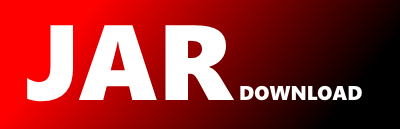
tensorflow.TpuProfiler Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: tensorflow/contrib/tpu/profiler/tpu_profiler.proto
package tensorflow;
public final class TpuProfiler {
private TpuProfiler() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface ProfileOptionsOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.ProfileOptions)
com.google.protobuf.MessageOrBuilder {
/**
*
* We don't collect the dataset ops by default for better trace-viewer
* scalability. The caller can mannually set this field to include the ops.
*
*
* bool include_dataset_ops = 1;
*/
boolean getIncludeDatasetOps();
}
/**
* Protobuf type {@code tensorflow.ProfileOptions}
*/
public static final class ProfileOptions extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.ProfileOptions)
ProfileOptionsOrBuilder {
private static final long serialVersionUID = 0L;
// Use ProfileOptions.newBuilder() to construct.
private ProfileOptions(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ProfileOptions() {
includeDatasetOps_ = false;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ProfileOptions(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
includeDatasetOps_ = input.readBool();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfiler.internal_static_tensorflow_ProfileOptions_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfiler.internal_static_tensorflow_ProfileOptions_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfiler.ProfileOptions.class, tensorflow.TpuProfiler.ProfileOptions.Builder.class);
}
public static final int INCLUDE_DATASET_OPS_FIELD_NUMBER = 1;
private boolean includeDatasetOps_;
/**
*
* We don't collect the dataset ops by default for better trace-viewer
* scalability. The caller can mannually set this field to include the ops.
*
*
* bool include_dataset_ops = 1;
*/
public boolean getIncludeDatasetOps() {
return includeDatasetOps_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (includeDatasetOps_ != false) {
output.writeBool(1, includeDatasetOps_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (includeDatasetOps_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(1, includeDatasetOps_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.TpuProfiler.ProfileOptions)) {
return super.equals(obj);
}
tensorflow.TpuProfiler.ProfileOptions other = (tensorflow.TpuProfiler.ProfileOptions) obj;
boolean result = true;
result = result && (getIncludeDatasetOps()
== other.getIncludeDatasetOps());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + INCLUDE_DATASET_OPS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIncludeDatasetOps());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.TpuProfiler.ProfileOptions parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfiler.ProfileOptions parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfiler.ProfileOptions parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfiler.ProfileOptions parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfiler.ProfileOptions parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfiler.ProfileOptions parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfiler.ProfileOptions parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfiler.ProfileOptions parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfiler.ProfileOptions parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.TpuProfiler.ProfileOptions parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfiler.ProfileOptions parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfiler.ProfileOptions parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.TpuProfiler.ProfileOptions prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.ProfileOptions}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.ProfileOptions)
tensorflow.TpuProfiler.ProfileOptionsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfiler.internal_static_tensorflow_ProfileOptions_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfiler.internal_static_tensorflow_ProfileOptions_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfiler.ProfileOptions.class, tensorflow.TpuProfiler.ProfileOptions.Builder.class);
}
// Construct using tensorflow.TpuProfiler.ProfileOptions.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
includeDatasetOps_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.TpuProfiler.internal_static_tensorflow_ProfileOptions_descriptor;
}
@java.lang.Override
public tensorflow.TpuProfiler.ProfileOptions getDefaultInstanceForType() {
return tensorflow.TpuProfiler.ProfileOptions.getDefaultInstance();
}
@java.lang.Override
public tensorflow.TpuProfiler.ProfileOptions build() {
tensorflow.TpuProfiler.ProfileOptions result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.TpuProfiler.ProfileOptions buildPartial() {
tensorflow.TpuProfiler.ProfileOptions result = new tensorflow.TpuProfiler.ProfileOptions(this);
result.includeDatasetOps_ = includeDatasetOps_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.TpuProfiler.ProfileOptions) {
return mergeFrom((tensorflow.TpuProfiler.ProfileOptions)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.TpuProfiler.ProfileOptions other) {
if (other == tensorflow.TpuProfiler.ProfileOptions.getDefaultInstance()) return this;
if (other.getIncludeDatasetOps() != false) {
setIncludeDatasetOps(other.getIncludeDatasetOps());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.TpuProfiler.ProfileOptions parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.TpuProfiler.ProfileOptions) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private boolean includeDatasetOps_ ;
/**
*
* We don't collect the dataset ops by default for better trace-viewer
* scalability. The caller can mannually set this field to include the ops.
*
*
* bool include_dataset_ops = 1;
*/
public boolean getIncludeDatasetOps() {
return includeDatasetOps_;
}
/**
*
* We don't collect the dataset ops by default for better trace-viewer
* scalability. The caller can mannually set this field to include the ops.
*
*
* bool include_dataset_ops = 1;
*/
public Builder setIncludeDatasetOps(boolean value) {
includeDatasetOps_ = value;
onChanged();
return this;
}
/**
*
* We don't collect the dataset ops by default for better trace-viewer
* scalability. The caller can mannually set this field to include the ops.
*
*
* bool include_dataset_ops = 1;
*/
public Builder clearIncludeDatasetOps() {
includeDatasetOps_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.ProfileOptions)
}
// @@protoc_insertion_point(class_scope:tensorflow.ProfileOptions)
private static final tensorflow.TpuProfiler.ProfileOptions DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.TpuProfiler.ProfileOptions();
}
public static tensorflow.TpuProfiler.ProfileOptions getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ProfileOptions parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ProfileOptions(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.TpuProfiler.ProfileOptions getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ToolRequestOptionsOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.ToolRequestOptions)
com.google.protobuf.MessageOrBuilder {
/**
*
* Required formats for the tool, it should be one of "json", "proto", "raw"
* etc. If not specified (backward compatible), use default format, i.e. most
* tools use json format.
*
*
* string output_formats = 2;
*/
java.lang.String getOutputFormats();
/**
*
* Required formats for the tool, it should be one of "json", "proto", "raw"
* etc. If not specified (backward compatible), use default format, i.e. most
* tools use json format.
*
*
* string output_formats = 2;
*/
com.google.protobuf.ByteString
getOutputFormatsBytes();
/**
*
* Whether save the result directly to repository or pass it back to caller.
* Default to false for backward compatibilities.
*
*
* bool save_to_repo = 3;
*/
boolean getSaveToRepo();
}
/**
* Protobuf type {@code tensorflow.ToolRequestOptions}
*/
public static final class ToolRequestOptions extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.ToolRequestOptions)
ToolRequestOptionsOrBuilder {
private static final long serialVersionUID = 0L;
// Use ToolRequestOptions.newBuilder() to construct.
private ToolRequestOptions(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ToolRequestOptions() {
outputFormats_ = "";
saveToRepo_ = false;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ToolRequestOptions(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 18: {
java.lang.String s = input.readStringRequireUtf8();
outputFormats_ = s;
break;
}
case 24: {
saveToRepo_ = input.readBool();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfiler.internal_static_tensorflow_ToolRequestOptions_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfiler.internal_static_tensorflow_ToolRequestOptions_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfiler.ToolRequestOptions.class, tensorflow.TpuProfiler.ToolRequestOptions.Builder.class);
}
public static final int OUTPUT_FORMATS_FIELD_NUMBER = 2;
private volatile java.lang.Object outputFormats_;
/**
*
* Required formats for the tool, it should be one of "json", "proto", "raw"
* etc. If not specified (backward compatible), use default format, i.e. most
* tools use json format.
*
*
* string output_formats = 2;
*/
public java.lang.String getOutputFormats() {
java.lang.Object ref = outputFormats_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
outputFormats_ = s;
return s;
}
}
/**
*
* Required formats for the tool, it should be one of "json", "proto", "raw"
* etc. If not specified (backward compatible), use default format, i.e. most
* tools use json format.
*
*
* string output_formats = 2;
*/
public com.google.protobuf.ByteString
getOutputFormatsBytes() {
java.lang.Object ref = outputFormats_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
outputFormats_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SAVE_TO_REPO_FIELD_NUMBER = 3;
private boolean saveToRepo_;
/**
*
* Whether save the result directly to repository or pass it back to caller.
* Default to false for backward compatibilities.
*
*
* bool save_to_repo = 3;
*/
public boolean getSaveToRepo() {
return saveToRepo_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getOutputFormatsBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, outputFormats_);
}
if (saveToRepo_ != false) {
output.writeBool(3, saveToRepo_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getOutputFormatsBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, outputFormats_);
}
if (saveToRepo_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, saveToRepo_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.TpuProfiler.ToolRequestOptions)) {
return super.equals(obj);
}
tensorflow.TpuProfiler.ToolRequestOptions other = (tensorflow.TpuProfiler.ToolRequestOptions) obj;
boolean result = true;
result = result && getOutputFormats()
.equals(other.getOutputFormats());
result = result && (getSaveToRepo()
== other.getSaveToRepo());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + OUTPUT_FORMATS_FIELD_NUMBER;
hash = (53 * hash) + getOutputFormats().hashCode();
hash = (37 * hash) + SAVE_TO_REPO_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getSaveToRepo());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.TpuProfiler.ToolRequestOptions parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfiler.ToolRequestOptions parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfiler.ToolRequestOptions parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfiler.ToolRequestOptions parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfiler.ToolRequestOptions parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfiler.ToolRequestOptions parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfiler.ToolRequestOptions parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfiler.ToolRequestOptions parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfiler.ToolRequestOptions parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.TpuProfiler.ToolRequestOptions parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfiler.ToolRequestOptions parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfiler.ToolRequestOptions parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.TpuProfiler.ToolRequestOptions prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.ToolRequestOptions}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.ToolRequestOptions)
tensorflow.TpuProfiler.ToolRequestOptionsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfiler.internal_static_tensorflow_ToolRequestOptions_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfiler.internal_static_tensorflow_ToolRequestOptions_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfiler.ToolRequestOptions.class, tensorflow.TpuProfiler.ToolRequestOptions.Builder.class);
}
// Construct using tensorflow.TpuProfiler.ToolRequestOptions.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
outputFormats_ = "";
saveToRepo_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.TpuProfiler.internal_static_tensorflow_ToolRequestOptions_descriptor;
}
@java.lang.Override
public tensorflow.TpuProfiler.ToolRequestOptions getDefaultInstanceForType() {
return tensorflow.TpuProfiler.ToolRequestOptions.getDefaultInstance();
}
@java.lang.Override
public tensorflow.TpuProfiler.ToolRequestOptions build() {
tensorflow.TpuProfiler.ToolRequestOptions result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.TpuProfiler.ToolRequestOptions buildPartial() {
tensorflow.TpuProfiler.ToolRequestOptions result = new tensorflow.TpuProfiler.ToolRequestOptions(this);
result.outputFormats_ = outputFormats_;
result.saveToRepo_ = saveToRepo_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.TpuProfiler.ToolRequestOptions) {
return mergeFrom((tensorflow.TpuProfiler.ToolRequestOptions)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.TpuProfiler.ToolRequestOptions other) {
if (other == tensorflow.TpuProfiler.ToolRequestOptions.getDefaultInstance()) return this;
if (!other.getOutputFormats().isEmpty()) {
outputFormats_ = other.outputFormats_;
onChanged();
}
if (other.getSaveToRepo() != false) {
setSaveToRepo(other.getSaveToRepo());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.TpuProfiler.ToolRequestOptions parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.TpuProfiler.ToolRequestOptions) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object outputFormats_ = "";
/**
*
* Required formats for the tool, it should be one of "json", "proto", "raw"
* etc. If not specified (backward compatible), use default format, i.e. most
* tools use json format.
*
*
* string output_formats = 2;
*/
public java.lang.String getOutputFormats() {
java.lang.Object ref = outputFormats_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
outputFormats_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Required formats for the tool, it should be one of "json", "proto", "raw"
* etc. If not specified (backward compatible), use default format, i.e. most
* tools use json format.
*
*
* string output_formats = 2;
*/
public com.google.protobuf.ByteString
getOutputFormatsBytes() {
java.lang.Object ref = outputFormats_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
outputFormats_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Required formats for the tool, it should be one of "json", "proto", "raw"
* etc. If not specified (backward compatible), use default format, i.e. most
* tools use json format.
*
*
* string output_formats = 2;
*/
public Builder setOutputFormats(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
outputFormats_ = value;
onChanged();
return this;
}
/**
*
* Required formats for the tool, it should be one of "json", "proto", "raw"
* etc. If not specified (backward compatible), use default format, i.e. most
* tools use json format.
*
*
* string output_formats = 2;
*/
public Builder clearOutputFormats() {
outputFormats_ = getDefaultInstance().getOutputFormats();
onChanged();
return this;
}
/**
*
* Required formats for the tool, it should be one of "json", "proto", "raw"
* etc. If not specified (backward compatible), use default format, i.e. most
* tools use json format.
*
*
* string output_formats = 2;
*/
public Builder setOutputFormatsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
outputFormats_ = value;
onChanged();
return this;
}
private boolean saveToRepo_ ;
/**
*
* Whether save the result directly to repository or pass it back to caller.
* Default to false for backward compatibilities.
*
*
* bool save_to_repo = 3;
*/
public boolean getSaveToRepo() {
return saveToRepo_;
}
/**
*
* Whether save the result directly to repository or pass it back to caller.
* Default to false for backward compatibilities.
*
*
* bool save_to_repo = 3;
*/
public Builder setSaveToRepo(boolean value) {
saveToRepo_ = value;
onChanged();
return this;
}
/**
*
* Whether save the result directly to repository or pass it back to caller.
* Default to false for backward compatibilities.
*
*
* bool save_to_repo = 3;
*/
public Builder clearSaveToRepo() {
saveToRepo_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.ToolRequestOptions)
}
// @@protoc_insertion_point(class_scope:tensorflow.ToolRequestOptions)
private static final tensorflow.TpuProfiler.ToolRequestOptions DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.TpuProfiler.ToolRequestOptions();
}
public static tensorflow.TpuProfiler.ToolRequestOptions getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ToolRequestOptions parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ToolRequestOptions(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.TpuProfiler.ToolRequestOptions getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ProfileRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.ProfileRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* In future, the caller will be able to customize when profiling starts and
* stops. For now, it collects `duration_ms` milliseconds worth of data.
*
*
* uint64 duration_ms = 1;
*/
long getDurationMs();
/**
*
* The maximum number of events to return. By default (value 0), return all
* events.
*
*
* uint64 max_events = 2;
*/
long getMaxEvents();
/**
*
* Required profiling tools name such as "input_pipeline_analyzer" etc
*
*
* repeated string tools = 3;
*/
java.util.List
getToolsList();
/**
*
* Required profiling tools name such as "input_pipeline_analyzer" etc
*
*
* repeated string tools = 3;
*/
int getToolsCount();
/**
*
* Required profiling tools name such as "input_pipeline_analyzer" etc
*
*
* repeated string tools = 3;
*/
java.lang.String getTools(int index);
/**
*
* Required profiling tools name such as "input_pipeline_analyzer" etc
*
*
* repeated string tools = 3;
*/
com.google.protobuf.ByteString
getToolsBytes(int index);
/**
*
* Specifies the requirement for each tools.
*
*
* map<string, .tensorflow.ToolRequestOptions> tool_options = 8;
*/
int getToolOptionsCount();
/**
*
* Specifies the requirement for each tools.
*
*
* map<string, .tensorflow.ToolRequestOptions> tool_options = 8;
*/
boolean containsToolOptions(
java.lang.String key);
/**
* Use {@link #getToolOptionsMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getToolOptions();
/**
*
* Specifies the requirement for each tools.
*
*
* map<string, .tensorflow.ToolRequestOptions> tool_options = 8;
*/
java.util.Map
getToolOptionsMap();
/**
*
* Specifies the requirement for each tools.
*
*
* map<string, .tensorflow.ToolRequestOptions> tool_options = 8;
*/
tensorflow.TpuProfiler.ToolRequestOptions getToolOptionsOrDefault(
java.lang.String key,
tensorflow.TpuProfiler.ToolRequestOptions defaultValue);
/**
*
* Specifies the requirement for each tools.
*
*
* map<string, .tensorflow.ToolRequestOptions> tool_options = 8;
*/
tensorflow.TpuProfiler.ToolRequestOptions getToolOptionsOrThrow(
java.lang.String key);
/**
*
* Optional profiling options that control how a TF session will be profiled.
*
*
* .tensorflow.ProfileOptions opts = 4;
*/
boolean hasOpts();
/**
*
* Optional profiling options that control how a TF session will be profiled.
*
*
* .tensorflow.ProfileOptions opts = 4;
*/
tensorflow.TpuProfiler.ProfileOptions getOpts();
/**
*
* Optional profiling options that control how a TF session will be profiled.
*
*
* .tensorflow.ProfileOptions opts = 4;
*/
tensorflow.TpuProfiler.ProfileOptionsOrBuilder getOptsOrBuilder();
/**
*
* The place where we will dump profile data. We will normally use
* MODEL_DIR/plugin/profile/ as our repository root.
*
*
* string repository_root = 5;
*/
java.lang.String getRepositoryRoot();
/**
*
* The place where we will dump profile data. We will normally use
* MODEL_DIR/plugin/profile/ as our repository root.
*
*
* string repository_root = 5;
*/
com.google.protobuf.ByteString
getRepositoryRootBytes();
/**
*
* The user provided profile session identifier.
*
*
* string session_id = 6;
*/
java.lang.String getSessionId();
/**
*
* The user provided profile session identifier.
*
*
* string session_id = 6;
*/
com.google.protobuf.ByteString
getSessionIdBytes();
/**
*
* The hostname of system where the profile should happen.
* We use it as identifier in part of our output filename.
*
*
* string host_name = 7;
*/
java.lang.String getHostName();
/**
*
* The hostname of system where the profile should happen.
* We use it as identifier in part of our output filename.
*
*
* string host_name = 7;
*/
com.google.protobuf.ByteString
getHostNameBytes();
}
/**
* Protobuf type {@code tensorflow.ProfileRequest}
*/
public static final class ProfileRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.ProfileRequest)
ProfileRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use ProfileRequest.newBuilder() to construct.
private ProfileRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ProfileRequest() {
durationMs_ = 0L;
maxEvents_ = 0L;
tools_ = com.google.protobuf.LazyStringArrayList.EMPTY;
repositoryRoot_ = "";
sessionId_ = "";
hostName_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ProfileRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
durationMs_ = input.readUInt64();
break;
}
case 16: {
maxEvents_ = input.readUInt64();
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
tools_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000004;
}
tools_.add(s);
break;
}
case 34: {
tensorflow.TpuProfiler.ProfileOptions.Builder subBuilder = null;
if (opts_ != null) {
subBuilder = opts_.toBuilder();
}
opts_ = input.readMessage(tensorflow.TpuProfiler.ProfileOptions.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(opts_);
opts_ = subBuilder.buildPartial();
}
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
repositoryRoot_ = s;
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
sessionId_ = s;
break;
}
case 58: {
java.lang.String s = input.readStringRequireUtf8();
hostName_ = s;
break;
}
case 66: {
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
toolOptions_ = com.google.protobuf.MapField.newMapField(
ToolOptionsDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000008;
}
com.google.protobuf.MapEntry
toolOptions__ = input.readMessage(
ToolOptionsDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
toolOptions_.getMutableMap().put(
toolOptions__.getKey(), toolOptions__.getValue());
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
tools_ = tools_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfiler.internal_static_tensorflow_ProfileRequest_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 8:
return internalGetToolOptions();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfiler.internal_static_tensorflow_ProfileRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfiler.ProfileRequest.class, tensorflow.TpuProfiler.ProfileRequest.Builder.class);
}
private int bitField0_;
public static final int DURATION_MS_FIELD_NUMBER = 1;
private long durationMs_;
/**
*
* In future, the caller will be able to customize when profiling starts and
* stops. For now, it collects `duration_ms` milliseconds worth of data.
*
*
* uint64 duration_ms = 1;
*/
public long getDurationMs() {
return durationMs_;
}
public static final int MAX_EVENTS_FIELD_NUMBER = 2;
private long maxEvents_;
/**
*
* The maximum number of events to return. By default (value 0), return all
* events.
*
*
* uint64 max_events = 2;
*/
public long getMaxEvents() {
return maxEvents_;
}
public static final int TOOLS_FIELD_NUMBER = 3;
private com.google.protobuf.LazyStringList tools_;
/**
*
* Required profiling tools name such as "input_pipeline_analyzer" etc
*
*
* repeated string tools = 3;
*/
public com.google.protobuf.ProtocolStringList
getToolsList() {
return tools_;
}
/**
*
* Required profiling tools name such as "input_pipeline_analyzer" etc
*
*
* repeated string tools = 3;
*/
public int getToolsCount() {
return tools_.size();
}
/**
*
* Required profiling tools name such as "input_pipeline_analyzer" etc
*
*
* repeated string tools = 3;
*/
public java.lang.String getTools(int index) {
return tools_.get(index);
}
/**
*
* Required profiling tools name such as "input_pipeline_analyzer" etc
*
*
* repeated string tools = 3;
*/
public com.google.protobuf.ByteString
getToolsBytes(int index) {
return tools_.getByteString(index);
}
public static final int TOOL_OPTIONS_FIELD_NUMBER = 8;
private static final class ToolOptionsDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, tensorflow.TpuProfiler.ToolRequestOptions> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
tensorflow.TpuProfiler.internal_static_tensorflow_ProfileRequest_ToolOptionsEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
tensorflow.TpuProfiler.ToolRequestOptions.getDefaultInstance());
}
private com.google.protobuf.MapField<
java.lang.String, tensorflow.TpuProfiler.ToolRequestOptions> toolOptions_;
private com.google.protobuf.MapField
internalGetToolOptions() {
if (toolOptions_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ToolOptionsDefaultEntryHolder.defaultEntry);
}
return toolOptions_;
}
public int getToolOptionsCount() {
return internalGetToolOptions().getMap().size();
}
/**
*
* Specifies the requirement for each tools.
*
*
* map<string, .tensorflow.ToolRequestOptions> tool_options = 8;
*/
public boolean containsToolOptions(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetToolOptions().getMap().containsKey(key);
}
/**
* Use {@link #getToolOptionsMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getToolOptions() {
return getToolOptionsMap();
}
/**
*
* Specifies the requirement for each tools.
*
*
* map<string, .tensorflow.ToolRequestOptions> tool_options = 8;
*/
public java.util.Map getToolOptionsMap() {
return internalGetToolOptions().getMap();
}
/**
*
* Specifies the requirement for each tools.
*
*
* map<string, .tensorflow.ToolRequestOptions> tool_options = 8;
*/
public tensorflow.TpuProfiler.ToolRequestOptions getToolOptionsOrDefault(
java.lang.String key,
tensorflow.TpuProfiler.ToolRequestOptions defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetToolOptions().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Specifies the requirement for each tools.
*
*
* map<string, .tensorflow.ToolRequestOptions> tool_options = 8;
*/
public tensorflow.TpuProfiler.ToolRequestOptions getToolOptionsOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetToolOptions().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int OPTS_FIELD_NUMBER = 4;
private tensorflow.TpuProfiler.ProfileOptions opts_;
/**
*
* Optional profiling options that control how a TF session will be profiled.
*
*
* .tensorflow.ProfileOptions opts = 4;
*/
public boolean hasOpts() {
return opts_ != null;
}
/**
*
* Optional profiling options that control how a TF session will be profiled.
*
*
* .tensorflow.ProfileOptions opts = 4;
*/
public tensorflow.TpuProfiler.ProfileOptions getOpts() {
return opts_ == null ? tensorflow.TpuProfiler.ProfileOptions.getDefaultInstance() : opts_;
}
/**
*
* Optional profiling options that control how a TF session will be profiled.
*
*
* .tensorflow.ProfileOptions opts = 4;
*/
public tensorflow.TpuProfiler.ProfileOptionsOrBuilder getOptsOrBuilder() {
return getOpts();
}
public static final int REPOSITORY_ROOT_FIELD_NUMBER = 5;
private volatile java.lang.Object repositoryRoot_;
/**
*
* The place where we will dump profile data. We will normally use
* MODEL_DIR/plugin/profile/ as our repository root.
*
*
* string repository_root = 5;
*/
public java.lang.String getRepositoryRoot() {
java.lang.Object ref = repositoryRoot_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
repositoryRoot_ = s;
return s;
}
}
/**
*
* The place where we will dump profile data. We will normally use
* MODEL_DIR/plugin/profile/ as our repository root.
*
*
* string repository_root = 5;
*/
public com.google.protobuf.ByteString
getRepositoryRootBytes() {
java.lang.Object ref = repositoryRoot_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
repositoryRoot_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SESSION_ID_FIELD_NUMBER = 6;
private volatile java.lang.Object sessionId_;
/**
*
* The user provided profile session identifier.
*
*
* string session_id = 6;
*/
public java.lang.String getSessionId() {
java.lang.Object ref = sessionId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sessionId_ = s;
return s;
}
}
/**
*
* The user provided profile session identifier.
*
*
* string session_id = 6;
*/
public com.google.protobuf.ByteString
getSessionIdBytes() {
java.lang.Object ref = sessionId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sessionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int HOST_NAME_FIELD_NUMBER = 7;
private volatile java.lang.Object hostName_;
/**
*
* The hostname of system where the profile should happen.
* We use it as identifier in part of our output filename.
*
*
* string host_name = 7;
*/
public java.lang.String getHostName() {
java.lang.Object ref = hostName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
hostName_ = s;
return s;
}
}
/**
*
* The hostname of system where the profile should happen.
* We use it as identifier in part of our output filename.
*
*
* string host_name = 7;
*/
public com.google.protobuf.ByteString
getHostNameBytes() {
java.lang.Object ref = hostName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
hostName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (durationMs_ != 0L) {
output.writeUInt64(1, durationMs_);
}
if (maxEvents_ != 0L) {
output.writeUInt64(2, maxEvents_);
}
for (int i = 0; i < tools_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, tools_.getRaw(i));
}
if (opts_ != null) {
output.writeMessage(4, getOpts());
}
if (!getRepositoryRootBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, repositoryRoot_);
}
if (!getSessionIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, sessionId_);
}
if (!getHostNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, hostName_);
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetToolOptions(),
ToolOptionsDefaultEntryHolder.defaultEntry,
8);
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (durationMs_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, durationMs_);
}
if (maxEvents_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, maxEvents_);
}
{
int dataSize = 0;
for (int i = 0; i < tools_.size(); i++) {
dataSize += computeStringSizeNoTag(tools_.getRaw(i));
}
size += dataSize;
size += 1 * getToolsList().size();
}
if (opts_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getOpts());
}
if (!getRepositoryRootBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, repositoryRoot_);
}
if (!getSessionIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, sessionId_);
}
if (!getHostNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, hostName_);
}
for (java.util.Map.Entry entry
: internalGetToolOptions().getMap().entrySet()) {
com.google.protobuf.MapEntry
toolOptions__ = ToolOptionsDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, toolOptions__);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.TpuProfiler.ProfileRequest)) {
return super.equals(obj);
}
tensorflow.TpuProfiler.ProfileRequest other = (tensorflow.TpuProfiler.ProfileRequest) obj;
boolean result = true;
result = result && (getDurationMs()
== other.getDurationMs());
result = result && (getMaxEvents()
== other.getMaxEvents());
result = result && getToolsList()
.equals(other.getToolsList());
result = result && internalGetToolOptions().equals(
other.internalGetToolOptions());
result = result && (hasOpts() == other.hasOpts());
if (hasOpts()) {
result = result && getOpts()
.equals(other.getOpts());
}
result = result && getRepositoryRoot()
.equals(other.getRepositoryRoot());
result = result && getSessionId()
.equals(other.getSessionId());
result = result && getHostName()
.equals(other.getHostName());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + DURATION_MS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getDurationMs());
hash = (37 * hash) + MAX_EVENTS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMaxEvents());
if (getToolsCount() > 0) {
hash = (37 * hash) + TOOLS_FIELD_NUMBER;
hash = (53 * hash) + getToolsList().hashCode();
}
if (!internalGetToolOptions().getMap().isEmpty()) {
hash = (37 * hash) + TOOL_OPTIONS_FIELD_NUMBER;
hash = (53 * hash) + internalGetToolOptions().hashCode();
}
if (hasOpts()) {
hash = (37 * hash) + OPTS_FIELD_NUMBER;
hash = (53 * hash) + getOpts().hashCode();
}
hash = (37 * hash) + REPOSITORY_ROOT_FIELD_NUMBER;
hash = (53 * hash) + getRepositoryRoot().hashCode();
hash = (37 * hash) + SESSION_ID_FIELD_NUMBER;
hash = (53 * hash) + getSessionId().hashCode();
hash = (37 * hash) + HOST_NAME_FIELD_NUMBER;
hash = (53 * hash) + getHostName().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.TpuProfiler.ProfileRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfiler.ProfileRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfiler.ProfileRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfiler.ProfileRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfiler.ProfileRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfiler.ProfileRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfiler.ProfileRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfiler.ProfileRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfiler.ProfileRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.TpuProfiler.ProfileRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfiler.ProfileRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfiler.ProfileRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.TpuProfiler.ProfileRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.ProfileRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.ProfileRequest)
tensorflow.TpuProfiler.ProfileRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfiler.internal_static_tensorflow_ProfileRequest_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 8:
return internalGetToolOptions();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 8:
return internalGetMutableToolOptions();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfiler.internal_static_tensorflow_ProfileRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfiler.ProfileRequest.class, tensorflow.TpuProfiler.ProfileRequest.Builder.class);
}
// Construct using tensorflow.TpuProfiler.ProfileRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
durationMs_ = 0L;
maxEvents_ = 0L;
tools_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
internalGetMutableToolOptions().clear();
if (optsBuilder_ == null) {
opts_ = null;
} else {
opts_ = null;
optsBuilder_ = null;
}
repositoryRoot_ = "";
sessionId_ = "";
hostName_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.TpuProfiler.internal_static_tensorflow_ProfileRequest_descriptor;
}
@java.lang.Override
public tensorflow.TpuProfiler.ProfileRequest getDefaultInstanceForType() {
return tensorflow.TpuProfiler.ProfileRequest.getDefaultInstance();
}
@java.lang.Override
public tensorflow.TpuProfiler.ProfileRequest build() {
tensorflow.TpuProfiler.ProfileRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.TpuProfiler.ProfileRequest buildPartial() {
tensorflow.TpuProfiler.ProfileRequest result = new tensorflow.TpuProfiler.ProfileRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.durationMs_ = durationMs_;
result.maxEvents_ = maxEvents_;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
tools_ = tools_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000004);
}
result.tools_ = tools_;
result.toolOptions_ = internalGetToolOptions();
result.toolOptions_.makeImmutable();
if (optsBuilder_ == null) {
result.opts_ = opts_;
} else {
result.opts_ = optsBuilder_.build();
}
result.repositoryRoot_ = repositoryRoot_;
result.sessionId_ = sessionId_;
result.hostName_ = hostName_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.TpuProfiler.ProfileRequest) {
return mergeFrom((tensorflow.TpuProfiler.ProfileRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.TpuProfiler.ProfileRequest other) {
if (other == tensorflow.TpuProfiler.ProfileRequest.getDefaultInstance()) return this;
if (other.getDurationMs() != 0L) {
setDurationMs(other.getDurationMs());
}
if (other.getMaxEvents() != 0L) {
setMaxEvents(other.getMaxEvents());
}
if (!other.tools_.isEmpty()) {
if (tools_.isEmpty()) {
tools_ = other.tools_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureToolsIsMutable();
tools_.addAll(other.tools_);
}
onChanged();
}
internalGetMutableToolOptions().mergeFrom(
other.internalGetToolOptions());
if (other.hasOpts()) {
mergeOpts(other.getOpts());
}
if (!other.getRepositoryRoot().isEmpty()) {
repositoryRoot_ = other.repositoryRoot_;
onChanged();
}
if (!other.getSessionId().isEmpty()) {
sessionId_ = other.sessionId_;
onChanged();
}
if (!other.getHostName().isEmpty()) {
hostName_ = other.hostName_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.TpuProfiler.ProfileRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.TpuProfiler.ProfileRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long durationMs_ ;
/**
*
* In future, the caller will be able to customize when profiling starts and
* stops. For now, it collects `duration_ms` milliseconds worth of data.
*
*
* uint64 duration_ms = 1;
*/
public long getDurationMs() {
return durationMs_;
}
/**
*
* In future, the caller will be able to customize when profiling starts and
* stops. For now, it collects `duration_ms` milliseconds worth of data.
*
*
* uint64 duration_ms = 1;
*/
public Builder setDurationMs(long value) {
durationMs_ = value;
onChanged();
return this;
}
/**
*
* In future, the caller will be able to customize when profiling starts and
* stops. For now, it collects `duration_ms` milliseconds worth of data.
*
*
* uint64 duration_ms = 1;
*/
public Builder clearDurationMs() {
durationMs_ = 0L;
onChanged();
return this;
}
private long maxEvents_ ;
/**
*
* The maximum number of events to return. By default (value 0), return all
* events.
*
*
* uint64 max_events = 2;
*/
public long getMaxEvents() {
return maxEvents_;
}
/**
*
* The maximum number of events to return. By default (value 0), return all
* events.
*
*
* uint64 max_events = 2;
*/
public Builder setMaxEvents(long value) {
maxEvents_ = value;
onChanged();
return this;
}
/**
*
* The maximum number of events to return. By default (value 0), return all
* events.
*
*
* uint64 max_events = 2;
*/
public Builder clearMaxEvents() {
maxEvents_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList tools_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureToolsIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
tools_ = new com.google.protobuf.LazyStringArrayList(tools_);
bitField0_ |= 0x00000004;
}
}
/**
*
* Required profiling tools name such as "input_pipeline_analyzer" etc
*
*
* repeated string tools = 3;
*/
public com.google.protobuf.ProtocolStringList
getToolsList() {
return tools_.getUnmodifiableView();
}
/**
*
* Required profiling tools name such as "input_pipeline_analyzer" etc
*
*
* repeated string tools = 3;
*/
public int getToolsCount() {
return tools_.size();
}
/**
*
* Required profiling tools name such as "input_pipeline_analyzer" etc
*
*
* repeated string tools = 3;
*/
public java.lang.String getTools(int index) {
return tools_.get(index);
}
/**
*
* Required profiling tools name such as "input_pipeline_analyzer" etc
*
*
* repeated string tools = 3;
*/
public com.google.protobuf.ByteString
getToolsBytes(int index) {
return tools_.getByteString(index);
}
/**
*
* Required profiling tools name such as "input_pipeline_analyzer" etc
*
*
* repeated string tools = 3;
*/
public Builder setTools(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureToolsIsMutable();
tools_.set(index, value);
onChanged();
return this;
}
/**
*
* Required profiling tools name such as "input_pipeline_analyzer" etc
*
*
* repeated string tools = 3;
*/
public Builder addTools(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureToolsIsMutable();
tools_.add(value);
onChanged();
return this;
}
/**
*
* Required profiling tools name such as "input_pipeline_analyzer" etc
*
*
* repeated string tools = 3;
*/
public Builder addAllTools(
java.lang.Iterable values) {
ensureToolsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, tools_);
onChanged();
return this;
}
/**
*
* Required profiling tools name such as "input_pipeline_analyzer" etc
*
*
* repeated string tools = 3;
*/
public Builder clearTools() {
tools_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* Required profiling tools name such as "input_pipeline_analyzer" etc
*
*
* repeated string tools = 3;
*/
public Builder addToolsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureToolsIsMutable();
tools_.add(value);
onChanged();
return this;
}
private com.google.protobuf.MapField<
java.lang.String, tensorflow.TpuProfiler.ToolRequestOptions> toolOptions_;
private com.google.protobuf.MapField
internalGetToolOptions() {
if (toolOptions_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ToolOptionsDefaultEntryHolder.defaultEntry);
}
return toolOptions_;
}
private com.google.protobuf.MapField
internalGetMutableToolOptions() {
onChanged();;
if (toolOptions_ == null) {
toolOptions_ = com.google.protobuf.MapField.newMapField(
ToolOptionsDefaultEntryHolder.defaultEntry);
}
if (!toolOptions_.isMutable()) {
toolOptions_ = toolOptions_.copy();
}
return toolOptions_;
}
public int getToolOptionsCount() {
return internalGetToolOptions().getMap().size();
}
/**
*
* Specifies the requirement for each tools.
*
*
* map<string, .tensorflow.ToolRequestOptions> tool_options = 8;
*/
public boolean containsToolOptions(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetToolOptions().getMap().containsKey(key);
}
/**
* Use {@link #getToolOptionsMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getToolOptions() {
return getToolOptionsMap();
}
/**
*
* Specifies the requirement for each tools.
*
*
* map<string, .tensorflow.ToolRequestOptions> tool_options = 8;
*/
public java.util.Map getToolOptionsMap() {
return internalGetToolOptions().getMap();
}
/**
*
* Specifies the requirement for each tools.
*
*
* map<string, .tensorflow.ToolRequestOptions> tool_options = 8;
*/
public tensorflow.TpuProfiler.ToolRequestOptions getToolOptionsOrDefault(
java.lang.String key,
tensorflow.TpuProfiler.ToolRequestOptions defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetToolOptions().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Specifies the requirement for each tools.
*
*
* map<string, .tensorflow.ToolRequestOptions> tool_options = 8;
*/
public tensorflow.TpuProfiler.ToolRequestOptions getToolOptionsOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetToolOptions().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearToolOptions() {
internalGetMutableToolOptions().getMutableMap()
.clear();
return this;
}
/**
*
* Specifies the requirement for each tools.
*
*
* map<string, .tensorflow.ToolRequestOptions> tool_options = 8;
*/
public Builder removeToolOptions(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableToolOptions().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableToolOptions() {
return internalGetMutableToolOptions().getMutableMap();
}
/**
*
* Specifies the requirement for each tools.
*
*
* map<string, .tensorflow.ToolRequestOptions> tool_options = 8;
*/
public Builder putToolOptions(
java.lang.String key,
tensorflow.TpuProfiler.ToolRequestOptions value) {
if (key == null) { throw new java.lang.NullPointerException(); }
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutableToolOptions().getMutableMap()
.put(key, value);
return this;
}
/**
*
* Specifies the requirement for each tools.
*
*
* map<string, .tensorflow.ToolRequestOptions> tool_options = 8;
*/
public Builder putAllToolOptions(
java.util.Map values) {
internalGetMutableToolOptions().getMutableMap()
.putAll(values);
return this;
}
private tensorflow.TpuProfiler.ProfileOptions opts_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.TpuProfiler.ProfileOptions, tensorflow.TpuProfiler.ProfileOptions.Builder, tensorflow.TpuProfiler.ProfileOptionsOrBuilder> optsBuilder_;
/**
*
* Optional profiling options that control how a TF session will be profiled.
*
*
* .tensorflow.ProfileOptions opts = 4;
*/
public boolean hasOpts() {
return optsBuilder_ != null || opts_ != null;
}
/**
*
* Optional profiling options that control how a TF session will be profiled.
*
*
* .tensorflow.ProfileOptions opts = 4;
*/
public tensorflow.TpuProfiler.ProfileOptions getOpts() {
if (optsBuilder_ == null) {
return opts_ == null ? tensorflow.TpuProfiler.ProfileOptions.getDefaultInstance() : opts_;
} else {
return optsBuilder_.getMessage();
}
}
/**
*
* Optional profiling options that control how a TF session will be profiled.
*
*
* .tensorflow.ProfileOptions opts = 4;
*/
public Builder setOpts(tensorflow.TpuProfiler.ProfileOptions value) {
if (optsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
opts_ = value;
onChanged();
} else {
optsBuilder_.setMessage(value);
}
return this;
}
/**
*
* Optional profiling options that control how a TF session will be profiled.
*
*
* .tensorflow.ProfileOptions opts = 4;
*/
public Builder setOpts(
tensorflow.TpuProfiler.ProfileOptions.Builder builderForValue) {
if (optsBuilder_ == null) {
opts_ = builderForValue.build();
onChanged();
} else {
optsBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Optional profiling options that control how a TF session will be profiled.
*
*
* .tensorflow.ProfileOptions opts = 4;
*/
public Builder mergeOpts(tensorflow.TpuProfiler.ProfileOptions value) {
if (optsBuilder_ == null) {
if (opts_ != null) {
opts_ =
tensorflow.TpuProfiler.ProfileOptions.newBuilder(opts_).mergeFrom(value).buildPartial();
} else {
opts_ = value;
}
onChanged();
} else {
optsBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Optional profiling options that control how a TF session will be profiled.
*
*
* .tensorflow.ProfileOptions opts = 4;
*/
public Builder clearOpts() {
if (optsBuilder_ == null) {
opts_ = null;
onChanged();
} else {
opts_ = null;
optsBuilder_ = null;
}
return this;
}
/**
*
* Optional profiling options that control how a TF session will be profiled.
*
*
* .tensorflow.ProfileOptions opts = 4;
*/
public tensorflow.TpuProfiler.ProfileOptions.Builder getOptsBuilder() {
onChanged();
return getOptsFieldBuilder().getBuilder();
}
/**
*
* Optional profiling options that control how a TF session will be profiled.
*
*
* .tensorflow.ProfileOptions opts = 4;
*/
public tensorflow.TpuProfiler.ProfileOptionsOrBuilder getOptsOrBuilder() {
if (optsBuilder_ != null) {
return optsBuilder_.getMessageOrBuilder();
} else {
return opts_ == null ?
tensorflow.TpuProfiler.ProfileOptions.getDefaultInstance() : opts_;
}
}
/**
*
* Optional profiling options that control how a TF session will be profiled.
*
*
* .tensorflow.ProfileOptions opts = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.TpuProfiler.ProfileOptions, tensorflow.TpuProfiler.ProfileOptions.Builder, tensorflow.TpuProfiler.ProfileOptionsOrBuilder>
getOptsFieldBuilder() {
if (optsBuilder_ == null) {
optsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.TpuProfiler.ProfileOptions, tensorflow.TpuProfiler.ProfileOptions.Builder, tensorflow.TpuProfiler.ProfileOptionsOrBuilder>(
getOpts(),
getParentForChildren(),
isClean());
opts_ = null;
}
return optsBuilder_;
}
private java.lang.Object repositoryRoot_ = "";
/**
*
* The place where we will dump profile data. We will normally use
* MODEL_DIR/plugin/profile/ as our repository root.
*
*
* string repository_root = 5;
*/
public java.lang.String getRepositoryRoot() {
java.lang.Object ref = repositoryRoot_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
repositoryRoot_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The place where we will dump profile data. We will normally use
* MODEL_DIR/plugin/profile/ as our repository root.
*
*
* string repository_root = 5;
*/
public com.google.protobuf.ByteString
getRepositoryRootBytes() {
java.lang.Object ref = repositoryRoot_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
repositoryRoot_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The place where we will dump profile data. We will normally use
* MODEL_DIR/plugin/profile/ as our repository root.
*
*
* string repository_root = 5;
*/
public Builder setRepositoryRoot(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
repositoryRoot_ = value;
onChanged();
return this;
}
/**
*
* The place where we will dump profile data. We will normally use
* MODEL_DIR/plugin/profile/ as our repository root.
*
*
* string repository_root = 5;
*/
public Builder clearRepositoryRoot() {
repositoryRoot_ = getDefaultInstance().getRepositoryRoot();
onChanged();
return this;
}
/**
*
* The place where we will dump profile data. We will normally use
* MODEL_DIR/plugin/profile/ as our repository root.
*
*
* string repository_root = 5;
*/
public Builder setRepositoryRootBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
repositoryRoot_ = value;
onChanged();
return this;
}
private java.lang.Object sessionId_ = "";
/**
*
* The user provided profile session identifier.
*
*
* string session_id = 6;
*/
public java.lang.String getSessionId() {
java.lang.Object ref = sessionId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sessionId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The user provided profile session identifier.
*
*
* string session_id = 6;
*/
public com.google.protobuf.ByteString
getSessionIdBytes() {
java.lang.Object ref = sessionId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sessionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The user provided profile session identifier.
*
*
* string session_id = 6;
*/
public Builder setSessionId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sessionId_ = value;
onChanged();
return this;
}
/**
*
* The user provided profile session identifier.
*
*
* string session_id = 6;
*/
public Builder clearSessionId() {
sessionId_ = getDefaultInstance().getSessionId();
onChanged();
return this;
}
/**
*
* The user provided profile session identifier.
*
*
* string session_id = 6;
*/
public Builder setSessionIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sessionId_ = value;
onChanged();
return this;
}
private java.lang.Object hostName_ = "";
/**
*
* The hostname of system where the profile should happen.
* We use it as identifier in part of our output filename.
*
*
* string host_name = 7;
*/
public java.lang.String getHostName() {
java.lang.Object ref = hostName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
hostName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The hostname of system where the profile should happen.
* We use it as identifier in part of our output filename.
*
*
* string host_name = 7;
*/
public com.google.protobuf.ByteString
getHostNameBytes() {
java.lang.Object ref = hostName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
hostName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The hostname of system where the profile should happen.
* We use it as identifier in part of our output filename.
*
*
* string host_name = 7;
*/
public Builder setHostName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
hostName_ = value;
onChanged();
return this;
}
/**
*
* The hostname of system where the profile should happen.
* We use it as identifier in part of our output filename.
*
*
* string host_name = 7;
*/
public Builder clearHostName() {
hostName_ = getDefaultInstance().getHostName();
onChanged();
return this;
}
/**
*
* The hostname of system where the profile should happen.
* We use it as identifier in part of our output filename.
*
*
* string host_name = 7;
*/
public Builder setHostNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
hostName_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.ProfileRequest)
}
// @@protoc_insertion_point(class_scope:tensorflow.ProfileRequest)
private static final tensorflow.TpuProfiler.ProfileRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.TpuProfiler.ProfileRequest();
}
public static tensorflow.TpuProfiler.ProfileRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ProfileRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ProfileRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.TpuProfiler.ProfileRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ProfileToolDataOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.ProfileToolData)
com.google.protobuf.MessageOrBuilder {
/**
*
* The file name which this data is associated (e.g. "input_pipeline.json",
* "cluster_xxx.memory_viewer.json").
*
*
* string name = 1;
*/
java.lang.String getName();
/**
*
* The file name which this data is associated (e.g. "input_pipeline.json",
* "cluster_xxx.memory_viewer.json").
*
*
* string name = 1;
*/
com.google.protobuf.ByteString
getNameBytes();
/**
*
* The data payload (likely json) for the specific tool.
*
*
* bytes data = 2;
*/
com.google.protobuf.ByteString getData();
}
/**
* Protobuf type {@code tensorflow.ProfileToolData}
*/
public static final class ProfileToolData extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.ProfileToolData)
ProfileToolDataOrBuilder {
private static final long serialVersionUID = 0L;
// Use ProfileToolData.newBuilder() to construct.
private ProfileToolData(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ProfileToolData() {
name_ = "";
data_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ProfileToolData(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 18: {
data_ = input.readBytes();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfiler.internal_static_tensorflow_ProfileToolData_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfiler.internal_static_tensorflow_ProfileToolData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfiler.ProfileToolData.class, tensorflow.TpuProfiler.ProfileToolData.Builder.class);
}
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
*
* The file name which this data is associated (e.g. "input_pipeline.json",
* "cluster_xxx.memory_viewer.json").
*
*
* string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
* The file name which this data is associated (e.g. "input_pipeline.json",
* "cluster_xxx.memory_viewer.json").
*
*
* string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DATA_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString data_;
/**
*
* The data payload (likely json) for the specific tool.
*
*
* bytes data = 2;
*/
public com.google.protobuf.ByteString getData() {
return data_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (!data_.isEmpty()) {
output.writeBytes(2, data_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (!data_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, data_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.TpuProfiler.ProfileToolData)) {
return super.equals(obj);
}
tensorflow.TpuProfiler.ProfileToolData other = (tensorflow.TpuProfiler.ProfileToolData) obj;
boolean result = true;
result = result && getName()
.equals(other.getName());
result = result && getData()
.equals(other.getData());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.TpuProfiler.ProfileToolData parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfiler.ProfileToolData parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfiler.ProfileToolData parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfiler.ProfileToolData parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfiler.ProfileToolData parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfiler.ProfileToolData parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfiler.ProfileToolData parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfiler.ProfileToolData parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfiler.ProfileToolData parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.TpuProfiler.ProfileToolData parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfiler.ProfileToolData parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfiler.ProfileToolData parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.TpuProfiler.ProfileToolData prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.ProfileToolData}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.ProfileToolData)
tensorflow.TpuProfiler.ProfileToolDataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfiler.internal_static_tensorflow_ProfileToolData_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfiler.internal_static_tensorflow_ProfileToolData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfiler.ProfileToolData.class, tensorflow.TpuProfiler.ProfileToolData.Builder.class);
}
// Construct using tensorflow.TpuProfiler.ProfileToolData.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
name_ = "";
data_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.TpuProfiler.internal_static_tensorflow_ProfileToolData_descriptor;
}
@java.lang.Override
public tensorflow.TpuProfiler.ProfileToolData getDefaultInstanceForType() {
return tensorflow.TpuProfiler.ProfileToolData.getDefaultInstance();
}
@java.lang.Override
public tensorflow.TpuProfiler.ProfileToolData build() {
tensorflow.TpuProfiler.ProfileToolData result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.TpuProfiler.ProfileToolData buildPartial() {
tensorflow.TpuProfiler.ProfileToolData result = new tensorflow.TpuProfiler.ProfileToolData(this);
result.name_ = name_;
result.data_ = data_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.TpuProfiler.ProfileToolData) {
return mergeFrom((tensorflow.TpuProfiler.ProfileToolData)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.TpuProfiler.ProfileToolData other) {
if (other == tensorflow.TpuProfiler.ProfileToolData.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (other.getData() != com.google.protobuf.ByteString.EMPTY) {
setData(other.getData());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.TpuProfiler.ProfileToolData parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.TpuProfiler.ProfileToolData) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object name_ = "";
/**
*
* The file name which this data is associated (e.g. "input_pipeline.json",
* "cluster_xxx.memory_viewer.json").
*
*
* string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The file name which this data is associated (e.g. "input_pipeline.json",
* "cluster_xxx.memory_viewer.json").
*
*
* string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The file name which this data is associated (e.g. "input_pipeline.json",
* "cluster_xxx.memory_viewer.json").
*
*
* string name = 1;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
*
* The file name which this data is associated (e.g. "input_pipeline.json",
* "cluster_xxx.memory_viewer.json").
*
*
* string name = 1;
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
*
* The file name which this data is associated (e.g. "input_pipeline.json",
* "cluster_xxx.memory_viewer.json").
*
*
* string name = 1;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* The data payload (likely json) for the specific tool.
*
*
* bytes data = 2;
*/
public com.google.protobuf.ByteString getData() {
return data_;
}
/**
*
* The data payload (likely json) for the specific tool.
*
*
* bytes data = 2;
*/
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
data_ = value;
onChanged();
return this;
}
/**
*
* The data payload (likely json) for the specific tool.
*
*
* bytes data = 2;
*/
public Builder clearData() {
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.ProfileToolData)
}
// @@protoc_insertion_point(class_scope:tensorflow.ProfileToolData)
private static final tensorflow.TpuProfiler.ProfileToolData DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.TpuProfiler.ProfileToolData();
}
public static tensorflow.TpuProfiler.ProfileToolData getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ProfileToolData parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ProfileToolData(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.TpuProfiler.ProfileToolData getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ProfileResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.ProfileResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
java.util.List
getComputationGraphList();
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
org.tensorflow.framework.GraphDef getComputationGraph(int index);
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
int getComputationGraphCount();
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
java.util.List extends org.tensorflow.framework.GraphDefOrBuilder>
getComputationGraphOrBuilderList();
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
org.tensorflow.framework.GraphDefOrBuilder getComputationGraphOrBuilder(
int index);
/**
*
* Performance profile that can be used to annotate HLO operations in the
* computation graph.
*
*
* .tensorflow.RunMetadata hlo_metadata = 5;
*/
boolean hasHloMetadata();
/**
*
* Performance profile that can be used to annotate HLO operations in the
* computation graph.
*
*
* .tensorflow.RunMetadata hlo_metadata = 5;
*/
org.tensorflow.framework.RunMetadata getHloMetadata();
/**
*
* Performance profile that can be used to annotate HLO operations in the
* computation graph.
*
*
* .tensorflow.RunMetadata hlo_metadata = 5;
*/
org.tensorflow.framework.RunMetadataOrBuilder getHloMetadataOrBuilder();
/**
*
* Encoded Trace proto message that contains metadata about the trace captured
* during the profiling period. Describes the devices and resources that
* 'trace_events' refers to.
*
*
* bytes encoded_trace = 3;
*/
com.google.protobuf.ByteString getEncodedTrace();
/**
*
* Assembles a hierarchical performance profile based on HLOs in trace events.
* If the trace covers multiple programs, the longest-running one is analyzed.
* See op_profile.proto for the detailed semantics of the returned profile.
*
*
* .tensorflow.tpu.op_profile.Profile op_profile = 4;
*/
boolean hasOpProfile();
/**
*
* Assembles a hierarchical performance profile based on HLOs in trace events.
* If the trace covers multiple programs, the longest-running one is analyzed.
* See op_profile.proto for the detailed semantics of the returned profile.
*
*
* .tensorflow.tpu.op_profile.Profile op_profile = 4;
*/
tensorflow.tpu.op_profile.OpProfile.Profile getOpProfile();
/**
*
* Assembles a hierarchical performance profile based on HLOs in trace events.
* If the trace covers multiple programs, the longest-running one is analyzed.
* See op_profile.proto for the detailed semantics of the returned profile.
*
*
* .tensorflow.tpu.op_profile.Profile op_profile = 4;
*/
tensorflow.tpu.op_profile.OpProfile.ProfileOrBuilder getOpProfileOrBuilder();
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
java.util.List
getToolDataList();
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
tensorflow.TpuProfiler.ProfileToolData getToolData(int index);
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
int getToolDataCount();
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
java.util.List extends tensorflow.TpuProfiler.ProfileToolDataOrBuilder>
getToolDataOrBuilderList();
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
tensorflow.TpuProfiler.ProfileToolDataOrBuilder getToolDataOrBuilder(
int index);
/**
*
* When we write profiling data directly to repository directory, we need a
* way to figure out whether the captured trace is empty (due to idle TPU).
*
*
* bool empty_trace = 7;
*/
boolean getEmptyTrace();
}
/**
* Protobuf type {@code tensorflow.ProfileResponse}
*/
public static final class ProfileResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.ProfileResponse)
ProfileResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use ProfileResponse.newBuilder() to construct.
private ProfileResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ProfileResponse() {
computationGraph_ = java.util.Collections.emptyList();
encodedTrace_ = com.google.protobuf.ByteString.EMPTY;
toolData_ = java.util.Collections.emptyList();
emptyTrace_ = false;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ProfileResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 18: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
computationGraph_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
computationGraph_.add(
input.readMessage(org.tensorflow.framework.GraphDef.parser(), extensionRegistry));
break;
}
case 26: {
encodedTrace_ = input.readBytes();
break;
}
case 34: {
tensorflow.tpu.op_profile.OpProfile.Profile.Builder subBuilder = null;
if (opProfile_ != null) {
subBuilder = opProfile_.toBuilder();
}
opProfile_ = input.readMessage(tensorflow.tpu.op_profile.OpProfile.Profile.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(opProfile_);
opProfile_ = subBuilder.buildPartial();
}
break;
}
case 42: {
org.tensorflow.framework.RunMetadata.Builder subBuilder = null;
if (hloMetadata_ != null) {
subBuilder = hloMetadata_.toBuilder();
}
hloMetadata_ = input.readMessage(org.tensorflow.framework.RunMetadata.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(hloMetadata_);
hloMetadata_ = subBuilder.buildPartial();
}
break;
}
case 50: {
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
toolData_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
toolData_.add(
input.readMessage(tensorflow.TpuProfiler.ProfileToolData.parser(), extensionRegistry));
break;
}
case 56: {
emptyTrace_ = input.readBool();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
computationGraph_ = java.util.Collections.unmodifiableList(computationGraph_);
}
if (((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
toolData_ = java.util.Collections.unmodifiableList(toolData_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfiler.internal_static_tensorflow_ProfileResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfiler.internal_static_tensorflow_ProfileResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfiler.ProfileResponse.class, tensorflow.TpuProfiler.ProfileResponse.Builder.class);
}
private int bitField0_;
public static final int COMPUTATION_GRAPH_FIELD_NUMBER = 2;
private java.util.List computationGraph_;
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
public java.util.List getComputationGraphList() {
return computationGraph_;
}
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
public java.util.List extends org.tensorflow.framework.GraphDefOrBuilder>
getComputationGraphOrBuilderList() {
return computationGraph_;
}
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
public int getComputationGraphCount() {
return computationGraph_.size();
}
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
public org.tensorflow.framework.GraphDef getComputationGraph(int index) {
return computationGraph_.get(index);
}
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
public org.tensorflow.framework.GraphDefOrBuilder getComputationGraphOrBuilder(
int index) {
return computationGraph_.get(index);
}
public static final int HLO_METADATA_FIELD_NUMBER = 5;
private org.tensorflow.framework.RunMetadata hloMetadata_;
/**
*
* Performance profile that can be used to annotate HLO operations in the
* computation graph.
*
*
* .tensorflow.RunMetadata hlo_metadata = 5;
*/
public boolean hasHloMetadata() {
return hloMetadata_ != null;
}
/**
*
* Performance profile that can be used to annotate HLO operations in the
* computation graph.
*
*
* .tensorflow.RunMetadata hlo_metadata = 5;
*/
public org.tensorflow.framework.RunMetadata getHloMetadata() {
return hloMetadata_ == null ? org.tensorflow.framework.RunMetadata.getDefaultInstance() : hloMetadata_;
}
/**
*
* Performance profile that can be used to annotate HLO operations in the
* computation graph.
*
*
* .tensorflow.RunMetadata hlo_metadata = 5;
*/
public org.tensorflow.framework.RunMetadataOrBuilder getHloMetadataOrBuilder() {
return getHloMetadata();
}
public static final int ENCODED_TRACE_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString encodedTrace_;
/**
*
* Encoded Trace proto message that contains metadata about the trace captured
* during the profiling period. Describes the devices and resources that
* 'trace_events' refers to.
*
*
* bytes encoded_trace = 3;
*/
public com.google.protobuf.ByteString getEncodedTrace() {
return encodedTrace_;
}
public static final int OP_PROFILE_FIELD_NUMBER = 4;
private tensorflow.tpu.op_profile.OpProfile.Profile opProfile_;
/**
*
* Assembles a hierarchical performance profile based on HLOs in trace events.
* If the trace covers multiple programs, the longest-running one is analyzed.
* See op_profile.proto for the detailed semantics of the returned profile.
*
*
* .tensorflow.tpu.op_profile.Profile op_profile = 4;
*/
public boolean hasOpProfile() {
return opProfile_ != null;
}
/**
*
* Assembles a hierarchical performance profile based on HLOs in trace events.
* If the trace covers multiple programs, the longest-running one is analyzed.
* See op_profile.proto for the detailed semantics of the returned profile.
*
*
* .tensorflow.tpu.op_profile.Profile op_profile = 4;
*/
public tensorflow.tpu.op_profile.OpProfile.Profile getOpProfile() {
return opProfile_ == null ? tensorflow.tpu.op_profile.OpProfile.Profile.getDefaultInstance() : opProfile_;
}
/**
*
* Assembles a hierarchical performance profile based on HLOs in trace events.
* If the trace covers multiple programs, the longest-running one is analyzed.
* See op_profile.proto for the detailed semantics of the returned profile.
*
*
* .tensorflow.tpu.op_profile.Profile op_profile = 4;
*/
public tensorflow.tpu.op_profile.OpProfile.ProfileOrBuilder getOpProfileOrBuilder() {
return getOpProfile();
}
public static final int TOOL_DATA_FIELD_NUMBER = 6;
private java.util.List toolData_;
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
public java.util.List getToolDataList() {
return toolData_;
}
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
public java.util.List extends tensorflow.TpuProfiler.ProfileToolDataOrBuilder>
getToolDataOrBuilderList() {
return toolData_;
}
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
public int getToolDataCount() {
return toolData_.size();
}
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
public tensorflow.TpuProfiler.ProfileToolData getToolData(int index) {
return toolData_.get(index);
}
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
public tensorflow.TpuProfiler.ProfileToolDataOrBuilder getToolDataOrBuilder(
int index) {
return toolData_.get(index);
}
public static final int EMPTY_TRACE_FIELD_NUMBER = 7;
private boolean emptyTrace_;
/**
*
* When we write profiling data directly to repository directory, we need a
* way to figure out whether the captured trace is empty (due to idle TPU).
*
*
* bool empty_trace = 7;
*/
public boolean getEmptyTrace() {
return emptyTrace_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < computationGraph_.size(); i++) {
output.writeMessage(2, computationGraph_.get(i));
}
if (!encodedTrace_.isEmpty()) {
output.writeBytes(3, encodedTrace_);
}
if (opProfile_ != null) {
output.writeMessage(4, getOpProfile());
}
if (hloMetadata_ != null) {
output.writeMessage(5, getHloMetadata());
}
for (int i = 0; i < toolData_.size(); i++) {
output.writeMessage(6, toolData_.get(i));
}
if (emptyTrace_ != false) {
output.writeBool(7, emptyTrace_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < computationGraph_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, computationGraph_.get(i));
}
if (!encodedTrace_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, encodedTrace_);
}
if (opProfile_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getOpProfile());
}
if (hloMetadata_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getHloMetadata());
}
for (int i = 0; i < toolData_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, toolData_.get(i));
}
if (emptyTrace_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(7, emptyTrace_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.TpuProfiler.ProfileResponse)) {
return super.equals(obj);
}
tensorflow.TpuProfiler.ProfileResponse other = (tensorflow.TpuProfiler.ProfileResponse) obj;
boolean result = true;
result = result && getComputationGraphList()
.equals(other.getComputationGraphList());
result = result && (hasHloMetadata() == other.hasHloMetadata());
if (hasHloMetadata()) {
result = result && getHloMetadata()
.equals(other.getHloMetadata());
}
result = result && getEncodedTrace()
.equals(other.getEncodedTrace());
result = result && (hasOpProfile() == other.hasOpProfile());
if (hasOpProfile()) {
result = result && getOpProfile()
.equals(other.getOpProfile());
}
result = result && getToolDataList()
.equals(other.getToolDataList());
result = result && (getEmptyTrace()
== other.getEmptyTrace());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getComputationGraphCount() > 0) {
hash = (37 * hash) + COMPUTATION_GRAPH_FIELD_NUMBER;
hash = (53 * hash) + getComputationGraphList().hashCode();
}
if (hasHloMetadata()) {
hash = (37 * hash) + HLO_METADATA_FIELD_NUMBER;
hash = (53 * hash) + getHloMetadata().hashCode();
}
hash = (37 * hash) + ENCODED_TRACE_FIELD_NUMBER;
hash = (53 * hash) + getEncodedTrace().hashCode();
if (hasOpProfile()) {
hash = (37 * hash) + OP_PROFILE_FIELD_NUMBER;
hash = (53 * hash) + getOpProfile().hashCode();
}
if (getToolDataCount() > 0) {
hash = (37 * hash) + TOOL_DATA_FIELD_NUMBER;
hash = (53 * hash) + getToolDataList().hashCode();
}
hash = (37 * hash) + EMPTY_TRACE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getEmptyTrace());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.TpuProfiler.ProfileResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfiler.ProfileResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfiler.ProfileResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfiler.ProfileResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfiler.ProfileResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfiler.ProfileResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfiler.ProfileResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfiler.ProfileResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfiler.ProfileResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.TpuProfiler.ProfileResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfiler.ProfileResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfiler.ProfileResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.TpuProfiler.ProfileResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.ProfileResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.ProfileResponse)
tensorflow.TpuProfiler.ProfileResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfiler.internal_static_tensorflow_ProfileResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfiler.internal_static_tensorflow_ProfileResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfiler.ProfileResponse.class, tensorflow.TpuProfiler.ProfileResponse.Builder.class);
}
// Construct using tensorflow.TpuProfiler.ProfileResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getComputationGraphFieldBuilder();
getToolDataFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (computationGraphBuilder_ == null) {
computationGraph_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
computationGraphBuilder_.clear();
}
if (hloMetadataBuilder_ == null) {
hloMetadata_ = null;
} else {
hloMetadata_ = null;
hloMetadataBuilder_ = null;
}
encodedTrace_ = com.google.protobuf.ByteString.EMPTY;
if (opProfileBuilder_ == null) {
opProfile_ = null;
} else {
opProfile_ = null;
opProfileBuilder_ = null;
}
if (toolDataBuilder_ == null) {
toolData_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
} else {
toolDataBuilder_.clear();
}
emptyTrace_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.TpuProfiler.internal_static_tensorflow_ProfileResponse_descriptor;
}
@java.lang.Override
public tensorflow.TpuProfiler.ProfileResponse getDefaultInstanceForType() {
return tensorflow.TpuProfiler.ProfileResponse.getDefaultInstance();
}
@java.lang.Override
public tensorflow.TpuProfiler.ProfileResponse build() {
tensorflow.TpuProfiler.ProfileResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.TpuProfiler.ProfileResponse buildPartial() {
tensorflow.TpuProfiler.ProfileResponse result = new tensorflow.TpuProfiler.ProfileResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (computationGraphBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
computationGraph_ = java.util.Collections.unmodifiableList(computationGraph_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.computationGraph_ = computationGraph_;
} else {
result.computationGraph_ = computationGraphBuilder_.build();
}
if (hloMetadataBuilder_ == null) {
result.hloMetadata_ = hloMetadata_;
} else {
result.hloMetadata_ = hloMetadataBuilder_.build();
}
result.encodedTrace_ = encodedTrace_;
if (opProfileBuilder_ == null) {
result.opProfile_ = opProfile_;
} else {
result.opProfile_ = opProfileBuilder_.build();
}
if (toolDataBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010)) {
toolData_ = java.util.Collections.unmodifiableList(toolData_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.toolData_ = toolData_;
} else {
result.toolData_ = toolDataBuilder_.build();
}
result.emptyTrace_ = emptyTrace_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.TpuProfiler.ProfileResponse) {
return mergeFrom((tensorflow.TpuProfiler.ProfileResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.TpuProfiler.ProfileResponse other) {
if (other == tensorflow.TpuProfiler.ProfileResponse.getDefaultInstance()) return this;
if (computationGraphBuilder_ == null) {
if (!other.computationGraph_.isEmpty()) {
if (computationGraph_.isEmpty()) {
computationGraph_ = other.computationGraph_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureComputationGraphIsMutable();
computationGraph_.addAll(other.computationGraph_);
}
onChanged();
}
} else {
if (!other.computationGraph_.isEmpty()) {
if (computationGraphBuilder_.isEmpty()) {
computationGraphBuilder_.dispose();
computationGraphBuilder_ = null;
computationGraph_ = other.computationGraph_;
bitField0_ = (bitField0_ & ~0x00000001);
computationGraphBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getComputationGraphFieldBuilder() : null;
} else {
computationGraphBuilder_.addAllMessages(other.computationGraph_);
}
}
}
if (other.hasHloMetadata()) {
mergeHloMetadata(other.getHloMetadata());
}
if (other.getEncodedTrace() != com.google.protobuf.ByteString.EMPTY) {
setEncodedTrace(other.getEncodedTrace());
}
if (other.hasOpProfile()) {
mergeOpProfile(other.getOpProfile());
}
if (toolDataBuilder_ == null) {
if (!other.toolData_.isEmpty()) {
if (toolData_.isEmpty()) {
toolData_ = other.toolData_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureToolDataIsMutable();
toolData_.addAll(other.toolData_);
}
onChanged();
}
} else {
if (!other.toolData_.isEmpty()) {
if (toolDataBuilder_.isEmpty()) {
toolDataBuilder_.dispose();
toolDataBuilder_ = null;
toolData_ = other.toolData_;
bitField0_ = (bitField0_ & ~0x00000010);
toolDataBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getToolDataFieldBuilder() : null;
} else {
toolDataBuilder_.addAllMessages(other.toolData_);
}
}
}
if (other.getEmptyTrace() != false) {
setEmptyTrace(other.getEmptyTrace());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.TpuProfiler.ProfileResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.TpuProfiler.ProfileResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List computationGraph_ =
java.util.Collections.emptyList();
private void ensureComputationGraphIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
computationGraph_ = new java.util.ArrayList(computationGraph_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.GraphDef, org.tensorflow.framework.GraphDef.Builder, org.tensorflow.framework.GraphDefOrBuilder> computationGraphBuilder_;
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
public java.util.List getComputationGraphList() {
if (computationGraphBuilder_ == null) {
return java.util.Collections.unmodifiableList(computationGraph_);
} else {
return computationGraphBuilder_.getMessageList();
}
}
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
public int getComputationGraphCount() {
if (computationGraphBuilder_ == null) {
return computationGraph_.size();
} else {
return computationGraphBuilder_.getCount();
}
}
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
public org.tensorflow.framework.GraphDef getComputationGraph(int index) {
if (computationGraphBuilder_ == null) {
return computationGraph_.get(index);
} else {
return computationGraphBuilder_.getMessage(index);
}
}
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
public Builder setComputationGraph(
int index, org.tensorflow.framework.GraphDef value) {
if (computationGraphBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureComputationGraphIsMutable();
computationGraph_.set(index, value);
onChanged();
} else {
computationGraphBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
public Builder setComputationGraph(
int index, org.tensorflow.framework.GraphDef.Builder builderForValue) {
if (computationGraphBuilder_ == null) {
ensureComputationGraphIsMutable();
computationGraph_.set(index, builderForValue.build());
onChanged();
} else {
computationGraphBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
public Builder addComputationGraph(org.tensorflow.framework.GraphDef value) {
if (computationGraphBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureComputationGraphIsMutable();
computationGraph_.add(value);
onChanged();
} else {
computationGraphBuilder_.addMessage(value);
}
return this;
}
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
public Builder addComputationGraph(
int index, org.tensorflow.framework.GraphDef value) {
if (computationGraphBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureComputationGraphIsMutable();
computationGraph_.add(index, value);
onChanged();
} else {
computationGraphBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
public Builder addComputationGraph(
org.tensorflow.framework.GraphDef.Builder builderForValue) {
if (computationGraphBuilder_ == null) {
ensureComputationGraphIsMutable();
computationGraph_.add(builderForValue.build());
onChanged();
} else {
computationGraphBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
public Builder addComputationGraph(
int index, org.tensorflow.framework.GraphDef.Builder builderForValue) {
if (computationGraphBuilder_ == null) {
ensureComputationGraphIsMutable();
computationGraph_.add(index, builderForValue.build());
onChanged();
} else {
computationGraphBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
public Builder addAllComputationGraph(
java.lang.Iterable extends org.tensorflow.framework.GraphDef> values) {
if (computationGraphBuilder_ == null) {
ensureComputationGraphIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, computationGraph_);
onChanged();
} else {
computationGraphBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
public Builder clearComputationGraph() {
if (computationGraphBuilder_ == null) {
computationGraph_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
computationGraphBuilder_.clear();
}
return this;
}
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
public Builder removeComputationGraph(int index) {
if (computationGraphBuilder_ == null) {
ensureComputationGraphIsMutable();
computationGraph_.remove(index);
onChanged();
} else {
computationGraphBuilder_.remove(index);
}
return this;
}
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
public org.tensorflow.framework.GraphDef.Builder getComputationGraphBuilder(
int index) {
return getComputationGraphFieldBuilder().getBuilder(index);
}
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
public org.tensorflow.framework.GraphDefOrBuilder getComputationGraphOrBuilder(
int index) {
if (computationGraphBuilder_ == null) {
return computationGraph_.get(index); } else {
return computationGraphBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
public java.util.List extends org.tensorflow.framework.GraphDefOrBuilder>
getComputationGraphOrBuilderList() {
if (computationGraphBuilder_ != null) {
return computationGraphBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(computationGraph_);
}
}
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
public org.tensorflow.framework.GraphDef.Builder addComputationGraphBuilder() {
return getComputationGraphFieldBuilder().addBuilder(
org.tensorflow.framework.GraphDef.getDefaultInstance());
}
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
public org.tensorflow.framework.GraphDef.Builder addComputationGraphBuilder(
int index) {
return getComputationGraphFieldBuilder().addBuilder(
index, org.tensorflow.framework.GraphDef.getDefaultInstance());
}
/**
*
* Graphs of programs executed on TPUs during the profiling period.
*
*
* repeated .tensorflow.GraphDef computation_graph = 2;
*/
public java.util.List
getComputationGraphBuilderList() {
return getComputationGraphFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.GraphDef, org.tensorflow.framework.GraphDef.Builder, org.tensorflow.framework.GraphDefOrBuilder>
getComputationGraphFieldBuilder() {
if (computationGraphBuilder_ == null) {
computationGraphBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.GraphDef, org.tensorflow.framework.GraphDef.Builder, org.tensorflow.framework.GraphDefOrBuilder>(
computationGraph_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
computationGraph_ = null;
}
return computationGraphBuilder_;
}
private org.tensorflow.framework.RunMetadata hloMetadata_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.RunMetadata, org.tensorflow.framework.RunMetadata.Builder, org.tensorflow.framework.RunMetadataOrBuilder> hloMetadataBuilder_;
/**
*
* Performance profile that can be used to annotate HLO operations in the
* computation graph.
*
*
* .tensorflow.RunMetadata hlo_metadata = 5;
*/
public boolean hasHloMetadata() {
return hloMetadataBuilder_ != null || hloMetadata_ != null;
}
/**
*
* Performance profile that can be used to annotate HLO operations in the
* computation graph.
*
*
* .tensorflow.RunMetadata hlo_metadata = 5;
*/
public org.tensorflow.framework.RunMetadata getHloMetadata() {
if (hloMetadataBuilder_ == null) {
return hloMetadata_ == null ? org.tensorflow.framework.RunMetadata.getDefaultInstance() : hloMetadata_;
} else {
return hloMetadataBuilder_.getMessage();
}
}
/**
*
* Performance profile that can be used to annotate HLO operations in the
* computation graph.
*
*
* .tensorflow.RunMetadata hlo_metadata = 5;
*/
public Builder setHloMetadata(org.tensorflow.framework.RunMetadata value) {
if (hloMetadataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
hloMetadata_ = value;
onChanged();
} else {
hloMetadataBuilder_.setMessage(value);
}
return this;
}
/**
*
* Performance profile that can be used to annotate HLO operations in the
* computation graph.
*
*
* .tensorflow.RunMetadata hlo_metadata = 5;
*/
public Builder setHloMetadata(
org.tensorflow.framework.RunMetadata.Builder builderForValue) {
if (hloMetadataBuilder_ == null) {
hloMetadata_ = builderForValue.build();
onChanged();
} else {
hloMetadataBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Performance profile that can be used to annotate HLO operations in the
* computation graph.
*
*
* .tensorflow.RunMetadata hlo_metadata = 5;
*/
public Builder mergeHloMetadata(org.tensorflow.framework.RunMetadata value) {
if (hloMetadataBuilder_ == null) {
if (hloMetadata_ != null) {
hloMetadata_ =
org.tensorflow.framework.RunMetadata.newBuilder(hloMetadata_).mergeFrom(value).buildPartial();
} else {
hloMetadata_ = value;
}
onChanged();
} else {
hloMetadataBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Performance profile that can be used to annotate HLO operations in the
* computation graph.
*
*
* .tensorflow.RunMetadata hlo_metadata = 5;
*/
public Builder clearHloMetadata() {
if (hloMetadataBuilder_ == null) {
hloMetadata_ = null;
onChanged();
} else {
hloMetadata_ = null;
hloMetadataBuilder_ = null;
}
return this;
}
/**
*
* Performance profile that can be used to annotate HLO operations in the
* computation graph.
*
*
* .tensorflow.RunMetadata hlo_metadata = 5;
*/
public org.tensorflow.framework.RunMetadata.Builder getHloMetadataBuilder() {
onChanged();
return getHloMetadataFieldBuilder().getBuilder();
}
/**
*
* Performance profile that can be used to annotate HLO operations in the
* computation graph.
*
*
* .tensorflow.RunMetadata hlo_metadata = 5;
*/
public org.tensorflow.framework.RunMetadataOrBuilder getHloMetadataOrBuilder() {
if (hloMetadataBuilder_ != null) {
return hloMetadataBuilder_.getMessageOrBuilder();
} else {
return hloMetadata_ == null ?
org.tensorflow.framework.RunMetadata.getDefaultInstance() : hloMetadata_;
}
}
/**
*
* Performance profile that can be used to annotate HLO operations in the
* computation graph.
*
*
* .tensorflow.RunMetadata hlo_metadata = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.RunMetadata, org.tensorflow.framework.RunMetadata.Builder, org.tensorflow.framework.RunMetadataOrBuilder>
getHloMetadataFieldBuilder() {
if (hloMetadataBuilder_ == null) {
hloMetadataBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.RunMetadata, org.tensorflow.framework.RunMetadata.Builder, org.tensorflow.framework.RunMetadataOrBuilder>(
getHloMetadata(),
getParentForChildren(),
isClean());
hloMetadata_ = null;
}
return hloMetadataBuilder_;
}
private com.google.protobuf.ByteString encodedTrace_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Encoded Trace proto message that contains metadata about the trace captured
* during the profiling period. Describes the devices and resources that
* 'trace_events' refers to.
*
*
* bytes encoded_trace = 3;
*/
public com.google.protobuf.ByteString getEncodedTrace() {
return encodedTrace_;
}
/**
*
* Encoded Trace proto message that contains metadata about the trace captured
* during the profiling period. Describes the devices and resources that
* 'trace_events' refers to.
*
*
* bytes encoded_trace = 3;
*/
public Builder setEncodedTrace(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
encodedTrace_ = value;
onChanged();
return this;
}
/**
*
* Encoded Trace proto message that contains metadata about the trace captured
* during the profiling period. Describes the devices and resources that
* 'trace_events' refers to.
*
*
* bytes encoded_trace = 3;
*/
public Builder clearEncodedTrace() {
encodedTrace_ = getDefaultInstance().getEncodedTrace();
onChanged();
return this;
}
private tensorflow.tpu.op_profile.OpProfile.Profile opProfile_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Profile, tensorflow.tpu.op_profile.OpProfile.Profile.Builder, tensorflow.tpu.op_profile.OpProfile.ProfileOrBuilder> opProfileBuilder_;
/**
*
* Assembles a hierarchical performance profile based on HLOs in trace events.
* If the trace covers multiple programs, the longest-running one is analyzed.
* See op_profile.proto for the detailed semantics of the returned profile.
*
*
* .tensorflow.tpu.op_profile.Profile op_profile = 4;
*/
public boolean hasOpProfile() {
return opProfileBuilder_ != null || opProfile_ != null;
}
/**
*
* Assembles a hierarchical performance profile based on HLOs in trace events.
* If the trace covers multiple programs, the longest-running one is analyzed.
* See op_profile.proto for the detailed semantics of the returned profile.
*
*
* .tensorflow.tpu.op_profile.Profile op_profile = 4;
*/
public tensorflow.tpu.op_profile.OpProfile.Profile getOpProfile() {
if (opProfileBuilder_ == null) {
return opProfile_ == null ? tensorflow.tpu.op_profile.OpProfile.Profile.getDefaultInstance() : opProfile_;
} else {
return opProfileBuilder_.getMessage();
}
}
/**
*
* Assembles a hierarchical performance profile based on HLOs in trace events.
* If the trace covers multiple programs, the longest-running one is analyzed.
* See op_profile.proto for the detailed semantics of the returned profile.
*
*
* .tensorflow.tpu.op_profile.Profile op_profile = 4;
*/
public Builder setOpProfile(tensorflow.tpu.op_profile.OpProfile.Profile value) {
if (opProfileBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
opProfile_ = value;
onChanged();
} else {
opProfileBuilder_.setMessage(value);
}
return this;
}
/**
*
* Assembles a hierarchical performance profile based on HLOs in trace events.
* If the trace covers multiple programs, the longest-running one is analyzed.
* See op_profile.proto for the detailed semantics of the returned profile.
*
*
* .tensorflow.tpu.op_profile.Profile op_profile = 4;
*/
public Builder setOpProfile(
tensorflow.tpu.op_profile.OpProfile.Profile.Builder builderForValue) {
if (opProfileBuilder_ == null) {
opProfile_ = builderForValue.build();
onChanged();
} else {
opProfileBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Assembles a hierarchical performance profile based on HLOs in trace events.
* If the trace covers multiple programs, the longest-running one is analyzed.
* See op_profile.proto for the detailed semantics of the returned profile.
*
*
* .tensorflow.tpu.op_profile.Profile op_profile = 4;
*/
public Builder mergeOpProfile(tensorflow.tpu.op_profile.OpProfile.Profile value) {
if (opProfileBuilder_ == null) {
if (opProfile_ != null) {
opProfile_ =
tensorflow.tpu.op_profile.OpProfile.Profile.newBuilder(opProfile_).mergeFrom(value).buildPartial();
} else {
opProfile_ = value;
}
onChanged();
} else {
opProfileBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Assembles a hierarchical performance profile based on HLOs in trace events.
* If the trace covers multiple programs, the longest-running one is analyzed.
* See op_profile.proto for the detailed semantics of the returned profile.
*
*
* .tensorflow.tpu.op_profile.Profile op_profile = 4;
*/
public Builder clearOpProfile() {
if (opProfileBuilder_ == null) {
opProfile_ = null;
onChanged();
} else {
opProfile_ = null;
opProfileBuilder_ = null;
}
return this;
}
/**
*
* Assembles a hierarchical performance profile based on HLOs in trace events.
* If the trace covers multiple programs, the longest-running one is analyzed.
* See op_profile.proto for the detailed semantics of the returned profile.
*
*
* .tensorflow.tpu.op_profile.Profile op_profile = 4;
*/
public tensorflow.tpu.op_profile.OpProfile.Profile.Builder getOpProfileBuilder() {
onChanged();
return getOpProfileFieldBuilder().getBuilder();
}
/**
*
* Assembles a hierarchical performance profile based on HLOs in trace events.
* If the trace covers multiple programs, the longest-running one is analyzed.
* See op_profile.proto for the detailed semantics of the returned profile.
*
*
* .tensorflow.tpu.op_profile.Profile op_profile = 4;
*/
public tensorflow.tpu.op_profile.OpProfile.ProfileOrBuilder getOpProfileOrBuilder() {
if (opProfileBuilder_ != null) {
return opProfileBuilder_.getMessageOrBuilder();
} else {
return opProfile_ == null ?
tensorflow.tpu.op_profile.OpProfile.Profile.getDefaultInstance() : opProfile_;
}
}
/**
*
* Assembles a hierarchical performance profile based on HLOs in trace events.
* If the trace covers multiple programs, the longest-running one is analyzed.
* See op_profile.proto for the detailed semantics of the returned profile.
*
*
* .tensorflow.tpu.op_profile.Profile op_profile = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Profile, tensorflow.tpu.op_profile.OpProfile.Profile.Builder, tensorflow.tpu.op_profile.OpProfile.ProfileOrBuilder>
getOpProfileFieldBuilder() {
if (opProfileBuilder_ == null) {
opProfileBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Profile, tensorflow.tpu.op_profile.OpProfile.Profile.Builder, tensorflow.tpu.op_profile.OpProfile.ProfileOrBuilder>(
getOpProfile(),
getParentForChildren(),
isClean());
opProfile_ = null;
}
return opProfileBuilder_;
}
private java.util.List toolData_ =
java.util.Collections.emptyList();
private void ensureToolDataIsMutable() {
if (!((bitField0_ & 0x00000010) == 0x00000010)) {
toolData_ = new java.util.ArrayList(toolData_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.TpuProfiler.ProfileToolData, tensorflow.TpuProfiler.ProfileToolData.Builder, tensorflow.TpuProfiler.ProfileToolDataOrBuilder> toolDataBuilder_;
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
public java.util.List getToolDataList() {
if (toolDataBuilder_ == null) {
return java.util.Collections.unmodifiableList(toolData_);
} else {
return toolDataBuilder_.getMessageList();
}
}
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
public int getToolDataCount() {
if (toolDataBuilder_ == null) {
return toolData_.size();
} else {
return toolDataBuilder_.getCount();
}
}
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
public tensorflow.TpuProfiler.ProfileToolData getToolData(int index) {
if (toolDataBuilder_ == null) {
return toolData_.get(index);
} else {
return toolDataBuilder_.getMessage(index);
}
}
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
public Builder setToolData(
int index, tensorflow.TpuProfiler.ProfileToolData value) {
if (toolDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureToolDataIsMutable();
toolData_.set(index, value);
onChanged();
} else {
toolDataBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
public Builder setToolData(
int index, tensorflow.TpuProfiler.ProfileToolData.Builder builderForValue) {
if (toolDataBuilder_ == null) {
ensureToolDataIsMutable();
toolData_.set(index, builderForValue.build());
onChanged();
} else {
toolDataBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
public Builder addToolData(tensorflow.TpuProfiler.ProfileToolData value) {
if (toolDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureToolDataIsMutable();
toolData_.add(value);
onChanged();
} else {
toolDataBuilder_.addMessage(value);
}
return this;
}
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
public Builder addToolData(
int index, tensorflow.TpuProfiler.ProfileToolData value) {
if (toolDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureToolDataIsMutable();
toolData_.add(index, value);
onChanged();
} else {
toolDataBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
public Builder addToolData(
tensorflow.TpuProfiler.ProfileToolData.Builder builderForValue) {
if (toolDataBuilder_ == null) {
ensureToolDataIsMutable();
toolData_.add(builderForValue.build());
onChanged();
} else {
toolDataBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
public Builder addToolData(
int index, tensorflow.TpuProfiler.ProfileToolData.Builder builderForValue) {
if (toolDataBuilder_ == null) {
ensureToolDataIsMutable();
toolData_.add(index, builderForValue.build());
onChanged();
} else {
toolDataBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
public Builder addAllToolData(
java.lang.Iterable extends tensorflow.TpuProfiler.ProfileToolData> values) {
if (toolDataBuilder_ == null) {
ensureToolDataIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, toolData_);
onChanged();
} else {
toolDataBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
public Builder clearToolData() {
if (toolDataBuilder_ == null) {
toolData_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
toolDataBuilder_.clear();
}
return this;
}
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
public Builder removeToolData(int index) {
if (toolDataBuilder_ == null) {
ensureToolDataIsMutable();
toolData_.remove(index);
onChanged();
} else {
toolDataBuilder_.remove(index);
}
return this;
}
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
public tensorflow.TpuProfiler.ProfileToolData.Builder getToolDataBuilder(
int index) {
return getToolDataFieldBuilder().getBuilder(index);
}
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
public tensorflow.TpuProfiler.ProfileToolDataOrBuilder getToolDataOrBuilder(
int index) {
if (toolDataBuilder_ == null) {
return toolData_.get(index); } else {
return toolDataBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
public java.util.List extends tensorflow.TpuProfiler.ProfileToolDataOrBuilder>
getToolDataOrBuilderList() {
if (toolDataBuilder_ != null) {
return toolDataBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(toolData_);
}
}
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
public tensorflow.TpuProfiler.ProfileToolData.Builder addToolDataBuilder() {
return getToolDataFieldBuilder().addBuilder(
tensorflow.TpuProfiler.ProfileToolData.getDefaultInstance());
}
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
public tensorflow.TpuProfiler.ProfileToolData.Builder addToolDataBuilder(
int index) {
return getToolDataFieldBuilder().addBuilder(
index, tensorflow.TpuProfiler.ProfileToolData.getDefaultInstance());
}
/**
*
* Data payload for each required tools.
*
*
* repeated .tensorflow.ProfileToolData tool_data = 6;
*/
public java.util.List
getToolDataBuilderList() {
return getToolDataFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.TpuProfiler.ProfileToolData, tensorflow.TpuProfiler.ProfileToolData.Builder, tensorflow.TpuProfiler.ProfileToolDataOrBuilder>
getToolDataFieldBuilder() {
if (toolDataBuilder_ == null) {
toolDataBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.TpuProfiler.ProfileToolData, tensorflow.TpuProfiler.ProfileToolData.Builder, tensorflow.TpuProfiler.ProfileToolDataOrBuilder>(
toolData_,
((bitField0_ & 0x00000010) == 0x00000010),
getParentForChildren(),
isClean());
toolData_ = null;
}
return toolDataBuilder_;
}
private boolean emptyTrace_ ;
/**
*
* When we write profiling data directly to repository directory, we need a
* way to figure out whether the captured trace is empty (due to idle TPU).
*
*
* bool empty_trace = 7;
*/
public boolean getEmptyTrace() {
return emptyTrace_;
}
/**
*
* When we write profiling data directly to repository directory, we need a
* way to figure out whether the captured trace is empty (due to idle TPU).
*
*
* bool empty_trace = 7;
*/
public Builder setEmptyTrace(boolean value) {
emptyTrace_ = value;
onChanged();
return this;
}
/**
*
* When we write profiling data directly to repository directory, we need a
* way to figure out whether the captured trace is empty (due to idle TPU).
*
*
* bool empty_trace = 7;
*/
public Builder clearEmptyTrace() {
emptyTrace_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.ProfileResponse)
}
// @@protoc_insertion_point(class_scope:tensorflow.ProfileResponse)
private static final tensorflow.TpuProfiler.ProfileResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.TpuProfiler.ProfileResponse();
}
public static tensorflow.TpuProfiler.ProfileResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ProfileResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ProfileResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.TpuProfiler.ProfileResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MonitorRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.MonitorRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* Duration for which to profile between each update.
*
*
* uint64 duration_ms = 1;
*/
long getDurationMs();
/**
*
* Indicates the level at which we want to monitor. Currently, two levels are
* supported:
* Level 1: An ultra lightweight mode that captures only some utilization
* metrics.
* Level 2: More verbose than level 1. Collects utilization metrics, device
* information, step time information, etc. Do not use this option if the TPU
* host is being very heavily used.
*
*
* int32 monitoring_level = 2;
*/
int getMonitoringLevel();
}
/**
* Protobuf type {@code tensorflow.MonitorRequest}
*/
public static final class MonitorRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.MonitorRequest)
MonitorRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use MonitorRequest.newBuilder() to construct.
private MonitorRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MonitorRequest() {
durationMs_ = 0L;
monitoringLevel_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MonitorRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
durationMs_ = input.readUInt64();
break;
}
case 16: {
monitoringLevel_ = input.readInt32();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfiler.internal_static_tensorflow_MonitorRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfiler.internal_static_tensorflow_MonitorRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfiler.MonitorRequest.class, tensorflow.TpuProfiler.MonitorRequest.Builder.class);
}
public static final int DURATION_MS_FIELD_NUMBER = 1;
private long durationMs_;
/**
*
* Duration for which to profile between each update.
*
*
* uint64 duration_ms = 1;
*/
public long getDurationMs() {
return durationMs_;
}
public static final int MONITORING_LEVEL_FIELD_NUMBER = 2;
private int monitoringLevel_;
/**
*
* Indicates the level at which we want to monitor. Currently, two levels are
* supported:
* Level 1: An ultra lightweight mode that captures only some utilization
* metrics.
* Level 2: More verbose than level 1. Collects utilization metrics, device
* information, step time information, etc. Do not use this option if the TPU
* host is being very heavily used.
*
*
* int32 monitoring_level = 2;
*/
public int getMonitoringLevel() {
return monitoringLevel_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (durationMs_ != 0L) {
output.writeUInt64(1, durationMs_);
}
if (monitoringLevel_ != 0) {
output.writeInt32(2, monitoringLevel_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (durationMs_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, durationMs_);
}
if (monitoringLevel_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, monitoringLevel_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.TpuProfiler.MonitorRequest)) {
return super.equals(obj);
}
tensorflow.TpuProfiler.MonitorRequest other = (tensorflow.TpuProfiler.MonitorRequest) obj;
boolean result = true;
result = result && (getDurationMs()
== other.getDurationMs());
result = result && (getMonitoringLevel()
== other.getMonitoringLevel());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + DURATION_MS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getDurationMs());
hash = (37 * hash) + MONITORING_LEVEL_FIELD_NUMBER;
hash = (53 * hash) + getMonitoringLevel();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.TpuProfiler.MonitorRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfiler.MonitorRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfiler.MonitorRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfiler.MonitorRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfiler.MonitorRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfiler.MonitorRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfiler.MonitorRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfiler.MonitorRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfiler.MonitorRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.TpuProfiler.MonitorRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfiler.MonitorRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfiler.MonitorRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.TpuProfiler.MonitorRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.MonitorRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.MonitorRequest)
tensorflow.TpuProfiler.MonitorRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfiler.internal_static_tensorflow_MonitorRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfiler.internal_static_tensorflow_MonitorRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfiler.MonitorRequest.class, tensorflow.TpuProfiler.MonitorRequest.Builder.class);
}
// Construct using tensorflow.TpuProfiler.MonitorRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
durationMs_ = 0L;
monitoringLevel_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.TpuProfiler.internal_static_tensorflow_MonitorRequest_descriptor;
}
@java.lang.Override
public tensorflow.TpuProfiler.MonitorRequest getDefaultInstanceForType() {
return tensorflow.TpuProfiler.MonitorRequest.getDefaultInstance();
}
@java.lang.Override
public tensorflow.TpuProfiler.MonitorRequest build() {
tensorflow.TpuProfiler.MonitorRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.TpuProfiler.MonitorRequest buildPartial() {
tensorflow.TpuProfiler.MonitorRequest result = new tensorflow.TpuProfiler.MonitorRequest(this);
result.durationMs_ = durationMs_;
result.monitoringLevel_ = monitoringLevel_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.TpuProfiler.MonitorRequest) {
return mergeFrom((tensorflow.TpuProfiler.MonitorRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.TpuProfiler.MonitorRequest other) {
if (other == tensorflow.TpuProfiler.MonitorRequest.getDefaultInstance()) return this;
if (other.getDurationMs() != 0L) {
setDurationMs(other.getDurationMs());
}
if (other.getMonitoringLevel() != 0) {
setMonitoringLevel(other.getMonitoringLevel());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.TpuProfiler.MonitorRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.TpuProfiler.MonitorRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long durationMs_ ;
/**
*
* Duration for which to profile between each update.
*
*
* uint64 duration_ms = 1;
*/
public long getDurationMs() {
return durationMs_;
}
/**
*
* Duration for which to profile between each update.
*
*
* uint64 duration_ms = 1;
*/
public Builder setDurationMs(long value) {
durationMs_ = value;
onChanged();
return this;
}
/**
*
* Duration for which to profile between each update.
*
*
* uint64 duration_ms = 1;
*/
public Builder clearDurationMs() {
durationMs_ = 0L;
onChanged();
return this;
}
private int monitoringLevel_ ;
/**
*
* Indicates the level at which we want to monitor. Currently, two levels are
* supported:
* Level 1: An ultra lightweight mode that captures only some utilization
* metrics.
* Level 2: More verbose than level 1. Collects utilization metrics, device
* information, step time information, etc. Do not use this option if the TPU
* host is being very heavily used.
*
*
* int32 monitoring_level = 2;
*/
public int getMonitoringLevel() {
return monitoringLevel_;
}
/**
*
* Indicates the level at which we want to monitor. Currently, two levels are
* supported:
* Level 1: An ultra lightweight mode that captures only some utilization
* metrics.
* Level 2: More verbose than level 1. Collects utilization metrics, device
* information, step time information, etc. Do not use this option if the TPU
* host is being very heavily used.
*
*
* int32 monitoring_level = 2;
*/
public Builder setMonitoringLevel(int value) {
monitoringLevel_ = value;
onChanged();
return this;
}
/**
*
* Indicates the level at which we want to monitor. Currently, two levels are
* supported:
* Level 1: An ultra lightweight mode that captures only some utilization
* metrics.
* Level 2: More verbose than level 1. Collects utilization metrics, device
* information, step time information, etc. Do not use this option if the TPU
* host is being very heavily used.
*
*
* int32 monitoring_level = 2;
*/
public Builder clearMonitoringLevel() {
monitoringLevel_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.MonitorRequest)
}
// @@protoc_insertion_point(class_scope:tensorflow.MonitorRequest)
private static final tensorflow.TpuProfiler.MonitorRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.TpuProfiler.MonitorRequest();
}
public static tensorflow.TpuProfiler.MonitorRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MonitorRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MonitorRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.TpuProfiler.MonitorRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MonitorResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.MonitorResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* Properly formatted string data that can be directly returned back to user.
*
*
* string data = 1;
*/
java.lang.String getData();
/**
*
* Properly formatted string data that can be directly returned back to user.
*
*
* string data = 1;
*/
com.google.protobuf.ByteString
getDataBytes();
}
/**
* Protobuf type {@code tensorflow.MonitorResponse}
*/
public static final class MonitorResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.MonitorResponse)
MonitorResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use MonitorResponse.newBuilder() to construct.
private MonitorResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MonitorResponse() {
data_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MonitorResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
data_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfiler.internal_static_tensorflow_MonitorResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfiler.internal_static_tensorflow_MonitorResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfiler.MonitorResponse.class, tensorflow.TpuProfiler.MonitorResponse.Builder.class);
}
public static final int DATA_FIELD_NUMBER = 1;
private volatile java.lang.Object data_;
/**
*
* Properly formatted string data that can be directly returned back to user.
*
*
* string data = 1;
*/
public java.lang.String getData() {
java.lang.Object ref = data_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
data_ = s;
return s;
}
}
/**
*
* Properly formatted string data that can be directly returned back to user.
*
*
* string data = 1;
*/
public com.google.protobuf.ByteString
getDataBytes() {
java.lang.Object ref = data_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
data_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getDataBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, data_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getDataBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, data_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.TpuProfiler.MonitorResponse)) {
return super.equals(obj);
}
tensorflow.TpuProfiler.MonitorResponse other = (tensorflow.TpuProfiler.MonitorResponse) obj;
boolean result = true;
result = result && getData()
.equals(other.getData());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.TpuProfiler.MonitorResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfiler.MonitorResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfiler.MonitorResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfiler.MonitorResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfiler.MonitorResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfiler.MonitorResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfiler.MonitorResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfiler.MonitorResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfiler.MonitorResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.TpuProfiler.MonitorResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfiler.MonitorResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfiler.MonitorResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.TpuProfiler.MonitorResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.MonitorResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.MonitorResponse)
tensorflow.TpuProfiler.MonitorResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfiler.internal_static_tensorflow_MonitorResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfiler.internal_static_tensorflow_MonitorResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfiler.MonitorResponse.class, tensorflow.TpuProfiler.MonitorResponse.Builder.class);
}
// Construct using tensorflow.TpuProfiler.MonitorResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
data_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.TpuProfiler.internal_static_tensorflow_MonitorResponse_descriptor;
}
@java.lang.Override
public tensorflow.TpuProfiler.MonitorResponse getDefaultInstanceForType() {
return tensorflow.TpuProfiler.MonitorResponse.getDefaultInstance();
}
@java.lang.Override
public tensorflow.TpuProfiler.MonitorResponse build() {
tensorflow.TpuProfiler.MonitorResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.TpuProfiler.MonitorResponse buildPartial() {
tensorflow.TpuProfiler.MonitorResponse result = new tensorflow.TpuProfiler.MonitorResponse(this);
result.data_ = data_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.TpuProfiler.MonitorResponse) {
return mergeFrom((tensorflow.TpuProfiler.MonitorResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.TpuProfiler.MonitorResponse other) {
if (other == tensorflow.TpuProfiler.MonitorResponse.getDefaultInstance()) return this;
if (!other.getData().isEmpty()) {
data_ = other.data_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.TpuProfiler.MonitorResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.TpuProfiler.MonitorResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object data_ = "";
/**
*
* Properly formatted string data that can be directly returned back to user.
*
*
* string data = 1;
*/
public java.lang.String getData() {
java.lang.Object ref = data_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
data_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Properly formatted string data that can be directly returned back to user.
*
*
* string data = 1;
*/
public com.google.protobuf.ByteString
getDataBytes() {
java.lang.Object ref = data_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
data_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Properly formatted string data that can be directly returned back to user.
*
*
* string data = 1;
*/
public Builder setData(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
data_ = value;
onChanged();
return this;
}
/**
*
* Properly formatted string data that can be directly returned back to user.
*
*
* string data = 1;
*/
public Builder clearData() {
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
/**
*
* Properly formatted string data that can be directly returned back to user.
*
*
* string data = 1;
*/
public Builder setDataBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
data_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.MonitorResponse)
}
// @@protoc_insertion_point(class_scope:tensorflow.MonitorResponse)
private static final tensorflow.TpuProfiler.MonitorResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.TpuProfiler.MonitorResponse();
}
public static tensorflow.TpuProfiler.MonitorResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MonitorResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MonitorResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.TpuProfiler.MonitorResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_ProfileOptions_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_ProfileOptions_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_ToolRequestOptions_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_ToolRequestOptions_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_ProfileRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_ProfileRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_ProfileRequest_ToolOptionsEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_ProfileRequest_ToolOptionsEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_ProfileToolData_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_ProfileToolData_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_ProfileResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_ProfileResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_MonitorRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_MonitorRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_MonitorResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_MonitorResponse_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n2tensorflow/contrib/tpu/profiler/tpu_pr" +
"ofiler.proto\022\ntensorflow\032%tensorflow/cor" +
"e/framework/graph.proto\032%tensorflow/core" +
"/protobuf/config.proto\0320tensorflow/contr" +
"ib/tpu/profiler/op_profile.proto\"-\n\016Prof" +
"ileOptions\022\033\n\023include_dataset_ops\030\001 \001(\010\"" +
"B\n\022ToolRequestOptions\022\026\n\016output_formats\030" +
"\002 \001(\t\022\024\n\014save_to_repo\030\003 \001(\010\"\311\002\n\016ProfileR" +
"equest\022\023\n\013duration_ms\030\001 \001(\004\022\022\n\nmax_event" +
"s\030\002 \001(\004\022\r\n\005tools\030\003 \003(\t\022A\n\014tool_options\030\010" +
" \003(\0132+.tensorflow.ProfileRequest.ToolOpt" +
"ionsEntry\022(\n\004opts\030\004 \001(\0132\032.tensorflow.Pro" +
"fileOptions\022\027\n\017repository_root\030\005 \001(\t\022\022\n\n" +
"session_id\030\006 \001(\t\022\021\n\thost_name\030\007 \001(\t\032R\n\020T" +
"oolOptionsEntry\022\013\n\003key\030\001 \001(\t\022-\n\005value\030\002 " +
"\001(\0132\036.tensorflow.ToolRequestOptions:\0028\001\"" +
"-\n\017ProfileToolData\022\014\n\004name\030\001 \001(\t\022\014\n\004data" +
"\030\002 \001(\014\"\213\002\n\017ProfileResponse\022/\n\021computatio" +
"n_graph\030\002 \003(\0132\024.tensorflow.GraphDef\022-\n\014h" +
"lo_metadata\030\005 \001(\0132\027.tensorflow.RunMetada" +
"ta\022\025\n\rencoded_trace\030\003 \001(\014\0226\n\nop_profile\030" +
"\004 \001(\0132\".tensorflow.tpu.op_profile.Profil" +
"e\022.\n\ttool_data\030\006 \003(\0132\033.tensorflow.Profil" +
"eToolData\022\023\n\013empty_trace\030\007 \001(\010J\004\010\001\020\002\"?\n\016" +
"MonitorRequest\022\023\n\013duration_ms\030\001 \001(\004\022\030\n\020m" +
"onitoring_level\030\002 \001(\005\"\037\n\017MonitorResponse" +
"\022\014\n\004data\030\001 \001(\t2\231\001\n\013TPUProfiler\022D\n\007Profil" +
"e\022\032.tensorflow.ProfileRequest\032\033.tensorfl" +
"ow.ProfileResponse\"\000\022D\n\007Monitor\022\032.tensor" +
"flow.MonitorRequest\032\033.tensorflow.Monitor" +
"Response\"\000b\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
org.tensorflow.framework.GraphProtos.getDescriptor(),
org.tensorflow.framework.ConfigProtos.getDescriptor(),
tensorflow.tpu.op_profile.OpProfile.getDescriptor(),
}, assigner);
internal_static_tensorflow_ProfileOptions_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_tensorflow_ProfileOptions_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_ProfileOptions_descriptor,
new java.lang.String[] { "IncludeDatasetOps", });
internal_static_tensorflow_ToolRequestOptions_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_tensorflow_ToolRequestOptions_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_ToolRequestOptions_descriptor,
new java.lang.String[] { "OutputFormats", "SaveToRepo", });
internal_static_tensorflow_ProfileRequest_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_tensorflow_ProfileRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_ProfileRequest_descriptor,
new java.lang.String[] { "DurationMs", "MaxEvents", "Tools", "ToolOptions", "Opts", "RepositoryRoot", "SessionId", "HostName", });
internal_static_tensorflow_ProfileRequest_ToolOptionsEntry_descriptor =
internal_static_tensorflow_ProfileRequest_descriptor.getNestedTypes().get(0);
internal_static_tensorflow_ProfileRequest_ToolOptionsEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_ProfileRequest_ToolOptionsEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_tensorflow_ProfileToolData_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_tensorflow_ProfileToolData_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_ProfileToolData_descriptor,
new java.lang.String[] { "Name", "Data", });
internal_static_tensorflow_ProfileResponse_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_tensorflow_ProfileResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_ProfileResponse_descriptor,
new java.lang.String[] { "ComputationGraph", "HloMetadata", "EncodedTrace", "OpProfile", "ToolData", "EmptyTrace", });
internal_static_tensorflow_MonitorRequest_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_tensorflow_MonitorRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_MonitorRequest_descriptor,
new java.lang.String[] { "DurationMs", "MonitoringLevel", });
internal_static_tensorflow_MonitorResponse_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_tensorflow_MonitorResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_MonitorResponse_descriptor,
new java.lang.String[] { "Data", });
org.tensorflow.framework.GraphProtos.getDescriptor();
org.tensorflow.framework.ConfigProtos.getDescriptor();
tensorflow.tpu.op_profile.OpProfile.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy