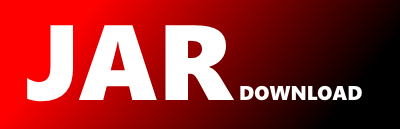
org.tensorflow.framework.NodeExecStats Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: tensorflow/core/framework/step_stats.proto
package org.tensorflow.framework;
/**
*
* Time/size stats recorded for a single execution of a graph node.
*
*
* Protobuf type {@code tensorflow.NodeExecStats}
*/
public final class NodeExecStats extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.NodeExecStats)
NodeExecStatsOrBuilder {
private static final long serialVersionUID = 0L;
// Use NodeExecStats.newBuilder() to construct.
private NodeExecStats(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private NodeExecStats() {
nodeName_ = "";
allStartMicros_ = 0L;
opStartRelMicros_ = 0L;
opEndRelMicros_ = 0L;
allEndRelMicros_ = 0L;
memory_ = java.util.Collections.emptyList();
output_ = java.util.Collections.emptyList();
timelineLabel_ = "";
scheduledMicros_ = 0L;
threadId_ = 0;
referencedTensor_ = java.util.Collections.emptyList();
allStartNanos_ = 0L;
opStartRelNanos_ = 0L;
opEndRelNanos_ = 0L;
allEndRelNanos_ = 0L;
scheduledNanos_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private NodeExecStats(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
nodeName_ = s;
break;
}
case 16: {
allStartMicros_ = input.readInt64();
break;
}
case 24: {
opStartRelMicros_ = input.readInt64();
break;
}
case 32: {
opEndRelMicros_ = input.readInt64();
break;
}
case 40: {
allEndRelMicros_ = input.readInt64();
break;
}
case 50: {
if (!((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
memory_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000020;
}
memory_.add(
input.readMessage(org.tensorflow.framework.AllocatorMemoryUsed.parser(), extensionRegistry));
break;
}
case 58: {
if (!((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
output_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
output_.add(
input.readMessage(org.tensorflow.framework.NodeOutput.parser(), extensionRegistry));
break;
}
case 66: {
java.lang.String s = input.readStringRequireUtf8();
timelineLabel_ = s;
break;
}
case 72: {
scheduledMicros_ = input.readInt64();
break;
}
case 80: {
threadId_ = input.readUInt32();
break;
}
case 90: {
if (!((mutable_bitField0_ & 0x00000400) == 0x00000400)) {
referencedTensor_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000400;
}
referencedTensor_.add(
input.readMessage(org.tensorflow.framework.AllocationDescription.parser(), extensionRegistry));
break;
}
case 98: {
org.tensorflow.framework.MemoryStats.Builder subBuilder = null;
if (memoryStats_ != null) {
subBuilder = memoryStats_.toBuilder();
}
memoryStats_ = input.readMessage(org.tensorflow.framework.MemoryStats.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(memoryStats_);
memoryStats_ = subBuilder.buildPartial();
}
break;
}
case 104: {
allStartNanos_ = input.readInt64();
break;
}
case 112: {
opStartRelNanos_ = input.readInt64();
break;
}
case 120: {
opEndRelNanos_ = input.readInt64();
break;
}
case 128: {
allEndRelNanos_ = input.readInt64();
break;
}
case 136: {
scheduledNanos_ = input.readInt64();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
memory_ = java.util.Collections.unmodifiableList(memory_);
}
if (((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
output_ = java.util.Collections.unmodifiableList(output_);
}
if (((mutable_bitField0_ & 0x00000400) == 0x00000400)) {
referencedTensor_ = java.util.Collections.unmodifiableList(referencedTensor_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.framework.StepStatsProtos.internal_static_tensorflow_NodeExecStats_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.framework.StepStatsProtos.internal_static_tensorflow_NodeExecStats_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.framework.NodeExecStats.class, org.tensorflow.framework.NodeExecStats.Builder.class);
}
private int bitField0_;
public static final int NODE_NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object nodeName_;
/**
*
* TODO(tucker): Use some more compact form of node identity than
* the full string name. Either all processes should agree on a
* global id (cost_id?) for each node, or we should use a hash of
* the name.
*
*
* string node_name = 1;
*/
public java.lang.String getNodeName() {
java.lang.Object ref = nodeName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
nodeName_ = s;
return s;
}
}
/**
*
* TODO(tucker): Use some more compact form of node identity than
* the full string name. Either all processes should agree on a
* global id (cost_id?) for each node, or we should use a hash of
* the name.
*
*
* string node_name = 1;
*/
public com.google.protobuf.ByteString
getNodeNameBytes() {
java.lang.Object ref = nodeName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nodeName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ALL_START_MICROS_FIELD_NUMBER = 2;
private long allStartMicros_;
/**
* int64 all_start_micros = 2;
*/
public long getAllStartMicros() {
return allStartMicros_;
}
public static final int OP_START_REL_MICROS_FIELD_NUMBER = 3;
private long opStartRelMicros_;
/**
* int64 op_start_rel_micros = 3;
*/
public long getOpStartRelMicros() {
return opStartRelMicros_;
}
public static final int OP_END_REL_MICROS_FIELD_NUMBER = 4;
private long opEndRelMicros_;
/**
* int64 op_end_rel_micros = 4;
*/
public long getOpEndRelMicros() {
return opEndRelMicros_;
}
public static final int ALL_END_REL_MICROS_FIELD_NUMBER = 5;
private long allEndRelMicros_;
/**
* int64 all_end_rel_micros = 5;
*/
public long getAllEndRelMicros() {
return allEndRelMicros_;
}
public static final int MEMORY_FIELD_NUMBER = 6;
private java.util.List memory_;
/**
* repeated .tensorflow.AllocatorMemoryUsed memory = 6;
*/
public java.util.List getMemoryList() {
return memory_;
}
/**
* repeated .tensorflow.AllocatorMemoryUsed memory = 6;
*/
public java.util.List extends org.tensorflow.framework.AllocatorMemoryUsedOrBuilder>
getMemoryOrBuilderList() {
return memory_;
}
/**
* repeated .tensorflow.AllocatorMemoryUsed memory = 6;
*/
public int getMemoryCount() {
return memory_.size();
}
/**
* repeated .tensorflow.AllocatorMemoryUsed memory = 6;
*/
public org.tensorflow.framework.AllocatorMemoryUsed getMemory(int index) {
return memory_.get(index);
}
/**
* repeated .tensorflow.AllocatorMemoryUsed memory = 6;
*/
public org.tensorflow.framework.AllocatorMemoryUsedOrBuilder getMemoryOrBuilder(
int index) {
return memory_.get(index);
}
public static final int OUTPUT_FIELD_NUMBER = 7;
private java.util.List output_;
/**
* repeated .tensorflow.NodeOutput output = 7;
*/
public java.util.List getOutputList() {
return output_;
}
/**
* repeated .tensorflow.NodeOutput output = 7;
*/
public java.util.List extends org.tensorflow.framework.NodeOutputOrBuilder>
getOutputOrBuilderList() {
return output_;
}
/**
* repeated .tensorflow.NodeOutput output = 7;
*/
public int getOutputCount() {
return output_.size();
}
/**
* repeated .tensorflow.NodeOutput output = 7;
*/
public org.tensorflow.framework.NodeOutput getOutput(int index) {
return output_.get(index);
}
/**
* repeated .tensorflow.NodeOutput output = 7;
*/
public org.tensorflow.framework.NodeOutputOrBuilder getOutputOrBuilder(
int index) {
return output_.get(index);
}
public static final int TIMELINE_LABEL_FIELD_NUMBER = 8;
private volatile java.lang.Object timelineLabel_;
/**
* string timeline_label = 8;
*/
public java.lang.String getTimelineLabel() {
java.lang.Object ref = timelineLabel_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
timelineLabel_ = s;
return s;
}
}
/**
* string timeline_label = 8;
*/
public com.google.protobuf.ByteString
getTimelineLabelBytes() {
java.lang.Object ref = timelineLabel_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
timelineLabel_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SCHEDULED_MICROS_FIELD_NUMBER = 9;
private long scheduledMicros_;
/**
* int64 scheduled_micros = 9;
*/
public long getScheduledMicros() {
return scheduledMicros_;
}
public static final int THREAD_ID_FIELD_NUMBER = 10;
private int threadId_;
/**
* uint32 thread_id = 10;
*/
public int getThreadId() {
return threadId_;
}
public static final int REFERENCED_TENSOR_FIELD_NUMBER = 11;
private java.util.List referencedTensor_;
/**
* repeated .tensorflow.AllocationDescription referenced_tensor = 11;
*/
public java.util.List getReferencedTensorList() {
return referencedTensor_;
}
/**
* repeated .tensorflow.AllocationDescription referenced_tensor = 11;
*/
public java.util.List extends org.tensorflow.framework.AllocationDescriptionOrBuilder>
getReferencedTensorOrBuilderList() {
return referencedTensor_;
}
/**
* repeated .tensorflow.AllocationDescription referenced_tensor = 11;
*/
public int getReferencedTensorCount() {
return referencedTensor_.size();
}
/**
* repeated .tensorflow.AllocationDescription referenced_tensor = 11;
*/
public org.tensorflow.framework.AllocationDescription getReferencedTensor(int index) {
return referencedTensor_.get(index);
}
/**
* repeated .tensorflow.AllocationDescription referenced_tensor = 11;
*/
public org.tensorflow.framework.AllocationDescriptionOrBuilder getReferencedTensorOrBuilder(
int index) {
return referencedTensor_.get(index);
}
public static final int MEMORY_STATS_FIELD_NUMBER = 12;
private org.tensorflow.framework.MemoryStats memoryStats_;
/**
* .tensorflow.MemoryStats memory_stats = 12;
*/
public boolean hasMemoryStats() {
return memoryStats_ != null;
}
/**
* .tensorflow.MemoryStats memory_stats = 12;
*/
public org.tensorflow.framework.MemoryStats getMemoryStats() {
return memoryStats_ == null ? org.tensorflow.framework.MemoryStats.getDefaultInstance() : memoryStats_;
}
/**
* .tensorflow.MemoryStats memory_stats = 12;
*/
public org.tensorflow.framework.MemoryStatsOrBuilder getMemoryStatsOrBuilder() {
return getMemoryStats();
}
public static final int ALL_START_NANOS_FIELD_NUMBER = 13;
private long allStartNanos_;
/**
* int64 all_start_nanos = 13;
*/
public long getAllStartNanos() {
return allStartNanos_;
}
public static final int OP_START_REL_NANOS_FIELD_NUMBER = 14;
private long opStartRelNanos_;
/**
* int64 op_start_rel_nanos = 14;
*/
public long getOpStartRelNanos() {
return opStartRelNanos_;
}
public static final int OP_END_REL_NANOS_FIELD_NUMBER = 15;
private long opEndRelNanos_;
/**
* int64 op_end_rel_nanos = 15;
*/
public long getOpEndRelNanos() {
return opEndRelNanos_;
}
public static final int ALL_END_REL_NANOS_FIELD_NUMBER = 16;
private long allEndRelNanos_;
/**
* int64 all_end_rel_nanos = 16;
*/
public long getAllEndRelNanos() {
return allEndRelNanos_;
}
public static final int SCHEDULED_NANOS_FIELD_NUMBER = 17;
private long scheduledNanos_;
/**
* int64 scheduled_nanos = 17;
*/
public long getScheduledNanos() {
return scheduledNanos_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getNodeNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, nodeName_);
}
if (allStartMicros_ != 0L) {
output.writeInt64(2, allStartMicros_);
}
if (opStartRelMicros_ != 0L) {
output.writeInt64(3, opStartRelMicros_);
}
if (opEndRelMicros_ != 0L) {
output.writeInt64(4, opEndRelMicros_);
}
if (allEndRelMicros_ != 0L) {
output.writeInt64(5, allEndRelMicros_);
}
for (int i = 0; i < memory_.size(); i++) {
output.writeMessage(6, memory_.get(i));
}
for (int i = 0; i < output_.size(); i++) {
output.writeMessage(7, output_.get(i));
}
if (!getTimelineLabelBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, timelineLabel_);
}
if (scheduledMicros_ != 0L) {
output.writeInt64(9, scheduledMicros_);
}
if (threadId_ != 0) {
output.writeUInt32(10, threadId_);
}
for (int i = 0; i < referencedTensor_.size(); i++) {
output.writeMessage(11, referencedTensor_.get(i));
}
if (memoryStats_ != null) {
output.writeMessage(12, getMemoryStats());
}
if (allStartNanos_ != 0L) {
output.writeInt64(13, allStartNanos_);
}
if (opStartRelNanos_ != 0L) {
output.writeInt64(14, opStartRelNanos_);
}
if (opEndRelNanos_ != 0L) {
output.writeInt64(15, opEndRelNanos_);
}
if (allEndRelNanos_ != 0L) {
output.writeInt64(16, allEndRelNanos_);
}
if (scheduledNanos_ != 0L) {
output.writeInt64(17, scheduledNanos_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getNodeNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, nodeName_);
}
if (allStartMicros_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, allStartMicros_);
}
if (opStartRelMicros_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, opStartRelMicros_);
}
if (opEndRelMicros_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, opEndRelMicros_);
}
if (allEndRelMicros_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(5, allEndRelMicros_);
}
for (int i = 0; i < memory_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, memory_.get(i));
}
for (int i = 0; i < output_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, output_.get(i));
}
if (!getTimelineLabelBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(8, timelineLabel_);
}
if (scheduledMicros_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(9, scheduledMicros_);
}
if (threadId_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(10, threadId_);
}
for (int i = 0; i < referencedTensor_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, referencedTensor_.get(i));
}
if (memoryStats_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, getMemoryStats());
}
if (allStartNanos_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(13, allStartNanos_);
}
if (opStartRelNanos_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(14, opStartRelNanos_);
}
if (opEndRelNanos_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(15, opEndRelNanos_);
}
if (allEndRelNanos_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(16, allEndRelNanos_);
}
if (scheduledNanos_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(17, scheduledNanos_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tensorflow.framework.NodeExecStats)) {
return super.equals(obj);
}
org.tensorflow.framework.NodeExecStats other = (org.tensorflow.framework.NodeExecStats) obj;
boolean result = true;
result = result && getNodeName()
.equals(other.getNodeName());
result = result && (getAllStartMicros()
== other.getAllStartMicros());
result = result && (getOpStartRelMicros()
== other.getOpStartRelMicros());
result = result && (getOpEndRelMicros()
== other.getOpEndRelMicros());
result = result && (getAllEndRelMicros()
== other.getAllEndRelMicros());
result = result && getMemoryList()
.equals(other.getMemoryList());
result = result && getOutputList()
.equals(other.getOutputList());
result = result && getTimelineLabel()
.equals(other.getTimelineLabel());
result = result && (getScheduledMicros()
== other.getScheduledMicros());
result = result && (getThreadId()
== other.getThreadId());
result = result && getReferencedTensorList()
.equals(other.getReferencedTensorList());
result = result && (hasMemoryStats() == other.hasMemoryStats());
if (hasMemoryStats()) {
result = result && getMemoryStats()
.equals(other.getMemoryStats());
}
result = result && (getAllStartNanos()
== other.getAllStartNanos());
result = result && (getOpStartRelNanos()
== other.getOpStartRelNanos());
result = result && (getOpEndRelNanos()
== other.getOpEndRelNanos());
result = result && (getAllEndRelNanos()
== other.getAllEndRelNanos());
result = result && (getScheduledNanos()
== other.getScheduledNanos());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NODE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getNodeName().hashCode();
hash = (37 * hash) + ALL_START_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getAllStartMicros());
hash = (37 * hash) + OP_START_REL_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOpStartRelMicros());
hash = (37 * hash) + OP_END_REL_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOpEndRelMicros());
hash = (37 * hash) + ALL_END_REL_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getAllEndRelMicros());
if (getMemoryCount() > 0) {
hash = (37 * hash) + MEMORY_FIELD_NUMBER;
hash = (53 * hash) + getMemoryList().hashCode();
}
if (getOutputCount() > 0) {
hash = (37 * hash) + OUTPUT_FIELD_NUMBER;
hash = (53 * hash) + getOutputList().hashCode();
}
hash = (37 * hash) + TIMELINE_LABEL_FIELD_NUMBER;
hash = (53 * hash) + getTimelineLabel().hashCode();
hash = (37 * hash) + SCHEDULED_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getScheduledMicros());
hash = (37 * hash) + THREAD_ID_FIELD_NUMBER;
hash = (53 * hash) + getThreadId();
if (getReferencedTensorCount() > 0) {
hash = (37 * hash) + REFERENCED_TENSOR_FIELD_NUMBER;
hash = (53 * hash) + getReferencedTensorList().hashCode();
}
if (hasMemoryStats()) {
hash = (37 * hash) + MEMORY_STATS_FIELD_NUMBER;
hash = (53 * hash) + getMemoryStats().hashCode();
}
hash = (37 * hash) + ALL_START_NANOS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getAllStartNanos());
hash = (37 * hash) + OP_START_REL_NANOS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOpStartRelNanos());
hash = (37 * hash) + OP_END_REL_NANOS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOpEndRelNanos());
hash = (37 * hash) + ALL_END_REL_NANOS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getAllEndRelNanos());
hash = (37 * hash) + SCHEDULED_NANOS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getScheduledNanos());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tensorflow.framework.NodeExecStats parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.NodeExecStats parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.NodeExecStats parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.NodeExecStats parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.NodeExecStats parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.NodeExecStats parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.NodeExecStats parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.framework.NodeExecStats parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.framework.NodeExecStats parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tensorflow.framework.NodeExecStats parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.framework.NodeExecStats parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.framework.NodeExecStats parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tensorflow.framework.NodeExecStats prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Time/size stats recorded for a single execution of a graph node.
*
*
* Protobuf type {@code tensorflow.NodeExecStats}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.NodeExecStats)
org.tensorflow.framework.NodeExecStatsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.framework.StepStatsProtos.internal_static_tensorflow_NodeExecStats_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.framework.StepStatsProtos.internal_static_tensorflow_NodeExecStats_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.framework.NodeExecStats.class, org.tensorflow.framework.NodeExecStats.Builder.class);
}
// Construct using org.tensorflow.framework.NodeExecStats.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getMemoryFieldBuilder();
getOutputFieldBuilder();
getReferencedTensorFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
nodeName_ = "";
allStartMicros_ = 0L;
opStartRelMicros_ = 0L;
opEndRelMicros_ = 0L;
allEndRelMicros_ = 0L;
if (memoryBuilder_ == null) {
memory_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
} else {
memoryBuilder_.clear();
}
if (outputBuilder_ == null) {
output_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
} else {
outputBuilder_.clear();
}
timelineLabel_ = "";
scheduledMicros_ = 0L;
threadId_ = 0;
if (referencedTensorBuilder_ == null) {
referencedTensor_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000400);
} else {
referencedTensorBuilder_.clear();
}
if (memoryStatsBuilder_ == null) {
memoryStats_ = null;
} else {
memoryStats_ = null;
memoryStatsBuilder_ = null;
}
allStartNanos_ = 0L;
opStartRelNanos_ = 0L;
opEndRelNanos_ = 0L;
allEndRelNanos_ = 0L;
scheduledNanos_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tensorflow.framework.StepStatsProtos.internal_static_tensorflow_NodeExecStats_descriptor;
}
@java.lang.Override
public org.tensorflow.framework.NodeExecStats getDefaultInstanceForType() {
return org.tensorflow.framework.NodeExecStats.getDefaultInstance();
}
@java.lang.Override
public org.tensorflow.framework.NodeExecStats build() {
org.tensorflow.framework.NodeExecStats result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.tensorflow.framework.NodeExecStats buildPartial() {
org.tensorflow.framework.NodeExecStats result = new org.tensorflow.framework.NodeExecStats(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.nodeName_ = nodeName_;
result.allStartMicros_ = allStartMicros_;
result.opStartRelMicros_ = opStartRelMicros_;
result.opEndRelMicros_ = opEndRelMicros_;
result.allEndRelMicros_ = allEndRelMicros_;
if (memoryBuilder_ == null) {
if (((bitField0_ & 0x00000020) == 0x00000020)) {
memory_ = java.util.Collections.unmodifiableList(memory_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.memory_ = memory_;
} else {
result.memory_ = memoryBuilder_.build();
}
if (outputBuilder_ == null) {
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output_ = java.util.Collections.unmodifiableList(output_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.output_ = output_;
} else {
result.output_ = outputBuilder_.build();
}
result.timelineLabel_ = timelineLabel_;
result.scheduledMicros_ = scheduledMicros_;
result.threadId_ = threadId_;
if (referencedTensorBuilder_ == null) {
if (((bitField0_ & 0x00000400) == 0x00000400)) {
referencedTensor_ = java.util.Collections.unmodifiableList(referencedTensor_);
bitField0_ = (bitField0_ & ~0x00000400);
}
result.referencedTensor_ = referencedTensor_;
} else {
result.referencedTensor_ = referencedTensorBuilder_.build();
}
if (memoryStatsBuilder_ == null) {
result.memoryStats_ = memoryStats_;
} else {
result.memoryStats_ = memoryStatsBuilder_.build();
}
result.allStartNanos_ = allStartNanos_;
result.opStartRelNanos_ = opStartRelNanos_;
result.opEndRelNanos_ = opEndRelNanos_;
result.allEndRelNanos_ = allEndRelNanos_;
result.scheduledNanos_ = scheduledNanos_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tensorflow.framework.NodeExecStats) {
return mergeFrom((org.tensorflow.framework.NodeExecStats)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tensorflow.framework.NodeExecStats other) {
if (other == org.tensorflow.framework.NodeExecStats.getDefaultInstance()) return this;
if (!other.getNodeName().isEmpty()) {
nodeName_ = other.nodeName_;
onChanged();
}
if (other.getAllStartMicros() != 0L) {
setAllStartMicros(other.getAllStartMicros());
}
if (other.getOpStartRelMicros() != 0L) {
setOpStartRelMicros(other.getOpStartRelMicros());
}
if (other.getOpEndRelMicros() != 0L) {
setOpEndRelMicros(other.getOpEndRelMicros());
}
if (other.getAllEndRelMicros() != 0L) {
setAllEndRelMicros(other.getAllEndRelMicros());
}
if (memoryBuilder_ == null) {
if (!other.memory_.isEmpty()) {
if (memory_.isEmpty()) {
memory_ = other.memory_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureMemoryIsMutable();
memory_.addAll(other.memory_);
}
onChanged();
}
} else {
if (!other.memory_.isEmpty()) {
if (memoryBuilder_.isEmpty()) {
memoryBuilder_.dispose();
memoryBuilder_ = null;
memory_ = other.memory_;
bitField0_ = (bitField0_ & ~0x00000020);
memoryBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getMemoryFieldBuilder() : null;
} else {
memoryBuilder_.addAllMessages(other.memory_);
}
}
}
if (outputBuilder_ == null) {
if (!other.output_.isEmpty()) {
if (output_.isEmpty()) {
output_ = other.output_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureOutputIsMutable();
output_.addAll(other.output_);
}
onChanged();
}
} else {
if (!other.output_.isEmpty()) {
if (outputBuilder_.isEmpty()) {
outputBuilder_.dispose();
outputBuilder_ = null;
output_ = other.output_;
bitField0_ = (bitField0_ & ~0x00000040);
outputBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getOutputFieldBuilder() : null;
} else {
outputBuilder_.addAllMessages(other.output_);
}
}
}
if (!other.getTimelineLabel().isEmpty()) {
timelineLabel_ = other.timelineLabel_;
onChanged();
}
if (other.getScheduledMicros() != 0L) {
setScheduledMicros(other.getScheduledMicros());
}
if (other.getThreadId() != 0) {
setThreadId(other.getThreadId());
}
if (referencedTensorBuilder_ == null) {
if (!other.referencedTensor_.isEmpty()) {
if (referencedTensor_.isEmpty()) {
referencedTensor_ = other.referencedTensor_;
bitField0_ = (bitField0_ & ~0x00000400);
} else {
ensureReferencedTensorIsMutable();
referencedTensor_.addAll(other.referencedTensor_);
}
onChanged();
}
} else {
if (!other.referencedTensor_.isEmpty()) {
if (referencedTensorBuilder_.isEmpty()) {
referencedTensorBuilder_.dispose();
referencedTensorBuilder_ = null;
referencedTensor_ = other.referencedTensor_;
bitField0_ = (bitField0_ & ~0x00000400);
referencedTensorBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getReferencedTensorFieldBuilder() : null;
} else {
referencedTensorBuilder_.addAllMessages(other.referencedTensor_);
}
}
}
if (other.hasMemoryStats()) {
mergeMemoryStats(other.getMemoryStats());
}
if (other.getAllStartNanos() != 0L) {
setAllStartNanos(other.getAllStartNanos());
}
if (other.getOpStartRelNanos() != 0L) {
setOpStartRelNanos(other.getOpStartRelNanos());
}
if (other.getOpEndRelNanos() != 0L) {
setOpEndRelNanos(other.getOpEndRelNanos());
}
if (other.getAllEndRelNanos() != 0L) {
setAllEndRelNanos(other.getAllEndRelNanos());
}
if (other.getScheduledNanos() != 0L) {
setScheduledNanos(other.getScheduledNanos());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tensorflow.framework.NodeExecStats parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tensorflow.framework.NodeExecStats) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object nodeName_ = "";
/**
*
* TODO(tucker): Use some more compact form of node identity than
* the full string name. Either all processes should agree on a
* global id (cost_id?) for each node, or we should use a hash of
* the name.
*
*
* string node_name = 1;
*/
public java.lang.String getNodeName() {
java.lang.Object ref = nodeName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
nodeName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* TODO(tucker): Use some more compact form of node identity than
* the full string name. Either all processes should agree on a
* global id (cost_id?) for each node, or we should use a hash of
* the name.
*
*
* string node_name = 1;
*/
public com.google.protobuf.ByteString
getNodeNameBytes() {
java.lang.Object ref = nodeName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nodeName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* TODO(tucker): Use some more compact form of node identity than
* the full string name. Either all processes should agree on a
* global id (cost_id?) for each node, or we should use a hash of
* the name.
*
*
* string node_name = 1;
*/
public Builder setNodeName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
nodeName_ = value;
onChanged();
return this;
}
/**
*
* TODO(tucker): Use some more compact form of node identity than
* the full string name. Either all processes should agree on a
* global id (cost_id?) for each node, or we should use a hash of
* the name.
*
*
* string node_name = 1;
*/
public Builder clearNodeName() {
nodeName_ = getDefaultInstance().getNodeName();
onChanged();
return this;
}
/**
*
* TODO(tucker): Use some more compact form of node identity than
* the full string name. Either all processes should agree on a
* global id (cost_id?) for each node, or we should use a hash of
* the name.
*
*
* string node_name = 1;
*/
public Builder setNodeNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
nodeName_ = value;
onChanged();
return this;
}
private long allStartMicros_ ;
/**
* int64 all_start_micros = 2;
*/
public long getAllStartMicros() {
return allStartMicros_;
}
/**
* int64 all_start_micros = 2;
*/
public Builder setAllStartMicros(long value) {
allStartMicros_ = value;
onChanged();
return this;
}
/**
* int64 all_start_micros = 2;
*/
public Builder clearAllStartMicros() {
allStartMicros_ = 0L;
onChanged();
return this;
}
private long opStartRelMicros_ ;
/**
* int64 op_start_rel_micros = 3;
*/
public long getOpStartRelMicros() {
return opStartRelMicros_;
}
/**
* int64 op_start_rel_micros = 3;
*/
public Builder setOpStartRelMicros(long value) {
opStartRelMicros_ = value;
onChanged();
return this;
}
/**
* int64 op_start_rel_micros = 3;
*/
public Builder clearOpStartRelMicros() {
opStartRelMicros_ = 0L;
onChanged();
return this;
}
private long opEndRelMicros_ ;
/**
* int64 op_end_rel_micros = 4;
*/
public long getOpEndRelMicros() {
return opEndRelMicros_;
}
/**
* int64 op_end_rel_micros = 4;
*/
public Builder setOpEndRelMicros(long value) {
opEndRelMicros_ = value;
onChanged();
return this;
}
/**
* int64 op_end_rel_micros = 4;
*/
public Builder clearOpEndRelMicros() {
opEndRelMicros_ = 0L;
onChanged();
return this;
}
private long allEndRelMicros_ ;
/**
* int64 all_end_rel_micros = 5;
*/
public long getAllEndRelMicros() {
return allEndRelMicros_;
}
/**
* int64 all_end_rel_micros = 5;
*/
public Builder setAllEndRelMicros(long value) {
allEndRelMicros_ = value;
onChanged();
return this;
}
/**
* int64 all_end_rel_micros = 5;
*/
public Builder clearAllEndRelMicros() {
allEndRelMicros_ = 0L;
onChanged();
return this;
}
private java.util.List memory_ =
java.util.Collections.emptyList();
private void ensureMemoryIsMutable() {
if (!((bitField0_ & 0x00000020) == 0x00000020)) {
memory_ = new java.util.ArrayList(memory_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.AllocatorMemoryUsed, org.tensorflow.framework.AllocatorMemoryUsed.Builder, org.tensorflow.framework.AllocatorMemoryUsedOrBuilder> memoryBuilder_;
/**
* repeated .tensorflow.AllocatorMemoryUsed memory = 6;
*/
public java.util.List getMemoryList() {
if (memoryBuilder_ == null) {
return java.util.Collections.unmodifiableList(memory_);
} else {
return memoryBuilder_.getMessageList();
}
}
/**
* repeated .tensorflow.AllocatorMemoryUsed memory = 6;
*/
public int getMemoryCount() {
if (memoryBuilder_ == null) {
return memory_.size();
} else {
return memoryBuilder_.getCount();
}
}
/**
* repeated .tensorflow.AllocatorMemoryUsed memory = 6;
*/
public org.tensorflow.framework.AllocatorMemoryUsed getMemory(int index) {
if (memoryBuilder_ == null) {
return memory_.get(index);
} else {
return memoryBuilder_.getMessage(index);
}
}
/**
* repeated .tensorflow.AllocatorMemoryUsed memory = 6;
*/
public Builder setMemory(
int index, org.tensorflow.framework.AllocatorMemoryUsed value) {
if (memoryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMemoryIsMutable();
memory_.set(index, value);
onChanged();
} else {
memoryBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .tensorflow.AllocatorMemoryUsed memory = 6;
*/
public Builder setMemory(
int index, org.tensorflow.framework.AllocatorMemoryUsed.Builder builderForValue) {
if (memoryBuilder_ == null) {
ensureMemoryIsMutable();
memory_.set(index, builderForValue.build());
onChanged();
} else {
memoryBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.AllocatorMemoryUsed memory = 6;
*/
public Builder addMemory(org.tensorflow.framework.AllocatorMemoryUsed value) {
if (memoryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMemoryIsMutable();
memory_.add(value);
onChanged();
} else {
memoryBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .tensorflow.AllocatorMemoryUsed memory = 6;
*/
public Builder addMemory(
int index, org.tensorflow.framework.AllocatorMemoryUsed value) {
if (memoryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMemoryIsMutable();
memory_.add(index, value);
onChanged();
} else {
memoryBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .tensorflow.AllocatorMemoryUsed memory = 6;
*/
public Builder addMemory(
org.tensorflow.framework.AllocatorMemoryUsed.Builder builderForValue) {
if (memoryBuilder_ == null) {
ensureMemoryIsMutable();
memory_.add(builderForValue.build());
onChanged();
} else {
memoryBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.AllocatorMemoryUsed memory = 6;
*/
public Builder addMemory(
int index, org.tensorflow.framework.AllocatorMemoryUsed.Builder builderForValue) {
if (memoryBuilder_ == null) {
ensureMemoryIsMutable();
memory_.add(index, builderForValue.build());
onChanged();
} else {
memoryBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.AllocatorMemoryUsed memory = 6;
*/
public Builder addAllMemory(
java.lang.Iterable extends org.tensorflow.framework.AllocatorMemoryUsed> values) {
if (memoryBuilder_ == null) {
ensureMemoryIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, memory_);
onChanged();
} else {
memoryBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .tensorflow.AllocatorMemoryUsed memory = 6;
*/
public Builder clearMemory() {
if (memoryBuilder_ == null) {
memory_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
memoryBuilder_.clear();
}
return this;
}
/**
* repeated .tensorflow.AllocatorMemoryUsed memory = 6;
*/
public Builder removeMemory(int index) {
if (memoryBuilder_ == null) {
ensureMemoryIsMutable();
memory_.remove(index);
onChanged();
} else {
memoryBuilder_.remove(index);
}
return this;
}
/**
* repeated .tensorflow.AllocatorMemoryUsed memory = 6;
*/
public org.tensorflow.framework.AllocatorMemoryUsed.Builder getMemoryBuilder(
int index) {
return getMemoryFieldBuilder().getBuilder(index);
}
/**
* repeated .tensorflow.AllocatorMemoryUsed memory = 6;
*/
public org.tensorflow.framework.AllocatorMemoryUsedOrBuilder getMemoryOrBuilder(
int index) {
if (memoryBuilder_ == null) {
return memory_.get(index); } else {
return memoryBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .tensorflow.AllocatorMemoryUsed memory = 6;
*/
public java.util.List extends org.tensorflow.framework.AllocatorMemoryUsedOrBuilder>
getMemoryOrBuilderList() {
if (memoryBuilder_ != null) {
return memoryBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(memory_);
}
}
/**
* repeated .tensorflow.AllocatorMemoryUsed memory = 6;
*/
public org.tensorflow.framework.AllocatorMemoryUsed.Builder addMemoryBuilder() {
return getMemoryFieldBuilder().addBuilder(
org.tensorflow.framework.AllocatorMemoryUsed.getDefaultInstance());
}
/**
* repeated .tensorflow.AllocatorMemoryUsed memory = 6;
*/
public org.tensorflow.framework.AllocatorMemoryUsed.Builder addMemoryBuilder(
int index) {
return getMemoryFieldBuilder().addBuilder(
index, org.tensorflow.framework.AllocatorMemoryUsed.getDefaultInstance());
}
/**
* repeated .tensorflow.AllocatorMemoryUsed memory = 6;
*/
public java.util.List
getMemoryBuilderList() {
return getMemoryFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.AllocatorMemoryUsed, org.tensorflow.framework.AllocatorMemoryUsed.Builder, org.tensorflow.framework.AllocatorMemoryUsedOrBuilder>
getMemoryFieldBuilder() {
if (memoryBuilder_ == null) {
memoryBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.AllocatorMemoryUsed, org.tensorflow.framework.AllocatorMemoryUsed.Builder, org.tensorflow.framework.AllocatorMemoryUsedOrBuilder>(
memory_,
((bitField0_ & 0x00000020) == 0x00000020),
getParentForChildren(),
isClean());
memory_ = null;
}
return memoryBuilder_;
}
private java.util.List output_ =
java.util.Collections.emptyList();
private void ensureOutputIsMutable() {
if (!((bitField0_ & 0x00000040) == 0x00000040)) {
output_ = new java.util.ArrayList(output_);
bitField0_ |= 0x00000040;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.NodeOutput, org.tensorflow.framework.NodeOutput.Builder, org.tensorflow.framework.NodeOutputOrBuilder> outputBuilder_;
/**
* repeated .tensorflow.NodeOutput output = 7;
*/
public java.util.List getOutputList() {
if (outputBuilder_ == null) {
return java.util.Collections.unmodifiableList(output_);
} else {
return outputBuilder_.getMessageList();
}
}
/**
* repeated .tensorflow.NodeOutput output = 7;
*/
public int getOutputCount() {
if (outputBuilder_ == null) {
return output_.size();
} else {
return outputBuilder_.getCount();
}
}
/**
* repeated .tensorflow.NodeOutput output = 7;
*/
public org.tensorflow.framework.NodeOutput getOutput(int index) {
if (outputBuilder_ == null) {
return output_.get(index);
} else {
return outputBuilder_.getMessage(index);
}
}
/**
* repeated .tensorflow.NodeOutput output = 7;
*/
public Builder setOutput(
int index, org.tensorflow.framework.NodeOutput value) {
if (outputBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputIsMutable();
output_.set(index, value);
onChanged();
} else {
outputBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .tensorflow.NodeOutput output = 7;
*/
public Builder setOutput(
int index, org.tensorflow.framework.NodeOutput.Builder builderForValue) {
if (outputBuilder_ == null) {
ensureOutputIsMutable();
output_.set(index, builderForValue.build());
onChanged();
} else {
outputBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.NodeOutput output = 7;
*/
public Builder addOutput(org.tensorflow.framework.NodeOutput value) {
if (outputBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputIsMutable();
output_.add(value);
onChanged();
} else {
outputBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .tensorflow.NodeOutput output = 7;
*/
public Builder addOutput(
int index, org.tensorflow.framework.NodeOutput value) {
if (outputBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputIsMutable();
output_.add(index, value);
onChanged();
} else {
outputBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .tensorflow.NodeOutput output = 7;
*/
public Builder addOutput(
org.tensorflow.framework.NodeOutput.Builder builderForValue) {
if (outputBuilder_ == null) {
ensureOutputIsMutable();
output_.add(builderForValue.build());
onChanged();
} else {
outputBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.NodeOutput output = 7;
*/
public Builder addOutput(
int index, org.tensorflow.framework.NodeOutput.Builder builderForValue) {
if (outputBuilder_ == null) {
ensureOutputIsMutable();
output_.add(index, builderForValue.build());
onChanged();
} else {
outputBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.NodeOutput output = 7;
*/
public Builder addAllOutput(
java.lang.Iterable extends org.tensorflow.framework.NodeOutput> values) {
if (outputBuilder_ == null) {
ensureOutputIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, output_);
onChanged();
} else {
outputBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .tensorflow.NodeOutput output = 7;
*/
public Builder clearOutput() {
if (outputBuilder_ == null) {
output_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
} else {
outputBuilder_.clear();
}
return this;
}
/**
* repeated .tensorflow.NodeOutput output = 7;
*/
public Builder removeOutput(int index) {
if (outputBuilder_ == null) {
ensureOutputIsMutable();
output_.remove(index);
onChanged();
} else {
outputBuilder_.remove(index);
}
return this;
}
/**
* repeated .tensorflow.NodeOutput output = 7;
*/
public org.tensorflow.framework.NodeOutput.Builder getOutputBuilder(
int index) {
return getOutputFieldBuilder().getBuilder(index);
}
/**
* repeated .tensorflow.NodeOutput output = 7;
*/
public org.tensorflow.framework.NodeOutputOrBuilder getOutputOrBuilder(
int index) {
if (outputBuilder_ == null) {
return output_.get(index); } else {
return outputBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .tensorflow.NodeOutput output = 7;
*/
public java.util.List extends org.tensorflow.framework.NodeOutputOrBuilder>
getOutputOrBuilderList() {
if (outputBuilder_ != null) {
return outputBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(output_);
}
}
/**
* repeated .tensorflow.NodeOutput output = 7;
*/
public org.tensorflow.framework.NodeOutput.Builder addOutputBuilder() {
return getOutputFieldBuilder().addBuilder(
org.tensorflow.framework.NodeOutput.getDefaultInstance());
}
/**
* repeated .tensorflow.NodeOutput output = 7;
*/
public org.tensorflow.framework.NodeOutput.Builder addOutputBuilder(
int index) {
return getOutputFieldBuilder().addBuilder(
index, org.tensorflow.framework.NodeOutput.getDefaultInstance());
}
/**
* repeated .tensorflow.NodeOutput output = 7;
*/
public java.util.List
getOutputBuilderList() {
return getOutputFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.NodeOutput, org.tensorflow.framework.NodeOutput.Builder, org.tensorflow.framework.NodeOutputOrBuilder>
getOutputFieldBuilder() {
if (outputBuilder_ == null) {
outputBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.NodeOutput, org.tensorflow.framework.NodeOutput.Builder, org.tensorflow.framework.NodeOutputOrBuilder>(
output_,
((bitField0_ & 0x00000040) == 0x00000040),
getParentForChildren(),
isClean());
output_ = null;
}
return outputBuilder_;
}
private java.lang.Object timelineLabel_ = "";
/**
* string timeline_label = 8;
*/
public java.lang.String getTimelineLabel() {
java.lang.Object ref = timelineLabel_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
timelineLabel_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string timeline_label = 8;
*/
public com.google.protobuf.ByteString
getTimelineLabelBytes() {
java.lang.Object ref = timelineLabel_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
timelineLabel_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string timeline_label = 8;
*/
public Builder setTimelineLabel(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
timelineLabel_ = value;
onChanged();
return this;
}
/**
* string timeline_label = 8;
*/
public Builder clearTimelineLabel() {
timelineLabel_ = getDefaultInstance().getTimelineLabel();
onChanged();
return this;
}
/**
* string timeline_label = 8;
*/
public Builder setTimelineLabelBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
timelineLabel_ = value;
onChanged();
return this;
}
private long scheduledMicros_ ;
/**
* int64 scheduled_micros = 9;
*/
public long getScheduledMicros() {
return scheduledMicros_;
}
/**
* int64 scheduled_micros = 9;
*/
public Builder setScheduledMicros(long value) {
scheduledMicros_ = value;
onChanged();
return this;
}
/**
* int64 scheduled_micros = 9;
*/
public Builder clearScheduledMicros() {
scheduledMicros_ = 0L;
onChanged();
return this;
}
private int threadId_ ;
/**
* uint32 thread_id = 10;
*/
public int getThreadId() {
return threadId_;
}
/**
* uint32 thread_id = 10;
*/
public Builder setThreadId(int value) {
threadId_ = value;
onChanged();
return this;
}
/**
* uint32 thread_id = 10;
*/
public Builder clearThreadId() {
threadId_ = 0;
onChanged();
return this;
}
private java.util.List referencedTensor_ =
java.util.Collections.emptyList();
private void ensureReferencedTensorIsMutable() {
if (!((bitField0_ & 0x00000400) == 0x00000400)) {
referencedTensor_ = new java.util.ArrayList(referencedTensor_);
bitField0_ |= 0x00000400;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.AllocationDescription, org.tensorflow.framework.AllocationDescription.Builder, org.tensorflow.framework.AllocationDescriptionOrBuilder> referencedTensorBuilder_;
/**
* repeated .tensorflow.AllocationDescription referenced_tensor = 11;
*/
public java.util.List getReferencedTensorList() {
if (referencedTensorBuilder_ == null) {
return java.util.Collections.unmodifiableList(referencedTensor_);
} else {
return referencedTensorBuilder_.getMessageList();
}
}
/**
* repeated .tensorflow.AllocationDescription referenced_tensor = 11;
*/
public int getReferencedTensorCount() {
if (referencedTensorBuilder_ == null) {
return referencedTensor_.size();
} else {
return referencedTensorBuilder_.getCount();
}
}
/**
* repeated .tensorflow.AllocationDescription referenced_tensor = 11;
*/
public org.tensorflow.framework.AllocationDescription getReferencedTensor(int index) {
if (referencedTensorBuilder_ == null) {
return referencedTensor_.get(index);
} else {
return referencedTensorBuilder_.getMessage(index);
}
}
/**
* repeated .tensorflow.AllocationDescription referenced_tensor = 11;
*/
public Builder setReferencedTensor(
int index, org.tensorflow.framework.AllocationDescription value) {
if (referencedTensorBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureReferencedTensorIsMutable();
referencedTensor_.set(index, value);
onChanged();
} else {
referencedTensorBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .tensorflow.AllocationDescription referenced_tensor = 11;
*/
public Builder setReferencedTensor(
int index, org.tensorflow.framework.AllocationDescription.Builder builderForValue) {
if (referencedTensorBuilder_ == null) {
ensureReferencedTensorIsMutable();
referencedTensor_.set(index, builderForValue.build());
onChanged();
} else {
referencedTensorBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.AllocationDescription referenced_tensor = 11;
*/
public Builder addReferencedTensor(org.tensorflow.framework.AllocationDescription value) {
if (referencedTensorBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureReferencedTensorIsMutable();
referencedTensor_.add(value);
onChanged();
} else {
referencedTensorBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .tensorflow.AllocationDescription referenced_tensor = 11;
*/
public Builder addReferencedTensor(
int index, org.tensorflow.framework.AllocationDescription value) {
if (referencedTensorBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureReferencedTensorIsMutable();
referencedTensor_.add(index, value);
onChanged();
} else {
referencedTensorBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .tensorflow.AllocationDescription referenced_tensor = 11;
*/
public Builder addReferencedTensor(
org.tensorflow.framework.AllocationDescription.Builder builderForValue) {
if (referencedTensorBuilder_ == null) {
ensureReferencedTensorIsMutable();
referencedTensor_.add(builderForValue.build());
onChanged();
} else {
referencedTensorBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.AllocationDescription referenced_tensor = 11;
*/
public Builder addReferencedTensor(
int index, org.tensorflow.framework.AllocationDescription.Builder builderForValue) {
if (referencedTensorBuilder_ == null) {
ensureReferencedTensorIsMutable();
referencedTensor_.add(index, builderForValue.build());
onChanged();
} else {
referencedTensorBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.AllocationDescription referenced_tensor = 11;
*/
public Builder addAllReferencedTensor(
java.lang.Iterable extends org.tensorflow.framework.AllocationDescription> values) {
if (referencedTensorBuilder_ == null) {
ensureReferencedTensorIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, referencedTensor_);
onChanged();
} else {
referencedTensorBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .tensorflow.AllocationDescription referenced_tensor = 11;
*/
public Builder clearReferencedTensor() {
if (referencedTensorBuilder_ == null) {
referencedTensor_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000400);
onChanged();
} else {
referencedTensorBuilder_.clear();
}
return this;
}
/**
* repeated .tensorflow.AllocationDescription referenced_tensor = 11;
*/
public Builder removeReferencedTensor(int index) {
if (referencedTensorBuilder_ == null) {
ensureReferencedTensorIsMutable();
referencedTensor_.remove(index);
onChanged();
} else {
referencedTensorBuilder_.remove(index);
}
return this;
}
/**
* repeated .tensorflow.AllocationDescription referenced_tensor = 11;
*/
public org.tensorflow.framework.AllocationDescription.Builder getReferencedTensorBuilder(
int index) {
return getReferencedTensorFieldBuilder().getBuilder(index);
}
/**
* repeated .tensorflow.AllocationDescription referenced_tensor = 11;
*/
public org.tensorflow.framework.AllocationDescriptionOrBuilder getReferencedTensorOrBuilder(
int index) {
if (referencedTensorBuilder_ == null) {
return referencedTensor_.get(index); } else {
return referencedTensorBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .tensorflow.AllocationDescription referenced_tensor = 11;
*/
public java.util.List extends org.tensorflow.framework.AllocationDescriptionOrBuilder>
getReferencedTensorOrBuilderList() {
if (referencedTensorBuilder_ != null) {
return referencedTensorBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(referencedTensor_);
}
}
/**
* repeated .tensorflow.AllocationDescription referenced_tensor = 11;
*/
public org.tensorflow.framework.AllocationDescription.Builder addReferencedTensorBuilder() {
return getReferencedTensorFieldBuilder().addBuilder(
org.tensorflow.framework.AllocationDescription.getDefaultInstance());
}
/**
* repeated .tensorflow.AllocationDescription referenced_tensor = 11;
*/
public org.tensorflow.framework.AllocationDescription.Builder addReferencedTensorBuilder(
int index) {
return getReferencedTensorFieldBuilder().addBuilder(
index, org.tensorflow.framework.AllocationDescription.getDefaultInstance());
}
/**
* repeated .tensorflow.AllocationDescription referenced_tensor = 11;
*/
public java.util.List
getReferencedTensorBuilderList() {
return getReferencedTensorFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.AllocationDescription, org.tensorflow.framework.AllocationDescription.Builder, org.tensorflow.framework.AllocationDescriptionOrBuilder>
getReferencedTensorFieldBuilder() {
if (referencedTensorBuilder_ == null) {
referencedTensorBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.AllocationDescription, org.tensorflow.framework.AllocationDescription.Builder, org.tensorflow.framework.AllocationDescriptionOrBuilder>(
referencedTensor_,
((bitField0_ & 0x00000400) == 0x00000400),
getParentForChildren(),
isClean());
referencedTensor_ = null;
}
return referencedTensorBuilder_;
}
private org.tensorflow.framework.MemoryStats memoryStats_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.MemoryStats, org.tensorflow.framework.MemoryStats.Builder, org.tensorflow.framework.MemoryStatsOrBuilder> memoryStatsBuilder_;
/**
* .tensorflow.MemoryStats memory_stats = 12;
*/
public boolean hasMemoryStats() {
return memoryStatsBuilder_ != null || memoryStats_ != null;
}
/**
* .tensorflow.MemoryStats memory_stats = 12;
*/
public org.tensorflow.framework.MemoryStats getMemoryStats() {
if (memoryStatsBuilder_ == null) {
return memoryStats_ == null ? org.tensorflow.framework.MemoryStats.getDefaultInstance() : memoryStats_;
} else {
return memoryStatsBuilder_.getMessage();
}
}
/**
* .tensorflow.MemoryStats memory_stats = 12;
*/
public Builder setMemoryStats(org.tensorflow.framework.MemoryStats value) {
if (memoryStatsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
memoryStats_ = value;
onChanged();
} else {
memoryStatsBuilder_.setMessage(value);
}
return this;
}
/**
* .tensorflow.MemoryStats memory_stats = 12;
*/
public Builder setMemoryStats(
org.tensorflow.framework.MemoryStats.Builder builderForValue) {
if (memoryStatsBuilder_ == null) {
memoryStats_ = builderForValue.build();
onChanged();
} else {
memoryStatsBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .tensorflow.MemoryStats memory_stats = 12;
*/
public Builder mergeMemoryStats(org.tensorflow.framework.MemoryStats value) {
if (memoryStatsBuilder_ == null) {
if (memoryStats_ != null) {
memoryStats_ =
org.tensorflow.framework.MemoryStats.newBuilder(memoryStats_).mergeFrom(value).buildPartial();
} else {
memoryStats_ = value;
}
onChanged();
} else {
memoryStatsBuilder_.mergeFrom(value);
}
return this;
}
/**
* .tensorflow.MemoryStats memory_stats = 12;
*/
public Builder clearMemoryStats() {
if (memoryStatsBuilder_ == null) {
memoryStats_ = null;
onChanged();
} else {
memoryStats_ = null;
memoryStatsBuilder_ = null;
}
return this;
}
/**
* .tensorflow.MemoryStats memory_stats = 12;
*/
public org.tensorflow.framework.MemoryStats.Builder getMemoryStatsBuilder() {
onChanged();
return getMemoryStatsFieldBuilder().getBuilder();
}
/**
* .tensorflow.MemoryStats memory_stats = 12;
*/
public org.tensorflow.framework.MemoryStatsOrBuilder getMemoryStatsOrBuilder() {
if (memoryStatsBuilder_ != null) {
return memoryStatsBuilder_.getMessageOrBuilder();
} else {
return memoryStats_ == null ?
org.tensorflow.framework.MemoryStats.getDefaultInstance() : memoryStats_;
}
}
/**
* .tensorflow.MemoryStats memory_stats = 12;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.MemoryStats, org.tensorflow.framework.MemoryStats.Builder, org.tensorflow.framework.MemoryStatsOrBuilder>
getMemoryStatsFieldBuilder() {
if (memoryStatsBuilder_ == null) {
memoryStatsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.MemoryStats, org.tensorflow.framework.MemoryStats.Builder, org.tensorflow.framework.MemoryStatsOrBuilder>(
getMemoryStats(),
getParentForChildren(),
isClean());
memoryStats_ = null;
}
return memoryStatsBuilder_;
}
private long allStartNanos_ ;
/**
* int64 all_start_nanos = 13;
*/
public long getAllStartNanos() {
return allStartNanos_;
}
/**
* int64 all_start_nanos = 13;
*/
public Builder setAllStartNanos(long value) {
allStartNanos_ = value;
onChanged();
return this;
}
/**
* int64 all_start_nanos = 13;
*/
public Builder clearAllStartNanos() {
allStartNanos_ = 0L;
onChanged();
return this;
}
private long opStartRelNanos_ ;
/**
* int64 op_start_rel_nanos = 14;
*/
public long getOpStartRelNanos() {
return opStartRelNanos_;
}
/**
* int64 op_start_rel_nanos = 14;
*/
public Builder setOpStartRelNanos(long value) {
opStartRelNanos_ = value;
onChanged();
return this;
}
/**
* int64 op_start_rel_nanos = 14;
*/
public Builder clearOpStartRelNanos() {
opStartRelNanos_ = 0L;
onChanged();
return this;
}
private long opEndRelNanos_ ;
/**
* int64 op_end_rel_nanos = 15;
*/
public long getOpEndRelNanos() {
return opEndRelNanos_;
}
/**
* int64 op_end_rel_nanos = 15;
*/
public Builder setOpEndRelNanos(long value) {
opEndRelNanos_ = value;
onChanged();
return this;
}
/**
* int64 op_end_rel_nanos = 15;
*/
public Builder clearOpEndRelNanos() {
opEndRelNanos_ = 0L;
onChanged();
return this;
}
private long allEndRelNanos_ ;
/**
* int64 all_end_rel_nanos = 16;
*/
public long getAllEndRelNanos() {
return allEndRelNanos_;
}
/**
* int64 all_end_rel_nanos = 16;
*/
public Builder setAllEndRelNanos(long value) {
allEndRelNanos_ = value;
onChanged();
return this;
}
/**
* int64 all_end_rel_nanos = 16;
*/
public Builder clearAllEndRelNanos() {
allEndRelNanos_ = 0L;
onChanged();
return this;
}
private long scheduledNanos_ ;
/**
* int64 scheduled_nanos = 17;
*/
public long getScheduledNanos() {
return scheduledNanos_;
}
/**
* int64 scheduled_nanos = 17;
*/
public Builder setScheduledNanos(long value) {
scheduledNanos_ = value;
onChanged();
return this;
}
/**
* int64 scheduled_nanos = 17;
*/
public Builder clearScheduledNanos() {
scheduledNanos_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.NodeExecStats)
}
// @@protoc_insertion_point(class_scope:tensorflow.NodeExecStats)
private static final org.tensorflow.framework.NodeExecStats DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tensorflow.framework.NodeExecStats();
}
public static org.tensorflow.framework.NodeExecStats getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public NodeExecStats parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new NodeExecStats(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.tensorflow.framework.NodeExecStats getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy