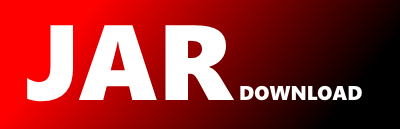
xin.xihc.jba.sql.Where Maven / Gradle / Ivy
package xin.xihc.jba.sql;
import xin.xihc.jba.sql.clause.Clause;
import java.util.LinkedList;
import java.util.List;
import static xin.xihc.jba.sql.SqlConstants.*;
/**
* Where子句
*
* @author Leo.Xi
* @date 2020/1/14
* @since 1.0
**/
public class Where implements SqlType {
private KV kv = new KV();
private final List wheres = new LinkedList<>();
public Where() {
}
public Where(KV kv) {
if (kv != null) {
this.kv = kv;
}
}
/**
* where的and条件
*
* @param clause 条件
* @return
*/
public Where and(Clause> clause) {
if (null != clause) {
wheres.add(AND);
wheres.add(clause.toSql(this.kv));
}
return this;
}
/**
* where的OR的条件列表
*
* @param clauses 条件列表
* @return
*/
public Where or(Clause>... clauses) {
if (clauses.length > 0) {
wheres.add(OR);
StringBuilder temp = new StringBuilder("(");
temp.append(clauses[0].toSql(this.kv));
for (int i = 1; i < clauses.length; i++) {
temp.append(AND).append(clauses[i].toSql(this.kv));
}
temp.append(")");
wheres.add(temp.toString());
}
return this;
}
public KV getKv() {
return this.kv;
}
/**
* 去除where首尾的AND、OR
*
* @author Leo.Xi
* @date 2020/1/18
* @since 0.0.1
*/
public List trimWhere() {
if (this.wheres.size() < 1) {
return this.wheres;
}
String first = this.wheres.get(0);
String last = this.wheres.get(this.wheres.size() - 1);
if (last.equals(AND) || last.equals(OR)) {
this.wheres.remove(this.wheres.size() - 1);
}
if (first.equals(AND) || first.equals(OR)) {
this.wheres.remove(0);
}
return this.wheres;
}
/**
* 转为SQL语句
*
* @return sql
* @author Leo.Xi
* @date 2020/1/14
* @since 0.0.1
*/
@Override
public String toSql() {
if (this.wheres.size() > 0) {
return action() + String.join("", trimWhere());
}
return BLANK;
}
/**
* 操作类型
*
* @return SELECT、WHERE、UPDATE、ORDER BY、
* @author Leo.Xi
* @date 2020/1/18
* @since 0.0.1
*/
@Override
public String action() {
return WHERE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy