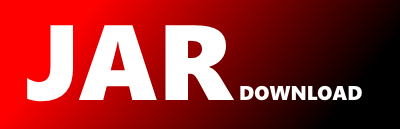
wtf.emulator.EwExecTask Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-plugin-core Show documentation
Show all versions of gradle-plugin-core Show documentation
With this Gradle plugin you can run your Android instrumentation tests with emulator.wtf
The newest version!
package wtf.emulator;
import org.gradle.api.DefaultTask;
import org.gradle.api.file.DirectoryProperty;
import org.gradle.api.file.FileCollection;
import org.gradle.api.file.RegularFileProperty;
import org.gradle.api.provider.ListProperty;
import org.gradle.api.provider.MapProperty;
import org.gradle.api.provider.Property;
import org.gradle.api.tasks.CacheableTask;
import org.gradle.api.tasks.Classpath;
import org.gradle.api.tasks.Input;
import org.gradle.api.tasks.InputFile;
import org.gradle.api.tasks.InputFiles;
import org.gradle.api.tasks.Internal;
import org.gradle.api.tasks.Optional;
import org.gradle.api.tasks.OutputDirectory;
import org.gradle.api.tasks.OutputFile;
import org.gradle.api.tasks.PathSensitive;
import org.gradle.api.tasks.PathSensitivity;
import org.gradle.api.tasks.TaskAction;
import org.gradle.workers.WorkQueue;
import org.gradle.workers.WorkerExecutor;
import wtf.emulator.exec.EwWorkAction;
import wtf.emulator.exec.EwWorkParameters;
import javax.inject.Inject;
import java.time.Duration;
import java.util.Map;
@CacheableTask
public abstract class EwExecTask extends DefaultTask {
@Classpath
@InputFiles
public abstract Property getClasspath();
@Input
public abstract Property getToken();
@Optional
@InputFiles
@PathSensitive(PathSensitivity.NONE)
public abstract Property getApks();
@Optional
@InputFile
@PathSensitive(PathSensitivity.NONE)
public abstract RegularFileProperty getTestApk();
@Optional
@InputFile
@PathSensitive(PathSensitivity.NONE)
public abstract RegularFileProperty getLibraryTestApk();
@Optional
@OutputDirectory
public abstract DirectoryProperty getOutputsDir();
@OutputFile
public abstract RegularFileProperty getOutputFile();
@Optional
@Input
public abstract ListProperty getOutputTypes();
@Optional
@Input
public abstract Property getRecordVideo();
@Optional
@Input
public abstract ListProperty
© 2015 - 2025 Weber Informatics LLC | Privacy Policy