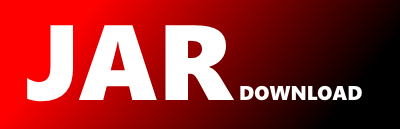
xyz.block.ftl.v1.CreateDeploymentRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ftl-runtime Show documentation
Show all versions of ftl-runtime Show documentation
Towards a 𝝺-calculus for large-scale systems
// Code generated by Wire protocol buffer compiler, do not edit.
// Source: xyz.block.ftl.v1.CreateDeploymentRequest in xyz/block/ftl/v1/ftl.proto
@file:Suppress("DEPRECATION")
package xyz.block.ftl.v1
import com.squareup.wire.FieldEncoding
import com.squareup.wire.Message
import com.squareup.wire.ProtoAdapter
import com.squareup.wire.ProtoReader
import com.squareup.wire.ProtoWriter
import com.squareup.wire.ReverseProtoWriter
import com.squareup.wire.Syntax.PROTO_3
import com.squareup.wire.WireField
import com.squareup.wire.`internal`.JvmField
import com.squareup.wire.`internal`.immutableCopyOf
import com.squareup.wire.`internal`.immutableCopyOfStruct
import com.squareup.wire.`internal`.redactElements
import kotlin.Any
import kotlin.AssertionError
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.DeprecationLevel
import kotlin.Int
import kotlin.Long
import kotlin.Nothing
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
import okio.ByteString
import xyz.block.ftl.v1.schema.Module
public class CreateDeploymentRequest(
@field:WireField(
tag = 1,
adapter = "xyz.block.ftl.v1.schema.Module#ADAPTER",
label = WireField.Label.OMIT_IDENTITY,
schemaIndex = 0,
)
public val schema: Module? = null,
artefacts: List = emptyList(),
labels: Map? = null,
unknownFields: ByteString = ByteString.EMPTY,
) : Message(ADAPTER, unknownFields) {
@field:WireField(
tag = 2,
adapter = "xyz.block.ftl.v1.DeploymentArtefact#ADAPTER",
label = WireField.Label.REPEATED,
schemaIndex = 1,
)
public val artefacts: List = immutableCopyOf("artefacts", artefacts)
/**
* Runner labels required to run this deployment.
*/
@field:WireField(
tag = 3,
adapter = "com.squareup.wire.ProtoAdapter#STRUCT_MAP",
schemaIndex = 2,
)
public val labels: Map? = immutableCopyOfStruct("labels", labels)
@Deprecated(
message = "Shouldn't be used in Kotlin",
level = DeprecationLevel.HIDDEN,
)
override fun newBuilder(): Nothing = throw
AssertionError("Builders are deprecated and only available in a javaInterop build; see https://square.github.io/wire/wire_compiler/#kotlin")
override fun equals(other: Any?): Boolean {
if (other === this) return true
if (other !is CreateDeploymentRequest) return false
if (unknownFields != other.unknownFields) return false
if (schema != other.schema) return false
if (artefacts != other.artefacts) return false
if (labels != other.labels) return false
return true
}
override fun hashCode(): Int {
var result = super.hashCode
if (result == 0) {
result = unknownFields.hashCode()
result = result * 37 + (schema?.hashCode() ?: 0)
result = result * 37 + artefacts.hashCode()
result = result * 37 + (labels?.hashCode() ?: 0)
super.hashCode = result
}
return result
}
override fun toString(): String {
val result = mutableListOf()
if (schema != null) result += """schema=$schema"""
if (artefacts.isNotEmpty()) result += """artefacts=$artefacts"""
if (labels != null) result += """labels=$labels"""
return result.joinToString(prefix = "CreateDeploymentRequest{", separator = ", ", postfix = "}")
}
public fun copy(
schema: Module? = this.schema,
artefacts: List = this.artefacts,
labels: Map? = this.labels,
unknownFields: ByteString = this.unknownFields,
): CreateDeploymentRequest = CreateDeploymentRequest(schema, artefacts, labels, unknownFields)
public companion object {
@JvmField
public val ADAPTER: ProtoAdapter = object :
ProtoAdapter(
FieldEncoding.LENGTH_DELIMITED,
CreateDeploymentRequest::class,
"type.googleapis.com/xyz.block.ftl.v1.CreateDeploymentRequest",
PROTO_3,
null,
"xyz/block/ftl/v1/ftl.proto"
) {
override fun encodedSize(`value`: CreateDeploymentRequest): Int {
var size = value.unknownFields.size
if (value.schema != null) size += Module.ADAPTER.encodedSizeWithTag(1, value.schema)
size += DeploymentArtefact.ADAPTER.asRepeated().encodedSizeWithTag(2, value.artefacts)
size += ProtoAdapter.STRUCT_MAP.encodedSizeWithTag(3, value.labels)
return size
}
override fun encode(writer: ProtoWriter, `value`: CreateDeploymentRequest) {
if (value.schema != null) Module.ADAPTER.encodeWithTag(writer, 1, value.schema)
DeploymentArtefact.ADAPTER.asRepeated().encodeWithTag(writer, 2, value.artefacts)
ProtoAdapter.STRUCT_MAP.encodeWithTag(writer, 3, value.labels)
writer.writeBytes(value.unknownFields)
}
override fun encode(writer: ReverseProtoWriter, `value`: CreateDeploymentRequest) {
writer.writeBytes(value.unknownFields)
ProtoAdapter.STRUCT_MAP.encodeWithTag(writer, 3, value.labels)
DeploymentArtefact.ADAPTER.asRepeated().encodeWithTag(writer, 2, value.artefacts)
if (value.schema != null) Module.ADAPTER.encodeWithTag(writer, 1, value.schema)
}
override fun decode(reader: ProtoReader): CreateDeploymentRequest {
var schema: Module? = null
val artefacts = mutableListOf()
var labels: Map? = null
val unknownFields = reader.forEachTag { tag ->
when (tag) {
1 -> schema = Module.ADAPTER.decode(reader)
2 -> artefacts.add(DeploymentArtefact.ADAPTER.decode(reader))
3 -> labels = ProtoAdapter.STRUCT_MAP.decode(reader)
else -> reader.readUnknownField(tag)
}
}
return CreateDeploymentRequest(
schema = schema,
artefacts = artefacts,
labels = labels,
unknownFields = unknownFields
)
}
override fun redact(`value`: CreateDeploymentRequest): CreateDeploymentRequest = value.copy(
schema = value.schema?.let(Module.ADAPTER::redact),
artefacts = value.artefacts.redactElements(DeploymentArtefact.ADAPTER),
labels = value.labels?.let(ProtoAdapter.STRUCT_MAP::redact),
unknownFields = ByteString.EMPTY
)
}
private const val serialVersionUID: Long = 0L
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy