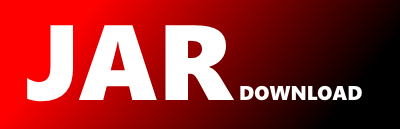
com.seal.quartz.controller.SysJobController Maven / Gradle / Ivy
package com.seal.quartz.controller;
import com.seal.common.annotation.Log;
import com.seal.common.core.controller.BaseController;
import com.seal.common.core.domain.AjaxResult;
import com.seal.common.core.page.TableDataInfo;
import com.seal.common.enums.BusinessType;
import com.seal.common.exception.job.TaskException;
import com.seal.common.utils.SecurityUtils;
import com.seal.common.utils.poi.ExcelUtil;
import com.seal.quartz.domain.SysJob;
import com.seal.quartz.service.ISysJobService;
import com.seal.quartz.util.CronUtils;
import org.quartz.SchedulerException;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.security.access.prepost.PreAuthorize;
import org.springframework.web.bind.annotation.*;
import java.util.List;
/**
* 调度任务信息操作处理
*
* @author silianpan
*/
@RestController
@RequestMapping("/monitor/job")
public class SysJobController extends BaseController {
@Autowired
private ISysJobService jobService;
/**
* 查询定时任务列表
* @param sysJob 作业
* @return 结果
*/
@PreAuthorize("@ss.hasPermi('monitor:job:list')")
@GetMapping("/list")
public TableDataInfo list(SysJob sysJob) {
startPage();
List list = jobService.selectJobList(sysJob);
return getDataTable(list);
}
/**
* 导出定时任务列表
* @param sysJob 作业
* @return 结果
*/
@PreAuthorize("@ss.hasPermi('monitor:job:export')")
@Log(title = "定时任务", businessType = BusinessType.EXPORT)
@GetMapping("/export")
public AjaxResult export(SysJob sysJob) {
List list = jobService.selectJobList(sysJob);
ExcelUtil util = new ExcelUtil(SysJob.class);
return util.exportExcel(list, "定时任务");
}
/**
* 获取定时任务详细信息
* @param jobId 作业ID
* @return 结果
*/
@PreAuthorize("@ss.hasPermi('monitor:job:query')")
@GetMapping(value = "/{jobId}")
public AjaxResult getInfo(@PathVariable("jobId") Long jobId) {
return AjaxResult.success(jobService.selectJobById(jobId));
}
/**
* 新增定时任务
* @param sysJob 作业
* @throws SchedulerException 异常
* @throws TaskException 异常
* @return 结果
*/
@PreAuthorize("@ss.hasPermi('monitor:job:add')")
@Log(title = "定时任务", businessType = BusinessType.INSERT)
@PostMapping
public AjaxResult add(@RequestBody SysJob sysJob) throws SchedulerException, TaskException {
if (!CronUtils.isValid(sysJob.getCronExpression())) {
return AjaxResult.error("cron表达式不正确");
}
sysJob.setCreateBy(SecurityUtils.getUsername());
return toAjax(jobService.insertJob(sysJob));
}
/**
* 修改定时任务
* @param sysJob 作业
* @throws SchedulerException 异常
* @throws TaskException 异常
* @return 结果
*/
@PreAuthorize("@ss.hasPermi('monitor:job:edit')")
@Log(title = "定时任务", businessType = BusinessType.UPDATE)
@PutMapping
public AjaxResult edit(@RequestBody SysJob sysJob) throws SchedulerException, TaskException {
if (!CronUtils.isValid(sysJob.getCronExpression())) {
return AjaxResult.error("cron表达式不正确");
}
sysJob.setUpdateBy(SecurityUtils.getUsername());
return toAjax(jobService.updateJob(sysJob));
}
/**
* 定时任务状态修改
* @param job 作业
* @throws SchedulerException 异常
* @return 结果
*/
@PreAuthorize("@ss.hasPermi('monitor:job:changeStatus')")
@Log(title = "定时任务", businessType = BusinessType.UPDATE)
@PutMapping("/changeStatus")
public AjaxResult changeStatus(@RequestBody SysJob job) throws SchedulerException {
SysJob newJob = jobService.selectJobById(job.getJobId());
newJob.setStatus(job.getStatus());
return toAjax(jobService.changeStatus(newJob));
}
/**
* 定时任务立即执行一次
* @param job 作业
* @throws SchedulerException 异常
* @return 结果
*/
@PreAuthorize("@ss.hasPermi('monitor:job:changeStatus')")
@Log(title = "定时任务", businessType = BusinessType.UPDATE)
@PutMapping("/run")
public AjaxResult run(@RequestBody SysJob job) throws SchedulerException {
jobService.run(job);
return AjaxResult.success();
}
/**
* 删除定时任务
* @param jobIds 作业ID数组
* @throws SchedulerException 异常
* @return 结果
*/
@PreAuthorize("@ss.hasPermi('monitor:job:remove')")
@Log(title = "定时任务", businessType = BusinessType.DELETE)
@DeleteMapping("/{jobIds}")
public AjaxResult remove(@PathVariable Long[] jobIds) throws SchedulerException {
jobService.deleteJobByIds(jobIds);
return AjaxResult.success();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy