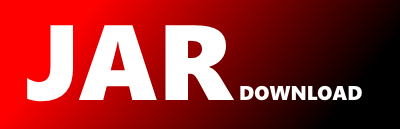
wtf.metio.yosql.models.configuration.ImmutableSqlParameter Maven / Gradle / Ivy
package wtf.metio.yosql.models.configuration;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.squareup.javapoet.TypeName;
import java.util.Objects;
import java.util.Optional;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link SqlParameter}.
*
* Use the builder to create immutable instances:
* {@code ImmutableSqlParameter.builder()}.
*/
@Generated(from = "SqlParameter", generator = "Immutables")
@SuppressWarnings({"all"})
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
public final class ImmutableSqlParameter implements SqlParameter {
private final String name;
private final String type;
private final int[] indices;
private final SqlParameterVariant variant;
private final Integer sqlType;
private final Integer scale;
private ImmutableSqlParameter(
String name,
String type,
int[] indices,
SqlParameterVariant variant,
Integer sqlType,
Integer scale) {
this.name = name;
this.type = type;
this.indices = indices;
this.variant = variant;
this.sqlType = sqlType;
this.scale = scale;
}
/**
* @return The name of the parameter.
*/
@JsonProperty("name")
@Override
public Optional name() {
return Optional.ofNullable(name);
}
/**
* @return The fully-qualified type name.
*/
@JsonProperty("type")
@Override
public Optional type() {
return Optional.ofNullable(type);
}
/**
* @return The indices in the SQL statement that match this parameter.
*/
@JsonProperty("indices")
@JsonIgnore
@Override
public Optional indices() {
return Optional.ofNullable(indices);
}
/**
* @return The variant of this parameter.
*/
@JsonProperty("variant")
@Override
public Optional variant() {
return Optional.ofNullable(variant);
}
/**
* @return The JDBC type of this parameter.
*/
@JsonProperty("sqlType")
@Override
public Optional sqlType() {
return Optional.ofNullable(sqlType);
}
/**
* @return The scale of this parameter in case its sqlType is numeric/decimal.
*/
@JsonProperty("scale")
@Override
public Optional scale() {
return Optional.ofNullable(scale);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SqlParameter#name() name} attribute.
* @param value The value for name
* @return A modified copy of {@code this} object
*/
public final ImmutableSqlParameter withName(String value) {
String newValue = Objects.requireNonNull(value, "name");
if (Objects.equals(this.name, newValue)) return this;
return new ImmutableSqlParameter(newValue, this.type, this.indices, this.variant, this.sqlType, this.scale);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SqlParameter#name() name} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for name
* @return A modified copy of {@code this} object
*/
public final ImmutableSqlParameter withName(Optional optional) {
String value = optional.orElse(null);
if (Objects.equals(this.name, value)) return this;
return new ImmutableSqlParameter(value, this.type, this.indices, this.variant, this.sqlType, this.scale);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SqlParameter#type() type} attribute.
* @param value The value for type
* @return A modified copy of {@code this} object
*/
public final ImmutableSqlParameter withType(String value) {
String newValue = Objects.requireNonNull(value, "type");
if (Objects.equals(this.type, newValue)) return this;
return new ImmutableSqlParameter(this.name, newValue, this.indices, this.variant, this.sqlType, this.scale);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SqlParameter#type() type} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for type
* @return A modified copy of {@code this} object
*/
public final ImmutableSqlParameter withType(Optional optional) {
String value = optional.orElse(null);
if (Objects.equals(this.type, value)) return this;
return new ImmutableSqlParameter(this.name, value, this.indices, this.variant, this.sqlType, this.scale);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SqlParameter#indices() indices} attribute.
* @param value The value for indices
* @return A modified copy of {@code this} object
*/
public final ImmutableSqlParameter withIndices(int[] value) {
int[] newValue = Objects.requireNonNull(value, "indices");
if (this.indices == newValue) return this;
return new ImmutableSqlParameter(this.name, this.type, newValue, this.variant, this.sqlType, this.scale);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SqlParameter#indices() indices} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for indices
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final ImmutableSqlParameter withIndices(Optional extends int[]> optional) {
int[] value = optional.orElse(null);
if (this.indices == value) return this;
return new ImmutableSqlParameter(this.name, this.type, value, this.variant, this.sqlType, this.scale);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SqlParameter#variant() variant} attribute.
* @param value The value for variant
* @return A modified copy of {@code this} object
*/
public final ImmutableSqlParameter withVariant(SqlParameterVariant value) {
SqlParameterVariant newValue = Objects.requireNonNull(value, "variant");
if (this.variant == newValue) return this;
return new ImmutableSqlParameter(this.name, this.type, this.indices, newValue, this.sqlType, this.scale);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SqlParameter#variant() variant} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for variant
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final ImmutableSqlParameter withVariant(Optional extends SqlParameterVariant> optional) {
SqlParameterVariant value = optional.orElse(null);
if (this.variant == value) return this;
return new ImmutableSqlParameter(this.name, this.type, this.indices, value, this.sqlType, this.scale);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SqlParameter#sqlType() sqlType} attribute.
* @param value The value for sqlType
* @return A modified copy of {@code this} object
*/
public final ImmutableSqlParameter withSqlType(int value) {
Integer newValue = value;
if (Objects.equals(this.sqlType, newValue)) return this;
return new ImmutableSqlParameter(this.name, this.type, this.indices, this.variant, newValue, this.scale);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SqlParameter#sqlType() sqlType} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for sqlType
* @return A modified copy of {@code this} object
*/
public final ImmutableSqlParameter withSqlType(Optional optional) {
Integer value = optional.orElse(null);
if (Objects.equals(this.sqlType, value)) return this;
return new ImmutableSqlParameter(this.name, this.type, this.indices, this.variant, value, this.scale);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SqlParameter#scale() scale} attribute.
* @param value The value for scale
* @return A modified copy of {@code this} object
*/
public final ImmutableSqlParameter withScale(int value) {
Integer newValue = value;
if (Objects.equals(this.scale, newValue)) return this;
return new ImmutableSqlParameter(this.name, this.type, this.indices, this.variant, this.sqlType, newValue);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SqlParameter#scale() scale} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for scale
* @return A modified copy of {@code this} object
*/
public final ImmutableSqlParameter withScale(Optional optional) {
Integer value = optional.orElse(null);
if (Objects.equals(this.scale, value)) return this;
return new ImmutableSqlParameter(this.name, this.type, this.indices, this.variant, this.sqlType, value);
}
/**
* This instance is equal to all instances of {@code ImmutableSqlParameter} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableSqlParameter
&& equalTo(0, (ImmutableSqlParameter) another);
}
private boolean equalTo(int synthetic, ImmutableSqlParameter another) {
return Objects.equals(name, another.name)
&& Objects.equals(type, another.type)
&& Objects.equals(indices, another.indices)
&& Objects.equals(variant, another.variant)
&& Objects.equals(sqlType, another.sqlType)
&& Objects.equals(scale, another.scale);
}
/**
* Computes a hash code from attributes: {@code name}, {@code type}, {@code indices}, {@code variant}, {@code sqlType}, {@code scale}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(name);
h += (h << 5) + Objects.hashCode(type);
h += (h << 5) + Objects.hashCode(indices);
h += (h << 5) + Objects.hashCode(variant);
h += (h << 5) + Objects.hashCode(sqlType);
h += (h << 5) + Objects.hashCode(scale);
return h;
}
/**
* Prints the immutable value {@code SqlParameter} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("SqlParameter{");
if (name != null) {
builder.append("name=").append(name);
}
if (type != null) {
if (builder.length() > 13) builder.append(", ");
builder.append("type=").append(type);
}
if (indices != null) {
if (builder.length() > 13) builder.append(", ");
builder.append("indices=").append(indices);
}
if (variant != null) {
if (builder.length() > 13) builder.append(", ");
builder.append("variant=").append(variant);
}
if (sqlType != null) {
if (builder.length() > 13) builder.append(", ");
builder.append("sqlType=").append(sqlType);
}
if (scale != null) {
if (builder.length() > 13) builder.append(", ");
builder.append("scale=").append(scale);
}
return builder.append("}").toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "SqlParameter", generator = "Immutables")
@Deprecated
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements SqlParameter {
Optional name = Optional.empty();
boolean nameIsSet;
Optional type = Optional.empty();
boolean typeIsSet;
Optional indices = Optional.empty();
boolean indicesIsSet;
Optional variant = Optional.empty();
boolean variantIsSet;
Optional sqlType = Optional.empty();
boolean sqlTypeIsSet;
Optional scale = Optional.empty();
boolean scaleIsSet;
@JsonProperty("name")
public void setName(Optional name) {
this.name = name;
this.nameIsSet = true;
}
@JsonProperty("type")
public void setType(Optional type) {
this.type = type;
this.typeIsSet = true;
}
@JsonProperty("indices")
@JsonIgnore
public void setIndices(Optional indices) {
this.indices = indices;
this.indicesIsSet = true;
}
@JsonProperty("variant")
public void setVariant(Optional variant) {
this.variant = variant;
this.variantIsSet = true;
}
@JsonProperty("sqlType")
public void setSqlType(Optional sqlType) {
this.sqlType = sqlType;
this.sqlTypeIsSet = true;
}
@JsonProperty("scale")
public void setScale(Optional scale) {
this.scale = scale;
this.scaleIsSet = true;
}
@Override
public Optional name() { throw new UnsupportedOperationException(); }
@Override
public Optional type() { throw new UnsupportedOperationException(); }
@Override
public Optional indices() { throw new UnsupportedOperationException(); }
@Override
public Optional variant() { throw new UnsupportedOperationException(); }
@Override
public Optional sqlType() { throw new UnsupportedOperationException(); }
@Override
public Optional scale() { throw new UnsupportedOperationException(); }
@Override
public Optional typeName() { throw new UnsupportedOperationException(); }
@Override
public boolean hasIndices() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableSqlParameter fromJson(Json json) {
ImmutableSqlParameter.Builder builder = ImmutableSqlParameter.builder();
if (json.nameIsSet) {
builder.setName(json.name);
}
if (json.typeIsSet) {
builder.setType(json.type);
}
if (json.indicesIsSet) {
builder.setIndices(json.indices);
}
if (json.variantIsSet) {
builder.setVariant(json.variant);
}
if (json.sqlTypeIsSet) {
builder.setSqlType(json.sqlType);
}
if (json.scaleIsSet) {
builder.setScale(json.scale);
}
return builder.build();
}
private transient volatile long lazyInitBitmap;
private static final long TYPE_NAME_LAZY_INIT_BIT = 0x1L;
private transient Optional typeName;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link SqlParameter#typeName() typeName} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* In case of any exception or error thrown by the lazy value initializer,
* the result will not be memoised (i.e. remembered) and on next call computation
* will be attempted again.
* @return A lazily initialized value of the {@code typeName} attribute
*/
@Override
public Optional typeName() {
if ((lazyInitBitmap & TYPE_NAME_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & TYPE_NAME_LAZY_INIT_BIT) == 0) {
this.typeName = Objects.requireNonNull(SqlParameter.super.typeName(), "typeName");
lazyInitBitmap |= TYPE_NAME_LAZY_INIT_BIT;
}
}
}
return typeName;
}
private static final long HAS_INDICES_LAZY_INIT_BIT = 0x2L;
private transient boolean hasIndices;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link SqlParameter#hasIndices() hasIndices} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* In case of any exception or error thrown by the lazy value initializer,
* the result will not be memoised (i.e. remembered) and on next call computation
* will be attempted again.
* @return A lazily initialized value of the {@code hasIndices} attribute
*/
@Override
public boolean hasIndices() {
if ((lazyInitBitmap & HAS_INDICES_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & HAS_INDICES_LAZY_INIT_BIT) == 0) {
this.hasIndices = SqlParameter.super.hasIndices();
lazyInitBitmap |= HAS_INDICES_LAZY_INIT_BIT;
}
}
}
return hasIndices;
}
/**
* Creates an immutable copy of a {@link SqlParameter} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable SqlParameter instance
*/
public static ImmutableSqlParameter copyOf(SqlParameter instance) {
if (instance instanceof ImmutableSqlParameter) {
return (ImmutableSqlParameter) instance;
}
return ImmutableSqlParameter.builder()
.setName(instance.name())
.setType(instance.type())
.setIndices(instance.indices())
.setVariant(instance.variant())
.setSqlType(instance.sqlType())
.setScale(instance.scale())
.build();
}
/**
* Creates a builder for {@link ImmutableSqlParameter ImmutableSqlParameter}.
*
* ImmutableSqlParameter.builder()
* .setName(String) // optional {@link SqlParameter#name() name}
* .setType(String) // optional {@link SqlParameter#type() type}
* .setIndices(int[]) // optional {@link SqlParameter#indices() indices}
* .setVariant(wtf.metio.yosql.models.configuration.SqlParameterVariant) // optional {@link SqlParameter#variant() variant}
* .setSqlType(Integer) // optional {@link SqlParameter#sqlType() sqlType}
* .setScale(Integer) // optional {@link SqlParameter#scale() scale}
* .build();
*
* @return A new ImmutableSqlParameter builder
*/
public static ImmutableSqlParameter.Builder builder() {
return new ImmutableSqlParameter.Builder();
}
/**
* Builds instances of type {@link ImmutableSqlParameter ImmutableSqlParameter}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "SqlParameter", generator = "Immutables")
public static final class Builder {
private static final long OPT_BIT_NAME = 0x1L;
private static final long OPT_BIT_TYPE = 0x2L;
private static final long OPT_BIT_INDICES = 0x4L;
private static final long OPT_BIT_VARIANT = 0x8L;
private static final long OPT_BIT_SQL_TYPE = 0x10L;
private static final long OPT_BIT_SCALE = 0x20L;
private long optBits;
private String name;
private String type;
private int[] indices;
private SqlParameterVariant variant;
private Integer sqlType;
private Integer scale;
private Builder() {
}
/**
* Initializes the optional value {@link SqlParameter#name() name} to name.
* @param name The value for name
* @return {@code this} builder for chained invocation
*/
public final Builder setName(String name) {
checkNotIsSet(nameIsSet(), "name");
this.name = Objects.requireNonNull(name, "name");
optBits |= OPT_BIT_NAME;
return this;
}
/**
* Initializes the optional value {@link SqlParameter#name() name} to name.
* @param name The value for name
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("name")
public final Builder setName(Optional name) {
checkNotIsSet(nameIsSet(), "name");
this.name = name.orElse(null);
optBits |= OPT_BIT_NAME;
return this;
}
/**
* Initializes the optional value {@link SqlParameter#type() type} to type.
* @param type The value for type
* @return {@code this} builder for chained invocation
*/
public final Builder setType(String type) {
checkNotIsSet(typeIsSet(), "type");
this.type = Objects.requireNonNull(type, "type");
optBits |= OPT_BIT_TYPE;
return this;
}
/**
* Initializes the optional value {@link SqlParameter#type() type} to type.
* @param type The value for type
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("type")
public final Builder setType(Optional type) {
checkNotIsSet(typeIsSet(), "type");
this.type = type.orElse(null);
optBits |= OPT_BIT_TYPE;
return this;
}
/**
* Initializes the optional value {@link SqlParameter#indices() indices} to indices.
* @param indices The value for indices
* @return {@code this} builder for chained invocation
*/
public final Builder setIndices(int[] indices) {
checkNotIsSet(indicesIsSet(), "indices");
this.indices = Objects.requireNonNull(indices, "indices");
optBits |= OPT_BIT_INDICES;
return this;
}
/**
* Initializes the optional value {@link SqlParameter#indices() indices} to indices.
* @param indices The value for indices
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("indices")
@JsonIgnore
public final Builder setIndices(Optional extends int[]> indices) {
checkNotIsSet(indicesIsSet(), "indices");
this.indices = indices.orElse(null);
optBits |= OPT_BIT_INDICES;
return this;
}
/**
* Initializes the optional value {@link SqlParameter#variant() variant} to variant.
* @param variant The value for variant
* @return {@code this} builder for chained invocation
*/
public final Builder setVariant(SqlParameterVariant variant) {
checkNotIsSet(variantIsSet(), "variant");
this.variant = Objects.requireNonNull(variant, "variant");
optBits |= OPT_BIT_VARIANT;
return this;
}
/**
* Initializes the optional value {@link SqlParameter#variant() variant} to variant.
* @param variant The value for variant
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("variant")
public final Builder setVariant(Optional extends SqlParameterVariant> variant) {
checkNotIsSet(variantIsSet(), "variant");
this.variant = variant.orElse(null);
optBits |= OPT_BIT_VARIANT;
return this;
}
/**
* Initializes the optional value {@link SqlParameter#sqlType() sqlType} to sqlType.
* @param sqlType The value for sqlType
* @return {@code this} builder for chained invocation
*/
public final Builder setSqlType(int sqlType) {
checkNotIsSet(sqlTypeIsSet(), "sqlType");
this.sqlType = sqlType;
optBits |= OPT_BIT_SQL_TYPE;
return this;
}
/**
* Initializes the optional value {@link SqlParameter#sqlType() sqlType} to sqlType.
* @param sqlType The value for sqlType
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("sqlType")
public final Builder setSqlType(Optional sqlType) {
checkNotIsSet(sqlTypeIsSet(), "sqlType");
this.sqlType = sqlType.orElse(null);
optBits |= OPT_BIT_SQL_TYPE;
return this;
}
/**
* Initializes the optional value {@link SqlParameter#scale() scale} to scale.
* @param scale The value for scale
* @return {@code this} builder for chained invocation
*/
public final Builder setScale(int scale) {
checkNotIsSet(scaleIsSet(), "scale");
this.scale = scale;
optBits |= OPT_BIT_SCALE;
return this;
}
/**
* Initializes the optional value {@link SqlParameter#scale() scale} to scale.
* @param scale The value for scale
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("scale")
public final Builder setScale(Optional scale) {
checkNotIsSet(scaleIsSet(), "scale");
this.scale = scale.orElse(null);
optBits |= OPT_BIT_SCALE;
return this;
}
/**
* Builds a new {@link ImmutableSqlParameter ImmutableSqlParameter}.
* @return An immutable instance of SqlParameter
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableSqlParameter build() {
return new ImmutableSqlParameter(name, type, indices, variant, sqlType, scale);
}
private boolean nameIsSet() {
return (optBits & OPT_BIT_NAME) != 0;
}
private boolean typeIsSet() {
return (optBits & OPT_BIT_TYPE) != 0;
}
private boolean indicesIsSet() {
return (optBits & OPT_BIT_INDICES) != 0;
}
private boolean variantIsSet() {
return (optBits & OPT_BIT_VARIANT) != 0;
}
private boolean sqlTypeIsSet() {
return (optBits & OPT_BIT_SQL_TYPE) != 0;
}
private boolean scaleIsSet() {
return (optBits & OPT_BIT_SCALE) != 0;
}
private static void checkNotIsSet(boolean isSet, String name) {
if (isSet) throw new IllegalStateException("Builder of SqlParameter is strict, attribute is already set: ".concat(name));
}
}
}