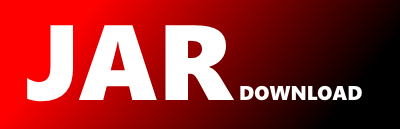
xyz.block.ftl.v1.language.LanguageServiceGrpc Maven / Gradle / Ivy
package xyz.block.ftl.v1.language;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*
* LanguageService allows a plugin to add support for a programming language.
*
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.65.1)",
comments = "Source: xyz/block/ftl/v1/language/language.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class LanguageServiceGrpc {
private LanguageServiceGrpc() {}
public static final java.lang.String SERVICE_NAME = "xyz.block.ftl.v1.language.LanguageService";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getPingMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Ping",
requestType = xyz.block.ftl.v1.PingRequest.class,
responseType = xyz.block.ftl.v1.PingResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getPingMethod() {
io.grpc.MethodDescriptor getPingMethod;
if ((getPingMethod = LanguageServiceGrpc.getPingMethod) == null) {
synchronized (LanguageServiceGrpc.class) {
if ((getPingMethod = LanguageServiceGrpc.getPingMethod) == null) {
LanguageServiceGrpc.getPingMethod = getPingMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Ping"))
.setSafe(true)
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xyz.block.ftl.v1.PingRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xyz.block.ftl.v1.PingResponse.getDefaultInstance()))
.setSchemaDescriptor(new LanguageServiceMethodDescriptorSupplier("Ping"))
.build();
}
}
}
return getPingMethod;
}
private static volatile io.grpc.MethodDescriptor getGetCreateModuleFlagsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetCreateModuleFlags",
requestType = xyz.block.ftl.v1.language.GetCreateModuleFlagsRequest.class,
responseType = xyz.block.ftl.v1.language.GetCreateModuleFlagsResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetCreateModuleFlagsMethod() {
io.grpc.MethodDescriptor getGetCreateModuleFlagsMethod;
if ((getGetCreateModuleFlagsMethod = LanguageServiceGrpc.getGetCreateModuleFlagsMethod) == null) {
synchronized (LanguageServiceGrpc.class) {
if ((getGetCreateModuleFlagsMethod = LanguageServiceGrpc.getGetCreateModuleFlagsMethod) == null) {
LanguageServiceGrpc.getGetCreateModuleFlagsMethod = getGetCreateModuleFlagsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetCreateModuleFlags"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xyz.block.ftl.v1.language.GetCreateModuleFlagsRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xyz.block.ftl.v1.language.GetCreateModuleFlagsResponse.getDefaultInstance()))
.setSchemaDescriptor(new LanguageServiceMethodDescriptorSupplier("GetCreateModuleFlags"))
.build();
}
}
}
return getGetCreateModuleFlagsMethod;
}
private static volatile io.grpc.MethodDescriptor getCreateModuleMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "CreateModule",
requestType = xyz.block.ftl.v1.language.CreateModuleRequest.class,
responseType = xyz.block.ftl.v1.language.CreateModuleResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getCreateModuleMethod() {
io.grpc.MethodDescriptor getCreateModuleMethod;
if ((getCreateModuleMethod = LanguageServiceGrpc.getCreateModuleMethod) == null) {
synchronized (LanguageServiceGrpc.class) {
if ((getCreateModuleMethod = LanguageServiceGrpc.getCreateModuleMethod) == null) {
LanguageServiceGrpc.getCreateModuleMethod = getCreateModuleMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "CreateModule"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xyz.block.ftl.v1.language.CreateModuleRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xyz.block.ftl.v1.language.CreateModuleResponse.getDefaultInstance()))
.setSchemaDescriptor(new LanguageServiceMethodDescriptorSupplier("CreateModule"))
.build();
}
}
}
return getCreateModuleMethod;
}
private static volatile io.grpc.MethodDescriptor getModuleConfigDefaultsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ModuleConfigDefaults",
requestType = xyz.block.ftl.v1.language.ModuleConfigDefaultsRequest.class,
responseType = xyz.block.ftl.v1.language.ModuleConfigDefaultsResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getModuleConfigDefaultsMethod() {
io.grpc.MethodDescriptor getModuleConfigDefaultsMethod;
if ((getModuleConfigDefaultsMethod = LanguageServiceGrpc.getModuleConfigDefaultsMethod) == null) {
synchronized (LanguageServiceGrpc.class) {
if ((getModuleConfigDefaultsMethod = LanguageServiceGrpc.getModuleConfigDefaultsMethod) == null) {
LanguageServiceGrpc.getModuleConfigDefaultsMethod = getModuleConfigDefaultsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ModuleConfigDefaults"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xyz.block.ftl.v1.language.ModuleConfigDefaultsRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xyz.block.ftl.v1.language.ModuleConfigDefaultsResponse.getDefaultInstance()))
.setSchemaDescriptor(new LanguageServiceMethodDescriptorSupplier("ModuleConfigDefaults"))
.build();
}
}
}
return getModuleConfigDefaultsMethod;
}
private static volatile io.grpc.MethodDescriptor getGetDependenciesMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetDependencies",
requestType = xyz.block.ftl.v1.language.DependenciesRequest.class,
responseType = xyz.block.ftl.v1.language.DependenciesResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetDependenciesMethod() {
io.grpc.MethodDescriptor getGetDependenciesMethod;
if ((getGetDependenciesMethod = LanguageServiceGrpc.getGetDependenciesMethod) == null) {
synchronized (LanguageServiceGrpc.class) {
if ((getGetDependenciesMethod = LanguageServiceGrpc.getGetDependenciesMethod) == null) {
LanguageServiceGrpc.getGetDependenciesMethod = getGetDependenciesMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetDependencies"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xyz.block.ftl.v1.language.DependenciesRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xyz.block.ftl.v1.language.DependenciesResponse.getDefaultInstance()))
.setSchemaDescriptor(new LanguageServiceMethodDescriptorSupplier("GetDependencies"))
.build();
}
}
}
return getGetDependenciesMethod;
}
private static volatile io.grpc.MethodDescriptor getBuildMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Build",
requestType = xyz.block.ftl.v1.language.BuildRequest.class,
responseType = xyz.block.ftl.v1.language.BuildEvent.class,
methodType = io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
public static io.grpc.MethodDescriptor getBuildMethod() {
io.grpc.MethodDescriptor getBuildMethod;
if ((getBuildMethod = LanguageServiceGrpc.getBuildMethod) == null) {
synchronized (LanguageServiceGrpc.class) {
if ((getBuildMethod = LanguageServiceGrpc.getBuildMethod) == null) {
LanguageServiceGrpc.getBuildMethod = getBuildMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Build"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xyz.block.ftl.v1.language.BuildRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xyz.block.ftl.v1.language.BuildEvent.getDefaultInstance()))
.setSchemaDescriptor(new LanguageServiceMethodDescriptorSupplier("Build"))
.build();
}
}
}
return getBuildMethod;
}
private static volatile io.grpc.MethodDescriptor getBuildContextUpdatedMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "BuildContextUpdated",
requestType = xyz.block.ftl.v1.language.BuildContextUpdatedRequest.class,
responseType = xyz.block.ftl.v1.language.BuildContextUpdatedResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getBuildContextUpdatedMethod() {
io.grpc.MethodDescriptor getBuildContextUpdatedMethod;
if ((getBuildContextUpdatedMethod = LanguageServiceGrpc.getBuildContextUpdatedMethod) == null) {
synchronized (LanguageServiceGrpc.class) {
if ((getBuildContextUpdatedMethod = LanguageServiceGrpc.getBuildContextUpdatedMethod) == null) {
LanguageServiceGrpc.getBuildContextUpdatedMethod = getBuildContextUpdatedMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "BuildContextUpdated"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xyz.block.ftl.v1.language.BuildContextUpdatedRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xyz.block.ftl.v1.language.BuildContextUpdatedResponse.getDefaultInstance()))
.setSchemaDescriptor(new LanguageServiceMethodDescriptorSupplier("BuildContextUpdated"))
.build();
}
}
}
return getBuildContextUpdatedMethod;
}
private static volatile io.grpc.MethodDescriptor getGenerateStubsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GenerateStubs",
requestType = xyz.block.ftl.v1.language.GenerateStubsRequest.class,
responseType = xyz.block.ftl.v1.language.GenerateStubsResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGenerateStubsMethod() {
io.grpc.MethodDescriptor getGenerateStubsMethod;
if ((getGenerateStubsMethod = LanguageServiceGrpc.getGenerateStubsMethod) == null) {
synchronized (LanguageServiceGrpc.class) {
if ((getGenerateStubsMethod = LanguageServiceGrpc.getGenerateStubsMethod) == null) {
LanguageServiceGrpc.getGenerateStubsMethod = getGenerateStubsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GenerateStubs"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xyz.block.ftl.v1.language.GenerateStubsRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xyz.block.ftl.v1.language.GenerateStubsResponse.getDefaultInstance()))
.setSchemaDescriptor(new LanguageServiceMethodDescriptorSupplier("GenerateStubs"))
.build();
}
}
}
return getGenerateStubsMethod;
}
private static volatile io.grpc.MethodDescriptor getSyncStubReferencesMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "SyncStubReferences",
requestType = xyz.block.ftl.v1.language.SyncStubReferencesRequest.class,
responseType = xyz.block.ftl.v1.language.SyncStubReferencesResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getSyncStubReferencesMethod() {
io.grpc.MethodDescriptor getSyncStubReferencesMethod;
if ((getSyncStubReferencesMethod = LanguageServiceGrpc.getSyncStubReferencesMethod) == null) {
synchronized (LanguageServiceGrpc.class) {
if ((getSyncStubReferencesMethod = LanguageServiceGrpc.getSyncStubReferencesMethod) == null) {
LanguageServiceGrpc.getSyncStubReferencesMethod = getSyncStubReferencesMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "SyncStubReferences"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xyz.block.ftl.v1.language.SyncStubReferencesRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xyz.block.ftl.v1.language.SyncStubReferencesResponse.getDefaultInstance()))
.setSchemaDescriptor(new LanguageServiceMethodDescriptorSupplier("SyncStubReferences"))
.build();
}
}
}
return getSyncStubReferencesMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static LanguageServiceStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public LanguageServiceStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new LanguageServiceStub(channel, callOptions);
}
};
return LanguageServiceStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static LanguageServiceBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public LanguageServiceBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new LanguageServiceBlockingStub(channel, callOptions);
}
};
return LanguageServiceBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static LanguageServiceFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public LanguageServiceFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new LanguageServiceFutureStub(channel, callOptions);
}
};
return LanguageServiceFutureStub.newStub(factory, channel);
}
/**
*
* LanguageService allows a plugin to add support for a programming language.
*
*/
public interface AsyncService {
/**
*
* Ping service for readiness.
*
*/
default void ping(xyz.block.ftl.v1.PingRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getPingMethod(), responseObserver);
}
/**
*
* Get language specific flags that can be used to create a new module.
*
*/
default void getCreateModuleFlags(xyz.block.ftl.v1.language.GetCreateModuleFlagsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetCreateModuleFlagsMethod(), responseObserver);
}
/**
*
* Generates files for a new module with the requested name
*
*/
default void createModule(xyz.block.ftl.v1.language.CreateModuleRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getCreateModuleMethod(), responseObserver);
}
/**
*
* Provide default values for ModuleConfig for values that are not configured in the ftl.toml file.
*
*/
default void moduleConfigDefaults(xyz.block.ftl.v1.language.ModuleConfigDefaultsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getModuleConfigDefaultsMethod(), responseObserver);
}
/**
*
* Extract dependencies for a module
* FTL will ensure that these dependencies are built before requesting a build for this module.
*
*/
default void getDependencies(xyz.block.ftl.v1.language.DependenciesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetDependenciesMethod(), responseObserver);
}
/**
*
* Build the module and stream back build events.
* A BuildSuccess or BuildFailure event must be streamed back with the request's context id to indicate the
* end of the build.
* The request can include the option to "rebuild_automatically". In this case the plugin should watch for
* file changes and automatically rebuild as needed as long as this build request is alive. Each automactic
* rebuild must include the latest build context id provided by the request or subsequent BuildContextUpdated
* calls.
*
*/
default void build(xyz.block.ftl.v1.language.BuildRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getBuildMethod(), responseObserver);
}
/**
*
* While a Build call with "rebuild_automatically" set is active, BuildContextUpdated is called whenever the
* build context is updated.
* Each time this call is made, the Build call must send back a corresponding BuildSuccess or BuildFailure
* event with the updated build context id with "is_automatic_rebuild" as false.
* If the plugin will not be able to return a BuildSuccess or BuildFailure, such as when there is no active
* build stream, it must fail the BuildContextUpdated call.
*
*/
default void buildContextUpdated(xyz.block.ftl.v1.language.BuildContextUpdatedRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getBuildContextUpdatedMethod(), responseObserver);
}
/**
*
* Generate stubs for a module.
* Stubs allow modules to import other module's exported interface. If a language does not need this step,
* then it is not required to do anything in this call.
* This call is not tied to the module that this plugin is responsible for. A plugin of each language will
* be chosen to generate stubs for each module.
*
*/
default void generateStubs(xyz.block.ftl.v1.language.GenerateStubsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGenerateStubsMethod(), responseObserver);
}
/**
*
* SyncStubReferences is called when module stubs have been updated. This allows the plugin to update
* references to external modules, regardless of whether they are dependencies.
* For example, go plugin adds references to all modules into the go.work file so that tools can automatically
* import the modules when users start reference them.
* It is optional to do anything with this call.
*
*/
default void syncStubReferences(xyz.block.ftl.v1.language.SyncStubReferencesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getSyncStubReferencesMethod(), responseObserver);
}
}
/**
* Base class for the server implementation of the service LanguageService.
*
* LanguageService allows a plugin to add support for a programming language.
*
*/
public static abstract class LanguageServiceImplBase
implements io.grpc.BindableService, AsyncService {
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return LanguageServiceGrpc.bindService(this);
}
}
/**
* A stub to allow clients to do asynchronous rpc calls to service LanguageService.
*
* LanguageService allows a plugin to add support for a programming language.
*
*/
public static final class LanguageServiceStub
extends io.grpc.stub.AbstractAsyncStub {
private LanguageServiceStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected LanguageServiceStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new LanguageServiceStub(channel, callOptions);
}
/**
*
* Ping service for readiness.
*
*/
public void ping(xyz.block.ftl.v1.PingRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getPingMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Get language specific flags that can be used to create a new module.
*
*/
public void getCreateModuleFlags(xyz.block.ftl.v1.language.GetCreateModuleFlagsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetCreateModuleFlagsMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Generates files for a new module with the requested name
*
*/
public void createModule(xyz.block.ftl.v1.language.CreateModuleRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getCreateModuleMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Provide default values for ModuleConfig for values that are not configured in the ftl.toml file.
*
*/
public void moduleConfigDefaults(xyz.block.ftl.v1.language.ModuleConfigDefaultsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getModuleConfigDefaultsMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Extract dependencies for a module
* FTL will ensure that these dependencies are built before requesting a build for this module.
*
*/
public void getDependencies(xyz.block.ftl.v1.language.DependenciesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetDependenciesMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Build the module and stream back build events.
* A BuildSuccess or BuildFailure event must be streamed back with the request's context id to indicate the
* end of the build.
* The request can include the option to "rebuild_automatically". In this case the plugin should watch for
* file changes and automatically rebuild as needed as long as this build request is alive. Each automactic
* rebuild must include the latest build context id provided by the request or subsequent BuildContextUpdated
* calls.
*
*/
public void build(xyz.block.ftl.v1.language.BuildRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncServerStreamingCall(
getChannel().newCall(getBuildMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* While a Build call with "rebuild_automatically" set is active, BuildContextUpdated is called whenever the
* build context is updated.
* Each time this call is made, the Build call must send back a corresponding BuildSuccess or BuildFailure
* event with the updated build context id with "is_automatic_rebuild" as false.
* If the plugin will not be able to return a BuildSuccess or BuildFailure, such as when there is no active
* build stream, it must fail the BuildContextUpdated call.
*
*/
public void buildContextUpdated(xyz.block.ftl.v1.language.BuildContextUpdatedRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getBuildContextUpdatedMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Generate stubs for a module.
* Stubs allow modules to import other module's exported interface. If a language does not need this step,
* then it is not required to do anything in this call.
* This call is not tied to the module that this plugin is responsible for. A plugin of each language will
* be chosen to generate stubs for each module.
*
*/
public void generateStubs(xyz.block.ftl.v1.language.GenerateStubsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGenerateStubsMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* SyncStubReferences is called when module stubs have been updated. This allows the plugin to update
* references to external modules, regardless of whether they are dependencies.
* For example, go plugin adds references to all modules into the go.work file so that tools can automatically
* import the modules when users start reference them.
* It is optional to do anything with this call.
*
*/
public void syncStubReferences(xyz.block.ftl.v1.language.SyncStubReferencesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getSyncStubReferencesMethod(), getCallOptions()), request, responseObserver);
}
}
/**
* A stub to allow clients to do synchronous rpc calls to service LanguageService.
*
* LanguageService allows a plugin to add support for a programming language.
*
*/
public static final class LanguageServiceBlockingStub
extends io.grpc.stub.AbstractBlockingStub {
private LanguageServiceBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected LanguageServiceBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new LanguageServiceBlockingStub(channel, callOptions);
}
/**
*
* Ping service for readiness.
*
*/
public xyz.block.ftl.v1.PingResponse ping(xyz.block.ftl.v1.PingRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getPingMethod(), getCallOptions(), request);
}
/**
*
* Get language specific flags that can be used to create a new module.
*
*/
public xyz.block.ftl.v1.language.GetCreateModuleFlagsResponse getCreateModuleFlags(xyz.block.ftl.v1.language.GetCreateModuleFlagsRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetCreateModuleFlagsMethod(), getCallOptions(), request);
}
/**
*
* Generates files for a new module with the requested name
*
*/
public xyz.block.ftl.v1.language.CreateModuleResponse createModule(xyz.block.ftl.v1.language.CreateModuleRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getCreateModuleMethod(), getCallOptions(), request);
}
/**
*
* Provide default values for ModuleConfig for values that are not configured in the ftl.toml file.
*
*/
public xyz.block.ftl.v1.language.ModuleConfigDefaultsResponse moduleConfigDefaults(xyz.block.ftl.v1.language.ModuleConfigDefaultsRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getModuleConfigDefaultsMethod(), getCallOptions(), request);
}
/**
*
* Extract dependencies for a module
* FTL will ensure that these dependencies are built before requesting a build for this module.
*
*/
public xyz.block.ftl.v1.language.DependenciesResponse getDependencies(xyz.block.ftl.v1.language.DependenciesRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetDependenciesMethod(), getCallOptions(), request);
}
/**
*
* Build the module and stream back build events.
* A BuildSuccess or BuildFailure event must be streamed back with the request's context id to indicate the
* end of the build.
* The request can include the option to "rebuild_automatically". In this case the plugin should watch for
* file changes and automatically rebuild as needed as long as this build request is alive. Each automactic
* rebuild must include the latest build context id provided by the request or subsequent BuildContextUpdated
* calls.
*
*/
public java.util.Iterator build(
xyz.block.ftl.v1.language.BuildRequest request) {
return io.grpc.stub.ClientCalls.blockingServerStreamingCall(
getChannel(), getBuildMethod(), getCallOptions(), request);
}
/**
*
* While a Build call with "rebuild_automatically" set is active, BuildContextUpdated is called whenever the
* build context is updated.
* Each time this call is made, the Build call must send back a corresponding BuildSuccess or BuildFailure
* event with the updated build context id with "is_automatic_rebuild" as false.
* If the plugin will not be able to return a BuildSuccess or BuildFailure, such as when there is no active
* build stream, it must fail the BuildContextUpdated call.
*
*/
public xyz.block.ftl.v1.language.BuildContextUpdatedResponse buildContextUpdated(xyz.block.ftl.v1.language.BuildContextUpdatedRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getBuildContextUpdatedMethod(), getCallOptions(), request);
}
/**
*
* Generate stubs for a module.
* Stubs allow modules to import other module's exported interface. If a language does not need this step,
* then it is not required to do anything in this call.
* This call is not tied to the module that this plugin is responsible for. A plugin of each language will
* be chosen to generate stubs for each module.
*
*/
public xyz.block.ftl.v1.language.GenerateStubsResponse generateStubs(xyz.block.ftl.v1.language.GenerateStubsRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGenerateStubsMethod(), getCallOptions(), request);
}
/**
*
* SyncStubReferences is called when module stubs have been updated. This allows the plugin to update
* references to external modules, regardless of whether they are dependencies.
* For example, go plugin adds references to all modules into the go.work file so that tools can automatically
* import the modules when users start reference them.
* It is optional to do anything with this call.
*
*/
public xyz.block.ftl.v1.language.SyncStubReferencesResponse syncStubReferences(xyz.block.ftl.v1.language.SyncStubReferencesRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getSyncStubReferencesMethod(), getCallOptions(), request);
}
}
/**
* A stub to allow clients to do ListenableFuture-style rpc calls to service LanguageService.
*
* LanguageService allows a plugin to add support for a programming language.
*
*/
public static final class LanguageServiceFutureStub
extends io.grpc.stub.AbstractFutureStub {
private LanguageServiceFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected LanguageServiceFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new LanguageServiceFutureStub(channel, callOptions);
}
/**
*
* Ping service for readiness.
*
*/
public com.google.common.util.concurrent.ListenableFuture ping(
xyz.block.ftl.v1.PingRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getPingMethod(), getCallOptions()), request);
}
/**
*
* Get language specific flags that can be used to create a new module.
*
*/
public com.google.common.util.concurrent.ListenableFuture getCreateModuleFlags(
xyz.block.ftl.v1.language.GetCreateModuleFlagsRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetCreateModuleFlagsMethod(), getCallOptions()), request);
}
/**
*
* Generates files for a new module with the requested name
*
*/
public com.google.common.util.concurrent.ListenableFuture createModule(
xyz.block.ftl.v1.language.CreateModuleRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getCreateModuleMethod(), getCallOptions()), request);
}
/**
*
* Provide default values for ModuleConfig for values that are not configured in the ftl.toml file.
*
*/
public com.google.common.util.concurrent.ListenableFuture moduleConfigDefaults(
xyz.block.ftl.v1.language.ModuleConfigDefaultsRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getModuleConfigDefaultsMethod(), getCallOptions()), request);
}
/**
*
* Extract dependencies for a module
* FTL will ensure that these dependencies are built before requesting a build for this module.
*
*/
public com.google.common.util.concurrent.ListenableFuture getDependencies(
xyz.block.ftl.v1.language.DependenciesRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetDependenciesMethod(), getCallOptions()), request);
}
/**
*
* While a Build call with "rebuild_automatically" set is active, BuildContextUpdated is called whenever the
* build context is updated.
* Each time this call is made, the Build call must send back a corresponding BuildSuccess or BuildFailure
* event with the updated build context id with "is_automatic_rebuild" as false.
* If the plugin will not be able to return a BuildSuccess or BuildFailure, such as when there is no active
* build stream, it must fail the BuildContextUpdated call.
*
*/
public com.google.common.util.concurrent.ListenableFuture buildContextUpdated(
xyz.block.ftl.v1.language.BuildContextUpdatedRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getBuildContextUpdatedMethod(), getCallOptions()), request);
}
/**
*
* Generate stubs for a module.
* Stubs allow modules to import other module's exported interface. If a language does not need this step,
* then it is not required to do anything in this call.
* This call is not tied to the module that this plugin is responsible for. A plugin of each language will
* be chosen to generate stubs for each module.
*
*/
public com.google.common.util.concurrent.ListenableFuture generateStubs(
xyz.block.ftl.v1.language.GenerateStubsRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGenerateStubsMethod(), getCallOptions()), request);
}
/**
*
* SyncStubReferences is called when module stubs have been updated. This allows the plugin to update
* references to external modules, regardless of whether they are dependencies.
* For example, go plugin adds references to all modules into the go.work file so that tools can automatically
* import the modules when users start reference them.
* It is optional to do anything with this call.
*
*/
public com.google.common.util.concurrent.ListenableFuture syncStubReferences(
xyz.block.ftl.v1.language.SyncStubReferencesRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getSyncStubReferencesMethod(), getCallOptions()), request);
}
}
private static final int METHODID_PING = 0;
private static final int METHODID_GET_CREATE_MODULE_FLAGS = 1;
private static final int METHODID_CREATE_MODULE = 2;
private static final int METHODID_MODULE_CONFIG_DEFAULTS = 3;
private static final int METHODID_GET_DEPENDENCIES = 4;
private static final int METHODID_BUILD = 5;
private static final int METHODID_BUILD_CONTEXT_UPDATED = 6;
private static final int METHODID_GENERATE_STUBS = 7;
private static final int METHODID_SYNC_STUB_REFERENCES = 8;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final AsyncService serviceImpl;
private final int methodId;
MethodHandlers(AsyncService serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_PING:
serviceImpl.ping((xyz.block.ftl.v1.PingRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_CREATE_MODULE_FLAGS:
serviceImpl.getCreateModuleFlags((xyz.block.ftl.v1.language.GetCreateModuleFlagsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_CREATE_MODULE:
serviceImpl.createModule((xyz.block.ftl.v1.language.CreateModuleRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_MODULE_CONFIG_DEFAULTS:
serviceImpl.moduleConfigDefaults((xyz.block.ftl.v1.language.ModuleConfigDefaultsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_DEPENDENCIES:
serviceImpl.getDependencies((xyz.block.ftl.v1.language.DependenciesRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_BUILD:
serviceImpl.build((xyz.block.ftl.v1.language.BuildRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_BUILD_CONTEXT_UPDATED:
serviceImpl.buildContextUpdated((xyz.block.ftl.v1.language.BuildContextUpdatedRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GENERATE_STUBS:
serviceImpl.generateStubs((xyz.block.ftl.v1.language.GenerateStubsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_SYNC_STUB_REFERENCES:
serviceImpl.syncStubReferences((xyz.block.ftl.v1.language.SyncStubReferencesRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
public static final io.grpc.ServerServiceDefinition bindService(AsyncService service) {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getPingMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
xyz.block.ftl.v1.PingRequest,
xyz.block.ftl.v1.PingResponse>(
service, METHODID_PING)))
.addMethod(
getGetCreateModuleFlagsMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
xyz.block.ftl.v1.language.GetCreateModuleFlagsRequest,
xyz.block.ftl.v1.language.GetCreateModuleFlagsResponse>(
service, METHODID_GET_CREATE_MODULE_FLAGS)))
.addMethod(
getCreateModuleMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
xyz.block.ftl.v1.language.CreateModuleRequest,
xyz.block.ftl.v1.language.CreateModuleResponse>(
service, METHODID_CREATE_MODULE)))
.addMethod(
getModuleConfigDefaultsMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
xyz.block.ftl.v1.language.ModuleConfigDefaultsRequest,
xyz.block.ftl.v1.language.ModuleConfigDefaultsResponse>(
service, METHODID_MODULE_CONFIG_DEFAULTS)))
.addMethod(
getGetDependenciesMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
xyz.block.ftl.v1.language.DependenciesRequest,
xyz.block.ftl.v1.language.DependenciesResponse>(
service, METHODID_GET_DEPENDENCIES)))
.addMethod(
getBuildMethod(),
io.grpc.stub.ServerCalls.asyncServerStreamingCall(
new MethodHandlers<
xyz.block.ftl.v1.language.BuildRequest,
xyz.block.ftl.v1.language.BuildEvent>(
service, METHODID_BUILD)))
.addMethod(
getBuildContextUpdatedMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
xyz.block.ftl.v1.language.BuildContextUpdatedRequest,
xyz.block.ftl.v1.language.BuildContextUpdatedResponse>(
service, METHODID_BUILD_CONTEXT_UPDATED)))
.addMethod(
getGenerateStubsMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
xyz.block.ftl.v1.language.GenerateStubsRequest,
xyz.block.ftl.v1.language.GenerateStubsResponse>(
service, METHODID_GENERATE_STUBS)))
.addMethod(
getSyncStubReferencesMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
xyz.block.ftl.v1.language.SyncStubReferencesRequest,
xyz.block.ftl.v1.language.SyncStubReferencesResponse>(
service, METHODID_SYNC_STUB_REFERENCES)))
.build();
}
private static abstract class LanguageServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
LanguageServiceBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return xyz.block.ftl.v1.language.Language.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("LanguageService");
}
}
private static final class LanguageServiceFileDescriptorSupplier
extends LanguageServiceBaseDescriptorSupplier {
LanguageServiceFileDescriptorSupplier() {}
}
private static final class LanguageServiceMethodDescriptorSupplier
extends LanguageServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final java.lang.String methodName;
LanguageServiceMethodDescriptorSupplier(java.lang.String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (LanguageServiceGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new LanguageServiceFileDescriptorSupplier())
.addMethod(getPingMethod())
.addMethod(getGetCreateModuleFlagsMethod())
.addMethod(getCreateModuleMethod())
.addMethod(getModuleConfigDefaultsMethod())
.addMethod(getGetDependenciesMethod())
.addMethod(getBuildMethod())
.addMethod(getBuildContextUpdatedMethod())
.addMethod(getGenerateStubsMethod())
.addMethod(getSyncStubReferencesMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy