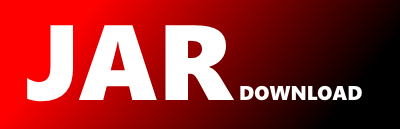
world.data.jdbc.internal.metadata.AbstractDatabaseMetaData Maven / Gradle / Ivy
/*
* dw-jdbc
* Copyright 2017 data.world, Inc.
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the
* License.
*
* You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*
* This product includes software developed at data.world, Inc.(http://www.data.world/).
*/
package world.data.jdbc.internal.metadata;
import world.data.jdbc.DataWorldConnection;
import world.data.jdbc.Driver;
import world.data.jdbc.internal.types.TypeMap;
import world.data.jdbc.internal.types.TypeMapping;
import world.data.jdbc.internal.util.Versions;
import world.data.jdbc.vocab.Xsd;
import java.sql.Connection;
import java.sql.DatabaseMetaData;
import java.sql.ResultSet;
import java.sql.RowIdLifetime;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import static java.util.Objects.requireNonNull;
import static world.data.jdbc.internal.util.Conditions.check;
import static world.data.jdbc.internal.util.Optionals.nullOrEquals;
import static world.data.jdbc.internal.util.Optionals.nullOrMatches;
import static world.data.jdbc.internal.util.Optionals.or;
/**
* Base class with functionality common to all query languages.
*/
abstract class AbstractDatabaseMetaData implements DatabaseMetaData {
/** Constant for the term used for catalogs. */
private static final String CATALOG_TERM = "Account";
/** Constant for the term used for schemas. */
private static final String SCHEMA_TERM = "Dataset";
private static final int NO_LIMIT = 0;
private static final int UNKNOWN_LIMIT = 0;
final DataWorldConnection connection;
final String catalog;
final String schema;
/**
* Creates new connection metadata
*
* @param connection Connection
*/
AbstractDatabaseMetaData(DataWorldConnection connection, String catalog, String schema) {
this.connection = requireNonNull(connection, "connection");
this.catalog = requireNonNull(catalog, "catalog");
this.schema = requireNonNull(schema, "schema");
}
@Override
public boolean isWrapperFor(Class> iface) throws SQLException {
return false;
}
@Override
public T unwrap(Class iface) throws SQLException {
check(isWrapperFor(iface), "Not a wrapper for the desired interface");
return iface.cast(this);
}
@Override
public boolean allProceduresAreCallable() {
return false;
}
@Override
public boolean allTablesAreSelectable() {
return true;
}
@Override
public boolean autoCommitFailureClosesAllResultSets() {
return false;
}
@Override
public boolean dataDefinitionCausesTransactionCommit() {
return true;
}
@Override
public boolean dataDefinitionIgnoredInTransactions() {
return false;
}
@Override
public boolean deletesAreDetected(int type) throws SQLException {
return false;
}
@Override
public boolean doesMaxRowSizeIncludeBlobs() {
// There is no max row size in RDF/SPARQL
return true;
}
@Override
public boolean generatedKeyAlwaysReturned() {
// We don't support returning keys
return false;
}
@Override
public ResultSet getAttributes(String catalog, String schemaPattern, String typeNamePattern, String attributeNamePattern) throws SQLException {
return MetaDataSchema.newResultSet(MetaDataSchema.ATTRIBUTE_COLUMNS);
}
@Override
public ResultSet getBestRowIdentifier(String catalog, String schema, String table, int scope, boolean nullable) throws SQLException {
return MetaDataSchema.newResultSet(MetaDataSchema.BEST_ROW_IDENTIFIER_COLUMNS);
}
@Override
public String getCatalogSeparator() {
return ".";
}
@Override
public String getCatalogTerm() {
return CATALOG_TERM;
}
@Override
public ResultSet getCatalogs() throws SQLException {
Object[][] rows = {{catalog}};
return MetaDataSchema.newResultSet(MetaDataSchema.CATALOG_COLUMNS, rows);
}
@Override
public ResultSet getClientInfoProperties() throws SQLException {
return MetaDataSchema.newResultSet(MetaDataSchema.CLIENT_INFO_PROPERTY_COLUMNS);
}
@Override
public ResultSet getColumnPrivileges(String catalog, String schema, String table, String columnNamePattern) throws SQLException {
return MetaDataSchema.newResultSet(MetaDataSchema.COLUMN_PRIVILEGE_COLUMNS);
}
@Override
public Connection getConnection() {
return connection;
}
@Override
public ResultSet getCrossReference(String parentCatalog, String parentSchema, String parentTable, String foreignCatalog, String foreignSchema, String foreignTable) throws SQLException {
return MetaDataSchema.newResultSet(MetaDataSchema.CROSS_REFERENCE_COLUMNS);
}
@Override
public boolean supportsTransactionIsolationLevel(int isolationLevel) {
return isolationLevel == Connection.TRANSACTION_NONE;
}
@Override
public int getDatabaseMajorVersion() {
return 1;
}
@Override
public int getDatabaseMinorVersion() {
return 0;
}
@Override
public String getDatabaseProductName() {
return "data.world";
}
@Override
public String getDatabaseProductVersion() {
return "1.0";
}
@Override
public int getDefaultTransactionIsolation() {
return Connection.TRANSACTION_NONE;
}
@Override
public int getDriverMajorVersion() {
return Versions.parseVersionNumbers(Driver.VERSION)[0];
}
@Override
public int getDriverMinorVersion() {
return Versions.parseVersionNumbers(Driver.VERSION)[1];
}
@Override
public String getDriverName() {
return "data.world JDBC driver";
}
@Override
public String getDriverVersion() {
return Driver.VERSION;
}
@Override
public ResultSet getExportedKeys(String catalog, String schema, String table) throws SQLException {
return MetaDataSchema.newResultSet(MetaDataSchema.EXPORTED_KEY_COLUMNS);
}
@Override
public String getExtraNameCharacters() {
// Since SPARQL doesn't really have a notion of identifiers like SQL
// does we return that there are no extra name characters
return "";
}
@Override
public ResultSet getFunctionColumns(String catalog, String schemaPattern, String functionNamePattern, String columnNamePattern) throws SQLException {
return MetaDataSchema.newResultSet(MetaDataSchema.FUNCTION_COLUMN_COLUMNS);
}
@Override
public ResultSet getFunctions(String catalog, String schemaPattern, String functionNamePattern) throws SQLException {
return MetaDataSchema.newResultSet(MetaDataSchema.FUNCTION_COLUMNS);
}
@Override
public ResultSet getImportedKeys(String catalog, String schema, String table) throws SQLException {
return MetaDataSchema.newResultSet(MetaDataSchema.IMPORTED_KEY_COLUMNS);
}
@Override
public ResultSet getIndexInfo(String catalog, String schema, String table, boolean unique, boolean approximate) throws SQLException {
return MetaDataSchema.newResultSet(MetaDataSchema.INDEX_INFO_COLUMNS);
}
@Override
public int getJDBCMajorVersion() {
return 4;
}
@Override
public int getJDBCMinorVersion() {
return 2;
}
@Override
public int getMaxBinaryLiteralLength() {
// No limit on RDF term sizes
return NO_LIMIT;
}
@Override
public int getMaxCatalogNameLength() {
return 31;
}
@Override
public int getMaxCharLiteralLength() {
// No limit on RDF term sizes
return NO_LIMIT;
}
@Override
public int getMaxColumnNameLength() {
// No limit on column name lengths
return NO_LIMIT;
}
@Override
public int getMaxColumnsInGroupBy() {
return NO_LIMIT;
}
@Override
public int getMaxColumnsInIndex() {
return NO_LIMIT;
}
@Override
public int getMaxColumnsInOrderBy() {
return NO_LIMIT;
}
@Override
public int getMaxColumnsInSelect() {
return NO_LIMIT;
}
@Override
public int getMaxColumnsInTable() {
return NO_LIMIT;
}
@Override
public int getMaxConnections() {
return NO_LIMIT;
}
@Override
public int getMaxCursorNameLength() {
return UNKNOWN_LIMIT;
}
@Override
public int getMaxIndexLength() {
return NO_LIMIT;
}
@Override
public int getMaxProcedureNameLength() {
return UNKNOWN_LIMIT;
}
@Override
public int getMaxRowSize() {
return NO_LIMIT;
}
@Override
public int getMaxSchemaNameLength() {
return 95;
}
@Override
public int getMaxStatementLength() {
return NO_LIMIT;
}
@Override
public int getMaxStatements() {
return NO_LIMIT;
}
@Override
public int getMaxTableNameLength() {
return NO_LIMIT;
}
@Override
public int getMaxTablesInSelect() {
return NO_LIMIT;
}
@Override
public int getMaxUserNameLength() {
return UNKNOWN_LIMIT;
}
@Override
public ResultSet getPrimaryKeys(String catalog, String schema, String table) throws SQLException {
return MetaDataSchema.newResultSet(MetaDataSchema.PRIMARY_KEY_COLUMNS);
}
@Override
public ResultSet getProcedureColumns(String catalog, String schemaPattern, String procedureNamePattern, String columnNamePattern) throws SQLException {
return MetaDataSchema.newResultSet(MetaDataSchema.PROCEDURE_COLUMN_COLUMNS);
}
@Override
public String getProcedureTerm() {
// Not supported
return null;
}
@Override
public ResultSet getProcedures(String catalog, String schemaPattern, String procedureNamePattern) throws SQLException {
return MetaDataSchema.newResultSet(MetaDataSchema.PROCEDURE_COLUMNS);
}
@Override
public ResultSet getPseudoColumns(String catalog, String schemaPattern, String tableNamePattern, String columnNamePattern) throws SQLException {
return MetaDataSchema.newResultSet(MetaDataSchema.PSUEDO_COLUMN_COLUMNS);
}
@Override
public int getResultSetHoldability() {
return ResultSet.CLOSE_CURSORS_AT_COMMIT;
}
@Override
public RowIdLifetime getRowIdLifetime() {
return RowIdLifetime.ROWID_UNSUPPORTED;
}
@Override
public int getSQLStateType() {
return sqlStateXOpen;
}
@Override
public String getSchemaTerm() {
return SCHEMA_TERM;
}
@Override
public ResultSet getSchemas() throws SQLException {
return getSchemas(null, null);
}
@Override
public ResultSet getSchemas(String catalog, String schemaPattern) throws SQLException {
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy