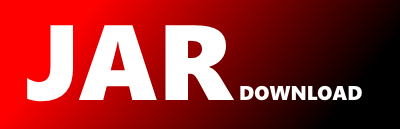
convex.net.message.MessageLocal Maven / Gradle / Ivy
package convex.net.message;
import java.util.function.Consumer;
import convex.core.Result;
import convex.core.data.ACell;
import convex.core.data.Hash;
import convex.core.data.Ref;
import convex.core.data.prim.CVMLong;
import convex.core.store.AStore;
import convex.net.MessageType;
import convex.peer.Server;
/**
* Class representing a message to a local Server instance
*/
public class MessageLocal extends Message {
protected Server server;
protected AStore store;
protected Consumer resultHandler;
protected MessageLocal(MessageType type, ACell payload, Server server, Consumer handler) {
super(type, payload);
this.server=server;
this.resultHandler=handler;
}
/**
* Create an instance with the given message data
* @param type Message type
* @param payload Message payload
* @param server Local server instance
* @param handler Handler for Results
* @return New MessageLocal instance
*/
public static MessageLocal create(MessageType type, ACell payload, Server server, Consumer handler) {
return new MessageLocal(type,payload,server,handler);
}
@Override
public boolean reportResult(Result res) {
resultHandler.accept(res);
return true;
}
@Override
public boolean reportResult(CVMLong id, ACell reply) {
resultHandler.accept(Result.create(id, reply));
return true;
}
@Override
public String getOriginString() {
return "Local Peer";
}
@Override
public boolean sendData(ACell data) {
if (data!=null) {
store.storeRef(data.getRef(), Ref.STORED, null);
}
return true;
}
@Override
public boolean sendMissingData(Hash hash) {
Ref ref=server.getStore().refForHash(hash);
if (ref!=null) {
store.storeRef(ref, Ref.STORED, null);
}
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy