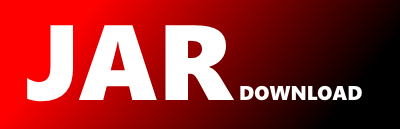
tensorflow.decision_trees.GenericTreeModel Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: tensorflow/contrib/decision_trees/proto/generic_tree_model.proto
package tensorflow.decision_trees;
public final class GenericTreeModel {
private GenericTreeModel() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface ModelOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.decision_trees.Model)
com.google.protobuf.MessageOrBuilder {
/**
* .tensorflow.decision_trees.DecisionTree decision_tree = 1;
*/
boolean hasDecisionTree();
/**
* .tensorflow.decision_trees.DecisionTree decision_tree = 1;
*/
tensorflow.decision_trees.GenericTreeModel.DecisionTree getDecisionTree();
/**
* .tensorflow.decision_trees.DecisionTree decision_tree = 1;
*/
tensorflow.decision_trees.GenericTreeModel.DecisionTreeOrBuilder getDecisionTreeOrBuilder();
/**
* .tensorflow.decision_trees.Ensemble ensemble = 2;
*/
boolean hasEnsemble();
/**
* .tensorflow.decision_trees.Ensemble ensemble = 2;
*/
tensorflow.decision_trees.GenericTreeModel.Ensemble getEnsemble();
/**
* .tensorflow.decision_trees.Ensemble ensemble = 2;
*/
tensorflow.decision_trees.GenericTreeModel.EnsembleOrBuilder getEnsembleOrBuilder();
/**
* .google.protobuf.Any custom_model = 3;
*/
boolean hasCustomModel();
/**
* .google.protobuf.Any custom_model = 3;
*/
com.google.protobuf.Any getCustomModel();
/**
* .google.protobuf.Any custom_model = 3;
*/
com.google.protobuf.AnyOrBuilder getCustomModelOrBuilder();
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
java.util.List
getAdditionalDataList();
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
com.google.protobuf.Any getAdditionalData(int index);
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
int getAdditionalDataCount();
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList();
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index);
public tensorflow.decision_trees.GenericTreeModel.Model.ModelCase getModelCase();
}
/**
*
* A generic handle for any type of model.
*
*
* Protobuf type {@code tensorflow.decision_trees.Model}
*/
public static final class Model extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.decision_trees.Model)
ModelOrBuilder {
private static final long serialVersionUID = 0L;
// Use Model.newBuilder() to construct.
private Model(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Model() {
additionalData_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Model(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
tensorflow.decision_trees.GenericTreeModel.DecisionTree.Builder subBuilder = null;
if (modelCase_ == 1) {
subBuilder = ((tensorflow.decision_trees.GenericTreeModel.DecisionTree) model_).toBuilder();
}
model_ =
input.readMessage(tensorflow.decision_trees.GenericTreeModel.DecisionTree.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((tensorflow.decision_trees.GenericTreeModel.DecisionTree) model_);
model_ = subBuilder.buildPartial();
}
modelCase_ = 1;
break;
}
case 18: {
tensorflow.decision_trees.GenericTreeModel.Ensemble.Builder subBuilder = null;
if (modelCase_ == 2) {
subBuilder = ((tensorflow.decision_trees.GenericTreeModel.Ensemble) model_).toBuilder();
}
model_ =
input.readMessage(tensorflow.decision_trees.GenericTreeModel.Ensemble.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((tensorflow.decision_trees.GenericTreeModel.Ensemble) model_);
model_ = subBuilder.buildPartial();
}
modelCase_ = 2;
break;
}
case 26: {
com.google.protobuf.Any.Builder subBuilder = null;
if (modelCase_ == 3) {
subBuilder = ((com.google.protobuf.Any) model_).toBuilder();
}
model_ =
input.readMessage(com.google.protobuf.Any.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((com.google.protobuf.Any) model_);
model_ = subBuilder.buildPartial();
}
modelCase_ = 3;
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
additionalData_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
additionalData_.add(
input.readMessage(com.google.protobuf.Any.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
additionalData_ = java.util.Collections.unmodifiableList(additionalData_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Model_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Model_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.Model.class, tensorflow.decision_trees.GenericTreeModel.Model.Builder.class);
}
private int bitField0_;
private int modelCase_ = 0;
private java.lang.Object model_;
public enum ModelCase
implements com.google.protobuf.Internal.EnumLite {
DECISION_TREE(1),
ENSEMBLE(2),
CUSTOM_MODEL(3),
MODEL_NOT_SET(0);
private final int value;
private ModelCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ModelCase valueOf(int value) {
return forNumber(value);
}
public static ModelCase forNumber(int value) {
switch (value) {
case 1: return DECISION_TREE;
case 2: return ENSEMBLE;
case 3: return CUSTOM_MODEL;
case 0: return MODEL_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ModelCase
getModelCase() {
return ModelCase.forNumber(
modelCase_);
}
public static final int DECISION_TREE_FIELD_NUMBER = 1;
/**
* .tensorflow.decision_trees.DecisionTree decision_tree = 1;
*/
public boolean hasDecisionTree() {
return modelCase_ == 1;
}
/**
* .tensorflow.decision_trees.DecisionTree decision_tree = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.DecisionTree getDecisionTree() {
if (modelCase_ == 1) {
return (tensorflow.decision_trees.GenericTreeModel.DecisionTree) model_;
}
return tensorflow.decision_trees.GenericTreeModel.DecisionTree.getDefaultInstance();
}
/**
* .tensorflow.decision_trees.DecisionTree decision_tree = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.DecisionTreeOrBuilder getDecisionTreeOrBuilder() {
if (modelCase_ == 1) {
return (tensorflow.decision_trees.GenericTreeModel.DecisionTree) model_;
}
return tensorflow.decision_trees.GenericTreeModel.DecisionTree.getDefaultInstance();
}
public static final int ENSEMBLE_FIELD_NUMBER = 2;
/**
* .tensorflow.decision_trees.Ensemble ensemble = 2;
*/
public boolean hasEnsemble() {
return modelCase_ == 2;
}
/**
* .tensorflow.decision_trees.Ensemble ensemble = 2;
*/
public tensorflow.decision_trees.GenericTreeModel.Ensemble getEnsemble() {
if (modelCase_ == 2) {
return (tensorflow.decision_trees.GenericTreeModel.Ensemble) model_;
}
return tensorflow.decision_trees.GenericTreeModel.Ensemble.getDefaultInstance();
}
/**
* .tensorflow.decision_trees.Ensemble ensemble = 2;
*/
public tensorflow.decision_trees.GenericTreeModel.EnsembleOrBuilder getEnsembleOrBuilder() {
if (modelCase_ == 2) {
return (tensorflow.decision_trees.GenericTreeModel.Ensemble) model_;
}
return tensorflow.decision_trees.GenericTreeModel.Ensemble.getDefaultInstance();
}
public static final int CUSTOM_MODEL_FIELD_NUMBER = 3;
/**
* .google.protobuf.Any custom_model = 3;
*/
public boolean hasCustomModel() {
return modelCase_ == 3;
}
/**
* .google.protobuf.Any custom_model = 3;
*/
public com.google.protobuf.Any getCustomModel() {
if (modelCase_ == 3) {
return (com.google.protobuf.Any) model_;
}
return com.google.protobuf.Any.getDefaultInstance();
}
/**
* .google.protobuf.Any custom_model = 3;
*/
public com.google.protobuf.AnyOrBuilder getCustomModelOrBuilder() {
if (modelCase_ == 3) {
return (com.google.protobuf.Any) model_;
}
return com.google.protobuf.Any.getDefaultInstance();
}
public static final int ADDITIONAL_DATA_FIELD_NUMBER = 4;
private java.util.List additionalData_;
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public java.util.List getAdditionalDataList() {
return additionalData_;
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList() {
return additionalData_;
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public int getAdditionalDataCount() {
return additionalData_.size();
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public com.google.protobuf.Any getAdditionalData(int index) {
return additionalData_.get(index);
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index) {
return additionalData_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (modelCase_ == 1) {
output.writeMessage(1, (tensorflow.decision_trees.GenericTreeModel.DecisionTree) model_);
}
if (modelCase_ == 2) {
output.writeMessage(2, (tensorflow.decision_trees.GenericTreeModel.Ensemble) model_);
}
if (modelCase_ == 3) {
output.writeMessage(3, (com.google.protobuf.Any) model_);
}
for (int i = 0; i < additionalData_.size(); i++) {
output.writeMessage(4, additionalData_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (modelCase_ == 1) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, (tensorflow.decision_trees.GenericTreeModel.DecisionTree) model_);
}
if (modelCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, (tensorflow.decision_trees.GenericTreeModel.Ensemble) model_);
}
if (modelCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, (com.google.protobuf.Any) model_);
}
for (int i = 0; i < additionalData_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, additionalData_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.decision_trees.GenericTreeModel.Model)) {
return super.equals(obj);
}
tensorflow.decision_trees.GenericTreeModel.Model other = (tensorflow.decision_trees.GenericTreeModel.Model) obj;
boolean result = true;
result = result && getAdditionalDataList()
.equals(other.getAdditionalDataList());
result = result && getModelCase().equals(
other.getModelCase());
if (!result) return false;
switch (modelCase_) {
case 1:
result = result && getDecisionTree()
.equals(other.getDecisionTree());
break;
case 2:
result = result && getEnsemble()
.equals(other.getEnsemble());
break;
case 3:
result = result && getCustomModel()
.equals(other.getCustomModel());
break;
case 0:
default:
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getAdditionalDataCount() > 0) {
hash = (37 * hash) + ADDITIONAL_DATA_FIELD_NUMBER;
hash = (53 * hash) + getAdditionalDataList().hashCode();
}
switch (modelCase_) {
case 1:
hash = (37 * hash) + DECISION_TREE_FIELD_NUMBER;
hash = (53 * hash) + getDecisionTree().hashCode();
break;
case 2:
hash = (37 * hash) + ENSEMBLE_FIELD_NUMBER;
hash = (53 * hash) + getEnsemble().hashCode();
break;
case 3:
hash = (37 * hash) + CUSTOM_MODEL_FIELD_NUMBER;
hash = (53 * hash) + getCustomModel().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.decision_trees.GenericTreeModel.Model parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.Model parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Model parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.Model parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Model parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.Model parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Model parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.Model parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Model parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.Model parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Model parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.Model parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.decision_trees.GenericTreeModel.Model prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A generic handle for any type of model.
*
*
* Protobuf type {@code tensorflow.decision_trees.Model}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.decision_trees.Model)
tensorflow.decision_trees.GenericTreeModel.ModelOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Model_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Model_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.Model.class, tensorflow.decision_trees.GenericTreeModel.Model.Builder.class);
}
// Construct using tensorflow.decision_trees.GenericTreeModel.Model.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getAdditionalDataFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (additionalDataBuilder_ == null) {
additionalData_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
} else {
additionalDataBuilder_.clear();
}
modelCase_ = 0;
model_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Model_descriptor;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Model getDefaultInstanceForType() {
return tensorflow.decision_trees.GenericTreeModel.Model.getDefaultInstance();
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Model build() {
tensorflow.decision_trees.GenericTreeModel.Model result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Model buildPartial() {
tensorflow.decision_trees.GenericTreeModel.Model result = new tensorflow.decision_trees.GenericTreeModel.Model(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (modelCase_ == 1) {
if (decisionTreeBuilder_ == null) {
result.model_ = model_;
} else {
result.model_ = decisionTreeBuilder_.build();
}
}
if (modelCase_ == 2) {
if (ensembleBuilder_ == null) {
result.model_ = model_;
} else {
result.model_ = ensembleBuilder_.build();
}
}
if (modelCase_ == 3) {
if (customModelBuilder_ == null) {
result.model_ = model_;
} else {
result.model_ = customModelBuilder_.build();
}
}
if (additionalDataBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008)) {
additionalData_ = java.util.Collections.unmodifiableList(additionalData_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.additionalData_ = additionalData_;
} else {
result.additionalData_ = additionalDataBuilder_.build();
}
result.bitField0_ = to_bitField0_;
result.modelCase_ = modelCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.decision_trees.GenericTreeModel.Model) {
return mergeFrom((tensorflow.decision_trees.GenericTreeModel.Model)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.decision_trees.GenericTreeModel.Model other) {
if (other == tensorflow.decision_trees.GenericTreeModel.Model.getDefaultInstance()) return this;
if (additionalDataBuilder_ == null) {
if (!other.additionalData_.isEmpty()) {
if (additionalData_.isEmpty()) {
additionalData_ = other.additionalData_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureAdditionalDataIsMutable();
additionalData_.addAll(other.additionalData_);
}
onChanged();
}
} else {
if (!other.additionalData_.isEmpty()) {
if (additionalDataBuilder_.isEmpty()) {
additionalDataBuilder_.dispose();
additionalDataBuilder_ = null;
additionalData_ = other.additionalData_;
bitField0_ = (bitField0_ & ~0x00000008);
additionalDataBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAdditionalDataFieldBuilder() : null;
} else {
additionalDataBuilder_.addAllMessages(other.additionalData_);
}
}
}
switch (other.getModelCase()) {
case DECISION_TREE: {
mergeDecisionTree(other.getDecisionTree());
break;
}
case ENSEMBLE: {
mergeEnsemble(other.getEnsemble());
break;
}
case CUSTOM_MODEL: {
mergeCustomModel(other.getCustomModel());
break;
}
case MODEL_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.decision_trees.GenericTreeModel.Model parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.decision_trees.GenericTreeModel.Model) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int modelCase_ = 0;
private java.lang.Object model_;
public ModelCase
getModelCase() {
return ModelCase.forNumber(
modelCase_);
}
public Builder clearModel() {
modelCase_ = 0;
model_ = null;
onChanged();
return this;
}
private int bitField0_;
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.DecisionTree, tensorflow.decision_trees.GenericTreeModel.DecisionTree.Builder, tensorflow.decision_trees.GenericTreeModel.DecisionTreeOrBuilder> decisionTreeBuilder_;
/**
* .tensorflow.decision_trees.DecisionTree decision_tree = 1;
*/
public boolean hasDecisionTree() {
return modelCase_ == 1;
}
/**
* .tensorflow.decision_trees.DecisionTree decision_tree = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.DecisionTree getDecisionTree() {
if (decisionTreeBuilder_ == null) {
if (modelCase_ == 1) {
return (tensorflow.decision_trees.GenericTreeModel.DecisionTree) model_;
}
return tensorflow.decision_trees.GenericTreeModel.DecisionTree.getDefaultInstance();
} else {
if (modelCase_ == 1) {
return decisionTreeBuilder_.getMessage();
}
return tensorflow.decision_trees.GenericTreeModel.DecisionTree.getDefaultInstance();
}
}
/**
* .tensorflow.decision_trees.DecisionTree decision_tree = 1;
*/
public Builder setDecisionTree(tensorflow.decision_trees.GenericTreeModel.DecisionTree value) {
if (decisionTreeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
model_ = value;
onChanged();
} else {
decisionTreeBuilder_.setMessage(value);
}
modelCase_ = 1;
return this;
}
/**
* .tensorflow.decision_trees.DecisionTree decision_tree = 1;
*/
public Builder setDecisionTree(
tensorflow.decision_trees.GenericTreeModel.DecisionTree.Builder builderForValue) {
if (decisionTreeBuilder_ == null) {
model_ = builderForValue.build();
onChanged();
} else {
decisionTreeBuilder_.setMessage(builderForValue.build());
}
modelCase_ = 1;
return this;
}
/**
* .tensorflow.decision_trees.DecisionTree decision_tree = 1;
*/
public Builder mergeDecisionTree(tensorflow.decision_trees.GenericTreeModel.DecisionTree value) {
if (decisionTreeBuilder_ == null) {
if (modelCase_ == 1 &&
model_ != tensorflow.decision_trees.GenericTreeModel.DecisionTree.getDefaultInstance()) {
model_ = tensorflow.decision_trees.GenericTreeModel.DecisionTree.newBuilder((tensorflow.decision_trees.GenericTreeModel.DecisionTree) model_)
.mergeFrom(value).buildPartial();
} else {
model_ = value;
}
onChanged();
} else {
if (modelCase_ == 1) {
decisionTreeBuilder_.mergeFrom(value);
}
decisionTreeBuilder_.setMessage(value);
}
modelCase_ = 1;
return this;
}
/**
* .tensorflow.decision_trees.DecisionTree decision_tree = 1;
*/
public Builder clearDecisionTree() {
if (decisionTreeBuilder_ == null) {
if (modelCase_ == 1) {
modelCase_ = 0;
model_ = null;
onChanged();
}
} else {
if (modelCase_ == 1) {
modelCase_ = 0;
model_ = null;
}
decisionTreeBuilder_.clear();
}
return this;
}
/**
* .tensorflow.decision_trees.DecisionTree decision_tree = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.DecisionTree.Builder getDecisionTreeBuilder() {
return getDecisionTreeFieldBuilder().getBuilder();
}
/**
* .tensorflow.decision_trees.DecisionTree decision_tree = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.DecisionTreeOrBuilder getDecisionTreeOrBuilder() {
if ((modelCase_ == 1) && (decisionTreeBuilder_ != null)) {
return decisionTreeBuilder_.getMessageOrBuilder();
} else {
if (modelCase_ == 1) {
return (tensorflow.decision_trees.GenericTreeModel.DecisionTree) model_;
}
return tensorflow.decision_trees.GenericTreeModel.DecisionTree.getDefaultInstance();
}
}
/**
* .tensorflow.decision_trees.DecisionTree decision_tree = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.DecisionTree, tensorflow.decision_trees.GenericTreeModel.DecisionTree.Builder, tensorflow.decision_trees.GenericTreeModel.DecisionTreeOrBuilder>
getDecisionTreeFieldBuilder() {
if (decisionTreeBuilder_ == null) {
if (!(modelCase_ == 1)) {
model_ = tensorflow.decision_trees.GenericTreeModel.DecisionTree.getDefaultInstance();
}
decisionTreeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.DecisionTree, tensorflow.decision_trees.GenericTreeModel.DecisionTree.Builder, tensorflow.decision_trees.GenericTreeModel.DecisionTreeOrBuilder>(
(tensorflow.decision_trees.GenericTreeModel.DecisionTree) model_,
getParentForChildren(),
isClean());
model_ = null;
}
modelCase_ = 1;
onChanged();;
return decisionTreeBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Ensemble, tensorflow.decision_trees.GenericTreeModel.Ensemble.Builder, tensorflow.decision_trees.GenericTreeModel.EnsembleOrBuilder> ensembleBuilder_;
/**
* .tensorflow.decision_trees.Ensemble ensemble = 2;
*/
public boolean hasEnsemble() {
return modelCase_ == 2;
}
/**
* .tensorflow.decision_trees.Ensemble ensemble = 2;
*/
public tensorflow.decision_trees.GenericTreeModel.Ensemble getEnsemble() {
if (ensembleBuilder_ == null) {
if (modelCase_ == 2) {
return (tensorflow.decision_trees.GenericTreeModel.Ensemble) model_;
}
return tensorflow.decision_trees.GenericTreeModel.Ensemble.getDefaultInstance();
} else {
if (modelCase_ == 2) {
return ensembleBuilder_.getMessage();
}
return tensorflow.decision_trees.GenericTreeModel.Ensemble.getDefaultInstance();
}
}
/**
* .tensorflow.decision_trees.Ensemble ensemble = 2;
*/
public Builder setEnsemble(tensorflow.decision_trees.GenericTreeModel.Ensemble value) {
if (ensembleBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
model_ = value;
onChanged();
} else {
ensembleBuilder_.setMessage(value);
}
modelCase_ = 2;
return this;
}
/**
* .tensorflow.decision_trees.Ensemble ensemble = 2;
*/
public Builder setEnsemble(
tensorflow.decision_trees.GenericTreeModel.Ensemble.Builder builderForValue) {
if (ensembleBuilder_ == null) {
model_ = builderForValue.build();
onChanged();
} else {
ensembleBuilder_.setMessage(builderForValue.build());
}
modelCase_ = 2;
return this;
}
/**
* .tensorflow.decision_trees.Ensemble ensemble = 2;
*/
public Builder mergeEnsemble(tensorflow.decision_trees.GenericTreeModel.Ensemble value) {
if (ensembleBuilder_ == null) {
if (modelCase_ == 2 &&
model_ != tensorflow.decision_trees.GenericTreeModel.Ensemble.getDefaultInstance()) {
model_ = tensorflow.decision_trees.GenericTreeModel.Ensemble.newBuilder((tensorflow.decision_trees.GenericTreeModel.Ensemble) model_)
.mergeFrom(value).buildPartial();
} else {
model_ = value;
}
onChanged();
} else {
if (modelCase_ == 2) {
ensembleBuilder_.mergeFrom(value);
}
ensembleBuilder_.setMessage(value);
}
modelCase_ = 2;
return this;
}
/**
* .tensorflow.decision_trees.Ensemble ensemble = 2;
*/
public Builder clearEnsemble() {
if (ensembleBuilder_ == null) {
if (modelCase_ == 2) {
modelCase_ = 0;
model_ = null;
onChanged();
}
} else {
if (modelCase_ == 2) {
modelCase_ = 0;
model_ = null;
}
ensembleBuilder_.clear();
}
return this;
}
/**
* .tensorflow.decision_trees.Ensemble ensemble = 2;
*/
public tensorflow.decision_trees.GenericTreeModel.Ensemble.Builder getEnsembleBuilder() {
return getEnsembleFieldBuilder().getBuilder();
}
/**
* .tensorflow.decision_trees.Ensemble ensemble = 2;
*/
public tensorflow.decision_trees.GenericTreeModel.EnsembleOrBuilder getEnsembleOrBuilder() {
if ((modelCase_ == 2) && (ensembleBuilder_ != null)) {
return ensembleBuilder_.getMessageOrBuilder();
} else {
if (modelCase_ == 2) {
return (tensorflow.decision_trees.GenericTreeModel.Ensemble) model_;
}
return tensorflow.decision_trees.GenericTreeModel.Ensemble.getDefaultInstance();
}
}
/**
* .tensorflow.decision_trees.Ensemble ensemble = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Ensemble, tensorflow.decision_trees.GenericTreeModel.Ensemble.Builder, tensorflow.decision_trees.GenericTreeModel.EnsembleOrBuilder>
getEnsembleFieldBuilder() {
if (ensembleBuilder_ == null) {
if (!(modelCase_ == 2)) {
model_ = tensorflow.decision_trees.GenericTreeModel.Ensemble.getDefaultInstance();
}
ensembleBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Ensemble, tensorflow.decision_trees.GenericTreeModel.Ensemble.Builder, tensorflow.decision_trees.GenericTreeModel.EnsembleOrBuilder>(
(tensorflow.decision_trees.GenericTreeModel.Ensemble) model_,
getParentForChildren(),
isClean());
model_ = null;
}
modelCase_ = 2;
onChanged();;
return ensembleBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> customModelBuilder_;
/**
* .google.protobuf.Any custom_model = 3;
*/
public boolean hasCustomModel() {
return modelCase_ == 3;
}
/**
* .google.protobuf.Any custom_model = 3;
*/
public com.google.protobuf.Any getCustomModel() {
if (customModelBuilder_ == null) {
if (modelCase_ == 3) {
return (com.google.protobuf.Any) model_;
}
return com.google.protobuf.Any.getDefaultInstance();
} else {
if (modelCase_ == 3) {
return customModelBuilder_.getMessage();
}
return com.google.protobuf.Any.getDefaultInstance();
}
}
/**
* .google.protobuf.Any custom_model = 3;
*/
public Builder setCustomModel(com.google.protobuf.Any value) {
if (customModelBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
model_ = value;
onChanged();
} else {
customModelBuilder_.setMessage(value);
}
modelCase_ = 3;
return this;
}
/**
* .google.protobuf.Any custom_model = 3;
*/
public Builder setCustomModel(
com.google.protobuf.Any.Builder builderForValue) {
if (customModelBuilder_ == null) {
model_ = builderForValue.build();
onChanged();
} else {
customModelBuilder_.setMessage(builderForValue.build());
}
modelCase_ = 3;
return this;
}
/**
* .google.protobuf.Any custom_model = 3;
*/
public Builder mergeCustomModel(com.google.protobuf.Any value) {
if (customModelBuilder_ == null) {
if (modelCase_ == 3 &&
model_ != com.google.protobuf.Any.getDefaultInstance()) {
model_ = com.google.protobuf.Any.newBuilder((com.google.protobuf.Any) model_)
.mergeFrom(value).buildPartial();
} else {
model_ = value;
}
onChanged();
} else {
if (modelCase_ == 3) {
customModelBuilder_.mergeFrom(value);
}
customModelBuilder_.setMessage(value);
}
modelCase_ = 3;
return this;
}
/**
* .google.protobuf.Any custom_model = 3;
*/
public Builder clearCustomModel() {
if (customModelBuilder_ == null) {
if (modelCase_ == 3) {
modelCase_ = 0;
model_ = null;
onChanged();
}
} else {
if (modelCase_ == 3) {
modelCase_ = 0;
model_ = null;
}
customModelBuilder_.clear();
}
return this;
}
/**
* .google.protobuf.Any custom_model = 3;
*/
public com.google.protobuf.Any.Builder getCustomModelBuilder() {
return getCustomModelFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Any custom_model = 3;
*/
public com.google.protobuf.AnyOrBuilder getCustomModelOrBuilder() {
if ((modelCase_ == 3) && (customModelBuilder_ != null)) {
return customModelBuilder_.getMessageOrBuilder();
} else {
if (modelCase_ == 3) {
return (com.google.protobuf.Any) model_;
}
return com.google.protobuf.Any.getDefaultInstance();
}
}
/**
* .google.protobuf.Any custom_model = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getCustomModelFieldBuilder() {
if (customModelBuilder_ == null) {
if (!(modelCase_ == 3)) {
model_ = com.google.protobuf.Any.getDefaultInstance();
}
customModelBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
(com.google.protobuf.Any) model_,
getParentForChildren(),
isClean());
model_ = null;
}
modelCase_ = 3;
onChanged();;
return customModelBuilder_;
}
private java.util.List additionalData_ =
java.util.Collections.emptyList();
private void ensureAdditionalDataIsMutable() {
if (!((bitField0_ & 0x00000008) == 0x00000008)) {
additionalData_ = new java.util.ArrayList(additionalData_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> additionalDataBuilder_;
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public java.util.List getAdditionalDataList() {
if (additionalDataBuilder_ == null) {
return java.util.Collections.unmodifiableList(additionalData_);
} else {
return additionalDataBuilder_.getMessageList();
}
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public int getAdditionalDataCount() {
if (additionalDataBuilder_ == null) {
return additionalData_.size();
} else {
return additionalDataBuilder_.getCount();
}
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public com.google.protobuf.Any getAdditionalData(int index) {
if (additionalDataBuilder_ == null) {
return additionalData_.get(index);
} else {
return additionalDataBuilder_.getMessage(index);
}
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public Builder setAdditionalData(
int index, com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.set(index, value);
onChanged();
} else {
additionalDataBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public Builder setAdditionalData(
int index, com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.set(index, builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public Builder addAdditionalData(com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.add(value);
onChanged();
} else {
additionalDataBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public Builder addAdditionalData(
int index, com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.add(index, value);
onChanged();
} else {
additionalDataBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public Builder addAdditionalData(
com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.add(builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public Builder addAdditionalData(
int index, com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.add(index, builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public Builder addAllAdditionalData(
java.lang.Iterable extends com.google.protobuf.Any> values) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, additionalData_);
onChanged();
} else {
additionalDataBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public Builder clearAdditionalData() {
if (additionalDataBuilder_ == null) {
additionalData_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
additionalDataBuilder_.clear();
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public Builder removeAdditionalData(int index) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.remove(index);
onChanged();
} else {
additionalDataBuilder_.remove(index);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public com.google.protobuf.Any.Builder getAdditionalDataBuilder(
int index) {
return getAdditionalDataFieldBuilder().getBuilder(index);
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index) {
if (additionalDataBuilder_ == null) {
return additionalData_.get(index); } else {
return additionalDataBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList() {
if (additionalDataBuilder_ != null) {
return additionalDataBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(additionalData_);
}
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public com.google.protobuf.Any.Builder addAdditionalDataBuilder() {
return getAdditionalDataFieldBuilder().addBuilder(
com.google.protobuf.Any.getDefaultInstance());
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public com.google.protobuf.Any.Builder addAdditionalDataBuilder(
int index) {
return getAdditionalDataFieldBuilder().addBuilder(
index, com.google.protobuf.Any.getDefaultInstance());
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public java.util.List
getAdditionalDataBuilderList() {
return getAdditionalDataFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getAdditionalDataFieldBuilder() {
if (additionalDataBuilder_ == null) {
additionalDataBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
additionalData_,
((bitField0_ & 0x00000008) == 0x00000008),
getParentForChildren(),
isClean());
additionalData_ = null;
}
return additionalDataBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.decision_trees.Model)
}
// @@protoc_insertion_point(class_scope:tensorflow.decision_trees.Model)
private static final tensorflow.decision_trees.GenericTreeModel.Model DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.decision_trees.GenericTreeModel.Model();
}
public static tensorflow.decision_trees.GenericTreeModel.Model getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Model parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Model(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Model getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ModelAndFeaturesOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.decision_trees.ModelAndFeatures)
com.google.protobuf.MessageOrBuilder {
/**
*
* Given a FeatureId feature_id, the feature's description is in
* features[feature_id.id.value].
*
*
* map<string, .tensorflow.decision_trees.ModelAndFeatures.Feature> features = 1;
*/
int getFeaturesCount();
/**
*
* Given a FeatureId feature_id, the feature's description is in
* features[feature_id.id.value].
*
*
* map<string, .tensorflow.decision_trees.ModelAndFeatures.Feature> features = 1;
*/
boolean containsFeatures(
java.lang.String key);
/**
* Use {@link #getFeaturesMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getFeatures();
/**
*
* Given a FeatureId feature_id, the feature's description is in
* features[feature_id.id.value].
*
*
* map<string, .tensorflow.decision_trees.ModelAndFeatures.Feature> features = 1;
*/
java.util.Map
getFeaturesMap();
/**
*
* Given a FeatureId feature_id, the feature's description is in
* features[feature_id.id.value].
*
*
* map<string, .tensorflow.decision_trees.ModelAndFeatures.Feature> features = 1;
*/
tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature getFeaturesOrDefault(
java.lang.String key,
tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature defaultValue);
/**
*
* Given a FeatureId feature_id, the feature's description is in
* features[feature_id.id.value].
*
*
* map<string, .tensorflow.decision_trees.ModelAndFeatures.Feature> features = 1;
*/
tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature getFeaturesOrThrow(
java.lang.String key);
/**
* .tensorflow.decision_trees.Model model = 2;
*/
boolean hasModel();
/**
* .tensorflow.decision_trees.Model model = 2;
*/
tensorflow.decision_trees.GenericTreeModel.Model getModel();
/**
* .tensorflow.decision_trees.Model model = 2;
*/
tensorflow.decision_trees.GenericTreeModel.ModelOrBuilder getModelOrBuilder();
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
java.util.List
getAdditionalDataList();
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
com.google.protobuf.Any getAdditionalData(int index);
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
int getAdditionalDataCount();
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList();
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index);
}
/**
* Protobuf type {@code tensorflow.decision_trees.ModelAndFeatures}
*/
public static final class ModelAndFeatures extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.decision_trees.ModelAndFeatures)
ModelAndFeaturesOrBuilder {
private static final long serialVersionUID = 0L;
// Use ModelAndFeatures.newBuilder() to construct.
private ModelAndFeatures(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ModelAndFeatures() {
additionalData_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ModelAndFeatures(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
features_ = com.google.protobuf.MapField.newMapField(
FeaturesDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000001;
}
com.google.protobuf.MapEntry
features__ = input.readMessage(
FeaturesDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
features_.getMutableMap().put(
features__.getKey(), features__.getValue());
break;
}
case 18: {
tensorflow.decision_trees.GenericTreeModel.Model.Builder subBuilder = null;
if (model_ != null) {
subBuilder = model_.toBuilder();
}
model_ = input.readMessage(tensorflow.decision_trees.GenericTreeModel.Model.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(model_);
model_ = subBuilder.buildPartial();
}
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
additionalData_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
additionalData_.add(
input.readMessage(com.google.protobuf.Any.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
additionalData_ = java.util.Collections.unmodifiableList(additionalData_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_ModelAndFeatures_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 1:
return internalGetFeatures();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_ModelAndFeatures_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.class, tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Builder.class);
}
public interface FeatureOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.decision_trees.ModelAndFeatures.Feature)
com.google.protobuf.MessageOrBuilder {
/**
*
* TODO(jonasz): Remove this field, as it's confusing. Ctx: cr/153569450.
*
*
* .tensorflow.decision_trees.FeatureId feature_id = 1 [deprecated = true];
*/
@java.lang.Deprecated boolean hasFeatureId();
/**
*
* TODO(jonasz): Remove this field, as it's confusing. Ctx: cr/153569450.
*
*
* .tensorflow.decision_trees.FeatureId feature_id = 1 [deprecated = true];
*/
@java.lang.Deprecated tensorflow.decision_trees.GenericTreeModel.FeatureId getFeatureId();
/**
*
* TODO(jonasz): Remove this field, as it's confusing. Ctx: cr/153569450.
*
*
* .tensorflow.decision_trees.FeatureId feature_id = 1 [deprecated = true];
*/
@java.lang.Deprecated tensorflow.decision_trees.GenericTreeModel.FeatureIdOrBuilder getFeatureIdOrBuilder();
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
java.util.List
getAdditionalDataList();
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
com.google.protobuf.Any getAdditionalData(int index);
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
int getAdditionalDataCount();
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList();
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index);
}
/**
* Protobuf type {@code tensorflow.decision_trees.ModelAndFeatures.Feature}
*/
public static final class Feature extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.decision_trees.ModelAndFeatures.Feature)
FeatureOrBuilder {
private static final long serialVersionUID = 0L;
// Use Feature.newBuilder() to construct.
private Feature(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Feature() {
additionalData_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Feature(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
tensorflow.decision_trees.GenericTreeModel.FeatureId.Builder subBuilder = null;
if (featureId_ != null) {
subBuilder = featureId_.toBuilder();
}
featureId_ = input.readMessage(tensorflow.decision_trees.GenericTreeModel.FeatureId.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(featureId_);
featureId_ = subBuilder.buildPartial();
}
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
additionalData_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
additionalData_.add(
input.readMessage(com.google.protobuf.Any.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
additionalData_ = java.util.Collections.unmodifiableList(additionalData_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_ModelAndFeatures_Feature_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_ModelAndFeatures_Feature_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature.class, tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature.Builder.class);
}
private int bitField0_;
public static final int FEATURE_ID_FIELD_NUMBER = 1;
private tensorflow.decision_trees.GenericTreeModel.FeatureId featureId_;
/**
*
* TODO(jonasz): Remove this field, as it's confusing. Ctx: cr/153569450.
*
*
* .tensorflow.decision_trees.FeatureId feature_id = 1 [deprecated = true];
*/
@java.lang.Deprecated public boolean hasFeatureId() {
return featureId_ != null;
}
/**
*
* TODO(jonasz): Remove this field, as it's confusing. Ctx: cr/153569450.
*
*
* .tensorflow.decision_trees.FeatureId feature_id = 1 [deprecated = true];
*/
@java.lang.Deprecated public tensorflow.decision_trees.GenericTreeModel.FeatureId getFeatureId() {
return featureId_ == null ? tensorflow.decision_trees.GenericTreeModel.FeatureId.getDefaultInstance() : featureId_;
}
/**
*
* TODO(jonasz): Remove this field, as it's confusing. Ctx: cr/153569450.
*
*
* .tensorflow.decision_trees.FeatureId feature_id = 1 [deprecated = true];
*/
@java.lang.Deprecated public tensorflow.decision_trees.GenericTreeModel.FeatureIdOrBuilder getFeatureIdOrBuilder() {
return getFeatureId();
}
public static final int ADDITIONAL_DATA_FIELD_NUMBER = 2;
private java.util.List additionalData_;
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public java.util.List getAdditionalDataList() {
return additionalData_;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList() {
return additionalData_;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public int getAdditionalDataCount() {
return additionalData_.size();
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public com.google.protobuf.Any getAdditionalData(int index) {
return additionalData_.get(index);
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index) {
return additionalData_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (featureId_ != null) {
output.writeMessage(1, getFeatureId());
}
for (int i = 0; i < additionalData_.size(); i++) {
output.writeMessage(2, additionalData_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (featureId_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getFeatureId());
}
for (int i = 0; i < additionalData_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, additionalData_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature)) {
return super.equals(obj);
}
tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature other = (tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature) obj;
boolean result = true;
result = result && (hasFeatureId() == other.hasFeatureId());
if (hasFeatureId()) {
result = result && getFeatureId()
.equals(other.getFeatureId());
}
result = result && getAdditionalDataList()
.equals(other.getAdditionalDataList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasFeatureId()) {
hash = (37 * hash) + FEATURE_ID_FIELD_NUMBER;
hash = (53 * hash) + getFeatureId().hashCode();
}
if (getAdditionalDataCount() > 0) {
hash = (37 * hash) + ADDITIONAL_DATA_FIELD_NUMBER;
hash = (53 * hash) + getAdditionalDataList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.decision_trees.ModelAndFeatures.Feature}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.decision_trees.ModelAndFeatures.Feature)
tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.FeatureOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_ModelAndFeatures_Feature_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_ModelAndFeatures_Feature_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature.class, tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature.Builder.class);
}
// Construct using tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getAdditionalDataFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (featureIdBuilder_ == null) {
featureId_ = null;
} else {
featureId_ = null;
featureIdBuilder_ = null;
}
if (additionalDataBuilder_ == null) {
additionalData_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
additionalDataBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_ModelAndFeatures_Feature_descriptor;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature getDefaultInstanceForType() {
return tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature.getDefaultInstance();
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature build() {
tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature buildPartial() {
tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature result = new tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (featureIdBuilder_ == null) {
result.featureId_ = featureId_;
} else {
result.featureId_ = featureIdBuilder_.build();
}
if (additionalDataBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
additionalData_ = java.util.Collections.unmodifiableList(additionalData_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.additionalData_ = additionalData_;
} else {
result.additionalData_ = additionalDataBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature) {
return mergeFrom((tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature other) {
if (other == tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature.getDefaultInstance()) return this;
if (other.hasFeatureId()) {
mergeFeatureId(other.getFeatureId());
}
if (additionalDataBuilder_ == null) {
if (!other.additionalData_.isEmpty()) {
if (additionalData_.isEmpty()) {
additionalData_ = other.additionalData_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureAdditionalDataIsMutable();
additionalData_.addAll(other.additionalData_);
}
onChanged();
}
} else {
if (!other.additionalData_.isEmpty()) {
if (additionalDataBuilder_.isEmpty()) {
additionalDataBuilder_.dispose();
additionalDataBuilder_ = null;
additionalData_ = other.additionalData_;
bitField0_ = (bitField0_ & ~0x00000002);
additionalDataBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAdditionalDataFieldBuilder() : null;
} else {
additionalDataBuilder_.addAllMessages(other.additionalData_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private tensorflow.decision_trees.GenericTreeModel.FeatureId featureId_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.FeatureId, tensorflow.decision_trees.GenericTreeModel.FeatureId.Builder, tensorflow.decision_trees.GenericTreeModel.FeatureIdOrBuilder> featureIdBuilder_;
/**
*
* TODO(jonasz): Remove this field, as it's confusing. Ctx: cr/153569450.
*
*
* .tensorflow.decision_trees.FeatureId feature_id = 1 [deprecated = true];
*/
@java.lang.Deprecated public boolean hasFeatureId() {
return featureIdBuilder_ != null || featureId_ != null;
}
/**
*
* TODO(jonasz): Remove this field, as it's confusing. Ctx: cr/153569450.
*
*
* .tensorflow.decision_trees.FeatureId feature_id = 1 [deprecated = true];
*/
@java.lang.Deprecated public tensorflow.decision_trees.GenericTreeModel.FeatureId getFeatureId() {
if (featureIdBuilder_ == null) {
return featureId_ == null ? tensorflow.decision_trees.GenericTreeModel.FeatureId.getDefaultInstance() : featureId_;
} else {
return featureIdBuilder_.getMessage();
}
}
/**
*
* TODO(jonasz): Remove this field, as it's confusing. Ctx: cr/153569450.
*
*
* .tensorflow.decision_trees.FeatureId feature_id = 1 [deprecated = true];
*/
@java.lang.Deprecated public Builder setFeatureId(tensorflow.decision_trees.GenericTreeModel.FeatureId value) {
if (featureIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
featureId_ = value;
onChanged();
} else {
featureIdBuilder_.setMessage(value);
}
return this;
}
/**
*
* TODO(jonasz): Remove this field, as it's confusing. Ctx: cr/153569450.
*
*
* .tensorflow.decision_trees.FeatureId feature_id = 1 [deprecated = true];
*/
@java.lang.Deprecated public Builder setFeatureId(
tensorflow.decision_trees.GenericTreeModel.FeatureId.Builder builderForValue) {
if (featureIdBuilder_ == null) {
featureId_ = builderForValue.build();
onChanged();
} else {
featureIdBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* TODO(jonasz): Remove this field, as it's confusing. Ctx: cr/153569450.
*
*
* .tensorflow.decision_trees.FeatureId feature_id = 1 [deprecated = true];
*/
@java.lang.Deprecated public Builder mergeFeatureId(tensorflow.decision_trees.GenericTreeModel.FeatureId value) {
if (featureIdBuilder_ == null) {
if (featureId_ != null) {
featureId_ =
tensorflow.decision_trees.GenericTreeModel.FeatureId.newBuilder(featureId_).mergeFrom(value).buildPartial();
} else {
featureId_ = value;
}
onChanged();
} else {
featureIdBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* TODO(jonasz): Remove this field, as it's confusing. Ctx: cr/153569450.
*
*
* .tensorflow.decision_trees.FeatureId feature_id = 1 [deprecated = true];
*/
@java.lang.Deprecated public Builder clearFeatureId() {
if (featureIdBuilder_ == null) {
featureId_ = null;
onChanged();
} else {
featureId_ = null;
featureIdBuilder_ = null;
}
return this;
}
/**
*
* TODO(jonasz): Remove this field, as it's confusing. Ctx: cr/153569450.
*
*
* .tensorflow.decision_trees.FeatureId feature_id = 1 [deprecated = true];
*/
@java.lang.Deprecated public tensorflow.decision_trees.GenericTreeModel.FeatureId.Builder getFeatureIdBuilder() {
onChanged();
return getFeatureIdFieldBuilder().getBuilder();
}
/**
*
* TODO(jonasz): Remove this field, as it's confusing. Ctx: cr/153569450.
*
*
* .tensorflow.decision_trees.FeatureId feature_id = 1 [deprecated = true];
*/
@java.lang.Deprecated public tensorflow.decision_trees.GenericTreeModel.FeatureIdOrBuilder getFeatureIdOrBuilder() {
if (featureIdBuilder_ != null) {
return featureIdBuilder_.getMessageOrBuilder();
} else {
return featureId_ == null ?
tensorflow.decision_trees.GenericTreeModel.FeatureId.getDefaultInstance() : featureId_;
}
}
/**
*
* TODO(jonasz): Remove this field, as it's confusing. Ctx: cr/153569450.
*
*
* .tensorflow.decision_trees.FeatureId feature_id = 1 [deprecated = true];
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.FeatureId, tensorflow.decision_trees.GenericTreeModel.FeatureId.Builder, tensorflow.decision_trees.GenericTreeModel.FeatureIdOrBuilder>
getFeatureIdFieldBuilder() {
if (featureIdBuilder_ == null) {
featureIdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.FeatureId, tensorflow.decision_trees.GenericTreeModel.FeatureId.Builder, tensorflow.decision_trees.GenericTreeModel.FeatureIdOrBuilder>(
getFeatureId(),
getParentForChildren(),
isClean());
featureId_ = null;
}
return featureIdBuilder_;
}
private java.util.List additionalData_ =
java.util.Collections.emptyList();
private void ensureAdditionalDataIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
additionalData_ = new java.util.ArrayList(additionalData_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> additionalDataBuilder_;
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public java.util.List getAdditionalDataList() {
if (additionalDataBuilder_ == null) {
return java.util.Collections.unmodifiableList(additionalData_);
} else {
return additionalDataBuilder_.getMessageList();
}
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public int getAdditionalDataCount() {
if (additionalDataBuilder_ == null) {
return additionalData_.size();
} else {
return additionalDataBuilder_.getCount();
}
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public com.google.protobuf.Any getAdditionalData(int index) {
if (additionalDataBuilder_ == null) {
return additionalData_.get(index);
} else {
return additionalDataBuilder_.getMessage(index);
}
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder setAdditionalData(
int index, com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.set(index, value);
onChanged();
} else {
additionalDataBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder setAdditionalData(
int index, com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.set(index, builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder addAdditionalData(com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.add(value);
onChanged();
} else {
additionalDataBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder addAdditionalData(
int index, com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.add(index, value);
onChanged();
} else {
additionalDataBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder addAdditionalData(
com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.add(builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder addAdditionalData(
int index, com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.add(index, builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder addAllAdditionalData(
java.lang.Iterable extends com.google.protobuf.Any> values) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, additionalData_);
onChanged();
} else {
additionalDataBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder clearAdditionalData() {
if (additionalDataBuilder_ == null) {
additionalData_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
additionalDataBuilder_.clear();
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder removeAdditionalData(int index) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.remove(index);
onChanged();
} else {
additionalDataBuilder_.remove(index);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public com.google.protobuf.Any.Builder getAdditionalDataBuilder(
int index) {
return getAdditionalDataFieldBuilder().getBuilder(index);
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index) {
if (additionalDataBuilder_ == null) {
return additionalData_.get(index); } else {
return additionalDataBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList() {
if (additionalDataBuilder_ != null) {
return additionalDataBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(additionalData_);
}
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public com.google.protobuf.Any.Builder addAdditionalDataBuilder() {
return getAdditionalDataFieldBuilder().addBuilder(
com.google.protobuf.Any.getDefaultInstance());
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public com.google.protobuf.Any.Builder addAdditionalDataBuilder(
int index) {
return getAdditionalDataFieldBuilder().addBuilder(
index, com.google.protobuf.Any.getDefaultInstance());
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public java.util.List
getAdditionalDataBuilderList() {
return getAdditionalDataFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getAdditionalDataFieldBuilder() {
if (additionalDataBuilder_ == null) {
additionalDataBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
additionalData_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
additionalData_ = null;
}
return additionalDataBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.decision_trees.ModelAndFeatures.Feature)
}
// @@protoc_insertion_point(class_scope:tensorflow.decision_trees.ModelAndFeatures.Feature)
private static final tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature();
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Feature parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Feature(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int FEATURES_FIELD_NUMBER = 1;
private static final class FeaturesDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_ModelAndFeatures_FeaturesEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature.getDefaultInstance());
}
private com.google.protobuf.MapField<
java.lang.String, tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature> features_;
private com.google.protobuf.MapField
internalGetFeatures() {
if (features_ == null) {
return com.google.protobuf.MapField.emptyMapField(
FeaturesDefaultEntryHolder.defaultEntry);
}
return features_;
}
public int getFeaturesCount() {
return internalGetFeatures().getMap().size();
}
/**
*
* Given a FeatureId feature_id, the feature's description is in
* features[feature_id.id.value].
*
*
* map<string, .tensorflow.decision_trees.ModelAndFeatures.Feature> features = 1;
*/
public boolean containsFeatures(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetFeatures().getMap().containsKey(key);
}
/**
* Use {@link #getFeaturesMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getFeatures() {
return getFeaturesMap();
}
/**
*
* Given a FeatureId feature_id, the feature's description is in
* features[feature_id.id.value].
*
*
* map<string, .tensorflow.decision_trees.ModelAndFeatures.Feature> features = 1;
*/
public java.util.Map getFeaturesMap() {
return internalGetFeatures().getMap();
}
/**
*
* Given a FeatureId feature_id, the feature's description is in
* features[feature_id.id.value].
*
*
* map<string, .tensorflow.decision_trees.ModelAndFeatures.Feature> features = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature getFeaturesOrDefault(
java.lang.String key,
tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetFeatures().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Given a FeatureId feature_id, the feature's description is in
* features[feature_id.id.value].
*
*
* map<string, .tensorflow.decision_trees.ModelAndFeatures.Feature> features = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature getFeaturesOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetFeatures().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int MODEL_FIELD_NUMBER = 2;
private tensorflow.decision_trees.GenericTreeModel.Model model_;
/**
* .tensorflow.decision_trees.Model model = 2;
*/
public boolean hasModel() {
return model_ != null;
}
/**
* .tensorflow.decision_trees.Model model = 2;
*/
public tensorflow.decision_trees.GenericTreeModel.Model getModel() {
return model_ == null ? tensorflow.decision_trees.GenericTreeModel.Model.getDefaultInstance() : model_;
}
/**
* .tensorflow.decision_trees.Model model = 2;
*/
public tensorflow.decision_trees.GenericTreeModel.ModelOrBuilder getModelOrBuilder() {
return getModel();
}
public static final int ADDITIONAL_DATA_FIELD_NUMBER = 3;
private java.util.List additionalData_;
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public java.util.List getAdditionalDataList() {
return additionalData_;
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList() {
return additionalData_;
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public int getAdditionalDataCount() {
return additionalData_.size();
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public com.google.protobuf.Any getAdditionalData(int index) {
return additionalData_.get(index);
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index) {
return additionalData_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetFeatures(),
FeaturesDefaultEntryHolder.defaultEntry,
1);
if (model_ != null) {
output.writeMessage(2, getModel());
}
for (int i = 0; i < additionalData_.size(); i++) {
output.writeMessage(3, additionalData_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (java.util.Map.Entry entry
: internalGetFeatures().getMap().entrySet()) {
com.google.protobuf.MapEntry
features__ = FeaturesDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, features__);
}
if (model_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getModel());
}
for (int i = 0; i < additionalData_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, additionalData_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures)) {
return super.equals(obj);
}
tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures other = (tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures) obj;
boolean result = true;
result = result && internalGetFeatures().equals(
other.internalGetFeatures());
result = result && (hasModel() == other.hasModel());
if (hasModel()) {
result = result && getModel()
.equals(other.getModel());
}
result = result && getAdditionalDataList()
.equals(other.getAdditionalDataList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (!internalGetFeatures().getMap().isEmpty()) {
hash = (37 * hash) + FEATURES_FIELD_NUMBER;
hash = (53 * hash) + internalGetFeatures().hashCode();
}
if (hasModel()) {
hash = (37 * hash) + MODEL_FIELD_NUMBER;
hash = (53 * hash) + getModel().hashCode();
}
if (getAdditionalDataCount() > 0) {
hash = (37 * hash) + ADDITIONAL_DATA_FIELD_NUMBER;
hash = (53 * hash) + getAdditionalDataList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.decision_trees.ModelAndFeatures}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.decision_trees.ModelAndFeatures)
tensorflow.decision_trees.GenericTreeModel.ModelAndFeaturesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_ModelAndFeatures_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 1:
return internalGetFeatures();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 1:
return internalGetMutableFeatures();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_ModelAndFeatures_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.class, tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Builder.class);
}
// Construct using tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getAdditionalDataFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
internalGetMutableFeatures().clear();
if (modelBuilder_ == null) {
model_ = null;
} else {
model_ = null;
modelBuilder_ = null;
}
if (additionalDataBuilder_ == null) {
additionalData_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
additionalDataBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_ModelAndFeatures_descriptor;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures getDefaultInstanceForType() {
return tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.getDefaultInstance();
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures build() {
tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures buildPartial() {
tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures result = new tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.features_ = internalGetFeatures();
result.features_.makeImmutable();
if (modelBuilder_ == null) {
result.model_ = model_;
} else {
result.model_ = modelBuilder_.build();
}
if (additionalDataBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004)) {
additionalData_ = java.util.Collections.unmodifiableList(additionalData_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.additionalData_ = additionalData_;
} else {
result.additionalData_ = additionalDataBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures) {
return mergeFrom((tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures other) {
if (other == tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.getDefaultInstance()) return this;
internalGetMutableFeatures().mergeFrom(
other.internalGetFeatures());
if (other.hasModel()) {
mergeModel(other.getModel());
}
if (additionalDataBuilder_ == null) {
if (!other.additionalData_.isEmpty()) {
if (additionalData_.isEmpty()) {
additionalData_ = other.additionalData_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureAdditionalDataIsMutable();
additionalData_.addAll(other.additionalData_);
}
onChanged();
}
} else {
if (!other.additionalData_.isEmpty()) {
if (additionalDataBuilder_.isEmpty()) {
additionalDataBuilder_.dispose();
additionalDataBuilder_ = null;
additionalData_ = other.additionalData_;
bitField0_ = (bitField0_ & ~0x00000004);
additionalDataBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAdditionalDataFieldBuilder() : null;
} else {
additionalDataBuilder_.addAllMessages(other.additionalData_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.MapField<
java.lang.String, tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature> features_;
private com.google.protobuf.MapField
internalGetFeatures() {
if (features_ == null) {
return com.google.protobuf.MapField.emptyMapField(
FeaturesDefaultEntryHolder.defaultEntry);
}
return features_;
}
private com.google.protobuf.MapField
internalGetMutableFeatures() {
onChanged();;
if (features_ == null) {
features_ = com.google.protobuf.MapField.newMapField(
FeaturesDefaultEntryHolder.defaultEntry);
}
if (!features_.isMutable()) {
features_ = features_.copy();
}
return features_;
}
public int getFeaturesCount() {
return internalGetFeatures().getMap().size();
}
/**
*
* Given a FeatureId feature_id, the feature's description is in
* features[feature_id.id.value].
*
*
* map<string, .tensorflow.decision_trees.ModelAndFeatures.Feature> features = 1;
*/
public boolean containsFeatures(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetFeatures().getMap().containsKey(key);
}
/**
* Use {@link #getFeaturesMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getFeatures() {
return getFeaturesMap();
}
/**
*
* Given a FeatureId feature_id, the feature's description is in
* features[feature_id.id.value].
*
*
* map<string, .tensorflow.decision_trees.ModelAndFeatures.Feature> features = 1;
*/
public java.util.Map getFeaturesMap() {
return internalGetFeatures().getMap();
}
/**
*
* Given a FeatureId feature_id, the feature's description is in
* features[feature_id.id.value].
*
*
* map<string, .tensorflow.decision_trees.ModelAndFeatures.Feature> features = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature getFeaturesOrDefault(
java.lang.String key,
tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetFeatures().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Given a FeatureId feature_id, the feature's description is in
* features[feature_id.id.value].
*
*
* map<string, .tensorflow.decision_trees.ModelAndFeatures.Feature> features = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature getFeaturesOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetFeatures().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearFeatures() {
internalGetMutableFeatures().getMutableMap()
.clear();
return this;
}
/**
*
* Given a FeatureId feature_id, the feature's description is in
* features[feature_id.id.value].
*
*
* map<string, .tensorflow.decision_trees.ModelAndFeatures.Feature> features = 1;
*/
public Builder removeFeatures(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableFeatures().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableFeatures() {
return internalGetMutableFeatures().getMutableMap();
}
/**
*
* Given a FeatureId feature_id, the feature's description is in
* features[feature_id.id.value].
*
*
* map<string, .tensorflow.decision_trees.ModelAndFeatures.Feature> features = 1;
*/
public Builder putFeatures(
java.lang.String key,
tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures.Feature value) {
if (key == null) { throw new java.lang.NullPointerException(); }
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutableFeatures().getMutableMap()
.put(key, value);
return this;
}
/**
*
* Given a FeatureId feature_id, the feature's description is in
* features[feature_id.id.value].
*
*
* map<string, .tensorflow.decision_trees.ModelAndFeatures.Feature> features = 1;
*/
public Builder putAllFeatures(
java.util.Map values) {
internalGetMutableFeatures().getMutableMap()
.putAll(values);
return this;
}
private tensorflow.decision_trees.GenericTreeModel.Model model_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Model, tensorflow.decision_trees.GenericTreeModel.Model.Builder, tensorflow.decision_trees.GenericTreeModel.ModelOrBuilder> modelBuilder_;
/**
* .tensorflow.decision_trees.Model model = 2;
*/
public boolean hasModel() {
return modelBuilder_ != null || model_ != null;
}
/**
* .tensorflow.decision_trees.Model model = 2;
*/
public tensorflow.decision_trees.GenericTreeModel.Model getModel() {
if (modelBuilder_ == null) {
return model_ == null ? tensorflow.decision_trees.GenericTreeModel.Model.getDefaultInstance() : model_;
} else {
return modelBuilder_.getMessage();
}
}
/**
* .tensorflow.decision_trees.Model model = 2;
*/
public Builder setModel(tensorflow.decision_trees.GenericTreeModel.Model value) {
if (modelBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
model_ = value;
onChanged();
} else {
modelBuilder_.setMessage(value);
}
return this;
}
/**
* .tensorflow.decision_trees.Model model = 2;
*/
public Builder setModel(
tensorflow.decision_trees.GenericTreeModel.Model.Builder builderForValue) {
if (modelBuilder_ == null) {
model_ = builderForValue.build();
onChanged();
} else {
modelBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .tensorflow.decision_trees.Model model = 2;
*/
public Builder mergeModel(tensorflow.decision_trees.GenericTreeModel.Model value) {
if (modelBuilder_ == null) {
if (model_ != null) {
model_ =
tensorflow.decision_trees.GenericTreeModel.Model.newBuilder(model_).mergeFrom(value).buildPartial();
} else {
model_ = value;
}
onChanged();
} else {
modelBuilder_.mergeFrom(value);
}
return this;
}
/**
* .tensorflow.decision_trees.Model model = 2;
*/
public Builder clearModel() {
if (modelBuilder_ == null) {
model_ = null;
onChanged();
} else {
model_ = null;
modelBuilder_ = null;
}
return this;
}
/**
* .tensorflow.decision_trees.Model model = 2;
*/
public tensorflow.decision_trees.GenericTreeModel.Model.Builder getModelBuilder() {
onChanged();
return getModelFieldBuilder().getBuilder();
}
/**
* .tensorflow.decision_trees.Model model = 2;
*/
public tensorflow.decision_trees.GenericTreeModel.ModelOrBuilder getModelOrBuilder() {
if (modelBuilder_ != null) {
return modelBuilder_.getMessageOrBuilder();
} else {
return model_ == null ?
tensorflow.decision_trees.GenericTreeModel.Model.getDefaultInstance() : model_;
}
}
/**
* .tensorflow.decision_trees.Model model = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Model, tensorflow.decision_trees.GenericTreeModel.Model.Builder, tensorflow.decision_trees.GenericTreeModel.ModelOrBuilder>
getModelFieldBuilder() {
if (modelBuilder_ == null) {
modelBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Model, tensorflow.decision_trees.GenericTreeModel.Model.Builder, tensorflow.decision_trees.GenericTreeModel.ModelOrBuilder>(
getModel(),
getParentForChildren(),
isClean());
model_ = null;
}
return modelBuilder_;
}
private java.util.List additionalData_ =
java.util.Collections.emptyList();
private void ensureAdditionalDataIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
additionalData_ = new java.util.ArrayList(additionalData_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> additionalDataBuilder_;
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public java.util.List getAdditionalDataList() {
if (additionalDataBuilder_ == null) {
return java.util.Collections.unmodifiableList(additionalData_);
} else {
return additionalDataBuilder_.getMessageList();
}
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public int getAdditionalDataCount() {
if (additionalDataBuilder_ == null) {
return additionalData_.size();
} else {
return additionalDataBuilder_.getCount();
}
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public com.google.protobuf.Any getAdditionalData(int index) {
if (additionalDataBuilder_ == null) {
return additionalData_.get(index);
} else {
return additionalDataBuilder_.getMessage(index);
}
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder setAdditionalData(
int index, com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.set(index, value);
onChanged();
} else {
additionalDataBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder setAdditionalData(
int index, com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.set(index, builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder addAdditionalData(com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.add(value);
onChanged();
} else {
additionalDataBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder addAdditionalData(
int index, com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.add(index, value);
onChanged();
} else {
additionalDataBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder addAdditionalData(
com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.add(builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder addAdditionalData(
int index, com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.add(index, builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder addAllAdditionalData(
java.lang.Iterable extends com.google.protobuf.Any> values) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, additionalData_);
onChanged();
} else {
additionalDataBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder clearAdditionalData() {
if (additionalDataBuilder_ == null) {
additionalData_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
additionalDataBuilder_.clear();
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder removeAdditionalData(int index) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.remove(index);
onChanged();
} else {
additionalDataBuilder_.remove(index);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public com.google.protobuf.Any.Builder getAdditionalDataBuilder(
int index) {
return getAdditionalDataFieldBuilder().getBuilder(index);
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index) {
if (additionalDataBuilder_ == null) {
return additionalData_.get(index); } else {
return additionalDataBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList() {
if (additionalDataBuilder_ != null) {
return additionalDataBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(additionalData_);
}
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public com.google.protobuf.Any.Builder addAdditionalDataBuilder() {
return getAdditionalDataFieldBuilder().addBuilder(
com.google.protobuf.Any.getDefaultInstance());
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public com.google.protobuf.Any.Builder addAdditionalDataBuilder(
int index) {
return getAdditionalDataFieldBuilder().addBuilder(
index, com.google.protobuf.Any.getDefaultInstance());
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public java.util.List
getAdditionalDataBuilderList() {
return getAdditionalDataFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getAdditionalDataFieldBuilder() {
if (additionalDataBuilder_ == null) {
additionalDataBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
additionalData_,
((bitField0_ & 0x00000004) == 0x00000004),
getParentForChildren(),
isClean());
additionalData_ = null;
}
return additionalDataBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.decision_trees.ModelAndFeatures)
}
// @@protoc_insertion_point(class_scope:tensorflow.decision_trees.ModelAndFeatures)
private static final tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures();
}
public static tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ModelAndFeatures parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ModelAndFeatures(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.ModelAndFeatures getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface EnsembleOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.decision_trees.Ensemble)
com.google.protobuf.MessageOrBuilder {
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
java.util.List
getMembersList();
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
tensorflow.decision_trees.GenericTreeModel.Ensemble.Member getMembers(int index);
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
int getMembersCount();
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
java.util.List extends tensorflow.decision_trees.GenericTreeModel.Ensemble.MemberOrBuilder>
getMembersOrBuilderList();
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
tensorflow.decision_trees.GenericTreeModel.Ensemble.MemberOrBuilder getMembersOrBuilder(
int index);
/**
* .tensorflow.decision_trees.Summation summation_combination_technique = 1;
*/
boolean hasSummationCombinationTechnique();
/**
* .tensorflow.decision_trees.Summation summation_combination_technique = 1;
*/
tensorflow.decision_trees.GenericTreeModel.Summation getSummationCombinationTechnique();
/**
* .tensorflow.decision_trees.Summation summation_combination_technique = 1;
*/
tensorflow.decision_trees.GenericTreeModel.SummationOrBuilder getSummationCombinationTechniqueOrBuilder();
/**
* .tensorflow.decision_trees.Averaging averaging_combination_technique = 2;
*/
boolean hasAveragingCombinationTechnique();
/**
* .tensorflow.decision_trees.Averaging averaging_combination_technique = 2;
*/
tensorflow.decision_trees.GenericTreeModel.Averaging getAveragingCombinationTechnique();
/**
* .tensorflow.decision_trees.Averaging averaging_combination_technique = 2;
*/
tensorflow.decision_trees.GenericTreeModel.AveragingOrBuilder getAveragingCombinationTechniqueOrBuilder();
/**
* .google.protobuf.Any custom_combination_technique = 3;
*/
boolean hasCustomCombinationTechnique();
/**
* .google.protobuf.Any custom_combination_technique = 3;
*/
com.google.protobuf.Any getCustomCombinationTechnique();
/**
* .google.protobuf.Any custom_combination_technique = 3;
*/
com.google.protobuf.AnyOrBuilder getCustomCombinationTechniqueOrBuilder();
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
java.util.List
getAdditionalDataList();
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
com.google.protobuf.Any getAdditionalData(int index);
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
int getAdditionalDataCount();
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList();
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index);
public tensorflow.decision_trees.GenericTreeModel.Ensemble.CombinationTechniqueCase getCombinationTechniqueCase();
}
/**
*
* An ordered sequence of models. This message can be used to express bagged or
* boosted models, as well as custom ensembles.
*
*
* Protobuf type {@code tensorflow.decision_trees.Ensemble}
*/
public static final class Ensemble extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.decision_trees.Ensemble)
EnsembleOrBuilder {
private static final long serialVersionUID = 0L;
// Use Ensemble.newBuilder() to construct.
private Ensemble(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Ensemble() {
members_ = java.util.Collections.emptyList();
additionalData_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Ensemble(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
tensorflow.decision_trees.GenericTreeModel.Summation.Builder subBuilder = null;
if (combinationTechniqueCase_ == 1) {
subBuilder = ((tensorflow.decision_trees.GenericTreeModel.Summation) combinationTechnique_).toBuilder();
}
combinationTechnique_ =
input.readMessage(tensorflow.decision_trees.GenericTreeModel.Summation.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((tensorflow.decision_trees.GenericTreeModel.Summation) combinationTechnique_);
combinationTechnique_ = subBuilder.buildPartial();
}
combinationTechniqueCase_ = 1;
break;
}
case 18: {
tensorflow.decision_trees.GenericTreeModel.Averaging.Builder subBuilder = null;
if (combinationTechniqueCase_ == 2) {
subBuilder = ((tensorflow.decision_trees.GenericTreeModel.Averaging) combinationTechnique_).toBuilder();
}
combinationTechnique_ =
input.readMessage(tensorflow.decision_trees.GenericTreeModel.Averaging.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((tensorflow.decision_trees.GenericTreeModel.Averaging) combinationTechnique_);
combinationTechnique_ = subBuilder.buildPartial();
}
combinationTechniqueCase_ = 2;
break;
}
case 26: {
com.google.protobuf.Any.Builder subBuilder = null;
if (combinationTechniqueCase_ == 3) {
subBuilder = ((com.google.protobuf.Any) combinationTechnique_).toBuilder();
}
combinationTechnique_ =
input.readMessage(com.google.protobuf.Any.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((com.google.protobuf.Any) combinationTechnique_);
combinationTechnique_ = subBuilder.buildPartial();
}
combinationTechniqueCase_ = 3;
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
additionalData_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
additionalData_.add(
input.readMessage(com.google.protobuf.Any.parser(), extensionRegistry));
break;
}
case 802: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
members_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
members_.add(
input.readMessage(tensorflow.decision_trees.GenericTreeModel.Ensemble.Member.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
additionalData_ = java.util.Collections.unmodifiableList(additionalData_);
}
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
members_ = java.util.Collections.unmodifiableList(members_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Ensemble_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Ensemble_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.Ensemble.class, tensorflow.decision_trees.GenericTreeModel.Ensemble.Builder.class);
}
public interface MemberOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.decision_trees.Ensemble.Member)
com.google.protobuf.MessageOrBuilder {
/**
* .tensorflow.decision_trees.Model submodel = 1;
*/
boolean hasSubmodel();
/**
* .tensorflow.decision_trees.Model submodel = 1;
*/
tensorflow.decision_trees.GenericTreeModel.Model getSubmodel();
/**
* .tensorflow.decision_trees.Model submodel = 1;
*/
tensorflow.decision_trees.GenericTreeModel.ModelOrBuilder getSubmodelOrBuilder();
/**
* .google.protobuf.Int32Value submodel_id = 2;
*/
boolean hasSubmodelId();
/**
* .google.protobuf.Int32Value submodel_id = 2;
*/
com.google.protobuf.Int32Value getSubmodelId();
/**
* .google.protobuf.Int32Value submodel_id = 2;
*/
com.google.protobuf.Int32ValueOrBuilder getSubmodelIdOrBuilder();
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
java.util.List
getAdditionalDataList();
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
com.google.protobuf.Any getAdditionalData(int index);
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
int getAdditionalDataCount();
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList();
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index);
}
/**
* Protobuf type {@code tensorflow.decision_trees.Ensemble.Member}
*/
public static final class Member extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.decision_trees.Ensemble.Member)
MemberOrBuilder {
private static final long serialVersionUID = 0L;
// Use Member.newBuilder() to construct.
private Member(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Member() {
additionalData_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Member(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
tensorflow.decision_trees.GenericTreeModel.Model.Builder subBuilder = null;
if (submodel_ != null) {
subBuilder = submodel_.toBuilder();
}
submodel_ = input.readMessage(tensorflow.decision_trees.GenericTreeModel.Model.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(submodel_);
submodel_ = subBuilder.buildPartial();
}
break;
}
case 18: {
com.google.protobuf.Int32Value.Builder subBuilder = null;
if (submodelId_ != null) {
subBuilder = submodelId_.toBuilder();
}
submodelId_ = input.readMessage(com.google.protobuf.Int32Value.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(submodelId_);
submodelId_ = subBuilder.buildPartial();
}
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
additionalData_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
additionalData_.add(
input.readMessage(com.google.protobuf.Any.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
additionalData_ = java.util.Collections.unmodifiableList(additionalData_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Ensemble_Member_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Ensemble_Member_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.Ensemble.Member.class, tensorflow.decision_trees.GenericTreeModel.Ensemble.Member.Builder.class);
}
private int bitField0_;
public static final int SUBMODEL_FIELD_NUMBER = 1;
private tensorflow.decision_trees.GenericTreeModel.Model submodel_;
/**
* .tensorflow.decision_trees.Model submodel = 1;
*/
public boolean hasSubmodel() {
return submodel_ != null;
}
/**
* .tensorflow.decision_trees.Model submodel = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.Model getSubmodel() {
return submodel_ == null ? tensorflow.decision_trees.GenericTreeModel.Model.getDefaultInstance() : submodel_;
}
/**
* .tensorflow.decision_trees.Model submodel = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.ModelOrBuilder getSubmodelOrBuilder() {
return getSubmodel();
}
public static final int SUBMODEL_ID_FIELD_NUMBER = 2;
private com.google.protobuf.Int32Value submodelId_;
/**
* .google.protobuf.Int32Value submodel_id = 2;
*/
public boolean hasSubmodelId() {
return submodelId_ != null;
}
/**
* .google.protobuf.Int32Value submodel_id = 2;
*/
public com.google.protobuf.Int32Value getSubmodelId() {
return submodelId_ == null ? com.google.protobuf.Int32Value.getDefaultInstance() : submodelId_;
}
/**
* .google.protobuf.Int32Value submodel_id = 2;
*/
public com.google.protobuf.Int32ValueOrBuilder getSubmodelIdOrBuilder() {
return getSubmodelId();
}
public static final int ADDITIONAL_DATA_FIELD_NUMBER = 3;
private java.util.List additionalData_;
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public java.util.List getAdditionalDataList() {
return additionalData_;
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList() {
return additionalData_;
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public int getAdditionalDataCount() {
return additionalData_.size();
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public com.google.protobuf.Any getAdditionalData(int index) {
return additionalData_.get(index);
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index) {
return additionalData_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (submodel_ != null) {
output.writeMessage(1, getSubmodel());
}
if (submodelId_ != null) {
output.writeMessage(2, getSubmodelId());
}
for (int i = 0; i < additionalData_.size(); i++) {
output.writeMessage(3, additionalData_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (submodel_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getSubmodel());
}
if (submodelId_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getSubmodelId());
}
for (int i = 0; i < additionalData_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, additionalData_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.decision_trees.GenericTreeModel.Ensemble.Member)) {
return super.equals(obj);
}
tensorflow.decision_trees.GenericTreeModel.Ensemble.Member other = (tensorflow.decision_trees.GenericTreeModel.Ensemble.Member) obj;
boolean result = true;
result = result && (hasSubmodel() == other.hasSubmodel());
if (hasSubmodel()) {
result = result && getSubmodel()
.equals(other.getSubmodel());
}
result = result && (hasSubmodelId() == other.hasSubmodelId());
if (hasSubmodelId()) {
result = result && getSubmodelId()
.equals(other.getSubmodelId());
}
result = result && getAdditionalDataList()
.equals(other.getAdditionalDataList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasSubmodel()) {
hash = (37 * hash) + SUBMODEL_FIELD_NUMBER;
hash = (53 * hash) + getSubmodel().hashCode();
}
if (hasSubmodelId()) {
hash = (37 * hash) + SUBMODEL_ID_FIELD_NUMBER;
hash = (53 * hash) + getSubmodelId().hashCode();
}
if (getAdditionalDataCount() > 0) {
hash = (37 * hash) + ADDITIONAL_DATA_FIELD_NUMBER;
hash = (53 * hash) + getAdditionalDataList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble.Member parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble.Member parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble.Member parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble.Member parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble.Member parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble.Member parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble.Member parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble.Member parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble.Member parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble.Member parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble.Member parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble.Member parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.decision_trees.GenericTreeModel.Ensemble.Member prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.decision_trees.Ensemble.Member}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.decision_trees.Ensemble.Member)
tensorflow.decision_trees.GenericTreeModel.Ensemble.MemberOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Ensemble_Member_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Ensemble_Member_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.Ensemble.Member.class, tensorflow.decision_trees.GenericTreeModel.Ensemble.Member.Builder.class);
}
// Construct using tensorflow.decision_trees.GenericTreeModel.Ensemble.Member.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getAdditionalDataFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (submodelBuilder_ == null) {
submodel_ = null;
} else {
submodel_ = null;
submodelBuilder_ = null;
}
if (submodelIdBuilder_ == null) {
submodelId_ = null;
} else {
submodelId_ = null;
submodelIdBuilder_ = null;
}
if (additionalDataBuilder_ == null) {
additionalData_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
additionalDataBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Ensemble_Member_descriptor;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Ensemble.Member getDefaultInstanceForType() {
return tensorflow.decision_trees.GenericTreeModel.Ensemble.Member.getDefaultInstance();
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Ensemble.Member build() {
tensorflow.decision_trees.GenericTreeModel.Ensemble.Member result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Ensemble.Member buildPartial() {
tensorflow.decision_trees.GenericTreeModel.Ensemble.Member result = new tensorflow.decision_trees.GenericTreeModel.Ensemble.Member(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (submodelBuilder_ == null) {
result.submodel_ = submodel_;
} else {
result.submodel_ = submodelBuilder_.build();
}
if (submodelIdBuilder_ == null) {
result.submodelId_ = submodelId_;
} else {
result.submodelId_ = submodelIdBuilder_.build();
}
if (additionalDataBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004)) {
additionalData_ = java.util.Collections.unmodifiableList(additionalData_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.additionalData_ = additionalData_;
} else {
result.additionalData_ = additionalDataBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.decision_trees.GenericTreeModel.Ensemble.Member) {
return mergeFrom((tensorflow.decision_trees.GenericTreeModel.Ensemble.Member)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.decision_trees.GenericTreeModel.Ensemble.Member other) {
if (other == tensorflow.decision_trees.GenericTreeModel.Ensemble.Member.getDefaultInstance()) return this;
if (other.hasSubmodel()) {
mergeSubmodel(other.getSubmodel());
}
if (other.hasSubmodelId()) {
mergeSubmodelId(other.getSubmodelId());
}
if (additionalDataBuilder_ == null) {
if (!other.additionalData_.isEmpty()) {
if (additionalData_.isEmpty()) {
additionalData_ = other.additionalData_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureAdditionalDataIsMutable();
additionalData_.addAll(other.additionalData_);
}
onChanged();
}
} else {
if (!other.additionalData_.isEmpty()) {
if (additionalDataBuilder_.isEmpty()) {
additionalDataBuilder_.dispose();
additionalDataBuilder_ = null;
additionalData_ = other.additionalData_;
bitField0_ = (bitField0_ & ~0x00000004);
additionalDataBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAdditionalDataFieldBuilder() : null;
} else {
additionalDataBuilder_.addAllMessages(other.additionalData_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.decision_trees.GenericTreeModel.Ensemble.Member parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.decision_trees.GenericTreeModel.Ensemble.Member) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private tensorflow.decision_trees.GenericTreeModel.Model submodel_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Model, tensorflow.decision_trees.GenericTreeModel.Model.Builder, tensorflow.decision_trees.GenericTreeModel.ModelOrBuilder> submodelBuilder_;
/**
* .tensorflow.decision_trees.Model submodel = 1;
*/
public boolean hasSubmodel() {
return submodelBuilder_ != null || submodel_ != null;
}
/**
* .tensorflow.decision_trees.Model submodel = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.Model getSubmodel() {
if (submodelBuilder_ == null) {
return submodel_ == null ? tensorflow.decision_trees.GenericTreeModel.Model.getDefaultInstance() : submodel_;
} else {
return submodelBuilder_.getMessage();
}
}
/**
* .tensorflow.decision_trees.Model submodel = 1;
*/
public Builder setSubmodel(tensorflow.decision_trees.GenericTreeModel.Model value) {
if (submodelBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
submodel_ = value;
onChanged();
} else {
submodelBuilder_.setMessage(value);
}
return this;
}
/**
* .tensorflow.decision_trees.Model submodel = 1;
*/
public Builder setSubmodel(
tensorflow.decision_trees.GenericTreeModel.Model.Builder builderForValue) {
if (submodelBuilder_ == null) {
submodel_ = builderForValue.build();
onChanged();
} else {
submodelBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .tensorflow.decision_trees.Model submodel = 1;
*/
public Builder mergeSubmodel(tensorflow.decision_trees.GenericTreeModel.Model value) {
if (submodelBuilder_ == null) {
if (submodel_ != null) {
submodel_ =
tensorflow.decision_trees.GenericTreeModel.Model.newBuilder(submodel_).mergeFrom(value).buildPartial();
} else {
submodel_ = value;
}
onChanged();
} else {
submodelBuilder_.mergeFrom(value);
}
return this;
}
/**
* .tensorflow.decision_trees.Model submodel = 1;
*/
public Builder clearSubmodel() {
if (submodelBuilder_ == null) {
submodel_ = null;
onChanged();
} else {
submodel_ = null;
submodelBuilder_ = null;
}
return this;
}
/**
* .tensorflow.decision_trees.Model submodel = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.Model.Builder getSubmodelBuilder() {
onChanged();
return getSubmodelFieldBuilder().getBuilder();
}
/**
* .tensorflow.decision_trees.Model submodel = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.ModelOrBuilder getSubmodelOrBuilder() {
if (submodelBuilder_ != null) {
return submodelBuilder_.getMessageOrBuilder();
} else {
return submodel_ == null ?
tensorflow.decision_trees.GenericTreeModel.Model.getDefaultInstance() : submodel_;
}
}
/**
* .tensorflow.decision_trees.Model submodel = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Model, tensorflow.decision_trees.GenericTreeModel.Model.Builder, tensorflow.decision_trees.GenericTreeModel.ModelOrBuilder>
getSubmodelFieldBuilder() {
if (submodelBuilder_ == null) {
submodelBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Model, tensorflow.decision_trees.GenericTreeModel.Model.Builder, tensorflow.decision_trees.GenericTreeModel.ModelOrBuilder>(
getSubmodel(),
getParentForChildren(),
isClean());
submodel_ = null;
}
return submodelBuilder_;
}
private com.google.protobuf.Int32Value submodelId_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int32Value, com.google.protobuf.Int32Value.Builder, com.google.protobuf.Int32ValueOrBuilder> submodelIdBuilder_;
/**
* .google.protobuf.Int32Value submodel_id = 2;
*/
public boolean hasSubmodelId() {
return submodelIdBuilder_ != null || submodelId_ != null;
}
/**
* .google.protobuf.Int32Value submodel_id = 2;
*/
public com.google.protobuf.Int32Value getSubmodelId() {
if (submodelIdBuilder_ == null) {
return submodelId_ == null ? com.google.protobuf.Int32Value.getDefaultInstance() : submodelId_;
} else {
return submodelIdBuilder_.getMessage();
}
}
/**
* .google.protobuf.Int32Value submodel_id = 2;
*/
public Builder setSubmodelId(com.google.protobuf.Int32Value value) {
if (submodelIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
submodelId_ = value;
onChanged();
} else {
submodelIdBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.Int32Value submodel_id = 2;
*/
public Builder setSubmodelId(
com.google.protobuf.Int32Value.Builder builderForValue) {
if (submodelIdBuilder_ == null) {
submodelId_ = builderForValue.build();
onChanged();
} else {
submodelIdBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.Int32Value submodel_id = 2;
*/
public Builder mergeSubmodelId(com.google.protobuf.Int32Value value) {
if (submodelIdBuilder_ == null) {
if (submodelId_ != null) {
submodelId_ =
com.google.protobuf.Int32Value.newBuilder(submodelId_).mergeFrom(value).buildPartial();
} else {
submodelId_ = value;
}
onChanged();
} else {
submodelIdBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.Int32Value submodel_id = 2;
*/
public Builder clearSubmodelId() {
if (submodelIdBuilder_ == null) {
submodelId_ = null;
onChanged();
} else {
submodelId_ = null;
submodelIdBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.Int32Value submodel_id = 2;
*/
public com.google.protobuf.Int32Value.Builder getSubmodelIdBuilder() {
onChanged();
return getSubmodelIdFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Int32Value submodel_id = 2;
*/
public com.google.protobuf.Int32ValueOrBuilder getSubmodelIdOrBuilder() {
if (submodelIdBuilder_ != null) {
return submodelIdBuilder_.getMessageOrBuilder();
} else {
return submodelId_ == null ?
com.google.protobuf.Int32Value.getDefaultInstance() : submodelId_;
}
}
/**
* .google.protobuf.Int32Value submodel_id = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int32Value, com.google.protobuf.Int32Value.Builder, com.google.protobuf.Int32ValueOrBuilder>
getSubmodelIdFieldBuilder() {
if (submodelIdBuilder_ == null) {
submodelIdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int32Value, com.google.protobuf.Int32Value.Builder, com.google.protobuf.Int32ValueOrBuilder>(
getSubmodelId(),
getParentForChildren(),
isClean());
submodelId_ = null;
}
return submodelIdBuilder_;
}
private java.util.List additionalData_ =
java.util.Collections.emptyList();
private void ensureAdditionalDataIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
additionalData_ = new java.util.ArrayList(additionalData_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> additionalDataBuilder_;
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public java.util.List getAdditionalDataList() {
if (additionalDataBuilder_ == null) {
return java.util.Collections.unmodifiableList(additionalData_);
} else {
return additionalDataBuilder_.getMessageList();
}
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public int getAdditionalDataCount() {
if (additionalDataBuilder_ == null) {
return additionalData_.size();
} else {
return additionalDataBuilder_.getCount();
}
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public com.google.protobuf.Any getAdditionalData(int index) {
if (additionalDataBuilder_ == null) {
return additionalData_.get(index);
} else {
return additionalDataBuilder_.getMessage(index);
}
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder setAdditionalData(
int index, com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.set(index, value);
onChanged();
} else {
additionalDataBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder setAdditionalData(
int index, com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.set(index, builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder addAdditionalData(com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.add(value);
onChanged();
} else {
additionalDataBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder addAdditionalData(
int index, com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.add(index, value);
onChanged();
} else {
additionalDataBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder addAdditionalData(
com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.add(builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder addAdditionalData(
int index, com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.add(index, builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder addAllAdditionalData(
java.lang.Iterable extends com.google.protobuf.Any> values) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, additionalData_);
onChanged();
} else {
additionalDataBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder clearAdditionalData() {
if (additionalDataBuilder_ == null) {
additionalData_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
additionalDataBuilder_.clear();
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder removeAdditionalData(int index) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.remove(index);
onChanged();
} else {
additionalDataBuilder_.remove(index);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public com.google.protobuf.Any.Builder getAdditionalDataBuilder(
int index) {
return getAdditionalDataFieldBuilder().getBuilder(index);
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index) {
if (additionalDataBuilder_ == null) {
return additionalData_.get(index); } else {
return additionalDataBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList() {
if (additionalDataBuilder_ != null) {
return additionalDataBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(additionalData_);
}
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public com.google.protobuf.Any.Builder addAdditionalDataBuilder() {
return getAdditionalDataFieldBuilder().addBuilder(
com.google.protobuf.Any.getDefaultInstance());
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public com.google.protobuf.Any.Builder addAdditionalDataBuilder(
int index) {
return getAdditionalDataFieldBuilder().addBuilder(
index, com.google.protobuf.Any.getDefaultInstance());
}
/**
* repeated .google.protobuf.Any additional_data = 3;
*/
public java.util.List
getAdditionalDataBuilderList() {
return getAdditionalDataFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getAdditionalDataFieldBuilder() {
if (additionalDataBuilder_ == null) {
additionalDataBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
additionalData_,
((bitField0_ & 0x00000004) == 0x00000004),
getParentForChildren(),
isClean());
additionalData_ = null;
}
return additionalDataBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.decision_trees.Ensemble.Member)
}
// @@protoc_insertion_point(class_scope:tensorflow.decision_trees.Ensemble.Member)
private static final tensorflow.decision_trees.GenericTreeModel.Ensemble.Member DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.decision_trees.GenericTreeModel.Ensemble.Member();
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble.Member getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Member parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Member(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Ensemble.Member getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
private int combinationTechniqueCase_ = 0;
private java.lang.Object combinationTechnique_;
public enum CombinationTechniqueCase
implements com.google.protobuf.Internal.EnumLite {
SUMMATION_COMBINATION_TECHNIQUE(1),
AVERAGING_COMBINATION_TECHNIQUE(2),
CUSTOM_COMBINATION_TECHNIQUE(3),
COMBINATIONTECHNIQUE_NOT_SET(0);
private final int value;
private CombinationTechniqueCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static CombinationTechniqueCase valueOf(int value) {
return forNumber(value);
}
public static CombinationTechniqueCase forNumber(int value) {
switch (value) {
case 1: return SUMMATION_COMBINATION_TECHNIQUE;
case 2: return AVERAGING_COMBINATION_TECHNIQUE;
case 3: return CUSTOM_COMBINATION_TECHNIQUE;
case 0: return COMBINATIONTECHNIQUE_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public CombinationTechniqueCase
getCombinationTechniqueCase() {
return CombinationTechniqueCase.forNumber(
combinationTechniqueCase_);
}
public static final int MEMBERS_FIELD_NUMBER = 100;
private java.util.List members_;
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
public java.util.List getMembersList() {
return members_;
}
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
public java.util.List extends tensorflow.decision_trees.GenericTreeModel.Ensemble.MemberOrBuilder>
getMembersOrBuilderList() {
return members_;
}
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
public int getMembersCount() {
return members_.size();
}
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
public tensorflow.decision_trees.GenericTreeModel.Ensemble.Member getMembers(int index) {
return members_.get(index);
}
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
public tensorflow.decision_trees.GenericTreeModel.Ensemble.MemberOrBuilder getMembersOrBuilder(
int index) {
return members_.get(index);
}
public static final int SUMMATION_COMBINATION_TECHNIQUE_FIELD_NUMBER = 1;
/**
* .tensorflow.decision_trees.Summation summation_combination_technique = 1;
*/
public boolean hasSummationCombinationTechnique() {
return combinationTechniqueCase_ == 1;
}
/**
* .tensorflow.decision_trees.Summation summation_combination_technique = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.Summation getSummationCombinationTechnique() {
if (combinationTechniqueCase_ == 1) {
return (tensorflow.decision_trees.GenericTreeModel.Summation) combinationTechnique_;
}
return tensorflow.decision_trees.GenericTreeModel.Summation.getDefaultInstance();
}
/**
* .tensorflow.decision_trees.Summation summation_combination_technique = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.SummationOrBuilder getSummationCombinationTechniqueOrBuilder() {
if (combinationTechniqueCase_ == 1) {
return (tensorflow.decision_trees.GenericTreeModel.Summation) combinationTechnique_;
}
return tensorflow.decision_trees.GenericTreeModel.Summation.getDefaultInstance();
}
public static final int AVERAGING_COMBINATION_TECHNIQUE_FIELD_NUMBER = 2;
/**
* .tensorflow.decision_trees.Averaging averaging_combination_technique = 2;
*/
public boolean hasAveragingCombinationTechnique() {
return combinationTechniqueCase_ == 2;
}
/**
* .tensorflow.decision_trees.Averaging averaging_combination_technique = 2;
*/
public tensorflow.decision_trees.GenericTreeModel.Averaging getAveragingCombinationTechnique() {
if (combinationTechniqueCase_ == 2) {
return (tensorflow.decision_trees.GenericTreeModel.Averaging) combinationTechnique_;
}
return tensorflow.decision_trees.GenericTreeModel.Averaging.getDefaultInstance();
}
/**
* .tensorflow.decision_trees.Averaging averaging_combination_technique = 2;
*/
public tensorflow.decision_trees.GenericTreeModel.AveragingOrBuilder getAveragingCombinationTechniqueOrBuilder() {
if (combinationTechniqueCase_ == 2) {
return (tensorflow.decision_trees.GenericTreeModel.Averaging) combinationTechnique_;
}
return tensorflow.decision_trees.GenericTreeModel.Averaging.getDefaultInstance();
}
public static final int CUSTOM_COMBINATION_TECHNIQUE_FIELD_NUMBER = 3;
/**
* .google.protobuf.Any custom_combination_technique = 3;
*/
public boolean hasCustomCombinationTechnique() {
return combinationTechniqueCase_ == 3;
}
/**
* .google.protobuf.Any custom_combination_technique = 3;
*/
public com.google.protobuf.Any getCustomCombinationTechnique() {
if (combinationTechniqueCase_ == 3) {
return (com.google.protobuf.Any) combinationTechnique_;
}
return com.google.protobuf.Any.getDefaultInstance();
}
/**
* .google.protobuf.Any custom_combination_technique = 3;
*/
public com.google.protobuf.AnyOrBuilder getCustomCombinationTechniqueOrBuilder() {
if (combinationTechniqueCase_ == 3) {
return (com.google.protobuf.Any) combinationTechnique_;
}
return com.google.protobuf.Any.getDefaultInstance();
}
public static final int ADDITIONAL_DATA_FIELD_NUMBER = 4;
private java.util.List additionalData_;
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public java.util.List getAdditionalDataList() {
return additionalData_;
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList() {
return additionalData_;
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public int getAdditionalDataCount() {
return additionalData_.size();
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public com.google.protobuf.Any getAdditionalData(int index) {
return additionalData_.get(index);
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index) {
return additionalData_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (combinationTechniqueCase_ == 1) {
output.writeMessage(1, (tensorflow.decision_trees.GenericTreeModel.Summation) combinationTechnique_);
}
if (combinationTechniqueCase_ == 2) {
output.writeMessage(2, (tensorflow.decision_trees.GenericTreeModel.Averaging) combinationTechnique_);
}
if (combinationTechniqueCase_ == 3) {
output.writeMessage(3, (com.google.protobuf.Any) combinationTechnique_);
}
for (int i = 0; i < additionalData_.size(); i++) {
output.writeMessage(4, additionalData_.get(i));
}
for (int i = 0; i < members_.size(); i++) {
output.writeMessage(100, members_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (combinationTechniqueCase_ == 1) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, (tensorflow.decision_trees.GenericTreeModel.Summation) combinationTechnique_);
}
if (combinationTechniqueCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, (tensorflow.decision_trees.GenericTreeModel.Averaging) combinationTechnique_);
}
if (combinationTechniqueCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, (com.google.protobuf.Any) combinationTechnique_);
}
for (int i = 0; i < additionalData_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, additionalData_.get(i));
}
for (int i = 0; i < members_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(100, members_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.decision_trees.GenericTreeModel.Ensemble)) {
return super.equals(obj);
}
tensorflow.decision_trees.GenericTreeModel.Ensemble other = (tensorflow.decision_trees.GenericTreeModel.Ensemble) obj;
boolean result = true;
result = result && getMembersList()
.equals(other.getMembersList());
result = result && getAdditionalDataList()
.equals(other.getAdditionalDataList());
result = result && getCombinationTechniqueCase().equals(
other.getCombinationTechniqueCase());
if (!result) return false;
switch (combinationTechniqueCase_) {
case 1:
result = result && getSummationCombinationTechnique()
.equals(other.getSummationCombinationTechnique());
break;
case 2:
result = result && getAveragingCombinationTechnique()
.equals(other.getAveragingCombinationTechnique());
break;
case 3:
result = result && getCustomCombinationTechnique()
.equals(other.getCustomCombinationTechnique());
break;
case 0:
default:
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getMembersCount() > 0) {
hash = (37 * hash) + MEMBERS_FIELD_NUMBER;
hash = (53 * hash) + getMembersList().hashCode();
}
if (getAdditionalDataCount() > 0) {
hash = (37 * hash) + ADDITIONAL_DATA_FIELD_NUMBER;
hash = (53 * hash) + getAdditionalDataList().hashCode();
}
switch (combinationTechniqueCase_) {
case 1:
hash = (37 * hash) + SUMMATION_COMBINATION_TECHNIQUE_FIELD_NUMBER;
hash = (53 * hash) + getSummationCombinationTechnique().hashCode();
break;
case 2:
hash = (37 * hash) + AVERAGING_COMBINATION_TECHNIQUE_FIELD_NUMBER;
hash = (53 * hash) + getAveragingCombinationTechnique().hashCode();
break;
case 3:
hash = (37 * hash) + CUSTOM_COMBINATION_TECHNIQUE_FIELD_NUMBER;
hash = (53 * hash) + getCustomCombinationTechnique().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.decision_trees.GenericTreeModel.Ensemble prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* An ordered sequence of models. This message can be used to express bagged or
* boosted models, as well as custom ensembles.
*
*
* Protobuf type {@code tensorflow.decision_trees.Ensemble}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.decision_trees.Ensemble)
tensorflow.decision_trees.GenericTreeModel.EnsembleOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Ensemble_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Ensemble_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.Ensemble.class, tensorflow.decision_trees.GenericTreeModel.Ensemble.Builder.class);
}
// Construct using tensorflow.decision_trees.GenericTreeModel.Ensemble.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getMembersFieldBuilder();
getAdditionalDataFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (membersBuilder_ == null) {
members_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
membersBuilder_.clear();
}
if (additionalDataBuilder_ == null) {
additionalData_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
} else {
additionalDataBuilder_.clear();
}
combinationTechniqueCase_ = 0;
combinationTechnique_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Ensemble_descriptor;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Ensemble getDefaultInstanceForType() {
return tensorflow.decision_trees.GenericTreeModel.Ensemble.getDefaultInstance();
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Ensemble build() {
tensorflow.decision_trees.GenericTreeModel.Ensemble result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Ensemble buildPartial() {
tensorflow.decision_trees.GenericTreeModel.Ensemble result = new tensorflow.decision_trees.GenericTreeModel.Ensemble(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (membersBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
members_ = java.util.Collections.unmodifiableList(members_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.members_ = members_;
} else {
result.members_ = membersBuilder_.build();
}
if (combinationTechniqueCase_ == 1) {
if (summationCombinationTechniqueBuilder_ == null) {
result.combinationTechnique_ = combinationTechnique_;
} else {
result.combinationTechnique_ = summationCombinationTechniqueBuilder_.build();
}
}
if (combinationTechniqueCase_ == 2) {
if (averagingCombinationTechniqueBuilder_ == null) {
result.combinationTechnique_ = combinationTechnique_;
} else {
result.combinationTechnique_ = averagingCombinationTechniqueBuilder_.build();
}
}
if (combinationTechniqueCase_ == 3) {
if (customCombinationTechniqueBuilder_ == null) {
result.combinationTechnique_ = combinationTechnique_;
} else {
result.combinationTechnique_ = customCombinationTechniqueBuilder_.build();
}
}
if (additionalDataBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010)) {
additionalData_ = java.util.Collections.unmodifiableList(additionalData_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.additionalData_ = additionalData_;
} else {
result.additionalData_ = additionalDataBuilder_.build();
}
result.bitField0_ = to_bitField0_;
result.combinationTechniqueCase_ = combinationTechniqueCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.decision_trees.GenericTreeModel.Ensemble) {
return mergeFrom((tensorflow.decision_trees.GenericTreeModel.Ensemble)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.decision_trees.GenericTreeModel.Ensemble other) {
if (other == tensorflow.decision_trees.GenericTreeModel.Ensemble.getDefaultInstance()) return this;
if (membersBuilder_ == null) {
if (!other.members_.isEmpty()) {
if (members_.isEmpty()) {
members_ = other.members_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureMembersIsMutable();
members_.addAll(other.members_);
}
onChanged();
}
} else {
if (!other.members_.isEmpty()) {
if (membersBuilder_.isEmpty()) {
membersBuilder_.dispose();
membersBuilder_ = null;
members_ = other.members_;
bitField0_ = (bitField0_ & ~0x00000001);
membersBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getMembersFieldBuilder() : null;
} else {
membersBuilder_.addAllMessages(other.members_);
}
}
}
if (additionalDataBuilder_ == null) {
if (!other.additionalData_.isEmpty()) {
if (additionalData_.isEmpty()) {
additionalData_ = other.additionalData_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureAdditionalDataIsMutable();
additionalData_.addAll(other.additionalData_);
}
onChanged();
}
} else {
if (!other.additionalData_.isEmpty()) {
if (additionalDataBuilder_.isEmpty()) {
additionalDataBuilder_.dispose();
additionalDataBuilder_ = null;
additionalData_ = other.additionalData_;
bitField0_ = (bitField0_ & ~0x00000010);
additionalDataBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAdditionalDataFieldBuilder() : null;
} else {
additionalDataBuilder_.addAllMessages(other.additionalData_);
}
}
}
switch (other.getCombinationTechniqueCase()) {
case SUMMATION_COMBINATION_TECHNIQUE: {
mergeSummationCombinationTechnique(other.getSummationCombinationTechnique());
break;
}
case AVERAGING_COMBINATION_TECHNIQUE: {
mergeAveragingCombinationTechnique(other.getAveragingCombinationTechnique());
break;
}
case CUSTOM_COMBINATION_TECHNIQUE: {
mergeCustomCombinationTechnique(other.getCustomCombinationTechnique());
break;
}
case COMBINATIONTECHNIQUE_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.decision_trees.GenericTreeModel.Ensemble parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.decision_trees.GenericTreeModel.Ensemble) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int combinationTechniqueCase_ = 0;
private java.lang.Object combinationTechnique_;
public CombinationTechniqueCase
getCombinationTechniqueCase() {
return CombinationTechniqueCase.forNumber(
combinationTechniqueCase_);
}
public Builder clearCombinationTechnique() {
combinationTechniqueCase_ = 0;
combinationTechnique_ = null;
onChanged();
return this;
}
private int bitField0_;
private java.util.List members_ =
java.util.Collections.emptyList();
private void ensureMembersIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
members_ = new java.util.ArrayList(members_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Ensemble.Member, tensorflow.decision_trees.GenericTreeModel.Ensemble.Member.Builder, tensorflow.decision_trees.GenericTreeModel.Ensemble.MemberOrBuilder> membersBuilder_;
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
public java.util.List getMembersList() {
if (membersBuilder_ == null) {
return java.util.Collections.unmodifiableList(members_);
} else {
return membersBuilder_.getMessageList();
}
}
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
public int getMembersCount() {
if (membersBuilder_ == null) {
return members_.size();
} else {
return membersBuilder_.getCount();
}
}
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
public tensorflow.decision_trees.GenericTreeModel.Ensemble.Member getMembers(int index) {
if (membersBuilder_ == null) {
return members_.get(index);
} else {
return membersBuilder_.getMessage(index);
}
}
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
public Builder setMembers(
int index, tensorflow.decision_trees.GenericTreeModel.Ensemble.Member value) {
if (membersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMembersIsMutable();
members_.set(index, value);
onChanged();
} else {
membersBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
public Builder setMembers(
int index, tensorflow.decision_trees.GenericTreeModel.Ensemble.Member.Builder builderForValue) {
if (membersBuilder_ == null) {
ensureMembersIsMutable();
members_.set(index, builderForValue.build());
onChanged();
} else {
membersBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
public Builder addMembers(tensorflow.decision_trees.GenericTreeModel.Ensemble.Member value) {
if (membersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMembersIsMutable();
members_.add(value);
onChanged();
} else {
membersBuilder_.addMessage(value);
}
return this;
}
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
public Builder addMembers(
int index, tensorflow.decision_trees.GenericTreeModel.Ensemble.Member value) {
if (membersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMembersIsMutable();
members_.add(index, value);
onChanged();
} else {
membersBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
public Builder addMembers(
tensorflow.decision_trees.GenericTreeModel.Ensemble.Member.Builder builderForValue) {
if (membersBuilder_ == null) {
ensureMembersIsMutable();
members_.add(builderForValue.build());
onChanged();
} else {
membersBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
public Builder addMembers(
int index, tensorflow.decision_trees.GenericTreeModel.Ensemble.Member.Builder builderForValue) {
if (membersBuilder_ == null) {
ensureMembersIsMutable();
members_.add(index, builderForValue.build());
onChanged();
} else {
membersBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
public Builder addAllMembers(
java.lang.Iterable extends tensorflow.decision_trees.GenericTreeModel.Ensemble.Member> values) {
if (membersBuilder_ == null) {
ensureMembersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, members_);
onChanged();
} else {
membersBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
public Builder clearMembers() {
if (membersBuilder_ == null) {
members_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
membersBuilder_.clear();
}
return this;
}
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
public Builder removeMembers(int index) {
if (membersBuilder_ == null) {
ensureMembersIsMutable();
members_.remove(index);
onChanged();
} else {
membersBuilder_.remove(index);
}
return this;
}
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
public tensorflow.decision_trees.GenericTreeModel.Ensemble.Member.Builder getMembersBuilder(
int index) {
return getMembersFieldBuilder().getBuilder(index);
}
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
public tensorflow.decision_trees.GenericTreeModel.Ensemble.MemberOrBuilder getMembersOrBuilder(
int index) {
if (membersBuilder_ == null) {
return members_.get(index); } else {
return membersBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
public java.util.List extends tensorflow.decision_trees.GenericTreeModel.Ensemble.MemberOrBuilder>
getMembersOrBuilderList() {
if (membersBuilder_ != null) {
return membersBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(members_);
}
}
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
public tensorflow.decision_trees.GenericTreeModel.Ensemble.Member.Builder addMembersBuilder() {
return getMembersFieldBuilder().addBuilder(
tensorflow.decision_trees.GenericTreeModel.Ensemble.Member.getDefaultInstance());
}
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
public tensorflow.decision_trees.GenericTreeModel.Ensemble.Member.Builder addMembersBuilder(
int index) {
return getMembersFieldBuilder().addBuilder(
index, tensorflow.decision_trees.GenericTreeModel.Ensemble.Member.getDefaultInstance());
}
/**
*
* A higher id for more readable printing.
*
*
* repeated .tensorflow.decision_trees.Ensemble.Member members = 100;
*/
public java.util.List
getMembersBuilderList() {
return getMembersFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Ensemble.Member, tensorflow.decision_trees.GenericTreeModel.Ensemble.Member.Builder, tensorflow.decision_trees.GenericTreeModel.Ensemble.MemberOrBuilder>
getMembersFieldBuilder() {
if (membersBuilder_ == null) {
membersBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Ensemble.Member, tensorflow.decision_trees.GenericTreeModel.Ensemble.Member.Builder, tensorflow.decision_trees.GenericTreeModel.Ensemble.MemberOrBuilder>(
members_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
members_ = null;
}
return membersBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Summation, tensorflow.decision_trees.GenericTreeModel.Summation.Builder, tensorflow.decision_trees.GenericTreeModel.SummationOrBuilder> summationCombinationTechniqueBuilder_;
/**
* .tensorflow.decision_trees.Summation summation_combination_technique = 1;
*/
public boolean hasSummationCombinationTechnique() {
return combinationTechniqueCase_ == 1;
}
/**
* .tensorflow.decision_trees.Summation summation_combination_technique = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.Summation getSummationCombinationTechnique() {
if (summationCombinationTechniqueBuilder_ == null) {
if (combinationTechniqueCase_ == 1) {
return (tensorflow.decision_trees.GenericTreeModel.Summation) combinationTechnique_;
}
return tensorflow.decision_trees.GenericTreeModel.Summation.getDefaultInstance();
} else {
if (combinationTechniqueCase_ == 1) {
return summationCombinationTechniqueBuilder_.getMessage();
}
return tensorflow.decision_trees.GenericTreeModel.Summation.getDefaultInstance();
}
}
/**
* .tensorflow.decision_trees.Summation summation_combination_technique = 1;
*/
public Builder setSummationCombinationTechnique(tensorflow.decision_trees.GenericTreeModel.Summation value) {
if (summationCombinationTechniqueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
combinationTechnique_ = value;
onChanged();
} else {
summationCombinationTechniqueBuilder_.setMessage(value);
}
combinationTechniqueCase_ = 1;
return this;
}
/**
* .tensorflow.decision_trees.Summation summation_combination_technique = 1;
*/
public Builder setSummationCombinationTechnique(
tensorflow.decision_trees.GenericTreeModel.Summation.Builder builderForValue) {
if (summationCombinationTechniqueBuilder_ == null) {
combinationTechnique_ = builderForValue.build();
onChanged();
} else {
summationCombinationTechniqueBuilder_.setMessage(builderForValue.build());
}
combinationTechniqueCase_ = 1;
return this;
}
/**
* .tensorflow.decision_trees.Summation summation_combination_technique = 1;
*/
public Builder mergeSummationCombinationTechnique(tensorflow.decision_trees.GenericTreeModel.Summation value) {
if (summationCombinationTechniqueBuilder_ == null) {
if (combinationTechniqueCase_ == 1 &&
combinationTechnique_ != tensorflow.decision_trees.GenericTreeModel.Summation.getDefaultInstance()) {
combinationTechnique_ = tensorflow.decision_trees.GenericTreeModel.Summation.newBuilder((tensorflow.decision_trees.GenericTreeModel.Summation) combinationTechnique_)
.mergeFrom(value).buildPartial();
} else {
combinationTechnique_ = value;
}
onChanged();
} else {
if (combinationTechniqueCase_ == 1) {
summationCombinationTechniqueBuilder_.mergeFrom(value);
}
summationCombinationTechniqueBuilder_.setMessage(value);
}
combinationTechniqueCase_ = 1;
return this;
}
/**
* .tensorflow.decision_trees.Summation summation_combination_technique = 1;
*/
public Builder clearSummationCombinationTechnique() {
if (summationCombinationTechniqueBuilder_ == null) {
if (combinationTechniqueCase_ == 1) {
combinationTechniqueCase_ = 0;
combinationTechnique_ = null;
onChanged();
}
} else {
if (combinationTechniqueCase_ == 1) {
combinationTechniqueCase_ = 0;
combinationTechnique_ = null;
}
summationCombinationTechniqueBuilder_.clear();
}
return this;
}
/**
* .tensorflow.decision_trees.Summation summation_combination_technique = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.Summation.Builder getSummationCombinationTechniqueBuilder() {
return getSummationCombinationTechniqueFieldBuilder().getBuilder();
}
/**
* .tensorflow.decision_trees.Summation summation_combination_technique = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.SummationOrBuilder getSummationCombinationTechniqueOrBuilder() {
if ((combinationTechniqueCase_ == 1) && (summationCombinationTechniqueBuilder_ != null)) {
return summationCombinationTechniqueBuilder_.getMessageOrBuilder();
} else {
if (combinationTechniqueCase_ == 1) {
return (tensorflow.decision_trees.GenericTreeModel.Summation) combinationTechnique_;
}
return tensorflow.decision_trees.GenericTreeModel.Summation.getDefaultInstance();
}
}
/**
* .tensorflow.decision_trees.Summation summation_combination_technique = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Summation, tensorflow.decision_trees.GenericTreeModel.Summation.Builder, tensorflow.decision_trees.GenericTreeModel.SummationOrBuilder>
getSummationCombinationTechniqueFieldBuilder() {
if (summationCombinationTechniqueBuilder_ == null) {
if (!(combinationTechniqueCase_ == 1)) {
combinationTechnique_ = tensorflow.decision_trees.GenericTreeModel.Summation.getDefaultInstance();
}
summationCombinationTechniqueBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Summation, tensorflow.decision_trees.GenericTreeModel.Summation.Builder, tensorflow.decision_trees.GenericTreeModel.SummationOrBuilder>(
(tensorflow.decision_trees.GenericTreeModel.Summation) combinationTechnique_,
getParentForChildren(),
isClean());
combinationTechnique_ = null;
}
combinationTechniqueCase_ = 1;
onChanged();;
return summationCombinationTechniqueBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Averaging, tensorflow.decision_trees.GenericTreeModel.Averaging.Builder, tensorflow.decision_trees.GenericTreeModel.AveragingOrBuilder> averagingCombinationTechniqueBuilder_;
/**
* .tensorflow.decision_trees.Averaging averaging_combination_technique = 2;
*/
public boolean hasAveragingCombinationTechnique() {
return combinationTechniqueCase_ == 2;
}
/**
* .tensorflow.decision_trees.Averaging averaging_combination_technique = 2;
*/
public tensorflow.decision_trees.GenericTreeModel.Averaging getAveragingCombinationTechnique() {
if (averagingCombinationTechniqueBuilder_ == null) {
if (combinationTechniqueCase_ == 2) {
return (tensorflow.decision_trees.GenericTreeModel.Averaging) combinationTechnique_;
}
return tensorflow.decision_trees.GenericTreeModel.Averaging.getDefaultInstance();
} else {
if (combinationTechniqueCase_ == 2) {
return averagingCombinationTechniqueBuilder_.getMessage();
}
return tensorflow.decision_trees.GenericTreeModel.Averaging.getDefaultInstance();
}
}
/**
* .tensorflow.decision_trees.Averaging averaging_combination_technique = 2;
*/
public Builder setAveragingCombinationTechnique(tensorflow.decision_trees.GenericTreeModel.Averaging value) {
if (averagingCombinationTechniqueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
combinationTechnique_ = value;
onChanged();
} else {
averagingCombinationTechniqueBuilder_.setMessage(value);
}
combinationTechniqueCase_ = 2;
return this;
}
/**
* .tensorflow.decision_trees.Averaging averaging_combination_technique = 2;
*/
public Builder setAveragingCombinationTechnique(
tensorflow.decision_trees.GenericTreeModel.Averaging.Builder builderForValue) {
if (averagingCombinationTechniqueBuilder_ == null) {
combinationTechnique_ = builderForValue.build();
onChanged();
} else {
averagingCombinationTechniqueBuilder_.setMessage(builderForValue.build());
}
combinationTechniqueCase_ = 2;
return this;
}
/**
* .tensorflow.decision_trees.Averaging averaging_combination_technique = 2;
*/
public Builder mergeAveragingCombinationTechnique(tensorflow.decision_trees.GenericTreeModel.Averaging value) {
if (averagingCombinationTechniqueBuilder_ == null) {
if (combinationTechniqueCase_ == 2 &&
combinationTechnique_ != tensorflow.decision_trees.GenericTreeModel.Averaging.getDefaultInstance()) {
combinationTechnique_ = tensorflow.decision_trees.GenericTreeModel.Averaging.newBuilder((tensorflow.decision_trees.GenericTreeModel.Averaging) combinationTechnique_)
.mergeFrom(value).buildPartial();
} else {
combinationTechnique_ = value;
}
onChanged();
} else {
if (combinationTechniqueCase_ == 2) {
averagingCombinationTechniqueBuilder_.mergeFrom(value);
}
averagingCombinationTechniqueBuilder_.setMessage(value);
}
combinationTechniqueCase_ = 2;
return this;
}
/**
* .tensorflow.decision_trees.Averaging averaging_combination_technique = 2;
*/
public Builder clearAveragingCombinationTechnique() {
if (averagingCombinationTechniqueBuilder_ == null) {
if (combinationTechniqueCase_ == 2) {
combinationTechniqueCase_ = 0;
combinationTechnique_ = null;
onChanged();
}
} else {
if (combinationTechniqueCase_ == 2) {
combinationTechniqueCase_ = 0;
combinationTechnique_ = null;
}
averagingCombinationTechniqueBuilder_.clear();
}
return this;
}
/**
* .tensorflow.decision_trees.Averaging averaging_combination_technique = 2;
*/
public tensorflow.decision_trees.GenericTreeModel.Averaging.Builder getAveragingCombinationTechniqueBuilder() {
return getAveragingCombinationTechniqueFieldBuilder().getBuilder();
}
/**
* .tensorflow.decision_trees.Averaging averaging_combination_technique = 2;
*/
public tensorflow.decision_trees.GenericTreeModel.AveragingOrBuilder getAveragingCombinationTechniqueOrBuilder() {
if ((combinationTechniqueCase_ == 2) && (averagingCombinationTechniqueBuilder_ != null)) {
return averagingCombinationTechniqueBuilder_.getMessageOrBuilder();
} else {
if (combinationTechniqueCase_ == 2) {
return (tensorflow.decision_trees.GenericTreeModel.Averaging) combinationTechnique_;
}
return tensorflow.decision_trees.GenericTreeModel.Averaging.getDefaultInstance();
}
}
/**
* .tensorflow.decision_trees.Averaging averaging_combination_technique = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Averaging, tensorflow.decision_trees.GenericTreeModel.Averaging.Builder, tensorflow.decision_trees.GenericTreeModel.AveragingOrBuilder>
getAveragingCombinationTechniqueFieldBuilder() {
if (averagingCombinationTechniqueBuilder_ == null) {
if (!(combinationTechniqueCase_ == 2)) {
combinationTechnique_ = tensorflow.decision_trees.GenericTreeModel.Averaging.getDefaultInstance();
}
averagingCombinationTechniqueBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Averaging, tensorflow.decision_trees.GenericTreeModel.Averaging.Builder, tensorflow.decision_trees.GenericTreeModel.AveragingOrBuilder>(
(tensorflow.decision_trees.GenericTreeModel.Averaging) combinationTechnique_,
getParentForChildren(),
isClean());
combinationTechnique_ = null;
}
combinationTechniqueCase_ = 2;
onChanged();;
return averagingCombinationTechniqueBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> customCombinationTechniqueBuilder_;
/**
* .google.protobuf.Any custom_combination_technique = 3;
*/
public boolean hasCustomCombinationTechnique() {
return combinationTechniqueCase_ == 3;
}
/**
* .google.protobuf.Any custom_combination_technique = 3;
*/
public com.google.protobuf.Any getCustomCombinationTechnique() {
if (customCombinationTechniqueBuilder_ == null) {
if (combinationTechniqueCase_ == 3) {
return (com.google.protobuf.Any) combinationTechnique_;
}
return com.google.protobuf.Any.getDefaultInstance();
} else {
if (combinationTechniqueCase_ == 3) {
return customCombinationTechniqueBuilder_.getMessage();
}
return com.google.protobuf.Any.getDefaultInstance();
}
}
/**
* .google.protobuf.Any custom_combination_technique = 3;
*/
public Builder setCustomCombinationTechnique(com.google.protobuf.Any value) {
if (customCombinationTechniqueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
combinationTechnique_ = value;
onChanged();
} else {
customCombinationTechniqueBuilder_.setMessage(value);
}
combinationTechniqueCase_ = 3;
return this;
}
/**
* .google.protobuf.Any custom_combination_technique = 3;
*/
public Builder setCustomCombinationTechnique(
com.google.protobuf.Any.Builder builderForValue) {
if (customCombinationTechniqueBuilder_ == null) {
combinationTechnique_ = builderForValue.build();
onChanged();
} else {
customCombinationTechniqueBuilder_.setMessage(builderForValue.build());
}
combinationTechniqueCase_ = 3;
return this;
}
/**
* .google.protobuf.Any custom_combination_technique = 3;
*/
public Builder mergeCustomCombinationTechnique(com.google.protobuf.Any value) {
if (customCombinationTechniqueBuilder_ == null) {
if (combinationTechniqueCase_ == 3 &&
combinationTechnique_ != com.google.protobuf.Any.getDefaultInstance()) {
combinationTechnique_ = com.google.protobuf.Any.newBuilder((com.google.protobuf.Any) combinationTechnique_)
.mergeFrom(value).buildPartial();
} else {
combinationTechnique_ = value;
}
onChanged();
} else {
if (combinationTechniqueCase_ == 3) {
customCombinationTechniqueBuilder_.mergeFrom(value);
}
customCombinationTechniqueBuilder_.setMessage(value);
}
combinationTechniqueCase_ = 3;
return this;
}
/**
* .google.protobuf.Any custom_combination_technique = 3;
*/
public Builder clearCustomCombinationTechnique() {
if (customCombinationTechniqueBuilder_ == null) {
if (combinationTechniqueCase_ == 3) {
combinationTechniqueCase_ = 0;
combinationTechnique_ = null;
onChanged();
}
} else {
if (combinationTechniqueCase_ == 3) {
combinationTechniqueCase_ = 0;
combinationTechnique_ = null;
}
customCombinationTechniqueBuilder_.clear();
}
return this;
}
/**
* .google.protobuf.Any custom_combination_technique = 3;
*/
public com.google.protobuf.Any.Builder getCustomCombinationTechniqueBuilder() {
return getCustomCombinationTechniqueFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Any custom_combination_technique = 3;
*/
public com.google.protobuf.AnyOrBuilder getCustomCombinationTechniqueOrBuilder() {
if ((combinationTechniqueCase_ == 3) && (customCombinationTechniqueBuilder_ != null)) {
return customCombinationTechniqueBuilder_.getMessageOrBuilder();
} else {
if (combinationTechniqueCase_ == 3) {
return (com.google.protobuf.Any) combinationTechnique_;
}
return com.google.protobuf.Any.getDefaultInstance();
}
}
/**
* .google.protobuf.Any custom_combination_technique = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getCustomCombinationTechniqueFieldBuilder() {
if (customCombinationTechniqueBuilder_ == null) {
if (!(combinationTechniqueCase_ == 3)) {
combinationTechnique_ = com.google.protobuf.Any.getDefaultInstance();
}
customCombinationTechniqueBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
(com.google.protobuf.Any) combinationTechnique_,
getParentForChildren(),
isClean());
combinationTechnique_ = null;
}
combinationTechniqueCase_ = 3;
onChanged();;
return customCombinationTechniqueBuilder_;
}
private java.util.List additionalData_ =
java.util.Collections.emptyList();
private void ensureAdditionalDataIsMutable() {
if (!((bitField0_ & 0x00000010) == 0x00000010)) {
additionalData_ = new java.util.ArrayList(additionalData_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> additionalDataBuilder_;
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public java.util.List getAdditionalDataList() {
if (additionalDataBuilder_ == null) {
return java.util.Collections.unmodifiableList(additionalData_);
} else {
return additionalDataBuilder_.getMessageList();
}
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public int getAdditionalDataCount() {
if (additionalDataBuilder_ == null) {
return additionalData_.size();
} else {
return additionalDataBuilder_.getCount();
}
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public com.google.protobuf.Any getAdditionalData(int index) {
if (additionalDataBuilder_ == null) {
return additionalData_.get(index);
} else {
return additionalDataBuilder_.getMessage(index);
}
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public Builder setAdditionalData(
int index, com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.set(index, value);
onChanged();
} else {
additionalDataBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public Builder setAdditionalData(
int index, com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.set(index, builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public Builder addAdditionalData(com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.add(value);
onChanged();
} else {
additionalDataBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public Builder addAdditionalData(
int index, com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.add(index, value);
onChanged();
} else {
additionalDataBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public Builder addAdditionalData(
com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.add(builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public Builder addAdditionalData(
int index, com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.add(index, builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public Builder addAllAdditionalData(
java.lang.Iterable extends com.google.protobuf.Any> values) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, additionalData_);
onChanged();
} else {
additionalDataBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public Builder clearAdditionalData() {
if (additionalDataBuilder_ == null) {
additionalData_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
additionalDataBuilder_.clear();
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public Builder removeAdditionalData(int index) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.remove(index);
onChanged();
} else {
additionalDataBuilder_.remove(index);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public com.google.protobuf.Any.Builder getAdditionalDataBuilder(
int index) {
return getAdditionalDataFieldBuilder().getBuilder(index);
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index) {
if (additionalDataBuilder_ == null) {
return additionalData_.get(index); } else {
return additionalDataBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList() {
if (additionalDataBuilder_ != null) {
return additionalDataBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(additionalData_);
}
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public com.google.protobuf.Any.Builder addAdditionalDataBuilder() {
return getAdditionalDataFieldBuilder().addBuilder(
com.google.protobuf.Any.getDefaultInstance());
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public com.google.protobuf.Any.Builder addAdditionalDataBuilder(
int index) {
return getAdditionalDataFieldBuilder().addBuilder(
index, com.google.protobuf.Any.getDefaultInstance());
}
/**
* repeated .google.protobuf.Any additional_data = 4;
*/
public java.util.List
getAdditionalDataBuilderList() {
return getAdditionalDataFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getAdditionalDataFieldBuilder() {
if (additionalDataBuilder_ == null) {
additionalDataBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
additionalData_,
((bitField0_ & 0x00000010) == 0x00000010),
getParentForChildren(),
isClean());
additionalData_ = null;
}
return additionalDataBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.decision_trees.Ensemble)
}
// @@protoc_insertion_point(class_scope:tensorflow.decision_trees.Ensemble)
private static final tensorflow.decision_trees.GenericTreeModel.Ensemble DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.decision_trees.GenericTreeModel.Ensemble();
}
public static tensorflow.decision_trees.GenericTreeModel.Ensemble getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Ensemble parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Ensemble(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Ensemble getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SummationOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.decision_trees.Summation)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
java.util.List
getAdditionalDataList();
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
com.google.protobuf.Any getAdditionalData(int index);
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
int getAdditionalDataCount();
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList();
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index);
}
/**
*
* When present, the Ensemble's output is the sum of member models' outputs.
*
*
* Protobuf type {@code tensorflow.decision_trees.Summation}
*/
public static final class Summation extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.decision_trees.Summation)
SummationOrBuilder {
private static final long serialVersionUID = 0L;
// Use Summation.newBuilder() to construct.
private Summation(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Summation() {
additionalData_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Summation(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
additionalData_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
additionalData_.add(
input.readMessage(com.google.protobuf.Any.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
additionalData_ = java.util.Collections.unmodifiableList(additionalData_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Summation_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Summation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.Summation.class, tensorflow.decision_trees.GenericTreeModel.Summation.Builder.class);
}
public static final int ADDITIONAL_DATA_FIELD_NUMBER = 1;
private java.util.List additionalData_;
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public java.util.List getAdditionalDataList() {
return additionalData_;
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList() {
return additionalData_;
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public int getAdditionalDataCount() {
return additionalData_.size();
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public com.google.protobuf.Any getAdditionalData(int index) {
return additionalData_.get(index);
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index) {
return additionalData_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < additionalData_.size(); i++) {
output.writeMessage(1, additionalData_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < additionalData_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, additionalData_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.decision_trees.GenericTreeModel.Summation)) {
return super.equals(obj);
}
tensorflow.decision_trees.GenericTreeModel.Summation other = (tensorflow.decision_trees.GenericTreeModel.Summation) obj;
boolean result = true;
result = result && getAdditionalDataList()
.equals(other.getAdditionalDataList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getAdditionalDataCount() > 0) {
hash = (37 * hash) + ADDITIONAL_DATA_FIELD_NUMBER;
hash = (53 * hash) + getAdditionalDataList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.decision_trees.GenericTreeModel.Summation parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.Summation parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Summation parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.Summation parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Summation parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.Summation parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Summation parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.Summation parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Summation parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.Summation parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Summation parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.Summation parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.decision_trees.GenericTreeModel.Summation prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* When present, the Ensemble's output is the sum of member models' outputs.
*
*
* Protobuf type {@code tensorflow.decision_trees.Summation}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.decision_trees.Summation)
tensorflow.decision_trees.GenericTreeModel.SummationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Summation_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Summation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.Summation.class, tensorflow.decision_trees.GenericTreeModel.Summation.Builder.class);
}
// Construct using tensorflow.decision_trees.GenericTreeModel.Summation.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getAdditionalDataFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (additionalDataBuilder_ == null) {
additionalData_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
additionalDataBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Summation_descriptor;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Summation getDefaultInstanceForType() {
return tensorflow.decision_trees.GenericTreeModel.Summation.getDefaultInstance();
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Summation build() {
tensorflow.decision_trees.GenericTreeModel.Summation result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Summation buildPartial() {
tensorflow.decision_trees.GenericTreeModel.Summation result = new tensorflow.decision_trees.GenericTreeModel.Summation(this);
int from_bitField0_ = bitField0_;
if (additionalDataBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
additionalData_ = java.util.Collections.unmodifiableList(additionalData_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.additionalData_ = additionalData_;
} else {
result.additionalData_ = additionalDataBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.decision_trees.GenericTreeModel.Summation) {
return mergeFrom((tensorflow.decision_trees.GenericTreeModel.Summation)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.decision_trees.GenericTreeModel.Summation other) {
if (other == tensorflow.decision_trees.GenericTreeModel.Summation.getDefaultInstance()) return this;
if (additionalDataBuilder_ == null) {
if (!other.additionalData_.isEmpty()) {
if (additionalData_.isEmpty()) {
additionalData_ = other.additionalData_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureAdditionalDataIsMutable();
additionalData_.addAll(other.additionalData_);
}
onChanged();
}
} else {
if (!other.additionalData_.isEmpty()) {
if (additionalDataBuilder_.isEmpty()) {
additionalDataBuilder_.dispose();
additionalDataBuilder_ = null;
additionalData_ = other.additionalData_;
bitField0_ = (bitField0_ & ~0x00000001);
additionalDataBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAdditionalDataFieldBuilder() : null;
} else {
additionalDataBuilder_.addAllMessages(other.additionalData_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.decision_trees.GenericTreeModel.Summation parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.decision_trees.GenericTreeModel.Summation) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List additionalData_ =
java.util.Collections.emptyList();
private void ensureAdditionalDataIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
additionalData_ = new java.util.ArrayList(additionalData_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> additionalDataBuilder_;
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public java.util.List getAdditionalDataList() {
if (additionalDataBuilder_ == null) {
return java.util.Collections.unmodifiableList(additionalData_);
} else {
return additionalDataBuilder_.getMessageList();
}
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public int getAdditionalDataCount() {
if (additionalDataBuilder_ == null) {
return additionalData_.size();
} else {
return additionalDataBuilder_.getCount();
}
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public com.google.protobuf.Any getAdditionalData(int index) {
if (additionalDataBuilder_ == null) {
return additionalData_.get(index);
} else {
return additionalDataBuilder_.getMessage(index);
}
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public Builder setAdditionalData(
int index, com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.set(index, value);
onChanged();
} else {
additionalDataBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public Builder setAdditionalData(
int index, com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.set(index, builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public Builder addAdditionalData(com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.add(value);
onChanged();
} else {
additionalDataBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public Builder addAdditionalData(
int index, com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.add(index, value);
onChanged();
} else {
additionalDataBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public Builder addAdditionalData(
com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.add(builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public Builder addAdditionalData(
int index, com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.add(index, builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public Builder addAllAdditionalData(
java.lang.Iterable extends com.google.protobuf.Any> values) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, additionalData_);
onChanged();
} else {
additionalDataBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public Builder clearAdditionalData() {
if (additionalDataBuilder_ == null) {
additionalData_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
additionalDataBuilder_.clear();
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public Builder removeAdditionalData(int index) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.remove(index);
onChanged();
} else {
additionalDataBuilder_.remove(index);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public com.google.protobuf.Any.Builder getAdditionalDataBuilder(
int index) {
return getAdditionalDataFieldBuilder().getBuilder(index);
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index) {
if (additionalDataBuilder_ == null) {
return additionalData_.get(index); } else {
return additionalDataBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList() {
if (additionalDataBuilder_ != null) {
return additionalDataBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(additionalData_);
}
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public com.google.protobuf.Any.Builder addAdditionalDataBuilder() {
return getAdditionalDataFieldBuilder().addBuilder(
com.google.protobuf.Any.getDefaultInstance());
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public com.google.protobuf.Any.Builder addAdditionalDataBuilder(
int index) {
return getAdditionalDataFieldBuilder().addBuilder(
index, com.google.protobuf.Any.getDefaultInstance());
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public java.util.List
getAdditionalDataBuilderList() {
return getAdditionalDataFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getAdditionalDataFieldBuilder() {
if (additionalDataBuilder_ == null) {
additionalDataBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
additionalData_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
additionalData_ = null;
}
return additionalDataBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.decision_trees.Summation)
}
// @@protoc_insertion_point(class_scope:tensorflow.decision_trees.Summation)
private static final tensorflow.decision_trees.GenericTreeModel.Summation DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.decision_trees.GenericTreeModel.Summation();
}
public static tensorflow.decision_trees.GenericTreeModel.Summation getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Summation parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Summation(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Summation getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AveragingOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.decision_trees.Averaging)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
java.util.List
getAdditionalDataList();
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
com.google.protobuf.Any getAdditionalData(int index);
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
int getAdditionalDataCount();
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList();
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index);
}
/**
*
* When present, the Ensemble's output is the average of member models' outputs.
*
*
* Protobuf type {@code tensorflow.decision_trees.Averaging}
*/
public static final class Averaging extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.decision_trees.Averaging)
AveragingOrBuilder {
private static final long serialVersionUID = 0L;
// Use Averaging.newBuilder() to construct.
private Averaging(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Averaging() {
additionalData_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Averaging(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
additionalData_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
additionalData_.add(
input.readMessage(com.google.protobuf.Any.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
additionalData_ = java.util.Collections.unmodifiableList(additionalData_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Averaging_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Averaging_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.Averaging.class, tensorflow.decision_trees.GenericTreeModel.Averaging.Builder.class);
}
public static final int ADDITIONAL_DATA_FIELD_NUMBER = 1;
private java.util.List additionalData_;
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public java.util.List getAdditionalDataList() {
return additionalData_;
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList() {
return additionalData_;
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public int getAdditionalDataCount() {
return additionalData_.size();
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public com.google.protobuf.Any getAdditionalData(int index) {
return additionalData_.get(index);
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index) {
return additionalData_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < additionalData_.size(); i++) {
output.writeMessage(1, additionalData_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < additionalData_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, additionalData_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.decision_trees.GenericTreeModel.Averaging)) {
return super.equals(obj);
}
tensorflow.decision_trees.GenericTreeModel.Averaging other = (tensorflow.decision_trees.GenericTreeModel.Averaging) obj;
boolean result = true;
result = result && getAdditionalDataList()
.equals(other.getAdditionalDataList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getAdditionalDataCount() > 0) {
hash = (37 * hash) + ADDITIONAL_DATA_FIELD_NUMBER;
hash = (53 * hash) + getAdditionalDataList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.decision_trees.GenericTreeModel.Averaging parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.Averaging parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Averaging parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.Averaging parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Averaging parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.Averaging parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Averaging parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.Averaging parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Averaging parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.Averaging parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Averaging parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.Averaging parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.decision_trees.GenericTreeModel.Averaging prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* When present, the Ensemble's output is the average of member models' outputs.
*
*
* Protobuf type {@code tensorflow.decision_trees.Averaging}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.decision_trees.Averaging)
tensorflow.decision_trees.GenericTreeModel.AveragingOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Averaging_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Averaging_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.Averaging.class, tensorflow.decision_trees.GenericTreeModel.Averaging.Builder.class);
}
// Construct using tensorflow.decision_trees.GenericTreeModel.Averaging.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getAdditionalDataFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (additionalDataBuilder_ == null) {
additionalData_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
additionalDataBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Averaging_descriptor;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Averaging getDefaultInstanceForType() {
return tensorflow.decision_trees.GenericTreeModel.Averaging.getDefaultInstance();
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Averaging build() {
tensorflow.decision_trees.GenericTreeModel.Averaging result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Averaging buildPartial() {
tensorflow.decision_trees.GenericTreeModel.Averaging result = new tensorflow.decision_trees.GenericTreeModel.Averaging(this);
int from_bitField0_ = bitField0_;
if (additionalDataBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
additionalData_ = java.util.Collections.unmodifiableList(additionalData_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.additionalData_ = additionalData_;
} else {
result.additionalData_ = additionalDataBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.decision_trees.GenericTreeModel.Averaging) {
return mergeFrom((tensorflow.decision_trees.GenericTreeModel.Averaging)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.decision_trees.GenericTreeModel.Averaging other) {
if (other == tensorflow.decision_trees.GenericTreeModel.Averaging.getDefaultInstance()) return this;
if (additionalDataBuilder_ == null) {
if (!other.additionalData_.isEmpty()) {
if (additionalData_.isEmpty()) {
additionalData_ = other.additionalData_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureAdditionalDataIsMutable();
additionalData_.addAll(other.additionalData_);
}
onChanged();
}
} else {
if (!other.additionalData_.isEmpty()) {
if (additionalDataBuilder_.isEmpty()) {
additionalDataBuilder_.dispose();
additionalDataBuilder_ = null;
additionalData_ = other.additionalData_;
bitField0_ = (bitField0_ & ~0x00000001);
additionalDataBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAdditionalDataFieldBuilder() : null;
} else {
additionalDataBuilder_.addAllMessages(other.additionalData_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.decision_trees.GenericTreeModel.Averaging parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.decision_trees.GenericTreeModel.Averaging) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List additionalData_ =
java.util.Collections.emptyList();
private void ensureAdditionalDataIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
additionalData_ = new java.util.ArrayList(additionalData_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> additionalDataBuilder_;
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public java.util.List getAdditionalDataList() {
if (additionalDataBuilder_ == null) {
return java.util.Collections.unmodifiableList(additionalData_);
} else {
return additionalDataBuilder_.getMessageList();
}
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public int getAdditionalDataCount() {
if (additionalDataBuilder_ == null) {
return additionalData_.size();
} else {
return additionalDataBuilder_.getCount();
}
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public com.google.protobuf.Any getAdditionalData(int index) {
if (additionalDataBuilder_ == null) {
return additionalData_.get(index);
} else {
return additionalDataBuilder_.getMessage(index);
}
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public Builder setAdditionalData(
int index, com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.set(index, value);
onChanged();
} else {
additionalDataBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public Builder setAdditionalData(
int index, com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.set(index, builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public Builder addAdditionalData(com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.add(value);
onChanged();
} else {
additionalDataBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public Builder addAdditionalData(
int index, com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.add(index, value);
onChanged();
} else {
additionalDataBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public Builder addAdditionalData(
com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.add(builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public Builder addAdditionalData(
int index, com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.add(index, builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public Builder addAllAdditionalData(
java.lang.Iterable extends com.google.protobuf.Any> values) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, additionalData_);
onChanged();
} else {
additionalDataBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public Builder clearAdditionalData() {
if (additionalDataBuilder_ == null) {
additionalData_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
additionalDataBuilder_.clear();
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public Builder removeAdditionalData(int index) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.remove(index);
onChanged();
} else {
additionalDataBuilder_.remove(index);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public com.google.protobuf.Any.Builder getAdditionalDataBuilder(
int index) {
return getAdditionalDataFieldBuilder().getBuilder(index);
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index) {
if (additionalDataBuilder_ == null) {
return additionalData_.get(index); } else {
return additionalDataBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList() {
if (additionalDataBuilder_ != null) {
return additionalDataBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(additionalData_);
}
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public com.google.protobuf.Any.Builder addAdditionalDataBuilder() {
return getAdditionalDataFieldBuilder().addBuilder(
com.google.protobuf.Any.getDefaultInstance());
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public com.google.protobuf.Any.Builder addAdditionalDataBuilder(
int index) {
return getAdditionalDataFieldBuilder().addBuilder(
index, com.google.protobuf.Any.getDefaultInstance());
}
/**
* repeated .google.protobuf.Any additional_data = 1;
*/
public java.util.List
getAdditionalDataBuilderList() {
return getAdditionalDataFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getAdditionalDataFieldBuilder() {
if (additionalDataBuilder_ == null) {
additionalDataBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
additionalData_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
additionalData_ = null;
}
return additionalDataBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.decision_trees.Averaging)
}
// @@protoc_insertion_point(class_scope:tensorflow.decision_trees.Averaging)
private static final tensorflow.decision_trees.GenericTreeModel.Averaging DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.decision_trees.GenericTreeModel.Averaging();
}
public static tensorflow.decision_trees.GenericTreeModel.Averaging getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Averaging parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Averaging(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Averaging getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DecisionTreeOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.decision_trees.DecisionTree)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
java.util.List
getNodesList();
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
tensorflow.decision_trees.GenericTreeModel.TreeNode getNodes(int index);
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
int getNodesCount();
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
java.util.List extends tensorflow.decision_trees.GenericTreeModel.TreeNodeOrBuilder>
getNodesOrBuilderList();
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
tensorflow.decision_trees.GenericTreeModel.TreeNodeOrBuilder getNodesOrBuilder(
int index);
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
java.util.List
getAdditionalDataList();
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
com.google.protobuf.Any getAdditionalData(int index);
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
int getAdditionalDataCount();
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList();
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index);
}
/**
* Protobuf type {@code tensorflow.decision_trees.DecisionTree}
*/
public static final class DecisionTree extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.decision_trees.DecisionTree)
DecisionTreeOrBuilder {
private static final long serialVersionUID = 0L;
// Use DecisionTree.newBuilder() to construct.
private DecisionTree(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DecisionTree() {
nodes_ = java.util.Collections.emptyList();
additionalData_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DecisionTree(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
nodes_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
nodes_.add(
input.readMessage(tensorflow.decision_trees.GenericTreeModel.TreeNode.parser(), extensionRegistry));
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
additionalData_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
additionalData_.add(
input.readMessage(com.google.protobuf.Any.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
nodes_ = java.util.Collections.unmodifiableList(nodes_);
}
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
additionalData_ = java.util.Collections.unmodifiableList(additionalData_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_DecisionTree_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_DecisionTree_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.DecisionTree.class, tensorflow.decision_trees.GenericTreeModel.DecisionTree.Builder.class);
}
public static final int NODES_FIELD_NUMBER = 1;
private java.util.List nodes_;
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
public java.util.List getNodesList() {
return nodes_;
}
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
public java.util.List extends tensorflow.decision_trees.GenericTreeModel.TreeNodeOrBuilder>
getNodesOrBuilderList() {
return nodes_;
}
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
public int getNodesCount() {
return nodes_.size();
}
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.TreeNode getNodes(int index) {
return nodes_.get(index);
}
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.TreeNodeOrBuilder getNodesOrBuilder(
int index) {
return nodes_.get(index);
}
public static final int ADDITIONAL_DATA_FIELD_NUMBER = 2;
private java.util.List additionalData_;
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public java.util.List getAdditionalDataList() {
return additionalData_;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList() {
return additionalData_;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public int getAdditionalDataCount() {
return additionalData_.size();
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public com.google.protobuf.Any getAdditionalData(int index) {
return additionalData_.get(index);
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index) {
return additionalData_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < nodes_.size(); i++) {
output.writeMessage(1, nodes_.get(i));
}
for (int i = 0; i < additionalData_.size(); i++) {
output.writeMessage(2, additionalData_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < nodes_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, nodes_.get(i));
}
for (int i = 0; i < additionalData_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, additionalData_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.decision_trees.GenericTreeModel.DecisionTree)) {
return super.equals(obj);
}
tensorflow.decision_trees.GenericTreeModel.DecisionTree other = (tensorflow.decision_trees.GenericTreeModel.DecisionTree) obj;
boolean result = true;
result = result && getNodesList()
.equals(other.getNodesList());
result = result && getAdditionalDataList()
.equals(other.getAdditionalDataList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getNodesCount() > 0) {
hash = (37 * hash) + NODES_FIELD_NUMBER;
hash = (53 * hash) + getNodesList().hashCode();
}
if (getAdditionalDataCount() > 0) {
hash = (37 * hash) + ADDITIONAL_DATA_FIELD_NUMBER;
hash = (53 * hash) + getAdditionalDataList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.decision_trees.GenericTreeModel.DecisionTree parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.DecisionTree parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.DecisionTree parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.DecisionTree parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.DecisionTree parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.DecisionTree parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.DecisionTree parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.DecisionTree parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.DecisionTree parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.DecisionTree parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.DecisionTree parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.DecisionTree parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.decision_trees.GenericTreeModel.DecisionTree prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.decision_trees.DecisionTree}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.decision_trees.DecisionTree)
tensorflow.decision_trees.GenericTreeModel.DecisionTreeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_DecisionTree_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_DecisionTree_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.DecisionTree.class, tensorflow.decision_trees.GenericTreeModel.DecisionTree.Builder.class);
}
// Construct using tensorflow.decision_trees.GenericTreeModel.DecisionTree.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getNodesFieldBuilder();
getAdditionalDataFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (nodesBuilder_ == null) {
nodes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
nodesBuilder_.clear();
}
if (additionalDataBuilder_ == null) {
additionalData_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
additionalDataBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_DecisionTree_descriptor;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.DecisionTree getDefaultInstanceForType() {
return tensorflow.decision_trees.GenericTreeModel.DecisionTree.getDefaultInstance();
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.DecisionTree build() {
tensorflow.decision_trees.GenericTreeModel.DecisionTree result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.DecisionTree buildPartial() {
tensorflow.decision_trees.GenericTreeModel.DecisionTree result = new tensorflow.decision_trees.GenericTreeModel.DecisionTree(this);
int from_bitField0_ = bitField0_;
if (nodesBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
nodes_ = java.util.Collections.unmodifiableList(nodes_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.nodes_ = nodes_;
} else {
result.nodes_ = nodesBuilder_.build();
}
if (additionalDataBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
additionalData_ = java.util.Collections.unmodifiableList(additionalData_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.additionalData_ = additionalData_;
} else {
result.additionalData_ = additionalDataBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.decision_trees.GenericTreeModel.DecisionTree) {
return mergeFrom((tensorflow.decision_trees.GenericTreeModel.DecisionTree)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.decision_trees.GenericTreeModel.DecisionTree other) {
if (other == tensorflow.decision_trees.GenericTreeModel.DecisionTree.getDefaultInstance()) return this;
if (nodesBuilder_ == null) {
if (!other.nodes_.isEmpty()) {
if (nodes_.isEmpty()) {
nodes_ = other.nodes_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureNodesIsMutable();
nodes_.addAll(other.nodes_);
}
onChanged();
}
} else {
if (!other.nodes_.isEmpty()) {
if (nodesBuilder_.isEmpty()) {
nodesBuilder_.dispose();
nodesBuilder_ = null;
nodes_ = other.nodes_;
bitField0_ = (bitField0_ & ~0x00000001);
nodesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getNodesFieldBuilder() : null;
} else {
nodesBuilder_.addAllMessages(other.nodes_);
}
}
}
if (additionalDataBuilder_ == null) {
if (!other.additionalData_.isEmpty()) {
if (additionalData_.isEmpty()) {
additionalData_ = other.additionalData_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureAdditionalDataIsMutable();
additionalData_.addAll(other.additionalData_);
}
onChanged();
}
} else {
if (!other.additionalData_.isEmpty()) {
if (additionalDataBuilder_.isEmpty()) {
additionalDataBuilder_.dispose();
additionalDataBuilder_ = null;
additionalData_ = other.additionalData_;
bitField0_ = (bitField0_ & ~0x00000002);
additionalDataBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAdditionalDataFieldBuilder() : null;
} else {
additionalDataBuilder_.addAllMessages(other.additionalData_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.decision_trees.GenericTreeModel.DecisionTree parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.decision_trees.GenericTreeModel.DecisionTree) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List nodes_ =
java.util.Collections.emptyList();
private void ensureNodesIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
nodes_ = new java.util.ArrayList(nodes_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.TreeNode, tensorflow.decision_trees.GenericTreeModel.TreeNode.Builder, tensorflow.decision_trees.GenericTreeModel.TreeNodeOrBuilder> nodesBuilder_;
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
public java.util.List getNodesList() {
if (nodesBuilder_ == null) {
return java.util.Collections.unmodifiableList(nodes_);
} else {
return nodesBuilder_.getMessageList();
}
}
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
public int getNodesCount() {
if (nodesBuilder_ == null) {
return nodes_.size();
} else {
return nodesBuilder_.getCount();
}
}
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.TreeNode getNodes(int index) {
if (nodesBuilder_ == null) {
return nodes_.get(index);
} else {
return nodesBuilder_.getMessage(index);
}
}
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
public Builder setNodes(
int index, tensorflow.decision_trees.GenericTreeModel.TreeNode value) {
if (nodesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNodesIsMutable();
nodes_.set(index, value);
onChanged();
} else {
nodesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
public Builder setNodes(
int index, tensorflow.decision_trees.GenericTreeModel.TreeNode.Builder builderForValue) {
if (nodesBuilder_ == null) {
ensureNodesIsMutable();
nodes_.set(index, builderForValue.build());
onChanged();
} else {
nodesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
public Builder addNodes(tensorflow.decision_trees.GenericTreeModel.TreeNode value) {
if (nodesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNodesIsMutable();
nodes_.add(value);
onChanged();
} else {
nodesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
public Builder addNodes(
int index, tensorflow.decision_trees.GenericTreeModel.TreeNode value) {
if (nodesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNodesIsMutable();
nodes_.add(index, value);
onChanged();
} else {
nodesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
public Builder addNodes(
tensorflow.decision_trees.GenericTreeModel.TreeNode.Builder builderForValue) {
if (nodesBuilder_ == null) {
ensureNodesIsMutable();
nodes_.add(builderForValue.build());
onChanged();
} else {
nodesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
public Builder addNodes(
int index, tensorflow.decision_trees.GenericTreeModel.TreeNode.Builder builderForValue) {
if (nodesBuilder_ == null) {
ensureNodesIsMutable();
nodes_.add(index, builderForValue.build());
onChanged();
} else {
nodesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
public Builder addAllNodes(
java.lang.Iterable extends tensorflow.decision_trees.GenericTreeModel.TreeNode> values) {
if (nodesBuilder_ == null) {
ensureNodesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, nodes_);
onChanged();
} else {
nodesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
public Builder clearNodes() {
if (nodesBuilder_ == null) {
nodes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
nodesBuilder_.clear();
}
return this;
}
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
public Builder removeNodes(int index) {
if (nodesBuilder_ == null) {
ensureNodesIsMutable();
nodes_.remove(index);
onChanged();
} else {
nodesBuilder_.remove(index);
}
return this;
}
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.TreeNode.Builder getNodesBuilder(
int index) {
return getNodesFieldBuilder().getBuilder(index);
}
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.TreeNodeOrBuilder getNodesOrBuilder(
int index) {
if (nodesBuilder_ == null) {
return nodes_.get(index); } else {
return nodesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
public java.util.List extends tensorflow.decision_trees.GenericTreeModel.TreeNodeOrBuilder>
getNodesOrBuilderList() {
if (nodesBuilder_ != null) {
return nodesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(nodes_);
}
}
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.TreeNode.Builder addNodesBuilder() {
return getNodesFieldBuilder().addBuilder(
tensorflow.decision_trees.GenericTreeModel.TreeNode.getDefaultInstance());
}
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.TreeNode.Builder addNodesBuilder(
int index) {
return getNodesFieldBuilder().addBuilder(
index, tensorflow.decision_trees.GenericTreeModel.TreeNode.getDefaultInstance());
}
/**
* repeated .tensorflow.decision_trees.TreeNode nodes = 1;
*/
public java.util.List
getNodesBuilderList() {
return getNodesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.TreeNode, tensorflow.decision_trees.GenericTreeModel.TreeNode.Builder, tensorflow.decision_trees.GenericTreeModel.TreeNodeOrBuilder>
getNodesFieldBuilder() {
if (nodesBuilder_ == null) {
nodesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.TreeNode, tensorflow.decision_trees.GenericTreeModel.TreeNode.Builder, tensorflow.decision_trees.GenericTreeModel.TreeNodeOrBuilder>(
nodes_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
nodes_ = null;
}
return nodesBuilder_;
}
private java.util.List additionalData_ =
java.util.Collections.emptyList();
private void ensureAdditionalDataIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
additionalData_ = new java.util.ArrayList(additionalData_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> additionalDataBuilder_;
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public java.util.List getAdditionalDataList() {
if (additionalDataBuilder_ == null) {
return java.util.Collections.unmodifiableList(additionalData_);
} else {
return additionalDataBuilder_.getMessageList();
}
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public int getAdditionalDataCount() {
if (additionalDataBuilder_ == null) {
return additionalData_.size();
} else {
return additionalDataBuilder_.getCount();
}
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public com.google.protobuf.Any getAdditionalData(int index) {
if (additionalDataBuilder_ == null) {
return additionalData_.get(index);
} else {
return additionalDataBuilder_.getMessage(index);
}
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder setAdditionalData(
int index, com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.set(index, value);
onChanged();
} else {
additionalDataBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder setAdditionalData(
int index, com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.set(index, builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder addAdditionalData(com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.add(value);
onChanged();
} else {
additionalDataBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder addAdditionalData(
int index, com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.add(index, value);
onChanged();
} else {
additionalDataBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder addAdditionalData(
com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.add(builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder addAdditionalData(
int index, com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.add(index, builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder addAllAdditionalData(
java.lang.Iterable extends com.google.protobuf.Any> values) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, additionalData_);
onChanged();
} else {
additionalDataBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder clearAdditionalData() {
if (additionalDataBuilder_ == null) {
additionalData_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
additionalDataBuilder_.clear();
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder removeAdditionalData(int index) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.remove(index);
onChanged();
} else {
additionalDataBuilder_.remove(index);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public com.google.protobuf.Any.Builder getAdditionalDataBuilder(
int index) {
return getAdditionalDataFieldBuilder().getBuilder(index);
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index) {
if (additionalDataBuilder_ == null) {
return additionalData_.get(index); } else {
return additionalDataBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList() {
if (additionalDataBuilder_ != null) {
return additionalDataBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(additionalData_);
}
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public com.google.protobuf.Any.Builder addAdditionalDataBuilder() {
return getAdditionalDataFieldBuilder().addBuilder(
com.google.protobuf.Any.getDefaultInstance());
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public com.google.protobuf.Any.Builder addAdditionalDataBuilder(
int index) {
return getAdditionalDataFieldBuilder().addBuilder(
index, com.google.protobuf.Any.getDefaultInstance());
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public java.util.List
getAdditionalDataBuilderList() {
return getAdditionalDataFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getAdditionalDataFieldBuilder() {
if (additionalDataBuilder_ == null) {
additionalDataBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
additionalData_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
additionalData_ = null;
}
return additionalDataBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.decision_trees.DecisionTree)
}
// @@protoc_insertion_point(class_scope:tensorflow.decision_trees.DecisionTree)
private static final tensorflow.decision_trees.GenericTreeModel.DecisionTree DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.decision_trees.GenericTreeModel.DecisionTree();
}
public static tensorflow.decision_trees.GenericTreeModel.DecisionTree getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DecisionTree parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DecisionTree(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.DecisionTree getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TreeNodeOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.decision_trees.TreeNode)
com.google.protobuf.MessageOrBuilder {
/**
*
* Following fields are provided for convenience and better readability.
* Filling them in is not required.
*
*
* .google.protobuf.Int32Value node_id = 1;
*/
boolean hasNodeId();
/**
*
* Following fields are provided for convenience and better readability.
* Filling them in is not required.
*
*
* .google.protobuf.Int32Value node_id = 1;
*/
com.google.protobuf.Int32Value getNodeId();
/**
*
* Following fields are provided for convenience and better readability.
* Filling them in is not required.
*
*
* .google.protobuf.Int32Value node_id = 1;
*/
com.google.protobuf.Int32ValueOrBuilder getNodeIdOrBuilder();
/**
* .google.protobuf.Int32Value depth = 2;
*/
boolean hasDepth();
/**
* .google.protobuf.Int32Value depth = 2;
*/
com.google.protobuf.Int32Value getDepth();
/**
* .google.protobuf.Int32Value depth = 2;
*/
com.google.protobuf.Int32ValueOrBuilder getDepthOrBuilder();
/**
* .google.protobuf.Int32Value subtree_size = 3;
*/
boolean hasSubtreeSize();
/**
* .google.protobuf.Int32Value subtree_size = 3;
*/
com.google.protobuf.Int32Value getSubtreeSize();
/**
* .google.protobuf.Int32Value subtree_size = 3;
*/
com.google.protobuf.Int32ValueOrBuilder getSubtreeSizeOrBuilder();
/**
* .tensorflow.decision_trees.BinaryNode binary_node = 4;
*/
boolean hasBinaryNode();
/**
* .tensorflow.decision_trees.BinaryNode binary_node = 4;
*/
tensorflow.decision_trees.GenericTreeModel.BinaryNode getBinaryNode();
/**
* .tensorflow.decision_trees.BinaryNode binary_node = 4;
*/
tensorflow.decision_trees.GenericTreeModel.BinaryNodeOrBuilder getBinaryNodeOrBuilder();
/**
* .tensorflow.decision_trees.Leaf leaf = 5;
*/
boolean hasLeaf();
/**
* .tensorflow.decision_trees.Leaf leaf = 5;
*/
tensorflow.decision_trees.GenericTreeModel.Leaf getLeaf();
/**
* .tensorflow.decision_trees.Leaf leaf = 5;
*/
tensorflow.decision_trees.GenericTreeModel.LeafOrBuilder getLeafOrBuilder();
/**
* .google.protobuf.Any custom_node_type = 6;
*/
boolean hasCustomNodeType();
/**
* .google.protobuf.Any custom_node_type = 6;
*/
com.google.protobuf.Any getCustomNodeType();
/**
* .google.protobuf.Any custom_node_type = 6;
*/
com.google.protobuf.AnyOrBuilder getCustomNodeTypeOrBuilder();
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
java.util.List
getAdditionalDataList();
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
com.google.protobuf.Any getAdditionalData(int index);
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
int getAdditionalDataCount();
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList();
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index);
public tensorflow.decision_trees.GenericTreeModel.TreeNode.NodeTypeCase getNodeTypeCase();
}
/**
* Protobuf type {@code tensorflow.decision_trees.TreeNode}
*/
public static final class TreeNode extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.decision_trees.TreeNode)
TreeNodeOrBuilder {
private static final long serialVersionUID = 0L;
// Use TreeNode.newBuilder() to construct.
private TreeNode(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TreeNode() {
additionalData_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TreeNode(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.Int32Value.Builder subBuilder = null;
if (nodeId_ != null) {
subBuilder = nodeId_.toBuilder();
}
nodeId_ = input.readMessage(com.google.protobuf.Int32Value.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(nodeId_);
nodeId_ = subBuilder.buildPartial();
}
break;
}
case 18: {
com.google.protobuf.Int32Value.Builder subBuilder = null;
if (depth_ != null) {
subBuilder = depth_.toBuilder();
}
depth_ = input.readMessage(com.google.protobuf.Int32Value.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(depth_);
depth_ = subBuilder.buildPartial();
}
break;
}
case 26: {
com.google.protobuf.Int32Value.Builder subBuilder = null;
if (subtreeSize_ != null) {
subBuilder = subtreeSize_.toBuilder();
}
subtreeSize_ = input.readMessage(com.google.protobuf.Int32Value.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(subtreeSize_);
subtreeSize_ = subBuilder.buildPartial();
}
break;
}
case 34: {
tensorflow.decision_trees.GenericTreeModel.BinaryNode.Builder subBuilder = null;
if (nodeTypeCase_ == 4) {
subBuilder = ((tensorflow.decision_trees.GenericTreeModel.BinaryNode) nodeType_).toBuilder();
}
nodeType_ =
input.readMessage(tensorflow.decision_trees.GenericTreeModel.BinaryNode.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((tensorflow.decision_trees.GenericTreeModel.BinaryNode) nodeType_);
nodeType_ = subBuilder.buildPartial();
}
nodeTypeCase_ = 4;
break;
}
case 42: {
tensorflow.decision_trees.GenericTreeModel.Leaf.Builder subBuilder = null;
if (nodeTypeCase_ == 5) {
subBuilder = ((tensorflow.decision_trees.GenericTreeModel.Leaf) nodeType_).toBuilder();
}
nodeType_ =
input.readMessage(tensorflow.decision_trees.GenericTreeModel.Leaf.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((tensorflow.decision_trees.GenericTreeModel.Leaf) nodeType_);
nodeType_ = subBuilder.buildPartial();
}
nodeTypeCase_ = 5;
break;
}
case 50: {
com.google.protobuf.Any.Builder subBuilder = null;
if (nodeTypeCase_ == 6) {
subBuilder = ((com.google.protobuf.Any) nodeType_).toBuilder();
}
nodeType_ =
input.readMessage(com.google.protobuf.Any.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((com.google.protobuf.Any) nodeType_);
nodeType_ = subBuilder.buildPartial();
}
nodeTypeCase_ = 6;
break;
}
case 58: {
if (!((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
additionalData_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
additionalData_.add(
input.readMessage(com.google.protobuf.Any.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
additionalData_ = java.util.Collections.unmodifiableList(additionalData_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_TreeNode_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_TreeNode_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.TreeNode.class, tensorflow.decision_trees.GenericTreeModel.TreeNode.Builder.class);
}
private int bitField0_;
private int nodeTypeCase_ = 0;
private java.lang.Object nodeType_;
public enum NodeTypeCase
implements com.google.protobuf.Internal.EnumLite {
BINARY_NODE(4),
LEAF(5),
CUSTOM_NODE_TYPE(6),
NODETYPE_NOT_SET(0);
private final int value;
private NodeTypeCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static NodeTypeCase valueOf(int value) {
return forNumber(value);
}
public static NodeTypeCase forNumber(int value) {
switch (value) {
case 4: return BINARY_NODE;
case 5: return LEAF;
case 6: return CUSTOM_NODE_TYPE;
case 0: return NODETYPE_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public NodeTypeCase
getNodeTypeCase() {
return NodeTypeCase.forNumber(
nodeTypeCase_);
}
public static final int NODE_ID_FIELD_NUMBER = 1;
private com.google.protobuf.Int32Value nodeId_;
/**
*
* Following fields are provided for convenience and better readability.
* Filling them in is not required.
*
*
* .google.protobuf.Int32Value node_id = 1;
*/
public boolean hasNodeId() {
return nodeId_ != null;
}
/**
*
* Following fields are provided for convenience and better readability.
* Filling them in is not required.
*
*
* .google.protobuf.Int32Value node_id = 1;
*/
public com.google.protobuf.Int32Value getNodeId() {
return nodeId_ == null ? com.google.protobuf.Int32Value.getDefaultInstance() : nodeId_;
}
/**
*
* Following fields are provided for convenience and better readability.
* Filling them in is not required.
*
*
* .google.protobuf.Int32Value node_id = 1;
*/
public com.google.protobuf.Int32ValueOrBuilder getNodeIdOrBuilder() {
return getNodeId();
}
public static final int DEPTH_FIELD_NUMBER = 2;
private com.google.protobuf.Int32Value depth_;
/**
* .google.protobuf.Int32Value depth = 2;
*/
public boolean hasDepth() {
return depth_ != null;
}
/**
* .google.protobuf.Int32Value depth = 2;
*/
public com.google.protobuf.Int32Value getDepth() {
return depth_ == null ? com.google.protobuf.Int32Value.getDefaultInstance() : depth_;
}
/**
* .google.protobuf.Int32Value depth = 2;
*/
public com.google.protobuf.Int32ValueOrBuilder getDepthOrBuilder() {
return getDepth();
}
public static final int SUBTREE_SIZE_FIELD_NUMBER = 3;
private com.google.protobuf.Int32Value subtreeSize_;
/**
* .google.protobuf.Int32Value subtree_size = 3;
*/
public boolean hasSubtreeSize() {
return subtreeSize_ != null;
}
/**
* .google.protobuf.Int32Value subtree_size = 3;
*/
public com.google.protobuf.Int32Value getSubtreeSize() {
return subtreeSize_ == null ? com.google.protobuf.Int32Value.getDefaultInstance() : subtreeSize_;
}
/**
* .google.protobuf.Int32Value subtree_size = 3;
*/
public com.google.protobuf.Int32ValueOrBuilder getSubtreeSizeOrBuilder() {
return getSubtreeSize();
}
public static final int BINARY_NODE_FIELD_NUMBER = 4;
/**
* .tensorflow.decision_trees.BinaryNode binary_node = 4;
*/
public boolean hasBinaryNode() {
return nodeTypeCase_ == 4;
}
/**
* .tensorflow.decision_trees.BinaryNode binary_node = 4;
*/
public tensorflow.decision_trees.GenericTreeModel.BinaryNode getBinaryNode() {
if (nodeTypeCase_ == 4) {
return (tensorflow.decision_trees.GenericTreeModel.BinaryNode) nodeType_;
}
return tensorflow.decision_trees.GenericTreeModel.BinaryNode.getDefaultInstance();
}
/**
* .tensorflow.decision_trees.BinaryNode binary_node = 4;
*/
public tensorflow.decision_trees.GenericTreeModel.BinaryNodeOrBuilder getBinaryNodeOrBuilder() {
if (nodeTypeCase_ == 4) {
return (tensorflow.decision_trees.GenericTreeModel.BinaryNode) nodeType_;
}
return tensorflow.decision_trees.GenericTreeModel.BinaryNode.getDefaultInstance();
}
public static final int LEAF_FIELD_NUMBER = 5;
/**
* .tensorflow.decision_trees.Leaf leaf = 5;
*/
public boolean hasLeaf() {
return nodeTypeCase_ == 5;
}
/**
* .tensorflow.decision_trees.Leaf leaf = 5;
*/
public tensorflow.decision_trees.GenericTreeModel.Leaf getLeaf() {
if (nodeTypeCase_ == 5) {
return (tensorflow.decision_trees.GenericTreeModel.Leaf) nodeType_;
}
return tensorflow.decision_trees.GenericTreeModel.Leaf.getDefaultInstance();
}
/**
* .tensorflow.decision_trees.Leaf leaf = 5;
*/
public tensorflow.decision_trees.GenericTreeModel.LeafOrBuilder getLeafOrBuilder() {
if (nodeTypeCase_ == 5) {
return (tensorflow.decision_trees.GenericTreeModel.Leaf) nodeType_;
}
return tensorflow.decision_trees.GenericTreeModel.Leaf.getDefaultInstance();
}
public static final int CUSTOM_NODE_TYPE_FIELD_NUMBER = 6;
/**
* .google.protobuf.Any custom_node_type = 6;
*/
public boolean hasCustomNodeType() {
return nodeTypeCase_ == 6;
}
/**
* .google.protobuf.Any custom_node_type = 6;
*/
public com.google.protobuf.Any getCustomNodeType() {
if (nodeTypeCase_ == 6) {
return (com.google.protobuf.Any) nodeType_;
}
return com.google.protobuf.Any.getDefaultInstance();
}
/**
* .google.protobuf.Any custom_node_type = 6;
*/
public com.google.protobuf.AnyOrBuilder getCustomNodeTypeOrBuilder() {
if (nodeTypeCase_ == 6) {
return (com.google.protobuf.Any) nodeType_;
}
return com.google.protobuf.Any.getDefaultInstance();
}
public static final int ADDITIONAL_DATA_FIELD_NUMBER = 7;
private java.util.List additionalData_;
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
public java.util.List getAdditionalDataList() {
return additionalData_;
}
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
public java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList() {
return additionalData_;
}
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
public int getAdditionalDataCount() {
return additionalData_.size();
}
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
public com.google.protobuf.Any getAdditionalData(int index) {
return additionalData_.get(index);
}
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
public com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index) {
return additionalData_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (nodeId_ != null) {
output.writeMessage(1, getNodeId());
}
if (depth_ != null) {
output.writeMessage(2, getDepth());
}
if (subtreeSize_ != null) {
output.writeMessage(3, getSubtreeSize());
}
if (nodeTypeCase_ == 4) {
output.writeMessage(4, (tensorflow.decision_trees.GenericTreeModel.BinaryNode) nodeType_);
}
if (nodeTypeCase_ == 5) {
output.writeMessage(5, (tensorflow.decision_trees.GenericTreeModel.Leaf) nodeType_);
}
if (nodeTypeCase_ == 6) {
output.writeMessage(6, (com.google.protobuf.Any) nodeType_);
}
for (int i = 0; i < additionalData_.size(); i++) {
output.writeMessage(7, additionalData_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (nodeId_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getNodeId());
}
if (depth_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getDepth());
}
if (subtreeSize_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getSubtreeSize());
}
if (nodeTypeCase_ == 4) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, (tensorflow.decision_trees.GenericTreeModel.BinaryNode) nodeType_);
}
if (nodeTypeCase_ == 5) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, (tensorflow.decision_trees.GenericTreeModel.Leaf) nodeType_);
}
if (nodeTypeCase_ == 6) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, (com.google.protobuf.Any) nodeType_);
}
for (int i = 0; i < additionalData_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, additionalData_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.decision_trees.GenericTreeModel.TreeNode)) {
return super.equals(obj);
}
tensorflow.decision_trees.GenericTreeModel.TreeNode other = (tensorflow.decision_trees.GenericTreeModel.TreeNode) obj;
boolean result = true;
result = result && (hasNodeId() == other.hasNodeId());
if (hasNodeId()) {
result = result && getNodeId()
.equals(other.getNodeId());
}
result = result && (hasDepth() == other.hasDepth());
if (hasDepth()) {
result = result && getDepth()
.equals(other.getDepth());
}
result = result && (hasSubtreeSize() == other.hasSubtreeSize());
if (hasSubtreeSize()) {
result = result && getSubtreeSize()
.equals(other.getSubtreeSize());
}
result = result && getAdditionalDataList()
.equals(other.getAdditionalDataList());
result = result && getNodeTypeCase().equals(
other.getNodeTypeCase());
if (!result) return false;
switch (nodeTypeCase_) {
case 4:
result = result && getBinaryNode()
.equals(other.getBinaryNode());
break;
case 5:
result = result && getLeaf()
.equals(other.getLeaf());
break;
case 6:
result = result && getCustomNodeType()
.equals(other.getCustomNodeType());
break;
case 0:
default:
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasNodeId()) {
hash = (37 * hash) + NODE_ID_FIELD_NUMBER;
hash = (53 * hash) + getNodeId().hashCode();
}
if (hasDepth()) {
hash = (37 * hash) + DEPTH_FIELD_NUMBER;
hash = (53 * hash) + getDepth().hashCode();
}
if (hasSubtreeSize()) {
hash = (37 * hash) + SUBTREE_SIZE_FIELD_NUMBER;
hash = (53 * hash) + getSubtreeSize().hashCode();
}
if (getAdditionalDataCount() > 0) {
hash = (37 * hash) + ADDITIONAL_DATA_FIELD_NUMBER;
hash = (53 * hash) + getAdditionalDataList().hashCode();
}
switch (nodeTypeCase_) {
case 4:
hash = (37 * hash) + BINARY_NODE_FIELD_NUMBER;
hash = (53 * hash) + getBinaryNode().hashCode();
break;
case 5:
hash = (37 * hash) + LEAF_FIELD_NUMBER;
hash = (53 * hash) + getLeaf().hashCode();
break;
case 6:
hash = (37 * hash) + CUSTOM_NODE_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getCustomNodeType().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.decision_trees.GenericTreeModel.TreeNode parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.TreeNode parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.TreeNode parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.TreeNode parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.TreeNode parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.TreeNode parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.TreeNode parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.TreeNode parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.TreeNode parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.TreeNode parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.TreeNode parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.TreeNode parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.decision_trees.GenericTreeModel.TreeNode prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.decision_trees.TreeNode}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.decision_trees.TreeNode)
tensorflow.decision_trees.GenericTreeModel.TreeNodeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_TreeNode_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_TreeNode_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.TreeNode.class, tensorflow.decision_trees.GenericTreeModel.TreeNode.Builder.class);
}
// Construct using tensorflow.decision_trees.GenericTreeModel.TreeNode.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getAdditionalDataFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (nodeIdBuilder_ == null) {
nodeId_ = null;
} else {
nodeId_ = null;
nodeIdBuilder_ = null;
}
if (depthBuilder_ == null) {
depth_ = null;
} else {
depth_ = null;
depthBuilder_ = null;
}
if (subtreeSizeBuilder_ == null) {
subtreeSize_ = null;
} else {
subtreeSize_ = null;
subtreeSizeBuilder_ = null;
}
if (additionalDataBuilder_ == null) {
additionalData_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
} else {
additionalDataBuilder_.clear();
}
nodeTypeCase_ = 0;
nodeType_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_TreeNode_descriptor;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.TreeNode getDefaultInstanceForType() {
return tensorflow.decision_trees.GenericTreeModel.TreeNode.getDefaultInstance();
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.TreeNode build() {
tensorflow.decision_trees.GenericTreeModel.TreeNode result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.TreeNode buildPartial() {
tensorflow.decision_trees.GenericTreeModel.TreeNode result = new tensorflow.decision_trees.GenericTreeModel.TreeNode(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (nodeIdBuilder_ == null) {
result.nodeId_ = nodeId_;
} else {
result.nodeId_ = nodeIdBuilder_.build();
}
if (depthBuilder_ == null) {
result.depth_ = depth_;
} else {
result.depth_ = depthBuilder_.build();
}
if (subtreeSizeBuilder_ == null) {
result.subtreeSize_ = subtreeSize_;
} else {
result.subtreeSize_ = subtreeSizeBuilder_.build();
}
if (nodeTypeCase_ == 4) {
if (binaryNodeBuilder_ == null) {
result.nodeType_ = nodeType_;
} else {
result.nodeType_ = binaryNodeBuilder_.build();
}
}
if (nodeTypeCase_ == 5) {
if (leafBuilder_ == null) {
result.nodeType_ = nodeType_;
} else {
result.nodeType_ = leafBuilder_.build();
}
}
if (nodeTypeCase_ == 6) {
if (customNodeTypeBuilder_ == null) {
result.nodeType_ = nodeType_;
} else {
result.nodeType_ = customNodeTypeBuilder_.build();
}
}
if (additionalDataBuilder_ == null) {
if (((bitField0_ & 0x00000040) == 0x00000040)) {
additionalData_ = java.util.Collections.unmodifiableList(additionalData_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.additionalData_ = additionalData_;
} else {
result.additionalData_ = additionalDataBuilder_.build();
}
result.bitField0_ = to_bitField0_;
result.nodeTypeCase_ = nodeTypeCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.decision_trees.GenericTreeModel.TreeNode) {
return mergeFrom((tensorflow.decision_trees.GenericTreeModel.TreeNode)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.decision_trees.GenericTreeModel.TreeNode other) {
if (other == tensorflow.decision_trees.GenericTreeModel.TreeNode.getDefaultInstance()) return this;
if (other.hasNodeId()) {
mergeNodeId(other.getNodeId());
}
if (other.hasDepth()) {
mergeDepth(other.getDepth());
}
if (other.hasSubtreeSize()) {
mergeSubtreeSize(other.getSubtreeSize());
}
if (additionalDataBuilder_ == null) {
if (!other.additionalData_.isEmpty()) {
if (additionalData_.isEmpty()) {
additionalData_ = other.additionalData_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureAdditionalDataIsMutable();
additionalData_.addAll(other.additionalData_);
}
onChanged();
}
} else {
if (!other.additionalData_.isEmpty()) {
if (additionalDataBuilder_.isEmpty()) {
additionalDataBuilder_.dispose();
additionalDataBuilder_ = null;
additionalData_ = other.additionalData_;
bitField0_ = (bitField0_ & ~0x00000040);
additionalDataBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAdditionalDataFieldBuilder() : null;
} else {
additionalDataBuilder_.addAllMessages(other.additionalData_);
}
}
}
switch (other.getNodeTypeCase()) {
case BINARY_NODE: {
mergeBinaryNode(other.getBinaryNode());
break;
}
case LEAF: {
mergeLeaf(other.getLeaf());
break;
}
case CUSTOM_NODE_TYPE: {
mergeCustomNodeType(other.getCustomNodeType());
break;
}
case NODETYPE_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.decision_trees.GenericTreeModel.TreeNode parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.decision_trees.GenericTreeModel.TreeNode) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int nodeTypeCase_ = 0;
private java.lang.Object nodeType_;
public NodeTypeCase
getNodeTypeCase() {
return NodeTypeCase.forNumber(
nodeTypeCase_);
}
public Builder clearNodeType() {
nodeTypeCase_ = 0;
nodeType_ = null;
onChanged();
return this;
}
private int bitField0_;
private com.google.protobuf.Int32Value nodeId_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int32Value, com.google.protobuf.Int32Value.Builder, com.google.protobuf.Int32ValueOrBuilder> nodeIdBuilder_;
/**
*
* Following fields are provided for convenience and better readability.
* Filling them in is not required.
*
*
* .google.protobuf.Int32Value node_id = 1;
*/
public boolean hasNodeId() {
return nodeIdBuilder_ != null || nodeId_ != null;
}
/**
*
* Following fields are provided for convenience and better readability.
* Filling them in is not required.
*
*
* .google.protobuf.Int32Value node_id = 1;
*/
public com.google.protobuf.Int32Value getNodeId() {
if (nodeIdBuilder_ == null) {
return nodeId_ == null ? com.google.protobuf.Int32Value.getDefaultInstance() : nodeId_;
} else {
return nodeIdBuilder_.getMessage();
}
}
/**
*
* Following fields are provided for convenience and better readability.
* Filling them in is not required.
*
*
* .google.protobuf.Int32Value node_id = 1;
*/
public Builder setNodeId(com.google.protobuf.Int32Value value) {
if (nodeIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
nodeId_ = value;
onChanged();
} else {
nodeIdBuilder_.setMessage(value);
}
return this;
}
/**
*
* Following fields are provided for convenience and better readability.
* Filling them in is not required.
*
*
* .google.protobuf.Int32Value node_id = 1;
*/
public Builder setNodeId(
com.google.protobuf.Int32Value.Builder builderForValue) {
if (nodeIdBuilder_ == null) {
nodeId_ = builderForValue.build();
onChanged();
} else {
nodeIdBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Following fields are provided for convenience and better readability.
* Filling them in is not required.
*
*
* .google.protobuf.Int32Value node_id = 1;
*/
public Builder mergeNodeId(com.google.protobuf.Int32Value value) {
if (nodeIdBuilder_ == null) {
if (nodeId_ != null) {
nodeId_ =
com.google.protobuf.Int32Value.newBuilder(nodeId_).mergeFrom(value).buildPartial();
} else {
nodeId_ = value;
}
onChanged();
} else {
nodeIdBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Following fields are provided for convenience and better readability.
* Filling them in is not required.
*
*
* .google.protobuf.Int32Value node_id = 1;
*/
public Builder clearNodeId() {
if (nodeIdBuilder_ == null) {
nodeId_ = null;
onChanged();
} else {
nodeId_ = null;
nodeIdBuilder_ = null;
}
return this;
}
/**
*
* Following fields are provided for convenience and better readability.
* Filling them in is not required.
*
*
* .google.protobuf.Int32Value node_id = 1;
*/
public com.google.protobuf.Int32Value.Builder getNodeIdBuilder() {
onChanged();
return getNodeIdFieldBuilder().getBuilder();
}
/**
*
* Following fields are provided for convenience and better readability.
* Filling them in is not required.
*
*
* .google.protobuf.Int32Value node_id = 1;
*/
public com.google.protobuf.Int32ValueOrBuilder getNodeIdOrBuilder() {
if (nodeIdBuilder_ != null) {
return nodeIdBuilder_.getMessageOrBuilder();
} else {
return nodeId_ == null ?
com.google.protobuf.Int32Value.getDefaultInstance() : nodeId_;
}
}
/**
*
* Following fields are provided for convenience and better readability.
* Filling them in is not required.
*
*
* .google.protobuf.Int32Value node_id = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int32Value, com.google.protobuf.Int32Value.Builder, com.google.protobuf.Int32ValueOrBuilder>
getNodeIdFieldBuilder() {
if (nodeIdBuilder_ == null) {
nodeIdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int32Value, com.google.protobuf.Int32Value.Builder, com.google.protobuf.Int32ValueOrBuilder>(
getNodeId(),
getParentForChildren(),
isClean());
nodeId_ = null;
}
return nodeIdBuilder_;
}
private com.google.protobuf.Int32Value depth_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int32Value, com.google.protobuf.Int32Value.Builder, com.google.protobuf.Int32ValueOrBuilder> depthBuilder_;
/**
* .google.protobuf.Int32Value depth = 2;
*/
public boolean hasDepth() {
return depthBuilder_ != null || depth_ != null;
}
/**
* .google.protobuf.Int32Value depth = 2;
*/
public com.google.protobuf.Int32Value getDepth() {
if (depthBuilder_ == null) {
return depth_ == null ? com.google.protobuf.Int32Value.getDefaultInstance() : depth_;
} else {
return depthBuilder_.getMessage();
}
}
/**
* .google.protobuf.Int32Value depth = 2;
*/
public Builder setDepth(com.google.protobuf.Int32Value value) {
if (depthBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
depth_ = value;
onChanged();
} else {
depthBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.Int32Value depth = 2;
*/
public Builder setDepth(
com.google.protobuf.Int32Value.Builder builderForValue) {
if (depthBuilder_ == null) {
depth_ = builderForValue.build();
onChanged();
} else {
depthBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.Int32Value depth = 2;
*/
public Builder mergeDepth(com.google.protobuf.Int32Value value) {
if (depthBuilder_ == null) {
if (depth_ != null) {
depth_ =
com.google.protobuf.Int32Value.newBuilder(depth_).mergeFrom(value).buildPartial();
} else {
depth_ = value;
}
onChanged();
} else {
depthBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.Int32Value depth = 2;
*/
public Builder clearDepth() {
if (depthBuilder_ == null) {
depth_ = null;
onChanged();
} else {
depth_ = null;
depthBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.Int32Value depth = 2;
*/
public com.google.protobuf.Int32Value.Builder getDepthBuilder() {
onChanged();
return getDepthFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Int32Value depth = 2;
*/
public com.google.protobuf.Int32ValueOrBuilder getDepthOrBuilder() {
if (depthBuilder_ != null) {
return depthBuilder_.getMessageOrBuilder();
} else {
return depth_ == null ?
com.google.protobuf.Int32Value.getDefaultInstance() : depth_;
}
}
/**
* .google.protobuf.Int32Value depth = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int32Value, com.google.protobuf.Int32Value.Builder, com.google.protobuf.Int32ValueOrBuilder>
getDepthFieldBuilder() {
if (depthBuilder_ == null) {
depthBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int32Value, com.google.protobuf.Int32Value.Builder, com.google.protobuf.Int32ValueOrBuilder>(
getDepth(),
getParentForChildren(),
isClean());
depth_ = null;
}
return depthBuilder_;
}
private com.google.protobuf.Int32Value subtreeSize_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int32Value, com.google.protobuf.Int32Value.Builder, com.google.protobuf.Int32ValueOrBuilder> subtreeSizeBuilder_;
/**
* .google.protobuf.Int32Value subtree_size = 3;
*/
public boolean hasSubtreeSize() {
return subtreeSizeBuilder_ != null || subtreeSize_ != null;
}
/**
* .google.protobuf.Int32Value subtree_size = 3;
*/
public com.google.protobuf.Int32Value getSubtreeSize() {
if (subtreeSizeBuilder_ == null) {
return subtreeSize_ == null ? com.google.protobuf.Int32Value.getDefaultInstance() : subtreeSize_;
} else {
return subtreeSizeBuilder_.getMessage();
}
}
/**
* .google.protobuf.Int32Value subtree_size = 3;
*/
public Builder setSubtreeSize(com.google.protobuf.Int32Value value) {
if (subtreeSizeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
subtreeSize_ = value;
onChanged();
} else {
subtreeSizeBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.Int32Value subtree_size = 3;
*/
public Builder setSubtreeSize(
com.google.protobuf.Int32Value.Builder builderForValue) {
if (subtreeSizeBuilder_ == null) {
subtreeSize_ = builderForValue.build();
onChanged();
} else {
subtreeSizeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.Int32Value subtree_size = 3;
*/
public Builder mergeSubtreeSize(com.google.protobuf.Int32Value value) {
if (subtreeSizeBuilder_ == null) {
if (subtreeSize_ != null) {
subtreeSize_ =
com.google.protobuf.Int32Value.newBuilder(subtreeSize_).mergeFrom(value).buildPartial();
} else {
subtreeSize_ = value;
}
onChanged();
} else {
subtreeSizeBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.Int32Value subtree_size = 3;
*/
public Builder clearSubtreeSize() {
if (subtreeSizeBuilder_ == null) {
subtreeSize_ = null;
onChanged();
} else {
subtreeSize_ = null;
subtreeSizeBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.Int32Value subtree_size = 3;
*/
public com.google.protobuf.Int32Value.Builder getSubtreeSizeBuilder() {
onChanged();
return getSubtreeSizeFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Int32Value subtree_size = 3;
*/
public com.google.protobuf.Int32ValueOrBuilder getSubtreeSizeOrBuilder() {
if (subtreeSizeBuilder_ != null) {
return subtreeSizeBuilder_.getMessageOrBuilder();
} else {
return subtreeSize_ == null ?
com.google.protobuf.Int32Value.getDefaultInstance() : subtreeSize_;
}
}
/**
* .google.protobuf.Int32Value subtree_size = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int32Value, com.google.protobuf.Int32Value.Builder, com.google.protobuf.Int32ValueOrBuilder>
getSubtreeSizeFieldBuilder() {
if (subtreeSizeBuilder_ == null) {
subtreeSizeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int32Value, com.google.protobuf.Int32Value.Builder, com.google.protobuf.Int32ValueOrBuilder>(
getSubtreeSize(),
getParentForChildren(),
isClean());
subtreeSize_ = null;
}
return subtreeSizeBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.BinaryNode, tensorflow.decision_trees.GenericTreeModel.BinaryNode.Builder, tensorflow.decision_trees.GenericTreeModel.BinaryNodeOrBuilder> binaryNodeBuilder_;
/**
* .tensorflow.decision_trees.BinaryNode binary_node = 4;
*/
public boolean hasBinaryNode() {
return nodeTypeCase_ == 4;
}
/**
* .tensorflow.decision_trees.BinaryNode binary_node = 4;
*/
public tensorflow.decision_trees.GenericTreeModel.BinaryNode getBinaryNode() {
if (binaryNodeBuilder_ == null) {
if (nodeTypeCase_ == 4) {
return (tensorflow.decision_trees.GenericTreeModel.BinaryNode) nodeType_;
}
return tensorflow.decision_trees.GenericTreeModel.BinaryNode.getDefaultInstance();
} else {
if (nodeTypeCase_ == 4) {
return binaryNodeBuilder_.getMessage();
}
return tensorflow.decision_trees.GenericTreeModel.BinaryNode.getDefaultInstance();
}
}
/**
* .tensorflow.decision_trees.BinaryNode binary_node = 4;
*/
public Builder setBinaryNode(tensorflow.decision_trees.GenericTreeModel.BinaryNode value) {
if (binaryNodeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
nodeType_ = value;
onChanged();
} else {
binaryNodeBuilder_.setMessage(value);
}
nodeTypeCase_ = 4;
return this;
}
/**
* .tensorflow.decision_trees.BinaryNode binary_node = 4;
*/
public Builder setBinaryNode(
tensorflow.decision_trees.GenericTreeModel.BinaryNode.Builder builderForValue) {
if (binaryNodeBuilder_ == null) {
nodeType_ = builderForValue.build();
onChanged();
} else {
binaryNodeBuilder_.setMessage(builderForValue.build());
}
nodeTypeCase_ = 4;
return this;
}
/**
* .tensorflow.decision_trees.BinaryNode binary_node = 4;
*/
public Builder mergeBinaryNode(tensorflow.decision_trees.GenericTreeModel.BinaryNode value) {
if (binaryNodeBuilder_ == null) {
if (nodeTypeCase_ == 4 &&
nodeType_ != tensorflow.decision_trees.GenericTreeModel.BinaryNode.getDefaultInstance()) {
nodeType_ = tensorflow.decision_trees.GenericTreeModel.BinaryNode.newBuilder((tensorflow.decision_trees.GenericTreeModel.BinaryNode) nodeType_)
.mergeFrom(value).buildPartial();
} else {
nodeType_ = value;
}
onChanged();
} else {
if (nodeTypeCase_ == 4) {
binaryNodeBuilder_.mergeFrom(value);
}
binaryNodeBuilder_.setMessage(value);
}
nodeTypeCase_ = 4;
return this;
}
/**
* .tensorflow.decision_trees.BinaryNode binary_node = 4;
*/
public Builder clearBinaryNode() {
if (binaryNodeBuilder_ == null) {
if (nodeTypeCase_ == 4) {
nodeTypeCase_ = 0;
nodeType_ = null;
onChanged();
}
} else {
if (nodeTypeCase_ == 4) {
nodeTypeCase_ = 0;
nodeType_ = null;
}
binaryNodeBuilder_.clear();
}
return this;
}
/**
* .tensorflow.decision_trees.BinaryNode binary_node = 4;
*/
public tensorflow.decision_trees.GenericTreeModel.BinaryNode.Builder getBinaryNodeBuilder() {
return getBinaryNodeFieldBuilder().getBuilder();
}
/**
* .tensorflow.decision_trees.BinaryNode binary_node = 4;
*/
public tensorflow.decision_trees.GenericTreeModel.BinaryNodeOrBuilder getBinaryNodeOrBuilder() {
if ((nodeTypeCase_ == 4) && (binaryNodeBuilder_ != null)) {
return binaryNodeBuilder_.getMessageOrBuilder();
} else {
if (nodeTypeCase_ == 4) {
return (tensorflow.decision_trees.GenericTreeModel.BinaryNode) nodeType_;
}
return tensorflow.decision_trees.GenericTreeModel.BinaryNode.getDefaultInstance();
}
}
/**
* .tensorflow.decision_trees.BinaryNode binary_node = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.BinaryNode, tensorflow.decision_trees.GenericTreeModel.BinaryNode.Builder, tensorflow.decision_trees.GenericTreeModel.BinaryNodeOrBuilder>
getBinaryNodeFieldBuilder() {
if (binaryNodeBuilder_ == null) {
if (!(nodeTypeCase_ == 4)) {
nodeType_ = tensorflow.decision_trees.GenericTreeModel.BinaryNode.getDefaultInstance();
}
binaryNodeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.BinaryNode, tensorflow.decision_trees.GenericTreeModel.BinaryNode.Builder, tensorflow.decision_trees.GenericTreeModel.BinaryNodeOrBuilder>(
(tensorflow.decision_trees.GenericTreeModel.BinaryNode) nodeType_,
getParentForChildren(),
isClean());
nodeType_ = null;
}
nodeTypeCase_ = 4;
onChanged();;
return binaryNodeBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Leaf, tensorflow.decision_trees.GenericTreeModel.Leaf.Builder, tensorflow.decision_trees.GenericTreeModel.LeafOrBuilder> leafBuilder_;
/**
* .tensorflow.decision_trees.Leaf leaf = 5;
*/
public boolean hasLeaf() {
return nodeTypeCase_ == 5;
}
/**
* .tensorflow.decision_trees.Leaf leaf = 5;
*/
public tensorflow.decision_trees.GenericTreeModel.Leaf getLeaf() {
if (leafBuilder_ == null) {
if (nodeTypeCase_ == 5) {
return (tensorflow.decision_trees.GenericTreeModel.Leaf) nodeType_;
}
return tensorflow.decision_trees.GenericTreeModel.Leaf.getDefaultInstance();
} else {
if (nodeTypeCase_ == 5) {
return leafBuilder_.getMessage();
}
return tensorflow.decision_trees.GenericTreeModel.Leaf.getDefaultInstance();
}
}
/**
* .tensorflow.decision_trees.Leaf leaf = 5;
*/
public Builder setLeaf(tensorflow.decision_trees.GenericTreeModel.Leaf value) {
if (leafBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
nodeType_ = value;
onChanged();
} else {
leafBuilder_.setMessage(value);
}
nodeTypeCase_ = 5;
return this;
}
/**
* .tensorflow.decision_trees.Leaf leaf = 5;
*/
public Builder setLeaf(
tensorflow.decision_trees.GenericTreeModel.Leaf.Builder builderForValue) {
if (leafBuilder_ == null) {
nodeType_ = builderForValue.build();
onChanged();
} else {
leafBuilder_.setMessage(builderForValue.build());
}
nodeTypeCase_ = 5;
return this;
}
/**
* .tensorflow.decision_trees.Leaf leaf = 5;
*/
public Builder mergeLeaf(tensorflow.decision_trees.GenericTreeModel.Leaf value) {
if (leafBuilder_ == null) {
if (nodeTypeCase_ == 5 &&
nodeType_ != tensorflow.decision_trees.GenericTreeModel.Leaf.getDefaultInstance()) {
nodeType_ = tensorflow.decision_trees.GenericTreeModel.Leaf.newBuilder((tensorflow.decision_trees.GenericTreeModel.Leaf) nodeType_)
.mergeFrom(value).buildPartial();
} else {
nodeType_ = value;
}
onChanged();
} else {
if (nodeTypeCase_ == 5) {
leafBuilder_.mergeFrom(value);
}
leafBuilder_.setMessage(value);
}
nodeTypeCase_ = 5;
return this;
}
/**
* .tensorflow.decision_trees.Leaf leaf = 5;
*/
public Builder clearLeaf() {
if (leafBuilder_ == null) {
if (nodeTypeCase_ == 5) {
nodeTypeCase_ = 0;
nodeType_ = null;
onChanged();
}
} else {
if (nodeTypeCase_ == 5) {
nodeTypeCase_ = 0;
nodeType_ = null;
}
leafBuilder_.clear();
}
return this;
}
/**
* .tensorflow.decision_trees.Leaf leaf = 5;
*/
public tensorflow.decision_trees.GenericTreeModel.Leaf.Builder getLeafBuilder() {
return getLeafFieldBuilder().getBuilder();
}
/**
* .tensorflow.decision_trees.Leaf leaf = 5;
*/
public tensorflow.decision_trees.GenericTreeModel.LeafOrBuilder getLeafOrBuilder() {
if ((nodeTypeCase_ == 5) && (leafBuilder_ != null)) {
return leafBuilder_.getMessageOrBuilder();
} else {
if (nodeTypeCase_ == 5) {
return (tensorflow.decision_trees.GenericTreeModel.Leaf) nodeType_;
}
return tensorflow.decision_trees.GenericTreeModel.Leaf.getDefaultInstance();
}
}
/**
* .tensorflow.decision_trees.Leaf leaf = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Leaf, tensorflow.decision_trees.GenericTreeModel.Leaf.Builder, tensorflow.decision_trees.GenericTreeModel.LeafOrBuilder>
getLeafFieldBuilder() {
if (leafBuilder_ == null) {
if (!(nodeTypeCase_ == 5)) {
nodeType_ = tensorflow.decision_trees.GenericTreeModel.Leaf.getDefaultInstance();
}
leafBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Leaf, tensorflow.decision_trees.GenericTreeModel.Leaf.Builder, tensorflow.decision_trees.GenericTreeModel.LeafOrBuilder>(
(tensorflow.decision_trees.GenericTreeModel.Leaf) nodeType_,
getParentForChildren(),
isClean());
nodeType_ = null;
}
nodeTypeCase_ = 5;
onChanged();;
return leafBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> customNodeTypeBuilder_;
/**
* .google.protobuf.Any custom_node_type = 6;
*/
public boolean hasCustomNodeType() {
return nodeTypeCase_ == 6;
}
/**
* .google.protobuf.Any custom_node_type = 6;
*/
public com.google.protobuf.Any getCustomNodeType() {
if (customNodeTypeBuilder_ == null) {
if (nodeTypeCase_ == 6) {
return (com.google.protobuf.Any) nodeType_;
}
return com.google.protobuf.Any.getDefaultInstance();
} else {
if (nodeTypeCase_ == 6) {
return customNodeTypeBuilder_.getMessage();
}
return com.google.protobuf.Any.getDefaultInstance();
}
}
/**
* .google.protobuf.Any custom_node_type = 6;
*/
public Builder setCustomNodeType(com.google.protobuf.Any value) {
if (customNodeTypeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
nodeType_ = value;
onChanged();
} else {
customNodeTypeBuilder_.setMessage(value);
}
nodeTypeCase_ = 6;
return this;
}
/**
* .google.protobuf.Any custom_node_type = 6;
*/
public Builder setCustomNodeType(
com.google.protobuf.Any.Builder builderForValue) {
if (customNodeTypeBuilder_ == null) {
nodeType_ = builderForValue.build();
onChanged();
} else {
customNodeTypeBuilder_.setMessage(builderForValue.build());
}
nodeTypeCase_ = 6;
return this;
}
/**
* .google.protobuf.Any custom_node_type = 6;
*/
public Builder mergeCustomNodeType(com.google.protobuf.Any value) {
if (customNodeTypeBuilder_ == null) {
if (nodeTypeCase_ == 6 &&
nodeType_ != com.google.protobuf.Any.getDefaultInstance()) {
nodeType_ = com.google.protobuf.Any.newBuilder((com.google.protobuf.Any) nodeType_)
.mergeFrom(value).buildPartial();
} else {
nodeType_ = value;
}
onChanged();
} else {
if (nodeTypeCase_ == 6) {
customNodeTypeBuilder_.mergeFrom(value);
}
customNodeTypeBuilder_.setMessage(value);
}
nodeTypeCase_ = 6;
return this;
}
/**
* .google.protobuf.Any custom_node_type = 6;
*/
public Builder clearCustomNodeType() {
if (customNodeTypeBuilder_ == null) {
if (nodeTypeCase_ == 6) {
nodeTypeCase_ = 0;
nodeType_ = null;
onChanged();
}
} else {
if (nodeTypeCase_ == 6) {
nodeTypeCase_ = 0;
nodeType_ = null;
}
customNodeTypeBuilder_.clear();
}
return this;
}
/**
* .google.protobuf.Any custom_node_type = 6;
*/
public com.google.protobuf.Any.Builder getCustomNodeTypeBuilder() {
return getCustomNodeTypeFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Any custom_node_type = 6;
*/
public com.google.protobuf.AnyOrBuilder getCustomNodeTypeOrBuilder() {
if ((nodeTypeCase_ == 6) && (customNodeTypeBuilder_ != null)) {
return customNodeTypeBuilder_.getMessageOrBuilder();
} else {
if (nodeTypeCase_ == 6) {
return (com.google.protobuf.Any) nodeType_;
}
return com.google.protobuf.Any.getDefaultInstance();
}
}
/**
* .google.protobuf.Any custom_node_type = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getCustomNodeTypeFieldBuilder() {
if (customNodeTypeBuilder_ == null) {
if (!(nodeTypeCase_ == 6)) {
nodeType_ = com.google.protobuf.Any.getDefaultInstance();
}
customNodeTypeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
(com.google.protobuf.Any) nodeType_,
getParentForChildren(),
isClean());
nodeType_ = null;
}
nodeTypeCase_ = 6;
onChanged();;
return customNodeTypeBuilder_;
}
private java.util.List additionalData_ =
java.util.Collections.emptyList();
private void ensureAdditionalDataIsMutable() {
if (!((bitField0_ & 0x00000040) == 0x00000040)) {
additionalData_ = new java.util.ArrayList(additionalData_);
bitField0_ |= 0x00000040;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> additionalDataBuilder_;
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
public java.util.List getAdditionalDataList() {
if (additionalDataBuilder_ == null) {
return java.util.Collections.unmodifiableList(additionalData_);
} else {
return additionalDataBuilder_.getMessageList();
}
}
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
public int getAdditionalDataCount() {
if (additionalDataBuilder_ == null) {
return additionalData_.size();
} else {
return additionalDataBuilder_.getCount();
}
}
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
public com.google.protobuf.Any getAdditionalData(int index) {
if (additionalDataBuilder_ == null) {
return additionalData_.get(index);
} else {
return additionalDataBuilder_.getMessage(index);
}
}
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
public Builder setAdditionalData(
int index, com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.set(index, value);
onChanged();
} else {
additionalDataBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
public Builder setAdditionalData(
int index, com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.set(index, builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
public Builder addAdditionalData(com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.add(value);
onChanged();
} else {
additionalDataBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
public Builder addAdditionalData(
int index, com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.add(index, value);
onChanged();
} else {
additionalDataBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
public Builder addAdditionalData(
com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.add(builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
public Builder addAdditionalData(
int index, com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.add(index, builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
public Builder addAllAdditionalData(
java.lang.Iterable extends com.google.protobuf.Any> values) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, additionalData_);
onChanged();
} else {
additionalDataBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
public Builder clearAdditionalData() {
if (additionalDataBuilder_ == null) {
additionalData_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
} else {
additionalDataBuilder_.clear();
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
public Builder removeAdditionalData(int index) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.remove(index);
onChanged();
} else {
additionalDataBuilder_.remove(index);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
public com.google.protobuf.Any.Builder getAdditionalDataBuilder(
int index) {
return getAdditionalDataFieldBuilder().getBuilder(index);
}
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
public com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index) {
if (additionalDataBuilder_ == null) {
return additionalData_.get(index); } else {
return additionalDataBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
public java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList() {
if (additionalDataBuilder_ != null) {
return additionalDataBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(additionalData_);
}
}
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
public com.google.protobuf.Any.Builder addAdditionalDataBuilder() {
return getAdditionalDataFieldBuilder().addBuilder(
com.google.protobuf.Any.getDefaultInstance());
}
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
public com.google.protobuf.Any.Builder addAdditionalDataBuilder(
int index) {
return getAdditionalDataFieldBuilder().addBuilder(
index, com.google.protobuf.Any.getDefaultInstance());
}
/**
* repeated .google.protobuf.Any additional_data = 7;
*/
public java.util.List
getAdditionalDataBuilderList() {
return getAdditionalDataFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getAdditionalDataFieldBuilder() {
if (additionalDataBuilder_ == null) {
additionalDataBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
additionalData_,
((bitField0_ & 0x00000040) == 0x00000040),
getParentForChildren(),
isClean());
additionalData_ = null;
}
return additionalDataBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.decision_trees.TreeNode)
}
// @@protoc_insertion_point(class_scope:tensorflow.decision_trees.TreeNode)
private static final tensorflow.decision_trees.GenericTreeModel.TreeNode DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.decision_trees.GenericTreeModel.TreeNode();
}
public static tensorflow.decision_trees.GenericTreeModel.TreeNode getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TreeNode parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TreeNode(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.TreeNode getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BinaryNodeOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.decision_trees.BinaryNode)
com.google.protobuf.MessageOrBuilder {
/**
* .google.protobuf.Int32Value left_child_id = 1;
*/
boolean hasLeftChildId();
/**
* .google.protobuf.Int32Value left_child_id = 1;
*/
com.google.protobuf.Int32Value getLeftChildId();
/**
* .google.protobuf.Int32Value left_child_id = 1;
*/
com.google.protobuf.Int32ValueOrBuilder getLeftChildIdOrBuilder();
/**
* .google.protobuf.Int32Value right_child_id = 2;
*/
boolean hasRightChildId();
/**
* .google.protobuf.Int32Value right_child_id = 2;
*/
com.google.protobuf.Int32Value getRightChildId();
/**
* .google.protobuf.Int32Value right_child_id = 2;
*/
com.google.protobuf.Int32ValueOrBuilder getRightChildIdOrBuilder();
/**
*
* When left_child_test is undefined for a particular datapoint (e.g. because
* it's not defined when feature value is missing), the datapoint should go
* in this direction.
*
*
* .tensorflow.decision_trees.BinaryNode.Direction default_direction = 3;
*/
int getDefaultDirectionValue();
/**
*
* When left_child_test is undefined for a particular datapoint (e.g. because
* it's not defined when feature value is missing), the datapoint should go
* in this direction.
*
*
* .tensorflow.decision_trees.BinaryNode.Direction default_direction = 3;
*/
tensorflow.decision_trees.GenericTreeModel.BinaryNode.Direction getDefaultDirection();
/**
* .tensorflow.decision_trees.InequalityTest inequality_left_child_test = 4;
*/
boolean hasInequalityLeftChildTest();
/**
* .tensorflow.decision_trees.InequalityTest inequality_left_child_test = 4;
*/
tensorflow.decision_trees.GenericTreeModel.InequalityTest getInequalityLeftChildTest();
/**
* .tensorflow.decision_trees.InequalityTest inequality_left_child_test = 4;
*/
tensorflow.decision_trees.GenericTreeModel.InequalityTestOrBuilder getInequalityLeftChildTestOrBuilder();
/**
* .google.protobuf.Any custom_left_child_test = 5;
*/
boolean hasCustomLeftChildTest();
/**
* .google.protobuf.Any custom_left_child_test = 5;
*/
com.google.protobuf.Any getCustomLeftChildTest();
/**
* .google.protobuf.Any custom_left_child_test = 5;
*/
com.google.protobuf.AnyOrBuilder getCustomLeftChildTestOrBuilder();
public tensorflow.decision_trees.GenericTreeModel.BinaryNode.LeftChildTestCase getLeftChildTestCase();
}
/**
* Protobuf type {@code tensorflow.decision_trees.BinaryNode}
*/
public static final class BinaryNode extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.decision_trees.BinaryNode)
BinaryNodeOrBuilder {
private static final long serialVersionUID = 0L;
// Use BinaryNode.newBuilder() to construct.
private BinaryNode(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BinaryNode() {
defaultDirection_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private BinaryNode(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.Int32Value.Builder subBuilder = null;
if (leftChildId_ != null) {
subBuilder = leftChildId_.toBuilder();
}
leftChildId_ = input.readMessage(com.google.protobuf.Int32Value.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(leftChildId_);
leftChildId_ = subBuilder.buildPartial();
}
break;
}
case 18: {
com.google.protobuf.Int32Value.Builder subBuilder = null;
if (rightChildId_ != null) {
subBuilder = rightChildId_.toBuilder();
}
rightChildId_ = input.readMessage(com.google.protobuf.Int32Value.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(rightChildId_);
rightChildId_ = subBuilder.buildPartial();
}
break;
}
case 24: {
int rawValue = input.readEnum();
defaultDirection_ = rawValue;
break;
}
case 34: {
tensorflow.decision_trees.GenericTreeModel.InequalityTest.Builder subBuilder = null;
if (leftChildTestCase_ == 4) {
subBuilder = ((tensorflow.decision_trees.GenericTreeModel.InequalityTest) leftChildTest_).toBuilder();
}
leftChildTest_ =
input.readMessage(tensorflow.decision_trees.GenericTreeModel.InequalityTest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((tensorflow.decision_trees.GenericTreeModel.InequalityTest) leftChildTest_);
leftChildTest_ = subBuilder.buildPartial();
}
leftChildTestCase_ = 4;
break;
}
case 42: {
com.google.protobuf.Any.Builder subBuilder = null;
if (leftChildTestCase_ == 5) {
subBuilder = ((com.google.protobuf.Any) leftChildTest_).toBuilder();
}
leftChildTest_ =
input.readMessage(com.google.protobuf.Any.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((com.google.protobuf.Any) leftChildTest_);
leftChildTest_ = subBuilder.buildPartial();
}
leftChildTestCase_ = 5;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_BinaryNode_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_BinaryNode_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.BinaryNode.class, tensorflow.decision_trees.GenericTreeModel.BinaryNode.Builder.class);
}
/**
* Protobuf enum {@code tensorflow.decision_trees.BinaryNode.Direction}
*/
public enum Direction
implements com.google.protobuf.ProtocolMessageEnum {
/**
* LEFT = 0;
*/
LEFT(0),
/**
* RIGHT = 1;
*/
RIGHT(1),
UNRECOGNIZED(-1),
;
/**
* LEFT = 0;
*/
public static final int LEFT_VALUE = 0;
/**
* RIGHT = 1;
*/
public static final int RIGHT_VALUE = 1;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Direction valueOf(int value) {
return forNumber(value);
}
public static Direction forNumber(int value) {
switch (value) {
case 0: return LEFT;
case 1: return RIGHT;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Direction> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Direction findValueByNumber(int number) {
return Direction.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.BinaryNode.getDescriptor().getEnumTypes().get(0);
}
private static final Direction[] VALUES = values();
public static Direction valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Direction(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:tensorflow.decision_trees.BinaryNode.Direction)
}
private int leftChildTestCase_ = 0;
private java.lang.Object leftChildTest_;
public enum LeftChildTestCase
implements com.google.protobuf.Internal.EnumLite {
INEQUALITY_LEFT_CHILD_TEST(4),
CUSTOM_LEFT_CHILD_TEST(5),
LEFTCHILDTEST_NOT_SET(0);
private final int value;
private LeftChildTestCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static LeftChildTestCase valueOf(int value) {
return forNumber(value);
}
public static LeftChildTestCase forNumber(int value) {
switch (value) {
case 4: return INEQUALITY_LEFT_CHILD_TEST;
case 5: return CUSTOM_LEFT_CHILD_TEST;
case 0: return LEFTCHILDTEST_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public LeftChildTestCase
getLeftChildTestCase() {
return LeftChildTestCase.forNumber(
leftChildTestCase_);
}
public static final int LEFT_CHILD_ID_FIELD_NUMBER = 1;
private com.google.protobuf.Int32Value leftChildId_;
/**
* .google.protobuf.Int32Value left_child_id = 1;
*/
public boolean hasLeftChildId() {
return leftChildId_ != null;
}
/**
* .google.protobuf.Int32Value left_child_id = 1;
*/
public com.google.protobuf.Int32Value getLeftChildId() {
return leftChildId_ == null ? com.google.protobuf.Int32Value.getDefaultInstance() : leftChildId_;
}
/**
* .google.protobuf.Int32Value left_child_id = 1;
*/
public com.google.protobuf.Int32ValueOrBuilder getLeftChildIdOrBuilder() {
return getLeftChildId();
}
public static final int RIGHT_CHILD_ID_FIELD_NUMBER = 2;
private com.google.protobuf.Int32Value rightChildId_;
/**
* .google.protobuf.Int32Value right_child_id = 2;
*/
public boolean hasRightChildId() {
return rightChildId_ != null;
}
/**
* .google.protobuf.Int32Value right_child_id = 2;
*/
public com.google.protobuf.Int32Value getRightChildId() {
return rightChildId_ == null ? com.google.protobuf.Int32Value.getDefaultInstance() : rightChildId_;
}
/**
* .google.protobuf.Int32Value right_child_id = 2;
*/
public com.google.protobuf.Int32ValueOrBuilder getRightChildIdOrBuilder() {
return getRightChildId();
}
public static final int DEFAULT_DIRECTION_FIELD_NUMBER = 3;
private int defaultDirection_;
/**
*
* When left_child_test is undefined for a particular datapoint (e.g. because
* it's not defined when feature value is missing), the datapoint should go
* in this direction.
*
*
* .tensorflow.decision_trees.BinaryNode.Direction default_direction = 3;
*/
public int getDefaultDirectionValue() {
return defaultDirection_;
}
/**
*
* When left_child_test is undefined for a particular datapoint (e.g. because
* it's not defined when feature value is missing), the datapoint should go
* in this direction.
*
*
* .tensorflow.decision_trees.BinaryNode.Direction default_direction = 3;
*/
public tensorflow.decision_trees.GenericTreeModel.BinaryNode.Direction getDefaultDirection() {
@SuppressWarnings("deprecation")
tensorflow.decision_trees.GenericTreeModel.BinaryNode.Direction result = tensorflow.decision_trees.GenericTreeModel.BinaryNode.Direction.valueOf(defaultDirection_);
return result == null ? tensorflow.decision_trees.GenericTreeModel.BinaryNode.Direction.UNRECOGNIZED : result;
}
public static final int INEQUALITY_LEFT_CHILD_TEST_FIELD_NUMBER = 4;
/**
* .tensorflow.decision_trees.InequalityTest inequality_left_child_test = 4;
*/
public boolean hasInequalityLeftChildTest() {
return leftChildTestCase_ == 4;
}
/**
* .tensorflow.decision_trees.InequalityTest inequality_left_child_test = 4;
*/
public tensorflow.decision_trees.GenericTreeModel.InequalityTest getInequalityLeftChildTest() {
if (leftChildTestCase_ == 4) {
return (tensorflow.decision_trees.GenericTreeModel.InequalityTest) leftChildTest_;
}
return tensorflow.decision_trees.GenericTreeModel.InequalityTest.getDefaultInstance();
}
/**
* .tensorflow.decision_trees.InequalityTest inequality_left_child_test = 4;
*/
public tensorflow.decision_trees.GenericTreeModel.InequalityTestOrBuilder getInequalityLeftChildTestOrBuilder() {
if (leftChildTestCase_ == 4) {
return (tensorflow.decision_trees.GenericTreeModel.InequalityTest) leftChildTest_;
}
return tensorflow.decision_trees.GenericTreeModel.InequalityTest.getDefaultInstance();
}
public static final int CUSTOM_LEFT_CHILD_TEST_FIELD_NUMBER = 5;
/**
* .google.protobuf.Any custom_left_child_test = 5;
*/
public boolean hasCustomLeftChildTest() {
return leftChildTestCase_ == 5;
}
/**
* .google.protobuf.Any custom_left_child_test = 5;
*/
public com.google.protobuf.Any getCustomLeftChildTest() {
if (leftChildTestCase_ == 5) {
return (com.google.protobuf.Any) leftChildTest_;
}
return com.google.protobuf.Any.getDefaultInstance();
}
/**
* .google.protobuf.Any custom_left_child_test = 5;
*/
public com.google.protobuf.AnyOrBuilder getCustomLeftChildTestOrBuilder() {
if (leftChildTestCase_ == 5) {
return (com.google.protobuf.Any) leftChildTest_;
}
return com.google.protobuf.Any.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (leftChildId_ != null) {
output.writeMessage(1, getLeftChildId());
}
if (rightChildId_ != null) {
output.writeMessage(2, getRightChildId());
}
if (defaultDirection_ != tensorflow.decision_trees.GenericTreeModel.BinaryNode.Direction.LEFT.getNumber()) {
output.writeEnum(3, defaultDirection_);
}
if (leftChildTestCase_ == 4) {
output.writeMessage(4, (tensorflow.decision_trees.GenericTreeModel.InequalityTest) leftChildTest_);
}
if (leftChildTestCase_ == 5) {
output.writeMessage(5, (com.google.protobuf.Any) leftChildTest_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (leftChildId_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getLeftChildId());
}
if (rightChildId_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getRightChildId());
}
if (defaultDirection_ != tensorflow.decision_trees.GenericTreeModel.BinaryNode.Direction.LEFT.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, defaultDirection_);
}
if (leftChildTestCase_ == 4) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, (tensorflow.decision_trees.GenericTreeModel.InequalityTest) leftChildTest_);
}
if (leftChildTestCase_ == 5) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, (com.google.protobuf.Any) leftChildTest_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.decision_trees.GenericTreeModel.BinaryNode)) {
return super.equals(obj);
}
tensorflow.decision_trees.GenericTreeModel.BinaryNode other = (tensorflow.decision_trees.GenericTreeModel.BinaryNode) obj;
boolean result = true;
result = result && (hasLeftChildId() == other.hasLeftChildId());
if (hasLeftChildId()) {
result = result && getLeftChildId()
.equals(other.getLeftChildId());
}
result = result && (hasRightChildId() == other.hasRightChildId());
if (hasRightChildId()) {
result = result && getRightChildId()
.equals(other.getRightChildId());
}
result = result && defaultDirection_ == other.defaultDirection_;
result = result && getLeftChildTestCase().equals(
other.getLeftChildTestCase());
if (!result) return false;
switch (leftChildTestCase_) {
case 4:
result = result && getInequalityLeftChildTest()
.equals(other.getInequalityLeftChildTest());
break;
case 5:
result = result && getCustomLeftChildTest()
.equals(other.getCustomLeftChildTest());
break;
case 0:
default:
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasLeftChildId()) {
hash = (37 * hash) + LEFT_CHILD_ID_FIELD_NUMBER;
hash = (53 * hash) + getLeftChildId().hashCode();
}
if (hasRightChildId()) {
hash = (37 * hash) + RIGHT_CHILD_ID_FIELD_NUMBER;
hash = (53 * hash) + getRightChildId().hashCode();
}
hash = (37 * hash) + DEFAULT_DIRECTION_FIELD_NUMBER;
hash = (53 * hash) + defaultDirection_;
switch (leftChildTestCase_) {
case 4:
hash = (37 * hash) + INEQUALITY_LEFT_CHILD_TEST_FIELD_NUMBER;
hash = (53 * hash) + getInequalityLeftChildTest().hashCode();
break;
case 5:
hash = (37 * hash) + CUSTOM_LEFT_CHILD_TEST_FIELD_NUMBER;
hash = (53 * hash) + getCustomLeftChildTest().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.decision_trees.GenericTreeModel.BinaryNode parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.BinaryNode parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.BinaryNode parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.BinaryNode parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.BinaryNode parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.BinaryNode parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.BinaryNode parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.BinaryNode parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.BinaryNode parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.BinaryNode parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.BinaryNode parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.BinaryNode parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.decision_trees.GenericTreeModel.BinaryNode prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.decision_trees.BinaryNode}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.decision_trees.BinaryNode)
tensorflow.decision_trees.GenericTreeModel.BinaryNodeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_BinaryNode_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_BinaryNode_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.BinaryNode.class, tensorflow.decision_trees.GenericTreeModel.BinaryNode.Builder.class);
}
// Construct using tensorflow.decision_trees.GenericTreeModel.BinaryNode.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (leftChildIdBuilder_ == null) {
leftChildId_ = null;
} else {
leftChildId_ = null;
leftChildIdBuilder_ = null;
}
if (rightChildIdBuilder_ == null) {
rightChildId_ = null;
} else {
rightChildId_ = null;
rightChildIdBuilder_ = null;
}
defaultDirection_ = 0;
leftChildTestCase_ = 0;
leftChildTest_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_BinaryNode_descriptor;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.BinaryNode getDefaultInstanceForType() {
return tensorflow.decision_trees.GenericTreeModel.BinaryNode.getDefaultInstance();
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.BinaryNode build() {
tensorflow.decision_trees.GenericTreeModel.BinaryNode result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.BinaryNode buildPartial() {
tensorflow.decision_trees.GenericTreeModel.BinaryNode result = new tensorflow.decision_trees.GenericTreeModel.BinaryNode(this);
if (leftChildIdBuilder_ == null) {
result.leftChildId_ = leftChildId_;
} else {
result.leftChildId_ = leftChildIdBuilder_.build();
}
if (rightChildIdBuilder_ == null) {
result.rightChildId_ = rightChildId_;
} else {
result.rightChildId_ = rightChildIdBuilder_.build();
}
result.defaultDirection_ = defaultDirection_;
if (leftChildTestCase_ == 4) {
if (inequalityLeftChildTestBuilder_ == null) {
result.leftChildTest_ = leftChildTest_;
} else {
result.leftChildTest_ = inequalityLeftChildTestBuilder_.build();
}
}
if (leftChildTestCase_ == 5) {
if (customLeftChildTestBuilder_ == null) {
result.leftChildTest_ = leftChildTest_;
} else {
result.leftChildTest_ = customLeftChildTestBuilder_.build();
}
}
result.leftChildTestCase_ = leftChildTestCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.decision_trees.GenericTreeModel.BinaryNode) {
return mergeFrom((tensorflow.decision_trees.GenericTreeModel.BinaryNode)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.decision_trees.GenericTreeModel.BinaryNode other) {
if (other == tensorflow.decision_trees.GenericTreeModel.BinaryNode.getDefaultInstance()) return this;
if (other.hasLeftChildId()) {
mergeLeftChildId(other.getLeftChildId());
}
if (other.hasRightChildId()) {
mergeRightChildId(other.getRightChildId());
}
if (other.defaultDirection_ != 0) {
setDefaultDirectionValue(other.getDefaultDirectionValue());
}
switch (other.getLeftChildTestCase()) {
case INEQUALITY_LEFT_CHILD_TEST: {
mergeInequalityLeftChildTest(other.getInequalityLeftChildTest());
break;
}
case CUSTOM_LEFT_CHILD_TEST: {
mergeCustomLeftChildTest(other.getCustomLeftChildTest());
break;
}
case LEFTCHILDTEST_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.decision_trees.GenericTreeModel.BinaryNode parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.decision_trees.GenericTreeModel.BinaryNode) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int leftChildTestCase_ = 0;
private java.lang.Object leftChildTest_;
public LeftChildTestCase
getLeftChildTestCase() {
return LeftChildTestCase.forNumber(
leftChildTestCase_);
}
public Builder clearLeftChildTest() {
leftChildTestCase_ = 0;
leftChildTest_ = null;
onChanged();
return this;
}
private com.google.protobuf.Int32Value leftChildId_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int32Value, com.google.protobuf.Int32Value.Builder, com.google.protobuf.Int32ValueOrBuilder> leftChildIdBuilder_;
/**
* .google.protobuf.Int32Value left_child_id = 1;
*/
public boolean hasLeftChildId() {
return leftChildIdBuilder_ != null || leftChildId_ != null;
}
/**
* .google.protobuf.Int32Value left_child_id = 1;
*/
public com.google.protobuf.Int32Value getLeftChildId() {
if (leftChildIdBuilder_ == null) {
return leftChildId_ == null ? com.google.protobuf.Int32Value.getDefaultInstance() : leftChildId_;
} else {
return leftChildIdBuilder_.getMessage();
}
}
/**
* .google.protobuf.Int32Value left_child_id = 1;
*/
public Builder setLeftChildId(com.google.protobuf.Int32Value value) {
if (leftChildIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
leftChildId_ = value;
onChanged();
} else {
leftChildIdBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.Int32Value left_child_id = 1;
*/
public Builder setLeftChildId(
com.google.protobuf.Int32Value.Builder builderForValue) {
if (leftChildIdBuilder_ == null) {
leftChildId_ = builderForValue.build();
onChanged();
} else {
leftChildIdBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.Int32Value left_child_id = 1;
*/
public Builder mergeLeftChildId(com.google.protobuf.Int32Value value) {
if (leftChildIdBuilder_ == null) {
if (leftChildId_ != null) {
leftChildId_ =
com.google.protobuf.Int32Value.newBuilder(leftChildId_).mergeFrom(value).buildPartial();
} else {
leftChildId_ = value;
}
onChanged();
} else {
leftChildIdBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.Int32Value left_child_id = 1;
*/
public Builder clearLeftChildId() {
if (leftChildIdBuilder_ == null) {
leftChildId_ = null;
onChanged();
} else {
leftChildId_ = null;
leftChildIdBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.Int32Value left_child_id = 1;
*/
public com.google.protobuf.Int32Value.Builder getLeftChildIdBuilder() {
onChanged();
return getLeftChildIdFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Int32Value left_child_id = 1;
*/
public com.google.protobuf.Int32ValueOrBuilder getLeftChildIdOrBuilder() {
if (leftChildIdBuilder_ != null) {
return leftChildIdBuilder_.getMessageOrBuilder();
} else {
return leftChildId_ == null ?
com.google.protobuf.Int32Value.getDefaultInstance() : leftChildId_;
}
}
/**
* .google.protobuf.Int32Value left_child_id = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int32Value, com.google.protobuf.Int32Value.Builder, com.google.protobuf.Int32ValueOrBuilder>
getLeftChildIdFieldBuilder() {
if (leftChildIdBuilder_ == null) {
leftChildIdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int32Value, com.google.protobuf.Int32Value.Builder, com.google.protobuf.Int32ValueOrBuilder>(
getLeftChildId(),
getParentForChildren(),
isClean());
leftChildId_ = null;
}
return leftChildIdBuilder_;
}
private com.google.protobuf.Int32Value rightChildId_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int32Value, com.google.protobuf.Int32Value.Builder, com.google.protobuf.Int32ValueOrBuilder> rightChildIdBuilder_;
/**
* .google.protobuf.Int32Value right_child_id = 2;
*/
public boolean hasRightChildId() {
return rightChildIdBuilder_ != null || rightChildId_ != null;
}
/**
* .google.protobuf.Int32Value right_child_id = 2;
*/
public com.google.protobuf.Int32Value getRightChildId() {
if (rightChildIdBuilder_ == null) {
return rightChildId_ == null ? com.google.protobuf.Int32Value.getDefaultInstance() : rightChildId_;
} else {
return rightChildIdBuilder_.getMessage();
}
}
/**
* .google.protobuf.Int32Value right_child_id = 2;
*/
public Builder setRightChildId(com.google.protobuf.Int32Value value) {
if (rightChildIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
rightChildId_ = value;
onChanged();
} else {
rightChildIdBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.Int32Value right_child_id = 2;
*/
public Builder setRightChildId(
com.google.protobuf.Int32Value.Builder builderForValue) {
if (rightChildIdBuilder_ == null) {
rightChildId_ = builderForValue.build();
onChanged();
} else {
rightChildIdBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.Int32Value right_child_id = 2;
*/
public Builder mergeRightChildId(com.google.protobuf.Int32Value value) {
if (rightChildIdBuilder_ == null) {
if (rightChildId_ != null) {
rightChildId_ =
com.google.protobuf.Int32Value.newBuilder(rightChildId_).mergeFrom(value).buildPartial();
} else {
rightChildId_ = value;
}
onChanged();
} else {
rightChildIdBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.Int32Value right_child_id = 2;
*/
public Builder clearRightChildId() {
if (rightChildIdBuilder_ == null) {
rightChildId_ = null;
onChanged();
} else {
rightChildId_ = null;
rightChildIdBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.Int32Value right_child_id = 2;
*/
public com.google.protobuf.Int32Value.Builder getRightChildIdBuilder() {
onChanged();
return getRightChildIdFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Int32Value right_child_id = 2;
*/
public com.google.protobuf.Int32ValueOrBuilder getRightChildIdOrBuilder() {
if (rightChildIdBuilder_ != null) {
return rightChildIdBuilder_.getMessageOrBuilder();
} else {
return rightChildId_ == null ?
com.google.protobuf.Int32Value.getDefaultInstance() : rightChildId_;
}
}
/**
* .google.protobuf.Int32Value right_child_id = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int32Value, com.google.protobuf.Int32Value.Builder, com.google.protobuf.Int32ValueOrBuilder>
getRightChildIdFieldBuilder() {
if (rightChildIdBuilder_ == null) {
rightChildIdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int32Value, com.google.protobuf.Int32Value.Builder, com.google.protobuf.Int32ValueOrBuilder>(
getRightChildId(),
getParentForChildren(),
isClean());
rightChildId_ = null;
}
return rightChildIdBuilder_;
}
private int defaultDirection_ = 0;
/**
*
* When left_child_test is undefined for a particular datapoint (e.g. because
* it's not defined when feature value is missing), the datapoint should go
* in this direction.
*
*
* .tensorflow.decision_trees.BinaryNode.Direction default_direction = 3;
*/
public int getDefaultDirectionValue() {
return defaultDirection_;
}
/**
*
* When left_child_test is undefined for a particular datapoint (e.g. because
* it's not defined when feature value is missing), the datapoint should go
* in this direction.
*
*
* .tensorflow.decision_trees.BinaryNode.Direction default_direction = 3;
*/
public Builder setDefaultDirectionValue(int value) {
defaultDirection_ = value;
onChanged();
return this;
}
/**
*
* When left_child_test is undefined for a particular datapoint (e.g. because
* it's not defined when feature value is missing), the datapoint should go
* in this direction.
*
*
* .tensorflow.decision_trees.BinaryNode.Direction default_direction = 3;
*/
public tensorflow.decision_trees.GenericTreeModel.BinaryNode.Direction getDefaultDirection() {
@SuppressWarnings("deprecation")
tensorflow.decision_trees.GenericTreeModel.BinaryNode.Direction result = tensorflow.decision_trees.GenericTreeModel.BinaryNode.Direction.valueOf(defaultDirection_);
return result == null ? tensorflow.decision_trees.GenericTreeModel.BinaryNode.Direction.UNRECOGNIZED : result;
}
/**
*
* When left_child_test is undefined for a particular datapoint (e.g. because
* it's not defined when feature value is missing), the datapoint should go
* in this direction.
*
*
* .tensorflow.decision_trees.BinaryNode.Direction default_direction = 3;
*/
public Builder setDefaultDirection(tensorflow.decision_trees.GenericTreeModel.BinaryNode.Direction value) {
if (value == null) {
throw new NullPointerException();
}
defaultDirection_ = value.getNumber();
onChanged();
return this;
}
/**
*
* When left_child_test is undefined for a particular datapoint (e.g. because
* it's not defined when feature value is missing), the datapoint should go
* in this direction.
*
*
* .tensorflow.decision_trees.BinaryNode.Direction default_direction = 3;
*/
public Builder clearDefaultDirection() {
defaultDirection_ = 0;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.InequalityTest, tensorflow.decision_trees.GenericTreeModel.InequalityTest.Builder, tensorflow.decision_trees.GenericTreeModel.InequalityTestOrBuilder> inequalityLeftChildTestBuilder_;
/**
* .tensorflow.decision_trees.InequalityTest inequality_left_child_test = 4;
*/
public boolean hasInequalityLeftChildTest() {
return leftChildTestCase_ == 4;
}
/**
* .tensorflow.decision_trees.InequalityTest inequality_left_child_test = 4;
*/
public tensorflow.decision_trees.GenericTreeModel.InequalityTest getInequalityLeftChildTest() {
if (inequalityLeftChildTestBuilder_ == null) {
if (leftChildTestCase_ == 4) {
return (tensorflow.decision_trees.GenericTreeModel.InequalityTest) leftChildTest_;
}
return tensorflow.decision_trees.GenericTreeModel.InequalityTest.getDefaultInstance();
} else {
if (leftChildTestCase_ == 4) {
return inequalityLeftChildTestBuilder_.getMessage();
}
return tensorflow.decision_trees.GenericTreeModel.InequalityTest.getDefaultInstance();
}
}
/**
* .tensorflow.decision_trees.InequalityTest inequality_left_child_test = 4;
*/
public Builder setInequalityLeftChildTest(tensorflow.decision_trees.GenericTreeModel.InequalityTest value) {
if (inequalityLeftChildTestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
leftChildTest_ = value;
onChanged();
} else {
inequalityLeftChildTestBuilder_.setMessage(value);
}
leftChildTestCase_ = 4;
return this;
}
/**
* .tensorflow.decision_trees.InequalityTest inequality_left_child_test = 4;
*/
public Builder setInequalityLeftChildTest(
tensorflow.decision_trees.GenericTreeModel.InequalityTest.Builder builderForValue) {
if (inequalityLeftChildTestBuilder_ == null) {
leftChildTest_ = builderForValue.build();
onChanged();
} else {
inequalityLeftChildTestBuilder_.setMessage(builderForValue.build());
}
leftChildTestCase_ = 4;
return this;
}
/**
* .tensorflow.decision_trees.InequalityTest inequality_left_child_test = 4;
*/
public Builder mergeInequalityLeftChildTest(tensorflow.decision_trees.GenericTreeModel.InequalityTest value) {
if (inequalityLeftChildTestBuilder_ == null) {
if (leftChildTestCase_ == 4 &&
leftChildTest_ != tensorflow.decision_trees.GenericTreeModel.InequalityTest.getDefaultInstance()) {
leftChildTest_ = tensorflow.decision_trees.GenericTreeModel.InequalityTest.newBuilder((tensorflow.decision_trees.GenericTreeModel.InequalityTest) leftChildTest_)
.mergeFrom(value).buildPartial();
} else {
leftChildTest_ = value;
}
onChanged();
} else {
if (leftChildTestCase_ == 4) {
inequalityLeftChildTestBuilder_.mergeFrom(value);
}
inequalityLeftChildTestBuilder_.setMessage(value);
}
leftChildTestCase_ = 4;
return this;
}
/**
* .tensorflow.decision_trees.InequalityTest inequality_left_child_test = 4;
*/
public Builder clearInequalityLeftChildTest() {
if (inequalityLeftChildTestBuilder_ == null) {
if (leftChildTestCase_ == 4) {
leftChildTestCase_ = 0;
leftChildTest_ = null;
onChanged();
}
} else {
if (leftChildTestCase_ == 4) {
leftChildTestCase_ = 0;
leftChildTest_ = null;
}
inequalityLeftChildTestBuilder_.clear();
}
return this;
}
/**
* .tensorflow.decision_trees.InequalityTest inequality_left_child_test = 4;
*/
public tensorflow.decision_trees.GenericTreeModel.InequalityTest.Builder getInequalityLeftChildTestBuilder() {
return getInequalityLeftChildTestFieldBuilder().getBuilder();
}
/**
* .tensorflow.decision_trees.InequalityTest inequality_left_child_test = 4;
*/
public tensorflow.decision_trees.GenericTreeModel.InequalityTestOrBuilder getInequalityLeftChildTestOrBuilder() {
if ((leftChildTestCase_ == 4) && (inequalityLeftChildTestBuilder_ != null)) {
return inequalityLeftChildTestBuilder_.getMessageOrBuilder();
} else {
if (leftChildTestCase_ == 4) {
return (tensorflow.decision_trees.GenericTreeModel.InequalityTest) leftChildTest_;
}
return tensorflow.decision_trees.GenericTreeModel.InequalityTest.getDefaultInstance();
}
}
/**
* .tensorflow.decision_trees.InequalityTest inequality_left_child_test = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.InequalityTest, tensorflow.decision_trees.GenericTreeModel.InequalityTest.Builder, tensorflow.decision_trees.GenericTreeModel.InequalityTestOrBuilder>
getInequalityLeftChildTestFieldBuilder() {
if (inequalityLeftChildTestBuilder_ == null) {
if (!(leftChildTestCase_ == 4)) {
leftChildTest_ = tensorflow.decision_trees.GenericTreeModel.InequalityTest.getDefaultInstance();
}
inequalityLeftChildTestBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.InequalityTest, tensorflow.decision_trees.GenericTreeModel.InequalityTest.Builder, tensorflow.decision_trees.GenericTreeModel.InequalityTestOrBuilder>(
(tensorflow.decision_trees.GenericTreeModel.InequalityTest) leftChildTest_,
getParentForChildren(),
isClean());
leftChildTest_ = null;
}
leftChildTestCase_ = 4;
onChanged();;
return inequalityLeftChildTestBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> customLeftChildTestBuilder_;
/**
* .google.protobuf.Any custom_left_child_test = 5;
*/
public boolean hasCustomLeftChildTest() {
return leftChildTestCase_ == 5;
}
/**
* .google.protobuf.Any custom_left_child_test = 5;
*/
public com.google.protobuf.Any getCustomLeftChildTest() {
if (customLeftChildTestBuilder_ == null) {
if (leftChildTestCase_ == 5) {
return (com.google.protobuf.Any) leftChildTest_;
}
return com.google.protobuf.Any.getDefaultInstance();
} else {
if (leftChildTestCase_ == 5) {
return customLeftChildTestBuilder_.getMessage();
}
return com.google.protobuf.Any.getDefaultInstance();
}
}
/**
* .google.protobuf.Any custom_left_child_test = 5;
*/
public Builder setCustomLeftChildTest(com.google.protobuf.Any value) {
if (customLeftChildTestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
leftChildTest_ = value;
onChanged();
} else {
customLeftChildTestBuilder_.setMessage(value);
}
leftChildTestCase_ = 5;
return this;
}
/**
* .google.protobuf.Any custom_left_child_test = 5;
*/
public Builder setCustomLeftChildTest(
com.google.protobuf.Any.Builder builderForValue) {
if (customLeftChildTestBuilder_ == null) {
leftChildTest_ = builderForValue.build();
onChanged();
} else {
customLeftChildTestBuilder_.setMessage(builderForValue.build());
}
leftChildTestCase_ = 5;
return this;
}
/**
* .google.protobuf.Any custom_left_child_test = 5;
*/
public Builder mergeCustomLeftChildTest(com.google.protobuf.Any value) {
if (customLeftChildTestBuilder_ == null) {
if (leftChildTestCase_ == 5 &&
leftChildTest_ != com.google.protobuf.Any.getDefaultInstance()) {
leftChildTest_ = com.google.protobuf.Any.newBuilder((com.google.protobuf.Any) leftChildTest_)
.mergeFrom(value).buildPartial();
} else {
leftChildTest_ = value;
}
onChanged();
} else {
if (leftChildTestCase_ == 5) {
customLeftChildTestBuilder_.mergeFrom(value);
}
customLeftChildTestBuilder_.setMessage(value);
}
leftChildTestCase_ = 5;
return this;
}
/**
* .google.protobuf.Any custom_left_child_test = 5;
*/
public Builder clearCustomLeftChildTest() {
if (customLeftChildTestBuilder_ == null) {
if (leftChildTestCase_ == 5) {
leftChildTestCase_ = 0;
leftChildTest_ = null;
onChanged();
}
} else {
if (leftChildTestCase_ == 5) {
leftChildTestCase_ = 0;
leftChildTest_ = null;
}
customLeftChildTestBuilder_.clear();
}
return this;
}
/**
* .google.protobuf.Any custom_left_child_test = 5;
*/
public com.google.protobuf.Any.Builder getCustomLeftChildTestBuilder() {
return getCustomLeftChildTestFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Any custom_left_child_test = 5;
*/
public com.google.protobuf.AnyOrBuilder getCustomLeftChildTestOrBuilder() {
if ((leftChildTestCase_ == 5) && (customLeftChildTestBuilder_ != null)) {
return customLeftChildTestBuilder_.getMessageOrBuilder();
} else {
if (leftChildTestCase_ == 5) {
return (com.google.protobuf.Any) leftChildTest_;
}
return com.google.protobuf.Any.getDefaultInstance();
}
}
/**
* .google.protobuf.Any custom_left_child_test = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getCustomLeftChildTestFieldBuilder() {
if (customLeftChildTestBuilder_ == null) {
if (!(leftChildTestCase_ == 5)) {
leftChildTest_ = com.google.protobuf.Any.getDefaultInstance();
}
customLeftChildTestBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
(com.google.protobuf.Any) leftChildTest_,
getParentForChildren(),
isClean());
leftChildTest_ = null;
}
leftChildTestCase_ = 5;
onChanged();;
return customLeftChildTestBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.decision_trees.BinaryNode)
}
// @@protoc_insertion_point(class_scope:tensorflow.decision_trees.BinaryNode)
private static final tensorflow.decision_trees.GenericTreeModel.BinaryNode DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.decision_trees.GenericTreeModel.BinaryNode();
}
public static tensorflow.decision_trees.GenericTreeModel.BinaryNode getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public BinaryNode parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BinaryNode(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.BinaryNode getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SparseVectorOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.decision_trees.SparseVector)
com.google.protobuf.MessageOrBuilder {
/**
* map<int64, .tensorflow.decision_trees.Value> sparse_value = 1;
*/
int getSparseValueCount();
/**
* map<int64, .tensorflow.decision_trees.Value> sparse_value = 1;
*/
boolean containsSparseValue(
long key);
/**
* Use {@link #getSparseValueMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getSparseValue();
/**
* map<int64, .tensorflow.decision_trees.Value> sparse_value = 1;
*/
java.util.Map
getSparseValueMap();
/**
* map<int64, .tensorflow.decision_trees.Value> sparse_value = 1;
*/
tensorflow.decision_trees.GenericTreeModel.Value getSparseValueOrDefault(
long key,
tensorflow.decision_trees.GenericTreeModel.Value defaultValue);
/**
* map<int64, .tensorflow.decision_trees.Value> sparse_value = 1;
*/
tensorflow.decision_trees.GenericTreeModel.Value getSparseValueOrThrow(
long key);
}
/**
*
* A SparseVector represents a vector in which only certain select elements
* are non-zero. Maps labels to values (e.g. class id to probability or count).
*
*
* Protobuf type {@code tensorflow.decision_trees.SparseVector}
*/
public static final class SparseVector extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.decision_trees.SparseVector)
SparseVectorOrBuilder {
private static final long serialVersionUID = 0L;
// Use SparseVector.newBuilder() to construct.
private SparseVector(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SparseVector() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SparseVector(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
sparseValue_ = com.google.protobuf.MapField.newMapField(
SparseValueDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000001;
}
com.google.protobuf.MapEntry
sparseValue__ = input.readMessage(
SparseValueDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
sparseValue_.getMutableMap().put(
sparseValue__.getKey(), sparseValue__.getValue());
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_SparseVector_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 1:
return internalGetSparseValue();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_SparseVector_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.SparseVector.class, tensorflow.decision_trees.GenericTreeModel.SparseVector.Builder.class);
}
public static final int SPARSE_VALUE_FIELD_NUMBER = 1;
private static final class SparseValueDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.Long, tensorflow.decision_trees.GenericTreeModel.Value> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_SparseVector_SparseValueEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.INT64,
0L,
com.google.protobuf.WireFormat.FieldType.MESSAGE,
tensorflow.decision_trees.GenericTreeModel.Value.getDefaultInstance());
}
private com.google.protobuf.MapField<
java.lang.Long, tensorflow.decision_trees.GenericTreeModel.Value> sparseValue_;
private com.google.protobuf.MapField
internalGetSparseValue() {
if (sparseValue_ == null) {
return com.google.protobuf.MapField.emptyMapField(
SparseValueDefaultEntryHolder.defaultEntry);
}
return sparseValue_;
}
public int getSparseValueCount() {
return internalGetSparseValue().getMap().size();
}
/**
* map<int64, .tensorflow.decision_trees.Value> sparse_value = 1;
*/
public boolean containsSparseValue(
long key) {
return internalGetSparseValue().getMap().containsKey(key);
}
/**
* Use {@link #getSparseValueMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getSparseValue() {
return getSparseValueMap();
}
/**
* map<int64, .tensorflow.decision_trees.Value> sparse_value = 1;
*/
public java.util.Map getSparseValueMap() {
return internalGetSparseValue().getMap();
}
/**
* map<int64, .tensorflow.decision_trees.Value> sparse_value = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.Value getSparseValueOrDefault(
long key,
tensorflow.decision_trees.GenericTreeModel.Value defaultValue) {
java.util.Map map =
internalGetSparseValue().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<int64, .tensorflow.decision_trees.Value> sparse_value = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.Value getSparseValueOrThrow(
long key) {
java.util.Map map =
internalGetSparseValue().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
com.google.protobuf.GeneratedMessageV3
.serializeLongMapTo(
output,
internalGetSparseValue(),
SparseValueDefaultEntryHolder.defaultEntry,
1);
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (java.util.Map.Entry entry
: internalGetSparseValue().getMap().entrySet()) {
com.google.protobuf.MapEntry
sparseValue__ = SparseValueDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, sparseValue__);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.decision_trees.GenericTreeModel.SparseVector)) {
return super.equals(obj);
}
tensorflow.decision_trees.GenericTreeModel.SparseVector other = (tensorflow.decision_trees.GenericTreeModel.SparseVector) obj;
boolean result = true;
result = result && internalGetSparseValue().equals(
other.internalGetSparseValue());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (!internalGetSparseValue().getMap().isEmpty()) {
hash = (37 * hash) + SPARSE_VALUE_FIELD_NUMBER;
hash = (53 * hash) + internalGetSparseValue().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.decision_trees.GenericTreeModel.SparseVector parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.SparseVector parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.SparseVector parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.SparseVector parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.SparseVector parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.SparseVector parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.SparseVector parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.SparseVector parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.SparseVector parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.SparseVector parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.SparseVector parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.SparseVector parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.decision_trees.GenericTreeModel.SparseVector prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A SparseVector represents a vector in which only certain select elements
* are non-zero. Maps labels to values (e.g. class id to probability or count).
*
*
* Protobuf type {@code tensorflow.decision_trees.SparseVector}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.decision_trees.SparseVector)
tensorflow.decision_trees.GenericTreeModel.SparseVectorOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_SparseVector_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 1:
return internalGetSparseValue();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 1:
return internalGetMutableSparseValue();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_SparseVector_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.SparseVector.class, tensorflow.decision_trees.GenericTreeModel.SparseVector.Builder.class);
}
// Construct using tensorflow.decision_trees.GenericTreeModel.SparseVector.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
internalGetMutableSparseValue().clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_SparseVector_descriptor;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.SparseVector getDefaultInstanceForType() {
return tensorflow.decision_trees.GenericTreeModel.SparseVector.getDefaultInstance();
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.SparseVector build() {
tensorflow.decision_trees.GenericTreeModel.SparseVector result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.SparseVector buildPartial() {
tensorflow.decision_trees.GenericTreeModel.SparseVector result = new tensorflow.decision_trees.GenericTreeModel.SparseVector(this);
int from_bitField0_ = bitField0_;
result.sparseValue_ = internalGetSparseValue();
result.sparseValue_.makeImmutable();
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.decision_trees.GenericTreeModel.SparseVector) {
return mergeFrom((tensorflow.decision_trees.GenericTreeModel.SparseVector)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.decision_trees.GenericTreeModel.SparseVector other) {
if (other == tensorflow.decision_trees.GenericTreeModel.SparseVector.getDefaultInstance()) return this;
internalGetMutableSparseValue().mergeFrom(
other.internalGetSparseValue());
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.decision_trees.GenericTreeModel.SparseVector parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.decision_trees.GenericTreeModel.SparseVector) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.MapField<
java.lang.Long, tensorflow.decision_trees.GenericTreeModel.Value> sparseValue_;
private com.google.protobuf.MapField
internalGetSparseValue() {
if (sparseValue_ == null) {
return com.google.protobuf.MapField.emptyMapField(
SparseValueDefaultEntryHolder.defaultEntry);
}
return sparseValue_;
}
private com.google.protobuf.MapField
internalGetMutableSparseValue() {
onChanged();;
if (sparseValue_ == null) {
sparseValue_ = com.google.protobuf.MapField.newMapField(
SparseValueDefaultEntryHolder.defaultEntry);
}
if (!sparseValue_.isMutable()) {
sparseValue_ = sparseValue_.copy();
}
return sparseValue_;
}
public int getSparseValueCount() {
return internalGetSparseValue().getMap().size();
}
/**
* map<int64, .tensorflow.decision_trees.Value> sparse_value = 1;
*/
public boolean containsSparseValue(
long key) {
return internalGetSparseValue().getMap().containsKey(key);
}
/**
* Use {@link #getSparseValueMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getSparseValue() {
return getSparseValueMap();
}
/**
* map<int64, .tensorflow.decision_trees.Value> sparse_value = 1;
*/
public java.util.Map getSparseValueMap() {
return internalGetSparseValue().getMap();
}
/**
* map<int64, .tensorflow.decision_trees.Value> sparse_value = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.Value getSparseValueOrDefault(
long key,
tensorflow.decision_trees.GenericTreeModel.Value defaultValue) {
java.util.Map map =
internalGetSparseValue().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<int64, .tensorflow.decision_trees.Value> sparse_value = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.Value getSparseValueOrThrow(
long key) {
java.util.Map map =
internalGetSparseValue().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearSparseValue() {
internalGetMutableSparseValue().getMutableMap()
.clear();
return this;
}
/**
* map<int64, .tensorflow.decision_trees.Value> sparse_value = 1;
*/
public Builder removeSparseValue(
long key) {
internalGetMutableSparseValue().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableSparseValue() {
return internalGetMutableSparseValue().getMutableMap();
}
/**
* map<int64, .tensorflow.decision_trees.Value> sparse_value = 1;
*/
public Builder putSparseValue(
long key,
tensorflow.decision_trees.GenericTreeModel.Value value) {
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutableSparseValue().getMutableMap()
.put(key, value);
return this;
}
/**
* map<int64, .tensorflow.decision_trees.Value> sparse_value = 1;
*/
public Builder putAllSparseValue(
java.util.Map values) {
internalGetMutableSparseValue().getMutableMap()
.putAll(values);
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.decision_trees.SparseVector)
}
// @@protoc_insertion_point(class_scope:tensorflow.decision_trees.SparseVector)
private static final tensorflow.decision_trees.GenericTreeModel.SparseVector DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.decision_trees.GenericTreeModel.SparseVector();
}
public static tensorflow.decision_trees.GenericTreeModel.SparseVector getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SparseVector parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SparseVector(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.SparseVector getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface VectorOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.decision_trees.Vector)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
java.util.List
getValueList();
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
tensorflow.decision_trees.GenericTreeModel.Value getValue(int index);
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
int getValueCount();
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
java.util.List extends tensorflow.decision_trees.GenericTreeModel.ValueOrBuilder>
getValueOrBuilderList();
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
tensorflow.decision_trees.GenericTreeModel.ValueOrBuilder getValueOrBuilder(
int index);
}
/**
* Protobuf type {@code tensorflow.decision_trees.Vector}
*/
public static final class Vector extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.decision_trees.Vector)
VectorOrBuilder {
private static final long serialVersionUID = 0L;
// Use Vector.newBuilder() to construct.
private Vector(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Vector() {
value_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Vector(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
value_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
value_.add(
input.readMessage(tensorflow.decision_trees.GenericTreeModel.Value.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
value_ = java.util.Collections.unmodifiableList(value_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Vector_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Vector_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.Vector.class, tensorflow.decision_trees.GenericTreeModel.Vector.Builder.class);
}
public static final int VALUE_FIELD_NUMBER = 1;
private java.util.List value_;
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
public java.util.List getValueList() {
return value_;
}
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
public java.util.List extends tensorflow.decision_trees.GenericTreeModel.ValueOrBuilder>
getValueOrBuilderList() {
return value_;
}
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
public int getValueCount() {
return value_.size();
}
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.Value getValue(int index) {
return value_.get(index);
}
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.ValueOrBuilder getValueOrBuilder(
int index) {
return value_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < value_.size(); i++) {
output.writeMessage(1, value_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < value_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, value_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.decision_trees.GenericTreeModel.Vector)) {
return super.equals(obj);
}
tensorflow.decision_trees.GenericTreeModel.Vector other = (tensorflow.decision_trees.GenericTreeModel.Vector) obj;
boolean result = true;
result = result && getValueList()
.equals(other.getValueList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getValueCount() > 0) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValueList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.decision_trees.GenericTreeModel.Vector parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.Vector parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Vector parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.Vector parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Vector parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.Vector parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Vector parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.Vector parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Vector parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.Vector parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Vector parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.Vector parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.decision_trees.GenericTreeModel.Vector prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.decision_trees.Vector}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.decision_trees.Vector)
tensorflow.decision_trees.GenericTreeModel.VectorOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Vector_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Vector_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.Vector.class, tensorflow.decision_trees.GenericTreeModel.Vector.Builder.class);
}
// Construct using tensorflow.decision_trees.GenericTreeModel.Vector.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getValueFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (valueBuilder_ == null) {
value_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
valueBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Vector_descriptor;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Vector getDefaultInstanceForType() {
return tensorflow.decision_trees.GenericTreeModel.Vector.getDefaultInstance();
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Vector build() {
tensorflow.decision_trees.GenericTreeModel.Vector result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Vector buildPartial() {
tensorflow.decision_trees.GenericTreeModel.Vector result = new tensorflow.decision_trees.GenericTreeModel.Vector(this);
int from_bitField0_ = bitField0_;
if (valueBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
value_ = java.util.Collections.unmodifiableList(value_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.value_ = value_;
} else {
result.value_ = valueBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.decision_trees.GenericTreeModel.Vector) {
return mergeFrom((tensorflow.decision_trees.GenericTreeModel.Vector)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.decision_trees.GenericTreeModel.Vector other) {
if (other == tensorflow.decision_trees.GenericTreeModel.Vector.getDefaultInstance()) return this;
if (valueBuilder_ == null) {
if (!other.value_.isEmpty()) {
if (value_.isEmpty()) {
value_ = other.value_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureValueIsMutable();
value_.addAll(other.value_);
}
onChanged();
}
} else {
if (!other.value_.isEmpty()) {
if (valueBuilder_.isEmpty()) {
valueBuilder_.dispose();
valueBuilder_ = null;
value_ = other.value_;
bitField0_ = (bitField0_ & ~0x00000001);
valueBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getValueFieldBuilder() : null;
} else {
valueBuilder_.addAllMessages(other.value_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.decision_trees.GenericTreeModel.Vector parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.decision_trees.GenericTreeModel.Vector) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List value_ =
java.util.Collections.emptyList();
private void ensureValueIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
value_ = new java.util.ArrayList(value_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Value, tensorflow.decision_trees.GenericTreeModel.Value.Builder, tensorflow.decision_trees.GenericTreeModel.ValueOrBuilder> valueBuilder_;
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
public java.util.List getValueList() {
if (valueBuilder_ == null) {
return java.util.Collections.unmodifiableList(value_);
} else {
return valueBuilder_.getMessageList();
}
}
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
public int getValueCount() {
if (valueBuilder_ == null) {
return value_.size();
} else {
return valueBuilder_.getCount();
}
}
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.Value getValue(int index) {
if (valueBuilder_ == null) {
return value_.get(index);
} else {
return valueBuilder_.getMessage(index);
}
}
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
public Builder setValue(
int index, tensorflow.decision_trees.GenericTreeModel.Value value) {
if (valueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValueIsMutable();
value_.set(index, value);
onChanged();
} else {
valueBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
public Builder setValue(
int index, tensorflow.decision_trees.GenericTreeModel.Value.Builder builderForValue) {
if (valueBuilder_ == null) {
ensureValueIsMutable();
value_.set(index, builderForValue.build());
onChanged();
} else {
valueBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
public Builder addValue(tensorflow.decision_trees.GenericTreeModel.Value value) {
if (valueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValueIsMutable();
value_.add(value);
onChanged();
} else {
valueBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
public Builder addValue(
int index, tensorflow.decision_trees.GenericTreeModel.Value value) {
if (valueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValueIsMutable();
value_.add(index, value);
onChanged();
} else {
valueBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
public Builder addValue(
tensorflow.decision_trees.GenericTreeModel.Value.Builder builderForValue) {
if (valueBuilder_ == null) {
ensureValueIsMutable();
value_.add(builderForValue.build());
onChanged();
} else {
valueBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
public Builder addValue(
int index, tensorflow.decision_trees.GenericTreeModel.Value.Builder builderForValue) {
if (valueBuilder_ == null) {
ensureValueIsMutable();
value_.add(index, builderForValue.build());
onChanged();
} else {
valueBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
public Builder addAllValue(
java.lang.Iterable extends tensorflow.decision_trees.GenericTreeModel.Value> values) {
if (valueBuilder_ == null) {
ensureValueIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, value_);
onChanged();
} else {
valueBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
public Builder clearValue() {
if (valueBuilder_ == null) {
value_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
valueBuilder_.clear();
}
return this;
}
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
public Builder removeValue(int index) {
if (valueBuilder_ == null) {
ensureValueIsMutable();
value_.remove(index);
onChanged();
} else {
valueBuilder_.remove(index);
}
return this;
}
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.Value.Builder getValueBuilder(
int index) {
return getValueFieldBuilder().getBuilder(index);
}
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.ValueOrBuilder getValueOrBuilder(
int index) {
if (valueBuilder_ == null) {
return value_.get(index); } else {
return valueBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
public java.util.List extends tensorflow.decision_trees.GenericTreeModel.ValueOrBuilder>
getValueOrBuilderList() {
if (valueBuilder_ != null) {
return valueBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(value_);
}
}
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.Value.Builder addValueBuilder() {
return getValueFieldBuilder().addBuilder(
tensorflow.decision_trees.GenericTreeModel.Value.getDefaultInstance());
}
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.Value.Builder addValueBuilder(
int index) {
return getValueFieldBuilder().addBuilder(
index, tensorflow.decision_trees.GenericTreeModel.Value.getDefaultInstance());
}
/**
* repeated .tensorflow.decision_trees.Value value = 1;
*/
public java.util.List
getValueBuilderList() {
return getValueFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Value, tensorflow.decision_trees.GenericTreeModel.Value.Builder, tensorflow.decision_trees.GenericTreeModel.ValueOrBuilder>
getValueFieldBuilder() {
if (valueBuilder_ == null) {
valueBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Value, tensorflow.decision_trees.GenericTreeModel.Value.Builder, tensorflow.decision_trees.GenericTreeModel.ValueOrBuilder>(
value_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
value_ = null;
}
return valueBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.decision_trees.Vector)
}
// @@protoc_insertion_point(class_scope:tensorflow.decision_trees.Vector)
private static final tensorflow.decision_trees.GenericTreeModel.Vector DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.decision_trees.GenericTreeModel.Vector();
}
public static tensorflow.decision_trees.GenericTreeModel.Vector getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Vector parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Vector(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Vector getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LeafOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.decision_trees.Leaf)
com.google.protobuf.MessageOrBuilder {
/**
*
* The interpretation of the values held in the leaves of a decision tree
* is application specific, but some common cases are:
* 1) len(vector) = 1, and the floating point value[0] holds the class 0
* probability in a two class classification problem.
* 2) len(vector) = 1, and the integer value[0] holds the class prediction.
* 3) The floating point value[i] holds the class i probability prediction.
* 4) The floating point value[i] holds the i-th component of the
* vector prediction in a regression problem.
* 5) sparse_vector holds the sparse class predictions for a classification
* problem with a large number of classes.
*
*
* .tensorflow.decision_trees.Vector vector = 1;
*/
boolean hasVector();
/**
*
* The interpretation of the values held in the leaves of a decision tree
* is application specific, but some common cases are:
* 1) len(vector) = 1, and the floating point value[0] holds the class 0
* probability in a two class classification problem.
* 2) len(vector) = 1, and the integer value[0] holds the class prediction.
* 3) The floating point value[i] holds the class i probability prediction.
* 4) The floating point value[i] holds the i-th component of the
* vector prediction in a regression problem.
* 5) sparse_vector holds the sparse class predictions for a classification
* problem with a large number of classes.
*
*
* .tensorflow.decision_trees.Vector vector = 1;
*/
tensorflow.decision_trees.GenericTreeModel.Vector getVector();
/**
*
* The interpretation of the values held in the leaves of a decision tree
* is application specific, but some common cases are:
* 1) len(vector) = 1, and the floating point value[0] holds the class 0
* probability in a two class classification problem.
* 2) len(vector) = 1, and the integer value[0] holds the class prediction.
* 3) The floating point value[i] holds the class i probability prediction.
* 4) The floating point value[i] holds the i-th component of the
* vector prediction in a regression problem.
* 5) sparse_vector holds the sparse class predictions for a classification
* problem with a large number of classes.
*
*
* .tensorflow.decision_trees.Vector vector = 1;
*/
tensorflow.decision_trees.GenericTreeModel.VectorOrBuilder getVectorOrBuilder();
/**
* .tensorflow.decision_trees.SparseVector sparse_vector = 2;
*/
boolean hasSparseVector();
/**
* .tensorflow.decision_trees.SparseVector sparse_vector = 2;
*/
tensorflow.decision_trees.GenericTreeModel.SparseVector getSparseVector();
/**
* .tensorflow.decision_trees.SparseVector sparse_vector = 2;
*/
tensorflow.decision_trees.GenericTreeModel.SparseVectorOrBuilder getSparseVectorOrBuilder();
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
java.util.List
getAdditionalDataList();
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
com.google.protobuf.Any getAdditionalData(int index);
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
int getAdditionalDataCount();
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList();
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index);
public tensorflow.decision_trees.GenericTreeModel.Leaf.LeafCase getLeafCase();
}
/**
* Protobuf type {@code tensorflow.decision_trees.Leaf}
*/
public static final class Leaf extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.decision_trees.Leaf)
LeafOrBuilder {
private static final long serialVersionUID = 0L;
// Use Leaf.newBuilder() to construct.
private Leaf(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Leaf() {
additionalData_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Leaf(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
tensorflow.decision_trees.GenericTreeModel.Vector.Builder subBuilder = null;
if (leafCase_ == 1) {
subBuilder = ((tensorflow.decision_trees.GenericTreeModel.Vector) leaf_).toBuilder();
}
leaf_ =
input.readMessage(tensorflow.decision_trees.GenericTreeModel.Vector.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((tensorflow.decision_trees.GenericTreeModel.Vector) leaf_);
leaf_ = subBuilder.buildPartial();
}
leafCase_ = 1;
break;
}
case 18: {
tensorflow.decision_trees.GenericTreeModel.SparseVector.Builder subBuilder = null;
if (leafCase_ == 2) {
subBuilder = ((tensorflow.decision_trees.GenericTreeModel.SparseVector) leaf_).toBuilder();
}
leaf_ =
input.readMessage(tensorflow.decision_trees.GenericTreeModel.SparseVector.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((tensorflow.decision_trees.GenericTreeModel.SparseVector) leaf_);
leaf_ = subBuilder.buildPartial();
}
leafCase_ = 2;
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
additionalData_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
additionalData_.add(
input.readMessage(com.google.protobuf.Any.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
additionalData_ = java.util.Collections.unmodifiableList(additionalData_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Leaf_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Leaf_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.Leaf.class, tensorflow.decision_trees.GenericTreeModel.Leaf.Builder.class);
}
private int bitField0_;
private int leafCase_ = 0;
private java.lang.Object leaf_;
public enum LeafCase
implements com.google.protobuf.Internal.EnumLite {
VECTOR(1),
SPARSE_VECTOR(2),
LEAF_NOT_SET(0);
private final int value;
private LeafCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static LeafCase valueOf(int value) {
return forNumber(value);
}
public static LeafCase forNumber(int value) {
switch (value) {
case 1: return VECTOR;
case 2: return SPARSE_VECTOR;
case 0: return LEAF_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public LeafCase
getLeafCase() {
return LeafCase.forNumber(
leafCase_);
}
public static final int VECTOR_FIELD_NUMBER = 1;
/**
*
* The interpretation of the values held in the leaves of a decision tree
* is application specific, but some common cases are:
* 1) len(vector) = 1, and the floating point value[0] holds the class 0
* probability in a two class classification problem.
* 2) len(vector) = 1, and the integer value[0] holds the class prediction.
* 3) The floating point value[i] holds the class i probability prediction.
* 4) The floating point value[i] holds the i-th component of the
* vector prediction in a regression problem.
* 5) sparse_vector holds the sparse class predictions for a classification
* problem with a large number of classes.
*
*
* .tensorflow.decision_trees.Vector vector = 1;
*/
public boolean hasVector() {
return leafCase_ == 1;
}
/**
*
* The interpretation of the values held in the leaves of a decision tree
* is application specific, but some common cases are:
* 1) len(vector) = 1, and the floating point value[0] holds the class 0
* probability in a two class classification problem.
* 2) len(vector) = 1, and the integer value[0] holds the class prediction.
* 3) The floating point value[i] holds the class i probability prediction.
* 4) The floating point value[i] holds the i-th component of the
* vector prediction in a regression problem.
* 5) sparse_vector holds the sparse class predictions for a classification
* problem with a large number of classes.
*
*
* .tensorflow.decision_trees.Vector vector = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.Vector getVector() {
if (leafCase_ == 1) {
return (tensorflow.decision_trees.GenericTreeModel.Vector) leaf_;
}
return tensorflow.decision_trees.GenericTreeModel.Vector.getDefaultInstance();
}
/**
*
* The interpretation of the values held in the leaves of a decision tree
* is application specific, but some common cases are:
* 1) len(vector) = 1, and the floating point value[0] holds the class 0
* probability in a two class classification problem.
* 2) len(vector) = 1, and the integer value[0] holds the class prediction.
* 3) The floating point value[i] holds the class i probability prediction.
* 4) The floating point value[i] holds the i-th component of the
* vector prediction in a regression problem.
* 5) sparse_vector holds the sparse class predictions for a classification
* problem with a large number of classes.
*
*
* .tensorflow.decision_trees.Vector vector = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.VectorOrBuilder getVectorOrBuilder() {
if (leafCase_ == 1) {
return (tensorflow.decision_trees.GenericTreeModel.Vector) leaf_;
}
return tensorflow.decision_trees.GenericTreeModel.Vector.getDefaultInstance();
}
public static final int SPARSE_VECTOR_FIELD_NUMBER = 2;
/**
* .tensorflow.decision_trees.SparseVector sparse_vector = 2;
*/
public boolean hasSparseVector() {
return leafCase_ == 2;
}
/**
* .tensorflow.decision_trees.SparseVector sparse_vector = 2;
*/
public tensorflow.decision_trees.GenericTreeModel.SparseVector getSparseVector() {
if (leafCase_ == 2) {
return (tensorflow.decision_trees.GenericTreeModel.SparseVector) leaf_;
}
return tensorflow.decision_trees.GenericTreeModel.SparseVector.getDefaultInstance();
}
/**
* .tensorflow.decision_trees.SparseVector sparse_vector = 2;
*/
public tensorflow.decision_trees.GenericTreeModel.SparseVectorOrBuilder getSparseVectorOrBuilder() {
if (leafCase_ == 2) {
return (tensorflow.decision_trees.GenericTreeModel.SparseVector) leaf_;
}
return tensorflow.decision_trees.GenericTreeModel.SparseVector.getDefaultInstance();
}
public static final int ADDITIONAL_DATA_FIELD_NUMBER = 3;
private java.util.List additionalData_;
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
public java.util.List getAdditionalDataList() {
return additionalData_;
}
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
public java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList() {
return additionalData_;
}
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
public int getAdditionalDataCount() {
return additionalData_.size();
}
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
public com.google.protobuf.Any getAdditionalData(int index) {
return additionalData_.get(index);
}
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
public com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index) {
return additionalData_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (leafCase_ == 1) {
output.writeMessage(1, (tensorflow.decision_trees.GenericTreeModel.Vector) leaf_);
}
if (leafCase_ == 2) {
output.writeMessage(2, (tensorflow.decision_trees.GenericTreeModel.SparseVector) leaf_);
}
for (int i = 0; i < additionalData_.size(); i++) {
output.writeMessage(3, additionalData_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (leafCase_ == 1) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, (tensorflow.decision_trees.GenericTreeModel.Vector) leaf_);
}
if (leafCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, (tensorflow.decision_trees.GenericTreeModel.SparseVector) leaf_);
}
for (int i = 0; i < additionalData_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, additionalData_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.decision_trees.GenericTreeModel.Leaf)) {
return super.equals(obj);
}
tensorflow.decision_trees.GenericTreeModel.Leaf other = (tensorflow.decision_trees.GenericTreeModel.Leaf) obj;
boolean result = true;
result = result && getAdditionalDataList()
.equals(other.getAdditionalDataList());
result = result && getLeafCase().equals(
other.getLeafCase());
if (!result) return false;
switch (leafCase_) {
case 1:
result = result && getVector()
.equals(other.getVector());
break;
case 2:
result = result && getSparseVector()
.equals(other.getSparseVector());
break;
case 0:
default:
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getAdditionalDataCount() > 0) {
hash = (37 * hash) + ADDITIONAL_DATA_FIELD_NUMBER;
hash = (53 * hash) + getAdditionalDataList().hashCode();
}
switch (leafCase_) {
case 1:
hash = (37 * hash) + VECTOR_FIELD_NUMBER;
hash = (53 * hash) + getVector().hashCode();
break;
case 2:
hash = (37 * hash) + SPARSE_VECTOR_FIELD_NUMBER;
hash = (53 * hash) + getSparseVector().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.decision_trees.GenericTreeModel.Leaf parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.Leaf parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Leaf parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.Leaf parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Leaf parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.Leaf parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Leaf parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.Leaf parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Leaf parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.Leaf parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.Leaf parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.Leaf parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.decision_trees.GenericTreeModel.Leaf prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.decision_trees.Leaf}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.decision_trees.Leaf)
tensorflow.decision_trees.GenericTreeModel.LeafOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Leaf_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Leaf_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.Leaf.class, tensorflow.decision_trees.GenericTreeModel.Leaf.Builder.class);
}
// Construct using tensorflow.decision_trees.GenericTreeModel.Leaf.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getAdditionalDataFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (additionalDataBuilder_ == null) {
additionalData_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
additionalDataBuilder_.clear();
}
leafCase_ = 0;
leaf_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_Leaf_descriptor;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Leaf getDefaultInstanceForType() {
return tensorflow.decision_trees.GenericTreeModel.Leaf.getDefaultInstance();
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Leaf build() {
tensorflow.decision_trees.GenericTreeModel.Leaf result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Leaf buildPartial() {
tensorflow.decision_trees.GenericTreeModel.Leaf result = new tensorflow.decision_trees.GenericTreeModel.Leaf(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (leafCase_ == 1) {
if (vectorBuilder_ == null) {
result.leaf_ = leaf_;
} else {
result.leaf_ = vectorBuilder_.build();
}
}
if (leafCase_ == 2) {
if (sparseVectorBuilder_ == null) {
result.leaf_ = leaf_;
} else {
result.leaf_ = sparseVectorBuilder_.build();
}
}
if (additionalDataBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004)) {
additionalData_ = java.util.Collections.unmodifiableList(additionalData_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.additionalData_ = additionalData_;
} else {
result.additionalData_ = additionalDataBuilder_.build();
}
result.bitField0_ = to_bitField0_;
result.leafCase_ = leafCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.decision_trees.GenericTreeModel.Leaf) {
return mergeFrom((tensorflow.decision_trees.GenericTreeModel.Leaf)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.decision_trees.GenericTreeModel.Leaf other) {
if (other == tensorflow.decision_trees.GenericTreeModel.Leaf.getDefaultInstance()) return this;
if (additionalDataBuilder_ == null) {
if (!other.additionalData_.isEmpty()) {
if (additionalData_.isEmpty()) {
additionalData_ = other.additionalData_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureAdditionalDataIsMutable();
additionalData_.addAll(other.additionalData_);
}
onChanged();
}
} else {
if (!other.additionalData_.isEmpty()) {
if (additionalDataBuilder_.isEmpty()) {
additionalDataBuilder_.dispose();
additionalDataBuilder_ = null;
additionalData_ = other.additionalData_;
bitField0_ = (bitField0_ & ~0x00000004);
additionalDataBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAdditionalDataFieldBuilder() : null;
} else {
additionalDataBuilder_.addAllMessages(other.additionalData_);
}
}
}
switch (other.getLeafCase()) {
case VECTOR: {
mergeVector(other.getVector());
break;
}
case SPARSE_VECTOR: {
mergeSparseVector(other.getSparseVector());
break;
}
case LEAF_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.decision_trees.GenericTreeModel.Leaf parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.decision_trees.GenericTreeModel.Leaf) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int leafCase_ = 0;
private java.lang.Object leaf_;
public LeafCase
getLeafCase() {
return LeafCase.forNumber(
leafCase_);
}
public Builder clearLeaf() {
leafCase_ = 0;
leaf_ = null;
onChanged();
return this;
}
private int bitField0_;
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Vector, tensorflow.decision_trees.GenericTreeModel.Vector.Builder, tensorflow.decision_trees.GenericTreeModel.VectorOrBuilder> vectorBuilder_;
/**
*
* The interpretation of the values held in the leaves of a decision tree
* is application specific, but some common cases are:
* 1) len(vector) = 1, and the floating point value[0] holds the class 0
* probability in a two class classification problem.
* 2) len(vector) = 1, and the integer value[0] holds the class prediction.
* 3) The floating point value[i] holds the class i probability prediction.
* 4) The floating point value[i] holds the i-th component of the
* vector prediction in a regression problem.
* 5) sparse_vector holds the sparse class predictions for a classification
* problem with a large number of classes.
*
*
* .tensorflow.decision_trees.Vector vector = 1;
*/
public boolean hasVector() {
return leafCase_ == 1;
}
/**
*
* The interpretation of the values held in the leaves of a decision tree
* is application specific, but some common cases are:
* 1) len(vector) = 1, and the floating point value[0] holds the class 0
* probability in a two class classification problem.
* 2) len(vector) = 1, and the integer value[0] holds the class prediction.
* 3) The floating point value[i] holds the class i probability prediction.
* 4) The floating point value[i] holds the i-th component of the
* vector prediction in a regression problem.
* 5) sparse_vector holds the sparse class predictions for a classification
* problem with a large number of classes.
*
*
* .tensorflow.decision_trees.Vector vector = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.Vector getVector() {
if (vectorBuilder_ == null) {
if (leafCase_ == 1) {
return (tensorflow.decision_trees.GenericTreeModel.Vector) leaf_;
}
return tensorflow.decision_trees.GenericTreeModel.Vector.getDefaultInstance();
} else {
if (leafCase_ == 1) {
return vectorBuilder_.getMessage();
}
return tensorflow.decision_trees.GenericTreeModel.Vector.getDefaultInstance();
}
}
/**
*
* The interpretation of the values held in the leaves of a decision tree
* is application specific, but some common cases are:
* 1) len(vector) = 1, and the floating point value[0] holds the class 0
* probability in a two class classification problem.
* 2) len(vector) = 1, and the integer value[0] holds the class prediction.
* 3) The floating point value[i] holds the class i probability prediction.
* 4) The floating point value[i] holds the i-th component of the
* vector prediction in a regression problem.
* 5) sparse_vector holds the sparse class predictions for a classification
* problem with a large number of classes.
*
*
* .tensorflow.decision_trees.Vector vector = 1;
*/
public Builder setVector(tensorflow.decision_trees.GenericTreeModel.Vector value) {
if (vectorBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
leaf_ = value;
onChanged();
} else {
vectorBuilder_.setMessage(value);
}
leafCase_ = 1;
return this;
}
/**
*
* The interpretation of the values held in the leaves of a decision tree
* is application specific, but some common cases are:
* 1) len(vector) = 1, and the floating point value[0] holds the class 0
* probability in a two class classification problem.
* 2) len(vector) = 1, and the integer value[0] holds the class prediction.
* 3) The floating point value[i] holds the class i probability prediction.
* 4) The floating point value[i] holds the i-th component of the
* vector prediction in a regression problem.
* 5) sparse_vector holds the sparse class predictions for a classification
* problem with a large number of classes.
*
*
* .tensorflow.decision_trees.Vector vector = 1;
*/
public Builder setVector(
tensorflow.decision_trees.GenericTreeModel.Vector.Builder builderForValue) {
if (vectorBuilder_ == null) {
leaf_ = builderForValue.build();
onChanged();
} else {
vectorBuilder_.setMessage(builderForValue.build());
}
leafCase_ = 1;
return this;
}
/**
*
* The interpretation of the values held in the leaves of a decision tree
* is application specific, but some common cases are:
* 1) len(vector) = 1, and the floating point value[0] holds the class 0
* probability in a two class classification problem.
* 2) len(vector) = 1, and the integer value[0] holds the class prediction.
* 3) The floating point value[i] holds the class i probability prediction.
* 4) The floating point value[i] holds the i-th component of the
* vector prediction in a regression problem.
* 5) sparse_vector holds the sparse class predictions for a classification
* problem with a large number of classes.
*
*
* .tensorflow.decision_trees.Vector vector = 1;
*/
public Builder mergeVector(tensorflow.decision_trees.GenericTreeModel.Vector value) {
if (vectorBuilder_ == null) {
if (leafCase_ == 1 &&
leaf_ != tensorflow.decision_trees.GenericTreeModel.Vector.getDefaultInstance()) {
leaf_ = tensorflow.decision_trees.GenericTreeModel.Vector.newBuilder((tensorflow.decision_trees.GenericTreeModel.Vector) leaf_)
.mergeFrom(value).buildPartial();
} else {
leaf_ = value;
}
onChanged();
} else {
if (leafCase_ == 1) {
vectorBuilder_.mergeFrom(value);
}
vectorBuilder_.setMessage(value);
}
leafCase_ = 1;
return this;
}
/**
*
* The interpretation of the values held in the leaves of a decision tree
* is application specific, but some common cases are:
* 1) len(vector) = 1, and the floating point value[0] holds the class 0
* probability in a two class classification problem.
* 2) len(vector) = 1, and the integer value[0] holds the class prediction.
* 3) The floating point value[i] holds the class i probability prediction.
* 4) The floating point value[i] holds the i-th component of the
* vector prediction in a regression problem.
* 5) sparse_vector holds the sparse class predictions for a classification
* problem with a large number of classes.
*
*
* .tensorflow.decision_trees.Vector vector = 1;
*/
public Builder clearVector() {
if (vectorBuilder_ == null) {
if (leafCase_ == 1) {
leafCase_ = 0;
leaf_ = null;
onChanged();
}
} else {
if (leafCase_ == 1) {
leafCase_ = 0;
leaf_ = null;
}
vectorBuilder_.clear();
}
return this;
}
/**
*
* The interpretation of the values held in the leaves of a decision tree
* is application specific, but some common cases are:
* 1) len(vector) = 1, and the floating point value[0] holds the class 0
* probability in a two class classification problem.
* 2) len(vector) = 1, and the integer value[0] holds the class prediction.
* 3) The floating point value[i] holds the class i probability prediction.
* 4) The floating point value[i] holds the i-th component of the
* vector prediction in a regression problem.
* 5) sparse_vector holds the sparse class predictions for a classification
* problem with a large number of classes.
*
*
* .tensorflow.decision_trees.Vector vector = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.Vector.Builder getVectorBuilder() {
return getVectorFieldBuilder().getBuilder();
}
/**
*
* The interpretation of the values held in the leaves of a decision tree
* is application specific, but some common cases are:
* 1) len(vector) = 1, and the floating point value[0] holds the class 0
* probability in a two class classification problem.
* 2) len(vector) = 1, and the integer value[0] holds the class prediction.
* 3) The floating point value[i] holds the class i probability prediction.
* 4) The floating point value[i] holds the i-th component of the
* vector prediction in a regression problem.
* 5) sparse_vector holds the sparse class predictions for a classification
* problem with a large number of classes.
*
*
* .tensorflow.decision_trees.Vector vector = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.VectorOrBuilder getVectorOrBuilder() {
if ((leafCase_ == 1) && (vectorBuilder_ != null)) {
return vectorBuilder_.getMessageOrBuilder();
} else {
if (leafCase_ == 1) {
return (tensorflow.decision_trees.GenericTreeModel.Vector) leaf_;
}
return tensorflow.decision_trees.GenericTreeModel.Vector.getDefaultInstance();
}
}
/**
*
* The interpretation of the values held in the leaves of a decision tree
* is application specific, but some common cases are:
* 1) len(vector) = 1, and the floating point value[0] holds the class 0
* probability in a two class classification problem.
* 2) len(vector) = 1, and the integer value[0] holds the class prediction.
* 3) The floating point value[i] holds the class i probability prediction.
* 4) The floating point value[i] holds the i-th component of the
* vector prediction in a regression problem.
* 5) sparse_vector holds the sparse class predictions for a classification
* problem with a large number of classes.
*
*
* .tensorflow.decision_trees.Vector vector = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Vector, tensorflow.decision_trees.GenericTreeModel.Vector.Builder, tensorflow.decision_trees.GenericTreeModel.VectorOrBuilder>
getVectorFieldBuilder() {
if (vectorBuilder_ == null) {
if (!(leafCase_ == 1)) {
leaf_ = tensorflow.decision_trees.GenericTreeModel.Vector.getDefaultInstance();
}
vectorBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Vector, tensorflow.decision_trees.GenericTreeModel.Vector.Builder, tensorflow.decision_trees.GenericTreeModel.VectorOrBuilder>(
(tensorflow.decision_trees.GenericTreeModel.Vector) leaf_,
getParentForChildren(),
isClean());
leaf_ = null;
}
leafCase_ = 1;
onChanged();;
return vectorBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.SparseVector, tensorflow.decision_trees.GenericTreeModel.SparseVector.Builder, tensorflow.decision_trees.GenericTreeModel.SparseVectorOrBuilder> sparseVectorBuilder_;
/**
* .tensorflow.decision_trees.SparseVector sparse_vector = 2;
*/
public boolean hasSparseVector() {
return leafCase_ == 2;
}
/**
* .tensorflow.decision_trees.SparseVector sparse_vector = 2;
*/
public tensorflow.decision_trees.GenericTreeModel.SparseVector getSparseVector() {
if (sparseVectorBuilder_ == null) {
if (leafCase_ == 2) {
return (tensorflow.decision_trees.GenericTreeModel.SparseVector) leaf_;
}
return tensorflow.decision_trees.GenericTreeModel.SparseVector.getDefaultInstance();
} else {
if (leafCase_ == 2) {
return sparseVectorBuilder_.getMessage();
}
return tensorflow.decision_trees.GenericTreeModel.SparseVector.getDefaultInstance();
}
}
/**
* .tensorflow.decision_trees.SparseVector sparse_vector = 2;
*/
public Builder setSparseVector(tensorflow.decision_trees.GenericTreeModel.SparseVector value) {
if (sparseVectorBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
leaf_ = value;
onChanged();
} else {
sparseVectorBuilder_.setMessage(value);
}
leafCase_ = 2;
return this;
}
/**
* .tensorflow.decision_trees.SparseVector sparse_vector = 2;
*/
public Builder setSparseVector(
tensorflow.decision_trees.GenericTreeModel.SparseVector.Builder builderForValue) {
if (sparseVectorBuilder_ == null) {
leaf_ = builderForValue.build();
onChanged();
} else {
sparseVectorBuilder_.setMessage(builderForValue.build());
}
leafCase_ = 2;
return this;
}
/**
* .tensorflow.decision_trees.SparseVector sparse_vector = 2;
*/
public Builder mergeSparseVector(tensorflow.decision_trees.GenericTreeModel.SparseVector value) {
if (sparseVectorBuilder_ == null) {
if (leafCase_ == 2 &&
leaf_ != tensorflow.decision_trees.GenericTreeModel.SparseVector.getDefaultInstance()) {
leaf_ = tensorflow.decision_trees.GenericTreeModel.SparseVector.newBuilder((tensorflow.decision_trees.GenericTreeModel.SparseVector) leaf_)
.mergeFrom(value).buildPartial();
} else {
leaf_ = value;
}
onChanged();
} else {
if (leafCase_ == 2) {
sparseVectorBuilder_.mergeFrom(value);
}
sparseVectorBuilder_.setMessage(value);
}
leafCase_ = 2;
return this;
}
/**
* .tensorflow.decision_trees.SparseVector sparse_vector = 2;
*/
public Builder clearSparseVector() {
if (sparseVectorBuilder_ == null) {
if (leafCase_ == 2) {
leafCase_ = 0;
leaf_ = null;
onChanged();
}
} else {
if (leafCase_ == 2) {
leafCase_ = 0;
leaf_ = null;
}
sparseVectorBuilder_.clear();
}
return this;
}
/**
* .tensorflow.decision_trees.SparseVector sparse_vector = 2;
*/
public tensorflow.decision_trees.GenericTreeModel.SparseVector.Builder getSparseVectorBuilder() {
return getSparseVectorFieldBuilder().getBuilder();
}
/**
* .tensorflow.decision_trees.SparseVector sparse_vector = 2;
*/
public tensorflow.decision_trees.GenericTreeModel.SparseVectorOrBuilder getSparseVectorOrBuilder() {
if ((leafCase_ == 2) && (sparseVectorBuilder_ != null)) {
return sparseVectorBuilder_.getMessageOrBuilder();
} else {
if (leafCase_ == 2) {
return (tensorflow.decision_trees.GenericTreeModel.SparseVector) leaf_;
}
return tensorflow.decision_trees.GenericTreeModel.SparseVector.getDefaultInstance();
}
}
/**
* .tensorflow.decision_trees.SparseVector sparse_vector = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.SparseVector, tensorflow.decision_trees.GenericTreeModel.SparseVector.Builder, tensorflow.decision_trees.GenericTreeModel.SparseVectorOrBuilder>
getSparseVectorFieldBuilder() {
if (sparseVectorBuilder_ == null) {
if (!(leafCase_ == 2)) {
leaf_ = tensorflow.decision_trees.GenericTreeModel.SparseVector.getDefaultInstance();
}
sparseVectorBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.SparseVector, tensorflow.decision_trees.GenericTreeModel.SparseVector.Builder, tensorflow.decision_trees.GenericTreeModel.SparseVectorOrBuilder>(
(tensorflow.decision_trees.GenericTreeModel.SparseVector) leaf_,
getParentForChildren(),
isClean());
leaf_ = null;
}
leafCase_ = 2;
onChanged();;
return sparseVectorBuilder_;
}
private java.util.List additionalData_ =
java.util.Collections.emptyList();
private void ensureAdditionalDataIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
additionalData_ = new java.util.ArrayList(additionalData_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> additionalDataBuilder_;
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
public java.util.List getAdditionalDataList() {
if (additionalDataBuilder_ == null) {
return java.util.Collections.unmodifiableList(additionalData_);
} else {
return additionalDataBuilder_.getMessageList();
}
}
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
public int getAdditionalDataCount() {
if (additionalDataBuilder_ == null) {
return additionalData_.size();
} else {
return additionalDataBuilder_.getCount();
}
}
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
public com.google.protobuf.Any getAdditionalData(int index) {
if (additionalDataBuilder_ == null) {
return additionalData_.get(index);
} else {
return additionalDataBuilder_.getMessage(index);
}
}
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder setAdditionalData(
int index, com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.set(index, value);
onChanged();
} else {
additionalDataBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder setAdditionalData(
int index, com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.set(index, builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder addAdditionalData(com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.add(value);
onChanged();
} else {
additionalDataBuilder_.addMessage(value);
}
return this;
}
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder addAdditionalData(
int index, com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.add(index, value);
onChanged();
} else {
additionalDataBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder addAdditionalData(
com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.add(builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder addAdditionalData(
int index, com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.add(index, builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder addAllAdditionalData(
java.lang.Iterable extends com.google.protobuf.Any> values) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, additionalData_);
onChanged();
} else {
additionalDataBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder clearAdditionalData() {
if (additionalDataBuilder_ == null) {
additionalData_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
additionalDataBuilder_.clear();
}
return this;
}
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
public Builder removeAdditionalData(int index) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.remove(index);
onChanged();
} else {
additionalDataBuilder_.remove(index);
}
return this;
}
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
public com.google.protobuf.Any.Builder getAdditionalDataBuilder(
int index) {
return getAdditionalDataFieldBuilder().getBuilder(index);
}
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
public com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index) {
if (additionalDataBuilder_ == null) {
return additionalData_.get(index); } else {
return additionalDataBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
public java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList() {
if (additionalDataBuilder_ != null) {
return additionalDataBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(additionalData_);
}
}
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
public com.google.protobuf.Any.Builder addAdditionalDataBuilder() {
return getAdditionalDataFieldBuilder().addBuilder(
com.google.protobuf.Any.getDefaultInstance());
}
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
public com.google.protobuf.Any.Builder addAdditionalDataBuilder(
int index) {
return getAdditionalDataFieldBuilder().addBuilder(
index, com.google.protobuf.Any.getDefaultInstance());
}
/**
*
* For non-standard handling of leaves.
*
*
* repeated .google.protobuf.Any additional_data = 3;
*/
public java.util.List
getAdditionalDataBuilderList() {
return getAdditionalDataFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getAdditionalDataFieldBuilder() {
if (additionalDataBuilder_ == null) {
additionalDataBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
additionalData_,
((bitField0_ & 0x00000004) == 0x00000004),
getParentForChildren(),
isClean());
additionalData_ = null;
}
return additionalDataBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.decision_trees.Leaf)
}
// @@protoc_insertion_point(class_scope:tensorflow.decision_trees.Leaf)
private static final tensorflow.decision_trees.GenericTreeModel.Leaf DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.decision_trees.GenericTreeModel.Leaf();
}
public static tensorflow.decision_trees.GenericTreeModel.Leaf getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Leaf parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Leaf(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.Leaf getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FeatureIdOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.decision_trees.FeatureId)
com.google.protobuf.MessageOrBuilder {
/**
* .google.protobuf.StringValue id = 1;
*/
boolean hasId();
/**
* .google.protobuf.StringValue id = 1;
*/
com.google.protobuf.StringValue getId();
/**
* .google.protobuf.StringValue id = 1;
*/
com.google.protobuf.StringValueOrBuilder getIdOrBuilder();
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
java.util.List
getAdditionalDataList();
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
com.google.protobuf.Any getAdditionalData(int index);
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
int getAdditionalDataCount();
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList();
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index);
}
/**
* Protobuf type {@code tensorflow.decision_trees.FeatureId}
*/
public static final class FeatureId extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.decision_trees.FeatureId)
FeatureIdOrBuilder {
private static final long serialVersionUID = 0L;
// Use FeatureId.newBuilder() to construct.
private FeatureId(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FeatureId() {
additionalData_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private FeatureId(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.StringValue.Builder subBuilder = null;
if (id_ != null) {
subBuilder = id_.toBuilder();
}
id_ = input.readMessage(com.google.protobuf.StringValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(id_);
id_ = subBuilder.buildPartial();
}
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
additionalData_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
additionalData_.add(
input.readMessage(com.google.protobuf.Any.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
additionalData_ = java.util.Collections.unmodifiableList(additionalData_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_FeatureId_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_FeatureId_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.FeatureId.class, tensorflow.decision_trees.GenericTreeModel.FeatureId.Builder.class);
}
private int bitField0_;
public static final int ID_FIELD_NUMBER = 1;
private com.google.protobuf.StringValue id_;
/**
* .google.protobuf.StringValue id = 1;
*/
public boolean hasId() {
return id_ != null;
}
/**
* .google.protobuf.StringValue id = 1;
*/
public com.google.protobuf.StringValue getId() {
return id_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : id_;
}
/**
* .google.protobuf.StringValue id = 1;
*/
public com.google.protobuf.StringValueOrBuilder getIdOrBuilder() {
return getId();
}
public static final int ADDITIONAL_DATA_FIELD_NUMBER = 2;
private java.util.List additionalData_;
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public java.util.List getAdditionalDataList() {
return additionalData_;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList() {
return additionalData_;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public int getAdditionalDataCount() {
return additionalData_.size();
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public com.google.protobuf.Any getAdditionalData(int index) {
return additionalData_.get(index);
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index) {
return additionalData_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (id_ != null) {
output.writeMessage(1, getId());
}
for (int i = 0; i < additionalData_.size(); i++) {
output.writeMessage(2, additionalData_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (id_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getId());
}
for (int i = 0; i < additionalData_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, additionalData_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.decision_trees.GenericTreeModel.FeatureId)) {
return super.equals(obj);
}
tensorflow.decision_trees.GenericTreeModel.FeatureId other = (tensorflow.decision_trees.GenericTreeModel.FeatureId) obj;
boolean result = true;
result = result && (hasId() == other.hasId());
if (hasId()) {
result = result && getId()
.equals(other.getId());
}
result = result && getAdditionalDataList()
.equals(other.getAdditionalDataList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
}
if (getAdditionalDataCount() > 0) {
hash = (37 * hash) + ADDITIONAL_DATA_FIELD_NUMBER;
hash = (53 * hash) + getAdditionalDataList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.decision_trees.GenericTreeModel.FeatureId parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.FeatureId parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.FeatureId parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.FeatureId parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.FeatureId parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.FeatureId parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.FeatureId parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.FeatureId parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.FeatureId parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.FeatureId parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.FeatureId parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.FeatureId parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.decision_trees.GenericTreeModel.FeatureId prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.decision_trees.FeatureId}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.decision_trees.FeatureId)
tensorflow.decision_trees.GenericTreeModel.FeatureIdOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_FeatureId_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_FeatureId_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.FeatureId.class, tensorflow.decision_trees.GenericTreeModel.FeatureId.Builder.class);
}
// Construct using tensorflow.decision_trees.GenericTreeModel.FeatureId.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getAdditionalDataFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (idBuilder_ == null) {
id_ = null;
} else {
id_ = null;
idBuilder_ = null;
}
if (additionalDataBuilder_ == null) {
additionalData_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
additionalDataBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_FeatureId_descriptor;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.FeatureId getDefaultInstanceForType() {
return tensorflow.decision_trees.GenericTreeModel.FeatureId.getDefaultInstance();
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.FeatureId build() {
tensorflow.decision_trees.GenericTreeModel.FeatureId result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.FeatureId buildPartial() {
tensorflow.decision_trees.GenericTreeModel.FeatureId result = new tensorflow.decision_trees.GenericTreeModel.FeatureId(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (idBuilder_ == null) {
result.id_ = id_;
} else {
result.id_ = idBuilder_.build();
}
if (additionalDataBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
additionalData_ = java.util.Collections.unmodifiableList(additionalData_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.additionalData_ = additionalData_;
} else {
result.additionalData_ = additionalDataBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.decision_trees.GenericTreeModel.FeatureId) {
return mergeFrom((tensorflow.decision_trees.GenericTreeModel.FeatureId)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.decision_trees.GenericTreeModel.FeatureId other) {
if (other == tensorflow.decision_trees.GenericTreeModel.FeatureId.getDefaultInstance()) return this;
if (other.hasId()) {
mergeId(other.getId());
}
if (additionalDataBuilder_ == null) {
if (!other.additionalData_.isEmpty()) {
if (additionalData_.isEmpty()) {
additionalData_ = other.additionalData_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureAdditionalDataIsMutable();
additionalData_.addAll(other.additionalData_);
}
onChanged();
}
} else {
if (!other.additionalData_.isEmpty()) {
if (additionalDataBuilder_.isEmpty()) {
additionalDataBuilder_.dispose();
additionalDataBuilder_ = null;
additionalData_ = other.additionalData_;
bitField0_ = (bitField0_ & ~0x00000002);
additionalDataBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAdditionalDataFieldBuilder() : null;
} else {
additionalDataBuilder_.addAllMessages(other.additionalData_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.decision_trees.GenericTreeModel.FeatureId parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.decision_trees.GenericTreeModel.FeatureId) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.StringValue id_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> idBuilder_;
/**
* .google.protobuf.StringValue id = 1;
*/
public boolean hasId() {
return idBuilder_ != null || id_ != null;
}
/**
* .google.protobuf.StringValue id = 1;
*/
public com.google.protobuf.StringValue getId() {
if (idBuilder_ == null) {
return id_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : id_;
} else {
return idBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue id = 1;
*/
public Builder setId(com.google.protobuf.StringValue value) {
if (idBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
} else {
idBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue id = 1;
*/
public Builder setId(
com.google.protobuf.StringValue.Builder builderForValue) {
if (idBuilder_ == null) {
id_ = builderForValue.build();
onChanged();
} else {
idBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue id = 1;
*/
public Builder mergeId(com.google.protobuf.StringValue value) {
if (idBuilder_ == null) {
if (id_ != null) {
id_ =
com.google.protobuf.StringValue.newBuilder(id_).mergeFrom(value).buildPartial();
} else {
id_ = value;
}
onChanged();
} else {
idBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue id = 1;
*/
public Builder clearId() {
if (idBuilder_ == null) {
id_ = null;
onChanged();
} else {
id_ = null;
idBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue id = 1;
*/
public com.google.protobuf.StringValue.Builder getIdBuilder() {
onChanged();
return getIdFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue id = 1;
*/
public com.google.protobuf.StringValueOrBuilder getIdOrBuilder() {
if (idBuilder_ != null) {
return idBuilder_.getMessageOrBuilder();
} else {
return id_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : id_;
}
}
/**
* .google.protobuf.StringValue id = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getIdFieldBuilder() {
if (idBuilder_ == null) {
idBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getId(),
getParentForChildren(),
isClean());
id_ = null;
}
return idBuilder_;
}
private java.util.List additionalData_ =
java.util.Collections.emptyList();
private void ensureAdditionalDataIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
additionalData_ = new java.util.ArrayList(additionalData_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> additionalDataBuilder_;
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public java.util.List getAdditionalDataList() {
if (additionalDataBuilder_ == null) {
return java.util.Collections.unmodifiableList(additionalData_);
} else {
return additionalDataBuilder_.getMessageList();
}
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public int getAdditionalDataCount() {
if (additionalDataBuilder_ == null) {
return additionalData_.size();
} else {
return additionalDataBuilder_.getCount();
}
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public com.google.protobuf.Any getAdditionalData(int index) {
if (additionalDataBuilder_ == null) {
return additionalData_.get(index);
} else {
return additionalDataBuilder_.getMessage(index);
}
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder setAdditionalData(
int index, com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.set(index, value);
onChanged();
} else {
additionalDataBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder setAdditionalData(
int index, com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.set(index, builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder addAdditionalData(com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.add(value);
onChanged();
} else {
additionalDataBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder addAdditionalData(
int index, com.google.protobuf.Any value) {
if (additionalDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalDataIsMutable();
additionalData_.add(index, value);
onChanged();
} else {
additionalDataBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder addAdditionalData(
com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.add(builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder addAdditionalData(
int index, com.google.protobuf.Any.Builder builderForValue) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.add(index, builderForValue.build());
onChanged();
} else {
additionalDataBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder addAllAdditionalData(
java.lang.Iterable extends com.google.protobuf.Any> values) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, additionalData_);
onChanged();
} else {
additionalDataBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder clearAdditionalData() {
if (additionalDataBuilder_ == null) {
additionalData_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
additionalDataBuilder_.clear();
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public Builder removeAdditionalData(int index) {
if (additionalDataBuilder_ == null) {
ensureAdditionalDataIsMutable();
additionalData_.remove(index);
onChanged();
} else {
additionalDataBuilder_.remove(index);
}
return this;
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public com.google.protobuf.Any.Builder getAdditionalDataBuilder(
int index) {
return getAdditionalDataFieldBuilder().getBuilder(index);
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public com.google.protobuf.AnyOrBuilder getAdditionalDataOrBuilder(
int index) {
if (additionalDataBuilder_ == null) {
return additionalData_.get(index); } else {
return additionalDataBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public java.util.List extends com.google.protobuf.AnyOrBuilder>
getAdditionalDataOrBuilderList() {
if (additionalDataBuilder_ != null) {
return additionalDataBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(additionalData_);
}
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public com.google.protobuf.Any.Builder addAdditionalDataBuilder() {
return getAdditionalDataFieldBuilder().addBuilder(
com.google.protobuf.Any.getDefaultInstance());
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public com.google.protobuf.Any.Builder addAdditionalDataBuilder(
int index) {
return getAdditionalDataFieldBuilder().addBuilder(
index, com.google.protobuf.Any.getDefaultInstance());
}
/**
* repeated .google.protobuf.Any additional_data = 2;
*/
public java.util.List
getAdditionalDataBuilderList() {
return getAdditionalDataFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getAdditionalDataFieldBuilder() {
if (additionalDataBuilder_ == null) {
additionalDataBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
additionalData_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
additionalData_ = null;
}
return additionalDataBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.decision_trees.FeatureId)
}
// @@protoc_insertion_point(class_scope:tensorflow.decision_trees.FeatureId)
private static final tensorflow.decision_trees.GenericTreeModel.FeatureId DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.decision_trees.GenericTreeModel.FeatureId();
}
public static tensorflow.decision_trees.GenericTreeModel.FeatureId getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FeatureId parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new FeatureId(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.FeatureId getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ObliqueFeaturesOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.decision_trees.ObliqueFeatures)
com.google.protobuf.MessageOrBuilder {
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
java.util.List
getFeaturesList();
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
tensorflow.decision_trees.GenericTreeModel.FeatureId getFeatures(int index);
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
int getFeaturesCount();
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
java.util.List extends tensorflow.decision_trees.GenericTreeModel.FeatureIdOrBuilder>
getFeaturesOrBuilderList();
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
tensorflow.decision_trees.GenericTreeModel.FeatureIdOrBuilder getFeaturesOrBuilder(
int index);
/**
* repeated float weights = 2;
*/
java.util.List getWeightsList();
/**
* repeated float weights = 2;
*/
int getWeightsCount();
/**
* repeated float weights = 2;
*/
float getWeights(int index);
}
/**
* Protobuf type {@code tensorflow.decision_trees.ObliqueFeatures}
*/
public static final class ObliqueFeatures extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.decision_trees.ObliqueFeatures)
ObliqueFeaturesOrBuilder {
private static final long serialVersionUID = 0L;
// Use ObliqueFeatures.newBuilder() to construct.
private ObliqueFeatures(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ObliqueFeatures() {
features_ = java.util.Collections.emptyList();
weights_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ObliqueFeatures(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
features_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
features_.add(
input.readMessage(tensorflow.decision_trees.GenericTreeModel.FeatureId.parser(), extensionRegistry));
break;
}
case 21: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
weights_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
weights_.add(input.readFloat());
break;
}
case 18: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002) && input.getBytesUntilLimit() > 0) {
weights_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
while (input.getBytesUntilLimit() > 0) {
weights_.add(input.readFloat());
}
input.popLimit(limit);
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
features_ = java.util.Collections.unmodifiableList(features_);
}
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
weights_ = java.util.Collections.unmodifiableList(weights_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_ObliqueFeatures_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_ObliqueFeatures_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures.class, tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures.Builder.class);
}
public static final int FEATURES_FIELD_NUMBER = 1;
private java.util.List features_;
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
public java.util.List getFeaturesList() {
return features_;
}
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
public java.util.List extends tensorflow.decision_trees.GenericTreeModel.FeatureIdOrBuilder>
getFeaturesOrBuilderList() {
return features_;
}
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
public int getFeaturesCount() {
return features_.size();
}
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.FeatureId getFeatures(int index) {
return features_.get(index);
}
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.FeatureIdOrBuilder getFeaturesOrBuilder(
int index) {
return features_.get(index);
}
public static final int WEIGHTS_FIELD_NUMBER = 2;
private java.util.List weights_;
/**
* repeated float weights = 2;
*/
public java.util.List
getWeightsList() {
return weights_;
}
/**
* repeated float weights = 2;
*/
public int getWeightsCount() {
return weights_.size();
}
/**
* repeated float weights = 2;
*/
public float getWeights(int index) {
return weights_.get(index);
}
private int weightsMemoizedSerializedSize = -1;
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < features_.size(); i++) {
output.writeMessage(1, features_.get(i));
}
if (getWeightsList().size() > 0) {
output.writeUInt32NoTag(18);
output.writeUInt32NoTag(weightsMemoizedSerializedSize);
}
for (int i = 0; i < weights_.size(); i++) {
output.writeFloatNoTag(weights_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < features_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, features_.get(i));
}
{
int dataSize = 0;
dataSize = 4 * getWeightsList().size();
size += dataSize;
if (!getWeightsList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
weightsMemoizedSerializedSize = dataSize;
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures)) {
return super.equals(obj);
}
tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures other = (tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures) obj;
boolean result = true;
result = result && getFeaturesList()
.equals(other.getFeaturesList());
result = result && getWeightsList()
.equals(other.getWeightsList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getFeaturesCount() > 0) {
hash = (37 * hash) + FEATURES_FIELD_NUMBER;
hash = (53 * hash) + getFeaturesList().hashCode();
}
if (getWeightsCount() > 0) {
hash = (37 * hash) + WEIGHTS_FIELD_NUMBER;
hash = (53 * hash) + getWeightsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.decision_trees.ObliqueFeatures}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.decision_trees.ObliqueFeatures)
tensorflow.decision_trees.GenericTreeModel.ObliqueFeaturesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_ObliqueFeatures_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_ObliqueFeatures_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures.class, tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures.Builder.class);
}
// Construct using tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getFeaturesFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (featuresBuilder_ == null) {
features_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
featuresBuilder_.clear();
}
weights_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_ObliqueFeatures_descriptor;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures getDefaultInstanceForType() {
return tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures.getDefaultInstance();
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures build() {
tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures buildPartial() {
tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures result = new tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures(this);
int from_bitField0_ = bitField0_;
if (featuresBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
features_ = java.util.Collections.unmodifiableList(features_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.features_ = features_;
} else {
result.features_ = featuresBuilder_.build();
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
weights_ = java.util.Collections.unmodifiableList(weights_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.weights_ = weights_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures) {
return mergeFrom((tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures other) {
if (other == tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures.getDefaultInstance()) return this;
if (featuresBuilder_ == null) {
if (!other.features_.isEmpty()) {
if (features_.isEmpty()) {
features_ = other.features_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureFeaturesIsMutable();
features_.addAll(other.features_);
}
onChanged();
}
} else {
if (!other.features_.isEmpty()) {
if (featuresBuilder_.isEmpty()) {
featuresBuilder_.dispose();
featuresBuilder_ = null;
features_ = other.features_;
bitField0_ = (bitField0_ & ~0x00000001);
featuresBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getFeaturesFieldBuilder() : null;
} else {
featuresBuilder_.addAllMessages(other.features_);
}
}
}
if (!other.weights_.isEmpty()) {
if (weights_.isEmpty()) {
weights_ = other.weights_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureWeightsIsMutable();
weights_.addAll(other.weights_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List features_ =
java.util.Collections.emptyList();
private void ensureFeaturesIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
features_ = new java.util.ArrayList(features_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.FeatureId, tensorflow.decision_trees.GenericTreeModel.FeatureId.Builder, tensorflow.decision_trees.GenericTreeModel.FeatureIdOrBuilder> featuresBuilder_;
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
public java.util.List getFeaturesList() {
if (featuresBuilder_ == null) {
return java.util.Collections.unmodifiableList(features_);
} else {
return featuresBuilder_.getMessageList();
}
}
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
public int getFeaturesCount() {
if (featuresBuilder_ == null) {
return features_.size();
} else {
return featuresBuilder_.getCount();
}
}
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.FeatureId getFeatures(int index) {
if (featuresBuilder_ == null) {
return features_.get(index);
} else {
return featuresBuilder_.getMessage(index);
}
}
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
public Builder setFeatures(
int index, tensorflow.decision_trees.GenericTreeModel.FeatureId value) {
if (featuresBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFeaturesIsMutable();
features_.set(index, value);
onChanged();
} else {
featuresBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
public Builder setFeatures(
int index, tensorflow.decision_trees.GenericTreeModel.FeatureId.Builder builderForValue) {
if (featuresBuilder_ == null) {
ensureFeaturesIsMutable();
features_.set(index, builderForValue.build());
onChanged();
} else {
featuresBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
public Builder addFeatures(tensorflow.decision_trees.GenericTreeModel.FeatureId value) {
if (featuresBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFeaturesIsMutable();
features_.add(value);
onChanged();
} else {
featuresBuilder_.addMessage(value);
}
return this;
}
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
public Builder addFeatures(
int index, tensorflow.decision_trees.GenericTreeModel.FeatureId value) {
if (featuresBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFeaturesIsMutable();
features_.add(index, value);
onChanged();
} else {
featuresBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
public Builder addFeatures(
tensorflow.decision_trees.GenericTreeModel.FeatureId.Builder builderForValue) {
if (featuresBuilder_ == null) {
ensureFeaturesIsMutable();
features_.add(builderForValue.build());
onChanged();
} else {
featuresBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
public Builder addFeatures(
int index, tensorflow.decision_trees.GenericTreeModel.FeatureId.Builder builderForValue) {
if (featuresBuilder_ == null) {
ensureFeaturesIsMutable();
features_.add(index, builderForValue.build());
onChanged();
} else {
featuresBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
public Builder addAllFeatures(
java.lang.Iterable extends tensorflow.decision_trees.GenericTreeModel.FeatureId> values) {
if (featuresBuilder_ == null) {
ensureFeaturesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, features_);
onChanged();
} else {
featuresBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
public Builder clearFeatures() {
if (featuresBuilder_ == null) {
features_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
featuresBuilder_.clear();
}
return this;
}
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
public Builder removeFeatures(int index) {
if (featuresBuilder_ == null) {
ensureFeaturesIsMutable();
features_.remove(index);
onChanged();
} else {
featuresBuilder_.remove(index);
}
return this;
}
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.FeatureId.Builder getFeaturesBuilder(
int index) {
return getFeaturesFieldBuilder().getBuilder(index);
}
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.FeatureIdOrBuilder getFeaturesOrBuilder(
int index) {
if (featuresBuilder_ == null) {
return features_.get(index); } else {
return featuresBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
public java.util.List extends tensorflow.decision_trees.GenericTreeModel.FeatureIdOrBuilder>
getFeaturesOrBuilderList() {
if (featuresBuilder_ != null) {
return featuresBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(features_);
}
}
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.FeatureId.Builder addFeaturesBuilder() {
return getFeaturesFieldBuilder().addBuilder(
tensorflow.decision_trees.GenericTreeModel.FeatureId.getDefaultInstance());
}
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.FeatureId.Builder addFeaturesBuilder(
int index) {
return getFeaturesFieldBuilder().addBuilder(
index, tensorflow.decision_trees.GenericTreeModel.FeatureId.getDefaultInstance());
}
/**
*
* total value is sum(features[i] * weights[i]).
*
*
* repeated .tensorflow.decision_trees.FeatureId features = 1;
*/
public java.util.List
getFeaturesBuilderList() {
return getFeaturesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.FeatureId, tensorflow.decision_trees.GenericTreeModel.FeatureId.Builder, tensorflow.decision_trees.GenericTreeModel.FeatureIdOrBuilder>
getFeaturesFieldBuilder() {
if (featuresBuilder_ == null) {
featuresBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.FeatureId, tensorflow.decision_trees.GenericTreeModel.FeatureId.Builder, tensorflow.decision_trees.GenericTreeModel.FeatureIdOrBuilder>(
features_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
features_ = null;
}
return featuresBuilder_;
}
private java.util.List weights_ = java.util.Collections.emptyList();
private void ensureWeightsIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
weights_ = new java.util.ArrayList(weights_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated float weights = 2;
*/
public java.util.List
getWeightsList() {
return java.util.Collections.unmodifiableList(weights_);
}
/**
* repeated float weights = 2;
*/
public int getWeightsCount() {
return weights_.size();
}
/**
* repeated float weights = 2;
*/
public float getWeights(int index) {
return weights_.get(index);
}
/**
* repeated float weights = 2;
*/
public Builder setWeights(
int index, float value) {
ensureWeightsIsMutable();
weights_.set(index, value);
onChanged();
return this;
}
/**
* repeated float weights = 2;
*/
public Builder addWeights(float value) {
ensureWeightsIsMutable();
weights_.add(value);
onChanged();
return this;
}
/**
* repeated float weights = 2;
*/
public Builder addAllWeights(
java.lang.Iterable extends java.lang.Float> values) {
ensureWeightsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, weights_);
onChanged();
return this;
}
/**
* repeated float weights = 2;
*/
public Builder clearWeights() {
weights_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.decision_trees.ObliqueFeatures)
}
// @@protoc_insertion_point(class_scope:tensorflow.decision_trees.ObliqueFeatures)
private static final tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures();
}
public static tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ObliqueFeatures parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ObliqueFeatures(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface InequalityTestOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.decision_trees.InequalityTest)
com.google.protobuf.MessageOrBuilder {
/**
* .tensorflow.decision_trees.FeatureId feature_id = 1;
*/
boolean hasFeatureId();
/**
* .tensorflow.decision_trees.FeatureId feature_id = 1;
*/
tensorflow.decision_trees.GenericTreeModel.FeatureId getFeatureId();
/**
* .tensorflow.decision_trees.FeatureId feature_id = 1;
*/
tensorflow.decision_trees.GenericTreeModel.FeatureIdOrBuilder getFeatureIdOrBuilder();
/**
* .tensorflow.decision_trees.ObliqueFeatures oblique = 4;
*/
boolean hasOblique();
/**
* .tensorflow.decision_trees.ObliqueFeatures oblique = 4;
*/
tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures getOblique();
/**
* .tensorflow.decision_trees.ObliqueFeatures oblique = 4;
*/
tensorflow.decision_trees.GenericTreeModel.ObliqueFeaturesOrBuilder getObliqueOrBuilder();
/**
* .tensorflow.decision_trees.InequalityTest.Type type = 2;
*/
int getTypeValue();
/**
* .tensorflow.decision_trees.InequalityTest.Type type = 2;
*/
tensorflow.decision_trees.GenericTreeModel.InequalityTest.Type getType();
/**
* .tensorflow.decision_trees.Value threshold = 3;
*/
boolean hasThreshold();
/**
* .tensorflow.decision_trees.Value threshold = 3;
*/
tensorflow.decision_trees.GenericTreeModel.Value getThreshold();
/**
* .tensorflow.decision_trees.Value threshold = 3;
*/
tensorflow.decision_trees.GenericTreeModel.ValueOrBuilder getThresholdOrBuilder();
public tensorflow.decision_trees.GenericTreeModel.InequalityTest.FeatureSumCase getFeatureSumCase();
}
/**
* Protobuf type {@code tensorflow.decision_trees.InequalityTest}
*/
public static final class InequalityTest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.decision_trees.InequalityTest)
InequalityTestOrBuilder {
private static final long serialVersionUID = 0L;
// Use InequalityTest.newBuilder() to construct.
private InequalityTest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private InequalityTest() {
type_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private InequalityTest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
tensorflow.decision_trees.GenericTreeModel.FeatureId.Builder subBuilder = null;
if (featureSumCase_ == 1) {
subBuilder = ((tensorflow.decision_trees.GenericTreeModel.FeatureId) featureSum_).toBuilder();
}
featureSum_ =
input.readMessage(tensorflow.decision_trees.GenericTreeModel.FeatureId.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((tensorflow.decision_trees.GenericTreeModel.FeatureId) featureSum_);
featureSum_ = subBuilder.buildPartial();
}
featureSumCase_ = 1;
break;
}
case 16: {
int rawValue = input.readEnum();
type_ = rawValue;
break;
}
case 26: {
tensorflow.decision_trees.GenericTreeModel.Value.Builder subBuilder = null;
if (threshold_ != null) {
subBuilder = threshold_.toBuilder();
}
threshold_ = input.readMessage(tensorflow.decision_trees.GenericTreeModel.Value.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(threshold_);
threshold_ = subBuilder.buildPartial();
}
break;
}
case 34: {
tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures.Builder subBuilder = null;
if (featureSumCase_ == 4) {
subBuilder = ((tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures) featureSum_).toBuilder();
}
featureSum_ =
input.readMessage(tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures) featureSum_);
featureSum_ = subBuilder.buildPartial();
}
featureSumCase_ = 4;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_InequalityTest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_InequalityTest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.InequalityTest.class, tensorflow.decision_trees.GenericTreeModel.InequalityTest.Builder.class);
}
/**
* Protobuf enum {@code tensorflow.decision_trees.InequalityTest.Type}
*/
public enum Type
implements com.google.protobuf.ProtocolMessageEnum {
/**
* LESS_OR_EQUAL = 0;
*/
LESS_OR_EQUAL(0),
/**
* LESS_THAN = 1;
*/
LESS_THAN(1),
/**
* GREATER_OR_EQUAL = 2;
*/
GREATER_OR_EQUAL(2),
/**
* GREATER_THAN = 3;
*/
GREATER_THAN(3),
UNRECOGNIZED(-1),
;
/**
* LESS_OR_EQUAL = 0;
*/
public static final int LESS_OR_EQUAL_VALUE = 0;
/**
* LESS_THAN = 1;
*/
public static final int LESS_THAN_VALUE = 1;
/**
* GREATER_OR_EQUAL = 2;
*/
public static final int GREATER_OR_EQUAL_VALUE = 2;
/**
* GREATER_THAN = 3;
*/
public static final int GREATER_THAN_VALUE = 3;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Type valueOf(int value) {
return forNumber(value);
}
public static Type forNumber(int value) {
switch (value) {
case 0: return LESS_OR_EQUAL;
case 1: return LESS_THAN;
case 2: return GREATER_OR_EQUAL;
case 3: return GREATER_THAN;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Type> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Type findValueByNumber(int number) {
return Type.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.InequalityTest.getDescriptor().getEnumTypes().get(0);
}
private static final Type[] VALUES = values();
public static Type valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Type(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:tensorflow.decision_trees.InequalityTest.Type)
}
private int featureSumCase_ = 0;
private java.lang.Object featureSum_;
public enum FeatureSumCase
implements com.google.protobuf.Internal.EnumLite {
FEATURE_ID(1),
OBLIQUE(4),
FEATURESUM_NOT_SET(0);
private final int value;
private FeatureSumCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static FeatureSumCase valueOf(int value) {
return forNumber(value);
}
public static FeatureSumCase forNumber(int value) {
switch (value) {
case 1: return FEATURE_ID;
case 4: return OBLIQUE;
case 0: return FEATURESUM_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public FeatureSumCase
getFeatureSumCase() {
return FeatureSumCase.forNumber(
featureSumCase_);
}
public static final int FEATURE_ID_FIELD_NUMBER = 1;
/**
* .tensorflow.decision_trees.FeatureId feature_id = 1;
*/
public boolean hasFeatureId() {
return featureSumCase_ == 1;
}
/**
* .tensorflow.decision_trees.FeatureId feature_id = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.FeatureId getFeatureId() {
if (featureSumCase_ == 1) {
return (tensorflow.decision_trees.GenericTreeModel.FeatureId) featureSum_;
}
return tensorflow.decision_trees.GenericTreeModel.FeatureId.getDefaultInstance();
}
/**
* .tensorflow.decision_trees.FeatureId feature_id = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.FeatureIdOrBuilder getFeatureIdOrBuilder() {
if (featureSumCase_ == 1) {
return (tensorflow.decision_trees.GenericTreeModel.FeatureId) featureSum_;
}
return tensorflow.decision_trees.GenericTreeModel.FeatureId.getDefaultInstance();
}
public static final int OBLIQUE_FIELD_NUMBER = 4;
/**
* .tensorflow.decision_trees.ObliqueFeatures oblique = 4;
*/
public boolean hasOblique() {
return featureSumCase_ == 4;
}
/**
* .tensorflow.decision_trees.ObliqueFeatures oblique = 4;
*/
public tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures getOblique() {
if (featureSumCase_ == 4) {
return (tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures) featureSum_;
}
return tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures.getDefaultInstance();
}
/**
* .tensorflow.decision_trees.ObliqueFeatures oblique = 4;
*/
public tensorflow.decision_trees.GenericTreeModel.ObliqueFeaturesOrBuilder getObliqueOrBuilder() {
if (featureSumCase_ == 4) {
return (tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures) featureSum_;
}
return tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures.getDefaultInstance();
}
public static final int TYPE_FIELD_NUMBER = 2;
private int type_;
/**
* .tensorflow.decision_trees.InequalityTest.Type type = 2;
*/
public int getTypeValue() {
return type_;
}
/**
* .tensorflow.decision_trees.InequalityTest.Type type = 2;
*/
public tensorflow.decision_trees.GenericTreeModel.InequalityTest.Type getType() {
@SuppressWarnings("deprecation")
tensorflow.decision_trees.GenericTreeModel.InequalityTest.Type result = tensorflow.decision_trees.GenericTreeModel.InequalityTest.Type.valueOf(type_);
return result == null ? tensorflow.decision_trees.GenericTreeModel.InequalityTest.Type.UNRECOGNIZED : result;
}
public static final int THRESHOLD_FIELD_NUMBER = 3;
private tensorflow.decision_trees.GenericTreeModel.Value threshold_;
/**
* .tensorflow.decision_trees.Value threshold = 3;
*/
public boolean hasThreshold() {
return threshold_ != null;
}
/**
* .tensorflow.decision_trees.Value threshold = 3;
*/
public tensorflow.decision_trees.GenericTreeModel.Value getThreshold() {
return threshold_ == null ? tensorflow.decision_trees.GenericTreeModel.Value.getDefaultInstance() : threshold_;
}
/**
* .tensorflow.decision_trees.Value threshold = 3;
*/
public tensorflow.decision_trees.GenericTreeModel.ValueOrBuilder getThresholdOrBuilder() {
return getThreshold();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (featureSumCase_ == 1) {
output.writeMessage(1, (tensorflow.decision_trees.GenericTreeModel.FeatureId) featureSum_);
}
if (type_ != tensorflow.decision_trees.GenericTreeModel.InequalityTest.Type.LESS_OR_EQUAL.getNumber()) {
output.writeEnum(2, type_);
}
if (threshold_ != null) {
output.writeMessage(3, getThreshold());
}
if (featureSumCase_ == 4) {
output.writeMessage(4, (tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures) featureSum_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (featureSumCase_ == 1) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, (tensorflow.decision_trees.GenericTreeModel.FeatureId) featureSum_);
}
if (type_ != tensorflow.decision_trees.GenericTreeModel.InequalityTest.Type.LESS_OR_EQUAL.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, type_);
}
if (threshold_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getThreshold());
}
if (featureSumCase_ == 4) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, (tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures) featureSum_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.decision_trees.GenericTreeModel.InequalityTest)) {
return super.equals(obj);
}
tensorflow.decision_trees.GenericTreeModel.InequalityTest other = (tensorflow.decision_trees.GenericTreeModel.InequalityTest) obj;
boolean result = true;
result = result && type_ == other.type_;
result = result && (hasThreshold() == other.hasThreshold());
if (hasThreshold()) {
result = result && getThreshold()
.equals(other.getThreshold());
}
result = result && getFeatureSumCase().equals(
other.getFeatureSumCase());
if (!result) return false;
switch (featureSumCase_) {
case 1:
result = result && getFeatureId()
.equals(other.getFeatureId());
break;
case 4:
result = result && getOblique()
.equals(other.getOblique());
break;
case 0:
default:
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
if (hasThreshold()) {
hash = (37 * hash) + THRESHOLD_FIELD_NUMBER;
hash = (53 * hash) + getThreshold().hashCode();
}
switch (featureSumCase_) {
case 1:
hash = (37 * hash) + FEATURE_ID_FIELD_NUMBER;
hash = (53 * hash) + getFeatureId().hashCode();
break;
case 4:
hash = (37 * hash) + OBLIQUE_FIELD_NUMBER;
hash = (53 * hash) + getOblique().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.decision_trees.GenericTreeModel.InequalityTest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.InequalityTest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.InequalityTest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.InequalityTest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.InequalityTest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.decision_trees.GenericTreeModel.InequalityTest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.InequalityTest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.InequalityTest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.InequalityTest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.InequalityTest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.decision_trees.GenericTreeModel.InequalityTest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.decision_trees.GenericTreeModel.InequalityTest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.decision_trees.GenericTreeModel.InequalityTest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.decision_trees.InequalityTest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.decision_trees.InequalityTest)
tensorflow.decision_trees.GenericTreeModel.InequalityTestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_InequalityTest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_InequalityTest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.decision_trees.GenericTreeModel.InequalityTest.class, tensorflow.decision_trees.GenericTreeModel.InequalityTest.Builder.class);
}
// Construct using tensorflow.decision_trees.GenericTreeModel.InequalityTest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
type_ = 0;
if (thresholdBuilder_ == null) {
threshold_ = null;
} else {
threshold_ = null;
thresholdBuilder_ = null;
}
featureSumCase_ = 0;
featureSum_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.decision_trees.GenericTreeModel.internal_static_tensorflow_decision_trees_InequalityTest_descriptor;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.InequalityTest getDefaultInstanceForType() {
return tensorflow.decision_trees.GenericTreeModel.InequalityTest.getDefaultInstance();
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.InequalityTest build() {
tensorflow.decision_trees.GenericTreeModel.InequalityTest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.InequalityTest buildPartial() {
tensorflow.decision_trees.GenericTreeModel.InequalityTest result = new tensorflow.decision_trees.GenericTreeModel.InequalityTest(this);
if (featureSumCase_ == 1) {
if (featureIdBuilder_ == null) {
result.featureSum_ = featureSum_;
} else {
result.featureSum_ = featureIdBuilder_.build();
}
}
if (featureSumCase_ == 4) {
if (obliqueBuilder_ == null) {
result.featureSum_ = featureSum_;
} else {
result.featureSum_ = obliqueBuilder_.build();
}
}
result.type_ = type_;
if (thresholdBuilder_ == null) {
result.threshold_ = threshold_;
} else {
result.threshold_ = thresholdBuilder_.build();
}
result.featureSumCase_ = featureSumCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.decision_trees.GenericTreeModel.InequalityTest) {
return mergeFrom((tensorflow.decision_trees.GenericTreeModel.InequalityTest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.decision_trees.GenericTreeModel.InequalityTest other) {
if (other == tensorflow.decision_trees.GenericTreeModel.InequalityTest.getDefaultInstance()) return this;
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
if (other.hasThreshold()) {
mergeThreshold(other.getThreshold());
}
switch (other.getFeatureSumCase()) {
case FEATURE_ID: {
mergeFeatureId(other.getFeatureId());
break;
}
case OBLIQUE: {
mergeOblique(other.getOblique());
break;
}
case FEATURESUM_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.decision_trees.GenericTreeModel.InequalityTest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.decision_trees.GenericTreeModel.InequalityTest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int featureSumCase_ = 0;
private java.lang.Object featureSum_;
public FeatureSumCase
getFeatureSumCase() {
return FeatureSumCase.forNumber(
featureSumCase_);
}
public Builder clearFeatureSum() {
featureSumCase_ = 0;
featureSum_ = null;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.FeatureId, tensorflow.decision_trees.GenericTreeModel.FeatureId.Builder, tensorflow.decision_trees.GenericTreeModel.FeatureIdOrBuilder> featureIdBuilder_;
/**
* .tensorflow.decision_trees.FeatureId feature_id = 1;
*/
public boolean hasFeatureId() {
return featureSumCase_ == 1;
}
/**
* .tensorflow.decision_trees.FeatureId feature_id = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.FeatureId getFeatureId() {
if (featureIdBuilder_ == null) {
if (featureSumCase_ == 1) {
return (tensorflow.decision_trees.GenericTreeModel.FeatureId) featureSum_;
}
return tensorflow.decision_trees.GenericTreeModel.FeatureId.getDefaultInstance();
} else {
if (featureSumCase_ == 1) {
return featureIdBuilder_.getMessage();
}
return tensorflow.decision_trees.GenericTreeModel.FeatureId.getDefaultInstance();
}
}
/**
* .tensorflow.decision_trees.FeatureId feature_id = 1;
*/
public Builder setFeatureId(tensorflow.decision_trees.GenericTreeModel.FeatureId value) {
if (featureIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
featureSum_ = value;
onChanged();
} else {
featureIdBuilder_.setMessage(value);
}
featureSumCase_ = 1;
return this;
}
/**
* .tensorflow.decision_trees.FeatureId feature_id = 1;
*/
public Builder setFeatureId(
tensorflow.decision_trees.GenericTreeModel.FeatureId.Builder builderForValue) {
if (featureIdBuilder_ == null) {
featureSum_ = builderForValue.build();
onChanged();
} else {
featureIdBuilder_.setMessage(builderForValue.build());
}
featureSumCase_ = 1;
return this;
}
/**
* .tensorflow.decision_trees.FeatureId feature_id = 1;
*/
public Builder mergeFeatureId(tensorflow.decision_trees.GenericTreeModel.FeatureId value) {
if (featureIdBuilder_ == null) {
if (featureSumCase_ == 1 &&
featureSum_ != tensorflow.decision_trees.GenericTreeModel.FeatureId.getDefaultInstance()) {
featureSum_ = tensorflow.decision_trees.GenericTreeModel.FeatureId.newBuilder((tensorflow.decision_trees.GenericTreeModel.FeatureId) featureSum_)
.mergeFrom(value).buildPartial();
} else {
featureSum_ = value;
}
onChanged();
} else {
if (featureSumCase_ == 1) {
featureIdBuilder_.mergeFrom(value);
}
featureIdBuilder_.setMessage(value);
}
featureSumCase_ = 1;
return this;
}
/**
* .tensorflow.decision_trees.FeatureId feature_id = 1;
*/
public Builder clearFeatureId() {
if (featureIdBuilder_ == null) {
if (featureSumCase_ == 1) {
featureSumCase_ = 0;
featureSum_ = null;
onChanged();
}
} else {
if (featureSumCase_ == 1) {
featureSumCase_ = 0;
featureSum_ = null;
}
featureIdBuilder_.clear();
}
return this;
}
/**
* .tensorflow.decision_trees.FeatureId feature_id = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.FeatureId.Builder getFeatureIdBuilder() {
return getFeatureIdFieldBuilder().getBuilder();
}
/**
* .tensorflow.decision_trees.FeatureId feature_id = 1;
*/
public tensorflow.decision_trees.GenericTreeModel.FeatureIdOrBuilder getFeatureIdOrBuilder() {
if ((featureSumCase_ == 1) && (featureIdBuilder_ != null)) {
return featureIdBuilder_.getMessageOrBuilder();
} else {
if (featureSumCase_ == 1) {
return (tensorflow.decision_trees.GenericTreeModel.FeatureId) featureSum_;
}
return tensorflow.decision_trees.GenericTreeModel.FeatureId.getDefaultInstance();
}
}
/**
* .tensorflow.decision_trees.FeatureId feature_id = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.FeatureId, tensorflow.decision_trees.GenericTreeModel.FeatureId.Builder, tensorflow.decision_trees.GenericTreeModel.FeatureIdOrBuilder>
getFeatureIdFieldBuilder() {
if (featureIdBuilder_ == null) {
if (!(featureSumCase_ == 1)) {
featureSum_ = tensorflow.decision_trees.GenericTreeModel.FeatureId.getDefaultInstance();
}
featureIdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.FeatureId, tensorflow.decision_trees.GenericTreeModel.FeatureId.Builder, tensorflow.decision_trees.GenericTreeModel.FeatureIdOrBuilder>(
(tensorflow.decision_trees.GenericTreeModel.FeatureId) featureSum_,
getParentForChildren(),
isClean());
featureSum_ = null;
}
featureSumCase_ = 1;
onChanged();;
return featureIdBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures, tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures.Builder, tensorflow.decision_trees.GenericTreeModel.ObliqueFeaturesOrBuilder> obliqueBuilder_;
/**
* .tensorflow.decision_trees.ObliqueFeatures oblique = 4;
*/
public boolean hasOblique() {
return featureSumCase_ == 4;
}
/**
* .tensorflow.decision_trees.ObliqueFeatures oblique = 4;
*/
public tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures getOblique() {
if (obliqueBuilder_ == null) {
if (featureSumCase_ == 4) {
return (tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures) featureSum_;
}
return tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures.getDefaultInstance();
} else {
if (featureSumCase_ == 4) {
return obliqueBuilder_.getMessage();
}
return tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures.getDefaultInstance();
}
}
/**
* .tensorflow.decision_trees.ObliqueFeatures oblique = 4;
*/
public Builder setOblique(tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures value) {
if (obliqueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
featureSum_ = value;
onChanged();
} else {
obliqueBuilder_.setMessage(value);
}
featureSumCase_ = 4;
return this;
}
/**
* .tensorflow.decision_trees.ObliqueFeatures oblique = 4;
*/
public Builder setOblique(
tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures.Builder builderForValue) {
if (obliqueBuilder_ == null) {
featureSum_ = builderForValue.build();
onChanged();
} else {
obliqueBuilder_.setMessage(builderForValue.build());
}
featureSumCase_ = 4;
return this;
}
/**
* .tensorflow.decision_trees.ObliqueFeatures oblique = 4;
*/
public Builder mergeOblique(tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures value) {
if (obliqueBuilder_ == null) {
if (featureSumCase_ == 4 &&
featureSum_ != tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures.getDefaultInstance()) {
featureSum_ = tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures.newBuilder((tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures) featureSum_)
.mergeFrom(value).buildPartial();
} else {
featureSum_ = value;
}
onChanged();
} else {
if (featureSumCase_ == 4) {
obliqueBuilder_.mergeFrom(value);
}
obliqueBuilder_.setMessage(value);
}
featureSumCase_ = 4;
return this;
}
/**
* .tensorflow.decision_trees.ObliqueFeatures oblique = 4;
*/
public Builder clearOblique() {
if (obliqueBuilder_ == null) {
if (featureSumCase_ == 4) {
featureSumCase_ = 0;
featureSum_ = null;
onChanged();
}
} else {
if (featureSumCase_ == 4) {
featureSumCase_ = 0;
featureSum_ = null;
}
obliqueBuilder_.clear();
}
return this;
}
/**
* .tensorflow.decision_trees.ObliqueFeatures oblique = 4;
*/
public tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures.Builder getObliqueBuilder() {
return getObliqueFieldBuilder().getBuilder();
}
/**
* .tensorflow.decision_trees.ObliqueFeatures oblique = 4;
*/
public tensorflow.decision_trees.GenericTreeModel.ObliqueFeaturesOrBuilder getObliqueOrBuilder() {
if ((featureSumCase_ == 4) && (obliqueBuilder_ != null)) {
return obliqueBuilder_.getMessageOrBuilder();
} else {
if (featureSumCase_ == 4) {
return (tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures) featureSum_;
}
return tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures.getDefaultInstance();
}
}
/**
* .tensorflow.decision_trees.ObliqueFeatures oblique = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures, tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures.Builder, tensorflow.decision_trees.GenericTreeModel.ObliqueFeaturesOrBuilder>
getObliqueFieldBuilder() {
if (obliqueBuilder_ == null) {
if (!(featureSumCase_ == 4)) {
featureSum_ = tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures.getDefaultInstance();
}
obliqueBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures, tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures.Builder, tensorflow.decision_trees.GenericTreeModel.ObliqueFeaturesOrBuilder>(
(tensorflow.decision_trees.GenericTreeModel.ObliqueFeatures) featureSum_,
getParentForChildren(),
isClean());
featureSum_ = null;
}
featureSumCase_ = 4;
onChanged();;
return obliqueBuilder_;
}
private int type_ = 0;
/**
* .tensorflow.decision_trees.InequalityTest.Type type = 2;
*/
public int getTypeValue() {
return type_;
}
/**
* .tensorflow.decision_trees.InequalityTest.Type type = 2;
*/
public Builder setTypeValue(int value) {
type_ = value;
onChanged();
return this;
}
/**
* .tensorflow.decision_trees.InequalityTest.Type type = 2;
*/
public tensorflow.decision_trees.GenericTreeModel.InequalityTest.Type getType() {
@SuppressWarnings("deprecation")
tensorflow.decision_trees.GenericTreeModel.InequalityTest.Type result = tensorflow.decision_trees.GenericTreeModel.InequalityTest.Type.valueOf(type_);
return result == null ? tensorflow.decision_trees.GenericTreeModel.InequalityTest.Type.UNRECOGNIZED : result;
}
/**
* .tensorflow.decision_trees.InequalityTest.Type type = 2;
*/
public Builder setType(tensorflow.decision_trees.GenericTreeModel.InequalityTest.Type value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value.getNumber();
onChanged();
return this;
}
/**
* .tensorflow.decision_trees.InequalityTest.Type type = 2;
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
private tensorflow.decision_trees.GenericTreeModel.Value threshold_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Value, tensorflow.decision_trees.GenericTreeModel.Value.Builder, tensorflow.decision_trees.GenericTreeModel.ValueOrBuilder> thresholdBuilder_;
/**
* .tensorflow.decision_trees.Value threshold = 3;
*/
public boolean hasThreshold() {
return thresholdBuilder_ != null || threshold_ != null;
}
/**
* .tensorflow.decision_trees.Value threshold = 3;
*/
public tensorflow.decision_trees.GenericTreeModel.Value getThreshold() {
if (thresholdBuilder_ == null) {
return threshold_ == null ? tensorflow.decision_trees.GenericTreeModel.Value.getDefaultInstance() : threshold_;
} else {
return thresholdBuilder_.getMessage();
}
}
/**
* .tensorflow.decision_trees.Value threshold = 3;
*/
public Builder setThreshold(tensorflow.decision_trees.GenericTreeModel.Value value) {
if (thresholdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
threshold_ = value;
onChanged();
} else {
thresholdBuilder_.setMessage(value);
}
return this;
}
/**
* .tensorflow.decision_trees.Value threshold = 3;
*/
public Builder setThreshold(
tensorflow.decision_trees.GenericTreeModel.Value.Builder builderForValue) {
if (thresholdBuilder_ == null) {
threshold_ = builderForValue.build();
onChanged();
} else {
thresholdBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .tensorflow.decision_trees.Value threshold = 3;
*/
public Builder mergeThreshold(tensorflow.decision_trees.GenericTreeModel.Value value) {
if (thresholdBuilder_ == null) {
if (threshold_ != null) {
threshold_ =
tensorflow.decision_trees.GenericTreeModel.Value.newBuilder(threshold_).mergeFrom(value).buildPartial();
} else {
threshold_ = value;
}
onChanged();
} else {
thresholdBuilder_.mergeFrom(value);
}
return this;
}
/**
* .tensorflow.decision_trees.Value threshold = 3;
*/
public Builder clearThreshold() {
if (thresholdBuilder_ == null) {
threshold_ = null;
onChanged();
} else {
threshold_ = null;
thresholdBuilder_ = null;
}
return this;
}
/**
* .tensorflow.decision_trees.Value threshold = 3;
*/
public tensorflow.decision_trees.GenericTreeModel.Value.Builder getThresholdBuilder() {
onChanged();
return getThresholdFieldBuilder().getBuilder();
}
/**
* .tensorflow.decision_trees.Value threshold = 3;
*/
public tensorflow.decision_trees.GenericTreeModel.ValueOrBuilder getThresholdOrBuilder() {
if (thresholdBuilder_ != null) {
return thresholdBuilder_.getMessageOrBuilder();
} else {
return threshold_ == null ?
tensorflow.decision_trees.GenericTreeModel.Value.getDefaultInstance() : threshold_;
}
}
/**
* .tensorflow.decision_trees.Value threshold = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Value, tensorflow.decision_trees.GenericTreeModel.Value.Builder, tensorflow.decision_trees.GenericTreeModel.ValueOrBuilder>
getThresholdFieldBuilder() {
if (thresholdBuilder_ == null) {
thresholdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.decision_trees.GenericTreeModel.Value, tensorflow.decision_trees.GenericTreeModel.Value.Builder, tensorflow.decision_trees.GenericTreeModel.ValueOrBuilder>(
getThreshold(),
getParentForChildren(),
isClean());
threshold_ = null;
}
return thresholdBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.decision_trees.InequalityTest)
}
// @@protoc_insertion_point(class_scope:tensorflow.decision_trees.InequalityTest)
private static final tensorflow.decision_trees.GenericTreeModel.InequalityTest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.decision_trees.GenericTreeModel.InequalityTest();
}
public static tensorflow.decision_trees.GenericTreeModel.InequalityTest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public InequalityTest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new InequalityTest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.decision_trees.GenericTreeModel.InequalityTest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ValueOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.decision_trees.Value)
com.google.protobuf.MessageOrBuilder {
/**
*