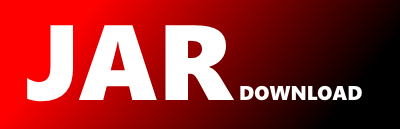
tensorflow.ReplayLog Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: tensorflow/core/protobuf/replay_log.proto
package tensorflow;
public final class ReplayLog {
private ReplayLog() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface NewReplaySessionOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.NewReplaySession)
com.google.protobuf.MessageOrBuilder {
/**
* .tensorflow.ListDevicesResponse devices = 1;
*/
boolean hasDevices();
/**
* .tensorflow.ListDevicesResponse devices = 1;
*/
org.tensorflow.distruntime.ListDevicesResponse getDevices();
/**
* .tensorflow.ListDevicesResponse devices = 1;
*/
org.tensorflow.distruntime.ListDevicesResponseOrBuilder getDevicesOrBuilder();
/**
* string session_handle = 2;
*/
java.lang.String getSessionHandle();
/**
* string session_handle = 2;
*/
com.google.protobuf.ByteString
getSessionHandleBytes();
}
/**
*
* Records the creation of a new replay session. We record the device listing
* here to capture the state of the cluster.
*
*
* Protobuf type {@code tensorflow.NewReplaySession}
*/
public static final class NewReplaySession extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.NewReplaySession)
NewReplaySessionOrBuilder {
private static final long serialVersionUID = 0L;
// Use NewReplaySession.newBuilder() to construct.
private NewReplaySession(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private NewReplaySession() {
sessionHandle_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private NewReplaySession(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
org.tensorflow.distruntime.ListDevicesResponse.Builder subBuilder = null;
if (devices_ != null) {
subBuilder = devices_.toBuilder();
}
devices_ = input.readMessage(org.tensorflow.distruntime.ListDevicesResponse.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(devices_);
devices_ = subBuilder.buildPartial();
}
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
sessionHandle_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.ReplayLog.internal_static_tensorflow_NewReplaySession_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.ReplayLog.internal_static_tensorflow_NewReplaySession_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.ReplayLog.NewReplaySession.class, tensorflow.ReplayLog.NewReplaySession.Builder.class);
}
public static final int DEVICES_FIELD_NUMBER = 1;
private org.tensorflow.distruntime.ListDevicesResponse devices_;
/**
* .tensorflow.ListDevicesResponse devices = 1;
*/
public boolean hasDevices() {
return devices_ != null;
}
/**
* .tensorflow.ListDevicesResponse devices = 1;
*/
public org.tensorflow.distruntime.ListDevicesResponse getDevices() {
return devices_ == null ? org.tensorflow.distruntime.ListDevicesResponse.getDefaultInstance() : devices_;
}
/**
* .tensorflow.ListDevicesResponse devices = 1;
*/
public org.tensorflow.distruntime.ListDevicesResponseOrBuilder getDevicesOrBuilder() {
return getDevices();
}
public static final int SESSION_HANDLE_FIELD_NUMBER = 2;
private volatile java.lang.Object sessionHandle_;
/**
* string session_handle = 2;
*/
public java.lang.String getSessionHandle() {
java.lang.Object ref = sessionHandle_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sessionHandle_ = s;
return s;
}
}
/**
* string session_handle = 2;
*/
public com.google.protobuf.ByteString
getSessionHandleBytes() {
java.lang.Object ref = sessionHandle_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sessionHandle_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (devices_ != null) {
output.writeMessage(1, getDevices());
}
if (!getSessionHandleBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, sessionHandle_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (devices_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getDevices());
}
if (!getSessionHandleBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, sessionHandle_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.ReplayLog.NewReplaySession)) {
return super.equals(obj);
}
tensorflow.ReplayLog.NewReplaySession other = (tensorflow.ReplayLog.NewReplaySession) obj;
boolean result = true;
result = result && (hasDevices() == other.hasDevices());
if (hasDevices()) {
result = result && getDevices()
.equals(other.getDevices());
}
result = result && getSessionHandle()
.equals(other.getSessionHandle());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasDevices()) {
hash = (37 * hash) + DEVICES_FIELD_NUMBER;
hash = (53 * hash) + getDevices().hashCode();
}
hash = (37 * hash) + SESSION_HANDLE_FIELD_NUMBER;
hash = (53 * hash) + getSessionHandle().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.ReplayLog.NewReplaySession parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.ReplayLog.NewReplaySession parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.ReplayLog.NewReplaySession parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.ReplayLog.NewReplaySession parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.ReplayLog.NewReplaySession parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.ReplayLog.NewReplaySession parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.ReplayLog.NewReplaySession parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.ReplayLog.NewReplaySession parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.ReplayLog.NewReplaySession parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.ReplayLog.NewReplaySession parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.ReplayLog.NewReplaySession parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.ReplayLog.NewReplaySession parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.ReplayLog.NewReplaySession prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Records the creation of a new replay session. We record the device listing
* here to capture the state of the cluster.
*
*
* Protobuf type {@code tensorflow.NewReplaySession}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.NewReplaySession)
tensorflow.ReplayLog.NewReplaySessionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.ReplayLog.internal_static_tensorflow_NewReplaySession_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.ReplayLog.internal_static_tensorflow_NewReplaySession_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.ReplayLog.NewReplaySession.class, tensorflow.ReplayLog.NewReplaySession.Builder.class);
}
// Construct using tensorflow.ReplayLog.NewReplaySession.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (devicesBuilder_ == null) {
devices_ = null;
} else {
devices_ = null;
devicesBuilder_ = null;
}
sessionHandle_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.ReplayLog.internal_static_tensorflow_NewReplaySession_descriptor;
}
@java.lang.Override
public tensorflow.ReplayLog.NewReplaySession getDefaultInstanceForType() {
return tensorflow.ReplayLog.NewReplaySession.getDefaultInstance();
}
@java.lang.Override
public tensorflow.ReplayLog.NewReplaySession build() {
tensorflow.ReplayLog.NewReplaySession result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.ReplayLog.NewReplaySession buildPartial() {
tensorflow.ReplayLog.NewReplaySession result = new tensorflow.ReplayLog.NewReplaySession(this);
if (devicesBuilder_ == null) {
result.devices_ = devices_;
} else {
result.devices_ = devicesBuilder_.build();
}
result.sessionHandle_ = sessionHandle_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.ReplayLog.NewReplaySession) {
return mergeFrom((tensorflow.ReplayLog.NewReplaySession)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.ReplayLog.NewReplaySession other) {
if (other == tensorflow.ReplayLog.NewReplaySession.getDefaultInstance()) return this;
if (other.hasDevices()) {
mergeDevices(other.getDevices());
}
if (!other.getSessionHandle().isEmpty()) {
sessionHandle_ = other.sessionHandle_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.ReplayLog.NewReplaySession parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.ReplayLog.NewReplaySession) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private org.tensorflow.distruntime.ListDevicesResponse devices_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ListDevicesResponse, org.tensorflow.distruntime.ListDevicesResponse.Builder, org.tensorflow.distruntime.ListDevicesResponseOrBuilder> devicesBuilder_;
/**
* .tensorflow.ListDevicesResponse devices = 1;
*/
public boolean hasDevices() {
return devicesBuilder_ != null || devices_ != null;
}
/**
* .tensorflow.ListDevicesResponse devices = 1;
*/
public org.tensorflow.distruntime.ListDevicesResponse getDevices() {
if (devicesBuilder_ == null) {
return devices_ == null ? org.tensorflow.distruntime.ListDevicesResponse.getDefaultInstance() : devices_;
} else {
return devicesBuilder_.getMessage();
}
}
/**
* .tensorflow.ListDevicesResponse devices = 1;
*/
public Builder setDevices(org.tensorflow.distruntime.ListDevicesResponse value) {
if (devicesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
devices_ = value;
onChanged();
} else {
devicesBuilder_.setMessage(value);
}
return this;
}
/**
* .tensorflow.ListDevicesResponse devices = 1;
*/
public Builder setDevices(
org.tensorflow.distruntime.ListDevicesResponse.Builder builderForValue) {
if (devicesBuilder_ == null) {
devices_ = builderForValue.build();
onChanged();
} else {
devicesBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .tensorflow.ListDevicesResponse devices = 1;
*/
public Builder mergeDevices(org.tensorflow.distruntime.ListDevicesResponse value) {
if (devicesBuilder_ == null) {
if (devices_ != null) {
devices_ =
org.tensorflow.distruntime.ListDevicesResponse.newBuilder(devices_).mergeFrom(value).buildPartial();
} else {
devices_ = value;
}
onChanged();
} else {
devicesBuilder_.mergeFrom(value);
}
return this;
}
/**
* .tensorflow.ListDevicesResponse devices = 1;
*/
public Builder clearDevices() {
if (devicesBuilder_ == null) {
devices_ = null;
onChanged();
} else {
devices_ = null;
devicesBuilder_ = null;
}
return this;
}
/**
* .tensorflow.ListDevicesResponse devices = 1;
*/
public org.tensorflow.distruntime.ListDevicesResponse.Builder getDevicesBuilder() {
onChanged();
return getDevicesFieldBuilder().getBuilder();
}
/**
* .tensorflow.ListDevicesResponse devices = 1;
*/
public org.tensorflow.distruntime.ListDevicesResponseOrBuilder getDevicesOrBuilder() {
if (devicesBuilder_ != null) {
return devicesBuilder_.getMessageOrBuilder();
} else {
return devices_ == null ?
org.tensorflow.distruntime.ListDevicesResponse.getDefaultInstance() : devices_;
}
}
/**
* .tensorflow.ListDevicesResponse devices = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ListDevicesResponse, org.tensorflow.distruntime.ListDevicesResponse.Builder, org.tensorflow.distruntime.ListDevicesResponseOrBuilder>
getDevicesFieldBuilder() {
if (devicesBuilder_ == null) {
devicesBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ListDevicesResponse, org.tensorflow.distruntime.ListDevicesResponse.Builder, org.tensorflow.distruntime.ListDevicesResponseOrBuilder>(
getDevices(),
getParentForChildren(),
isClean());
devices_ = null;
}
return devicesBuilder_;
}
private java.lang.Object sessionHandle_ = "";
/**
* string session_handle = 2;
*/
public java.lang.String getSessionHandle() {
java.lang.Object ref = sessionHandle_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sessionHandle_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string session_handle = 2;
*/
public com.google.protobuf.ByteString
getSessionHandleBytes() {
java.lang.Object ref = sessionHandle_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sessionHandle_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string session_handle = 2;
*/
public Builder setSessionHandle(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sessionHandle_ = value;
onChanged();
return this;
}
/**
* string session_handle = 2;
*/
public Builder clearSessionHandle() {
sessionHandle_ = getDefaultInstance().getSessionHandle();
onChanged();
return this;
}
/**
* string session_handle = 2;
*/
public Builder setSessionHandleBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sessionHandle_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.NewReplaySession)
}
// @@protoc_insertion_point(class_scope:tensorflow.NewReplaySession)
private static final tensorflow.ReplayLog.NewReplaySession DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.ReplayLog.NewReplaySession();
}
public static tensorflow.ReplayLog.NewReplaySession getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public NewReplaySession parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new NewReplaySession(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.ReplayLog.NewReplaySession getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ReplayOpOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.ReplayOp)
com.google.protobuf.MessageOrBuilder {
/**
* double start_time_us = 31;
*/
double getStartTimeUs();
/**
* double end_time_us = 32;
*/
double getEndTimeUs();
/**
* .tensorflow.CreateSessionRequest create_session = 1;
*/
boolean hasCreateSession();
/**
* .tensorflow.CreateSessionRequest create_session = 1;
*/
org.tensorflow.distruntime.CreateSessionRequest getCreateSession();
/**
* .tensorflow.CreateSessionRequest create_session = 1;
*/
org.tensorflow.distruntime.CreateSessionRequestOrBuilder getCreateSessionOrBuilder();
/**
* .tensorflow.ExtendSessionRequest extend_session = 2;
*/
boolean hasExtendSession();
/**
* .tensorflow.ExtendSessionRequest extend_session = 2;
*/
org.tensorflow.distruntime.ExtendSessionRequest getExtendSession();
/**
* .tensorflow.ExtendSessionRequest extend_session = 2;
*/
org.tensorflow.distruntime.ExtendSessionRequestOrBuilder getExtendSessionOrBuilder();
/**
* .tensorflow.PartialRunSetupRequest partial_run_setup = 3;
*/
boolean hasPartialRunSetup();
/**
* .tensorflow.PartialRunSetupRequest partial_run_setup = 3;
*/
org.tensorflow.distruntime.PartialRunSetupRequest getPartialRunSetup();
/**
* .tensorflow.PartialRunSetupRequest partial_run_setup = 3;
*/
org.tensorflow.distruntime.PartialRunSetupRequestOrBuilder getPartialRunSetupOrBuilder();
/**
* .tensorflow.RunStepRequest run_step = 4;
*/
boolean hasRunStep();
/**
* .tensorflow.RunStepRequest run_step = 4;
*/
org.tensorflow.distruntime.RunStepRequest getRunStep();
/**
* .tensorflow.RunStepRequest run_step = 4;
*/
org.tensorflow.distruntime.RunStepRequestOrBuilder getRunStepOrBuilder();
/**
* .tensorflow.CloseSessionRequest close_session = 5;
*/
boolean hasCloseSession();
/**
* .tensorflow.CloseSessionRequest close_session = 5;
*/
org.tensorflow.distruntime.CloseSessionRequest getCloseSession();
/**
* .tensorflow.CloseSessionRequest close_session = 5;
*/
org.tensorflow.distruntime.CloseSessionRequestOrBuilder getCloseSessionOrBuilder();
/**
* .tensorflow.ListDevicesRequest list_devices = 6;
*/
boolean hasListDevices();
/**
* .tensorflow.ListDevicesRequest list_devices = 6;
*/
org.tensorflow.distruntime.ListDevicesRequest getListDevices();
/**
* .tensorflow.ListDevicesRequest list_devices = 6;
*/
org.tensorflow.distruntime.ListDevicesRequestOrBuilder getListDevicesOrBuilder();
/**
* .tensorflow.ResetRequest reset_request = 7;
*/
boolean hasResetRequest();
/**
* .tensorflow.ResetRequest reset_request = 7;
*/
org.tensorflow.distruntime.ResetRequest getResetRequest();
/**
* .tensorflow.ResetRequest reset_request = 7;
*/
org.tensorflow.distruntime.ResetRequestOrBuilder getResetRequestOrBuilder();
/**
* .tensorflow.MakeCallableRequest make_callable = 8;
*/
boolean hasMakeCallable();
/**
* .tensorflow.MakeCallableRequest make_callable = 8;
*/
org.tensorflow.distruntime.MakeCallableRequest getMakeCallable();
/**
* .tensorflow.MakeCallableRequest make_callable = 8;
*/
org.tensorflow.distruntime.MakeCallableRequestOrBuilder getMakeCallableOrBuilder();
/**
* .tensorflow.RunCallableRequest run_callable = 9;
*/
boolean hasRunCallable();
/**
* .tensorflow.RunCallableRequest run_callable = 9;
*/
org.tensorflow.distruntime.RunCallableRequest getRunCallable();
/**
* .tensorflow.RunCallableRequest run_callable = 9;
*/
org.tensorflow.distruntime.RunCallableRequestOrBuilder getRunCallableOrBuilder();
/**
* .tensorflow.ReleaseCallableRequest release_callable = 10;
*/
boolean hasReleaseCallable();
/**
* .tensorflow.ReleaseCallableRequest release_callable = 10;
*/
org.tensorflow.distruntime.ReleaseCallableRequest getReleaseCallable();
/**
* .tensorflow.ReleaseCallableRequest release_callable = 10;
*/
org.tensorflow.distruntime.ReleaseCallableRequestOrBuilder getReleaseCallableOrBuilder();
/**
* .tensorflow.NewReplaySession new_replay_session = 11;
*/
boolean hasNewReplaySession();
/**
* .tensorflow.NewReplaySession new_replay_session = 11;
*/
tensorflow.ReplayLog.NewReplaySession getNewReplaySession();
/**
* .tensorflow.NewReplaySession new_replay_session = 11;
*/
tensorflow.ReplayLog.NewReplaySessionOrBuilder getNewReplaySessionOrBuilder();
/**
* .tensorflow.CreateSessionResponse create_session_response = 21;
*/
boolean hasCreateSessionResponse();
/**
* .tensorflow.CreateSessionResponse create_session_response = 21;
*/
org.tensorflow.distruntime.CreateSessionResponse getCreateSessionResponse();
/**
* .tensorflow.CreateSessionResponse create_session_response = 21;
*/
org.tensorflow.distruntime.CreateSessionResponseOrBuilder getCreateSessionResponseOrBuilder();
/**
* .tensorflow.ExtendSessionResponse extend_session_response = 22;
*/
boolean hasExtendSessionResponse();
/**
* .tensorflow.ExtendSessionResponse extend_session_response = 22;
*/
org.tensorflow.distruntime.ExtendSessionResponse getExtendSessionResponse();
/**
* .tensorflow.ExtendSessionResponse extend_session_response = 22;
*/
org.tensorflow.distruntime.ExtendSessionResponseOrBuilder getExtendSessionResponseOrBuilder();
/**
* .tensorflow.PartialRunSetupResponse partial_run_setup_response = 23;
*/
boolean hasPartialRunSetupResponse();
/**
* .tensorflow.PartialRunSetupResponse partial_run_setup_response = 23;
*/
org.tensorflow.distruntime.PartialRunSetupResponse getPartialRunSetupResponse();
/**
* .tensorflow.PartialRunSetupResponse partial_run_setup_response = 23;
*/
org.tensorflow.distruntime.PartialRunSetupResponseOrBuilder getPartialRunSetupResponseOrBuilder();
/**
* .tensorflow.RunStepResponse run_step_response = 24;
*/
boolean hasRunStepResponse();
/**
* .tensorflow.RunStepResponse run_step_response = 24;
*/
org.tensorflow.distruntime.RunStepResponse getRunStepResponse();
/**
* .tensorflow.RunStepResponse run_step_response = 24;
*/
org.tensorflow.distruntime.RunStepResponseOrBuilder getRunStepResponseOrBuilder();
/**
* .tensorflow.CloseSessionResponse close_session_response = 25;
*/
boolean hasCloseSessionResponse();
/**
* .tensorflow.CloseSessionResponse close_session_response = 25;
*/
org.tensorflow.distruntime.CloseSessionResponse getCloseSessionResponse();
/**
* .tensorflow.CloseSessionResponse close_session_response = 25;
*/
org.tensorflow.distruntime.CloseSessionResponseOrBuilder getCloseSessionResponseOrBuilder();
/**
* .tensorflow.ListDevicesResponse list_devices_response = 26;
*/
boolean hasListDevicesResponse();
/**
* .tensorflow.ListDevicesResponse list_devices_response = 26;
*/
org.tensorflow.distruntime.ListDevicesResponse getListDevicesResponse();
/**
* .tensorflow.ListDevicesResponse list_devices_response = 26;
*/
org.tensorflow.distruntime.ListDevicesResponseOrBuilder getListDevicesResponseOrBuilder();
/**
* .tensorflow.ResetResponse reset_request_response = 27;
*/
boolean hasResetRequestResponse();
/**
* .tensorflow.ResetResponse reset_request_response = 27;
*/
org.tensorflow.distruntime.ResetResponse getResetRequestResponse();
/**
* .tensorflow.ResetResponse reset_request_response = 27;
*/
org.tensorflow.distruntime.ResetResponseOrBuilder getResetRequestResponseOrBuilder();
/**
* .tensorflow.MakeCallableResponse make_callable_response = 28;
*/
boolean hasMakeCallableResponse();
/**
* .tensorflow.MakeCallableResponse make_callable_response = 28;
*/
org.tensorflow.distruntime.MakeCallableResponse getMakeCallableResponse();
/**
* .tensorflow.MakeCallableResponse make_callable_response = 28;
*/
org.tensorflow.distruntime.MakeCallableResponseOrBuilder getMakeCallableResponseOrBuilder();
/**
* .tensorflow.RunCallableResponse run_callable_response = 29;
*/
boolean hasRunCallableResponse();
/**
* .tensorflow.RunCallableResponse run_callable_response = 29;
*/
org.tensorflow.distruntime.RunCallableResponse getRunCallableResponse();
/**
* .tensorflow.RunCallableResponse run_callable_response = 29;
*/
org.tensorflow.distruntime.RunCallableResponseOrBuilder getRunCallableResponseOrBuilder();
/**
* .tensorflow.ReleaseCallableResponse release_callable_response = 30;
*/
boolean hasReleaseCallableResponse();
/**
* .tensorflow.ReleaseCallableResponse release_callable_response = 30;
*/
org.tensorflow.distruntime.ReleaseCallableResponse getReleaseCallableResponse();
/**
* .tensorflow.ReleaseCallableResponse release_callable_response = 30;
*/
org.tensorflow.distruntime.ReleaseCallableResponseOrBuilder getReleaseCallableResponseOrBuilder();
public tensorflow.ReplayLog.ReplayOp.OpCase getOpCase();
public tensorflow.ReplayLog.ReplayOp.ResponseCase getResponseCase();
}
/**
* Protobuf type {@code tensorflow.ReplayOp}
*/
public static final class ReplayOp extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.ReplayOp)
ReplayOpOrBuilder {
private static final long serialVersionUID = 0L;
// Use ReplayOp.newBuilder() to construct.
private ReplayOp(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ReplayOp() {
startTimeUs_ = 0D;
endTimeUs_ = 0D;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ReplayOp(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
org.tensorflow.distruntime.CreateSessionRequest.Builder subBuilder = null;
if (opCase_ == 1) {
subBuilder = ((org.tensorflow.distruntime.CreateSessionRequest) op_).toBuilder();
}
op_ =
input.readMessage(org.tensorflow.distruntime.CreateSessionRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.tensorflow.distruntime.CreateSessionRequest) op_);
op_ = subBuilder.buildPartial();
}
opCase_ = 1;
break;
}
case 18: {
org.tensorflow.distruntime.ExtendSessionRequest.Builder subBuilder = null;
if (opCase_ == 2) {
subBuilder = ((org.tensorflow.distruntime.ExtendSessionRequest) op_).toBuilder();
}
op_ =
input.readMessage(org.tensorflow.distruntime.ExtendSessionRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.tensorflow.distruntime.ExtendSessionRequest) op_);
op_ = subBuilder.buildPartial();
}
opCase_ = 2;
break;
}
case 26: {
org.tensorflow.distruntime.PartialRunSetupRequest.Builder subBuilder = null;
if (opCase_ == 3) {
subBuilder = ((org.tensorflow.distruntime.PartialRunSetupRequest) op_).toBuilder();
}
op_ =
input.readMessage(org.tensorflow.distruntime.PartialRunSetupRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.tensorflow.distruntime.PartialRunSetupRequest) op_);
op_ = subBuilder.buildPartial();
}
opCase_ = 3;
break;
}
case 34: {
org.tensorflow.distruntime.RunStepRequest.Builder subBuilder = null;
if (opCase_ == 4) {
subBuilder = ((org.tensorflow.distruntime.RunStepRequest) op_).toBuilder();
}
op_ =
input.readMessage(org.tensorflow.distruntime.RunStepRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.tensorflow.distruntime.RunStepRequest) op_);
op_ = subBuilder.buildPartial();
}
opCase_ = 4;
break;
}
case 42: {
org.tensorflow.distruntime.CloseSessionRequest.Builder subBuilder = null;
if (opCase_ == 5) {
subBuilder = ((org.tensorflow.distruntime.CloseSessionRequest) op_).toBuilder();
}
op_ =
input.readMessage(org.tensorflow.distruntime.CloseSessionRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.tensorflow.distruntime.CloseSessionRequest) op_);
op_ = subBuilder.buildPartial();
}
opCase_ = 5;
break;
}
case 50: {
org.tensorflow.distruntime.ListDevicesRequest.Builder subBuilder = null;
if (opCase_ == 6) {
subBuilder = ((org.tensorflow.distruntime.ListDevicesRequest) op_).toBuilder();
}
op_ =
input.readMessage(org.tensorflow.distruntime.ListDevicesRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.tensorflow.distruntime.ListDevicesRequest) op_);
op_ = subBuilder.buildPartial();
}
opCase_ = 6;
break;
}
case 58: {
org.tensorflow.distruntime.ResetRequest.Builder subBuilder = null;
if (opCase_ == 7) {
subBuilder = ((org.tensorflow.distruntime.ResetRequest) op_).toBuilder();
}
op_ =
input.readMessage(org.tensorflow.distruntime.ResetRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.tensorflow.distruntime.ResetRequest) op_);
op_ = subBuilder.buildPartial();
}
opCase_ = 7;
break;
}
case 66: {
org.tensorflow.distruntime.MakeCallableRequest.Builder subBuilder = null;
if (opCase_ == 8) {
subBuilder = ((org.tensorflow.distruntime.MakeCallableRequest) op_).toBuilder();
}
op_ =
input.readMessage(org.tensorflow.distruntime.MakeCallableRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.tensorflow.distruntime.MakeCallableRequest) op_);
op_ = subBuilder.buildPartial();
}
opCase_ = 8;
break;
}
case 74: {
org.tensorflow.distruntime.RunCallableRequest.Builder subBuilder = null;
if (opCase_ == 9) {
subBuilder = ((org.tensorflow.distruntime.RunCallableRequest) op_).toBuilder();
}
op_ =
input.readMessage(org.tensorflow.distruntime.RunCallableRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.tensorflow.distruntime.RunCallableRequest) op_);
op_ = subBuilder.buildPartial();
}
opCase_ = 9;
break;
}
case 82: {
org.tensorflow.distruntime.ReleaseCallableRequest.Builder subBuilder = null;
if (opCase_ == 10) {
subBuilder = ((org.tensorflow.distruntime.ReleaseCallableRequest) op_).toBuilder();
}
op_ =
input.readMessage(org.tensorflow.distruntime.ReleaseCallableRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.tensorflow.distruntime.ReleaseCallableRequest) op_);
op_ = subBuilder.buildPartial();
}
opCase_ = 10;
break;
}
case 90: {
tensorflow.ReplayLog.NewReplaySession.Builder subBuilder = null;
if (opCase_ == 11) {
subBuilder = ((tensorflow.ReplayLog.NewReplaySession) op_).toBuilder();
}
op_ =
input.readMessage(tensorflow.ReplayLog.NewReplaySession.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((tensorflow.ReplayLog.NewReplaySession) op_);
op_ = subBuilder.buildPartial();
}
opCase_ = 11;
break;
}
case 170: {
org.tensorflow.distruntime.CreateSessionResponse.Builder subBuilder = null;
if (responseCase_ == 21) {
subBuilder = ((org.tensorflow.distruntime.CreateSessionResponse) response_).toBuilder();
}
response_ =
input.readMessage(org.tensorflow.distruntime.CreateSessionResponse.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.tensorflow.distruntime.CreateSessionResponse) response_);
response_ = subBuilder.buildPartial();
}
responseCase_ = 21;
break;
}
case 178: {
org.tensorflow.distruntime.ExtendSessionResponse.Builder subBuilder = null;
if (responseCase_ == 22) {
subBuilder = ((org.tensorflow.distruntime.ExtendSessionResponse) response_).toBuilder();
}
response_ =
input.readMessage(org.tensorflow.distruntime.ExtendSessionResponse.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.tensorflow.distruntime.ExtendSessionResponse) response_);
response_ = subBuilder.buildPartial();
}
responseCase_ = 22;
break;
}
case 186: {
org.tensorflow.distruntime.PartialRunSetupResponse.Builder subBuilder = null;
if (responseCase_ == 23) {
subBuilder = ((org.tensorflow.distruntime.PartialRunSetupResponse) response_).toBuilder();
}
response_ =
input.readMessage(org.tensorflow.distruntime.PartialRunSetupResponse.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.tensorflow.distruntime.PartialRunSetupResponse) response_);
response_ = subBuilder.buildPartial();
}
responseCase_ = 23;
break;
}
case 194: {
org.tensorflow.distruntime.RunStepResponse.Builder subBuilder = null;
if (responseCase_ == 24) {
subBuilder = ((org.tensorflow.distruntime.RunStepResponse) response_).toBuilder();
}
response_ =
input.readMessage(org.tensorflow.distruntime.RunStepResponse.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.tensorflow.distruntime.RunStepResponse) response_);
response_ = subBuilder.buildPartial();
}
responseCase_ = 24;
break;
}
case 202: {
org.tensorflow.distruntime.CloseSessionResponse.Builder subBuilder = null;
if (responseCase_ == 25) {
subBuilder = ((org.tensorflow.distruntime.CloseSessionResponse) response_).toBuilder();
}
response_ =
input.readMessage(org.tensorflow.distruntime.CloseSessionResponse.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.tensorflow.distruntime.CloseSessionResponse) response_);
response_ = subBuilder.buildPartial();
}
responseCase_ = 25;
break;
}
case 210: {
org.tensorflow.distruntime.ListDevicesResponse.Builder subBuilder = null;
if (responseCase_ == 26) {
subBuilder = ((org.tensorflow.distruntime.ListDevicesResponse) response_).toBuilder();
}
response_ =
input.readMessage(org.tensorflow.distruntime.ListDevicesResponse.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.tensorflow.distruntime.ListDevicesResponse) response_);
response_ = subBuilder.buildPartial();
}
responseCase_ = 26;
break;
}
case 218: {
org.tensorflow.distruntime.ResetResponse.Builder subBuilder = null;
if (responseCase_ == 27) {
subBuilder = ((org.tensorflow.distruntime.ResetResponse) response_).toBuilder();
}
response_ =
input.readMessage(org.tensorflow.distruntime.ResetResponse.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.tensorflow.distruntime.ResetResponse) response_);
response_ = subBuilder.buildPartial();
}
responseCase_ = 27;
break;
}
case 226: {
org.tensorflow.distruntime.MakeCallableResponse.Builder subBuilder = null;
if (responseCase_ == 28) {
subBuilder = ((org.tensorflow.distruntime.MakeCallableResponse) response_).toBuilder();
}
response_ =
input.readMessage(org.tensorflow.distruntime.MakeCallableResponse.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.tensorflow.distruntime.MakeCallableResponse) response_);
response_ = subBuilder.buildPartial();
}
responseCase_ = 28;
break;
}
case 234: {
org.tensorflow.distruntime.RunCallableResponse.Builder subBuilder = null;
if (responseCase_ == 29) {
subBuilder = ((org.tensorflow.distruntime.RunCallableResponse) response_).toBuilder();
}
response_ =
input.readMessage(org.tensorflow.distruntime.RunCallableResponse.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.tensorflow.distruntime.RunCallableResponse) response_);
response_ = subBuilder.buildPartial();
}
responseCase_ = 29;
break;
}
case 242: {
org.tensorflow.distruntime.ReleaseCallableResponse.Builder subBuilder = null;
if (responseCase_ == 30) {
subBuilder = ((org.tensorflow.distruntime.ReleaseCallableResponse) response_).toBuilder();
}
response_ =
input.readMessage(org.tensorflow.distruntime.ReleaseCallableResponse.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.tensorflow.distruntime.ReleaseCallableResponse) response_);
response_ = subBuilder.buildPartial();
}
responseCase_ = 30;
break;
}
case 249: {
startTimeUs_ = input.readDouble();
break;
}
case 257: {
endTimeUs_ = input.readDouble();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.ReplayLog.internal_static_tensorflow_ReplayOp_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.ReplayLog.internal_static_tensorflow_ReplayOp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.ReplayLog.ReplayOp.class, tensorflow.ReplayLog.ReplayOp.Builder.class);
}
private int opCase_ = 0;
private java.lang.Object op_;
public enum OpCase
implements com.google.protobuf.Internal.EnumLite {
CREATE_SESSION(1),
EXTEND_SESSION(2),
PARTIAL_RUN_SETUP(3),
RUN_STEP(4),
CLOSE_SESSION(5),
LIST_DEVICES(6),
RESET_REQUEST(7),
MAKE_CALLABLE(8),
RUN_CALLABLE(9),
RELEASE_CALLABLE(10),
NEW_REPLAY_SESSION(11),
OP_NOT_SET(0);
private final int value;
private OpCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static OpCase valueOf(int value) {
return forNumber(value);
}
public static OpCase forNumber(int value) {
switch (value) {
case 1: return CREATE_SESSION;
case 2: return EXTEND_SESSION;
case 3: return PARTIAL_RUN_SETUP;
case 4: return RUN_STEP;
case 5: return CLOSE_SESSION;
case 6: return LIST_DEVICES;
case 7: return RESET_REQUEST;
case 8: return MAKE_CALLABLE;
case 9: return RUN_CALLABLE;
case 10: return RELEASE_CALLABLE;
case 11: return NEW_REPLAY_SESSION;
case 0: return OP_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public OpCase
getOpCase() {
return OpCase.forNumber(
opCase_);
}
private int responseCase_ = 0;
private java.lang.Object response_;
public enum ResponseCase
implements com.google.protobuf.Internal.EnumLite {
CREATE_SESSION_RESPONSE(21),
EXTEND_SESSION_RESPONSE(22),
PARTIAL_RUN_SETUP_RESPONSE(23),
RUN_STEP_RESPONSE(24),
CLOSE_SESSION_RESPONSE(25),
LIST_DEVICES_RESPONSE(26),
RESET_REQUEST_RESPONSE(27),
MAKE_CALLABLE_RESPONSE(28),
RUN_CALLABLE_RESPONSE(29),
RELEASE_CALLABLE_RESPONSE(30),
RESPONSE_NOT_SET(0);
private final int value;
private ResponseCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ResponseCase valueOf(int value) {
return forNumber(value);
}
public static ResponseCase forNumber(int value) {
switch (value) {
case 21: return CREATE_SESSION_RESPONSE;
case 22: return EXTEND_SESSION_RESPONSE;
case 23: return PARTIAL_RUN_SETUP_RESPONSE;
case 24: return RUN_STEP_RESPONSE;
case 25: return CLOSE_SESSION_RESPONSE;
case 26: return LIST_DEVICES_RESPONSE;
case 27: return RESET_REQUEST_RESPONSE;
case 28: return MAKE_CALLABLE_RESPONSE;
case 29: return RUN_CALLABLE_RESPONSE;
case 30: return RELEASE_CALLABLE_RESPONSE;
case 0: return RESPONSE_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ResponseCase
getResponseCase() {
return ResponseCase.forNumber(
responseCase_);
}
public static final int START_TIME_US_FIELD_NUMBER = 31;
private double startTimeUs_;
/**
* double start_time_us = 31;
*/
public double getStartTimeUs() {
return startTimeUs_;
}
public static final int END_TIME_US_FIELD_NUMBER = 32;
private double endTimeUs_;
/**
* double end_time_us = 32;
*/
public double getEndTimeUs() {
return endTimeUs_;
}
public static final int CREATE_SESSION_FIELD_NUMBER = 1;
/**
* .tensorflow.CreateSessionRequest create_session = 1;
*/
public boolean hasCreateSession() {
return opCase_ == 1;
}
/**
* .tensorflow.CreateSessionRequest create_session = 1;
*/
public org.tensorflow.distruntime.CreateSessionRequest getCreateSession() {
if (opCase_ == 1) {
return (org.tensorflow.distruntime.CreateSessionRequest) op_;
}
return org.tensorflow.distruntime.CreateSessionRequest.getDefaultInstance();
}
/**
* .tensorflow.CreateSessionRequest create_session = 1;
*/
public org.tensorflow.distruntime.CreateSessionRequestOrBuilder getCreateSessionOrBuilder() {
if (opCase_ == 1) {
return (org.tensorflow.distruntime.CreateSessionRequest) op_;
}
return org.tensorflow.distruntime.CreateSessionRequest.getDefaultInstance();
}
public static final int EXTEND_SESSION_FIELD_NUMBER = 2;
/**
* .tensorflow.ExtendSessionRequest extend_session = 2;
*/
public boolean hasExtendSession() {
return opCase_ == 2;
}
/**
* .tensorflow.ExtendSessionRequest extend_session = 2;
*/
public org.tensorflow.distruntime.ExtendSessionRequest getExtendSession() {
if (opCase_ == 2) {
return (org.tensorflow.distruntime.ExtendSessionRequest) op_;
}
return org.tensorflow.distruntime.ExtendSessionRequest.getDefaultInstance();
}
/**
* .tensorflow.ExtendSessionRequest extend_session = 2;
*/
public org.tensorflow.distruntime.ExtendSessionRequestOrBuilder getExtendSessionOrBuilder() {
if (opCase_ == 2) {
return (org.tensorflow.distruntime.ExtendSessionRequest) op_;
}
return org.tensorflow.distruntime.ExtendSessionRequest.getDefaultInstance();
}
public static final int PARTIAL_RUN_SETUP_FIELD_NUMBER = 3;
/**
* .tensorflow.PartialRunSetupRequest partial_run_setup = 3;
*/
public boolean hasPartialRunSetup() {
return opCase_ == 3;
}
/**
* .tensorflow.PartialRunSetupRequest partial_run_setup = 3;
*/
public org.tensorflow.distruntime.PartialRunSetupRequest getPartialRunSetup() {
if (opCase_ == 3) {
return (org.tensorflow.distruntime.PartialRunSetupRequest) op_;
}
return org.tensorflow.distruntime.PartialRunSetupRequest.getDefaultInstance();
}
/**
* .tensorflow.PartialRunSetupRequest partial_run_setup = 3;
*/
public org.tensorflow.distruntime.PartialRunSetupRequestOrBuilder getPartialRunSetupOrBuilder() {
if (opCase_ == 3) {
return (org.tensorflow.distruntime.PartialRunSetupRequest) op_;
}
return org.tensorflow.distruntime.PartialRunSetupRequest.getDefaultInstance();
}
public static final int RUN_STEP_FIELD_NUMBER = 4;
/**
* .tensorflow.RunStepRequest run_step = 4;
*/
public boolean hasRunStep() {
return opCase_ == 4;
}
/**
* .tensorflow.RunStepRequest run_step = 4;
*/
public org.tensorflow.distruntime.RunStepRequest getRunStep() {
if (opCase_ == 4) {
return (org.tensorflow.distruntime.RunStepRequest) op_;
}
return org.tensorflow.distruntime.RunStepRequest.getDefaultInstance();
}
/**
* .tensorflow.RunStepRequest run_step = 4;
*/
public org.tensorflow.distruntime.RunStepRequestOrBuilder getRunStepOrBuilder() {
if (opCase_ == 4) {
return (org.tensorflow.distruntime.RunStepRequest) op_;
}
return org.tensorflow.distruntime.RunStepRequest.getDefaultInstance();
}
public static final int CLOSE_SESSION_FIELD_NUMBER = 5;
/**
* .tensorflow.CloseSessionRequest close_session = 5;
*/
public boolean hasCloseSession() {
return opCase_ == 5;
}
/**
* .tensorflow.CloseSessionRequest close_session = 5;
*/
public org.tensorflow.distruntime.CloseSessionRequest getCloseSession() {
if (opCase_ == 5) {
return (org.tensorflow.distruntime.CloseSessionRequest) op_;
}
return org.tensorflow.distruntime.CloseSessionRequest.getDefaultInstance();
}
/**
* .tensorflow.CloseSessionRequest close_session = 5;
*/
public org.tensorflow.distruntime.CloseSessionRequestOrBuilder getCloseSessionOrBuilder() {
if (opCase_ == 5) {
return (org.tensorflow.distruntime.CloseSessionRequest) op_;
}
return org.tensorflow.distruntime.CloseSessionRequest.getDefaultInstance();
}
public static final int LIST_DEVICES_FIELD_NUMBER = 6;
/**
* .tensorflow.ListDevicesRequest list_devices = 6;
*/
public boolean hasListDevices() {
return opCase_ == 6;
}
/**
* .tensorflow.ListDevicesRequest list_devices = 6;
*/
public org.tensorflow.distruntime.ListDevicesRequest getListDevices() {
if (opCase_ == 6) {
return (org.tensorflow.distruntime.ListDevicesRequest) op_;
}
return org.tensorflow.distruntime.ListDevicesRequest.getDefaultInstance();
}
/**
* .tensorflow.ListDevicesRequest list_devices = 6;
*/
public org.tensorflow.distruntime.ListDevicesRequestOrBuilder getListDevicesOrBuilder() {
if (opCase_ == 6) {
return (org.tensorflow.distruntime.ListDevicesRequest) op_;
}
return org.tensorflow.distruntime.ListDevicesRequest.getDefaultInstance();
}
public static final int RESET_REQUEST_FIELD_NUMBER = 7;
/**
* .tensorflow.ResetRequest reset_request = 7;
*/
public boolean hasResetRequest() {
return opCase_ == 7;
}
/**
* .tensorflow.ResetRequest reset_request = 7;
*/
public org.tensorflow.distruntime.ResetRequest getResetRequest() {
if (opCase_ == 7) {
return (org.tensorflow.distruntime.ResetRequest) op_;
}
return org.tensorflow.distruntime.ResetRequest.getDefaultInstance();
}
/**
* .tensorflow.ResetRequest reset_request = 7;
*/
public org.tensorflow.distruntime.ResetRequestOrBuilder getResetRequestOrBuilder() {
if (opCase_ == 7) {
return (org.tensorflow.distruntime.ResetRequest) op_;
}
return org.tensorflow.distruntime.ResetRequest.getDefaultInstance();
}
public static final int MAKE_CALLABLE_FIELD_NUMBER = 8;
/**
* .tensorflow.MakeCallableRequest make_callable = 8;
*/
public boolean hasMakeCallable() {
return opCase_ == 8;
}
/**
* .tensorflow.MakeCallableRequest make_callable = 8;
*/
public org.tensorflow.distruntime.MakeCallableRequest getMakeCallable() {
if (opCase_ == 8) {
return (org.tensorflow.distruntime.MakeCallableRequest) op_;
}
return org.tensorflow.distruntime.MakeCallableRequest.getDefaultInstance();
}
/**
* .tensorflow.MakeCallableRequest make_callable = 8;
*/
public org.tensorflow.distruntime.MakeCallableRequestOrBuilder getMakeCallableOrBuilder() {
if (opCase_ == 8) {
return (org.tensorflow.distruntime.MakeCallableRequest) op_;
}
return org.tensorflow.distruntime.MakeCallableRequest.getDefaultInstance();
}
public static final int RUN_CALLABLE_FIELD_NUMBER = 9;
/**
* .tensorflow.RunCallableRequest run_callable = 9;
*/
public boolean hasRunCallable() {
return opCase_ == 9;
}
/**
* .tensorflow.RunCallableRequest run_callable = 9;
*/
public org.tensorflow.distruntime.RunCallableRequest getRunCallable() {
if (opCase_ == 9) {
return (org.tensorflow.distruntime.RunCallableRequest) op_;
}
return org.tensorflow.distruntime.RunCallableRequest.getDefaultInstance();
}
/**
* .tensorflow.RunCallableRequest run_callable = 9;
*/
public org.tensorflow.distruntime.RunCallableRequestOrBuilder getRunCallableOrBuilder() {
if (opCase_ == 9) {
return (org.tensorflow.distruntime.RunCallableRequest) op_;
}
return org.tensorflow.distruntime.RunCallableRequest.getDefaultInstance();
}
public static final int RELEASE_CALLABLE_FIELD_NUMBER = 10;
/**
* .tensorflow.ReleaseCallableRequest release_callable = 10;
*/
public boolean hasReleaseCallable() {
return opCase_ == 10;
}
/**
* .tensorflow.ReleaseCallableRequest release_callable = 10;
*/
public org.tensorflow.distruntime.ReleaseCallableRequest getReleaseCallable() {
if (opCase_ == 10) {
return (org.tensorflow.distruntime.ReleaseCallableRequest) op_;
}
return org.tensorflow.distruntime.ReleaseCallableRequest.getDefaultInstance();
}
/**
* .tensorflow.ReleaseCallableRequest release_callable = 10;
*/
public org.tensorflow.distruntime.ReleaseCallableRequestOrBuilder getReleaseCallableOrBuilder() {
if (opCase_ == 10) {
return (org.tensorflow.distruntime.ReleaseCallableRequest) op_;
}
return org.tensorflow.distruntime.ReleaseCallableRequest.getDefaultInstance();
}
public static final int NEW_REPLAY_SESSION_FIELD_NUMBER = 11;
/**
* .tensorflow.NewReplaySession new_replay_session = 11;
*/
public boolean hasNewReplaySession() {
return opCase_ == 11;
}
/**
* .tensorflow.NewReplaySession new_replay_session = 11;
*/
public tensorflow.ReplayLog.NewReplaySession getNewReplaySession() {
if (opCase_ == 11) {
return (tensorflow.ReplayLog.NewReplaySession) op_;
}
return tensorflow.ReplayLog.NewReplaySession.getDefaultInstance();
}
/**
* .tensorflow.NewReplaySession new_replay_session = 11;
*/
public tensorflow.ReplayLog.NewReplaySessionOrBuilder getNewReplaySessionOrBuilder() {
if (opCase_ == 11) {
return (tensorflow.ReplayLog.NewReplaySession) op_;
}
return tensorflow.ReplayLog.NewReplaySession.getDefaultInstance();
}
public static final int CREATE_SESSION_RESPONSE_FIELD_NUMBER = 21;
/**
* .tensorflow.CreateSessionResponse create_session_response = 21;
*/
public boolean hasCreateSessionResponse() {
return responseCase_ == 21;
}
/**
* .tensorflow.CreateSessionResponse create_session_response = 21;
*/
public org.tensorflow.distruntime.CreateSessionResponse getCreateSessionResponse() {
if (responseCase_ == 21) {
return (org.tensorflow.distruntime.CreateSessionResponse) response_;
}
return org.tensorflow.distruntime.CreateSessionResponse.getDefaultInstance();
}
/**
* .tensorflow.CreateSessionResponse create_session_response = 21;
*/
public org.tensorflow.distruntime.CreateSessionResponseOrBuilder getCreateSessionResponseOrBuilder() {
if (responseCase_ == 21) {
return (org.tensorflow.distruntime.CreateSessionResponse) response_;
}
return org.tensorflow.distruntime.CreateSessionResponse.getDefaultInstance();
}
public static final int EXTEND_SESSION_RESPONSE_FIELD_NUMBER = 22;
/**
* .tensorflow.ExtendSessionResponse extend_session_response = 22;
*/
public boolean hasExtendSessionResponse() {
return responseCase_ == 22;
}
/**
* .tensorflow.ExtendSessionResponse extend_session_response = 22;
*/
public org.tensorflow.distruntime.ExtendSessionResponse getExtendSessionResponse() {
if (responseCase_ == 22) {
return (org.tensorflow.distruntime.ExtendSessionResponse) response_;
}
return org.tensorflow.distruntime.ExtendSessionResponse.getDefaultInstance();
}
/**
* .tensorflow.ExtendSessionResponse extend_session_response = 22;
*/
public org.tensorflow.distruntime.ExtendSessionResponseOrBuilder getExtendSessionResponseOrBuilder() {
if (responseCase_ == 22) {
return (org.tensorflow.distruntime.ExtendSessionResponse) response_;
}
return org.tensorflow.distruntime.ExtendSessionResponse.getDefaultInstance();
}
public static final int PARTIAL_RUN_SETUP_RESPONSE_FIELD_NUMBER = 23;
/**
* .tensorflow.PartialRunSetupResponse partial_run_setup_response = 23;
*/
public boolean hasPartialRunSetupResponse() {
return responseCase_ == 23;
}
/**
* .tensorflow.PartialRunSetupResponse partial_run_setup_response = 23;
*/
public org.tensorflow.distruntime.PartialRunSetupResponse getPartialRunSetupResponse() {
if (responseCase_ == 23) {
return (org.tensorflow.distruntime.PartialRunSetupResponse) response_;
}
return org.tensorflow.distruntime.PartialRunSetupResponse.getDefaultInstance();
}
/**
* .tensorflow.PartialRunSetupResponse partial_run_setup_response = 23;
*/
public org.tensorflow.distruntime.PartialRunSetupResponseOrBuilder getPartialRunSetupResponseOrBuilder() {
if (responseCase_ == 23) {
return (org.tensorflow.distruntime.PartialRunSetupResponse) response_;
}
return org.tensorflow.distruntime.PartialRunSetupResponse.getDefaultInstance();
}
public static final int RUN_STEP_RESPONSE_FIELD_NUMBER = 24;
/**
* .tensorflow.RunStepResponse run_step_response = 24;
*/
public boolean hasRunStepResponse() {
return responseCase_ == 24;
}
/**
* .tensorflow.RunStepResponse run_step_response = 24;
*/
public org.tensorflow.distruntime.RunStepResponse getRunStepResponse() {
if (responseCase_ == 24) {
return (org.tensorflow.distruntime.RunStepResponse) response_;
}
return org.tensorflow.distruntime.RunStepResponse.getDefaultInstance();
}
/**
* .tensorflow.RunStepResponse run_step_response = 24;
*/
public org.tensorflow.distruntime.RunStepResponseOrBuilder getRunStepResponseOrBuilder() {
if (responseCase_ == 24) {
return (org.tensorflow.distruntime.RunStepResponse) response_;
}
return org.tensorflow.distruntime.RunStepResponse.getDefaultInstance();
}
public static final int CLOSE_SESSION_RESPONSE_FIELD_NUMBER = 25;
/**
* .tensorflow.CloseSessionResponse close_session_response = 25;
*/
public boolean hasCloseSessionResponse() {
return responseCase_ == 25;
}
/**
* .tensorflow.CloseSessionResponse close_session_response = 25;
*/
public org.tensorflow.distruntime.CloseSessionResponse getCloseSessionResponse() {
if (responseCase_ == 25) {
return (org.tensorflow.distruntime.CloseSessionResponse) response_;
}
return org.tensorflow.distruntime.CloseSessionResponse.getDefaultInstance();
}
/**
* .tensorflow.CloseSessionResponse close_session_response = 25;
*/
public org.tensorflow.distruntime.CloseSessionResponseOrBuilder getCloseSessionResponseOrBuilder() {
if (responseCase_ == 25) {
return (org.tensorflow.distruntime.CloseSessionResponse) response_;
}
return org.tensorflow.distruntime.CloseSessionResponse.getDefaultInstance();
}
public static final int LIST_DEVICES_RESPONSE_FIELD_NUMBER = 26;
/**
* .tensorflow.ListDevicesResponse list_devices_response = 26;
*/
public boolean hasListDevicesResponse() {
return responseCase_ == 26;
}
/**
* .tensorflow.ListDevicesResponse list_devices_response = 26;
*/
public org.tensorflow.distruntime.ListDevicesResponse getListDevicesResponse() {
if (responseCase_ == 26) {
return (org.tensorflow.distruntime.ListDevicesResponse) response_;
}
return org.tensorflow.distruntime.ListDevicesResponse.getDefaultInstance();
}
/**
* .tensorflow.ListDevicesResponse list_devices_response = 26;
*/
public org.tensorflow.distruntime.ListDevicesResponseOrBuilder getListDevicesResponseOrBuilder() {
if (responseCase_ == 26) {
return (org.tensorflow.distruntime.ListDevicesResponse) response_;
}
return org.tensorflow.distruntime.ListDevicesResponse.getDefaultInstance();
}
public static final int RESET_REQUEST_RESPONSE_FIELD_NUMBER = 27;
/**
* .tensorflow.ResetResponse reset_request_response = 27;
*/
public boolean hasResetRequestResponse() {
return responseCase_ == 27;
}
/**
* .tensorflow.ResetResponse reset_request_response = 27;
*/
public org.tensorflow.distruntime.ResetResponse getResetRequestResponse() {
if (responseCase_ == 27) {
return (org.tensorflow.distruntime.ResetResponse) response_;
}
return org.tensorflow.distruntime.ResetResponse.getDefaultInstance();
}
/**
* .tensorflow.ResetResponse reset_request_response = 27;
*/
public org.tensorflow.distruntime.ResetResponseOrBuilder getResetRequestResponseOrBuilder() {
if (responseCase_ == 27) {
return (org.tensorflow.distruntime.ResetResponse) response_;
}
return org.tensorflow.distruntime.ResetResponse.getDefaultInstance();
}
public static final int MAKE_CALLABLE_RESPONSE_FIELD_NUMBER = 28;
/**
* .tensorflow.MakeCallableResponse make_callable_response = 28;
*/
public boolean hasMakeCallableResponse() {
return responseCase_ == 28;
}
/**
* .tensorflow.MakeCallableResponse make_callable_response = 28;
*/
public org.tensorflow.distruntime.MakeCallableResponse getMakeCallableResponse() {
if (responseCase_ == 28) {
return (org.tensorflow.distruntime.MakeCallableResponse) response_;
}
return org.tensorflow.distruntime.MakeCallableResponse.getDefaultInstance();
}
/**
* .tensorflow.MakeCallableResponse make_callable_response = 28;
*/
public org.tensorflow.distruntime.MakeCallableResponseOrBuilder getMakeCallableResponseOrBuilder() {
if (responseCase_ == 28) {
return (org.tensorflow.distruntime.MakeCallableResponse) response_;
}
return org.tensorflow.distruntime.MakeCallableResponse.getDefaultInstance();
}
public static final int RUN_CALLABLE_RESPONSE_FIELD_NUMBER = 29;
/**
* .tensorflow.RunCallableResponse run_callable_response = 29;
*/
public boolean hasRunCallableResponse() {
return responseCase_ == 29;
}
/**
* .tensorflow.RunCallableResponse run_callable_response = 29;
*/
public org.tensorflow.distruntime.RunCallableResponse getRunCallableResponse() {
if (responseCase_ == 29) {
return (org.tensorflow.distruntime.RunCallableResponse) response_;
}
return org.tensorflow.distruntime.RunCallableResponse.getDefaultInstance();
}
/**
* .tensorflow.RunCallableResponse run_callable_response = 29;
*/
public org.tensorflow.distruntime.RunCallableResponseOrBuilder getRunCallableResponseOrBuilder() {
if (responseCase_ == 29) {
return (org.tensorflow.distruntime.RunCallableResponse) response_;
}
return org.tensorflow.distruntime.RunCallableResponse.getDefaultInstance();
}
public static final int RELEASE_CALLABLE_RESPONSE_FIELD_NUMBER = 30;
/**
* .tensorflow.ReleaseCallableResponse release_callable_response = 30;
*/
public boolean hasReleaseCallableResponse() {
return responseCase_ == 30;
}
/**
* .tensorflow.ReleaseCallableResponse release_callable_response = 30;
*/
public org.tensorflow.distruntime.ReleaseCallableResponse getReleaseCallableResponse() {
if (responseCase_ == 30) {
return (org.tensorflow.distruntime.ReleaseCallableResponse) response_;
}
return org.tensorflow.distruntime.ReleaseCallableResponse.getDefaultInstance();
}
/**
* .tensorflow.ReleaseCallableResponse release_callable_response = 30;
*/
public org.tensorflow.distruntime.ReleaseCallableResponseOrBuilder getReleaseCallableResponseOrBuilder() {
if (responseCase_ == 30) {
return (org.tensorflow.distruntime.ReleaseCallableResponse) response_;
}
return org.tensorflow.distruntime.ReleaseCallableResponse.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (opCase_ == 1) {
output.writeMessage(1, (org.tensorflow.distruntime.CreateSessionRequest) op_);
}
if (opCase_ == 2) {
output.writeMessage(2, (org.tensorflow.distruntime.ExtendSessionRequest) op_);
}
if (opCase_ == 3) {
output.writeMessage(3, (org.tensorflow.distruntime.PartialRunSetupRequest) op_);
}
if (opCase_ == 4) {
output.writeMessage(4, (org.tensorflow.distruntime.RunStepRequest) op_);
}
if (opCase_ == 5) {
output.writeMessage(5, (org.tensorflow.distruntime.CloseSessionRequest) op_);
}
if (opCase_ == 6) {
output.writeMessage(6, (org.tensorflow.distruntime.ListDevicesRequest) op_);
}
if (opCase_ == 7) {
output.writeMessage(7, (org.tensorflow.distruntime.ResetRequest) op_);
}
if (opCase_ == 8) {
output.writeMessage(8, (org.tensorflow.distruntime.MakeCallableRequest) op_);
}
if (opCase_ == 9) {
output.writeMessage(9, (org.tensorflow.distruntime.RunCallableRequest) op_);
}
if (opCase_ == 10) {
output.writeMessage(10, (org.tensorflow.distruntime.ReleaseCallableRequest) op_);
}
if (opCase_ == 11) {
output.writeMessage(11, (tensorflow.ReplayLog.NewReplaySession) op_);
}
if (responseCase_ == 21) {
output.writeMessage(21, (org.tensorflow.distruntime.CreateSessionResponse) response_);
}
if (responseCase_ == 22) {
output.writeMessage(22, (org.tensorflow.distruntime.ExtendSessionResponse) response_);
}
if (responseCase_ == 23) {
output.writeMessage(23, (org.tensorflow.distruntime.PartialRunSetupResponse) response_);
}
if (responseCase_ == 24) {
output.writeMessage(24, (org.tensorflow.distruntime.RunStepResponse) response_);
}
if (responseCase_ == 25) {
output.writeMessage(25, (org.tensorflow.distruntime.CloseSessionResponse) response_);
}
if (responseCase_ == 26) {
output.writeMessage(26, (org.tensorflow.distruntime.ListDevicesResponse) response_);
}
if (responseCase_ == 27) {
output.writeMessage(27, (org.tensorflow.distruntime.ResetResponse) response_);
}
if (responseCase_ == 28) {
output.writeMessage(28, (org.tensorflow.distruntime.MakeCallableResponse) response_);
}
if (responseCase_ == 29) {
output.writeMessage(29, (org.tensorflow.distruntime.RunCallableResponse) response_);
}
if (responseCase_ == 30) {
output.writeMessage(30, (org.tensorflow.distruntime.ReleaseCallableResponse) response_);
}
if (startTimeUs_ != 0D) {
output.writeDouble(31, startTimeUs_);
}
if (endTimeUs_ != 0D) {
output.writeDouble(32, endTimeUs_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (opCase_ == 1) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, (org.tensorflow.distruntime.CreateSessionRequest) op_);
}
if (opCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, (org.tensorflow.distruntime.ExtendSessionRequest) op_);
}
if (opCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, (org.tensorflow.distruntime.PartialRunSetupRequest) op_);
}
if (opCase_ == 4) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, (org.tensorflow.distruntime.RunStepRequest) op_);
}
if (opCase_ == 5) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, (org.tensorflow.distruntime.CloseSessionRequest) op_);
}
if (opCase_ == 6) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, (org.tensorflow.distruntime.ListDevicesRequest) op_);
}
if (opCase_ == 7) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, (org.tensorflow.distruntime.ResetRequest) op_);
}
if (opCase_ == 8) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, (org.tensorflow.distruntime.MakeCallableRequest) op_);
}
if (opCase_ == 9) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, (org.tensorflow.distruntime.RunCallableRequest) op_);
}
if (opCase_ == 10) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, (org.tensorflow.distruntime.ReleaseCallableRequest) op_);
}
if (opCase_ == 11) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, (tensorflow.ReplayLog.NewReplaySession) op_);
}
if (responseCase_ == 21) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(21, (org.tensorflow.distruntime.CreateSessionResponse) response_);
}
if (responseCase_ == 22) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(22, (org.tensorflow.distruntime.ExtendSessionResponse) response_);
}
if (responseCase_ == 23) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(23, (org.tensorflow.distruntime.PartialRunSetupResponse) response_);
}
if (responseCase_ == 24) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(24, (org.tensorflow.distruntime.RunStepResponse) response_);
}
if (responseCase_ == 25) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(25, (org.tensorflow.distruntime.CloseSessionResponse) response_);
}
if (responseCase_ == 26) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(26, (org.tensorflow.distruntime.ListDevicesResponse) response_);
}
if (responseCase_ == 27) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(27, (org.tensorflow.distruntime.ResetResponse) response_);
}
if (responseCase_ == 28) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(28, (org.tensorflow.distruntime.MakeCallableResponse) response_);
}
if (responseCase_ == 29) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(29, (org.tensorflow.distruntime.RunCallableResponse) response_);
}
if (responseCase_ == 30) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(30, (org.tensorflow.distruntime.ReleaseCallableResponse) response_);
}
if (startTimeUs_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(31, startTimeUs_);
}
if (endTimeUs_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(32, endTimeUs_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.ReplayLog.ReplayOp)) {
return super.equals(obj);
}
tensorflow.ReplayLog.ReplayOp other = (tensorflow.ReplayLog.ReplayOp) obj;
boolean result = true;
result = result && (
java.lang.Double.doubleToLongBits(getStartTimeUs())
== java.lang.Double.doubleToLongBits(
other.getStartTimeUs()));
result = result && (
java.lang.Double.doubleToLongBits(getEndTimeUs())
== java.lang.Double.doubleToLongBits(
other.getEndTimeUs()));
result = result && getOpCase().equals(
other.getOpCase());
if (!result) return false;
switch (opCase_) {
case 1:
result = result && getCreateSession()
.equals(other.getCreateSession());
break;
case 2:
result = result && getExtendSession()
.equals(other.getExtendSession());
break;
case 3:
result = result && getPartialRunSetup()
.equals(other.getPartialRunSetup());
break;
case 4:
result = result && getRunStep()
.equals(other.getRunStep());
break;
case 5:
result = result && getCloseSession()
.equals(other.getCloseSession());
break;
case 6:
result = result && getListDevices()
.equals(other.getListDevices());
break;
case 7:
result = result && getResetRequest()
.equals(other.getResetRequest());
break;
case 8:
result = result && getMakeCallable()
.equals(other.getMakeCallable());
break;
case 9:
result = result && getRunCallable()
.equals(other.getRunCallable());
break;
case 10:
result = result && getReleaseCallable()
.equals(other.getReleaseCallable());
break;
case 11:
result = result && getNewReplaySession()
.equals(other.getNewReplaySession());
break;
case 0:
default:
}
result = result && getResponseCase().equals(
other.getResponseCase());
if (!result) return false;
switch (responseCase_) {
case 21:
result = result && getCreateSessionResponse()
.equals(other.getCreateSessionResponse());
break;
case 22:
result = result && getExtendSessionResponse()
.equals(other.getExtendSessionResponse());
break;
case 23:
result = result && getPartialRunSetupResponse()
.equals(other.getPartialRunSetupResponse());
break;
case 24:
result = result && getRunStepResponse()
.equals(other.getRunStepResponse());
break;
case 25:
result = result && getCloseSessionResponse()
.equals(other.getCloseSessionResponse());
break;
case 26:
result = result && getListDevicesResponse()
.equals(other.getListDevicesResponse());
break;
case 27:
result = result && getResetRequestResponse()
.equals(other.getResetRequestResponse());
break;
case 28:
result = result && getMakeCallableResponse()
.equals(other.getMakeCallableResponse());
break;
case 29:
result = result && getRunCallableResponse()
.equals(other.getRunCallableResponse());
break;
case 30:
result = result && getReleaseCallableResponse()
.equals(other.getReleaseCallableResponse());
break;
case 0:
default:
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + START_TIME_US_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getStartTimeUs()));
hash = (37 * hash) + END_TIME_US_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getEndTimeUs()));
switch (opCase_) {
case 1:
hash = (37 * hash) + CREATE_SESSION_FIELD_NUMBER;
hash = (53 * hash) + getCreateSession().hashCode();
break;
case 2:
hash = (37 * hash) + EXTEND_SESSION_FIELD_NUMBER;
hash = (53 * hash) + getExtendSession().hashCode();
break;
case 3:
hash = (37 * hash) + PARTIAL_RUN_SETUP_FIELD_NUMBER;
hash = (53 * hash) + getPartialRunSetup().hashCode();
break;
case 4:
hash = (37 * hash) + RUN_STEP_FIELD_NUMBER;
hash = (53 * hash) + getRunStep().hashCode();
break;
case 5:
hash = (37 * hash) + CLOSE_SESSION_FIELD_NUMBER;
hash = (53 * hash) + getCloseSession().hashCode();
break;
case 6:
hash = (37 * hash) + LIST_DEVICES_FIELD_NUMBER;
hash = (53 * hash) + getListDevices().hashCode();
break;
case 7:
hash = (37 * hash) + RESET_REQUEST_FIELD_NUMBER;
hash = (53 * hash) + getResetRequest().hashCode();
break;
case 8:
hash = (37 * hash) + MAKE_CALLABLE_FIELD_NUMBER;
hash = (53 * hash) + getMakeCallable().hashCode();
break;
case 9:
hash = (37 * hash) + RUN_CALLABLE_FIELD_NUMBER;
hash = (53 * hash) + getRunCallable().hashCode();
break;
case 10:
hash = (37 * hash) + RELEASE_CALLABLE_FIELD_NUMBER;
hash = (53 * hash) + getReleaseCallable().hashCode();
break;
case 11:
hash = (37 * hash) + NEW_REPLAY_SESSION_FIELD_NUMBER;
hash = (53 * hash) + getNewReplaySession().hashCode();
break;
case 0:
default:
}
switch (responseCase_) {
case 21:
hash = (37 * hash) + CREATE_SESSION_RESPONSE_FIELD_NUMBER;
hash = (53 * hash) + getCreateSessionResponse().hashCode();
break;
case 22:
hash = (37 * hash) + EXTEND_SESSION_RESPONSE_FIELD_NUMBER;
hash = (53 * hash) + getExtendSessionResponse().hashCode();
break;
case 23:
hash = (37 * hash) + PARTIAL_RUN_SETUP_RESPONSE_FIELD_NUMBER;
hash = (53 * hash) + getPartialRunSetupResponse().hashCode();
break;
case 24:
hash = (37 * hash) + RUN_STEP_RESPONSE_FIELD_NUMBER;
hash = (53 * hash) + getRunStepResponse().hashCode();
break;
case 25:
hash = (37 * hash) + CLOSE_SESSION_RESPONSE_FIELD_NUMBER;
hash = (53 * hash) + getCloseSessionResponse().hashCode();
break;
case 26:
hash = (37 * hash) + LIST_DEVICES_RESPONSE_FIELD_NUMBER;
hash = (53 * hash) + getListDevicesResponse().hashCode();
break;
case 27:
hash = (37 * hash) + RESET_REQUEST_RESPONSE_FIELD_NUMBER;
hash = (53 * hash) + getResetRequestResponse().hashCode();
break;
case 28:
hash = (37 * hash) + MAKE_CALLABLE_RESPONSE_FIELD_NUMBER;
hash = (53 * hash) + getMakeCallableResponse().hashCode();
break;
case 29:
hash = (37 * hash) + RUN_CALLABLE_RESPONSE_FIELD_NUMBER;
hash = (53 * hash) + getRunCallableResponse().hashCode();
break;
case 30:
hash = (37 * hash) + RELEASE_CALLABLE_RESPONSE_FIELD_NUMBER;
hash = (53 * hash) + getReleaseCallableResponse().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.ReplayLog.ReplayOp parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.ReplayLog.ReplayOp parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.ReplayLog.ReplayOp parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.ReplayLog.ReplayOp parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.ReplayLog.ReplayOp parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.ReplayLog.ReplayOp parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.ReplayLog.ReplayOp parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.ReplayLog.ReplayOp parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.ReplayLog.ReplayOp parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.ReplayLog.ReplayOp parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.ReplayLog.ReplayOp parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.ReplayLog.ReplayOp parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.ReplayLog.ReplayOp prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.ReplayOp}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.ReplayOp)
tensorflow.ReplayLog.ReplayOpOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.ReplayLog.internal_static_tensorflow_ReplayOp_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.ReplayLog.internal_static_tensorflow_ReplayOp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.ReplayLog.ReplayOp.class, tensorflow.ReplayLog.ReplayOp.Builder.class);
}
// Construct using tensorflow.ReplayLog.ReplayOp.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
startTimeUs_ = 0D;
endTimeUs_ = 0D;
opCase_ = 0;
op_ = null;
responseCase_ = 0;
response_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.ReplayLog.internal_static_tensorflow_ReplayOp_descriptor;
}
@java.lang.Override
public tensorflow.ReplayLog.ReplayOp getDefaultInstanceForType() {
return tensorflow.ReplayLog.ReplayOp.getDefaultInstance();
}
@java.lang.Override
public tensorflow.ReplayLog.ReplayOp build() {
tensorflow.ReplayLog.ReplayOp result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.ReplayLog.ReplayOp buildPartial() {
tensorflow.ReplayLog.ReplayOp result = new tensorflow.ReplayLog.ReplayOp(this);
result.startTimeUs_ = startTimeUs_;
result.endTimeUs_ = endTimeUs_;
if (opCase_ == 1) {
if (createSessionBuilder_ == null) {
result.op_ = op_;
} else {
result.op_ = createSessionBuilder_.build();
}
}
if (opCase_ == 2) {
if (extendSessionBuilder_ == null) {
result.op_ = op_;
} else {
result.op_ = extendSessionBuilder_.build();
}
}
if (opCase_ == 3) {
if (partialRunSetupBuilder_ == null) {
result.op_ = op_;
} else {
result.op_ = partialRunSetupBuilder_.build();
}
}
if (opCase_ == 4) {
if (runStepBuilder_ == null) {
result.op_ = op_;
} else {
result.op_ = runStepBuilder_.build();
}
}
if (opCase_ == 5) {
if (closeSessionBuilder_ == null) {
result.op_ = op_;
} else {
result.op_ = closeSessionBuilder_.build();
}
}
if (opCase_ == 6) {
if (listDevicesBuilder_ == null) {
result.op_ = op_;
} else {
result.op_ = listDevicesBuilder_.build();
}
}
if (opCase_ == 7) {
if (resetRequestBuilder_ == null) {
result.op_ = op_;
} else {
result.op_ = resetRequestBuilder_.build();
}
}
if (opCase_ == 8) {
if (makeCallableBuilder_ == null) {
result.op_ = op_;
} else {
result.op_ = makeCallableBuilder_.build();
}
}
if (opCase_ == 9) {
if (runCallableBuilder_ == null) {
result.op_ = op_;
} else {
result.op_ = runCallableBuilder_.build();
}
}
if (opCase_ == 10) {
if (releaseCallableBuilder_ == null) {
result.op_ = op_;
} else {
result.op_ = releaseCallableBuilder_.build();
}
}
if (opCase_ == 11) {
if (newReplaySessionBuilder_ == null) {
result.op_ = op_;
} else {
result.op_ = newReplaySessionBuilder_.build();
}
}
if (responseCase_ == 21) {
if (createSessionResponseBuilder_ == null) {
result.response_ = response_;
} else {
result.response_ = createSessionResponseBuilder_.build();
}
}
if (responseCase_ == 22) {
if (extendSessionResponseBuilder_ == null) {
result.response_ = response_;
} else {
result.response_ = extendSessionResponseBuilder_.build();
}
}
if (responseCase_ == 23) {
if (partialRunSetupResponseBuilder_ == null) {
result.response_ = response_;
} else {
result.response_ = partialRunSetupResponseBuilder_.build();
}
}
if (responseCase_ == 24) {
if (runStepResponseBuilder_ == null) {
result.response_ = response_;
} else {
result.response_ = runStepResponseBuilder_.build();
}
}
if (responseCase_ == 25) {
if (closeSessionResponseBuilder_ == null) {
result.response_ = response_;
} else {
result.response_ = closeSessionResponseBuilder_.build();
}
}
if (responseCase_ == 26) {
if (listDevicesResponseBuilder_ == null) {
result.response_ = response_;
} else {
result.response_ = listDevicesResponseBuilder_.build();
}
}
if (responseCase_ == 27) {
if (resetRequestResponseBuilder_ == null) {
result.response_ = response_;
} else {
result.response_ = resetRequestResponseBuilder_.build();
}
}
if (responseCase_ == 28) {
if (makeCallableResponseBuilder_ == null) {
result.response_ = response_;
} else {
result.response_ = makeCallableResponseBuilder_.build();
}
}
if (responseCase_ == 29) {
if (runCallableResponseBuilder_ == null) {
result.response_ = response_;
} else {
result.response_ = runCallableResponseBuilder_.build();
}
}
if (responseCase_ == 30) {
if (releaseCallableResponseBuilder_ == null) {
result.response_ = response_;
} else {
result.response_ = releaseCallableResponseBuilder_.build();
}
}
result.opCase_ = opCase_;
result.responseCase_ = responseCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.ReplayLog.ReplayOp) {
return mergeFrom((tensorflow.ReplayLog.ReplayOp)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.ReplayLog.ReplayOp other) {
if (other == tensorflow.ReplayLog.ReplayOp.getDefaultInstance()) return this;
if (other.getStartTimeUs() != 0D) {
setStartTimeUs(other.getStartTimeUs());
}
if (other.getEndTimeUs() != 0D) {
setEndTimeUs(other.getEndTimeUs());
}
switch (other.getOpCase()) {
case CREATE_SESSION: {
mergeCreateSession(other.getCreateSession());
break;
}
case EXTEND_SESSION: {
mergeExtendSession(other.getExtendSession());
break;
}
case PARTIAL_RUN_SETUP: {
mergePartialRunSetup(other.getPartialRunSetup());
break;
}
case RUN_STEP: {
mergeRunStep(other.getRunStep());
break;
}
case CLOSE_SESSION: {
mergeCloseSession(other.getCloseSession());
break;
}
case LIST_DEVICES: {
mergeListDevices(other.getListDevices());
break;
}
case RESET_REQUEST: {
mergeResetRequest(other.getResetRequest());
break;
}
case MAKE_CALLABLE: {
mergeMakeCallable(other.getMakeCallable());
break;
}
case RUN_CALLABLE: {
mergeRunCallable(other.getRunCallable());
break;
}
case RELEASE_CALLABLE: {
mergeReleaseCallable(other.getReleaseCallable());
break;
}
case NEW_REPLAY_SESSION: {
mergeNewReplaySession(other.getNewReplaySession());
break;
}
case OP_NOT_SET: {
break;
}
}
switch (other.getResponseCase()) {
case CREATE_SESSION_RESPONSE: {
mergeCreateSessionResponse(other.getCreateSessionResponse());
break;
}
case EXTEND_SESSION_RESPONSE: {
mergeExtendSessionResponse(other.getExtendSessionResponse());
break;
}
case PARTIAL_RUN_SETUP_RESPONSE: {
mergePartialRunSetupResponse(other.getPartialRunSetupResponse());
break;
}
case RUN_STEP_RESPONSE: {
mergeRunStepResponse(other.getRunStepResponse());
break;
}
case CLOSE_SESSION_RESPONSE: {
mergeCloseSessionResponse(other.getCloseSessionResponse());
break;
}
case LIST_DEVICES_RESPONSE: {
mergeListDevicesResponse(other.getListDevicesResponse());
break;
}
case RESET_REQUEST_RESPONSE: {
mergeResetRequestResponse(other.getResetRequestResponse());
break;
}
case MAKE_CALLABLE_RESPONSE: {
mergeMakeCallableResponse(other.getMakeCallableResponse());
break;
}
case RUN_CALLABLE_RESPONSE: {
mergeRunCallableResponse(other.getRunCallableResponse());
break;
}
case RELEASE_CALLABLE_RESPONSE: {
mergeReleaseCallableResponse(other.getReleaseCallableResponse());
break;
}
case RESPONSE_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.ReplayLog.ReplayOp parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.ReplayLog.ReplayOp) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int opCase_ = 0;
private java.lang.Object op_;
public OpCase
getOpCase() {
return OpCase.forNumber(
opCase_);
}
public Builder clearOp() {
opCase_ = 0;
op_ = null;
onChanged();
return this;
}
private int responseCase_ = 0;
private java.lang.Object response_;
public ResponseCase
getResponseCase() {
return ResponseCase.forNumber(
responseCase_);
}
public Builder clearResponse() {
responseCase_ = 0;
response_ = null;
onChanged();
return this;
}
private double startTimeUs_ ;
/**
* double start_time_us = 31;
*/
public double getStartTimeUs() {
return startTimeUs_;
}
/**
* double start_time_us = 31;
*/
public Builder setStartTimeUs(double value) {
startTimeUs_ = value;
onChanged();
return this;
}
/**
* double start_time_us = 31;
*/
public Builder clearStartTimeUs() {
startTimeUs_ = 0D;
onChanged();
return this;
}
private double endTimeUs_ ;
/**
* double end_time_us = 32;
*/
public double getEndTimeUs() {
return endTimeUs_;
}
/**
* double end_time_us = 32;
*/
public Builder setEndTimeUs(double value) {
endTimeUs_ = value;
onChanged();
return this;
}
/**
* double end_time_us = 32;
*/
public Builder clearEndTimeUs() {
endTimeUs_ = 0D;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.CreateSessionRequest, org.tensorflow.distruntime.CreateSessionRequest.Builder, org.tensorflow.distruntime.CreateSessionRequestOrBuilder> createSessionBuilder_;
/**
* .tensorflow.CreateSessionRequest create_session = 1;
*/
public boolean hasCreateSession() {
return opCase_ == 1;
}
/**
* .tensorflow.CreateSessionRequest create_session = 1;
*/
public org.tensorflow.distruntime.CreateSessionRequest getCreateSession() {
if (createSessionBuilder_ == null) {
if (opCase_ == 1) {
return (org.tensorflow.distruntime.CreateSessionRequest) op_;
}
return org.tensorflow.distruntime.CreateSessionRequest.getDefaultInstance();
} else {
if (opCase_ == 1) {
return createSessionBuilder_.getMessage();
}
return org.tensorflow.distruntime.CreateSessionRequest.getDefaultInstance();
}
}
/**
* .tensorflow.CreateSessionRequest create_session = 1;
*/
public Builder setCreateSession(org.tensorflow.distruntime.CreateSessionRequest value) {
if (createSessionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
op_ = value;
onChanged();
} else {
createSessionBuilder_.setMessage(value);
}
opCase_ = 1;
return this;
}
/**
* .tensorflow.CreateSessionRequest create_session = 1;
*/
public Builder setCreateSession(
org.tensorflow.distruntime.CreateSessionRequest.Builder builderForValue) {
if (createSessionBuilder_ == null) {
op_ = builderForValue.build();
onChanged();
} else {
createSessionBuilder_.setMessage(builderForValue.build());
}
opCase_ = 1;
return this;
}
/**
* .tensorflow.CreateSessionRequest create_session = 1;
*/
public Builder mergeCreateSession(org.tensorflow.distruntime.CreateSessionRequest value) {
if (createSessionBuilder_ == null) {
if (opCase_ == 1 &&
op_ != org.tensorflow.distruntime.CreateSessionRequest.getDefaultInstance()) {
op_ = org.tensorflow.distruntime.CreateSessionRequest.newBuilder((org.tensorflow.distruntime.CreateSessionRequest) op_)
.mergeFrom(value).buildPartial();
} else {
op_ = value;
}
onChanged();
} else {
if (opCase_ == 1) {
createSessionBuilder_.mergeFrom(value);
}
createSessionBuilder_.setMessage(value);
}
opCase_ = 1;
return this;
}
/**
* .tensorflow.CreateSessionRequest create_session = 1;
*/
public Builder clearCreateSession() {
if (createSessionBuilder_ == null) {
if (opCase_ == 1) {
opCase_ = 0;
op_ = null;
onChanged();
}
} else {
if (opCase_ == 1) {
opCase_ = 0;
op_ = null;
}
createSessionBuilder_.clear();
}
return this;
}
/**
* .tensorflow.CreateSessionRequest create_session = 1;
*/
public org.tensorflow.distruntime.CreateSessionRequest.Builder getCreateSessionBuilder() {
return getCreateSessionFieldBuilder().getBuilder();
}
/**
* .tensorflow.CreateSessionRequest create_session = 1;
*/
public org.tensorflow.distruntime.CreateSessionRequestOrBuilder getCreateSessionOrBuilder() {
if ((opCase_ == 1) && (createSessionBuilder_ != null)) {
return createSessionBuilder_.getMessageOrBuilder();
} else {
if (opCase_ == 1) {
return (org.tensorflow.distruntime.CreateSessionRequest) op_;
}
return org.tensorflow.distruntime.CreateSessionRequest.getDefaultInstance();
}
}
/**
* .tensorflow.CreateSessionRequest create_session = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.CreateSessionRequest, org.tensorflow.distruntime.CreateSessionRequest.Builder, org.tensorflow.distruntime.CreateSessionRequestOrBuilder>
getCreateSessionFieldBuilder() {
if (createSessionBuilder_ == null) {
if (!(opCase_ == 1)) {
op_ = org.tensorflow.distruntime.CreateSessionRequest.getDefaultInstance();
}
createSessionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.CreateSessionRequest, org.tensorflow.distruntime.CreateSessionRequest.Builder, org.tensorflow.distruntime.CreateSessionRequestOrBuilder>(
(org.tensorflow.distruntime.CreateSessionRequest) op_,
getParentForChildren(),
isClean());
op_ = null;
}
opCase_ = 1;
onChanged();;
return createSessionBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ExtendSessionRequest, org.tensorflow.distruntime.ExtendSessionRequest.Builder, org.tensorflow.distruntime.ExtendSessionRequestOrBuilder> extendSessionBuilder_;
/**
* .tensorflow.ExtendSessionRequest extend_session = 2;
*/
public boolean hasExtendSession() {
return opCase_ == 2;
}
/**
* .tensorflow.ExtendSessionRequest extend_session = 2;
*/
public org.tensorflow.distruntime.ExtendSessionRequest getExtendSession() {
if (extendSessionBuilder_ == null) {
if (opCase_ == 2) {
return (org.tensorflow.distruntime.ExtendSessionRequest) op_;
}
return org.tensorflow.distruntime.ExtendSessionRequest.getDefaultInstance();
} else {
if (opCase_ == 2) {
return extendSessionBuilder_.getMessage();
}
return org.tensorflow.distruntime.ExtendSessionRequest.getDefaultInstance();
}
}
/**
* .tensorflow.ExtendSessionRequest extend_session = 2;
*/
public Builder setExtendSession(org.tensorflow.distruntime.ExtendSessionRequest value) {
if (extendSessionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
op_ = value;
onChanged();
} else {
extendSessionBuilder_.setMessage(value);
}
opCase_ = 2;
return this;
}
/**
* .tensorflow.ExtendSessionRequest extend_session = 2;
*/
public Builder setExtendSession(
org.tensorflow.distruntime.ExtendSessionRequest.Builder builderForValue) {
if (extendSessionBuilder_ == null) {
op_ = builderForValue.build();
onChanged();
} else {
extendSessionBuilder_.setMessage(builderForValue.build());
}
opCase_ = 2;
return this;
}
/**
* .tensorflow.ExtendSessionRequest extend_session = 2;
*/
public Builder mergeExtendSession(org.tensorflow.distruntime.ExtendSessionRequest value) {
if (extendSessionBuilder_ == null) {
if (opCase_ == 2 &&
op_ != org.tensorflow.distruntime.ExtendSessionRequest.getDefaultInstance()) {
op_ = org.tensorflow.distruntime.ExtendSessionRequest.newBuilder((org.tensorflow.distruntime.ExtendSessionRequest) op_)
.mergeFrom(value).buildPartial();
} else {
op_ = value;
}
onChanged();
} else {
if (opCase_ == 2) {
extendSessionBuilder_.mergeFrom(value);
}
extendSessionBuilder_.setMessage(value);
}
opCase_ = 2;
return this;
}
/**
* .tensorflow.ExtendSessionRequest extend_session = 2;
*/
public Builder clearExtendSession() {
if (extendSessionBuilder_ == null) {
if (opCase_ == 2) {
opCase_ = 0;
op_ = null;
onChanged();
}
} else {
if (opCase_ == 2) {
opCase_ = 0;
op_ = null;
}
extendSessionBuilder_.clear();
}
return this;
}
/**
* .tensorflow.ExtendSessionRequest extend_session = 2;
*/
public org.tensorflow.distruntime.ExtendSessionRequest.Builder getExtendSessionBuilder() {
return getExtendSessionFieldBuilder().getBuilder();
}
/**
* .tensorflow.ExtendSessionRequest extend_session = 2;
*/
public org.tensorflow.distruntime.ExtendSessionRequestOrBuilder getExtendSessionOrBuilder() {
if ((opCase_ == 2) && (extendSessionBuilder_ != null)) {
return extendSessionBuilder_.getMessageOrBuilder();
} else {
if (opCase_ == 2) {
return (org.tensorflow.distruntime.ExtendSessionRequest) op_;
}
return org.tensorflow.distruntime.ExtendSessionRequest.getDefaultInstance();
}
}
/**
* .tensorflow.ExtendSessionRequest extend_session = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ExtendSessionRequest, org.tensorflow.distruntime.ExtendSessionRequest.Builder, org.tensorflow.distruntime.ExtendSessionRequestOrBuilder>
getExtendSessionFieldBuilder() {
if (extendSessionBuilder_ == null) {
if (!(opCase_ == 2)) {
op_ = org.tensorflow.distruntime.ExtendSessionRequest.getDefaultInstance();
}
extendSessionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ExtendSessionRequest, org.tensorflow.distruntime.ExtendSessionRequest.Builder, org.tensorflow.distruntime.ExtendSessionRequestOrBuilder>(
(org.tensorflow.distruntime.ExtendSessionRequest) op_,
getParentForChildren(),
isClean());
op_ = null;
}
opCase_ = 2;
onChanged();;
return extendSessionBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.PartialRunSetupRequest, org.tensorflow.distruntime.PartialRunSetupRequest.Builder, org.tensorflow.distruntime.PartialRunSetupRequestOrBuilder> partialRunSetupBuilder_;
/**
* .tensorflow.PartialRunSetupRequest partial_run_setup = 3;
*/
public boolean hasPartialRunSetup() {
return opCase_ == 3;
}
/**
* .tensorflow.PartialRunSetupRequest partial_run_setup = 3;
*/
public org.tensorflow.distruntime.PartialRunSetupRequest getPartialRunSetup() {
if (partialRunSetupBuilder_ == null) {
if (opCase_ == 3) {
return (org.tensorflow.distruntime.PartialRunSetupRequest) op_;
}
return org.tensorflow.distruntime.PartialRunSetupRequest.getDefaultInstance();
} else {
if (opCase_ == 3) {
return partialRunSetupBuilder_.getMessage();
}
return org.tensorflow.distruntime.PartialRunSetupRequest.getDefaultInstance();
}
}
/**
* .tensorflow.PartialRunSetupRequest partial_run_setup = 3;
*/
public Builder setPartialRunSetup(org.tensorflow.distruntime.PartialRunSetupRequest value) {
if (partialRunSetupBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
op_ = value;
onChanged();
} else {
partialRunSetupBuilder_.setMessage(value);
}
opCase_ = 3;
return this;
}
/**
* .tensorflow.PartialRunSetupRequest partial_run_setup = 3;
*/
public Builder setPartialRunSetup(
org.tensorflow.distruntime.PartialRunSetupRequest.Builder builderForValue) {
if (partialRunSetupBuilder_ == null) {
op_ = builderForValue.build();
onChanged();
} else {
partialRunSetupBuilder_.setMessage(builderForValue.build());
}
opCase_ = 3;
return this;
}
/**
* .tensorflow.PartialRunSetupRequest partial_run_setup = 3;
*/
public Builder mergePartialRunSetup(org.tensorflow.distruntime.PartialRunSetupRequest value) {
if (partialRunSetupBuilder_ == null) {
if (opCase_ == 3 &&
op_ != org.tensorflow.distruntime.PartialRunSetupRequest.getDefaultInstance()) {
op_ = org.tensorflow.distruntime.PartialRunSetupRequest.newBuilder((org.tensorflow.distruntime.PartialRunSetupRequest) op_)
.mergeFrom(value).buildPartial();
} else {
op_ = value;
}
onChanged();
} else {
if (opCase_ == 3) {
partialRunSetupBuilder_.mergeFrom(value);
}
partialRunSetupBuilder_.setMessage(value);
}
opCase_ = 3;
return this;
}
/**
* .tensorflow.PartialRunSetupRequest partial_run_setup = 3;
*/
public Builder clearPartialRunSetup() {
if (partialRunSetupBuilder_ == null) {
if (opCase_ == 3) {
opCase_ = 0;
op_ = null;
onChanged();
}
} else {
if (opCase_ == 3) {
opCase_ = 0;
op_ = null;
}
partialRunSetupBuilder_.clear();
}
return this;
}
/**
* .tensorflow.PartialRunSetupRequest partial_run_setup = 3;
*/
public org.tensorflow.distruntime.PartialRunSetupRequest.Builder getPartialRunSetupBuilder() {
return getPartialRunSetupFieldBuilder().getBuilder();
}
/**
* .tensorflow.PartialRunSetupRequest partial_run_setup = 3;
*/
public org.tensorflow.distruntime.PartialRunSetupRequestOrBuilder getPartialRunSetupOrBuilder() {
if ((opCase_ == 3) && (partialRunSetupBuilder_ != null)) {
return partialRunSetupBuilder_.getMessageOrBuilder();
} else {
if (opCase_ == 3) {
return (org.tensorflow.distruntime.PartialRunSetupRequest) op_;
}
return org.tensorflow.distruntime.PartialRunSetupRequest.getDefaultInstance();
}
}
/**
* .tensorflow.PartialRunSetupRequest partial_run_setup = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.PartialRunSetupRequest, org.tensorflow.distruntime.PartialRunSetupRequest.Builder, org.tensorflow.distruntime.PartialRunSetupRequestOrBuilder>
getPartialRunSetupFieldBuilder() {
if (partialRunSetupBuilder_ == null) {
if (!(opCase_ == 3)) {
op_ = org.tensorflow.distruntime.PartialRunSetupRequest.getDefaultInstance();
}
partialRunSetupBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.PartialRunSetupRequest, org.tensorflow.distruntime.PartialRunSetupRequest.Builder, org.tensorflow.distruntime.PartialRunSetupRequestOrBuilder>(
(org.tensorflow.distruntime.PartialRunSetupRequest) op_,
getParentForChildren(),
isClean());
op_ = null;
}
opCase_ = 3;
onChanged();;
return partialRunSetupBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.RunStepRequest, org.tensorflow.distruntime.RunStepRequest.Builder, org.tensorflow.distruntime.RunStepRequestOrBuilder> runStepBuilder_;
/**
* .tensorflow.RunStepRequest run_step = 4;
*/
public boolean hasRunStep() {
return opCase_ == 4;
}
/**
* .tensorflow.RunStepRequest run_step = 4;
*/
public org.tensorflow.distruntime.RunStepRequest getRunStep() {
if (runStepBuilder_ == null) {
if (opCase_ == 4) {
return (org.tensorflow.distruntime.RunStepRequest) op_;
}
return org.tensorflow.distruntime.RunStepRequest.getDefaultInstance();
} else {
if (opCase_ == 4) {
return runStepBuilder_.getMessage();
}
return org.tensorflow.distruntime.RunStepRequest.getDefaultInstance();
}
}
/**
* .tensorflow.RunStepRequest run_step = 4;
*/
public Builder setRunStep(org.tensorflow.distruntime.RunStepRequest value) {
if (runStepBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
op_ = value;
onChanged();
} else {
runStepBuilder_.setMessage(value);
}
opCase_ = 4;
return this;
}
/**
* .tensorflow.RunStepRequest run_step = 4;
*/
public Builder setRunStep(
org.tensorflow.distruntime.RunStepRequest.Builder builderForValue) {
if (runStepBuilder_ == null) {
op_ = builderForValue.build();
onChanged();
} else {
runStepBuilder_.setMessage(builderForValue.build());
}
opCase_ = 4;
return this;
}
/**
* .tensorflow.RunStepRequest run_step = 4;
*/
public Builder mergeRunStep(org.tensorflow.distruntime.RunStepRequest value) {
if (runStepBuilder_ == null) {
if (opCase_ == 4 &&
op_ != org.tensorflow.distruntime.RunStepRequest.getDefaultInstance()) {
op_ = org.tensorflow.distruntime.RunStepRequest.newBuilder((org.tensorflow.distruntime.RunStepRequest) op_)
.mergeFrom(value).buildPartial();
} else {
op_ = value;
}
onChanged();
} else {
if (opCase_ == 4) {
runStepBuilder_.mergeFrom(value);
}
runStepBuilder_.setMessage(value);
}
opCase_ = 4;
return this;
}
/**
* .tensorflow.RunStepRequest run_step = 4;
*/
public Builder clearRunStep() {
if (runStepBuilder_ == null) {
if (opCase_ == 4) {
opCase_ = 0;
op_ = null;
onChanged();
}
} else {
if (opCase_ == 4) {
opCase_ = 0;
op_ = null;
}
runStepBuilder_.clear();
}
return this;
}
/**
* .tensorflow.RunStepRequest run_step = 4;
*/
public org.tensorflow.distruntime.RunStepRequest.Builder getRunStepBuilder() {
return getRunStepFieldBuilder().getBuilder();
}
/**
* .tensorflow.RunStepRequest run_step = 4;
*/
public org.tensorflow.distruntime.RunStepRequestOrBuilder getRunStepOrBuilder() {
if ((opCase_ == 4) && (runStepBuilder_ != null)) {
return runStepBuilder_.getMessageOrBuilder();
} else {
if (opCase_ == 4) {
return (org.tensorflow.distruntime.RunStepRequest) op_;
}
return org.tensorflow.distruntime.RunStepRequest.getDefaultInstance();
}
}
/**
* .tensorflow.RunStepRequest run_step = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.RunStepRequest, org.tensorflow.distruntime.RunStepRequest.Builder, org.tensorflow.distruntime.RunStepRequestOrBuilder>
getRunStepFieldBuilder() {
if (runStepBuilder_ == null) {
if (!(opCase_ == 4)) {
op_ = org.tensorflow.distruntime.RunStepRequest.getDefaultInstance();
}
runStepBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.RunStepRequest, org.tensorflow.distruntime.RunStepRequest.Builder, org.tensorflow.distruntime.RunStepRequestOrBuilder>(
(org.tensorflow.distruntime.RunStepRequest) op_,
getParentForChildren(),
isClean());
op_ = null;
}
opCase_ = 4;
onChanged();;
return runStepBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.CloseSessionRequest, org.tensorflow.distruntime.CloseSessionRequest.Builder, org.tensorflow.distruntime.CloseSessionRequestOrBuilder> closeSessionBuilder_;
/**
* .tensorflow.CloseSessionRequest close_session = 5;
*/
public boolean hasCloseSession() {
return opCase_ == 5;
}
/**
* .tensorflow.CloseSessionRequest close_session = 5;
*/
public org.tensorflow.distruntime.CloseSessionRequest getCloseSession() {
if (closeSessionBuilder_ == null) {
if (opCase_ == 5) {
return (org.tensorflow.distruntime.CloseSessionRequest) op_;
}
return org.tensorflow.distruntime.CloseSessionRequest.getDefaultInstance();
} else {
if (opCase_ == 5) {
return closeSessionBuilder_.getMessage();
}
return org.tensorflow.distruntime.CloseSessionRequest.getDefaultInstance();
}
}
/**
* .tensorflow.CloseSessionRequest close_session = 5;
*/
public Builder setCloseSession(org.tensorflow.distruntime.CloseSessionRequest value) {
if (closeSessionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
op_ = value;
onChanged();
} else {
closeSessionBuilder_.setMessage(value);
}
opCase_ = 5;
return this;
}
/**
* .tensorflow.CloseSessionRequest close_session = 5;
*/
public Builder setCloseSession(
org.tensorflow.distruntime.CloseSessionRequest.Builder builderForValue) {
if (closeSessionBuilder_ == null) {
op_ = builderForValue.build();
onChanged();
} else {
closeSessionBuilder_.setMessage(builderForValue.build());
}
opCase_ = 5;
return this;
}
/**
* .tensorflow.CloseSessionRequest close_session = 5;
*/
public Builder mergeCloseSession(org.tensorflow.distruntime.CloseSessionRequest value) {
if (closeSessionBuilder_ == null) {
if (opCase_ == 5 &&
op_ != org.tensorflow.distruntime.CloseSessionRequest.getDefaultInstance()) {
op_ = org.tensorflow.distruntime.CloseSessionRequest.newBuilder((org.tensorflow.distruntime.CloseSessionRequest) op_)
.mergeFrom(value).buildPartial();
} else {
op_ = value;
}
onChanged();
} else {
if (opCase_ == 5) {
closeSessionBuilder_.mergeFrom(value);
}
closeSessionBuilder_.setMessage(value);
}
opCase_ = 5;
return this;
}
/**
* .tensorflow.CloseSessionRequest close_session = 5;
*/
public Builder clearCloseSession() {
if (closeSessionBuilder_ == null) {
if (opCase_ == 5) {
opCase_ = 0;
op_ = null;
onChanged();
}
} else {
if (opCase_ == 5) {
opCase_ = 0;
op_ = null;
}
closeSessionBuilder_.clear();
}
return this;
}
/**
* .tensorflow.CloseSessionRequest close_session = 5;
*/
public org.tensorflow.distruntime.CloseSessionRequest.Builder getCloseSessionBuilder() {
return getCloseSessionFieldBuilder().getBuilder();
}
/**
* .tensorflow.CloseSessionRequest close_session = 5;
*/
public org.tensorflow.distruntime.CloseSessionRequestOrBuilder getCloseSessionOrBuilder() {
if ((opCase_ == 5) && (closeSessionBuilder_ != null)) {
return closeSessionBuilder_.getMessageOrBuilder();
} else {
if (opCase_ == 5) {
return (org.tensorflow.distruntime.CloseSessionRequest) op_;
}
return org.tensorflow.distruntime.CloseSessionRequest.getDefaultInstance();
}
}
/**
* .tensorflow.CloseSessionRequest close_session = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.CloseSessionRequest, org.tensorflow.distruntime.CloseSessionRequest.Builder, org.tensorflow.distruntime.CloseSessionRequestOrBuilder>
getCloseSessionFieldBuilder() {
if (closeSessionBuilder_ == null) {
if (!(opCase_ == 5)) {
op_ = org.tensorflow.distruntime.CloseSessionRequest.getDefaultInstance();
}
closeSessionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.CloseSessionRequest, org.tensorflow.distruntime.CloseSessionRequest.Builder, org.tensorflow.distruntime.CloseSessionRequestOrBuilder>(
(org.tensorflow.distruntime.CloseSessionRequest) op_,
getParentForChildren(),
isClean());
op_ = null;
}
opCase_ = 5;
onChanged();;
return closeSessionBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ListDevicesRequest, org.tensorflow.distruntime.ListDevicesRequest.Builder, org.tensorflow.distruntime.ListDevicesRequestOrBuilder> listDevicesBuilder_;
/**
* .tensorflow.ListDevicesRequest list_devices = 6;
*/
public boolean hasListDevices() {
return opCase_ == 6;
}
/**
* .tensorflow.ListDevicesRequest list_devices = 6;
*/
public org.tensorflow.distruntime.ListDevicesRequest getListDevices() {
if (listDevicesBuilder_ == null) {
if (opCase_ == 6) {
return (org.tensorflow.distruntime.ListDevicesRequest) op_;
}
return org.tensorflow.distruntime.ListDevicesRequest.getDefaultInstance();
} else {
if (opCase_ == 6) {
return listDevicesBuilder_.getMessage();
}
return org.tensorflow.distruntime.ListDevicesRequest.getDefaultInstance();
}
}
/**
* .tensorflow.ListDevicesRequest list_devices = 6;
*/
public Builder setListDevices(org.tensorflow.distruntime.ListDevicesRequest value) {
if (listDevicesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
op_ = value;
onChanged();
} else {
listDevicesBuilder_.setMessage(value);
}
opCase_ = 6;
return this;
}
/**
* .tensorflow.ListDevicesRequest list_devices = 6;
*/
public Builder setListDevices(
org.tensorflow.distruntime.ListDevicesRequest.Builder builderForValue) {
if (listDevicesBuilder_ == null) {
op_ = builderForValue.build();
onChanged();
} else {
listDevicesBuilder_.setMessage(builderForValue.build());
}
opCase_ = 6;
return this;
}
/**
* .tensorflow.ListDevicesRequest list_devices = 6;
*/
public Builder mergeListDevices(org.tensorflow.distruntime.ListDevicesRequest value) {
if (listDevicesBuilder_ == null) {
if (opCase_ == 6 &&
op_ != org.tensorflow.distruntime.ListDevicesRequest.getDefaultInstance()) {
op_ = org.tensorflow.distruntime.ListDevicesRequest.newBuilder((org.tensorflow.distruntime.ListDevicesRequest) op_)
.mergeFrom(value).buildPartial();
} else {
op_ = value;
}
onChanged();
} else {
if (opCase_ == 6) {
listDevicesBuilder_.mergeFrom(value);
}
listDevicesBuilder_.setMessage(value);
}
opCase_ = 6;
return this;
}
/**
* .tensorflow.ListDevicesRequest list_devices = 6;
*/
public Builder clearListDevices() {
if (listDevicesBuilder_ == null) {
if (opCase_ == 6) {
opCase_ = 0;
op_ = null;
onChanged();
}
} else {
if (opCase_ == 6) {
opCase_ = 0;
op_ = null;
}
listDevicesBuilder_.clear();
}
return this;
}
/**
* .tensorflow.ListDevicesRequest list_devices = 6;
*/
public org.tensorflow.distruntime.ListDevicesRequest.Builder getListDevicesBuilder() {
return getListDevicesFieldBuilder().getBuilder();
}
/**
* .tensorflow.ListDevicesRequest list_devices = 6;
*/
public org.tensorflow.distruntime.ListDevicesRequestOrBuilder getListDevicesOrBuilder() {
if ((opCase_ == 6) && (listDevicesBuilder_ != null)) {
return listDevicesBuilder_.getMessageOrBuilder();
} else {
if (opCase_ == 6) {
return (org.tensorflow.distruntime.ListDevicesRequest) op_;
}
return org.tensorflow.distruntime.ListDevicesRequest.getDefaultInstance();
}
}
/**
* .tensorflow.ListDevicesRequest list_devices = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ListDevicesRequest, org.tensorflow.distruntime.ListDevicesRequest.Builder, org.tensorflow.distruntime.ListDevicesRequestOrBuilder>
getListDevicesFieldBuilder() {
if (listDevicesBuilder_ == null) {
if (!(opCase_ == 6)) {
op_ = org.tensorflow.distruntime.ListDevicesRequest.getDefaultInstance();
}
listDevicesBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ListDevicesRequest, org.tensorflow.distruntime.ListDevicesRequest.Builder, org.tensorflow.distruntime.ListDevicesRequestOrBuilder>(
(org.tensorflow.distruntime.ListDevicesRequest) op_,
getParentForChildren(),
isClean());
op_ = null;
}
opCase_ = 6;
onChanged();;
return listDevicesBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ResetRequest, org.tensorflow.distruntime.ResetRequest.Builder, org.tensorflow.distruntime.ResetRequestOrBuilder> resetRequestBuilder_;
/**
* .tensorflow.ResetRequest reset_request = 7;
*/
public boolean hasResetRequest() {
return opCase_ == 7;
}
/**
* .tensorflow.ResetRequest reset_request = 7;
*/
public org.tensorflow.distruntime.ResetRequest getResetRequest() {
if (resetRequestBuilder_ == null) {
if (opCase_ == 7) {
return (org.tensorflow.distruntime.ResetRequest) op_;
}
return org.tensorflow.distruntime.ResetRequest.getDefaultInstance();
} else {
if (opCase_ == 7) {
return resetRequestBuilder_.getMessage();
}
return org.tensorflow.distruntime.ResetRequest.getDefaultInstance();
}
}
/**
* .tensorflow.ResetRequest reset_request = 7;
*/
public Builder setResetRequest(org.tensorflow.distruntime.ResetRequest value) {
if (resetRequestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
op_ = value;
onChanged();
} else {
resetRequestBuilder_.setMessage(value);
}
opCase_ = 7;
return this;
}
/**
* .tensorflow.ResetRequest reset_request = 7;
*/
public Builder setResetRequest(
org.tensorflow.distruntime.ResetRequest.Builder builderForValue) {
if (resetRequestBuilder_ == null) {
op_ = builderForValue.build();
onChanged();
} else {
resetRequestBuilder_.setMessage(builderForValue.build());
}
opCase_ = 7;
return this;
}
/**
* .tensorflow.ResetRequest reset_request = 7;
*/
public Builder mergeResetRequest(org.tensorflow.distruntime.ResetRequest value) {
if (resetRequestBuilder_ == null) {
if (opCase_ == 7 &&
op_ != org.tensorflow.distruntime.ResetRequest.getDefaultInstance()) {
op_ = org.tensorflow.distruntime.ResetRequest.newBuilder((org.tensorflow.distruntime.ResetRequest) op_)
.mergeFrom(value).buildPartial();
} else {
op_ = value;
}
onChanged();
} else {
if (opCase_ == 7) {
resetRequestBuilder_.mergeFrom(value);
}
resetRequestBuilder_.setMessage(value);
}
opCase_ = 7;
return this;
}
/**
* .tensorflow.ResetRequest reset_request = 7;
*/
public Builder clearResetRequest() {
if (resetRequestBuilder_ == null) {
if (opCase_ == 7) {
opCase_ = 0;
op_ = null;
onChanged();
}
} else {
if (opCase_ == 7) {
opCase_ = 0;
op_ = null;
}
resetRequestBuilder_.clear();
}
return this;
}
/**
* .tensorflow.ResetRequest reset_request = 7;
*/
public org.tensorflow.distruntime.ResetRequest.Builder getResetRequestBuilder() {
return getResetRequestFieldBuilder().getBuilder();
}
/**
* .tensorflow.ResetRequest reset_request = 7;
*/
public org.tensorflow.distruntime.ResetRequestOrBuilder getResetRequestOrBuilder() {
if ((opCase_ == 7) && (resetRequestBuilder_ != null)) {
return resetRequestBuilder_.getMessageOrBuilder();
} else {
if (opCase_ == 7) {
return (org.tensorflow.distruntime.ResetRequest) op_;
}
return org.tensorflow.distruntime.ResetRequest.getDefaultInstance();
}
}
/**
* .tensorflow.ResetRequest reset_request = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ResetRequest, org.tensorflow.distruntime.ResetRequest.Builder, org.tensorflow.distruntime.ResetRequestOrBuilder>
getResetRequestFieldBuilder() {
if (resetRequestBuilder_ == null) {
if (!(opCase_ == 7)) {
op_ = org.tensorflow.distruntime.ResetRequest.getDefaultInstance();
}
resetRequestBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ResetRequest, org.tensorflow.distruntime.ResetRequest.Builder, org.tensorflow.distruntime.ResetRequestOrBuilder>(
(org.tensorflow.distruntime.ResetRequest) op_,
getParentForChildren(),
isClean());
op_ = null;
}
opCase_ = 7;
onChanged();;
return resetRequestBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.MakeCallableRequest, org.tensorflow.distruntime.MakeCallableRequest.Builder, org.tensorflow.distruntime.MakeCallableRequestOrBuilder> makeCallableBuilder_;
/**
* .tensorflow.MakeCallableRequest make_callable = 8;
*/
public boolean hasMakeCallable() {
return opCase_ == 8;
}
/**
* .tensorflow.MakeCallableRequest make_callable = 8;
*/
public org.tensorflow.distruntime.MakeCallableRequest getMakeCallable() {
if (makeCallableBuilder_ == null) {
if (opCase_ == 8) {
return (org.tensorflow.distruntime.MakeCallableRequest) op_;
}
return org.tensorflow.distruntime.MakeCallableRequest.getDefaultInstance();
} else {
if (opCase_ == 8) {
return makeCallableBuilder_.getMessage();
}
return org.tensorflow.distruntime.MakeCallableRequest.getDefaultInstance();
}
}
/**
* .tensorflow.MakeCallableRequest make_callable = 8;
*/
public Builder setMakeCallable(org.tensorflow.distruntime.MakeCallableRequest value) {
if (makeCallableBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
op_ = value;
onChanged();
} else {
makeCallableBuilder_.setMessage(value);
}
opCase_ = 8;
return this;
}
/**
* .tensorflow.MakeCallableRequest make_callable = 8;
*/
public Builder setMakeCallable(
org.tensorflow.distruntime.MakeCallableRequest.Builder builderForValue) {
if (makeCallableBuilder_ == null) {
op_ = builderForValue.build();
onChanged();
} else {
makeCallableBuilder_.setMessage(builderForValue.build());
}
opCase_ = 8;
return this;
}
/**
* .tensorflow.MakeCallableRequest make_callable = 8;
*/
public Builder mergeMakeCallable(org.tensorflow.distruntime.MakeCallableRequest value) {
if (makeCallableBuilder_ == null) {
if (opCase_ == 8 &&
op_ != org.tensorflow.distruntime.MakeCallableRequest.getDefaultInstance()) {
op_ = org.tensorflow.distruntime.MakeCallableRequest.newBuilder((org.tensorflow.distruntime.MakeCallableRequest) op_)
.mergeFrom(value).buildPartial();
} else {
op_ = value;
}
onChanged();
} else {
if (opCase_ == 8) {
makeCallableBuilder_.mergeFrom(value);
}
makeCallableBuilder_.setMessage(value);
}
opCase_ = 8;
return this;
}
/**
* .tensorflow.MakeCallableRequest make_callable = 8;
*/
public Builder clearMakeCallable() {
if (makeCallableBuilder_ == null) {
if (opCase_ == 8) {
opCase_ = 0;
op_ = null;
onChanged();
}
} else {
if (opCase_ == 8) {
opCase_ = 0;
op_ = null;
}
makeCallableBuilder_.clear();
}
return this;
}
/**
* .tensorflow.MakeCallableRequest make_callable = 8;
*/
public org.tensorflow.distruntime.MakeCallableRequest.Builder getMakeCallableBuilder() {
return getMakeCallableFieldBuilder().getBuilder();
}
/**
* .tensorflow.MakeCallableRequest make_callable = 8;
*/
public org.tensorflow.distruntime.MakeCallableRequestOrBuilder getMakeCallableOrBuilder() {
if ((opCase_ == 8) && (makeCallableBuilder_ != null)) {
return makeCallableBuilder_.getMessageOrBuilder();
} else {
if (opCase_ == 8) {
return (org.tensorflow.distruntime.MakeCallableRequest) op_;
}
return org.tensorflow.distruntime.MakeCallableRequest.getDefaultInstance();
}
}
/**
* .tensorflow.MakeCallableRequest make_callable = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.MakeCallableRequest, org.tensorflow.distruntime.MakeCallableRequest.Builder, org.tensorflow.distruntime.MakeCallableRequestOrBuilder>
getMakeCallableFieldBuilder() {
if (makeCallableBuilder_ == null) {
if (!(opCase_ == 8)) {
op_ = org.tensorflow.distruntime.MakeCallableRequest.getDefaultInstance();
}
makeCallableBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.MakeCallableRequest, org.tensorflow.distruntime.MakeCallableRequest.Builder, org.tensorflow.distruntime.MakeCallableRequestOrBuilder>(
(org.tensorflow.distruntime.MakeCallableRequest) op_,
getParentForChildren(),
isClean());
op_ = null;
}
opCase_ = 8;
onChanged();;
return makeCallableBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.RunCallableRequest, org.tensorflow.distruntime.RunCallableRequest.Builder, org.tensorflow.distruntime.RunCallableRequestOrBuilder> runCallableBuilder_;
/**
* .tensorflow.RunCallableRequest run_callable = 9;
*/
public boolean hasRunCallable() {
return opCase_ == 9;
}
/**
* .tensorflow.RunCallableRequest run_callable = 9;
*/
public org.tensorflow.distruntime.RunCallableRequest getRunCallable() {
if (runCallableBuilder_ == null) {
if (opCase_ == 9) {
return (org.tensorflow.distruntime.RunCallableRequest) op_;
}
return org.tensorflow.distruntime.RunCallableRequest.getDefaultInstance();
} else {
if (opCase_ == 9) {
return runCallableBuilder_.getMessage();
}
return org.tensorflow.distruntime.RunCallableRequest.getDefaultInstance();
}
}
/**
* .tensorflow.RunCallableRequest run_callable = 9;
*/
public Builder setRunCallable(org.tensorflow.distruntime.RunCallableRequest value) {
if (runCallableBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
op_ = value;
onChanged();
} else {
runCallableBuilder_.setMessage(value);
}
opCase_ = 9;
return this;
}
/**
* .tensorflow.RunCallableRequest run_callable = 9;
*/
public Builder setRunCallable(
org.tensorflow.distruntime.RunCallableRequest.Builder builderForValue) {
if (runCallableBuilder_ == null) {
op_ = builderForValue.build();
onChanged();
} else {
runCallableBuilder_.setMessage(builderForValue.build());
}
opCase_ = 9;
return this;
}
/**
* .tensorflow.RunCallableRequest run_callable = 9;
*/
public Builder mergeRunCallable(org.tensorflow.distruntime.RunCallableRequest value) {
if (runCallableBuilder_ == null) {
if (opCase_ == 9 &&
op_ != org.tensorflow.distruntime.RunCallableRequest.getDefaultInstance()) {
op_ = org.tensorflow.distruntime.RunCallableRequest.newBuilder((org.tensorflow.distruntime.RunCallableRequest) op_)
.mergeFrom(value).buildPartial();
} else {
op_ = value;
}
onChanged();
} else {
if (opCase_ == 9) {
runCallableBuilder_.mergeFrom(value);
}
runCallableBuilder_.setMessage(value);
}
opCase_ = 9;
return this;
}
/**
* .tensorflow.RunCallableRequest run_callable = 9;
*/
public Builder clearRunCallable() {
if (runCallableBuilder_ == null) {
if (opCase_ == 9) {
opCase_ = 0;
op_ = null;
onChanged();
}
} else {
if (opCase_ == 9) {
opCase_ = 0;
op_ = null;
}
runCallableBuilder_.clear();
}
return this;
}
/**
* .tensorflow.RunCallableRequest run_callable = 9;
*/
public org.tensorflow.distruntime.RunCallableRequest.Builder getRunCallableBuilder() {
return getRunCallableFieldBuilder().getBuilder();
}
/**
* .tensorflow.RunCallableRequest run_callable = 9;
*/
public org.tensorflow.distruntime.RunCallableRequestOrBuilder getRunCallableOrBuilder() {
if ((opCase_ == 9) && (runCallableBuilder_ != null)) {
return runCallableBuilder_.getMessageOrBuilder();
} else {
if (opCase_ == 9) {
return (org.tensorflow.distruntime.RunCallableRequest) op_;
}
return org.tensorflow.distruntime.RunCallableRequest.getDefaultInstance();
}
}
/**
* .tensorflow.RunCallableRequest run_callable = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.RunCallableRequest, org.tensorflow.distruntime.RunCallableRequest.Builder, org.tensorflow.distruntime.RunCallableRequestOrBuilder>
getRunCallableFieldBuilder() {
if (runCallableBuilder_ == null) {
if (!(opCase_ == 9)) {
op_ = org.tensorflow.distruntime.RunCallableRequest.getDefaultInstance();
}
runCallableBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.RunCallableRequest, org.tensorflow.distruntime.RunCallableRequest.Builder, org.tensorflow.distruntime.RunCallableRequestOrBuilder>(
(org.tensorflow.distruntime.RunCallableRequest) op_,
getParentForChildren(),
isClean());
op_ = null;
}
opCase_ = 9;
onChanged();;
return runCallableBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ReleaseCallableRequest, org.tensorflow.distruntime.ReleaseCallableRequest.Builder, org.tensorflow.distruntime.ReleaseCallableRequestOrBuilder> releaseCallableBuilder_;
/**
* .tensorflow.ReleaseCallableRequest release_callable = 10;
*/
public boolean hasReleaseCallable() {
return opCase_ == 10;
}
/**
* .tensorflow.ReleaseCallableRequest release_callable = 10;
*/
public org.tensorflow.distruntime.ReleaseCallableRequest getReleaseCallable() {
if (releaseCallableBuilder_ == null) {
if (opCase_ == 10) {
return (org.tensorflow.distruntime.ReleaseCallableRequest) op_;
}
return org.tensorflow.distruntime.ReleaseCallableRequest.getDefaultInstance();
} else {
if (opCase_ == 10) {
return releaseCallableBuilder_.getMessage();
}
return org.tensorflow.distruntime.ReleaseCallableRequest.getDefaultInstance();
}
}
/**
* .tensorflow.ReleaseCallableRequest release_callable = 10;
*/
public Builder setReleaseCallable(org.tensorflow.distruntime.ReleaseCallableRequest value) {
if (releaseCallableBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
op_ = value;
onChanged();
} else {
releaseCallableBuilder_.setMessage(value);
}
opCase_ = 10;
return this;
}
/**
* .tensorflow.ReleaseCallableRequest release_callable = 10;
*/
public Builder setReleaseCallable(
org.tensorflow.distruntime.ReleaseCallableRequest.Builder builderForValue) {
if (releaseCallableBuilder_ == null) {
op_ = builderForValue.build();
onChanged();
} else {
releaseCallableBuilder_.setMessage(builderForValue.build());
}
opCase_ = 10;
return this;
}
/**
* .tensorflow.ReleaseCallableRequest release_callable = 10;
*/
public Builder mergeReleaseCallable(org.tensorflow.distruntime.ReleaseCallableRequest value) {
if (releaseCallableBuilder_ == null) {
if (opCase_ == 10 &&
op_ != org.tensorflow.distruntime.ReleaseCallableRequest.getDefaultInstance()) {
op_ = org.tensorflow.distruntime.ReleaseCallableRequest.newBuilder((org.tensorflow.distruntime.ReleaseCallableRequest) op_)
.mergeFrom(value).buildPartial();
} else {
op_ = value;
}
onChanged();
} else {
if (opCase_ == 10) {
releaseCallableBuilder_.mergeFrom(value);
}
releaseCallableBuilder_.setMessage(value);
}
opCase_ = 10;
return this;
}
/**
* .tensorflow.ReleaseCallableRequest release_callable = 10;
*/
public Builder clearReleaseCallable() {
if (releaseCallableBuilder_ == null) {
if (opCase_ == 10) {
opCase_ = 0;
op_ = null;
onChanged();
}
} else {
if (opCase_ == 10) {
opCase_ = 0;
op_ = null;
}
releaseCallableBuilder_.clear();
}
return this;
}
/**
* .tensorflow.ReleaseCallableRequest release_callable = 10;
*/
public org.tensorflow.distruntime.ReleaseCallableRequest.Builder getReleaseCallableBuilder() {
return getReleaseCallableFieldBuilder().getBuilder();
}
/**
* .tensorflow.ReleaseCallableRequest release_callable = 10;
*/
public org.tensorflow.distruntime.ReleaseCallableRequestOrBuilder getReleaseCallableOrBuilder() {
if ((opCase_ == 10) && (releaseCallableBuilder_ != null)) {
return releaseCallableBuilder_.getMessageOrBuilder();
} else {
if (opCase_ == 10) {
return (org.tensorflow.distruntime.ReleaseCallableRequest) op_;
}
return org.tensorflow.distruntime.ReleaseCallableRequest.getDefaultInstance();
}
}
/**
* .tensorflow.ReleaseCallableRequest release_callable = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ReleaseCallableRequest, org.tensorflow.distruntime.ReleaseCallableRequest.Builder, org.tensorflow.distruntime.ReleaseCallableRequestOrBuilder>
getReleaseCallableFieldBuilder() {
if (releaseCallableBuilder_ == null) {
if (!(opCase_ == 10)) {
op_ = org.tensorflow.distruntime.ReleaseCallableRequest.getDefaultInstance();
}
releaseCallableBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ReleaseCallableRequest, org.tensorflow.distruntime.ReleaseCallableRequest.Builder, org.tensorflow.distruntime.ReleaseCallableRequestOrBuilder>(
(org.tensorflow.distruntime.ReleaseCallableRequest) op_,
getParentForChildren(),
isClean());
op_ = null;
}
opCase_ = 10;
onChanged();;
return releaseCallableBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.ReplayLog.NewReplaySession, tensorflow.ReplayLog.NewReplaySession.Builder, tensorflow.ReplayLog.NewReplaySessionOrBuilder> newReplaySessionBuilder_;
/**
* .tensorflow.NewReplaySession new_replay_session = 11;
*/
public boolean hasNewReplaySession() {
return opCase_ == 11;
}
/**
* .tensorflow.NewReplaySession new_replay_session = 11;
*/
public tensorflow.ReplayLog.NewReplaySession getNewReplaySession() {
if (newReplaySessionBuilder_ == null) {
if (opCase_ == 11) {
return (tensorflow.ReplayLog.NewReplaySession) op_;
}
return tensorflow.ReplayLog.NewReplaySession.getDefaultInstance();
} else {
if (opCase_ == 11) {
return newReplaySessionBuilder_.getMessage();
}
return tensorflow.ReplayLog.NewReplaySession.getDefaultInstance();
}
}
/**
* .tensorflow.NewReplaySession new_replay_session = 11;
*/
public Builder setNewReplaySession(tensorflow.ReplayLog.NewReplaySession value) {
if (newReplaySessionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
op_ = value;
onChanged();
} else {
newReplaySessionBuilder_.setMessage(value);
}
opCase_ = 11;
return this;
}
/**
* .tensorflow.NewReplaySession new_replay_session = 11;
*/
public Builder setNewReplaySession(
tensorflow.ReplayLog.NewReplaySession.Builder builderForValue) {
if (newReplaySessionBuilder_ == null) {
op_ = builderForValue.build();
onChanged();
} else {
newReplaySessionBuilder_.setMessage(builderForValue.build());
}
opCase_ = 11;
return this;
}
/**
* .tensorflow.NewReplaySession new_replay_session = 11;
*/
public Builder mergeNewReplaySession(tensorflow.ReplayLog.NewReplaySession value) {
if (newReplaySessionBuilder_ == null) {
if (opCase_ == 11 &&
op_ != tensorflow.ReplayLog.NewReplaySession.getDefaultInstance()) {
op_ = tensorflow.ReplayLog.NewReplaySession.newBuilder((tensorflow.ReplayLog.NewReplaySession) op_)
.mergeFrom(value).buildPartial();
} else {
op_ = value;
}
onChanged();
} else {
if (opCase_ == 11) {
newReplaySessionBuilder_.mergeFrom(value);
}
newReplaySessionBuilder_.setMessage(value);
}
opCase_ = 11;
return this;
}
/**
* .tensorflow.NewReplaySession new_replay_session = 11;
*/
public Builder clearNewReplaySession() {
if (newReplaySessionBuilder_ == null) {
if (opCase_ == 11) {
opCase_ = 0;
op_ = null;
onChanged();
}
} else {
if (opCase_ == 11) {
opCase_ = 0;
op_ = null;
}
newReplaySessionBuilder_.clear();
}
return this;
}
/**
* .tensorflow.NewReplaySession new_replay_session = 11;
*/
public tensorflow.ReplayLog.NewReplaySession.Builder getNewReplaySessionBuilder() {
return getNewReplaySessionFieldBuilder().getBuilder();
}
/**
* .tensorflow.NewReplaySession new_replay_session = 11;
*/
public tensorflow.ReplayLog.NewReplaySessionOrBuilder getNewReplaySessionOrBuilder() {
if ((opCase_ == 11) && (newReplaySessionBuilder_ != null)) {
return newReplaySessionBuilder_.getMessageOrBuilder();
} else {
if (opCase_ == 11) {
return (tensorflow.ReplayLog.NewReplaySession) op_;
}
return tensorflow.ReplayLog.NewReplaySession.getDefaultInstance();
}
}
/**
* .tensorflow.NewReplaySession new_replay_session = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.ReplayLog.NewReplaySession, tensorflow.ReplayLog.NewReplaySession.Builder, tensorflow.ReplayLog.NewReplaySessionOrBuilder>
getNewReplaySessionFieldBuilder() {
if (newReplaySessionBuilder_ == null) {
if (!(opCase_ == 11)) {
op_ = tensorflow.ReplayLog.NewReplaySession.getDefaultInstance();
}
newReplaySessionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.ReplayLog.NewReplaySession, tensorflow.ReplayLog.NewReplaySession.Builder, tensorflow.ReplayLog.NewReplaySessionOrBuilder>(
(tensorflow.ReplayLog.NewReplaySession) op_,
getParentForChildren(),
isClean());
op_ = null;
}
opCase_ = 11;
onChanged();;
return newReplaySessionBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.CreateSessionResponse, org.tensorflow.distruntime.CreateSessionResponse.Builder, org.tensorflow.distruntime.CreateSessionResponseOrBuilder> createSessionResponseBuilder_;
/**
* .tensorflow.CreateSessionResponse create_session_response = 21;
*/
public boolean hasCreateSessionResponse() {
return responseCase_ == 21;
}
/**
* .tensorflow.CreateSessionResponse create_session_response = 21;
*/
public org.tensorflow.distruntime.CreateSessionResponse getCreateSessionResponse() {
if (createSessionResponseBuilder_ == null) {
if (responseCase_ == 21) {
return (org.tensorflow.distruntime.CreateSessionResponse) response_;
}
return org.tensorflow.distruntime.CreateSessionResponse.getDefaultInstance();
} else {
if (responseCase_ == 21) {
return createSessionResponseBuilder_.getMessage();
}
return org.tensorflow.distruntime.CreateSessionResponse.getDefaultInstance();
}
}
/**
* .tensorflow.CreateSessionResponse create_session_response = 21;
*/
public Builder setCreateSessionResponse(org.tensorflow.distruntime.CreateSessionResponse value) {
if (createSessionResponseBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
response_ = value;
onChanged();
} else {
createSessionResponseBuilder_.setMessage(value);
}
responseCase_ = 21;
return this;
}
/**
* .tensorflow.CreateSessionResponse create_session_response = 21;
*/
public Builder setCreateSessionResponse(
org.tensorflow.distruntime.CreateSessionResponse.Builder builderForValue) {
if (createSessionResponseBuilder_ == null) {
response_ = builderForValue.build();
onChanged();
} else {
createSessionResponseBuilder_.setMessage(builderForValue.build());
}
responseCase_ = 21;
return this;
}
/**
* .tensorflow.CreateSessionResponse create_session_response = 21;
*/
public Builder mergeCreateSessionResponse(org.tensorflow.distruntime.CreateSessionResponse value) {
if (createSessionResponseBuilder_ == null) {
if (responseCase_ == 21 &&
response_ != org.tensorflow.distruntime.CreateSessionResponse.getDefaultInstance()) {
response_ = org.tensorflow.distruntime.CreateSessionResponse.newBuilder((org.tensorflow.distruntime.CreateSessionResponse) response_)
.mergeFrom(value).buildPartial();
} else {
response_ = value;
}
onChanged();
} else {
if (responseCase_ == 21) {
createSessionResponseBuilder_.mergeFrom(value);
}
createSessionResponseBuilder_.setMessage(value);
}
responseCase_ = 21;
return this;
}
/**
* .tensorflow.CreateSessionResponse create_session_response = 21;
*/
public Builder clearCreateSessionResponse() {
if (createSessionResponseBuilder_ == null) {
if (responseCase_ == 21) {
responseCase_ = 0;
response_ = null;
onChanged();
}
} else {
if (responseCase_ == 21) {
responseCase_ = 0;
response_ = null;
}
createSessionResponseBuilder_.clear();
}
return this;
}
/**
* .tensorflow.CreateSessionResponse create_session_response = 21;
*/
public org.tensorflow.distruntime.CreateSessionResponse.Builder getCreateSessionResponseBuilder() {
return getCreateSessionResponseFieldBuilder().getBuilder();
}
/**
* .tensorflow.CreateSessionResponse create_session_response = 21;
*/
public org.tensorflow.distruntime.CreateSessionResponseOrBuilder getCreateSessionResponseOrBuilder() {
if ((responseCase_ == 21) && (createSessionResponseBuilder_ != null)) {
return createSessionResponseBuilder_.getMessageOrBuilder();
} else {
if (responseCase_ == 21) {
return (org.tensorflow.distruntime.CreateSessionResponse) response_;
}
return org.tensorflow.distruntime.CreateSessionResponse.getDefaultInstance();
}
}
/**
* .tensorflow.CreateSessionResponse create_session_response = 21;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.CreateSessionResponse, org.tensorflow.distruntime.CreateSessionResponse.Builder, org.tensorflow.distruntime.CreateSessionResponseOrBuilder>
getCreateSessionResponseFieldBuilder() {
if (createSessionResponseBuilder_ == null) {
if (!(responseCase_ == 21)) {
response_ = org.tensorflow.distruntime.CreateSessionResponse.getDefaultInstance();
}
createSessionResponseBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.CreateSessionResponse, org.tensorflow.distruntime.CreateSessionResponse.Builder, org.tensorflow.distruntime.CreateSessionResponseOrBuilder>(
(org.tensorflow.distruntime.CreateSessionResponse) response_,
getParentForChildren(),
isClean());
response_ = null;
}
responseCase_ = 21;
onChanged();;
return createSessionResponseBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ExtendSessionResponse, org.tensorflow.distruntime.ExtendSessionResponse.Builder, org.tensorflow.distruntime.ExtendSessionResponseOrBuilder> extendSessionResponseBuilder_;
/**
* .tensorflow.ExtendSessionResponse extend_session_response = 22;
*/
public boolean hasExtendSessionResponse() {
return responseCase_ == 22;
}
/**
* .tensorflow.ExtendSessionResponse extend_session_response = 22;
*/
public org.tensorflow.distruntime.ExtendSessionResponse getExtendSessionResponse() {
if (extendSessionResponseBuilder_ == null) {
if (responseCase_ == 22) {
return (org.tensorflow.distruntime.ExtendSessionResponse) response_;
}
return org.tensorflow.distruntime.ExtendSessionResponse.getDefaultInstance();
} else {
if (responseCase_ == 22) {
return extendSessionResponseBuilder_.getMessage();
}
return org.tensorflow.distruntime.ExtendSessionResponse.getDefaultInstance();
}
}
/**
* .tensorflow.ExtendSessionResponse extend_session_response = 22;
*/
public Builder setExtendSessionResponse(org.tensorflow.distruntime.ExtendSessionResponse value) {
if (extendSessionResponseBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
response_ = value;
onChanged();
} else {
extendSessionResponseBuilder_.setMessage(value);
}
responseCase_ = 22;
return this;
}
/**
* .tensorflow.ExtendSessionResponse extend_session_response = 22;
*/
public Builder setExtendSessionResponse(
org.tensorflow.distruntime.ExtendSessionResponse.Builder builderForValue) {
if (extendSessionResponseBuilder_ == null) {
response_ = builderForValue.build();
onChanged();
} else {
extendSessionResponseBuilder_.setMessage(builderForValue.build());
}
responseCase_ = 22;
return this;
}
/**
* .tensorflow.ExtendSessionResponse extend_session_response = 22;
*/
public Builder mergeExtendSessionResponse(org.tensorflow.distruntime.ExtendSessionResponse value) {
if (extendSessionResponseBuilder_ == null) {
if (responseCase_ == 22 &&
response_ != org.tensorflow.distruntime.ExtendSessionResponse.getDefaultInstance()) {
response_ = org.tensorflow.distruntime.ExtendSessionResponse.newBuilder((org.tensorflow.distruntime.ExtendSessionResponse) response_)
.mergeFrom(value).buildPartial();
} else {
response_ = value;
}
onChanged();
} else {
if (responseCase_ == 22) {
extendSessionResponseBuilder_.mergeFrom(value);
}
extendSessionResponseBuilder_.setMessage(value);
}
responseCase_ = 22;
return this;
}
/**
* .tensorflow.ExtendSessionResponse extend_session_response = 22;
*/
public Builder clearExtendSessionResponse() {
if (extendSessionResponseBuilder_ == null) {
if (responseCase_ == 22) {
responseCase_ = 0;
response_ = null;
onChanged();
}
} else {
if (responseCase_ == 22) {
responseCase_ = 0;
response_ = null;
}
extendSessionResponseBuilder_.clear();
}
return this;
}
/**
* .tensorflow.ExtendSessionResponse extend_session_response = 22;
*/
public org.tensorflow.distruntime.ExtendSessionResponse.Builder getExtendSessionResponseBuilder() {
return getExtendSessionResponseFieldBuilder().getBuilder();
}
/**
* .tensorflow.ExtendSessionResponse extend_session_response = 22;
*/
public org.tensorflow.distruntime.ExtendSessionResponseOrBuilder getExtendSessionResponseOrBuilder() {
if ((responseCase_ == 22) && (extendSessionResponseBuilder_ != null)) {
return extendSessionResponseBuilder_.getMessageOrBuilder();
} else {
if (responseCase_ == 22) {
return (org.tensorflow.distruntime.ExtendSessionResponse) response_;
}
return org.tensorflow.distruntime.ExtendSessionResponse.getDefaultInstance();
}
}
/**
* .tensorflow.ExtendSessionResponse extend_session_response = 22;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ExtendSessionResponse, org.tensorflow.distruntime.ExtendSessionResponse.Builder, org.tensorflow.distruntime.ExtendSessionResponseOrBuilder>
getExtendSessionResponseFieldBuilder() {
if (extendSessionResponseBuilder_ == null) {
if (!(responseCase_ == 22)) {
response_ = org.tensorflow.distruntime.ExtendSessionResponse.getDefaultInstance();
}
extendSessionResponseBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ExtendSessionResponse, org.tensorflow.distruntime.ExtendSessionResponse.Builder, org.tensorflow.distruntime.ExtendSessionResponseOrBuilder>(
(org.tensorflow.distruntime.ExtendSessionResponse) response_,
getParentForChildren(),
isClean());
response_ = null;
}
responseCase_ = 22;
onChanged();;
return extendSessionResponseBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.PartialRunSetupResponse, org.tensorflow.distruntime.PartialRunSetupResponse.Builder, org.tensorflow.distruntime.PartialRunSetupResponseOrBuilder> partialRunSetupResponseBuilder_;
/**
* .tensorflow.PartialRunSetupResponse partial_run_setup_response = 23;
*/
public boolean hasPartialRunSetupResponse() {
return responseCase_ == 23;
}
/**
* .tensorflow.PartialRunSetupResponse partial_run_setup_response = 23;
*/
public org.tensorflow.distruntime.PartialRunSetupResponse getPartialRunSetupResponse() {
if (partialRunSetupResponseBuilder_ == null) {
if (responseCase_ == 23) {
return (org.tensorflow.distruntime.PartialRunSetupResponse) response_;
}
return org.tensorflow.distruntime.PartialRunSetupResponse.getDefaultInstance();
} else {
if (responseCase_ == 23) {
return partialRunSetupResponseBuilder_.getMessage();
}
return org.tensorflow.distruntime.PartialRunSetupResponse.getDefaultInstance();
}
}
/**
* .tensorflow.PartialRunSetupResponse partial_run_setup_response = 23;
*/
public Builder setPartialRunSetupResponse(org.tensorflow.distruntime.PartialRunSetupResponse value) {
if (partialRunSetupResponseBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
response_ = value;
onChanged();
} else {
partialRunSetupResponseBuilder_.setMessage(value);
}
responseCase_ = 23;
return this;
}
/**
* .tensorflow.PartialRunSetupResponse partial_run_setup_response = 23;
*/
public Builder setPartialRunSetupResponse(
org.tensorflow.distruntime.PartialRunSetupResponse.Builder builderForValue) {
if (partialRunSetupResponseBuilder_ == null) {
response_ = builderForValue.build();
onChanged();
} else {
partialRunSetupResponseBuilder_.setMessage(builderForValue.build());
}
responseCase_ = 23;
return this;
}
/**
* .tensorflow.PartialRunSetupResponse partial_run_setup_response = 23;
*/
public Builder mergePartialRunSetupResponse(org.tensorflow.distruntime.PartialRunSetupResponse value) {
if (partialRunSetupResponseBuilder_ == null) {
if (responseCase_ == 23 &&
response_ != org.tensorflow.distruntime.PartialRunSetupResponse.getDefaultInstance()) {
response_ = org.tensorflow.distruntime.PartialRunSetupResponse.newBuilder((org.tensorflow.distruntime.PartialRunSetupResponse) response_)
.mergeFrom(value).buildPartial();
} else {
response_ = value;
}
onChanged();
} else {
if (responseCase_ == 23) {
partialRunSetupResponseBuilder_.mergeFrom(value);
}
partialRunSetupResponseBuilder_.setMessage(value);
}
responseCase_ = 23;
return this;
}
/**
* .tensorflow.PartialRunSetupResponse partial_run_setup_response = 23;
*/
public Builder clearPartialRunSetupResponse() {
if (partialRunSetupResponseBuilder_ == null) {
if (responseCase_ == 23) {
responseCase_ = 0;
response_ = null;
onChanged();
}
} else {
if (responseCase_ == 23) {
responseCase_ = 0;
response_ = null;
}
partialRunSetupResponseBuilder_.clear();
}
return this;
}
/**
* .tensorflow.PartialRunSetupResponse partial_run_setup_response = 23;
*/
public org.tensorflow.distruntime.PartialRunSetupResponse.Builder getPartialRunSetupResponseBuilder() {
return getPartialRunSetupResponseFieldBuilder().getBuilder();
}
/**
* .tensorflow.PartialRunSetupResponse partial_run_setup_response = 23;
*/
public org.tensorflow.distruntime.PartialRunSetupResponseOrBuilder getPartialRunSetupResponseOrBuilder() {
if ((responseCase_ == 23) && (partialRunSetupResponseBuilder_ != null)) {
return partialRunSetupResponseBuilder_.getMessageOrBuilder();
} else {
if (responseCase_ == 23) {
return (org.tensorflow.distruntime.PartialRunSetupResponse) response_;
}
return org.tensorflow.distruntime.PartialRunSetupResponse.getDefaultInstance();
}
}
/**
* .tensorflow.PartialRunSetupResponse partial_run_setup_response = 23;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.PartialRunSetupResponse, org.tensorflow.distruntime.PartialRunSetupResponse.Builder, org.tensorflow.distruntime.PartialRunSetupResponseOrBuilder>
getPartialRunSetupResponseFieldBuilder() {
if (partialRunSetupResponseBuilder_ == null) {
if (!(responseCase_ == 23)) {
response_ = org.tensorflow.distruntime.PartialRunSetupResponse.getDefaultInstance();
}
partialRunSetupResponseBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.PartialRunSetupResponse, org.tensorflow.distruntime.PartialRunSetupResponse.Builder, org.tensorflow.distruntime.PartialRunSetupResponseOrBuilder>(
(org.tensorflow.distruntime.PartialRunSetupResponse) response_,
getParentForChildren(),
isClean());
response_ = null;
}
responseCase_ = 23;
onChanged();;
return partialRunSetupResponseBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.RunStepResponse, org.tensorflow.distruntime.RunStepResponse.Builder, org.tensorflow.distruntime.RunStepResponseOrBuilder> runStepResponseBuilder_;
/**
* .tensorflow.RunStepResponse run_step_response = 24;
*/
public boolean hasRunStepResponse() {
return responseCase_ == 24;
}
/**
* .tensorflow.RunStepResponse run_step_response = 24;
*/
public org.tensorflow.distruntime.RunStepResponse getRunStepResponse() {
if (runStepResponseBuilder_ == null) {
if (responseCase_ == 24) {
return (org.tensorflow.distruntime.RunStepResponse) response_;
}
return org.tensorflow.distruntime.RunStepResponse.getDefaultInstance();
} else {
if (responseCase_ == 24) {
return runStepResponseBuilder_.getMessage();
}
return org.tensorflow.distruntime.RunStepResponse.getDefaultInstance();
}
}
/**
* .tensorflow.RunStepResponse run_step_response = 24;
*/
public Builder setRunStepResponse(org.tensorflow.distruntime.RunStepResponse value) {
if (runStepResponseBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
response_ = value;
onChanged();
} else {
runStepResponseBuilder_.setMessage(value);
}
responseCase_ = 24;
return this;
}
/**
* .tensorflow.RunStepResponse run_step_response = 24;
*/
public Builder setRunStepResponse(
org.tensorflow.distruntime.RunStepResponse.Builder builderForValue) {
if (runStepResponseBuilder_ == null) {
response_ = builderForValue.build();
onChanged();
} else {
runStepResponseBuilder_.setMessage(builderForValue.build());
}
responseCase_ = 24;
return this;
}
/**
* .tensorflow.RunStepResponse run_step_response = 24;
*/
public Builder mergeRunStepResponse(org.tensorflow.distruntime.RunStepResponse value) {
if (runStepResponseBuilder_ == null) {
if (responseCase_ == 24 &&
response_ != org.tensorflow.distruntime.RunStepResponse.getDefaultInstance()) {
response_ = org.tensorflow.distruntime.RunStepResponse.newBuilder((org.tensorflow.distruntime.RunStepResponse) response_)
.mergeFrom(value).buildPartial();
} else {
response_ = value;
}
onChanged();
} else {
if (responseCase_ == 24) {
runStepResponseBuilder_.mergeFrom(value);
}
runStepResponseBuilder_.setMessage(value);
}
responseCase_ = 24;
return this;
}
/**
* .tensorflow.RunStepResponse run_step_response = 24;
*/
public Builder clearRunStepResponse() {
if (runStepResponseBuilder_ == null) {
if (responseCase_ == 24) {
responseCase_ = 0;
response_ = null;
onChanged();
}
} else {
if (responseCase_ == 24) {
responseCase_ = 0;
response_ = null;
}
runStepResponseBuilder_.clear();
}
return this;
}
/**
* .tensorflow.RunStepResponse run_step_response = 24;
*/
public org.tensorflow.distruntime.RunStepResponse.Builder getRunStepResponseBuilder() {
return getRunStepResponseFieldBuilder().getBuilder();
}
/**
* .tensorflow.RunStepResponse run_step_response = 24;
*/
public org.tensorflow.distruntime.RunStepResponseOrBuilder getRunStepResponseOrBuilder() {
if ((responseCase_ == 24) && (runStepResponseBuilder_ != null)) {
return runStepResponseBuilder_.getMessageOrBuilder();
} else {
if (responseCase_ == 24) {
return (org.tensorflow.distruntime.RunStepResponse) response_;
}
return org.tensorflow.distruntime.RunStepResponse.getDefaultInstance();
}
}
/**
* .tensorflow.RunStepResponse run_step_response = 24;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.RunStepResponse, org.tensorflow.distruntime.RunStepResponse.Builder, org.tensorflow.distruntime.RunStepResponseOrBuilder>
getRunStepResponseFieldBuilder() {
if (runStepResponseBuilder_ == null) {
if (!(responseCase_ == 24)) {
response_ = org.tensorflow.distruntime.RunStepResponse.getDefaultInstance();
}
runStepResponseBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.RunStepResponse, org.tensorflow.distruntime.RunStepResponse.Builder, org.tensorflow.distruntime.RunStepResponseOrBuilder>(
(org.tensorflow.distruntime.RunStepResponse) response_,
getParentForChildren(),
isClean());
response_ = null;
}
responseCase_ = 24;
onChanged();;
return runStepResponseBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.CloseSessionResponse, org.tensorflow.distruntime.CloseSessionResponse.Builder, org.tensorflow.distruntime.CloseSessionResponseOrBuilder> closeSessionResponseBuilder_;
/**
* .tensorflow.CloseSessionResponse close_session_response = 25;
*/
public boolean hasCloseSessionResponse() {
return responseCase_ == 25;
}
/**
* .tensorflow.CloseSessionResponse close_session_response = 25;
*/
public org.tensorflow.distruntime.CloseSessionResponse getCloseSessionResponse() {
if (closeSessionResponseBuilder_ == null) {
if (responseCase_ == 25) {
return (org.tensorflow.distruntime.CloseSessionResponse) response_;
}
return org.tensorflow.distruntime.CloseSessionResponse.getDefaultInstance();
} else {
if (responseCase_ == 25) {
return closeSessionResponseBuilder_.getMessage();
}
return org.tensorflow.distruntime.CloseSessionResponse.getDefaultInstance();
}
}
/**
* .tensorflow.CloseSessionResponse close_session_response = 25;
*/
public Builder setCloseSessionResponse(org.tensorflow.distruntime.CloseSessionResponse value) {
if (closeSessionResponseBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
response_ = value;
onChanged();
} else {
closeSessionResponseBuilder_.setMessage(value);
}
responseCase_ = 25;
return this;
}
/**
* .tensorflow.CloseSessionResponse close_session_response = 25;
*/
public Builder setCloseSessionResponse(
org.tensorflow.distruntime.CloseSessionResponse.Builder builderForValue) {
if (closeSessionResponseBuilder_ == null) {
response_ = builderForValue.build();
onChanged();
} else {
closeSessionResponseBuilder_.setMessage(builderForValue.build());
}
responseCase_ = 25;
return this;
}
/**
* .tensorflow.CloseSessionResponse close_session_response = 25;
*/
public Builder mergeCloseSessionResponse(org.tensorflow.distruntime.CloseSessionResponse value) {
if (closeSessionResponseBuilder_ == null) {
if (responseCase_ == 25 &&
response_ != org.tensorflow.distruntime.CloseSessionResponse.getDefaultInstance()) {
response_ = org.tensorflow.distruntime.CloseSessionResponse.newBuilder((org.tensorflow.distruntime.CloseSessionResponse) response_)
.mergeFrom(value).buildPartial();
} else {
response_ = value;
}
onChanged();
} else {
if (responseCase_ == 25) {
closeSessionResponseBuilder_.mergeFrom(value);
}
closeSessionResponseBuilder_.setMessage(value);
}
responseCase_ = 25;
return this;
}
/**
* .tensorflow.CloseSessionResponse close_session_response = 25;
*/
public Builder clearCloseSessionResponse() {
if (closeSessionResponseBuilder_ == null) {
if (responseCase_ == 25) {
responseCase_ = 0;
response_ = null;
onChanged();
}
} else {
if (responseCase_ == 25) {
responseCase_ = 0;
response_ = null;
}
closeSessionResponseBuilder_.clear();
}
return this;
}
/**
* .tensorflow.CloseSessionResponse close_session_response = 25;
*/
public org.tensorflow.distruntime.CloseSessionResponse.Builder getCloseSessionResponseBuilder() {
return getCloseSessionResponseFieldBuilder().getBuilder();
}
/**
* .tensorflow.CloseSessionResponse close_session_response = 25;
*/
public org.tensorflow.distruntime.CloseSessionResponseOrBuilder getCloseSessionResponseOrBuilder() {
if ((responseCase_ == 25) && (closeSessionResponseBuilder_ != null)) {
return closeSessionResponseBuilder_.getMessageOrBuilder();
} else {
if (responseCase_ == 25) {
return (org.tensorflow.distruntime.CloseSessionResponse) response_;
}
return org.tensorflow.distruntime.CloseSessionResponse.getDefaultInstance();
}
}
/**
* .tensorflow.CloseSessionResponse close_session_response = 25;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.CloseSessionResponse, org.tensorflow.distruntime.CloseSessionResponse.Builder, org.tensorflow.distruntime.CloseSessionResponseOrBuilder>
getCloseSessionResponseFieldBuilder() {
if (closeSessionResponseBuilder_ == null) {
if (!(responseCase_ == 25)) {
response_ = org.tensorflow.distruntime.CloseSessionResponse.getDefaultInstance();
}
closeSessionResponseBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.CloseSessionResponse, org.tensorflow.distruntime.CloseSessionResponse.Builder, org.tensorflow.distruntime.CloseSessionResponseOrBuilder>(
(org.tensorflow.distruntime.CloseSessionResponse) response_,
getParentForChildren(),
isClean());
response_ = null;
}
responseCase_ = 25;
onChanged();;
return closeSessionResponseBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ListDevicesResponse, org.tensorflow.distruntime.ListDevicesResponse.Builder, org.tensorflow.distruntime.ListDevicesResponseOrBuilder> listDevicesResponseBuilder_;
/**
* .tensorflow.ListDevicesResponse list_devices_response = 26;
*/
public boolean hasListDevicesResponse() {
return responseCase_ == 26;
}
/**
* .tensorflow.ListDevicesResponse list_devices_response = 26;
*/
public org.tensorflow.distruntime.ListDevicesResponse getListDevicesResponse() {
if (listDevicesResponseBuilder_ == null) {
if (responseCase_ == 26) {
return (org.tensorflow.distruntime.ListDevicesResponse) response_;
}
return org.tensorflow.distruntime.ListDevicesResponse.getDefaultInstance();
} else {
if (responseCase_ == 26) {
return listDevicesResponseBuilder_.getMessage();
}
return org.tensorflow.distruntime.ListDevicesResponse.getDefaultInstance();
}
}
/**
* .tensorflow.ListDevicesResponse list_devices_response = 26;
*/
public Builder setListDevicesResponse(org.tensorflow.distruntime.ListDevicesResponse value) {
if (listDevicesResponseBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
response_ = value;
onChanged();
} else {
listDevicesResponseBuilder_.setMessage(value);
}
responseCase_ = 26;
return this;
}
/**
* .tensorflow.ListDevicesResponse list_devices_response = 26;
*/
public Builder setListDevicesResponse(
org.tensorflow.distruntime.ListDevicesResponse.Builder builderForValue) {
if (listDevicesResponseBuilder_ == null) {
response_ = builderForValue.build();
onChanged();
} else {
listDevicesResponseBuilder_.setMessage(builderForValue.build());
}
responseCase_ = 26;
return this;
}
/**
* .tensorflow.ListDevicesResponse list_devices_response = 26;
*/
public Builder mergeListDevicesResponse(org.tensorflow.distruntime.ListDevicesResponse value) {
if (listDevicesResponseBuilder_ == null) {
if (responseCase_ == 26 &&
response_ != org.tensorflow.distruntime.ListDevicesResponse.getDefaultInstance()) {
response_ = org.tensorflow.distruntime.ListDevicesResponse.newBuilder((org.tensorflow.distruntime.ListDevicesResponse) response_)
.mergeFrom(value).buildPartial();
} else {
response_ = value;
}
onChanged();
} else {
if (responseCase_ == 26) {
listDevicesResponseBuilder_.mergeFrom(value);
}
listDevicesResponseBuilder_.setMessage(value);
}
responseCase_ = 26;
return this;
}
/**
* .tensorflow.ListDevicesResponse list_devices_response = 26;
*/
public Builder clearListDevicesResponse() {
if (listDevicesResponseBuilder_ == null) {
if (responseCase_ == 26) {
responseCase_ = 0;
response_ = null;
onChanged();
}
} else {
if (responseCase_ == 26) {
responseCase_ = 0;
response_ = null;
}
listDevicesResponseBuilder_.clear();
}
return this;
}
/**
* .tensorflow.ListDevicesResponse list_devices_response = 26;
*/
public org.tensorflow.distruntime.ListDevicesResponse.Builder getListDevicesResponseBuilder() {
return getListDevicesResponseFieldBuilder().getBuilder();
}
/**
* .tensorflow.ListDevicesResponse list_devices_response = 26;
*/
public org.tensorflow.distruntime.ListDevicesResponseOrBuilder getListDevicesResponseOrBuilder() {
if ((responseCase_ == 26) && (listDevicesResponseBuilder_ != null)) {
return listDevicesResponseBuilder_.getMessageOrBuilder();
} else {
if (responseCase_ == 26) {
return (org.tensorflow.distruntime.ListDevicesResponse) response_;
}
return org.tensorflow.distruntime.ListDevicesResponse.getDefaultInstance();
}
}
/**
* .tensorflow.ListDevicesResponse list_devices_response = 26;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ListDevicesResponse, org.tensorflow.distruntime.ListDevicesResponse.Builder, org.tensorflow.distruntime.ListDevicesResponseOrBuilder>
getListDevicesResponseFieldBuilder() {
if (listDevicesResponseBuilder_ == null) {
if (!(responseCase_ == 26)) {
response_ = org.tensorflow.distruntime.ListDevicesResponse.getDefaultInstance();
}
listDevicesResponseBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ListDevicesResponse, org.tensorflow.distruntime.ListDevicesResponse.Builder, org.tensorflow.distruntime.ListDevicesResponseOrBuilder>(
(org.tensorflow.distruntime.ListDevicesResponse) response_,
getParentForChildren(),
isClean());
response_ = null;
}
responseCase_ = 26;
onChanged();;
return listDevicesResponseBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ResetResponse, org.tensorflow.distruntime.ResetResponse.Builder, org.tensorflow.distruntime.ResetResponseOrBuilder> resetRequestResponseBuilder_;
/**
* .tensorflow.ResetResponse reset_request_response = 27;
*/
public boolean hasResetRequestResponse() {
return responseCase_ == 27;
}
/**
* .tensorflow.ResetResponse reset_request_response = 27;
*/
public org.tensorflow.distruntime.ResetResponse getResetRequestResponse() {
if (resetRequestResponseBuilder_ == null) {
if (responseCase_ == 27) {
return (org.tensorflow.distruntime.ResetResponse) response_;
}
return org.tensorflow.distruntime.ResetResponse.getDefaultInstance();
} else {
if (responseCase_ == 27) {
return resetRequestResponseBuilder_.getMessage();
}
return org.tensorflow.distruntime.ResetResponse.getDefaultInstance();
}
}
/**
* .tensorflow.ResetResponse reset_request_response = 27;
*/
public Builder setResetRequestResponse(org.tensorflow.distruntime.ResetResponse value) {
if (resetRequestResponseBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
response_ = value;
onChanged();
} else {
resetRequestResponseBuilder_.setMessage(value);
}
responseCase_ = 27;
return this;
}
/**
* .tensorflow.ResetResponse reset_request_response = 27;
*/
public Builder setResetRequestResponse(
org.tensorflow.distruntime.ResetResponse.Builder builderForValue) {
if (resetRequestResponseBuilder_ == null) {
response_ = builderForValue.build();
onChanged();
} else {
resetRequestResponseBuilder_.setMessage(builderForValue.build());
}
responseCase_ = 27;
return this;
}
/**
* .tensorflow.ResetResponse reset_request_response = 27;
*/
public Builder mergeResetRequestResponse(org.tensorflow.distruntime.ResetResponse value) {
if (resetRequestResponseBuilder_ == null) {
if (responseCase_ == 27 &&
response_ != org.tensorflow.distruntime.ResetResponse.getDefaultInstance()) {
response_ = org.tensorflow.distruntime.ResetResponse.newBuilder((org.tensorflow.distruntime.ResetResponse) response_)
.mergeFrom(value).buildPartial();
} else {
response_ = value;
}
onChanged();
} else {
if (responseCase_ == 27) {
resetRequestResponseBuilder_.mergeFrom(value);
}
resetRequestResponseBuilder_.setMessage(value);
}
responseCase_ = 27;
return this;
}
/**
* .tensorflow.ResetResponse reset_request_response = 27;
*/
public Builder clearResetRequestResponse() {
if (resetRequestResponseBuilder_ == null) {
if (responseCase_ == 27) {
responseCase_ = 0;
response_ = null;
onChanged();
}
} else {
if (responseCase_ == 27) {
responseCase_ = 0;
response_ = null;
}
resetRequestResponseBuilder_.clear();
}
return this;
}
/**
* .tensorflow.ResetResponse reset_request_response = 27;
*/
public org.tensorflow.distruntime.ResetResponse.Builder getResetRequestResponseBuilder() {
return getResetRequestResponseFieldBuilder().getBuilder();
}
/**
* .tensorflow.ResetResponse reset_request_response = 27;
*/
public org.tensorflow.distruntime.ResetResponseOrBuilder getResetRequestResponseOrBuilder() {
if ((responseCase_ == 27) && (resetRequestResponseBuilder_ != null)) {
return resetRequestResponseBuilder_.getMessageOrBuilder();
} else {
if (responseCase_ == 27) {
return (org.tensorflow.distruntime.ResetResponse) response_;
}
return org.tensorflow.distruntime.ResetResponse.getDefaultInstance();
}
}
/**
* .tensorflow.ResetResponse reset_request_response = 27;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ResetResponse, org.tensorflow.distruntime.ResetResponse.Builder, org.tensorflow.distruntime.ResetResponseOrBuilder>
getResetRequestResponseFieldBuilder() {
if (resetRequestResponseBuilder_ == null) {
if (!(responseCase_ == 27)) {
response_ = org.tensorflow.distruntime.ResetResponse.getDefaultInstance();
}
resetRequestResponseBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ResetResponse, org.tensorflow.distruntime.ResetResponse.Builder, org.tensorflow.distruntime.ResetResponseOrBuilder>(
(org.tensorflow.distruntime.ResetResponse) response_,
getParentForChildren(),
isClean());
response_ = null;
}
responseCase_ = 27;
onChanged();;
return resetRequestResponseBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.MakeCallableResponse, org.tensorflow.distruntime.MakeCallableResponse.Builder, org.tensorflow.distruntime.MakeCallableResponseOrBuilder> makeCallableResponseBuilder_;
/**
* .tensorflow.MakeCallableResponse make_callable_response = 28;
*/
public boolean hasMakeCallableResponse() {
return responseCase_ == 28;
}
/**
* .tensorflow.MakeCallableResponse make_callable_response = 28;
*/
public org.tensorflow.distruntime.MakeCallableResponse getMakeCallableResponse() {
if (makeCallableResponseBuilder_ == null) {
if (responseCase_ == 28) {
return (org.tensorflow.distruntime.MakeCallableResponse) response_;
}
return org.tensorflow.distruntime.MakeCallableResponse.getDefaultInstance();
} else {
if (responseCase_ == 28) {
return makeCallableResponseBuilder_.getMessage();
}
return org.tensorflow.distruntime.MakeCallableResponse.getDefaultInstance();
}
}
/**
* .tensorflow.MakeCallableResponse make_callable_response = 28;
*/
public Builder setMakeCallableResponse(org.tensorflow.distruntime.MakeCallableResponse value) {
if (makeCallableResponseBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
response_ = value;
onChanged();
} else {
makeCallableResponseBuilder_.setMessage(value);
}
responseCase_ = 28;
return this;
}
/**
* .tensorflow.MakeCallableResponse make_callable_response = 28;
*/
public Builder setMakeCallableResponse(
org.tensorflow.distruntime.MakeCallableResponse.Builder builderForValue) {
if (makeCallableResponseBuilder_ == null) {
response_ = builderForValue.build();
onChanged();
} else {
makeCallableResponseBuilder_.setMessage(builderForValue.build());
}
responseCase_ = 28;
return this;
}
/**
* .tensorflow.MakeCallableResponse make_callable_response = 28;
*/
public Builder mergeMakeCallableResponse(org.tensorflow.distruntime.MakeCallableResponse value) {
if (makeCallableResponseBuilder_ == null) {
if (responseCase_ == 28 &&
response_ != org.tensorflow.distruntime.MakeCallableResponse.getDefaultInstance()) {
response_ = org.tensorflow.distruntime.MakeCallableResponse.newBuilder((org.tensorflow.distruntime.MakeCallableResponse) response_)
.mergeFrom(value).buildPartial();
} else {
response_ = value;
}
onChanged();
} else {
if (responseCase_ == 28) {
makeCallableResponseBuilder_.mergeFrom(value);
}
makeCallableResponseBuilder_.setMessage(value);
}
responseCase_ = 28;
return this;
}
/**
* .tensorflow.MakeCallableResponse make_callable_response = 28;
*/
public Builder clearMakeCallableResponse() {
if (makeCallableResponseBuilder_ == null) {
if (responseCase_ == 28) {
responseCase_ = 0;
response_ = null;
onChanged();
}
} else {
if (responseCase_ == 28) {
responseCase_ = 0;
response_ = null;
}
makeCallableResponseBuilder_.clear();
}
return this;
}
/**
* .tensorflow.MakeCallableResponse make_callable_response = 28;
*/
public org.tensorflow.distruntime.MakeCallableResponse.Builder getMakeCallableResponseBuilder() {
return getMakeCallableResponseFieldBuilder().getBuilder();
}
/**
* .tensorflow.MakeCallableResponse make_callable_response = 28;
*/
public org.tensorflow.distruntime.MakeCallableResponseOrBuilder getMakeCallableResponseOrBuilder() {
if ((responseCase_ == 28) && (makeCallableResponseBuilder_ != null)) {
return makeCallableResponseBuilder_.getMessageOrBuilder();
} else {
if (responseCase_ == 28) {
return (org.tensorflow.distruntime.MakeCallableResponse) response_;
}
return org.tensorflow.distruntime.MakeCallableResponse.getDefaultInstance();
}
}
/**
* .tensorflow.MakeCallableResponse make_callable_response = 28;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.MakeCallableResponse, org.tensorflow.distruntime.MakeCallableResponse.Builder, org.tensorflow.distruntime.MakeCallableResponseOrBuilder>
getMakeCallableResponseFieldBuilder() {
if (makeCallableResponseBuilder_ == null) {
if (!(responseCase_ == 28)) {
response_ = org.tensorflow.distruntime.MakeCallableResponse.getDefaultInstance();
}
makeCallableResponseBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.MakeCallableResponse, org.tensorflow.distruntime.MakeCallableResponse.Builder, org.tensorflow.distruntime.MakeCallableResponseOrBuilder>(
(org.tensorflow.distruntime.MakeCallableResponse) response_,
getParentForChildren(),
isClean());
response_ = null;
}
responseCase_ = 28;
onChanged();;
return makeCallableResponseBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.RunCallableResponse, org.tensorflow.distruntime.RunCallableResponse.Builder, org.tensorflow.distruntime.RunCallableResponseOrBuilder> runCallableResponseBuilder_;
/**
* .tensorflow.RunCallableResponse run_callable_response = 29;
*/
public boolean hasRunCallableResponse() {
return responseCase_ == 29;
}
/**
* .tensorflow.RunCallableResponse run_callable_response = 29;
*/
public org.tensorflow.distruntime.RunCallableResponse getRunCallableResponse() {
if (runCallableResponseBuilder_ == null) {
if (responseCase_ == 29) {
return (org.tensorflow.distruntime.RunCallableResponse) response_;
}
return org.tensorflow.distruntime.RunCallableResponse.getDefaultInstance();
} else {
if (responseCase_ == 29) {
return runCallableResponseBuilder_.getMessage();
}
return org.tensorflow.distruntime.RunCallableResponse.getDefaultInstance();
}
}
/**
* .tensorflow.RunCallableResponse run_callable_response = 29;
*/
public Builder setRunCallableResponse(org.tensorflow.distruntime.RunCallableResponse value) {
if (runCallableResponseBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
response_ = value;
onChanged();
} else {
runCallableResponseBuilder_.setMessage(value);
}
responseCase_ = 29;
return this;
}
/**
* .tensorflow.RunCallableResponse run_callable_response = 29;
*/
public Builder setRunCallableResponse(
org.tensorflow.distruntime.RunCallableResponse.Builder builderForValue) {
if (runCallableResponseBuilder_ == null) {
response_ = builderForValue.build();
onChanged();
} else {
runCallableResponseBuilder_.setMessage(builderForValue.build());
}
responseCase_ = 29;
return this;
}
/**
* .tensorflow.RunCallableResponse run_callable_response = 29;
*/
public Builder mergeRunCallableResponse(org.tensorflow.distruntime.RunCallableResponse value) {
if (runCallableResponseBuilder_ == null) {
if (responseCase_ == 29 &&
response_ != org.tensorflow.distruntime.RunCallableResponse.getDefaultInstance()) {
response_ = org.tensorflow.distruntime.RunCallableResponse.newBuilder((org.tensorflow.distruntime.RunCallableResponse) response_)
.mergeFrom(value).buildPartial();
} else {
response_ = value;
}
onChanged();
} else {
if (responseCase_ == 29) {
runCallableResponseBuilder_.mergeFrom(value);
}
runCallableResponseBuilder_.setMessage(value);
}
responseCase_ = 29;
return this;
}
/**
* .tensorflow.RunCallableResponse run_callable_response = 29;
*/
public Builder clearRunCallableResponse() {
if (runCallableResponseBuilder_ == null) {
if (responseCase_ == 29) {
responseCase_ = 0;
response_ = null;
onChanged();
}
} else {
if (responseCase_ == 29) {
responseCase_ = 0;
response_ = null;
}
runCallableResponseBuilder_.clear();
}
return this;
}
/**
* .tensorflow.RunCallableResponse run_callable_response = 29;
*/
public org.tensorflow.distruntime.RunCallableResponse.Builder getRunCallableResponseBuilder() {
return getRunCallableResponseFieldBuilder().getBuilder();
}
/**
* .tensorflow.RunCallableResponse run_callable_response = 29;
*/
public org.tensorflow.distruntime.RunCallableResponseOrBuilder getRunCallableResponseOrBuilder() {
if ((responseCase_ == 29) && (runCallableResponseBuilder_ != null)) {
return runCallableResponseBuilder_.getMessageOrBuilder();
} else {
if (responseCase_ == 29) {
return (org.tensorflow.distruntime.RunCallableResponse) response_;
}
return org.tensorflow.distruntime.RunCallableResponse.getDefaultInstance();
}
}
/**
* .tensorflow.RunCallableResponse run_callable_response = 29;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.RunCallableResponse, org.tensorflow.distruntime.RunCallableResponse.Builder, org.tensorflow.distruntime.RunCallableResponseOrBuilder>
getRunCallableResponseFieldBuilder() {
if (runCallableResponseBuilder_ == null) {
if (!(responseCase_ == 29)) {
response_ = org.tensorflow.distruntime.RunCallableResponse.getDefaultInstance();
}
runCallableResponseBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.RunCallableResponse, org.tensorflow.distruntime.RunCallableResponse.Builder, org.tensorflow.distruntime.RunCallableResponseOrBuilder>(
(org.tensorflow.distruntime.RunCallableResponse) response_,
getParentForChildren(),
isClean());
response_ = null;
}
responseCase_ = 29;
onChanged();;
return runCallableResponseBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ReleaseCallableResponse, org.tensorflow.distruntime.ReleaseCallableResponse.Builder, org.tensorflow.distruntime.ReleaseCallableResponseOrBuilder> releaseCallableResponseBuilder_;
/**
* .tensorflow.ReleaseCallableResponse release_callable_response = 30;
*/
public boolean hasReleaseCallableResponse() {
return responseCase_ == 30;
}
/**
* .tensorflow.ReleaseCallableResponse release_callable_response = 30;
*/
public org.tensorflow.distruntime.ReleaseCallableResponse getReleaseCallableResponse() {
if (releaseCallableResponseBuilder_ == null) {
if (responseCase_ == 30) {
return (org.tensorflow.distruntime.ReleaseCallableResponse) response_;
}
return org.tensorflow.distruntime.ReleaseCallableResponse.getDefaultInstance();
} else {
if (responseCase_ == 30) {
return releaseCallableResponseBuilder_.getMessage();
}
return org.tensorflow.distruntime.ReleaseCallableResponse.getDefaultInstance();
}
}
/**
* .tensorflow.ReleaseCallableResponse release_callable_response = 30;
*/
public Builder setReleaseCallableResponse(org.tensorflow.distruntime.ReleaseCallableResponse value) {
if (releaseCallableResponseBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
response_ = value;
onChanged();
} else {
releaseCallableResponseBuilder_.setMessage(value);
}
responseCase_ = 30;
return this;
}
/**
* .tensorflow.ReleaseCallableResponse release_callable_response = 30;
*/
public Builder setReleaseCallableResponse(
org.tensorflow.distruntime.ReleaseCallableResponse.Builder builderForValue) {
if (releaseCallableResponseBuilder_ == null) {
response_ = builderForValue.build();
onChanged();
} else {
releaseCallableResponseBuilder_.setMessage(builderForValue.build());
}
responseCase_ = 30;
return this;
}
/**
* .tensorflow.ReleaseCallableResponse release_callable_response = 30;
*/
public Builder mergeReleaseCallableResponse(org.tensorflow.distruntime.ReleaseCallableResponse value) {
if (releaseCallableResponseBuilder_ == null) {
if (responseCase_ == 30 &&
response_ != org.tensorflow.distruntime.ReleaseCallableResponse.getDefaultInstance()) {
response_ = org.tensorflow.distruntime.ReleaseCallableResponse.newBuilder((org.tensorflow.distruntime.ReleaseCallableResponse) response_)
.mergeFrom(value).buildPartial();
} else {
response_ = value;
}
onChanged();
} else {
if (responseCase_ == 30) {
releaseCallableResponseBuilder_.mergeFrom(value);
}
releaseCallableResponseBuilder_.setMessage(value);
}
responseCase_ = 30;
return this;
}
/**
* .tensorflow.ReleaseCallableResponse release_callable_response = 30;
*/
public Builder clearReleaseCallableResponse() {
if (releaseCallableResponseBuilder_ == null) {
if (responseCase_ == 30) {
responseCase_ = 0;
response_ = null;
onChanged();
}
} else {
if (responseCase_ == 30) {
responseCase_ = 0;
response_ = null;
}
releaseCallableResponseBuilder_.clear();
}
return this;
}
/**
* .tensorflow.ReleaseCallableResponse release_callable_response = 30;
*/
public org.tensorflow.distruntime.ReleaseCallableResponse.Builder getReleaseCallableResponseBuilder() {
return getReleaseCallableResponseFieldBuilder().getBuilder();
}
/**
* .tensorflow.ReleaseCallableResponse release_callable_response = 30;
*/
public org.tensorflow.distruntime.ReleaseCallableResponseOrBuilder getReleaseCallableResponseOrBuilder() {
if ((responseCase_ == 30) && (releaseCallableResponseBuilder_ != null)) {
return releaseCallableResponseBuilder_.getMessageOrBuilder();
} else {
if (responseCase_ == 30) {
return (org.tensorflow.distruntime.ReleaseCallableResponse) response_;
}
return org.tensorflow.distruntime.ReleaseCallableResponse.getDefaultInstance();
}
}
/**
* .tensorflow.ReleaseCallableResponse release_callable_response = 30;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ReleaseCallableResponse, org.tensorflow.distruntime.ReleaseCallableResponse.Builder, org.tensorflow.distruntime.ReleaseCallableResponseOrBuilder>
getReleaseCallableResponseFieldBuilder() {
if (releaseCallableResponseBuilder_ == null) {
if (!(responseCase_ == 30)) {
response_ = org.tensorflow.distruntime.ReleaseCallableResponse.getDefaultInstance();
}
releaseCallableResponseBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.distruntime.ReleaseCallableResponse, org.tensorflow.distruntime.ReleaseCallableResponse.Builder, org.tensorflow.distruntime.ReleaseCallableResponseOrBuilder>(
(org.tensorflow.distruntime.ReleaseCallableResponse) response_,
getParentForChildren(),
isClean());
response_ = null;
}
responseCase_ = 30;
onChanged();;
return releaseCallableResponseBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.ReplayOp)
}
// @@protoc_insertion_point(class_scope:tensorflow.ReplayOp)
private static final tensorflow.ReplayLog.ReplayOp DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.ReplayLog.ReplayOp();
}
public static tensorflow.ReplayLog.ReplayOp getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ReplayOp parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ReplayOp(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.ReplayLog.ReplayOp getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_NewReplaySession_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_NewReplaySession_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_ReplayOp_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_ReplayOp_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n)tensorflow/core/protobuf/replay_log.pr" +
"oto\022\ntensorflow\032%tensorflow/core/framewo" +
"rk/graph.proto\032&tensorflow/core/protobuf" +
"/cluster.proto\032%tensorflow/core/protobuf" +
"/master.proto\"\\\n\020NewReplaySession\0220\n\007dev" +
"ices\030\001 \001(\0132\037.tensorflow.ListDevicesRespo" +
"nse\022\026\n\016session_handle\030\002 \001(\t\"\350\n\n\010ReplayOp" +
"\022\025\n\rstart_time_us\030\037 \001(\001\022\023\n\013end_time_us\030 " +
" \001(\001\022:\n\016create_session\030\001 \001(\0132 .tensorflo" +
"w.CreateSessionRequestH\000\022:\n\016extend_sessi" +
"on\030\002 \001(\0132 .tensorflow.ExtendSessionReque" +
"stH\000\022?\n\021partial_run_setup\030\003 \001(\0132\".tensor" +
"flow.PartialRunSetupRequestH\000\022.\n\010run_ste" +
"p\030\004 \001(\0132\032.tensorflow.RunStepRequestH\000\0228\n" +
"\rclose_session\030\005 \001(\0132\037.tensorflow.CloseS" +
"essionRequestH\000\0226\n\014list_devices\030\006 \001(\0132\036." +
"tensorflow.ListDevicesRequestH\000\0221\n\rreset" +
"_request\030\007 \001(\0132\030.tensorflow.ResetRequest" +
"H\000\0228\n\rmake_callable\030\010 \001(\0132\037.tensorflow.M" +
"akeCallableRequestH\000\0226\n\014run_callable\030\t \001" +
"(\0132\036.tensorflow.RunCallableRequestH\000\022>\n\020" +
"release_callable\030\n \001(\0132\".tensorflow.Rele" +
"aseCallableRequestH\000\022:\n\022new_replay_sessi" +
"on\030\013 \001(\0132\034.tensorflow.NewReplaySessionH\000" +
"\022D\n\027create_session_response\030\025 \001(\0132!.tens" +
"orflow.CreateSessionResponseH\001\022D\n\027extend" +
"_session_response\030\026 \001(\0132!.tensorflow.Ext" +
"endSessionResponseH\001\022I\n\032partial_run_setu" +
"p_response\030\027 \001(\0132#.tensorflow.PartialRun" +
"SetupResponseH\001\0228\n\021run_step_response\030\030 \001" +
"(\0132\033.tensorflow.RunStepResponseH\001\022B\n\026clo" +
"se_session_response\030\031 \001(\0132 .tensorflow.C" +
"loseSessionResponseH\001\022@\n\025list_devices_re" +
"sponse\030\032 \001(\0132\037.tensorflow.ListDevicesRes" +
"ponseH\001\022;\n\026reset_request_response\030\033 \001(\0132" +
"\031.tensorflow.ResetResponseH\001\022B\n\026make_cal" +
"lable_response\030\034 \001(\0132 .tensorflow.MakeCa" +
"llableResponseH\001\022@\n\025run_callable_respons" +
"e\030\035 \001(\0132\037.tensorflow.RunCallableResponse" +
"H\001\022H\n\031release_callable_response\030\036 \001(\0132#." +
"tensorflow.ReleaseCallableResponseH\001B\004\n\002" +
"opB\n\n\010responseB\003\370\001\001b\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
org.tensorflow.framework.GraphProtos.getDescriptor(),
org.tensorflow.distruntime.ClusterProtos.getDescriptor(),
org.tensorflow.distruntime.DistributedRuntimeProtos.getDescriptor(),
}, assigner);
internal_static_tensorflow_NewReplaySession_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_tensorflow_NewReplaySession_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_NewReplaySession_descriptor,
new java.lang.String[] { "Devices", "SessionHandle", });
internal_static_tensorflow_ReplayOp_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_tensorflow_ReplayOp_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_ReplayOp_descriptor,
new java.lang.String[] { "StartTimeUs", "EndTimeUs", "CreateSession", "ExtendSession", "PartialRunSetup", "RunStep", "CloseSession", "ListDevices", "ResetRequest", "MakeCallable", "RunCallable", "ReleaseCallable", "NewReplaySession", "CreateSessionResponse", "ExtendSessionResponse", "PartialRunSetupResponse", "RunStepResponse", "CloseSessionResponse", "ListDevicesResponse", "ResetRequestResponse", "MakeCallableResponse", "RunCallableResponse", "ReleaseCallableResponse", "Op", "Response", });
org.tensorflow.framework.GraphProtos.getDescriptor();
org.tensorflow.distruntime.ClusterProtos.getDescriptor();
org.tensorflow.distruntime.DistributedRuntimeProtos.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy