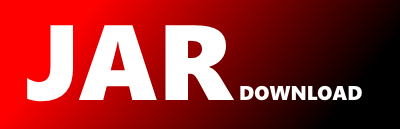
ws.wamp.jawampa.connection.WampConnectionPromise Maven / Gradle / Ivy
package ws.wamp.jawampa.connection;
/**
* A lightweight promise type which is used for the implementation
* of WAMP connection adapters.
* The promise is not thread-safe and supports no synchronization.
* This means it is not allowed to call fulfill or reject more than once and
* to access the result without external synchronization.
*/
public class WampConnectionPromise implements IWampConnectionPromise {
Throwable error = null;
T result = null;
Object state;
ICompletionCallback callback;
/**
* Creates a new promise
* @param callback The callback which will be invoked when {@link WampConnectionPromise#fulfill(Object)}
* or {@link WampConnectionPromise#reject(Throwable)} is called.
* @param state An arbitrary state object which is stored inside the promise.
*/
public WampConnectionPromise(ICompletionCallback callback, Object state) {
this.callback = callback;
this.state = state;
}
@Override
public Object state() {
return state;
}
@Override
public void fulfill(T value) {
this.result = value;
callback.onCompletion(this);
}
@Override
public void reject(Throwable error) {
this.error = error;
callback.onCompletion(this);
}
/**
* Resets a promises state.
* This can be used to reuse a promise.
* This may only be used if it's guaranteed that the promise and the
* associated future is no longer used by anyone else.
*/
public void reset(ICompletionCallback callback, Object state) {
this.error = null;
this.result = null;
this.callback = callback;
this.state = state;
}
@Override
public boolean isSuccess() {
return error == null;
}
@Override
public T result() {
return result;
}
@Override
public Throwable error() {
return error;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy