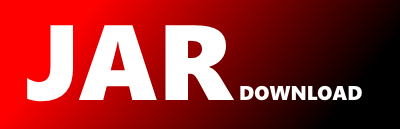
tensorflow.TpuProfilerAnalysis Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: tensorflow/contrib/tpu/profiler/tpu_profiler_analysis.proto
package tensorflow;
public final class TpuProfilerAnalysis {
private TpuProfilerAnalysis() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface NewProfileSessionRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.NewProfileSessionRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .tensorflow.ProfileRequest request = 1;
*/
boolean hasRequest();
/**
* .tensorflow.ProfileRequest request = 1;
*/
tensorflow.TpuProfiler.ProfileRequest getRequest();
/**
* .tensorflow.ProfileRequest request = 1;
*/
tensorflow.TpuProfiler.ProfileRequestOrBuilder getRequestOrBuilder();
/**
* string repository_root = 2;
*/
java.lang.String getRepositoryRoot();
/**
* string repository_root = 2;
*/
com.google.protobuf.ByteString
getRepositoryRootBytes();
/**
* repeated string hosts = 3;
*/
java.util.List
getHostsList();
/**
* repeated string hosts = 3;
*/
int getHostsCount();
/**
* repeated string hosts = 3;
*/
java.lang.String getHosts(int index);
/**
* repeated string hosts = 3;
*/
com.google.protobuf.ByteString
getHostsBytes(int index);
/**
* string session_id = 4;
*/
java.lang.String getSessionId();
/**
* string session_id = 4;
*/
com.google.protobuf.ByteString
getSessionIdBytes();
}
/**
* Protobuf type {@code tensorflow.NewProfileSessionRequest}
*/
public static final class NewProfileSessionRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.NewProfileSessionRequest)
NewProfileSessionRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use NewProfileSessionRequest.newBuilder() to construct.
private NewProfileSessionRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private NewProfileSessionRequest() {
repositoryRoot_ = "";
hosts_ = com.google.protobuf.LazyStringArrayList.EMPTY;
sessionId_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private NewProfileSessionRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
tensorflow.TpuProfiler.ProfileRequest.Builder subBuilder = null;
if (request_ != null) {
subBuilder = request_.toBuilder();
}
request_ = input.readMessage(tensorflow.TpuProfiler.ProfileRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(request_);
request_ = subBuilder.buildPartial();
}
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
repositoryRoot_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
hosts_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000004;
}
hosts_.add(s);
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
sessionId_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
hosts_ = hosts_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_NewProfileSessionRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_NewProfileSessionRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest.class, tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest.Builder.class);
}
private int bitField0_;
public static final int REQUEST_FIELD_NUMBER = 1;
private tensorflow.TpuProfiler.ProfileRequest request_;
/**
* .tensorflow.ProfileRequest request = 1;
*/
public boolean hasRequest() {
return request_ != null;
}
/**
* .tensorflow.ProfileRequest request = 1;
*/
public tensorflow.TpuProfiler.ProfileRequest getRequest() {
return request_ == null ? tensorflow.TpuProfiler.ProfileRequest.getDefaultInstance() : request_;
}
/**
* .tensorflow.ProfileRequest request = 1;
*/
public tensorflow.TpuProfiler.ProfileRequestOrBuilder getRequestOrBuilder() {
return getRequest();
}
public static final int REPOSITORY_ROOT_FIELD_NUMBER = 2;
private volatile java.lang.Object repositoryRoot_;
/**
* string repository_root = 2;
*/
public java.lang.String getRepositoryRoot() {
java.lang.Object ref = repositoryRoot_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
repositoryRoot_ = s;
return s;
}
}
/**
* string repository_root = 2;
*/
public com.google.protobuf.ByteString
getRepositoryRootBytes() {
java.lang.Object ref = repositoryRoot_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
repositoryRoot_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int HOSTS_FIELD_NUMBER = 3;
private com.google.protobuf.LazyStringList hosts_;
/**
* repeated string hosts = 3;
*/
public com.google.protobuf.ProtocolStringList
getHostsList() {
return hosts_;
}
/**
* repeated string hosts = 3;
*/
public int getHostsCount() {
return hosts_.size();
}
/**
* repeated string hosts = 3;
*/
public java.lang.String getHosts(int index) {
return hosts_.get(index);
}
/**
* repeated string hosts = 3;
*/
public com.google.protobuf.ByteString
getHostsBytes(int index) {
return hosts_.getByteString(index);
}
public static final int SESSION_ID_FIELD_NUMBER = 4;
private volatile java.lang.Object sessionId_;
/**
* string session_id = 4;
*/
public java.lang.String getSessionId() {
java.lang.Object ref = sessionId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sessionId_ = s;
return s;
}
}
/**
* string session_id = 4;
*/
public com.google.protobuf.ByteString
getSessionIdBytes() {
java.lang.Object ref = sessionId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sessionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (request_ != null) {
output.writeMessage(1, getRequest());
}
if (!getRepositoryRootBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, repositoryRoot_);
}
for (int i = 0; i < hosts_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, hosts_.getRaw(i));
}
if (!getSessionIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, sessionId_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (request_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getRequest());
}
if (!getRepositoryRootBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, repositoryRoot_);
}
{
int dataSize = 0;
for (int i = 0; i < hosts_.size(); i++) {
dataSize += computeStringSizeNoTag(hosts_.getRaw(i));
}
size += dataSize;
size += 1 * getHostsList().size();
}
if (!getSessionIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, sessionId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest)) {
return super.equals(obj);
}
tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest other = (tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest) obj;
boolean result = true;
result = result && (hasRequest() == other.hasRequest());
if (hasRequest()) {
result = result && getRequest()
.equals(other.getRequest());
}
result = result && getRepositoryRoot()
.equals(other.getRepositoryRoot());
result = result && getHostsList()
.equals(other.getHostsList());
result = result && getSessionId()
.equals(other.getSessionId());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasRequest()) {
hash = (37 * hash) + REQUEST_FIELD_NUMBER;
hash = (53 * hash) + getRequest().hashCode();
}
hash = (37 * hash) + REPOSITORY_ROOT_FIELD_NUMBER;
hash = (53 * hash) + getRepositoryRoot().hashCode();
if (getHostsCount() > 0) {
hash = (37 * hash) + HOSTS_FIELD_NUMBER;
hash = (53 * hash) + getHostsList().hashCode();
}
hash = (37 * hash) + SESSION_ID_FIELD_NUMBER;
hash = (53 * hash) + getSessionId().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.NewProfileSessionRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.NewProfileSessionRequest)
tensorflow.TpuProfilerAnalysis.NewProfileSessionRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_NewProfileSessionRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_NewProfileSessionRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest.class, tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest.Builder.class);
}
// Construct using tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (requestBuilder_ == null) {
request_ = null;
} else {
request_ = null;
requestBuilder_ = null;
}
repositoryRoot_ = "";
hosts_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
sessionId_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_NewProfileSessionRequest_descriptor;
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest getDefaultInstanceForType() {
return tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest.getDefaultInstance();
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest build() {
tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest buildPartial() {
tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest result = new tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (requestBuilder_ == null) {
result.request_ = request_;
} else {
result.request_ = requestBuilder_.build();
}
result.repositoryRoot_ = repositoryRoot_;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
hosts_ = hosts_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000004);
}
result.hosts_ = hosts_;
result.sessionId_ = sessionId_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest) {
return mergeFrom((tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest other) {
if (other == tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest.getDefaultInstance()) return this;
if (other.hasRequest()) {
mergeRequest(other.getRequest());
}
if (!other.getRepositoryRoot().isEmpty()) {
repositoryRoot_ = other.repositoryRoot_;
onChanged();
}
if (!other.hosts_.isEmpty()) {
if (hosts_.isEmpty()) {
hosts_ = other.hosts_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureHostsIsMutable();
hosts_.addAll(other.hosts_);
}
onChanged();
}
if (!other.getSessionId().isEmpty()) {
sessionId_ = other.sessionId_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private tensorflow.TpuProfiler.ProfileRequest request_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.TpuProfiler.ProfileRequest, tensorflow.TpuProfiler.ProfileRequest.Builder, tensorflow.TpuProfiler.ProfileRequestOrBuilder> requestBuilder_;
/**
* .tensorflow.ProfileRequest request = 1;
*/
public boolean hasRequest() {
return requestBuilder_ != null || request_ != null;
}
/**
* .tensorflow.ProfileRequest request = 1;
*/
public tensorflow.TpuProfiler.ProfileRequest getRequest() {
if (requestBuilder_ == null) {
return request_ == null ? tensorflow.TpuProfiler.ProfileRequest.getDefaultInstance() : request_;
} else {
return requestBuilder_.getMessage();
}
}
/**
* .tensorflow.ProfileRequest request = 1;
*/
public Builder setRequest(tensorflow.TpuProfiler.ProfileRequest value) {
if (requestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
request_ = value;
onChanged();
} else {
requestBuilder_.setMessage(value);
}
return this;
}
/**
* .tensorflow.ProfileRequest request = 1;
*/
public Builder setRequest(
tensorflow.TpuProfiler.ProfileRequest.Builder builderForValue) {
if (requestBuilder_ == null) {
request_ = builderForValue.build();
onChanged();
} else {
requestBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .tensorflow.ProfileRequest request = 1;
*/
public Builder mergeRequest(tensorflow.TpuProfiler.ProfileRequest value) {
if (requestBuilder_ == null) {
if (request_ != null) {
request_ =
tensorflow.TpuProfiler.ProfileRequest.newBuilder(request_).mergeFrom(value).buildPartial();
} else {
request_ = value;
}
onChanged();
} else {
requestBuilder_.mergeFrom(value);
}
return this;
}
/**
* .tensorflow.ProfileRequest request = 1;
*/
public Builder clearRequest() {
if (requestBuilder_ == null) {
request_ = null;
onChanged();
} else {
request_ = null;
requestBuilder_ = null;
}
return this;
}
/**
* .tensorflow.ProfileRequest request = 1;
*/
public tensorflow.TpuProfiler.ProfileRequest.Builder getRequestBuilder() {
onChanged();
return getRequestFieldBuilder().getBuilder();
}
/**
* .tensorflow.ProfileRequest request = 1;
*/
public tensorflow.TpuProfiler.ProfileRequestOrBuilder getRequestOrBuilder() {
if (requestBuilder_ != null) {
return requestBuilder_.getMessageOrBuilder();
} else {
return request_ == null ?
tensorflow.TpuProfiler.ProfileRequest.getDefaultInstance() : request_;
}
}
/**
* .tensorflow.ProfileRequest request = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.TpuProfiler.ProfileRequest, tensorflow.TpuProfiler.ProfileRequest.Builder, tensorflow.TpuProfiler.ProfileRequestOrBuilder>
getRequestFieldBuilder() {
if (requestBuilder_ == null) {
requestBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.TpuProfiler.ProfileRequest, tensorflow.TpuProfiler.ProfileRequest.Builder, tensorflow.TpuProfiler.ProfileRequestOrBuilder>(
getRequest(),
getParentForChildren(),
isClean());
request_ = null;
}
return requestBuilder_;
}
private java.lang.Object repositoryRoot_ = "";
/**
* string repository_root = 2;
*/
public java.lang.String getRepositoryRoot() {
java.lang.Object ref = repositoryRoot_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
repositoryRoot_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string repository_root = 2;
*/
public com.google.protobuf.ByteString
getRepositoryRootBytes() {
java.lang.Object ref = repositoryRoot_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
repositoryRoot_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string repository_root = 2;
*/
public Builder setRepositoryRoot(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
repositoryRoot_ = value;
onChanged();
return this;
}
/**
* string repository_root = 2;
*/
public Builder clearRepositoryRoot() {
repositoryRoot_ = getDefaultInstance().getRepositoryRoot();
onChanged();
return this;
}
/**
* string repository_root = 2;
*/
public Builder setRepositoryRootBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
repositoryRoot_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList hosts_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureHostsIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
hosts_ = new com.google.protobuf.LazyStringArrayList(hosts_);
bitField0_ |= 0x00000004;
}
}
/**
* repeated string hosts = 3;
*/
public com.google.protobuf.ProtocolStringList
getHostsList() {
return hosts_.getUnmodifiableView();
}
/**
* repeated string hosts = 3;
*/
public int getHostsCount() {
return hosts_.size();
}
/**
* repeated string hosts = 3;
*/
public java.lang.String getHosts(int index) {
return hosts_.get(index);
}
/**
* repeated string hosts = 3;
*/
public com.google.protobuf.ByteString
getHostsBytes(int index) {
return hosts_.getByteString(index);
}
/**
* repeated string hosts = 3;
*/
public Builder setHosts(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureHostsIsMutable();
hosts_.set(index, value);
onChanged();
return this;
}
/**
* repeated string hosts = 3;
*/
public Builder addHosts(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureHostsIsMutable();
hosts_.add(value);
onChanged();
return this;
}
/**
* repeated string hosts = 3;
*/
public Builder addAllHosts(
java.lang.Iterable values) {
ensureHostsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, hosts_);
onChanged();
return this;
}
/**
* repeated string hosts = 3;
*/
public Builder clearHosts() {
hosts_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
* repeated string hosts = 3;
*/
public Builder addHostsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureHostsIsMutable();
hosts_.add(value);
onChanged();
return this;
}
private java.lang.Object sessionId_ = "";
/**
* string session_id = 4;
*/
public java.lang.String getSessionId() {
java.lang.Object ref = sessionId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sessionId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string session_id = 4;
*/
public com.google.protobuf.ByteString
getSessionIdBytes() {
java.lang.Object ref = sessionId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sessionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string session_id = 4;
*/
public Builder setSessionId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sessionId_ = value;
onChanged();
return this;
}
/**
* string session_id = 4;
*/
public Builder clearSessionId() {
sessionId_ = getDefaultInstance().getSessionId();
onChanged();
return this;
}
/**
* string session_id = 4;
*/
public Builder setSessionIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sessionId_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.NewProfileSessionRequest)
}
// @@protoc_insertion_point(class_scope:tensorflow.NewProfileSessionRequest)
private static final tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest();
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public NewProfileSessionRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new NewProfileSessionRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.NewProfileSessionRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface NewProfileSessionResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.NewProfileSessionResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
java.lang.String getErrorMessage();
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
com.google.protobuf.ByteString
getErrorMessageBytes();
/**
*
* Whether all hosts had returned a empty trace.
*
*
* bool empty_trace = 2;
*/
boolean getEmptyTrace();
}
/**
* Protobuf type {@code tensorflow.NewProfileSessionResponse}
*/
public static final class NewProfileSessionResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.NewProfileSessionResponse)
NewProfileSessionResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use NewProfileSessionResponse.newBuilder() to construct.
private NewProfileSessionResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private NewProfileSessionResponse() {
errorMessage_ = "";
emptyTrace_ = false;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private NewProfileSessionResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
errorMessage_ = s;
break;
}
case 16: {
emptyTrace_ = input.readBool();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_NewProfileSessionResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_NewProfileSessionResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse.class, tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse.Builder.class);
}
public static final int ERROR_MESSAGE_FIELD_NUMBER = 1;
private volatile java.lang.Object errorMessage_;
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
public java.lang.String getErrorMessage() {
java.lang.Object ref = errorMessage_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
errorMessage_ = s;
return s;
}
}
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
public com.google.protobuf.ByteString
getErrorMessageBytes() {
java.lang.Object ref = errorMessage_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
errorMessage_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int EMPTY_TRACE_FIELD_NUMBER = 2;
private boolean emptyTrace_;
/**
*
* Whether all hosts had returned a empty trace.
*
*
* bool empty_trace = 2;
*/
public boolean getEmptyTrace() {
return emptyTrace_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getErrorMessageBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, errorMessage_);
}
if (emptyTrace_ != false) {
output.writeBool(2, emptyTrace_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getErrorMessageBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, errorMessage_);
}
if (emptyTrace_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, emptyTrace_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse)) {
return super.equals(obj);
}
tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse other = (tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse) obj;
boolean result = true;
result = result && getErrorMessage()
.equals(other.getErrorMessage());
result = result && (getEmptyTrace()
== other.getEmptyTrace());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ERROR_MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getErrorMessage().hashCode();
hash = (37 * hash) + EMPTY_TRACE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getEmptyTrace());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.NewProfileSessionResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.NewProfileSessionResponse)
tensorflow.TpuProfilerAnalysis.NewProfileSessionResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_NewProfileSessionResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_NewProfileSessionResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse.class, tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse.Builder.class);
}
// Construct using tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
errorMessage_ = "";
emptyTrace_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_NewProfileSessionResponse_descriptor;
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse getDefaultInstanceForType() {
return tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse.getDefaultInstance();
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse build() {
tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse buildPartial() {
tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse result = new tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse(this);
result.errorMessage_ = errorMessage_;
result.emptyTrace_ = emptyTrace_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse) {
return mergeFrom((tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse other) {
if (other == tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse.getDefaultInstance()) return this;
if (!other.getErrorMessage().isEmpty()) {
errorMessage_ = other.errorMessage_;
onChanged();
}
if (other.getEmptyTrace() != false) {
setEmptyTrace(other.getEmptyTrace());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object errorMessage_ = "";
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
public java.lang.String getErrorMessage() {
java.lang.Object ref = errorMessage_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
errorMessage_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
public com.google.protobuf.ByteString
getErrorMessageBytes() {
java.lang.Object ref = errorMessage_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
errorMessage_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
public Builder setErrorMessage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
errorMessage_ = value;
onChanged();
return this;
}
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
public Builder clearErrorMessage() {
errorMessage_ = getDefaultInstance().getErrorMessage();
onChanged();
return this;
}
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
public Builder setErrorMessageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
errorMessage_ = value;
onChanged();
return this;
}
private boolean emptyTrace_ ;
/**
*
* Whether all hosts had returned a empty trace.
*
*
* bool empty_trace = 2;
*/
public boolean getEmptyTrace() {
return emptyTrace_;
}
/**
*
* Whether all hosts had returned a empty trace.
*
*
* bool empty_trace = 2;
*/
public Builder setEmptyTrace(boolean value) {
emptyTrace_ = value;
onChanged();
return this;
}
/**
*
* Whether all hosts had returned a empty trace.
*
*
* bool empty_trace = 2;
*/
public Builder clearEmptyTrace() {
emptyTrace_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.NewProfileSessionResponse)
}
// @@protoc_insertion_point(class_scope:tensorflow.NewProfileSessionResponse)
private static final tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse();
}
public static tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public NewProfileSessionResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new NewProfileSessionResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.NewProfileSessionResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface EnumProfileSessionsAndToolsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.EnumProfileSessionsAndToolsRequest)
com.google.protobuf.MessageOrBuilder {
/**
* string repository_root = 1;
*/
java.lang.String getRepositoryRoot();
/**
* string repository_root = 1;
*/
com.google.protobuf.ByteString
getRepositoryRootBytes();
}
/**
* Protobuf type {@code tensorflow.EnumProfileSessionsAndToolsRequest}
*/
public static final class EnumProfileSessionsAndToolsRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.EnumProfileSessionsAndToolsRequest)
EnumProfileSessionsAndToolsRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use EnumProfileSessionsAndToolsRequest.newBuilder() to construct.
private EnumProfileSessionsAndToolsRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private EnumProfileSessionsAndToolsRequest() {
repositoryRoot_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private EnumProfileSessionsAndToolsRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
repositoryRoot_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_EnumProfileSessionsAndToolsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_EnumProfileSessionsAndToolsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest.class, tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest.Builder.class);
}
public static final int REPOSITORY_ROOT_FIELD_NUMBER = 1;
private volatile java.lang.Object repositoryRoot_;
/**
* string repository_root = 1;
*/
public java.lang.String getRepositoryRoot() {
java.lang.Object ref = repositoryRoot_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
repositoryRoot_ = s;
return s;
}
}
/**
* string repository_root = 1;
*/
public com.google.protobuf.ByteString
getRepositoryRootBytes() {
java.lang.Object ref = repositoryRoot_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
repositoryRoot_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getRepositoryRootBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, repositoryRoot_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getRepositoryRootBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, repositoryRoot_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest)) {
return super.equals(obj);
}
tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest other = (tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest) obj;
boolean result = true;
result = result && getRepositoryRoot()
.equals(other.getRepositoryRoot());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + REPOSITORY_ROOT_FIELD_NUMBER;
hash = (53 * hash) + getRepositoryRoot().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.EnumProfileSessionsAndToolsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.EnumProfileSessionsAndToolsRequest)
tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_EnumProfileSessionsAndToolsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_EnumProfileSessionsAndToolsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest.class, tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest.Builder.class);
}
// Construct using tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
repositoryRoot_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_EnumProfileSessionsAndToolsRequest_descriptor;
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest getDefaultInstanceForType() {
return tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest.getDefaultInstance();
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest build() {
tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest buildPartial() {
tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest result = new tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest(this);
result.repositoryRoot_ = repositoryRoot_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest) {
return mergeFrom((tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest other) {
if (other == tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest.getDefaultInstance()) return this;
if (!other.getRepositoryRoot().isEmpty()) {
repositoryRoot_ = other.repositoryRoot_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object repositoryRoot_ = "";
/**
* string repository_root = 1;
*/
public java.lang.String getRepositoryRoot() {
java.lang.Object ref = repositoryRoot_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
repositoryRoot_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string repository_root = 1;
*/
public com.google.protobuf.ByteString
getRepositoryRootBytes() {
java.lang.Object ref = repositoryRoot_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
repositoryRoot_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string repository_root = 1;
*/
public Builder setRepositoryRoot(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
repositoryRoot_ = value;
onChanged();
return this;
}
/**
* string repository_root = 1;
*/
public Builder clearRepositoryRoot() {
repositoryRoot_ = getDefaultInstance().getRepositoryRoot();
onChanged();
return this;
}
/**
* string repository_root = 1;
*/
public Builder setRepositoryRootBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
repositoryRoot_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.EnumProfileSessionsAndToolsRequest)
}
// @@protoc_insertion_point(class_scope:tensorflow.EnumProfileSessionsAndToolsRequest)
private static final tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest();
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public EnumProfileSessionsAndToolsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new EnumProfileSessionsAndToolsRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ProfileSessionInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.ProfileSessionInfo)
com.google.protobuf.MessageOrBuilder {
/**
* string session_id = 1;
*/
java.lang.String getSessionId();
/**
* string session_id = 1;
*/
com.google.protobuf.ByteString
getSessionIdBytes();
/**
*
* Which tool data is available for consumption.
*
*
* repeated string available_tools = 2;
*/
java.util.List
getAvailableToolsList();
/**
*
* Which tool data is available for consumption.
*
*
* repeated string available_tools = 2;
*/
int getAvailableToolsCount();
/**
*
* Which tool data is available for consumption.
*
*
* repeated string available_tools = 2;
*/
java.lang.String getAvailableTools(int index);
/**
*
* Which tool data is available for consumption.
*
*
* repeated string available_tools = 2;
*/
com.google.protobuf.ByteString
getAvailableToolsBytes(int index);
}
/**
* Protobuf type {@code tensorflow.ProfileSessionInfo}
*/
public static final class ProfileSessionInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.ProfileSessionInfo)
ProfileSessionInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use ProfileSessionInfo.newBuilder() to construct.
private ProfileSessionInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ProfileSessionInfo() {
sessionId_ = "";
availableTools_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ProfileSessionInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
sessionId_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
availableTools_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000002;
}
availableTools_.add(s);
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
availableTools_ = availableTools_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_ProfileSessionInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_ProfileSessionInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfilerAnalysis.ProfileSessionInfo.class, tensorflow.TpuProfilerAnalysis.ProfileSessionInfo.Builder.class);
}
private int bitField0_;
public static final int SESSION_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object sessionId_;
/**
* string session_id = 1;
*/
public java.lang.String getSessionId() {
java.lang.Object ref = sessionId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sessionId_ = s;
return s;
}
}
/**
* string session_id = 1;
*/
public com.google.protobuf.ByteString
getSessionIdBytes() {
java.lang.Object ref = sessionId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sessionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int AVAILABLE_TOOLS_FIELD_NUMBER = 2;
private com.google.protobuf.LazyStringList availableTools_;
/**
*
* Which tool data is available for consumption.
*
*
* repeated string available_tools = 2;
*/
public com.google.protobuf.ProtocolStringList
getAvailableToolsList() {
return availableTools_;
}
/**
*
* Which tool data is available for consumption.
*
*
* repeated string available_tools = 2;
*/
public int getAvailableToolsCount() {
return availableTools_.size();
}
/**
*
* Which tool data is available for consumption.
*
*
* repeated string available_tools = 2;
*/
public java.lang.String getAvailableTools(int index) {
return availableTools_.get(index);
}
/**
*
* Which tool data is available for consumption.
*
*
* repeated string available_tools = 2;
*/
public com.google.protobuf.ByteString
getAvailableToolsBytes(int index) {
return availableTools_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getSessionIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, sessionId_);
}
for (int i = 0; i < availableTools_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, availableTools_.getRaw(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getSessionIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, sessionId_);
}
{
int dataSize = 0;
for (int i = 0; i < availableTools_.size(); i++) {
dataSize += computeStringSizeNoTag(availableTools_.getRaw(i));
}
size += dataSize;
size += 1 * getAvailableToolsList().size();
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.TpuProfilerAnalysis.ProfileSessionInfo)) {
return super.equals(obj);
}
tensorflow.TpuProfilerAnalysis.ProfileSessionInfo other = (tensorflow.TpuProfilerAnalysis.ProfileSessionInfo) obj;
boolean result = true;
result = result && getSessionId()
.equals(other.getSessionId());
result = result && getAvailableToolsList()
.equals(other.getAvailableToolsList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + SESSION_ID_FIELD_NUMBER;
hash = (53 * hash) + getSessionId().hashCode();
if (getAvailableToolsCount() > 0) {
hash = (37 * hash) + AVAILABLE_TOOLS_FIELD_NUMBER;
hash = (53 * hash) + getAvailableToolsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.TpuProfilerAnalysis.ProfileSessionInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.ProfileSessionInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.ProfileSessionInfo)
tensorflow.TpuProfilerAnalysis.ProfileSessionInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_ProfileSessionInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_ProfileSessionInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfilerAnalysis.ProfileSessionInfo.class, tensorflow.TpuProfilerAnalysis.ProfileSessionInfo.Builder.class);
}
// Construct using tensorflow.TpuProfilerAnalysis.ProfileSessionInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
sessionId_ = "";
availableTools_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_ProfileSessionInfo_descriptor;
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.ProfileSessionInfo getDefaultInstanceForType() {
return tensorflow.TpuProfilerAnalysis.ProfileSessionInfo.getDefaultInstance();
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.ProfileSessionInfo build() {
tensorflow.TpuProfilerAnalysis.ProfileSessionInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.ProfileSessionInfo buildPartial() {
tensorflow.TpuProfilerAnalysis.ProfileSessionInfo result = new tensorflow.TpuProfilerAnalysis.ProfileSessionInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.sessionId_ = sessionId_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
availableTools_ = availableTools_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.availableTools_ = availableTools_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.TpuProfilerAnalysis.ProfileSessionInfo) {
return mergeFrom((tensorflow.TpuProfilerAnalysis.ProfileSessionInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.TpuProfilerAnalysis.ProfileSessionInfo other) {
if (other == tensorflow.TpuProfilerAnalysis.ProfileSessionInfo.getDefaultInstance()) return this;
if (!other.getSessionId().isEmpty()) {
sessionId_ = other.sessionId_;
onChanged();
}
if (!other.availableTools_.isEmpty()) {
if (availableTools_.isEmpty()) {
availableTools_ = other.availableTools_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureAvailableToolsIsMutable();
availableTools_.addAll(other.availableTools_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.TpuProfilerAnalysis.ProfileSessionInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.TpuProfilerAnalysis.ProfileSessionInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object sessionId_ = "";
/**
* string session_id = 1;
*/
public java.lang.String getSessionId() {
java.lang.Object ref = sessionId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sessionId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string session_id = 1;
*/
public com.google.protobuf.ByteString
getSessionIdBytes() {
java.lang.Object ref = sessionId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sessionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string session_id = 1;
*/
public Builder setSessionId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sessionId_ = value;
onChanged();
return this;
}
/**
* string session_id = 1;
*/
public Builder clearSessionId() {
sessionId_ = getDefaultInstance().getSessionId();
onChanged();
return this;
}
/**
* string session_id = 1;
*/
public Builder setSessionIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sessionId_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList availableTools_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureAvailableToolsIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
availableTools_ = new com.google.protobuf.LazyStringArrayList(availableTools_);
bitField0_ |= 0x00000002;
}
}
/**
*
* Which tool data is available for consumption.
*
*
* repeated string available_tools = 2;
*/
public com.google.protobuf.ProtocolStringList
getAvailableToolsList() {
return availableTools_.getUnmodifiableView();
}
/**
*
* Which tool data is available for consumption.
*
*
* repeated string available_tools = 2;
*/
public int getAvailableToolsCount() {
return availableTools_.size();
}
/**
*
* Which tool data is available for consumption.
*
*
* repeated string available_tools = 2;
*/
public java.lang.String getAvailableTools(int index) {
return availableTools_.get(index);
}
/**
*
* Which tool data is available for consumption.
*
*
* repeated string available_tools = 2;
*/
public com.google.protobuf.ByteString
getAvailableToolsBytes(int index) {
return availableTools_.getByteString(index);
}
/**
*
* Which tool data is available for consumption.
*
*
* repeated string available_tools = 2;
*/
public Builder setAvailableTools(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureAvailableToolsIsMutable();
availableTools_.set(index, value);
onChanged();
return this;
}
/**
*
* Which tool data is available for consumption.
*
*
* repeated string available_tools = 2;
*/
public Builder addAvailableTools(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureAvailableToolsIsMutable();
availableTools_.add(value);
onChanged();
return this;
}
/**
*
* Which tool data is available for consumption.
*
*
* repeated string available_tools = 2;
*/
public Builder addAllAvailableTools(
java.lang.Iterable values) {
ensureAvailableToolsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, availableTools_);
onChanged();
return this;
}
/**
*
* Which tool data is available for consumption.
*
*
* repeated string available_tools = 2;
*/
public Builder clearAvailableTools() {
availableTools_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* Which tool data is available for consumption.
*
*
* repeated string available_tools = 2;
*/
public Builder addAvailableToolsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureAvailableToolsIsMutable();
availableTools_.add(value);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.ProfileSessionInfo)
}
// @@protoc_insertion_point(class_scope:tensorflow.ProfileSessionInfo)
private static final tensorflow.TpuProfilerAnalysis.ProfileSessionInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.TpuProfilerAnalysis.ProfileSessionInfo();
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ProfileSessionInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ProfileSessionInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.ProfileSessionInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface EnumProfileSessionsAndToolsResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.EnumProfileSessionsAndToolsResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
java.lang.String getErrorMessage();
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
com.google.protobuf.ByteString
getErrorMessageBytes();
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
java.util.List
getSessionsList();
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
tensorflow.TpuProfilerAnalysis.ProfileSessionInfo getSessions(int index);
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
int getSessionsCount();
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
java.util.List extends tensorflow.TpuProfilerAnalysis.ProfileSessionInfoOrBuilder>
getSessionsOrBuilderList();
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
tensorflow.TpuProfilerAnalysis.ProfileSessionInfoOrBuilder getSessionsOrBuilder(
int index);
}
/**
* Protobuf type {@code tensorflow.EnumProfileSessionsAndToolsResponse}
*/
public static final class EnumProfileSessionsAndToolsResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.EnumProfileSessionsAndToolsResponse)
EnumProfileSessionsAndToolsResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use EnumProfileSessionsAndToolsResponse.newBuilder() to construct.
private EnumProfileSessionsAndToolsResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private EnumProfileSessionsAndToolsResponse() {
errorMessage_ = "";
sessions_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private EnumProfileSessionsAndToolsResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
errorMessage_ = s;
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
sessions_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
sessions_.add(
input.readMessage(tensorflow.TpuProfilerAnalysis.ProfileSessionInfo.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
sessions_ = java.util.Collections.unmodifiableList(sessions_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_EnumProfileSessionsAndToolsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_EnumProfileSessionsAndToolsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse.class, tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse.Builder.class);
}
private int bitField0_;
public static final int ERROR_MESSAGE_FIELD_NUMBER = 1;
private volatile java.lang.Object errorMessage_;
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
public java.lang.String getErrorMessage() {
java.lang.Object ref = errorMessage_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
errorMessage_ = s;
return s;
}
}
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
public com.google.protobuf.ByteString
getErrorMessageBytes() {
java.lang.Object ref = errorMessage_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
errorMessage_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SESSIONS_FIELD_NUMBER = 2;
private java.util.List sessions_;
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
public java.util.List getSessionsList() {
return sessions_;
}
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
public java.util.List extends tensorflow.TpuProfilerAnalysis.ProfileSessionInfoOrBuilder>
getSessionsOrBuilderList() {
return sessions_;
}
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
public int getSessionsCount() {
return sessions_.size();
}
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
public tensorflow.TpuProfilerAnalysis.ProfileSessionInfo getSessions(int index) {
return sessions_.get(index);
}
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
public tensorflow.TpuProfilerAnalysis.ProfileSessionInfoOrBuilder getSessionsOrBuilder(
int index) {
return sessions_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getErrorMessageBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, errorMessage_);
}
for (int i = 0; i < sessions_.size(); i++) {
output.writeMessage(2, sessions_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getErrorMessageBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, errorMessage_);
}
for (int i = 0; i < sessions_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, sessions_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse)) {
return super.equals(obj);
}
tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse other = (tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse) obj;
boolean result = true;
result = result && getErrorMessage()
.equals(other.getErrorMessage());
result = result && getSessionsList()
.equals(other.getSessionsList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ERROR_MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getErrorMessage().hashCode();
if (getSessionsCount() > 0) {
hash = (37 * hash) + SESSIONS_FIELD_NUMBER;
hash = (53 * hash) + getSessionsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.EnumProfileSessionsAndToolsResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.EnumProfileSessionsAndToolsResponse)
tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_EnumProfileSessionsAndToolsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_EnumProfileSessionsAndToolsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse.class, tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse.Builder.class);
}
// Construct using tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getSessionsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
errorMessage_ = "";
if (sessionsBuilder_ == null) {
sessions_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
sessionsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_EnumProfileSessionsAndToolsResponse_descriptor;
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse getDefaultInstanceForType() {
return tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse.getDefaultInstance();
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse build() {
tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse buildPartial() {
tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse result = new tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.errorMessage_ = errorMessage_;
if (sessionsBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
sessions_ = java.util.Collections.unmodifiableList(sessions_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.sessions_ = sessions_;
} else {
result.sessions_ = sessionsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse) {
return mergeFrom((tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse other) {
if (other == tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse.getDefaultInstance()) return this;
if (!other.getErrorMessage().isEmpty()) {
errorMessage_ = other.errorMessage_;
onChanged();
}
if (sessionsBuilder_ == null) {
if (!other.sessions_.isEmpty()) {
if (sessions_.isEmpty()) {
sessions_ = other.sessions_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureSessionsIsMutable();
sessions_.addAll(other.sessions_);
}
onChanged();
}
} else {
if (!other.sessions_.isEmpty()) {
if (sessionsBuilder_.isEmpty()) {
sessionsBuilder_.dispose();
sessionsBuilder_ = null;
sessions_ = other.sessions_;
bitField0_ = (bitField0_ & ~0x00000002);
sessionsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getSessionsFieldBuilder() : null;
} else {
sessionsBuilder_.addAllMessages(other.sessions_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object errorMessage_ = "";
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
public java.lang.String getErrorMessage() {
java.lang.Object ref = errorMessage_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
errorMessage_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
public com.google.protobuf.ByteString
getErrorMessageBytes() {
java.lang.Object ref = errorMessage_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
errorMessage_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
public Builder setErrorMessage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
errorMessage_ = value;
onChanged();
return this;
}
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
public Builder clearErrorMessage() {
errorMessage_ = getDefaultInstance().getErrorMessage();
onChanged();
return this;
}
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
public Builder setErrorMessageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
errorMessage_ = value;
onChanged();
return this;
}
private java.util.List sessions_ =
java.util.Collections.emptyList();
private void ensureSessionsIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
sessions_ = new java.util.ArrayList(sessions_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.TpuProfilerAnalysis.ProfileSessionInfo, tensorflow.TpuProfilerAnalysis.ProfileSessionInfo.Builder, tensorflow.TpuProfilerAnalysis.ProfileSessionInfoOrBuilder> sessionsBuilder_;
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
public java.util.List getSessionsList() {
if (sessionsBuilder_ == null) {
return java.util.Collections.unmodifiableList(sessions_);
} else {
return sessionsBuilder_.getMessageList();
}
}
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
public int getSessionsCount() {
if (sessionsBuilder_ == null) {
return sessions_.size();
} else {
return sessionsBuilder_.getCount();
}
}
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
public tensorflow.TpuProfilerAnalysis.ProfileSessionInfo getSessions(int index) {
if (sessionsBuilder_ == null) {
return sessions_.get(index);
} else {
return sessionsBuilder_.getMessage(index);
}
}
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
public Builder setSessions(
int index, tensorflow.TpuProfilerAnalysis.ProfileSessionInfo value) {
if (sessionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSessionsIsMutable();
sessions_.set(index, value);
onChanged();
} else {
sessionsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
public Builder setSessions(
int index, tensorflow.TpuProfilerAnalysis.ProfileSessionInfo.Builder builderForValue) {
if (sessionsBuilder_ == null) {
ensureSessionsIsMutable();
sessions_.set(index, builderForValue.build());
onChanged();
} else {
sessionsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
public Builder addSessions(tensorflow.TpuProfilerAnalysis.ProfileSessionInfo value) {
if (sessionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSessionsIsMutable();
sessions_.add(value);
onChanged();
} else {
sessionsBuilder_.addMessage(value);
}
return this;
}
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
public Builder addSessions(
int index, tensorflow.TpuProfilerAnalysis.ProfileSessionInfo value) {
if (sessionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSessionsIsMutable();
sessions_.add(index, value);
onChanged();
} else {
sessionsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
public Builder addSessions(
tensorflow.TpuProfilerAnalysis.ProfileSessionInfo.Builder builderForValue) {
if (sessionsBuilder_ == null) {
ensureSessionsIsMutable();
sessions_.add(builderForValue.build());
onChanged();
} else {
sessionsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
public Builder addSessions(
int index, tensorflow.TpuProfilerAnalysis.ProfileSessionInfo.Builder builderForValue) {
if (sessionsBuilder_ == null) {
ensureSessionsIsMutable();
sessions_.add(index, builderForValue.build());
onChanged();
} else {
sessionsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
public Builder addAllSessions(
java.lang.Iterable extends tensorflow.TpuProfilerAnalysis.ProfileSessionInfo> values) {
if (sessionsBuilder_ == null) {
ensureSessionsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, sessions_);
onChanged();
} else {
sessionsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
public Builder clearSessions() {
if (sessionsBuilder_ == null) {
sessions_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
sessionsBuilder_.clear();
}
return this;
}
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
public Builder removeSessions(int index) {
if (sessionsBuilder_ == null) {
ensureSessionsIsMutable();
sessions_.remove(index);
onChanged();
} else {
sessionsBuilder_.remove(index);
}
return this;
}
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
public tensorflow.TpuProfilerAnalysis.ProfileSessionInfo.Builder getSessionsBuilder(
int index) {
return getSessionsFieldBuilder().getBuilder(index);
}
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
public tensorflow.TpuProfilerAnalysis.ProfileSessionInfoOrBuilder getSessionsOrBuilder(
int index) {
if (sessionsBuilder_ == null) {
return sessions_.get(index); } else {
return sessionsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
public java.util.List extends tensorflow.TpuProfilerAnalysis.ProfileSessionInfoOrBuilder>
getSessionsOrBuilderList() {
if (sessionsBuilder_ != null) {
return sessionsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(sessions_);
}
}
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
public tensorflow.TpuProfilerAnalysis.ProfileSessionInfo.Builder addSessionsBuilder() {
return getSessionsFieldBuilder().addBuilder(
tensorflow.TpuProfilerAnalysis.ProfileSessionInfo.getDefaultInstance());
}
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
public tensorflow.TpuProfilerAnalysis.ProfileSessionInfo.Builder addSessionsBuilder(
int index) {
return getSessionsFieldBuilder().addBuilder(
index, tensorflow.TpuProfilerAnalysis.ProfileSessionInfo.getDefaultInstance());
}
/**
*
* If success, the returned sessions information are stored here.
*
*
* repeated .tensorflow.ProfileSessionInfo sessions = 2;
*/
public java.util.List
getSessionsBuilderList() {
return getSessionsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.TpuProfilerAnalysis.ProfileSessionInfo, tensorflow.TpuProfilerAnalysis.ProfileSessionInfo.Builder, tensorflow.TpuProfilerAnalysis.ProfileSessionInfoOrBuilder>
getSessionsFieldBuilder() {
if (sessionsBuilder_ == null) {
sessionsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.TpuProfilerAnalysis.ProfileSessionInfo, tensorflow.TpuProfilerAnalysis.ProfileSessionInfo.Builder, tensorflow.TpuProfilerAnalysis.ProfileSessionInfoOrBuilder>(
sessions_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
sessions_ = null;
}
return sessionsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.EnumProfileSessionsAndToolsResponse)
}
// @@protoc_insertion_point(class_scope:tensorflow.EnumProfileSessionsAndToolsResponse)
private static final tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse();
}
public static tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public EnumProfileSessionsAndToolsResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new EnumProfileSessionsAndToolsResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.EnumProfileSessionsAndToolsResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ProfileSessionDataRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.ProfileSessionDataRequest)
com.google.protobuf.MessageOrBuilder {
/**
* string repository_root = 1;
*/
java.lang.String getRepositoryRoot();
/**
* string repository_root = 1;
*/
com.google.protobuf.ByteString
getRepositoryRootBytes();
/**
* string session_id = 2;
*/
java.lang.String getSessionId();
/**
* string session_id = 2;
*/
com.google.protobuf.ByteString
getSessionIdBytes();
/**
*
* Which host the data is associated. if empty, data from all hosts are
* aggregated.
*
*
* string host_name = 5;
*/
java.lang.String getHostName();
/**
*
* Which host the data is associated. if empty, data from all hosts are
* aggregated.
*
*
* string host_name = 5;
*/
com.google.protobuf.ByteString
getHostNameBytes();
/**
*
* Which tool
*
*
* string tool_name = 3;
*/
java.lang.String getToolName();
/**
*
* Which tool
*
*
* string tool_name = 3;
*/
com.google.protobuf.ByteString
getToolNameBytes();
/**
*
* Tool's specific parameters. e.g. TraceViewer's viewport etc
*
*
* map<string, string> parameters = 4;
*/
int getParametersCount();
/**
*
* Tool's specific parameters. e.g. TraceViewer's viewport etc
*
*
* map<string, string> parameters = 4;
*/
boolean containsParameters(
java.lang.String key);
/**
* Use {@link #getParametersMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getParameters();
/**
*
* Tool's specific parameters. e.g. TraceViewer's viewport etc
*
*
* map<string, string> parameters = 4;
*/
java.util.Map
getParametersMap();
/**
*
* Tool's specific parameters. e.g. TraceViewer's viewport etc
*
*
* map<string, string> parameters = 4;
*/
java.lang.String getParametersOrDefault(
java.lang.String key,
java.lang.String defaultValue);
/**
*
* Tool's specific parameters. e.g. TraceViewer's viewport etc
*
*
* map<string, string> parameters = 4;
*/
java.lang.String getParametersOrThrow(
java.lang.String key);
}
/**
* Protobuf type {@code tensorflow.ProfileSessionDataRequest}
*/
public static final class ProfileSessionDataRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.ProfileSessionDataRequest)
ProfileSessionDataRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use ProfileSessionDataRequest.newBuilder() to construct.
private ProfileSessionDataRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ProfileSessionDataRequest() {
repositoryRoot_ = "";
sessionId_ = "";
hostName_ = "";
toolName_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ProfileSessionDataRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
repositoryRoot_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
sessionId_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
toolName_ = s;
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
parameters_ = com.google.protobuf.MapField.newMapField(
ParametersDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000010;
}
com.google.protobuf.MapEntry
parameters__ = input.readMessage(
ParametersDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
parameters_.getMutableMap().put(
parameters__.getKey(), parameters__.getValue());
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
hostName_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_ProfileSessionDataRequest_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 4:
return internalGetParameters();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_ProfileSessionDataRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest.class, tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest.Builder.class);
}
private int bitField0_;
public static final int REPOSITORY_ROOT_FIELD_NUMBER = 1;
private volatile java.lang.Object repositoryRoot_;
/**
* string repository_root = 1;
*/
public java.lang.String getRepositoryRoot() {
java.lang.Object ref = repositoryRoot_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
repositoryRoot_ = s;
return s;
}
}
/**
* string repository_root = 1;
*/
public com.google.protobuf.ByteString
getRepositoryRootBytes() {
java.lang.Object ref = repositoryRoot_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
repositoryRoot_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SESSION_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object sessionId_;
/**
* string session_id = 2;
*/
public java.lang.String getSessionId() {
java.lang.Object ref = sessionId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sessionId_ = s;
return s;
}
}
/**
* string session_id = 2;
*/
public com.google.protobuf.ByteString
getSessionIdBytes() {
java.lang.Object ref = sessionId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sessionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int HOST_NAME_FIELD_NUMBER = 5;
private volatile java.lang.Object hostName_;
/**
*
* Which host the data is associated. if empty, data from all hosts are
* aggregated.
*
*
* string host_name = 5;
*/
public java.lang.String getHostName() {
java.lang.Object ref = hostName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
hostName_ = s;
return s;
}
}
/**
*
* Which host the data is associated. if empty, data from all hosts are
* aggregated.
*
*
* string host_name = 5;
*/
public com.google.protobuf.ByteString
getHostNameBytes() {
java.lang.Object ref = hostName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
hostName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TOOL_NAME_FIELD_NUMBER = 3;
private volatile java.lang.Object toolName_;
/**
*
* Which tool
*
*
* string tool_name = 3;
*/
public java.lang.String getToolName() {
java.lang.Object ref = toolName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
toolName_ = s;
return s;
}
}
/**
*
* Which tool
*
*
* string tool_name = 3;
*/
public com.google.protobuf.ByteString
getToolNameBytes() {
java.lang.Object ref = toolName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
toolName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PARAMETERS_FIELD_NUMBER = 4;
private static final class ParametersDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_ProfileSessionDataRequest_ParametersEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> parameters_;
private com.google.protobuf.MapField
internalGetParameters() {
if (parameters_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ParametersDefaultEntryHolder.defaultEntry);
}
return parameters_;
}
public int getParametersCount() {
return internalGetParameters().getMap().size();
}
/**
*
* Tool's specific parameters. e.g. TraceViewer's viewport etc
*
*
* map<string, string> parameters = 4;
*/
public boolean containsParameters(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetParameters().getMap().containsKey(key);
}
/**
* Use {@link #getParametersMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getParameters() {
return getParametersMap();
}
/**
*
* Tool's specific parameters. e.g. TraceViewer's viewport etc
*
*
* map<string, string> parameters = 4;
*/
public java.util.Map getParametersMap() {
return internalGetParameters().getMap();
}
/**
*
* Tool's specific parameters. e.g. TraceViewer's viewport etc
*
*
* map<string, string> parameters = 4;
*/
public java.lang.String getParametersOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetParameters().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Tool's specific parameters. e.g. TraceViewer's viewport etc
*
*
* map<string, string> parameters = 4;
*/
public java.lang.String getParametersOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetParameters().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getRepositoryRootBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, repositoryRoot_);
}
if (!getSessionIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, sessionId_);
}
if (!getToolNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, toolName_);
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetParameters(),
ParametersDefaultEntryHolder.defaultEntry,
4);
if (!getHostNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, hostName_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getRepositoryRootBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, repositoryRoot_);
}
if (!getSessionIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, sessionId_);
}
if (!getToolNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, toolName_);
}
for (java.util.Map.Entry entry
: internalGetParameters().getMap().entrySet()) {
com.google.protobuf.MapEntry
parameters__ = ParametersDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, parameters__);
}
if (!getHostNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, hostName_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest)) {
return super.equals(obj);
}
tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest other = (tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest) obj;
boolean result = true;
result = result && getRepositoryRoot()
.equals(other.getRepositoryRoot());
result = result && getSessionId()
.equals(other.getSessionId());
result = result && getHostName()
.equals(other.getHostName());
result = result && getToolName()
.equals(other.getToolName());
result = result && internalGetParameters().equals(
other.internalGetParameters());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + REPOSITORY_ROOT_FIELD_NUMBER;
hash = (53 * hash) + getRepositoryRoot().hashCode();
hash = (37 * hash) + SESSION_ID_FIELD_NUMBER;
hash = (53 * hash) + getSessionId().hashCode();
hash = (37 * hash) + HOST_NAME_FIELD_NUMBER;
hash = (53 * hash) + getHostName().hashCode();
hash = (37 * hash) + TOOL_NAME_FIELD_NUMBER;
hash = (53 * hash) + getToolName().hashCode();
if (!internalGetParameters().getMap().isEmpty()) {
hash = (37 * hash) + PARAMETERS_FIELD_NUMBER;
hash = (53 * hash) + internalGetParameters().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.ProfileSessionDataRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.ProfileSessionDataRequest)
tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_ProfileSessionDataRequest_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 4:
return internalGetParameters();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 4:
return internalGetMutableParameters();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_ProfileSessionDataRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest.class, tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest.Builder.class);
}
// Construct using tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
repositoryRoot_ = "";
sessionId_ = "";
hostName_ = "";
toolName_ = "";
internalGetMutableParameters().clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_ProfileSessionDataRequest_descriptor;
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest getDefaultInstanceForType() {
return tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest.getDefaultInstance();
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest build() {
tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest buildPartial() {
tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest result = new tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.repositoryRoot_ = repositoryRoot_;
result.sessionId_ = sessionId_;
result.hostName_ = hostName_;
result.toolName_ = toolName_;
result.parameters_ = internalGetParameters();
result.parameters_.makeImmutable();
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest) {
return mergeFrom((tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest other) {
if (other == tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest.getDefaultInstance()) return this;
if (!other.getRepositoryRoot().isEmpty()) {
repositoryRoot_ = other.repositoryRoot_;
onChanged();
}
if (!other.getSessionId().isEmpty()) {
sessionId_ = other.sessionId_;
onChanged();
}
if (!other.getHostName().isEmpty()) {
hostName_ = other.hostName_;
onChanged();
}
if (!other.getToolName().isEmpty()) {
toolName_ = other.toolName_;
onChanged();
}
internalGetMutableParameters().mergeFrom(
other.internalGetParameters());
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object repositoryRoot_ = "";
/**
* string repository_root = 1;
*/
public java.lang.String getRepositoryRoot() {
java.lang.Object ref = repositoryRoot_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
repositoryRoot_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string repository_root = 1;
*/
public com.google.protobuf.ByteString
getRepositoryRootBytes() {
java.lang.Object ref = repositoryRoot_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
repositoryRoot_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string repository_root = 1;
*/
public Builder setRepositoryRoot(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
repositoryRoot_ = value;
onChanged();
return this;
}
/**
* string repository_root = 1;
*/
public Builder clearRepositoryRoot() {
repositoryRoot_ = getDefaultInstance().getRepositoryRoot();
onChanged();
return this;
}
/**
* string repository_root = 1;
*/
public Builder setRepositoryRootBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
repositoryRoot_ = value;
onChanged();
return this;
}
private java.lang.Object sessionId_ = "";
/**
* string session_id = 2;
*/
public java.lang.String getSessionId() {
java.lang.Object ref = sessionId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sessionId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string session_id = 2;
*/
public com.google.protobuf.ByteString
getSessionIdBytes() {
java.lang.Object ref = sessionId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sessionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string session_id = 2;
*/
public Builder setSessionId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sessionId_ = value;
onChanged();
return this;
}
/**
* string session_id = 2;
*/
public Builder clearSessionId() {
sessionId_ = getDefaultInstance().getSessionId();
onChanged();
return this;
}
/**
* string session_id = 2;
*/
public Builder setSessionIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sessionId_ = value;
onChanged();
return this;
}
private java.lang.Object hostName_ = "";
/**
*
* Which host the data is associated. if empty, data from all hosts are
* aggregated.
*
*
* string host_name = 5;
*/
public java.lang.String getHostName() {
java.lang.Object ref = hostName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
hostName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Which host the data is associated. if empty, data from all hosts are
* aggregated.
*
*
* string host_name = 5;
*/
public com.google.protobuf.ByteString
getHostNameBytes() {
java.lang.Object ref = hostName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
hostName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Which host the data is associated. if empty, data from all hosts are
* aggregated.
*
*
* string host_name = 5;
*/
public Builder setHostName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
hostName_ = value;
onChanged();
return this;
}
/**
*
* Which host the data is associated. if empty, data from all hosts are
* aggregated.
*
*
* string host_name = 5;
*/
public Builder clearHostName() {
hostName_ = getDefaultInstance().getHostName();
onChanged();
return this;
}
/**
*
* Which host the data is associated. if empty, data from all hosts are
* aggregated.
*
*
* string host_name = 5;
*/
public Builder setHostNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
hostName_ = value;
onChanged();
return this;
}
private java.lang.Object toolName_ = "";
/**
*
* Which tool
*
*
* string tool_name = 3;
*/
public java.lang.String getToolName() {
java.lang.Object ref = toolName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
toolName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Which tool
*
*
* string tool_name = 3;
*/
public com.google.protobuf.ByteString
getToolNameBytes() {
java.lang.Object ref = toolName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
toolName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Which tool
*
*
* string tool_name = 3;
*/
public Builder setToolName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
toolName_ = value;
onChanged();
return this;
}
/**
*
* Which tool
*
*
* string tool_name = 3;
*/
public Builder clearToolName() {
toolName_ = getDefaultInstance().getToolName();
onChanged();
return this;
}
/**
*
* Which tool
*
*
* string tool_name = 3;
*/
public Builder setToolNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
toolName_ = value;
onChanged();
return this;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> parameters_;
private com.google.protobuf.MapField
internalGetParameters() {
if (parameters_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ParametersDefaultEntryHolder.defaultEntry);
}
return parameters_;
}
private com.google.protobuf.MapField
internalGetMutableParameters() {
onChanged();;
if (parameters_ == null) {
parameters_ = com.google.protobuf.MapField.newMapField(
ParametersDefaultEntryHolder.defaultEntry);
}
if (!parameters_.isMutable()) {
parameters_ = parameters_.copy();
}
return parameters_;
}
public int getParametersCount() {
return internalGetParameters().getMap().size();
}
/**
*
* Tool's specific parameters. e.g. TraceViewer's viewport etc
*
*
* map<string, string> parameters = 4;
*/
public boolean containsParameters(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetParameters().getMap().containsKey(key);
}
/**
* Use {@link #getParametersMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getParameters() {
return getParametersMap();
}
/**
*
* Tool's specific parameters. e.g. TraceViewer's viewport etc
*
*
* map<string, string> parameters = 4;
*/
public java.util.Map getParametersMap() {
return internalGetParameters().getMap();
}
/**
*
* Tool's specific parameters. e.g. TraceViewer's viewport etc
*
*
* map<string, string> parameters = 4;
*/
public java.lang.String getParametersOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetParameters().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Tool's specific parameters. e.g. TraceViewer's viewport etc
*
*
* map<string, string> parameters = 4;
*/
public java.lang.String getParametersOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetParameters().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearParameters() {
internalGetMutableParameters().getMutableMap()
.clear();
return this;
}
/**
*
* Tool's specific parameters. e.g. TraceViewer's viewport etc
*
*
* map<string, string> parameters = 4;
*/
public Builder removeParameters(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableParameters().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableParameters() {
return internalGetMutableParameters().getMutableMap();
}
/**
*
* Tool's specific parameters. e.g. TraceViewer's viewport etc
*
*
* map<string, string> parameters = 4;
*/
public Builder putParameters(
java.lang.String key,
java.lang.String value) {
if (key == null) { throw new java.lang.NullPointerException(); }
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutableParameters().getMutableMap()
.put(key, value);
return this;
}
/**
*
* Tool's specific parameters. e.g. TraceViewer's viewport etc
*
*
* map<string, string> parameters = 4;
*/
public Builder putAllParameters(
java.util.Map values) {
internalGetMutableParameters().getMutableMap()
.putAll(values);
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.ProfileSessionDataRequest)
}
// @@protoc_insertion_point(class_scope:tensorflow.ProfileSessionDataRequest)
private static final tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest();
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ProfileSessionDataRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ProfileSessionDataRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.ProfileSessionDataRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ProfileSessionDataResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.ProfileSessionDataResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
java.lang.String getErrorMessage();
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
com.google.protobuf.ByteString
getErrorMessageBytes();
/**
*
* Output format. e.g. "json" or "proto" or "blob"
*
*
* string output_format = 2;
*/
java.lang.String getOutputFormat();
/**
*
* Output format. e.g. "json" or "proto" or "blob"
*
*
* string output_format = 2;
*/
com.google.protobuf.ByteString
getOutputFormatBytes();
/**
*
* TODO(jiesun): figure out whether to put bytes or oneof tool specific proto.
*
*
* bytes output = 3;
*/
com.google.protobuf.ByteString getOutput();
}
/**
* Protobuf type {@code tensorflow.ProfileSessionDataResponse}
*/
public static final class ProfileSessionDataResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.ProfileSessionDataResponse)
ProfileSessionDataResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use ProfileSessionDataResponse.newBuilder() to construct.
private ProfileSessionDataResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ProfileSessionDataResponse() {
errorMessage_ = "";
outputFormat_ = "";
output_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ProfileSessionDataResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
errorMessage_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
outputFormat_ = s;
break;
}
case 26: {
output_ = input.readBytes();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_ProfileSessionDataResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_ProfileSessionDataResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse.class, tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse.Builder.class);
}
public static final int ERROR_MESSAGE_FIELD_NUMBER = 1;
private volatile java.lang.Object errorMessage_;
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
public java.lang.String getErrorMessage() {
java.lang.Object ref = errorMessage_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
errorMessage_ = s;
return s;
}
}
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
public com.google.protobuf.ByteString
getErrorMessageBytes() {
java.lang.Object ref = errorMessage_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
errorMessage_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OUTPUT_FORMAT_FIELD_NUMBER = 2;
private volatile java.lang.Object outputFormat_;
/**
*
* Output format. e.g. "json" or "proto" or "blob"
*
*
* string output_format = 2;
*/
public java.lang.String getOutputFormat() {
java.lang.Object ref = outputFormat_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
outputFormat_ = s;
return s;
}
}
/**
*
* Output format. e.g. "json" or "proto" or "blob"
*
*
* string output_format = 2;
*/
public com.google.protobuf.ByteString
getOutputFormatBytes() {
java.lang.Object ref = outputFormat_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
outputFormat_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OUTPUT_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString output_;
/**
*
* TODO(jiesun): figure out whether to put bytes or oneof tool specific proto.
*
*
* bytes output = 3;
*/
public com.google.protobuf.ByteString getOutput() {
return output_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getErrorMessageBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, errorMessage_);
}
if (!getOutputFormatBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, outputFormat_);
}
if (!output_.isEmpty()) {
output.writeBytes(3, output_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getErrorMessageBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, errorMessage_);
}
if (!getOutputFormatBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, outputFormat_);
}
if (!output_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, output_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse)) {
return super.equals(obj);
}
tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse other = (tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse) obj;
boolean result = true;
result = result && getErrorMessage()
.equals(other.getErrorMessage());
result = result && getOutputFormat()
.equals(other.getOutputFormat());
result = result && getOutput()
.equals(other.getOutput());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ERROR_MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getErrorMessage().hashCode();
hash = (37 * hash) + OUTPUT_FORMAT_FIELD_NUMBER;
hash = (53 * hash) + getOutputFormat().hashCode();
hash = (37 * hash) + OUTPUT_FIELD_NUMBER;
hash = (53 * hash) + getOutput().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.ProfileSessionDataResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.ProfileSessionDataResponse)
tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_ProfileSessionDataResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_ProfileSessionDataResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse.class, tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse.Builder.class);
}
// Construct using tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
errorMessage_ = "";
outputFormat_ = "";
output_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.TpuProfilerAnalysis.internal_static_tensorflow_ProfileSessionDataResponse_descriptor;
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse getDefaultInstanceForType() {
return tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse.getDefaultInstance();
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse build() {
tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse buildPartial() {
tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse result = new tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse(this);
result.errorMessage_ = errorMessage_;
result.outputFormat_ = outputFormat_;
result.output_ = output_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse) {
return mergeFrom((tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse other) {
if (other == tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse.getDefaultInstance()) return this;
if (!other.getErrorMessage().isEmpty()) {
errorMessage_ = other.errorMessage_;
onChanged();
}
if (!other.getOutputFormat().isEmpty()) {
outputFormat_ = other.outputFormat_;
onChanged();
}
if (other.getOutput() != com.google.protobuf.ByteString.EMPTY) {
setOutput(other.getOutput());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object errorMessage_ = "";
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
public java.lang.String getErrorMessage() {
java.lang.Object ref = errorMessage_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
errorMessage_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
public com.google.protobuf.ByteString
getErrorMessageBytes() {
java.lang.Object ref = errorMessage_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
errorMessage_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
public Builder setErrorMessage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
errorMessage_ = value;
onChanged();
return this;
}
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
public Builder clearErrorMessage() {
errorMessage_ = getDefaultInstance().getErrorMessage();
onChanged();
return this;
}
/**
*
* Auxiliary error_message.
*
*
* string error_message = 1;
*/
public Builder setErrorMessageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
errorMessage_ = value;
onChanged();
return this;
}
private java.lang.Object outputFormat_ = "";
/**
*
* Output format. e.g. "json" or "proto" or "blob"
*
*
* string output_format = 2;
*/
public java.lang.String getOutputFormat() {
java.lang.Object ref = outputFormat_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
outputFormat_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output format. e.g. "json" or "proto" or "blob"
*
*
* string output_format = 2;
*/
public com.google.protobuf.ByteString
getOutputFormatBytes() {
java.lang.Object ref = outputFormat_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
outputFormat_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output format. e.g. "json" or "proto" or "blob"
*
*
* string output_format = 2;
*/
public Builder setOutputFormat(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
outputFormat_ = value;
onChanged();
return this;
}
/**
*
* Output format. e.g. "json" or "proto" or "blob"
*
*
* string output_format = 2;
*/
public Builder clearOutputFormat() {
outputFormat_ = getDefaultInstance().getOutputFormat();
onChanged();
return this;
}
/**
*
* Output format. e.g. "json" or "proto" or "blob"
*
*
* string output_format = 2;
*/
public Builder setOutputFormatBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
outputFormat_ = value;
onChanged();
return this;
}
private com.google.protobuf.ByteString output_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* TODO(jiesun): figure out whether to put bytes or oneof tool specific proto.
*
*
* bytes output = 3;
*/
public com.google.protobuf.ByteString getOutput() {
return output_;
}
/**
*
* TODO(jiesun): figure out whether to put bytes or oneof tool specific proto.
*
*
* bytes output = 3;
*/
public Builder setOutput(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
output_ = value;
onChanged();
return this;
}
/**
*
* TODO(jiesun): figure out whether to put bytes or oneof tool specific proto.
*
*
* bytes output = 3;
*/
public Builder clearOutput() {
output_ = getDefaultInstance().getOutput();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.ProfileSessionDataResponse)
}
// @@protoc_insertion_point(class_scope:tensorflow.ProfileSessionDataResponse)
private static final tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse();
}
public static tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ProfileSessionDataResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ProfileSessionDataResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.TpuProfilerAnalysis.ProfileSessionDataResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_NewProfileSessionRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_NewProfileSessionRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_NewProfileSessionResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_NewProfileSessionResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_EnumProfileSessionsAndToolsRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_EnumProfileSessionsAndToolsRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_ProfileSessionInfo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_ProfileSessionInfo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_EnumProfileSessionsAndToolsResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_EnumProfileSessionsAndToolsResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_ProfileSessionDataRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_ProfileSessionDataRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_ProfileSessionDataRequest_ParametersEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_ProfileSessionDataRequest_ParametersEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_ProfileSessionDataResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_ProfileSessionDataResponse_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n;tensorflow/contrib/tpu/profiler/tpu_pr" +
"ofiler_analysis.proto\022\ntensorflow\0322tenso" +
"rflow/contrib/tpu/profiler/tpu_profiler." +
"proto\"\203\001\n\030NewProfileSessionRequest\022+\n\007re" +
"quest\030\001 \001(\0132\032.tensorflow.ProfileRequest\022" +
"\027\n\017repository_root\030\002 \001(\t\022\r\n\005hosts\030\003 \003(\t\022" +
"\022\n\nsession_id\030\004 \001(\t\"G\n\031NewProfileSession" +
"Response\022\025\n\rerror_message\030\001 \001(\t\022\023\n\013empty" +
"_trace\030\002 \001(\010\"=\n\"EnumProfileSessionsAndTo" +
"olsRequest\022\027\n\017repository_root\030\001 \001(\t\"A\n\022P" +
"rofileSessionInfo\022\022\n\nsession_id\030\001 \001(\t\022\027\n" +
"\017available_tools\030\002 \003(\t\"n\n#EnumProfileSes" +
"sionsAndToolsResponse\022\025\n\rerror_message\030\001" +
" \001(\t\0220\n\010sessions\030\002 \003(\0132\036.tensorflow.Prof" +
"ileSessionInfo\"\354\001\n\031ProfileSessionDataReq" +
"uest\022\027\n\017repository_root\030\001 \001(\t\022\022\n\nsession" +
"_id\030\002 \001(\t\022\021\n\thost_name\030\005 \001(\t\022\021\n\ttool_nam" +
"e\030\003 \001(\t\022I\n\nparameters\030\004 \003(\01325.tensorflow" +
".ProfileSessionDataRequest.ParametersEnt" +
"ry\0321\n\017ParametersEntry\022\013\n\003key\030\001 \001(\t\022\r\n\005va" +
"lue\030\002 \001(\t:\0028\001\"Z\n\032ProfileSessionDataRespo" +
"nse\022\025\n\rerror_message\030\001 \001(\t\022\025\n\routput_for" +
"mat\030\002 \001(\t\022\016\n\006output\030\003 \001(\0142\313\002\n\022TPUProfile" +
"Analysis\022[\n\nNewSession\022$.tensorflow.NewP" +
"rofileSessionRequest\032%.tensorflow.NewPro" +
"fileSessionResponse\"\000\022q\n\014EnumSessions\022.." +
"tensorflow.EnumProfileSessionsAndToolsRe" +
"quest\032/.tensorflow.EnumProfileSessionsAn" +
"dToolsResponse\"\000\022e\n\022GetSessionToolData\022%" +
".tensorflow.ProfileSessionDataRequest\032&." +
"tensorflow.ProfileSessionDataResponse\"\000b" +
"\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
tensorflow.TpuProfiler.getDescriptor(),
}, assigner);
internal_static_tensorflow_NewProfileSessionRequest_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_tensorflow_NewProfileSessionRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_NewProfileSessionRequest_descriptor,
new java.lang.String[] { "Request", "RepositoryRoot", "Hosts", "SessionId", });
internal_static_tensorflow_NewProfileSessionResponse_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_tensorflow_NewProfileSessionResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_NewProfileSessionResponse_descriptor,
new java.lang.String[] { "ErrorMessage", "EmptyTrace", });
internal_static_tensorflow_EnumProfileSessionsAndToolsRequest_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_tensorflow_EnumProfileSessionsAndToolsRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_EnumProfileSessionsAndToolsRequest_descriptor,
new java.lang.String[] { "RepositoryRoot", });
internal_static_tensorflow_ProfileSessionInfo_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_tensorflow_ProfileSessionInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_ProfileSessionInfo_descriptor,
new java.lang.String[] { "SessionId", "AvailableTools", });
internal_static_tensorflow_EnumProfileSessionsAndToolsResponse_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_tensorflow_EnumProfileSessionsAndToolsResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_EnumProfileSessionsAndToolsResponse_descriptor,
new java.lang.String[] { "ErrorMessage", "Sessions", });
internal_static_tensorflow_ProfileSessionDataRequest_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_tensorflow_ProfileSessionDataRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_ProfileSessionDataRequest_descriptor,
new java.lang.String[] { "RepositoryRoot", "SessionId", "HostName", "ToolName", "Parameters", });
internal_static_tensorflow_ProfileSessionDataRequest_ParametersEntry_descriptor =
internal_static_tensorflow_ProfileSessionDataRequest_descriptor.getNestedTypes().get(0);
internal_static_tensorflow_ProfileSessionDataRequest_ParametersEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_ProfileSessionDataRequest_ParametersEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_tensorflow_ProfileSessionDataResponse_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_tensorflow_ProfileSessionDataResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_ProfileSessionDataResponse_descriptor,
new java.lang.String[] { "ErrorMessage", "OutputFormat", "Output", });
tensorflow.TpuProfiler.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy