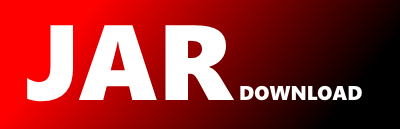
work.eddiejamsession.file.utils.FileUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jam-file-utils Show documentation
Show all versions of jam-file-utils Show documentation
Project helps to work with Java IO
package work.eddiejamsession.file.utils;
import java.io.File;
import java.io.IOException;
import java.util.Base64;
import java.util.Optional;
import java.util.function.Supplier;
import java.util.logging.Level;
import java.util.logging.Logger;
public class FileUtils {
//public api
public static void createIfNotExists(String filename) throws IOException {
File file = new File(filename);
if (!file.exists()) {
file.createNewFile();
}
}
public static byte[] computeIfAbsentBytes(String fileName, Supplier data) {
return Base64.getDecoder().decode(computeIfAbsent(fileName, () -> Base64.getEncoder().encodeToString(data.get())));
}
public static Optional getData(String fileName) {
try {
File file = new File(fileName);
createIfNotExists(fileName);
String strip = null;
strip = org.apache.commons.io.FileUtils.readFileToString(file).trim().strip();
if (strip.isBlank() || strip.isEmpty()) {
return Optional.empty();
} else {
return Optional.of(strip);
}
} catch (IOException e) {
throw new RuntimeException(e);
}
}
public static String computeIfAbsent(String fileName, Supplier stringData) {
try {
File file = new File(fileName);
createIfNotExists(fileName);
String strip;
strip = org.apache.commons.io.FileUtils.readFileToString(file).trim().strip();
if (strip.isBlank() || strip.isEmpty()) {
String d = stringData.get();
writeToFile(fileName, d);
return d;
} else {
return strip;
}
} catch (IOException e) {
throw new RuntimeException(e);
}
}
public static void writeToFile(String fileName, String data) {
try {
createIfNotExists(fileName);
org.apache.commons.io.FileUtils.writeStringToFile(new File(fileName), data);
} catch (IOException e) {
logException(e);
}
}
///--
private static void logException(IOException e) {
Logger.getGlobal().log(Level.SEVERE, e, () -> "");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy