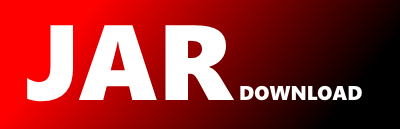
xyz.capybara.clamav.commands.scan.ScanCommand.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clamav-client Show documentation
Show all versions of clamav-client Show documentation
Java Client for the ClamAV antivirus service.
package xyz.capybara.clamav.commands.scan
import xyz.capybara.clamav.commands.scan.result.ScanResult
import xyz.capybara.clamav.commands.Command
import xyz.capybara.clamav.InvalidResponseException
import xyz.capybara.clamav.ScanFailureException
abstract class ScanCommand : Command() {
private val RESPONSE_OK = Regex(
"(.+) OK$",
RegexOption.UNIX_LINES
)
private val RESPONSE_VIRUS_FOUND = Regex(
"(.+) FOUND$",
setOf(RegexOption.MULTILINE, RegexOption.UNIX_LINES)
)
private val RESPONSE_ERROR = Regex(
"(.+) ERROR",
RegexOption.UNIX_LINES
)
private val RESPONSE_VIRUS_FOUND_LINE = Regex(
"(.+: )?(.+): (.+) FOUND$",
RegexOption.UNIX_LINES
)
override fun parseResponse(responseString: String): ScanResult {
try {
return when {
RESPONSE_OK matches responseString -> ScanResult(ScanResult.Status.OK)
RESPONSE_VIRUS_FOUND.containsMatchIn(responseString) -> {
// add every found viruses to the scan result, grouped by infected file
val foundViruses = responseString.split("\n".toRegex())
.mapNotNull(RESPONSE_VIRUS_FOUND_LINE::matchEntire)
.map { it.groups.reversed() }
// key: file path
// value: virus name
.groupBy({ it[1]!!.value }, { it[0]!!.value })
ScanResult(ScanResult.Status.VIRUS_FOUND, foundViruses)
}
RESPONSE_ERROR matches responseString -> throw ScanFailureException(responseString)
else -> throw InvalidResponseException(responseString)
}
} catch (e: IllegalStateException) {
throw InvalidResponseException(responseString)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy