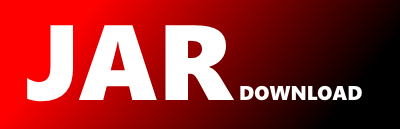
xin.xihc.jba.sql.Update Maven / Gradle / Ivy
package xin.xihc.jba.sql;
import xin.xihc.jba.scan.TableManager;
import xin.xihc.jba.sql.clause.Eq;
import xin.xihc.jba.sql.clause.NativeExp;
import java.util.LinkedList;
import java.util.List;
/**
* Update语句生成器
*
* @author Leo.Xi
* @date 2020/3/5
* @since 1.0
**/
public class Update implements SqlType {
/**
* TABLE 表
*/
private String tableName;
/**
* 排序字段列表
*/
private final List sets = new LinkedList<>();
private KV kv = new KV("t");
private Where where;
private Update() {
}
/**
* 更新的表
*
* @param clazz 实体类
* @return
*/
public static Update from(Class> clazz) {
Update update = new Update();
update.tableName = TableManager.getTable(clazz).getTableName();
return update;
}
/**
* 更新的表
*
* @param tableName 表名
* @return
*/
public static Update from(String tableName) {
Update update = new Update();
update.tableName = tableName;
return update;
}
/**
* set的属性
*
* @param eq 属性表达式
* @return
*/
public Update set(T eq) {
this.sets.add(eq.toSql(this.kv));
return this;
}
/**
* set的属性
*
* @param eq 属性表达式
* @return
*/
public Update set(NativeExp eq) {
this.sets.add(eq.toSql(this.kv));
return this;
}
/**
* where表达式
*
* @param where where子句
* @return
*/
public Update where(Where where) {
this.where = where;
if (null != where) {
this.kv.merge(where.getKv());
}
return this;
}
public KV getKv() {
return this.kv;
}
/**
* 转为SQL语句
*
* @return sql
* @author Leo.Xi
* @date 2020/1/14
* @since 0.0.1
*/
@Override
public String toSql() {
String sql = action() + this.tableName + SqlConstants.SET + String.join(",", this.sets);
if (this.where != null) {
sql += this.where.toSql();
}
return sql;
}
/**
* 操作类型
*
* @return SELECT、WHERE、UPDATE、ORDER BY、
* @author Leo.Xi
* @date 2020/1/18
* @since 0.0.1
*/
@Override
public String action() {
return SqlConstants.UPDATE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy