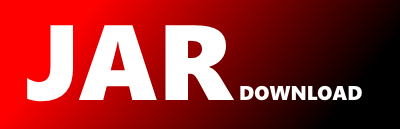
wtf.metio.memoization.jool.MemoizeJool Maven / Gradle / Ivy
/*
* SPDX-FileCopyrightText: The memoization.java Authors
* SPDX-License-Identifier: 0BSD
*/
package wtf.metio.memoization.jool;
import edu.umd.cs.findbugs.annotations.CheckReturnValue;
import org.jooq.lambda.fi.lang.CheckedRunnable;
import org.jooq.lambda.fi.util.concurrent.CheckedCallable;
import org.jooq.lambda.fi.util.function.CheckedBiConsumer;
import org.jooq.lambda.function.*;
import wtf.metio.memoization.core.MemoizationDefaults;
import java.util.Map;
import java.util.function.BiFunction;
import java.util.function.Function;
import java.util.function.Supplier;
import static java.util.Collections.emptyMap;
import static java.util.function.Function.identity;
import static wtf.metio.memoization.core.ConcurrentMaps.asConcurrentMap;
import static wtf.metio.memoization.core.MemoizationDefaults.staticKey;
/**
*
* Factory for lightweight wrappers that store the result of a potentially expensive function call. Each method of this
* class exposes two of the following features:
*
* Default cache
*
* The memoizer uses the default cache of this factory. Current implementation creates a new
* {@link java.util.concurrent.ConcurrentMap} per memoizer.
*
* Default cache key
*
* The memoizer uses the default key function or {@link Supplier} to calculate the cache key for each call. Either
* uses the natural key (e.g. the input itself) or one of the methods in {@link MemoizationDefaults}.
*
* Custom cache
*
* The memoizer uses a user-provided {@link java.util.concurrent.ConcurrentMap} as its cache. It is possible to add
* values to the cache both before and after the memoizer was created. In case a {@link Map} subtype is provided that is
* not a subclass of {@link java.util.concurrent.ConcurrentMap} as well, the map entries will be copied to a new
* {@link java.util.concurrent.ConcurrentHashMap}.
*
* Custom cache key
*
* The memoizer uses a user-defined function or {@link Supplier} to calculate the cache key for each call.
* Take a look at {@link MemoizationDefaults} for a possible key functions and suppliers.
*
*
* @see CheckedBiConsumer
* @see CheckedCallable
* @see CheckedRunnable
* @see Consumer0
* @see Consumer1
* @see Consumer2
* @see Consumer3
* @see Consumer4
* @see Consumer5
* @see Consumer6
* @see Consumer7
* @see Consumer8
* @see Consumer9
* @see Consumer10
* @see Consumer11
* @see Consumer12
* @see Consumer13
* @see Consumer14
* @see Consumer15
* @see Consumer16
* @see Function0
* @see Function1
* @see Function2
* @see Function3
* @see Function4
* @see Function5
* @see Function6
* @see Function7
* @see Function8
* @see Function9
* @see Function10
* @see Function11
* @see Function12
* @see Function13
* @see Function14
* @see Function15
* @see Function16
* @see Wikipedia: Memoization
*/
public final class MemoizeJool {
private MemoizeJool() {
// factory class
}
/**
*
* Memoizes a {@link Consumer0} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Default cache
* - Default cache key
*
*
* @param consumer The {@link Consumer0} to memoize.
* @return The wrapped {@link Consumer0}.
*/
@CheckReturnValue
public static Consumer0 consumer0(final Consumer0 consumer) {
return consumer0(consumer, emptyMap());
}
/**
*
* Memoizes a {@link Consumer0} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Custom cache
* - Default cache key
*
*
* @param consumer The {@link Consumer0} to memoize.
* @param cache The {@link Map} based cache to use.
* @return The wrapped {@link Consumer0}.
*/
@CheckReturnValue
public static Consumer0 consumer0(
final Consumer0 consumer,
final Map cache) {
return consumer0(consumer, staticKey(), cache);
}
/**
*
* Memoizes a {@link Consumer0} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Default cache
* - Custom cache key
*
*
* @param The type of the cache key.
* @param consumer The {@link Consumer0} to memoize.
* @param keySupplier The {@link Supplier} to get the cache key.
* @return The wrapped {@link Consumer0}.
*/
@CheckReturnValue
public static Consumer0 consumer0(
final Consumer0 consumer,
final Supplier keySupplier) {
return consumer0(consumer, keySupplier, emptyMap());
}
/**
*
* Memoizes a {@link Consumer0} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Custom cache
* - Custom cache key
*
*
* @param The type of the cache key.
* @param consumer The {@link Consumer0} to memoize.
* @param keySupplier The {@link Supplier} to get the cache key.
* @param cache The {@link Map} based cache to use.
* @return The wrapped {@link Consumer0}.
*/
@CheckReturnValue
public static Consumer0 consumer0(
final Consumer0 consumer,
final Supplier keySupplier,
final Map cache) {
return new Consumer0Memoizer<>(asConcurrentMap(cache), keySupplier, consumer);
}
/**
*
* Memoizes a {@link Consumer1} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Default cache
* - Default cache key
*
*
* @param The type of the first parameter.
* @param consumer The {@link Consumer1} to memoize.
* @return The wrapped {@link Consumer1}.
*/
@CheckReturnValue
public static Consumer1 consumer1(final Consumer1 consumer) {
return consumer1(consumer, emptyMap());
}
/**
*
* Memoizes a {@link Consumer1} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Custom cache
* - Default cache key
*
*
* @param The type of the first parameter.
* @param consumer The {@link Consumer1} to memoize.
* @param cache The {@link Map} based cache to use.
* @return The wrapped {@link Consumer1}.
*/
@CheckReturnValue
public static Consumer1 consumer1(
final Consumer1 consumer,
final Map cache) {
return consumer1(consumer, identity(), cache);
}
/**
*
* Memoizes a {@link Consumer1} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Default cache
* - Custom cache key
*
*
* @param The type of the cache key.
* @param The type of the first parameter.
* @param consumer The {@link Consumer1} to memoize.
* @param keyFunction The {@link Function} to compute the cache key.
* @return The wrapped {@link Consumer1}.
*/
@CheckReturnValue
public static Consumer1 consumer1(
final Consumer1 consumer,
final Function keyFunction) {
return consumer1(consumer, keyFunction, emptyMap());
}
/**
*
* Memoizes a {@link Consumer1} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Custom cache
* - Custom cache key
*
*
* @param The type of the cache key.
* @param The type of the first parameter.
* @param consumer The {@link Consumer1} to memoize.
* @param keyFunction The {@link Function} to get the cache key.
* @param cache The {@link Map} based cache to use.
* @return The wrapped {@link Consumer1}.
*/
@CheckReturnValue
public static Consumer1 consumer1(
final Consumer1 consumer,
final Function keyFunction,
final Map cache) {
return new Consumer1Memoizer<>(asConcurrentMap(cache), keyFunction, consumer);
}
/**
*
* Memoizes a {@link Consumer2} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Default cache
* - Default cache key
*
*
* @param The type of the first parameter.
* @param The type of the second parameter.
* @param consumer The {@link Consumer2} to memoize.
* @return The wrapped {@link Consumer2}.
*/
@CheckReturnValue
public static Consumer2 consumer2(final Consumer2 consumer) {
return consumer2(consumer, emptyMap());
}
/**
*
* Memoizes a {@link Consumer2} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Custom cache
* - Default cache key
*
*
* @param The type of the first parameter.
* @param The type of the second parameter.
* @param consumer The {@link Consumer2} to memoize.
* @param cache The {@link Map} based cache to use.
* @return The wrapped {@link Consumer2}.
*/
@CheckReturnValue
public static Consumer2 consumer2(
final Consumer2 consumer,
final Map cache) {
return consumer2(consumer, MemoizationDefaults::hashCodes, cache);
}
/**
*
* Memoizes a {@link Consumer2} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Default cache
* - Custom cache key
*
*
* @param The type of the cache key.
* @param The type of the first parameter.
* @param The type of the second parameter.
* @param consumer The {@link Consumer2} to memoize.
* @param keyFunction The {@link BiFunction} to compute the cache key.
* @return The wrapped {@link Consumer2}.
*/
@CheckReturnValue
public static Consumer2 consumer2(
final Consumer2 consumer,
final BiFunction keyFunction) {
return consumer2(consumer, keyFunction, emptyMap());
}
/**
*
* Memoizes a {@link Consumer2} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Custom cache
* - Custom cache key
*
*
* @param The type of the cache key.
* @param The type of the first parameter.
* @param The type of the second parameter.
* @param consumer The {@link Consumer2} to memoize.
* @param keyFunction The {@link BiFunction} to get the cache key.
* @param cache The {@link Map} based cache to use.
* @return The wrapped {@link Consumer2}.
*/
@CheckReturnValue
public static Consumer2 consumer2(
final Consumer2 consumer,
final BiFunction keyFunction,
final Map cache) {
return new Consumer2Memoizer<>(asConcurrentMap(cache), keyFunction, consumer);
}
/**
*
* Memoizes a {@link Consumer3} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Default cache
* - Default cache key
*
*
* @param The type of the first parameter.
* @param The type of the second parameter.
* @param The type of the third parameter.
* @param consumer The {@link Consumer3} to memoize.
* @return The wrapped {@link Consumer3}.
*/
@CheckReturnValue
public static Consumer3 consumer3(
final Consumer3 consumer) {
return consumer3(consumer, emptyMap());
}
/**
*
* Memoizes a {@link Consumer3} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Custom cache
* - Default cache key
*
*
* @param The type of the first parameter.
* @param The type of the second parameter.
* @param The type of the third parameter.
* @param consumer The {@link Consumer3} to memoize.
* @param cache The {@link Map} based cache to use.
* @return The wrapped {@link Consumer3}.
*/
@CheckReturnValue
public static Consumer3 consumer3(
final Consumer3 consumer,
final Map cache) {
return consumer3(consumer, MemoizationDefaults::hashCodes, cache);
}
/**
*
* Memoizes a {@link Consumer3} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Default cache
* - Custom cache key
*
*
* @param The type of the cache key.
* @param The type of the first parameter.
* @param The type of the second parameter.
* @param The type of the third parameter.
* @param consumer The {@link Consumer3} to memoize.
* @param keyFunction The {@link Function3} to compute the cache key.
* @return The wrapped {@link Consumer3}.
*/
@CheckReturnValue
public static Consumer3 consumer3(
final Consumer3 consumer,
final Function3 keyFunction) {
return consumer3(consumer, keyFunction, emptyMap());
}
/**
*
* Memoizes a {@link Consumer3} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Custom cache
* - Custom cache key
*
*
* @param The type of the cache key.
* @param The type of the first parameter.
* @param The type of the second parameter.
* @param The type of the third parameter.
* @param consumer The {@link Consumer3} to memoize.
* @param keyFunction The {@link Function3} to get the cache key.
* @param cache The {@link Map} based cache to use.
* @return The wrapped {@link Consumer3}.
*/
@CheckReturnValue
public static Consumer3 consumer3(
final Consumer3 consumer,
final Function3 keyFunction,
final Map cache) {
return new Consumer3Memoizer<>(asConcurrentMap(cache), keyFunction, consumer);
}
/**
*
* Memoizes a {@link Consumer4} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Default cache
* - Default cache key
*
*
* @param The type of the first parameter.
* @param The type of the second parameter.
* @param The type of the third parameter.
* @param The type of the fourth parameter.
* @param consumer The {@link Consumer4} to memoize.
* @return The wrapped {@link Consumer4}.
*/
@CheckReturnValue
public static Consumer4 consumer4(
final Consumer4 consumer) {
return consumer4(consumer, emptyMap());
}
/**
*
* Memoizes a {@link Consumer4} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Custom cache
* - Default cache key
*
*
* @param The type of the first parameter.
* @param The type of the second parameter.
* @param The type of the third parameter.
* @param The type of the fourth parameter.
* @param consumer The {@link Consumer4} to memoize.
* @param cache The {@link Map} based cache to use.
* @return The wrapped {@link Consumer4}.
*/
@CheckReturnValue
public static Consumer4 consumer4(
final Consumer4 consumer,
final Map cache) {
return consumer4(consumer, MemoizationDefaults::hashCodes, cache);
}
/**
*
* Memoizes a {@link Consumer4} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Default cache
* - Custom cache key
*
*
* @param The type of the cache key.
* @param The type of the first parameter.
* @param The type of the second parameter.
* @param The type of the third parameter.
* @param The type of the fourth parameter.
* @param consumer The {@link Consumer4} to memoize.
* @param keyFunction The {@link Function4} to compute the cache key.
* @return The wrapped {@link Consumer4}.
*/
@CheckReturnValue
public static Consumer4 consumer4(
final Consumer4 consumer,
final Function4 keyFunction) {
return consumer4(consumer, keyFunction, emptyMap());
}
/**
*
* Memoizes a {@link Consumer4} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Custom cache
* - Custom cache key
*
*
* @param The type of the cache key.
* @param The type of the first parameter.
* @param The type of the second parameter.
* @param The type of the third parameter.
* @param The type of the fourth parameter.
* @param consumer The {@link Consumer4} to memoize.
* @param keyFunction The {@link Function4} to get the cache key.
* @param cache The {@link Map} based cache to use.
* @return The wrapped {@link Consumer4}.
*/
@CheckReturnValue
public static Consumer4 consumer4(
final Consumer4 consumer,
final Function4 keyFunction,
final Map cache) {
return new Consumer4Memoizer<>(asConcurrentMap(cache), keyFunction, consumer);
}
/**
*
* Memoizes a {@link Consumer5} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Default cache
* - Default cache key
*
*
* @param The type of the first parameter.
* @param The type of the second parameter.
* @param The type of the third parameter.
* @param The type of the fourth parameter.
* @param The type of the fifth parameter.
* @param consumer The {@link Consumer5} to memoize.
* @return The wrapped {@link Consumer5}.
*/
@CheckReturnValue
public static Consumer5 consumer5(
final Consumer5 consumer) {
return consumer5(consumer, emptyMap());
}
/**
*
* Memoizes a {@link Consumer5} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Custom cache
* - Default cache key
*
*
* @param The type of the first parameter.
* @param The type of the second parameter.
* @param The type of the third parameter.
* @param The type of the fourth parameter.
* @param The type of the fifth parameter.
* @param consumer The {@link Consumer5} to memoize.
* @param cache The {@link Map} based cache to use.
* @return The wrapped {@link Consumer5}.
*/
@CheckReturnValue
public static Consumer5 consumer5(
final Consumer5 consumer,
final Map cache) {
return consumer5(consumer, MemoizationDefaults::hashCodes, cache);
}
/**
*
* Memoizes a {@link Consumer5} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Default cache
* - Custom cache key
*
*
* @param The type of the cache key.
* @param The type of the first parameter.
* @param The type of the second parameter.
* @param The type of the third parameter.
* @param The type of the fourth parameter.
* @param The type of the fifth parameter.
* @param consumer The {@link Consumer5} to memoize.
* @param keyFunction The {@link Function5} to compute the cache key.
* @return The wrapped {@link Consumer5}.
*/
@CheckReturnValue
public static Consumer5 consumer5(
final Consumer5 consumer,
final Function5 keyFunction) {
return consumer5(consumer, keyFunction, emptyMap());
}
/**
*
* Memoizes a {@link Consumer5} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Custom cache
* - Custom cache key
*
*
* @param The type of the cache key.
* @param The type of the first parameter.
* @param The type of the second parameter.
* @param The type of the third parameter.
* @param The type of the fourth parameter.
* @param The type of the fifth parameter.
* @param consumer The {@link Consumer5} to memoize.
* @param keyFunction The {@link Function5} to get the cache key.
* @param cache The {@link Map} based cache to use.
* @return The wrapped {@link Consumer5}.
*/
@CheckReturnValue
public static Consumer5 consumer5(
final Consumer5 consumer,
final Function5 keyFunction,
final Map cache) {
return new Consumer5Memoizer<>(asConcurrentMap(cache), keyFunction, consumer);
}
/**
*
* Memoizes a {@link Consumer6} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Default cache
* - Default cache key
*
*
* @param The type of the first parameter.
* @param The type of the second parameter.
* @param The type of the third parameter.
* @param The type of the fourth parameter.
* @param The type of the fifth parameter.
* @param The type of the sixth parameter.
* @param consumer The {@link Consumer6} to memoize.
* @return The wrapped {@link Consumer6}.
*/
@CheckReturnValue
public static Consumer6 consumer6(
final Consumer6 consumer) {
return consumer6(consumer, emptyMap());
}
/**
*
* Memoizes a {@link Consumer6} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Custom cache
* - Default cache key
*
*
* @param The type of the first parameter.
* @param The type of the second parameter.
* @param The type of the third parameter.
* @param The type of the fourth parameter.
* @param The type of the fifth parameter.
* @param The type of the sixth parameter.
* @param consumer The {@link Consumer6} to memoize.
* @param cache The {@link Map} based cache to use.
* @return The wrapped {@link Consumer6}.
*/
@CheckReturnValue
public static Consumer6 consumer6(
final Consumer6 consumer,
final Map cache) {
return consumer6(consumer, MemoizationDefaults::hashCodes, cache);
}
/**
*
* Memoizes a {@link Consumer6} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Default cache
* - Custom cache key
*
*
* @param The type of the cache key.
* @param The type of the first parameter.
* @param The type of the second parameter.
* @param The type of the third parameter.
* @param The type of the fourth parameter.
* @param The type of the fifth parameter.
* @param The type of the sixth parameter.
* @param consumer The {@link Consumer6} to memoize.
* @param keyFunction The {@link Function6} to compute the cache key.
* @return The wrapped {@link Consumer6}.
*/
@CheckReturnValue
public static Consumer6 consumer6(
final Consumer6 consumer,
final Function6 keyFunction) {
return consumer6(consumer, keyFunction, emptyMap());
}
/**
*
* Memoizes a {@link Consumer6} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Custom cache
* - Custom cache key
*
*
* @param The type of the cache key.
* @param The type of the first parameter.
* @param The type of the second parameter.
* @param The type of the third parameter.
* @param The type of the fourth parameter.
* @param The type of the fifth parameter.
* @param The type of the sixth parameter.
* @param consumer The {@link Consumer6} to memoize.
* @param keyFunction The {@link Function6} to get the cache key.
* @param cache The {@link Map} based cache to use.
* @return The wrapped {@link Consumer6}.
*/
@CheckReturnValue
public static Consumer6 consumer6(
final Consumer6 consumer,
final Function6 keyFunction,
final Map cache) {
return new Consumer6Memoizer<>(asConcurrentMap(cache), keyFunction, consumer);
}
/**
*
* Memoizes a {@link Consumer7} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Default cache
* - Default cache key
*
*
* @param The type of the first parameter.
* @param The type of the second parameter.
* @param The type of the third parameter.
* @param The type of the fourth parameter.
* @param The type of the fifth parameter.
* @param The type of the sixth parameter.
* @param The type of the seventh parameter.
* @param consumer The {@link Consumer7} to memoize.
* @return The wrapped {@link Consumer7}.
*/
@CheckReturnValue
public static Consumer7 consumer7(
final Consumer7 consumer) {
return consumer7(consumer, emptyMap());
}
/**
*
* Memoizes a {@link Consumer7} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Custom cache
* - Default cache key
*
*
* @param The type of the first parameter.
* @param The type of the second parameter.
* @param The type of the third parameter.
* @param The type of the fourth parameter.
* @param The type of the fifth parameter.
* @param The type of the sixth parameter.
* @param The type of the seventh parameter.
* @param consumer The {@link Consumer7} to memoize.
* @param cache The {@link Map} based cache to use.
* @return The wrapped {@link Consumer7}.
*/
@CheckReturnValue
public static Consumer7 consumer7(
final Consumer7 consumer,
final Map cache) {
return consumer7(consumer, MemoizationDefaults::hashCodes, cache);
}
/**
*
* Memoizes a {@link Consumer7} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Default cache
* - Custom cache key
*
*
* @param The type of the cache key.
* @param The type of the first parameter.
* @param The type of the second parameter.
* @param The type of the third parameter.
* @param The type of the fourth parameter.
* @param The type of the fifth parameter.
* @param The type of the sixth parameter.
* @param The type of the seventh parameter.
* @param consumer The {@link Consumer7} to memoize.
* @param keyFunction The {@link Function7} to compute the cache key.
* @return The wrapped {@link Consumer7}.
*/
@CheckReturnValue
public static Consumer7 consumer7(
final Consumer7 consumer,
final Function7 keyFunction) {
return consumer7(consumer, keyFunction, emptyMap());
}
/**
*
* Memoizes a {@link Consumer7} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Custom cache
* - Custom cache key
*
*
* @param The type of the cache key.
* @param The type of the first parameter.
* @param The type of the second parameter.
* @param The type of the third parameter.
* @param The type of the fourth parameter.
* @param The type of the fifth parameter.
* @param The type of the sixth parameter.
* @param The type of the seventh parameter.
* @param consumer The {@link Consumer7} to memoize.
* @param keyFunction The {@link Function7} to get the cache key.
* @param cache The {@link Map} based cache to use.
* @return The wrapped {@link Consumer7}.
*/
@CheckReturnValue
public static Consumer7 consumer7(
final Consumer7 consumer,
final Function7 keyFunction,
final Map cache) {
return new Consumer7Memoizer<>(asConcurrentMap(cache), keyFunction, consumer);
}
/**
*
* Memoizes a {@link Consumer8} in a {@link java.util.concurrent.ConcurrentMap}.
*
*
*
* - Default cache
* - Default cache key
*
*
* @param The type of the first parameter.
* @param The type of the second parameter.
* @param The type of the third parameter.
* @param The type of the fourth parameter.
* @param