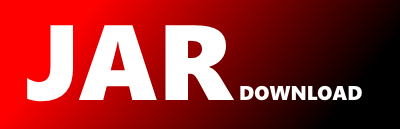
convex.core.metadata.cvx Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of convex-core Show documentation
Show all versions of convex-core Show documentation
Convex core libraries and common utilities
;;
;;
;; Metadata for core symbols that are implemented at the level of the CVM:
;;
;; - Core Functions defined in Java code
;; - Convex Lisp special forms
;;
;; Conventions:
;; - Explicit values quotes as `nil`
;; - Capitalised names used for types e.g. Long
;; - Brevity preferred, this is on-chain data
{*address*
{:doc {:description "Returns the address of the current account (user address in regular transaction, actor address in actor calls)."
:examples [{:code "(address? *address*)"}]}
:special true}
*balance*
{:doc {:description ["Returns the available balance of the current account (in Convex coins)."
"The available balance excludes reserved balance for transaction execution, so this number may be somewhat less than the total account balance during transaction execution."]
:examples [{:code "*balance*"}]}
:special true}
*caller*
{:doc {:description "During an actor call, returns the address of the account doing the call. Returns `nil` otherwise."
:examples [{:code "*caller*"}]}
:special true}
*controller*
{:doc {:description "Returns the controller of the current account."
:examples [{:code "*controller*"}]}
:special true}
*depth*
{:doc {:description ["Returns the CVM execution stack depth, at the point the `*depth*` operation is executed. If the depth becomes too deep, the transaction will fail with a `:DEPTH` exception."
"In most cases, the allowable depth should be sufficient."]
:examples [{:code "*depth*"}]}
:special true}
*holdings*
{:doc {:description ["Returns the holdings Index for this account."
"Holdings are data values controlled by other accounts (usually actors). They can be used to indicate that an account may have special rights or asset holdings with respect to a specific actor, for instance."
"Holdings should NOT be trusted if they are provided by an untrusted account."
"See `get-holding`, `set-holding`."]
:examples [{:code "*holdings*"}]}
:special true}
*initial-expander*
{:doc {:description "Initial expander used to expand forms, before compilation."
:examples [{:code "(expand '(if 1 2 3) *initial-expander*)"}]
:signature [{:params [form cont]}]}}
*juice*
{:doc {:description ["Returns the amount of execution juice consumed at this point of the current transaction."
"Juice is required for every CVM operation executed, and the transaction will fail immediately with a `:JUICE` error if an attempt is made to consume juice beyond this value."]
:examples [{:code "*juice*"}]}
:special true}
*juice-limit*
{:doc {:description ["Returns the limit of execution juice available."
"Juice is required for every CVM operation executed, and the transaction will fail immediately with a `:JUICE` error if an attempt is made to consume juice beyond this value."]
:examples [{:code "*juice-limit*"}]}
:special true}
*key*
{:doc {:description "Returns the public key for this account. Returns nil in the case of an actor since actors are accounts without keys."
:examples [{:code "*key*"}]}
:special true}
*memory*
{:doc {:description "Returns the current memory allowance for this account. May be zero - in which case any new memory allocations will be charged at the current memory exchange pool price."
:examples [{:code "*memory*"}]}
:special true}
*memory-price*
{:doc {:description "Returns the current memory price, as a double value"
:examples [{:code "*memory-price*"}]}
:special true}
*nop*
{:doc {:description "Does nothing."
:examples [{:code "*nop*"}]}
:special true}
*offer*
{:doc {:description "Returns the amount of native coin offered by `*caller*` during a call. See `call`. Will usually be zero, unless the caller has included an offer with a 'call' expression."
:examples [{:code "*offer*"}]}
:special true}
*origin*
{:doc {:description ["Similar to `*caller*` but returns the address of the account that initially signed this transaction."
"In a chain of calls, this address is the very first link."
"Usually, should NOT be used for access control, since a rogue actor can potentially trick a user into creating a transaction that allows code to be indirectly executed. Consider using `*caller*` for access control instead."]
:examples [{:code "*origin*"}]}
:special true}
*parent*
{:doc {:description "Returns the parent of the current account. Determines the default location for symbolic lookup."
:examples [{:code "*parent*"}]}
:special true}
*result*
{:doc {:description "Returns the result of the last CVM operation executed. Can be used, in some cases, to access the value of the previous expression. Will be nil for new transactions, or at the start of an actor call."
:examples [{:code "(do 1 *result*)"}]}
:special true}
*sequence*
{:doc {:description "Returns the sequence number for the current transaction. The sequence number is the number of transactions executed on this account *including* the current transaction / query. The next valid transction must increment *sequence* by one."
:examples [{:code "*sequence*"}]}
:special true}
*state*
{:doc {:description "Returns the current CVM state record. This is a large object, and should normally only be used temporarily to look up relevant values."
:examples [{:code "(keys *state*)"}
{:code "(get-in *state* [:accounts *address* :balance])"}]}
:special true}
*timestamp*
{:doc {:description ["Returns the current timestamp."
"The timestamp is a `Long` value that is equal to the greatest timestamp of any block executed (including the current block)."
"A timestamp can be interpreted as the number of milliseconds since January 1, 1970, 00:00:00 GMT. The block timestamp should always be less than or equal to the Unix timestamp of peers that are in consensus."
"Commonly used with `schedule`."]
:examples [{:code "*timestamp*"}]}
:special true}
<
{:doc {:description "Tests if numeric arguments are in strict increasing order. Reads as 'less-than'."
:examples [{:code "(< 1 2 3)"}]
:signature [{:params [& xs]
:return Boolean}]}}
>
{:doc {:description "Tests if numeric arguments are in strict decreasing order. Reads as 'greater-than'."
:examples [{:code "(> 3 2 1)"}]
:signature [{:params [& xs]
:return Boolean}]}}
<=
{:doc {:description "Tests if numeric arguments are in increasing order. Reads as 'less-than-or-equal'."
:examples [{:code "(<= 1 1 3)"}]
:signature [{:params [& xs]
:return Boolean}]}}
>=
{:doc {:description "Tests if numeric arguments are in decreasing order. Reads as 'greater-than-or-equal'."
:examples [{:code "(>= 3 2 2)"}]
:signature [{:params [& xs]
:return Boolean}]}}
==
{:doc {:description ["Tests if arguments are equal in numerical value."
"Difference with `=` is that types are erased (eg. `Long` value is comparable with a `double` value)."]
:examples [{:code "(== 2 2.0)"
:return "true"}]
:signature [{:params [& xs]
:return Boolean}]}}
!=
{:doc {:description ["Tests if arguments are not equal in numerical value. Inverse of ==."]
:examples [{:code "(!= 2 2.0)"
:return "false"}]
:signature [{:params [& xs]
:return Boolean}]}}
=
{:doc {:description "Returns `true` if and only if arguments are precisely equal in value."
:examples [{:code "(= :foo :foo)"
:return "true"}]
:signature [{:params [& vals]
:return Boolean}]}}
+
{:doc {:description "Adds numerical arguments. Result will be a `Long` if all arguments are integers, or a double if any floating point values are included."
:examples [{:code "(+ 1 2 3)"}]
:signature [{:params [& xs]}]}}
-
{:doc {:description "Subtracts numerical arguments from the first argument. Negates a single argument."
:examples [{:code "(- 10 7)"}]
:signature [{:params [x]}
{:params [x y & more]}]}}
*
{:doc {:description "Multiplies numeric arguments. Result will be a `Double` if any arguments are floating point values, otherwise it will be a `long`."
:examples [{:code "(* 1 2 3 4 5)"}]
:signature [{:params [& xs]}]}}
/
{:doc {:description "Double precision point divide. With a single argument, returns the reciprocal of a number. With multiple arguments, divides the first argument by the others in order."
:examples [{:code "(/ 10 3)"}]
:signature [{:params [divisor]}
{:params [numerator divisor]}
{:params [numerator divisor & more]}]}}
abs
{:doc {:description "Computes the absolute value of a numerical argument. Supports `double` and `long` results."
:errors {:CAST "If the parameter is not a number."}
:examples [{:code "(abs -1.5)"}
{:code "(abs 100)"}]
:signature [{:params [x]
:return Number}]}}
accept
{:doc {:description ["Used during a `call`, accepts offered coins up to the amount of `*offer*` from `*caller*`. Returns the amount accepted if successful."
"Amount must cast to `Long`. If successful, the amount will be added immediately to the `*balance*` of the current `*address*`."
"This is the recommended way of transferring balance between actors, as it requires a positive action to confirm receipt."]
:errors {:ARGUMENT "If accepted amount is negative"
:CAST "If accepted amount is not a `long`"
:STATE "If ´*caller*´ has not offered sufficient coins to fulfil the offer"}
:examples [{:code "(accept *offer*)"}]
:signature [{:params [amount]
:return Number}]}}
account
{:doc {:description "Returns the account record for a given address, or `nil` if the account does not exist. Argument must be an Address."
:errors {:CAST "If the argument is not a valid Address."}
:examples [{:code "(account *address*)"}]
:signature [{:params [address]
:return Account}]}}
address
{:doc {:description "Casts the argument to an Address. Valid arguments are hex strings, a Long, an Address or a Blob with the correct length (8 bytes)."
:errors {:CAST "If the argument is not castable to an Address."}
:examples [{:code "(address 451)"}]
:signature [{:params [x]
:return Address}]}}
address?
{:doc {:description "Returns true if the argument is an actual `address`."
:examples [{:code "(address? #777)"}
{:code "(address? :foo)"}]
:signature [{:params [x]
:return Boolean}]}}
apply
{:doc {:description ["Applies a function to the specified arguments, after flattening the last argument. Last argument must be a sequential collection, or 'nil' which is considered an empty collection."
"Only useful in particular cases when interacting with variadic functions."]
:errors {:ARITY "If the additional arguments cause an arity error in the applied function."
:CAST "If the first argument is not castable to a valid function."}
:examples [{:code "(apply + [1 2 3])"}
{:code "(apply + 1 2 [3 4 5])"}]
:signature [{:params [f & args more-args]
:return Any}]}}
assoc
{:doc {:description "Adds entries into an associative data structure, taking each two arguments as key/value pairs. A nil data structure is considered as an empty map. A set can only take the boolean values (`true` and `false`) indicating set inclusion and exclusion respectively."
:errors {:ARITY "If the additional arguments are not an even number (key and value pairs)."
:ARGUMENT "If one or more of the supplied keys or values is invalid for the data structure."
:CAST "If the first argument is not a valid DataStructure."}
:examples [{:code "(assoc {1 2} 3 4)"}]
:signature [{:params [coll & kvs]
:return DataStructure}]}}
assoc-in
{:doc {:description "Associates a value into a nested associative data structure, as if using `assoc` at each level."
:errors {:ARGUMENT "If one or more of the supplied keys is invalid for the data structure."
:CAST "If the first argument is not a valid data structure, or the second argument is not a a sequential data structure."}
:examples [{:code "(assoc-in {1 [1 2 3]} [1 2] 4)"}]
:signature [{:params [coll keys v]
:return DataStructure}]}}
balance
{:doc {:description "Returns the Convex Coin balance of the specified Account, which must be identified with a valid `Address`. Returns `nil` if and only if the Account does not exist."
:errors {:CAST "If the argument is not a valid address."}
:examples [{:code "(balance *caller*)"}]
:signature [{:params [address]
:return Long}]}}
bit-and
{:doc {:description "Returns the bitwise AND of two Long values."
:errors {:CAST "If an argument is not implicitly castable to Long."}
:examples [{:code "(bit-and 7 12)"}]
:signature [{:params [a b]
:return Long}]}}
bit-not
{:doc {:description "Returns the bitwise NOT of a Long value."
:errors {:CAST "If the argument is not implicitly castable to Long."}
:examples [{:code "(bit-not (long 0xfffffffffffffff0))"}]
:signature [{:params [a b]
:return Long}]}}
bit-or
{:doc {:description "Returns the bitwise OR of two Long values."
:errors {:CAST "If an argument is not implicitly castable to Long."}
:examples [{:code "(bit-or 7 12)"}]
:signature [{:params [a b]
:return Long}]}}
bit-xor
{:doc {:description "Returns the bitwise XOR of two Long values."
:errors {:CAST "If an argument is not implicitly castable to Long."}
:examples [{:code "(bit-xor 7 12)"}]
:signature [{:params [a b]
:return Long}]}}
blob
{:doc {:description "Casts the argument to a canonical Blob. Arugment can be an Address, a hex String, a Long value, or another Blob value."
:errors {:CAST "If the argument is not castable to a blob."}
:examples [{:code "(blob \"1234abcd\")"}]
:signature [{:params [address]
:return Blob}]}}
index
{:doc {:description ["Creates an Index. Indexes are specialised maps that support blob-like types as keys only (see `blob`)."
"Optional arguments must be pairs of blob-like keys and values to be included in the blob map."]
:errors {:ARGUMENT "If any of the keys supplied is not castable to a blob."
:ARITY "If there is not an even number of arguments (key and value pairs)."}
:examples [{:code "(index 0x1234 :foo)"}]
:signature [{:params [& kvs]
:return Index}]}}
blob?
{:doc {:description "Returns true if and only if the argument is a Blob."
:examples [{:code "(blob? 0x1234)"}]
:signature [{:params [x]
:return Boolean}]}}
boolean
{:doc {:description "Casts any value to a Boolean. Returns false if value is `nil` or `false`, true in any other case."
:examples [{:code "(boolean 123)"}]
:signature [{:params [x]
:return Boolean}]}}
boolean?
{:doc {:description "Returns true if the argument is a Boolean (either `true` or `false`). Any other value (including nil) returns false."
:examples [{:code "(boolean? false)"}]
:signature [{:params [x]
:return Boolean}]}}
byte
{:doc {:description "Casts a value to the unsigned byte range 0-255. Discards high bits of larger integer / blob types."
:errors {:CAST "If the argument is not castable to an integer."}
:examples [{:code "(byte 1234)"}]
:signature [{:params [x]
:return Integer}]}}
call*
{:doc {:description ["Behaves like `call` but implemented as a function. Instead of a form, takes a symbol referring to the function, and then arguments separately."
"Same kind of errors may happen."]
:errors {:ARITY "If the supplied arguments are the wrong arity for the called function."
:CAST "If the address argument is an Address, the offer is not a Long, or the function name is not a Symbol."
:STATE "If the address does not refer to an Account with the callable function specified by fn-name."
:ARGUMENT "If the offer is negative."}
:examples [{:code "(call* some-actor 1000 'contract-fn arg1 arg2)"}]
:signature [{:params [address offer fn-name & args]
:return Any}]}}
callable?
{:doc {:description "Returns true if the target is callable. If symbol is provided, also checks that this symbol references a callable function in the specified account. See `call`."
:errors {:CAST "If the symbol argument is not a Symbol."}
:examples [{:code "(callable? actor-address 'function-name)"}]
:signature [{:params [target]}
{:params [target symbol]}]}}
ceil
{:doc {:description "Computes the mathematical ceiling (rounding up towards positive infinity) for a numerical argument. Uses double precision mathematics."
:errors {:CAST "If the argument is not a number."}
:examples [{:code "(ceil 16.3)"}]
:signature [{:params [x]
:return Double}]}}
char
{:doc {:description "Casts a value to a Character. Treats integers as Unicode code points. Treats blob-like values as UTF-8 strings."
:errors {:CAST "If the argument type is not castable to a character."
:ARGUMENT "If the argument does not precisely specify a valid Unicode code point"}
:examples [{:code "(char 97)"}]
:signature [{:params [x]
:return Character}]}}
coll?
{:doc {:description "Returns `true` if and only if the argument is a collection: List, Map, Set, or Vector. Returns false otherwise."
:examples [{:code "(coll? [1 2 3])"}]
:signature [{:params [x]
:return Boolean}]}}
cond
{:doc {:description ["Performs conditional tests on successive pairs of `test` -> `result`, returning the `result` for the first `test` that succeeds."
"Performs short-circuit evaluation: result expressions that are not used and any test expressions after the first success will not be executed."
"In the case that no test succeeds, a single aditional argument may be added as a fallback value. If no fallback value is available, nil will be returned."]
:examples [{:code "(cond test-1 result-1 else-value)"}
{:code "(cond test-1 result-1 test-2 result-2 test-3 result--3)"}]
:signature [{:params []}
{:params [test]}
{:params [test result]}
{:params [test result fallback-value]}
{:params [test-1 result-1 test-2 result-2 & more]}]}
:special true}
compile
{:doc {:description "Compiles a form, returning an Op. See `eval`."
:errors {:COMPILE "If a compiler error occurs."}
:examples [{:code "(compile '(fn [x] (* x 2)))"}]
:signature [{:params [form]
:return Op}]}}
concat
{:doc {:description "Concatenates sequential data structures, returning a new sequential data structure of the same type as the first non-nil argument. `nil` is treated as an empty sequence."
:errors {:CAST "If any of the arguments is neither a sequential data structure nor nil."}
:examples [{:code "(concat [1 2] [3 4])"}]
:signature [{:params [& seqs]
:return DataStructure}]}}
conj
{:doc {:description "Adds elements to a data structure, in the natural mode of addition for the data structure. Supports sequential collections, sets and maps. `nil` is treated as an empty vector."
:errors {:ARGUMENT "If a provided element is not of correct type for the given data structure."
:CAST "If the first argument is not a data structure (or nil)."}
:examples [{:code "(conj [1 2] 3)"}
{:code "(conj {1 2} [3 4])"}
{:code "(conj #{1 2} 3)"}]
:signature [{:params [coll & elems]
:return DataStructure}]}}
cons
{:doc {:description "Constructs a List, by prepending the leading arguments to the last argument. The last argument must be coercable to a sequential data structure. `nil` is treated as an empty list."
:errors {:CAST "If the last argument is not a sequence."}
:examples [{:code "(cons 1 '(2 3))"}
{:code "(cons 1 2 '(3 4))"}]
:signature [{:params [arg & more-args coll]}]}}
contains-key?
{:doc {:description "Returns true if the given associative data structure contains the given key, false otherwise."
:examples [{:code "(contains-key? {:foo 1 :bar 2} :foo)"}]
:signature [{:params [coll key]
:return Boolean}]}}
create-peer
{:doc {:description "Creates a new peer record for the network. The peer must have an account number and sufficient balance to place a stake amount"
:errors {:CAST "If the first argument is not a valid account-key."}
:examples [{:code "(create-peer account-key 700000000)"}]
:signature [{:params [account-key stake-amount]
:return stake-amount}]}}
count
{:doc {:description "Returns the number of elements in the given data structure or other countable value."
:errors {:CAST "If the argument is not a countable object."}
:examples [{:code "(count [1 2 3])"}]
:signature [{:params [coll]
:return Long}]}}
countable?
{:doc {:description "Returns true if the argument is countable."
:examples [{:code "(countable? 0x1234)"}]
:signature [{:params [a]
:return Boolean}]}}
dec
{:doc {:description "Decrements the given Integer by 1."
:errors {:CAST "If the argument is not an Integer."}
:examples [{:code "(dec 10)"}]
:signature [{:params [long]
:return Long}]}}
def
{:doc {:description ["Creates a definition in the current environment. This value will persist in the environment owned by the current account."
"The name argument must be a symbol, or a Symbol wrapped in a syntax object with optional metadata."]
:errors {:CAST "If the argument is neither a valid symbol name nor a syntax containing a symbol value."}
:examples [{:code "(def a 10)"}]
:signature [{:params [name value]}]}
:special true}
deploy
{:doc {:description "Deploys an actor. The code provided will be executed to initialise the actor's account. More than one code form may be provided, in which case they will be compiled and evaluated separately. Returns the Address of the deployed actor."
:errors {:COMPILE "If a compiler error occurred deploying the given code."}
:examples [{:code "(deploy '(defn my-fn [x y] (+ x y)))"}]
:signature [{:params [code & more-code]
:return Address}]}}
difference
{:doc {:description "Computes the difference of one or more sets. Nil arguments are treated as an empty set."
:errors {:CAST "If any of the arguments is neither a set nor nil."}
:examples [{:code "(difference #{1 2} #{2 3})"}]
:signature [{:params [set & more]
:return Set}]}}
disj
{:doc {:description "Removes the specified key(s) from a set. `nil` is treated as the empty Set."
:errors {:CAST "If the first argument is not a set."}
:examples [{:code "(disj #{1 2 3} 1)"}]
:signature [{:params [coll key]}]}}
dissoc
{:doc {:description "Removes entries with the specified key(s) from a Map (including Indexes). `nil` is treated as the empty Map."
:errors {:CAST "If the first argument is not a Map."}
:examples [{:code "(dissoc {1 2 3 4} 3)"}]
:signature [{:params [coll & keys]
:return Map}]}}
div
{:doc {:description "Returns the integer value of a numerator divided by a divisor. Performs Euclidian division (i.e. non-negative remainder)."
:errors {:CAST "If any argument is not an Integer."}
:examples [{:code "(div 14 3)"}]
:signature [{:params [num denom]
:return Integer}]}}
do
{:doc {:description "Executes multiple expressions sequentially, and returns the value of the final expression."
:examples [{:code "(do (count [1 2 3]) :done)"}]
:signature [{:params [& expressions]}]}
:special true}
double
{:doc {:description "Casts any numerical value to a `Double`."
:errors {:CAST "If the argument is not castable to double."}
:examples [{:code "(double 3)"}]
:signature [{:params [x]
:return Double}]}}
double?
{:doc {:description "Returnes `true` if the argument is a value of Double type, `false` otherwise."
:examples [{:code "(double? 1.0)"}]
:signature [{:params [x]
:return Boolean}]}}
empty
{:doc {:description "Returns an empty collection of the same type as the argument. `(empty nil)` returns nil."
:errors {:CAST "If the argument is neither nil nor a data structure."}
:examples [{:code "(empty [1 2 3])"}]
:signature [{:params [coll]
:return DataStructure}]}}
empty?
{:doc {:description "Checks if the argument is an empty data structure. nil is considered empty. Returns false if not a data structures."
:examples [{:code "(empty? [])"}]
:signature [{:params [coll]
:return Boolean}]}}
encoding
{:doc {:description ["Returns the byte encoding for a given value as a Blob."
"The encoding is the unique canonical binary representation of a value. Encodings may change between Convex versions - it is unwise to rely on the exact representation."]
:examples [{:code "(encoding {1 2})"}]
:signature [{:params [value]
:return Blob}]}}
eval
{:doc {:description "Evaluates code in the current context, expanding and compiling the form if necessary (see `expand`,`compile`)."
:errors {:COMPILE "If a compiler error occurred evaluating the form."
:EXPAND "If an expander error occurred while expanding the form."}
:examples [{:code "(eval '(+ 1 2))"}]
:signature [{:params [form]}]}}
eval-as
{:doc {:description "Like `eval` but evaluates code in the environment of the specifed account. The current account must have controller privileges to execute this operation (see `set-controller`)."
:examples [{:code "(eval-as #666 '(+ 1 2))"}]
:signature [{:params [address form]}]}}
exp
{:doc {:description "Returns `e` raised to the power of the given numerical argument."
:errors {:CAST "If the argument is not a number."}
:examples [{:code "(exp 1.0)"}]
:signature [{:params [x]
:return Double}]}}
expand
{:doc {:description ["Expands the given form."
"Uses the specified expander (including macros) as the primary expander if provided, the default `*initial-expander*` otherwise."
"If also provided, a continuation expander will be passed to the primary expander, otherwise the primary will be used as its own continuation."
"Expanders are an advanced feature."]
:examples [{:code "(expand '(if a :truthy :falsey))"}]
:signature [{:params [form]}
{:params [form expander]}
{:params [form expander cont]}]}}
fail
{:doc {:description ["Causes execution to fail at the current position."
"Error type defaults to `:ASSERT` if not specified, and cannot be nil. Typically a keyword, it can actually be any value."
"Error message defaults to nil if not specified. The message may be any value, but the use of short descriptive strings is recommended."]
:examples [{:code "(fail :ASSERT \"Assertion failed\")"}]
:signature [{:params []}
{:params [message]}
{:params [error-type message]}]}}
first
{:doc {:description "Returns the first element from a Countable collection which must contain at least one element. Also see `empty?`"
:errors {:BOUNDS "If the collection is empty."
:CAST "If the first argument is not a Countable collection."}
:examples [{:code "(first [1 2 3])"}]
:signature [{:params [coll]}]}}
floor
{:doc {:description "Computes the mathematical floor (rounding down towards negative infinity) for a numerical argument. Uses double precision mathematics."
:examples [{:code "(floor 16.3)"}]
:signature [{:params [x]
:return Double}]}}
fn
{:doc {:description "Creates an anonymous function (closure) with the specified argument list and function body. Will close over variables in the current lexical scope."
:examples [{:code "(let [f (fn [x y] (* x y))] (f 10 7))"}]
:signature [{:params [args & body]}]}
:special true}
fn?
{:doc {:description "Returns `true` if and only if the argument is a function. Some values may operate as functions but are not functions themselves (eg. maps and vectors)."
:examples [{:code "(fn? count)"}]
:signature [{:params [x]
:return Boolean}]}}
get
{:doc {:description ["Gets an element from a collection at the specified index value. Works on all collection types including maps, sets and sequences. Nil is treated as an empty collection."
"If the index is not present, returns `not-found` value (nil by default)."]
:examples [{:code "(get {:foo 10 :bar 15} :foo)"}]
:signature [{:params [coll key]}
{:params [coll key not-found]}]}}
get-holding
{:doc {:description "Gets the holding value for a specified owner account address. Owner account must exist. Holding will be null by default. See `*holdings*`, `set-holding`."
:errors {:CAST "If the argument is not an address."}
:examples [{:code "(get-holding *caller*)"}]
:signature [{:params [owner]}]}}
get-in
{:doc {:description "Gets an element by successively looking up keys in nested collections according to the logic of `get`. If any lookup does not find the appropriate key, will return `not-found` (nil by default)."
:errors {:CAST "If the first argument is not an associative collection."}
:examples [{:code "(get-in [[1 2] [3 4]] [1 1])"}]
:signature [{:params [coll keys]}
{:params [coll keys not-found]}]}}
halt
{:doc {:description ["Completes execution in the current context with the specified result, or null if not provided. Does not roll back any state changes made."
"If the currently executing context is an actor, the result will be used as the return value from the actor call."]
:examples [{:code "(halt :we-are-finished-here)"}]
:signature [{:params []}
{:params [result]}]}}
hash
{:doc {:description "Calculates the 32-byte SHA3-256 cryptographic hash of a `blob` or an `address` (which is a specialized type of `blob`). Returns a 32-byte `blob`."
:examples [{:code "(hash 0x1234)"}
{:code "(hash (encoding :foo))"}]
:signature [{:params [value]
:return Blob}]}}
keccak256
{:doc {:description "Calculates the 32-byte Keccak-256 cryptographic hash of a `blob` or an `address` (which is a specialized type of `blob`). Returns a 32-byte `blob`."
:examples [{:code "(keccak256 0x1234)"}]
:signature [{:params [value]
:return Blob}]}}
sha256
{:doc {:description "Calculates the 32-byte SHA-256 cryptographic hash of a `blob` or an `address` (which is a specialized type of `blob`). Returns a 32-byte `blob`."
:examples [{:code "(sha256 0x1234)"}]
:signature [{:params [value]
:return Blob}]}}
hash-map
{:doc {:description "Constructs a map with the given keys and values. If a key is repeated, the last value will overwrite previous ones."
:errors {:ARITY "If the number of arguments is not even (key-value pairs)."}
:examples [{:code "(hash-map 1 2 3 4)"}]
:signature [{:params [& kvs]}]}}
hash-set
{:doc {:description "Constructs a Set with the given values. If a value is repeated, it will be included only once in the set."
:examples [{:code "(hash-set 1 2 3)"}]
:signature [{:params [& values]}]}}
inc
{:doc {:description "Increments the given Long value by 1."
:errors {:CAST "If the actor argument is not castable to Long."}
:examples [{:code "(inc 10)"}]
:signature [{:params [num]
:return Long}]}}
intersection
{:doc {:description "Computes the intersection of one or more sets. Nil is treated as an empty set."
:errors {:CAST "If any of the arguments is neither a set nor nil."}
:examples [{:code "(intersection #{1 2} #{2 3})"}]
:signature [{:params [set & more]
:return Set}]}}
int
{:doc {:description "Casts the given argument to an Integer)."
:errors {:CAST "If the argument is not castable to Integer."}
:examples [{:code "(int 10)"}]
:signature [{:params [num]}]}}
int?
{:doc {:description "Returnes `true` if the argument is an Integer value, `false` otherwise."
:examples [{:code "(int? 1234)"}]
:signature [{:params [x]
:return Boolean}]}}
into
{:doc {:description "Adds elements to a collection, in a collection-defined manner as with `conj`."
:errors {:ARGUMENT "If any of the elements is not a valid type for the given data structure."
:CAST "If either argument is not a data structure."}
:examples [{:code "(into {} [[1 2] [3 4]])"}]
:signature [{:params [coll elements]
:return DataStructure}]}}
join
{:doc {:description "Joins a sequence of Strings into a single String, using the provided Character separator."
:errors {:CAST "If the first argument is not a sequence of Strings, or the second arcgument is not a Character."}
:examples [{:code "(join [\"foo\" \"bar\"] \\.)"}]
:signature [{:params [strs separator]
:return String}]}}
keys
{:doc {:description "Returns a Vector of keys in the given Map, in the map defined order. Also see `values`."
:errors {:CAST "If the argument is not a Map."}
:examples [{:code "(keys {:foo 1 :bar 2})"}]
:signature [{:params [m]
:return Vector}]}}
keyword
{:doc {:description "Casts the argument to a Keyword: must be a name between 1 and 128 UTF-8 bytes."
:errors {:ARGUMENT "If the Keyword name is of illegal length."
:CAST "If the argument does not specify a name for the Keyword."}
:examples [{:code "(keyword \"foo\")"}]
:signature [{:params [name]
:return Keyword}]}}
keyword?
{:doc {:description "Returns `true` if and only if the argument is a Keyword."
:examples [{:code "(keyword? :foo)"}]
:signature [{:params [x]
:return Boolean}]}}
last
{:doc {:description "Returns the last element of a Countable data structure, in collection-defined order."
:errors {:CAST "If the argument is not coercible to a sequential data structure (list or vector)."
:BOUNDS "If there are no elements in the data structure, i.e. the last element does not exist."}
:examples [{:code "(last [1 2 3])"}]
:signature [{:params [coll]}]}}
let
{:doc {:description "Binds local variables according to symbol-expression pairs in a binding vectors, then execute following expressions in an implicit do block."
:examples [{:code "(let [x 10] (* x x))"}]
:signature [{:params [bindings & exps]}]}
:special true}
list
{:doc {:description "Creates a List containing the given arguments as elements."
:examples [{:code "(list 1 2 3)"}]
:signature [{:params [& elements]
:return List}]}}
list?
{:doc {:description "Returns `true` if and only if the argument is a List."
:examples [{:code "(list? :foo)"}]
:signature [{:params [x]
:return Boolean}]}}
log
{:doc {:description ["Outputs a sequence of values to the CVM log for the current account. Any valid CVM values can be logged. Returns a vector containing logged values."
"The CVM log is NOT stored on chain. It may be used by peers for external audits."]
:examples [{:code "(log :EVENT 123 [:some :data])"}]
:signature [{:params [& values]
:return Vector}]}}
long
{:doc {:description "Casts the given argument to a 64-bit Long (signed integer)."
:errors {:CAST "If the argument is not castable to Long."}
:examples [{:code "(long 10)"}]
:signature [{:params [num]}]}}
long?
{:doc {:description "Returnes `true` if the argument is a value of Long type, `false` otherwise."
:examples [{:code "(long? 1234)"}]
:signature [{:params [x]
:return Boolean}]}}
lookup
{:doc {:description "Looks up the value of a Symbol in the current execution environment, or the Account of the given Address if specified."
:errors {:NOBODY "If the target Account for lookup does not exist."}
:examples [{:code "(do (def a 13) (lookup a))"}
{:code "(lookup #8 count)"}]
:signature [{:params [sym]}
{:params [address sym]}]}
:special true}
lookup-meta
{:doc {:description "Looks up metadata for a Symbol in the current execution environment, or the Account of the given Address if specified. Returns nil if not found."
:examples [{:code "(lookup-meta 'count)"}]
:signature [{:params [name]}
{:params [address name]}]}}
loop
{:doc {:description ["Creates a loop body, binding one or more loop variables in a manner similar to `let`."
"Within the loop body, `recur` can be used to return to the start of the loop while re-binding the loop variables with new values. Does not consume stack."]
:examples [{:code "(loop [i 10 acc 1] (if (> i 1) (recur (dec i) (* acc i)) acc))"}]
:signature [{:params [bindings & body]}]}
:special true}
map
{:doc {:description "Applies a Function to each element of a data structure in sequence, and returns a vector of results. Additional collections may be provided to call a function with higher arity."
:examples [{:code "(map inc [1 2 3])"}]
:signature [{:params [f coll]}
{:params [f coll1 coll2 & more-colls ]}]}}
map?
{:doc {:description "Returns true if and only if the argument is a Map."
:examples [{:code "(map? {1 2})"}]
:signature [{:params [coll]
:return Boolean}]}}
max
{:doc {:description "Returns the numerical maximum of the given values. If two or more values are equal to the minimum, the first is returned."
:examples [{:code "(max 1 2 3)"}]
:signature [{:params [& numbers]}]}}
merge
{:doc {:description "Merges zero or more Maps (not Indexes!), replacing existing values. Nil is considered as an empty map."
:examples [{:code "(merge {1 2 3 4} {3 5 7 9})"}]
:signature [{:params [& maps]}]}}
meta
{:doc {:description "Returns metadata for a Syntax Object. Returns `nil` if the argument is not a syntax object."
:examples [{:code "(meta (syntax 'foo {:bar 1}))"}]
:signature [{:params [syntax]
:return Map}]}}
min
{:doc {:description "Returns the numerical minimum of the given values. If two or more values are equal to the minimum, the first is returned."
:examples [{:code "(min 1 2 3)"}]
:signature [{:params [& numbers]}]}}
mod
{:doc {:description "Returns the integer modulus of a numerator divided by a divisor. The result will always be positive, consistent with Euclidean Divsion."
:examples [{:code "(mod 13 5)"}]
:signature [{:params [num div]}]}}
nan?
{:doc {:description "Returns `true` if and only if the argment is the Double value `##NaN`."
:examples [{:code "(nan? ##NaN)"}]
:signature [{:params [x]
:return Boolean}]}}
name
{:doc {:description "Gets the String name of an object: a Keyword, a Symbol, or a String."
:errors {:CAST "If the argument is not castable to a String name."}
:examples [{:code "(name :foo)"
:return "\"foo\""}]
:signature [{:params [named-object]
:return String}]}}
next
{:doc {:description "Returns the elements of a sequential data structure after the first element, or `nil` if no more elements remain."
:errors {:CAST "If the argument is not a sequential data structure."}
:examples [{:code "(next [1 2 3])"}]
:signature [{:params [coll]
:return Sequence}]}}
nil?
{:doc {:description "Returns `true` if and only if the argument is the value `nil`."
:examples [{:code "(nil? nil)"}]
:signature [{:params [x]
:return Boolean}]}}
not
{:doc {:description "Inverts a truth value. Returns `true` for `false` or `nil`, returns `false` for any other value."
:examples [{:code "(not true)"}
{:code "(not nil)"}]
:signature [{:params [b]
:return Boolean}]}}
nth
{:doc {:description ["Gets the nth element of a Countable data structure."
"The index must be a valid integer between 0 (inclusive) and the element count of the collection (exclusive)."]
:errors {:CAST "If the first argument is not countable data structure."
:BOUNDS "If the element index is out of range."}
:examples [{:code "(nth [1 2 3] 2)"}]
:signature [{:params [coll index]}]}}
number?
{:doc {:description "Returns `true` if and only if the argument is a numeric value."
:examples [{:code "(number? 2.3)"}]
:signature [{:params [x]
:return Boolean}]}}
pow
{:doc {:description "Returns the first argument raised to the power of the second argument. Uses double precision maths."
:errors {:CAST "If the argument is not a Number."}
:examples [{:code "(pow 2 3)"}]
:signature [{:params [x y]
:return Double}]}}
print
{:doc {:description "Prints any value in its canonical readable representation as a String."
:errors {:JUICE "If the printed representation is to long for available Juice."}
:examples [{:code "(print [\\a :foo 'bar])"}]
:signature [{:params [a]
:return String}]}}
quasiquote
{:doc {:description "Returns the quoted value of a form, without evaluating it. Like `quote`, but elements within the form may be unquoted via `unquote`."
:examples [{:code "(quasiquote foo)"
:return "foo"}
{:code "(quasiquote (:a :b (unquote (+ 2 3))))"
:return "(:a :b 5)"}]
:signature [{:params [form]}]}
:expander true
:special true}
query
{:doc {:description "Runs forms in query mode. When returning a result, any State changes will be rolled back as if nothing happened."
:examples [{:code "(query (def a 10) a)"}
{:code "(query (call unsafe-actor (do-something)))"}]
:signature [{:params [& forms]}]}
:special true}
query-as
{:doc {:description "Like `eval-as` but executes the code in a query context, discarding any state changes."
:examples [{:code "(query-as #666 '(def a (+ 2 3)))"}]
:signature [{:params [address form]}]}}
quot
{:doc {:description "Returns the quotient of a numerator divided by a divisor. Performs truncated division (ie. rounds towards zero)."
:examples [{:code "(quot 13 5)"}]
:signature [{:params [num div]}]}}
quote
{:doc {:description "Returns the quoted value of a form, without evaluating it. For example, you can quote a symbol to get the symbol itself rather than the value in the environment that it refers to."
:examples [{:code "(quote foo)"}
{:code "(eval (quote (+ 1 2 3)))"}]
:signature [{:params [form]}]}
:expander true
:special true}
recur
{:doc {:description "Escapes from the currently executing code and recurs at the level of the next loop or function body."
:examples [{:code "(recur acc (dec i))"}]
:signature [{:params [x y]}]}
:special true}
reduce
{:doc {:description ["Efficient and convenient looping mechanism."
"Reduces over a collection, calling `f` with an accumulator value and, successively each element of that collection (see example showing a summation)."
"Looping can be stopped at any moment by calling `reduced`. Otherwise, all elements in the collection are processed."
"If an initial accumulator value is not supplied, the initial value will be determined by calling the function on the first 0, 1 or 2 elements of the collection (however many are available)."]
:errors {:CAST "If the first argument is not a function, or the final argument is not a sequential collection."}
:examples [{:code "(reduce (fn [acc item] (+ acc item)) 0 [1 2 3 4 5])"}]
:signature [{:params [f coll]}
{:params [f init coll]}]}}
reduced
{:doc {:description "Returns immediately from the enclosing `reduce` function, providing the given value as the result of the whole `reduce` operation. This can be used to terminate a reduction early, saving transaction costs."
:examples [{:code "(reduce (fn [acc x] (reduced :exit)) 1 [1 2 3 4 5])"}]
:signature [{:params [result]}]}}
rem
{:doc {:description "Returns the remainder of a numerator divided by a divisor, consistent with division performed by `quot`. The remainder will therefore have the same sign as the numerator."
:examples [{:code "(rem 13 5)"}]
:signature [{:params [num div]}]}}
return
{:doc {:description "Escapes from the currently executing code and returns the specified value from the current function. Expressions following `return` will not be executed."
:examples [{:code "(do (return :finished) 42)"}]
:signature [{:params [value]}]}
:special true}
reverse
{:doc {:description "Reverses a sequential data structure. Lists are converted to vectors, and vice versa for efficieny reasons. Nil is treated as an empty vector."
:examples [{:code "(reverse [1 2 3])"}]
:signature [{:params [value]
:return Sequence}]}
:special true}
rollback
{:doc {:description "Escapes from the currently executing smart contract. Rolls back any state changes, as if nothing happened during that transaction. Returns the given value."
:examples [{:code "(rollback :aborted)"}]
:signature [{:params [value]}]}
:special true}
schedule*
{:doc {:description "Schedules a Op for future execution under this account. Op will not be executed until the specified timestamp. `compile` may be used to produce an Op from a normal code form."
:examples [{:code "(schedule* (+ *timestamp* 1000) (compile '(transfer my-friend 1000000)))"}]
:signature [{:params [timestamp code]}]}}
second
{:doc {:description "Returns the second element of a countable collection."
:errors {:BOUNDS "If the argument does not have a second value"
:CAST "If the argument is not a countable collection."}
:examples [{:code "(second [1 2 3])"}]
:signature [{:params [coll]}]}}
set
{:doc {:description "Coerces any data structure to a `Set`."
:errors {:CAST "If the argument is not a countable data structure."}
:examples [{:code "(set [1 2 3])"}]
:signature [{:params [coll]
:return Set}]}}
set!
{:doc {:description ["Sets a binding to the given value."
"A local change will be be visible until the scope leaves the current binding form (eg. `let` binding, function body or recur)."
"Returns the value assigned to the local binding if successful."]
:errors {:SYNTAX "If the symbol is not valid."
:UNDECLARED "If the symbol is not decalred."}
:examples [{:code "(let [a 10] (set! a 20) a)"}]
:signature [{:params [sym value]}]}
:special true}
set-controller
{:doc {:description ["Sets the controller for the current account."
"Controller account is granted powerful access privileges including the ability to run `eval-as`. Setting to nil disable such control (default value)."]
:errors {:CAST "If the argument is neither a valid (scoped) Address nor nil"
:NOBODY "If the address does not refer to an existing account."}
:examples [{:code "(set-controller #9)"}]
:signature [{:params [addr]}]}}
set-holding
{:doc {:description "Sets the holding value for a specified owner account address. Owner account must exist. Returns the new holding value."
:errors {:CAST "If the first argument is not an address."}
:examples [{:code "(set-holding *caller* 1000)"}]
:signature [{:params [owner value]}]}}
set-key
{:doc {:description ["Sets the public key (32-byte blob) for the current Account. May set to `nil` to turn this Account into an Actor and disable future external transactions."
"WARNING: You may lose access to the Account if you do not have access to the associated private key."]
:signature [{:params [new-key]}]}}
set-memory
{:doc {:description "Sets the free memory allowance for the current account address, in number of bytes. Increases in memory allowance may cost coin balance. Decreases in memory allowance may earn a coin refund. Returns the price paid (negative for refund)."
:examples [{:code "(set-memory 10000)"}]
:signature [{:params [mem]}]}}
set-parent
{:doc {:description ["Sets the parent for the current account."
"Parent account is the account for which symbolic lookups will default."]
:errors {:CAST "If the argument is neither a valid address nor nil"
:ARGUMENT "If the parent is the current address"
:NOBODY "If the address does not refer to an existing account."}
:examples [{:code "(set-parent #8)"}]
:signature [{:params [addr]}]}}
set-peer-data
{:doc {:description "Sets metadata on a given peer. Metadata must be a map. Peer must exist, with a public account key."
:errors {:CAST "If the first argument is not a valid peer Key, or the second argument is not a hash map."}
:examples [{:code "(set-peer-data peer-key {:url \"my-peer.com:4242\"})"}]
:signature [{:params [peer map]}]}}
set-peer-stake
{:doc {:description "Sets the peer stake. Stake must be a `long`. Peer must exist, with a public account key."
:errors {:CAST "If the first argument is not a valid peer key, or the second argument is not a `long`."}
:examples [{:code "(set-peer-data peer-key {:url \"my-peer.com:4242\"})"}]
:signature [{:params [peer stake]}]}}
set?
{:doc {:description "Returns true if the argument is a set, false otherwise."
:examples [{:code "(set? #{1 2 3})"}]
:signature [{:params [x]
:return Boolean}]}}
signum
{:doc {:description "Returns the signum of a numeric value, defined to be -1, 0 or 1."
:errors {:CAST "If the argument is not a number (including `##NaN`)."}
:examples [{:code "(signum -1)"}]
:signature [{:params [x]
:return Long}]}}
slice
{:doc {:description ["Takes a slice of a countable data structure between start (inclusive) and end (exclusive) indexes."]
:signature [{:params [a start end]}]
:errors {:BOUNDS "If the start or end indexes are out of bounds."}
:examples [{:code "(slice \"Hello World\" 6 11)"}]}}
split
{ :doc {:description "Splits a string by the given character separator, returning a Vector of substrings excluding the separator(s). Separator may be a character, Long codepoint value or a 1-character UTF-8 String."
:examples [{:code "(split \"foo|bar\" \\|)"}]
:signature [{:params [str separator]
:return Vector}]}}
sqrt
{ :doc {:description "Computes the square root of a numerical argument. Uses double precision mathematics. May return `##NaN` for negative values."
:examples [{:code "(sqrt 16.0)"}]
:signature [{:params [x]
:return Double}]}}
stake
{:doc {:description "Sets the stake on a given peer. Peer must exist, and funds must be available to set the stake to the specified level. Setting stake to zero removes the stake entirely."
:examples [{:code "(stake trusted-peer-account-key 7000000000)"}]
:signature [{:params [account-key amount]}]}}
str
{:doc {:description "Coerces values into strings and concatenates them."
:examples [{:code "(str \"Hello \" name)"}]
:signature [{:params [& args]
:return String}]}}
str?
{:doc {:description "Returns true if the argument is a string, false otherwise."
:examples [{:code "(str? name)"}]
:signature [{:params [x]
:return Boolean}]}}
subset?
{:doc {:description "Returns true if `set-1` is a subset of `set-2`. Both arguments must be sets, nil being considered as an empty set."
:examples [{:code "(subset? #{1} #{1 2 3})"}]
:signature [{:params [set-1 set-2]
:return Boolean}]}}
symbol
{:doc {:description "Creates a symbol from keyword, a symbol, or a string between 1 and 64 character"
:examples [{:code "(symbol :foo)"}]
:signature [{:params [name]
:return Symbol}]}}
str?
{:doc {:description "Returns true if the argument is a string, false otherwise."
:examples [{:code "(str? \"foo\")"}]
:signature [{:params [x]
:return Boolean}]}}
symbol?
{:doc {:description "Returns true if the argument is a symbol, false otherwise."
:examples [{:code "(symbol? 'foo)"}]
:signature [{:params [x]
:return Boolean}]}}
syntax
{:doc {:description "Wraps a value as a syntax object, if it is not already one. If metadata is provided, merge the metadata into the resulting syntax object."
:examples [{:code "(syntax 'bar)"}
{:code "(syntax 'bar {:some :metadata})"}]
:signature [{:params [value]
:return Syntax}
{:params [value meta]
:return Syntax}]}}
syntax?
{:doc {:description "Returns true if the argument is a syntax object."
:examples [{:code "(syntax? form)"}]
:signature [{:params [x]
:return Boolean}]}}
tailcall*
{:doc {:description "Like `tailcall` but lower-level. Instead of a form, takes a function and then arguments separately."
:examples [{:code "(tailcall* + 1 2 3)"}]
:signature [{:params [f & args] }]}}
transfer
{:doc {:description "Transfers the specified amount of coins to the target `Address`. Returns the amount transferred if successful, which may be less than the amount requested if the target does not accept all."
:errors {:FUNDS "If there is insufficient balance in the sender's account."
:STATE "If the receiver is an actor that is unable to accept funds."}
:examples [{:code "(transfer #42 12345678)"}]
:signature [{:params [address amount]
:return Long}]}}
transfer-memory
{:doc {:description "Transfers the specified amount of memory allowance to the target `address`. Returns the amount transferred if successful."
:errors {:MEMORY "If there is insufficient balance in the sender's account."}
:examples [{:code "(transfer-memory my-friend-address 100)"}]
:signature [{:params [address amount]
:return Long}]}}
undef*
{:doc {:description "Undefines a symbol, removing the mapping from the current environment if it exists. Helper function for the 'undef' macro."
:examples [{:code "(undef* 'a)"}]
:signature [{:params [sym]}]}}
union
{:doc {:description "Computes the union of zero or more sets. Nil is treated as an empty set when used as an input, but any result will always be a valid non-nil Set."
:examples [{:code "(union #{1 2} #{2 3})"}]
:signature [{:params [& sets]
:return Set}]}}
unsyntax
{:doc {:description "Unwraps a value from a syntax object. If the argument is not a syntax object, returns it unchanged."
:examples [{:code "(unsyntax form)"}]
:signature [{:params [form]}]}}
values
{:doc {:description "Gets the values from a map. Also see `keys`."
:examples [{:code "(values {1 2 3 4})"}]
:signature [{:params [map]
:return Vector}]}}
vec
{:doc {:description "Coerces the argument to a vector. Arguement must be coercible to a sequential data structure."
:examples [{:code "(vec #{1 2 3 4})"}]
:signature [{:params [coll]
:return Vector}]}}
vector
{:doc {:description "Creates a vector with the given elements."
:examples [{:code "(vector 1 2 3)"}]
:signature [{:params [& elements]
:return Vector}]}}
vector?
{:doc {:description "Returns true if the argument is a vector, false otherwise."
:examples [{:code "(vector? [1 2 3])"}]
:signature [{:params [x]
:return Boolean}]}}
zero?
{:doc {:description "Returns true if the argument has the numeric value zero, false otherwise."
:examples [{:code "(zero? 0.1)"}]
:signature [{:params [x]
:return Boolean}]}}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy