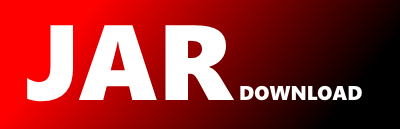
ws.ament.hammock.jpa.flyway.FlywayBean Maven / Gradle / Ivy
/*
* Copyright 2016 Hammock and its contributors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied.
*
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package ws.ament.hammock.jpa.flyway;
import javax.enterprise.context.Dependent;
import javax.enterprise.context.spi.CreationalContext;
import javax.enterprise.inject.spi.Bean;
import javax.enterprise.inject.spi.InjectionPoint;
import java.lang.annotation.Annotation;
import java.lang.reflect.Type;
import java.util.HashSet;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
import org.apache.deltaspike.core.api.config.ConfigResolver;
import org.flywaydb.core.Flyway;
import org.jboss.logging.Logger;
import ws.ament.hammock.core.config.ConfigLoader;
import static java.util.Collections.emptySet;
public class FlywayBean implements Bean {
private static Logger LOG = Logger.getLogger(FlywayBean.class);
private final Flyway flyway;
FlywayBean() {
Map properties = ConfigLoader.loadAllProperties("flyway", false);
this.flyway = new Flyway();
Properties props = new Properties();
props.putAll(properties);
flyway.configure(props);
this.postCreate();
}
private void postCreate() {
String executions = ConfigResolver.getProjectStageAwarePropertyValue("flyway.execute","migrate");
String[] methods = executions.split(",");
for(String method :methods) {
switch (method.toLowerCase()) {
case "repair":
flyway.repair();
break;
case "migrate":
flyway.migrate();
break;
case "clean":
flyway.clean();
break;
case "validate":
flyway.validate();
break;
case "baseline":
flyway.baseline();
break;
default:
LOG.warn("Invalid callback method "+method);
break;
}
}
}
@Override
public Class> getBeanClass() {
return Flyway.class;
}
@Override
public Set getInjectionPoints() {
return emptySet();
}
@Override
public boolean isNullable() {
return false;
}
@Override
public Flyway create(CreationalContext creationalContext) {
return flyway;
}
@Override
public void destroy(Flyway flyway, CreationalContext creationalContext) {
}
@Override
public Set getTypes() {
Set types = new HashSet<>();
types.add(Flyway.class);
types.add(Object.class);
return types;
}
@Override
public Set getQualifiers() {
return emptySet();
}
@Override
public Class extends Annotation> getScope() {
return Dependent.class;
}
@Override
public String getName() {
return "flyway";
}
@Override
public Set> getStereotypes() {
return emptySet();
}
@Override
public boolean isAlternative() {
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy