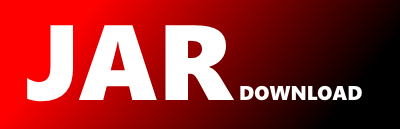
tensorflow.test.Test Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: tensorflow/tools/proto_text/test.proto
package tensorflow.test;
public final class Test {
private Test() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code tensorflow.test.ForeignEnum}
*/
public enum ForeignEnum
implements com.google.protobuf.ProtocolMessageEnum {
/**
* FOREIGN_ZERO = 0;
*/
FOREIGN_ZERO(0),
/**
* FOREIGN_FOO = 4;
*/
FOREIGN_FOO(4),
/**
* FOREIGN_BAR = 5;
*/
FOREIGN_BAR(5),
/**
* FOREIGN_BAZ = 6;
*/
FOREIGN_BAZ(6),
UNRECOGNIZED(-1),
;
/**
* FOREIGN_ZERO = 0;
*/
public static final int FOREIGN_ZERO_VALUE = 0;
/**
* FOREIGN_FOO = 4;
*/
public static final int FOREIGN_FOO_VALUE = 4;
/**
* FOREIGN_BAR = 5;
*/
public static final int FOREIGN_BAR_VALUE = 5;
/**
* FOREIGN_BAZ = 6;
*/
public static final int FOREIGN_BAZ_VALUE = 6;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ForeignEnum valueOf(int value) {
return forNumber(value);
}
public static ForeignEnum forNumber(int value) {
switch (value) {
case 0: return FOREIGN_ZERO;
case 4: return FOREIGN_FOO;
case 5: return FOREIGN_BAR;
case 6: return FOREIGN_BAZ;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ForeignEnum> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ForeignEnum findValueByNumber(int number) {
return ForeignEnum.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return tensorflow.test.Test.getDescriptor().getEnumTypes().get(0);
}
private static final ForeignEnum[] VALUES = values();
public static ForeignEnum valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private ForeignEnum(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:tensorflow.test.ForeignEnum)
}
public interface TestAllTypesOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.test.TestAllTypes)
com.google.protobuf.MessageOrBuilder {
/**
*
* Singular
*
*
* int32 optional_int32 = 1000;
*/
int getOptionalInt32();
/**
* int64 optional_int64 = 2;
*/
long getOptionalInt64();
/**
* uint32 optional_uint32 = 3;
*/
int getOptionalUint32();
/**
*
* use large tag to test output order.
*
*
* uint64 optional_uint64 = 999;
*/
long getOptionalUint64();
/**
* sint32 optional_sint32 = 5;
*/
int getOptionalSint32();
/**
* sint64 optional_sint64 = 6;
*/
long getOptionalSint64();
/**
* fixed32 optional_fixed32 = 7;
*/
int getOptionalFixed32();
/**
* fixed64 optional_fixed64 = 8;
*/
long getOptionalFixed64();
/**
* sfixed32 optional_sfixed32 = 9;
*/
int getOptionalSfixed32();
/**
* sfixed64 optional_sfixed64 = 10;
*/
long getOptionalSfixed64();
/**
* float optional_float = 11;
*/
float getOptionalFloat();
/**
* double optional_double = 12;
*/
double getOptionalDouble();
/**
* bool optional_bool = 13;
*/
boolean getOptionalBool();
/**
* string optional_string = 14;
*/
java.lang.String getOptionalString();
/**
* string optional_string = 14;
*/
com.google.protobuf.ByteString
getOptionalStringBytes();
/**
* bytes optional_bytes = 15;
*/
com.google.protobuf.ByteString getOptionalBytes();
/**
* .tensorflow.test.TestAllTypes.NestedMessage optional_nested_message = 18;
*/
boolean hasOptionalNestedMessage();
/**
* .tensorflow.test.TestAllTypes.NestedMessage optional_nested_message = 18;
*/
tensorflow.test.Test.TestAllTypes.NestedMessage getOptionalNestedMessage();
/**
* .tensorflow.test.TestAllTypes.NestedMessage optional_nested_message = 18;
*/
tensorflow.test.Test.TestAllTypes.NestedMessageOrBuilder getOptionalNestedMessageOrBuilder();
/**
* .tensorflow.test.ForeignMessage optional_foreign_message = 19;
*/
boolean hasOptionalForeignMessage();
/**
* .tensorflow.test.ForeignMessage optional_foreign_message = 19;
*/
tensorflow.test.Test.ForeignMessage getOptionalForeignMessage();
/**
* .tensorflow.test.ForeignMessage optional_foreign_message = 19;
*/
tensorflow.test.Test.ForeignMessageOrBuilder getOptionalForeignMessageOrBuilder();
/**
* .tensorflow.test.TestAllTypes.NestedEnum optional_nested_enum = 21;
*/
int getOptionalNestedEnumValue();
/**
* .tensorflow.test.TestAllTypes.NestedEnum optional_nested_enum = 21;
*/
tensorflow.test.Test.TestAllTypes.NestedEnum getOptionalNestedEnum();
/**
* .tensorflow.test.ForeignEnum optional_foreign_enum = 22;
*/
int getOptionalForeignEnumValue();
/**
* .tensorflow.test.ForeignEnum optional_foreign_enum = 22;
*/
tensorflow.test.Test.ForeignEnum getOptionalForeignEnum();
/**
* string optional_cord = 25;
*/
java.lang.String getOptionalCord();
/**
* string optional_cord = 25;
*/
com.google.protobuf.ByteString
getOptionalCordBytes();
/**
*
* Repeated
*
*
* repeated int32 repeated_int32 = 31;
*/
java.util.List getRepeatedInt32List();
/**
*
* Repeated
*
*
* repeated int32 repeated_int32 = 31;
*/
int getRepeatedInt32Count();
/**
*
* Repeated
*
*
* repeated int32 repeated_int32 = 31;
*/
int getRepeatedInt32(int index);
/**
* repeated int64 repeated_int64 = 32;
*/
java.util.List getRepeatedInt64List();
/**
* repeated int64 repeated_int64 = 32;
*/
int getRepeatedInt64Count();
/**
* repeated int64 repeated_int64 = 32;
*/
long getRepeatedInt64(int index);
/**
* repeated uint32 repeated_uint32 = 33;
*/
java.util.List getRepeatedUint32List();
/**
* repeated uint32 repeated_uint32 = 33;
*/
int getRepeatedUint32Count();
/**
* repeated uint32 repeated_uint32 = 33;
*/
int getRepeatedUint32(int index);
/**
* repeated uint64 repeated_uint64 = 34;
*/
java.util.List getRepeatedUint64List();
/**
* repeated uint64 repeated_uint64 = 34;
*/
int getRepeatedUint64Count();
/**
* repeated uint64 repeated_uint64 = 34;
*/
long getRepeatedUint64(int index);
/**
* repeated sint32 repeated_sint32 = 35;
*/
java.util.List getRepeatedSint32List();
/**
* repeated sint32 repeated_sint32 = 35;
*/
int getRepeatedSint32Count();
/**
* repeated sint32 repeated_sint32 = 35;
*/
int getRepeatedSint32(int index);
/**
* repeated sint64 repeated_sint64 = 36;
*/
java.util.List getRepeatedSint64List();
/**
* repeated sint64 repeated_sint64 = 36;
*/
int getRepeatedSint64Count();
/**
* repeated sint64 repeated_sint64 = 36;
*/
long getRepeatedSint64(int index);
/**
* repeated fixed32 repeated_fixed32 = 37;
*/
java.util.List getRepeatedFixed32List();
/**
* repeated fixed32 repeated_fixed32 = 37;
*/
int getRepeatedFixed32Count();
/**
* repeated fixed32 repeated_fixed32 = 37;
*/
int getRepeatedFixed32(int index);
/**
* repeated fixed64 repeated_fixed64 = 38;
*/
java.util.List getRepeatedFixed64List();
/**
* repeated fixed64 repeated_fixed64 = 38;
*/
int getRepeatedFixed64Count();
/**
* repeated fixed64 repeated_fixed64 = 38;
*/
long getRepeatedFixed64(int index);
/**
* repeated sfixed32 repeated_sfixed32 = 39;
*/
java.util.List getRepeatedSfixed32List();
/**
* repeated sfixed32 repeated_sfixed32 = 39;
*/
int getRepeatedSfixed32Count();
/**
* repeated sfixed32 repeated_sfixed32 = 39;
*/
int getRepeatedSfixed32(int index);
/**
* repeated sfixed64 repeated_sfixed64 = 40;
*/
java.util.List getRepeatedSfixed64List();
/**
* repeated sfixed64 repeated_sfixed64 = 40;
*/
int getRepeatedSfixed64Count();
/**
* repeated sfixed64 repeated_sfixed64 = 40;
*/
long getRepeatedSfixed64(int index);
/**
* repeated float repeated_float = 41;
*/
java.util.List getRepeatedFloatList();
/**
* repeated float repeated_float = 41;
*/
int getRepeatedFloatCount();
/**
* repeated float repeated_float = 41;
*/
float getRepeatedFloat(int index);
/**
* repeated double repeated_double = 42;
*/
java.util.List getRepeatedDoubleList();
/**
* repeated double repeated_double = 42;
*/
int getRepeatedDoubleCount();
/**
* repeated double repeated_double = 42;
*/
double getRepeatedDouble(int index);
/**
* repeated bool repeated_bool = 43;
*/
java.util.List getRepeatedBoolList();
/**
* repeated bool repeated_bool = 43;
*/
int getRepeatedBoolCount();
/**
* repeated bool repeated_bool = 43;
*/
boolean getRepeatedBool(int index);
/**
* repeated string repeated_string = 44;
*/
java.util.List
getRepeatedStringList();
/**
* repeated string repeated_string = 44;
*/
int getRepeatedStringCount();
/**
* repeated string repeated_string = 44;
*/
java.lang.String getRepeatedString(int index);
/**
* repeated string repeated_string = 44;
*/
com.google.protobuf.ByteString
getRepeatedStringBytes(int index);
/**
* repeated bytes repeated_bytes = 45;
*/
java.util.List getRepeatedBytesList();
/**
* repeated bytes repeated_bytes = 45;
*/
int getRepeatedBytesCount();
/**
* repeated bytes repeated_bytes = 45;
*/
com.google.protobuf.ByteString getRepeatedBytes(int index);
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
java.util.List
getRepeatedNestedMessageList();
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
tensorflow.test.Test.TestAllTypes.NestedMessage getRepeatedNestedMessage(int index);
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
int getRepeatedNestedMessageCount();
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
java.util.List extends tensorflow.test.Test.TestAllTypes.NestedMessageOrBuilder>
getRepeatedNestedMessageOrBuilderList();
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
tensorflow.test.Test.TestAllTypes.NestedMessageOrBuilder getRepeatedNestedMessageOrBuilder(
int index);
/**
* repeated .tensorflow.test.TestAllTypes.NestedEnum repeated_nested_enum = 51;
*/
java.util.List getRepeatedNestedEnumList();
/**
* repeated .tensorflow.test.TestAllTypes.NestedEnum repeated_nested_enum = 51;
*/
int getRepeatedNestedEnumCount();
/**
* repeated .tensorflow.test.TestAllTypes.NestedEnum repeated_nested_enum = 51;
*/
tensorflow.test.Test.TestAllTypes.NestedEnum getRepeatedNestedEnum(int index);
/**
* repeated .tensorflow.test.TestAllTypes.NestedEnum repeated_nested_enum = 51;
*/
java.util.List
getRepeatedNestedEnumValueList();
/**
* repeated .tensorflow.test.TestAllTypes.NestedEnum repeated_nested_enum = 51;
*/
int getRepeatedNestedEnumValue(int index);
/**
* repeated string repeated_cord = 55;
*/
java.util.List
getRepeatedCordList();
/**
* repeated string repeated_cord = 55;
*/
int getRepeatedCordCount();
/**
* repeated string repeated_cord = 55;
*/
java.lang.String getRepeatedCord(int index);
/**
* repeated string repeated_cord = 55;
*/
com.google.protobuf.ByteString
getRepeatedCordBytes(int index);
/**
* uint32 oneof_uint32 = 111;
*/
int getOneofUint32();
/**
* .tensorflow.test.TestAllTypes.NestedMessage oneof_nested_message = 112;
*/
boolean hasOneofNestedMessage();
/**
* .tensorflow.test.TestAllTypes.NestedMessage oneof_nested_message = 112;
*/
tensorflow.test.Test.TestAllTypes.NestedMessage getOneofNestedMessage();
/**
* .tensorflow.test.TestAllTypes.NestedMessage oneof_nested_message = 112;
*/
tensorflow.test.Test.TestAllTypes.NestedMessageOrBuilder getOneofNestedMessageOrBuilder();
/**
* string oneof_string = 113;
*/
java.lang.String getOneofString();
/**
* string oneof_string = 113;
*/
com.google.protobuf.ByteString
getOneofStringBytes();
/**
* bytes oneof_bytes = 114;
*/
com.google.protobuf.ByteString getOneofBytes();
/**
* .tensorflow.test.TestAllTypes.NestedEnum oneof_enum = 100;
*/
int getOneofEnumValue();
/**
* .tensorflow.test.TestAllTypes.NestedEnum oneof_enum = 100;
*/
tensorflow.test.Test.TestAllTypes.NestedEnum getOneofEnum();
/**
* map<string, .tensorflow.test.TestAllTypes.NestedMessage> map_string_to_message = 58;
*/
int getMapStringToMessageCount();
/**
* map<string, .tensorflow.test.TestAllTypes.NestedMessage> map_string_to_message = 58;
*/
boolean containsMapStringToMessage(
java.lang.String key);
/**
* Use {@link #getMapStringToMessageMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getMapStringToMessage();
/**
* map<string, .tensorflow.test.TestAllTypes.NestedMessage> map_string_to_message = 58;
*/
java.util.Map
getMapStringToMessageMap();
/**
* map<string, .tensorflow.test.TestAllTypes.NestedMessage> map_string_to_message = 58;
*/
tensorflow.test.Test.TestAllTypes.NestedMessage getMapStringToMessageOrDefault(
java.lang.String key,
tensorflow.test.Test.TestAllTypes.NestedMessage defaultValue);
/**
* map<string, .tensorflow.test.TestAllTypes.NestedMessage> map_string_to_message = 58;
*/
tensorflow.test.Test.TestAllTypes.NestedMessage getMapStringToMessageOrThrow(
java.lang.String key);
/**
* map<int32, .tensorflow.test.TestAllTypes.NestedMessage> map_int32_to_message = 59;
*/
int getMapInt32ToMessageCount();
/**
* map<int32, .tensorflow.test.TestAllTypes.NestedMessage> map_int32_to_message = 59;
*/
boolean containsMapInt32ToMessage(
int key);
/**
* Use {@link #getMapInt32ToMessageMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getMapInt32ToMessage();
/**
* map<int32, .tensorflow.test.TestAllTypes.NestedMessage> map_int32_to_message = 59;
*/
java.util.Map
getMapInt32ToMessageMap();
/**
* map<int32, .tensorflow.test.TestAllTypes.NestedMessage> map_int32_to_message = 59;
*/
tensorflow.test.Test.TestAllTypes.NestedMessage getMapInt32ToMessageOrDefault(
int key,
tensorflow.test.Test.TestAllTypes.NestedMessage defaultValue);
/**
* map<int32, .tensorflow.test.TestAllTypes.NestedMessage> map_int32_to_message = 59;
*/
tensorflow.test.Test.TestAllTypes.NestedMessage getMapInt32ToMessageOrThrow(
int key);
/**
* map<int64, .tensorflow.test.TestAllTypes.NestedMessage> map_int64_to_message = 60;
*/
int getMapInt64ToMessageCount();
/**
* map<int64, .tensorflow.test.TestAllTypes.NestedMessage> map_int64_to_message = 60;
*/
boolean containsMapInt64ToMessage(
long key);
/**
* Use {@link #getMapInt64ToMessageMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getMapInt64ToMessage();
/**
* map<int64, .tensorflow.test.TestAllTypes.NestedMessage> map_int64_to_message = 60;
*/
java.util.Map
getMapInt64ToMessageMap();
/**
* map<int64, .tensorflow.test.TestAllTypes.NestedMessage> map_int64_to_message = 60;
*/
tensorflow.test.Test.TestAllTypes.NestedMessage getMapInt64ToMessageOrDefault(
long key,
tensorflow.test.Test.TestAllTypes.NestedMessage defaultValue);
/**
* map<int64, .tensorflow.test.TestAllTypes.NestedMessage> map_int64_to_message = 60;
*/
tensorflow.test.Test.TestAllTypes.NestedMessage getMapInt64ToMessageOrThrow(
long key);
/**
* map<bool, .tensorflow.test.TestAllTypes.NestedMessage> map_bool_to_message = 61;
*/
int getMapBoolToMessageCount();
/**
* map<bool, .tensorflow.test.TestAllTypes.NestedMessage> map_bool_to_message = 61;
*/
boolean containsMapBoolToMessage(
boolean key);
/**
* Use {@link #getMapBoolToMessageMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getMapBoolToMessage();
/**
* map<bool, .tensorflow.test.TestAllTypes.NestedMessage> map_bool_to_message = 61;
*/
java.util.Map
getMapBoolToMessageMap();
/**
* map<bool, .tensorflow.test.TestAllTypes.NestedMessage> map_bool_to_message = 61;
*/
tensorflow.test.Test.TestAllTypes.NestedMessage getMapBoolToMessageOrDefault(
boolean key,
tensorflow.test.Test.TestAllTypes.NestedMessage defaultValue);
/**
* map<bool, .tensorflow.test.TestAllTypes.NestedMessage> map_bool_to_message = 61;
*/
tensorflow.test.Test.TestAllTypes.NestedMessage getMapBoolToMessageOrThrow(
boolean key);
/**
* map<string, int64> map_string_to_int64 = 62;
*/
int getMapStringToInt64Count();
/**
* map<string, int64> map_string_to_int64 = 62;
*/
boolean containsMapStringToInt64(
java.lang.String key);
/**
* Use {@link #getMapStringToInt64Map()} instead.
*/
@java.lang.Deprecated
java.util.Map
getMapStringToInt64();
/**
* map<string, int64> map_string_to_int64 = 62;
*/
java.util.Map
getMapStringToInt64Map();
/**
* map<string, int64> map_string_to_int64 = 62;
*/
long getMapStringToInt64OrDefault(
java.lang.String key,
long defaultValue);
/**
* map<string, int64> map_string_to_int64 = 62;
*/
long getMapStringToInt64OrThrow(
java.lang.String key);
/**
* map<int64, string> map_int64_to_string = 63;
*/
int getMapInt64ToStringCount();
/**
* map<int64, string> map_int64_to_string = 63;
*/
boolean containsMapInt64ToString(
long key);
/**
* Use {@link #getMapInt64ToStringMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getMapInt64ToString();
/**
* map<int64, string> map_int64_to_string = 63;
*/
java.util.Map
getMapInt64ToStringMap();
/**
* map<int64, string> map_int64_to_string = 63;
*/
java.lang.String getMapInt64ToStringOrDefault(
long key,
java.lang.String defaultValue);
/**
* map<int64, string> map_int64_to_string = 63;
*/
java.lang.String getMapInt64ToStringOrThrow(
long key);
/**
* map<string, .tensorflow.test.TestAllTypes.NestedMessage> another_map_string_to_message = 65;
*/
int getAnotherMapStringToMessageCount();
/**
* map<string, .tensorflow.test.TestAllTypes.NestedMessage> another_map_string_to_message = 65;
*/
boolean containsAnotherMapStringToMessage(
java.lang.String key);
/**
* Use {@link #getAnotherMapStringToMessageMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getAnotherMapStringToMessage();
/**
* map<string, .tensorflow.test.TestAllTypes.NestedMessage> another_map_string_to_message = 65;
*/
java.util.Map
getAnotherMapStringToMessageMap();
/**
* map<string, .tensorflow.test.TestAllTypes.NestedMessage> another_map_string_to_message = 65;
*/
tensorflow.test.Test.TestAllTypes.NestedMessage getAnotherMapStringToMessageOrDefault(
java.lang.String key,
tensorflow.test.Test.TestAllTypes.NestedMessage defaultValue);
/**
* map<string, .tensorflow.test.TestAllTypes.NestedMessage> another_map_string_to_message = 65;
*/
tensorflow.test.Test.TestAllTypes.NestedMessage getAnotherMapStringToMessageOrThrow(
java.lang.String key);
/**
* repeated int64 packed_repeated_int64 = 64 [packed = true];
*/
java.util.List getPackedRepeatedInt64List();
/**
* repeated int64 packed_repeated_int64 = 64 [packed = true];
*/
int getPackedRepeatedInt64Count();
/**
* repeated int64 packed_repeated_int64 = 64 [packed = true];
*/
long getPackedRepeatedInt64(int index);
public tensorflow.test.Test.TestAllTypes.OneofFieldCase getOneofFieldCase();
}
/**
* Protobuf type {@code tensorflow.test.TestAllTypes}
*/
public static final class TestAllTypes extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.test.TestAllTypes)
TestAllTypesOrBuilder {
private static final long serialVersionUID = 0L;
// Use TestAllTypes.newBuilder() to construct.
private TestAllTypes(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TestAllTypes() {
optionalInt32_ = 0;
optionalInt64_ = 0L;
optionalUint32_ = 0;
optionalUint64_ = 0L;
optionalSint32_ = 0;
optionalSint64_ = 0L;
optionalFixed32_ = 0;
optionalFixed64_ = 0L;
optionalSfixed32_ = 0;
optionalSfixed64_ = 0L;
optionalFloat_ = 0F;
optionalDouble_ = 0D;
optionalBool_ = false;
optionalString_ = "";
optionalBytes_ = com.google.protobuf.ByteString.EMPTY;
optionalNestedEnum_ = 0;
optionalForeignEnum_ = 0;
optionalCord_ = "";
repeatedInt32_ = java.util.Collections.emptyList();
repeatedInt64_ = java.util.Collections.emptyList();
repeatedUint32_ = java.util.Collections.emptyList();
repeatedUint64_ = java.util.Collections.emptyList();
repeatedSint32_ = java.util.Collections.emptyList();
repeatedSint64_ = java.util.Collections.emptyList();
repeatedFixed32_ = java.util.Collections.emptyList();
repeatedFixed64_ = java.util.Collections.emptyList();
repeatedSfixed32_ = java.util.Collections.emptyList();
repeatedSfixed64_ = java.util.Collections.emptyList();
repeatedFloat_ = java.util.Collections.emptyList();
repeatedDouble_ = java.util.Collections.emptyList();
repeatedBool_ = java.util.Collections.emptyList();
repeatedString_ = com.google.protobuf.LazyStringArrayList.EMPTY;
repeatedBytes_ = java.util.Collections.emptyList();
repeatedNestedMessage_ = java.util.Collections.emptyList();
repeatedNestedEnum_ = java.util.Collections.emptyList();
repeatedCord_ = com.google.protobuf.LazyStringArrayList.EMPTY;
packedRepeatedInt64_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TestAllTypes(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
int mutable_bitField1_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 16: {
optionalInt64_ = input.readInt64();
break;
}
case 24: {
optionalUint32_ = input.readUInt32();
break;
}
case 40: {
optionalSint32_ = input.readSInt32();
break;
}
case 48: {
optionalSint64_ = input.readSInt64();
break;
}
case 61: {
optionalFixed32_ = input.readFixed32();
break;
}
case 65: {
optionalFixed64_ = input.readFixed64();
break;
}
case 77: {
optionalSfixed32_ = input.readSFixed32();
break;
}
case 81: {
optionalSfixed64_ = input.readSFixed64();
break;
}
case 93: {
optionalFloat_ = input.readFloat();
break;
}
case 97: {
optionalDouble_ = input.readDouble();
break;
}
case 104: {
optionalBool_ = input.readBool();
break;
}
case 114: {
java.lang.String s = input.readStringRequireUtf8();
optionalString_ = s;
break;
}
case 122: {
optionalBytes_ = input.readBytes();
break;
}
case 146: {
tensorflow.test.Test.TestAllTypes.NestedMessage.Builder subBuilder = null;
if (optionalNestedMessage_ != null) {
subBuilder = optionalNestedMessage_.toBuilder();
}
optionalNestedMessage_ = input.readMessage(tensorflow.test.Test.TestAllTypes.NestedMessage.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(optionalNestedMessage_);
optionalNestedMessage_ = subBuilder.buildPartial();
}
break;
}
case 154: {
tensorflow.test.Test.ForeignMessage.Builder subBuilder = null;
if (optionalForeignMessage_ != null) {
subBuilder = optionalForeignMessage_.toBuilder();
}
optionalForeignMessage_ = input.readMessage(tensorflow.test.Test.ForeignMessage.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(optionalForeignMessage_);
optionalForeignMessage_ = subBuilder.buildPartial();
}
break;
}
case 168: {
int rawValue = input.readEnum();
optionalNestedEnum_ = rawValue;
break;
}
case 176: {
int rawValue = input.readEnum();
optionalForeignEnum_ = rawValue;
break;
}
case 202: {
java.lang.String s = input.readStringRequireUtf8();
optionalCord_ = s;
break;
}
case 248: {
if (!((mutable_bitField0_ & 0x00100000) == 0x00100000)) {
repeatedInt32_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00100000;
}
repeatedInt32_.add(input.readInt32());
break;
}
case 250: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00100000) == 0x00100000) && input.getBytesUntilLimit() > 0) {
repeatedInt32_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00100000;
}
while (input.getBytesUntilLimit() > 0) {
repeatedInt32_.add(input.readInt32());
}
input.popLimit(limit);
break;
}
case 256: {
if (!((mutable_bitField0_ & 0x00200000) == 0x00200000)) {
repeatedInt64_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00200000;
}
repeatedInt64_.add(input.readInt64());
break;
}
case 258: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00200000) == 0x00200000) && input.getBytesUntilLimit() > 0) {
repeatedInt64_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00200000;
}
while (input.getBytesUntilLimit() > 0) {
repeatedInt64_.add(input.readInt64());
}
input.popLimit(limit);
break;
}
case 264: {
if (!((mutable_bitField0_ & 0x00400000) == 0x00400000)) {
repeatedUint32_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00400000;
}
repeatedUint32_.add(input.readUInt32());
break;
}
case 266: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00400000) == 0x00400000) && input.getBytesUntilLimit() > 0) {
repeatedUint32_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00400000;
}
while (input.getBytesUntilLimit() > 0) {
repeatedUint32_.add(input.readUInt32());
}
input.popLimit(limit);
break;
}
case 272: {
if (!((mutable_bitField0_ & 0x00800000) == 0x00800000)) {
repeatedUint64_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00800000;
}
repeatedUint64_.add(input.readUInt64());
break;
}
case 274: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00800000) == 0x00800000) && input.getBytesUntilLimit() > 0) {
repeatedUint64_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00800000;
}
while (input.getBytesUntilLimit() > 0) {
repeatedUint64_.add(input.readUInt64());
}
input.popLimit(limit);
break;
}
case 280: {
if (!((mutable_bitField0_ & 0x01000000) == 0x01000000)) {
repeatedSint32_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x01000000;
}
repeatedSint32_.add(input.readSInt32());
break;
}
case 282: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x01000000) == 0x01000000) && input.getBytesUntilLimit() > 0) {
repeatedSint32_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x01000000;
}
while (input.getBytesUntilLimit() > 0) {
repeatedSint32_.add(input.readSInt32());
}
input.popLimit(limit);
break;
}
case 288: {
if (!((mutable_bitField0_ & 0x02000000) == 0x02000000)) {
repeatedSint64_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x02000000;
}
repeatedSint64_.add(input.readSInt64());
break;
}
case 290: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x02000000) == 0x02000000) && input.getBytesUntilLimit() > 0) {
repeatedSint64_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x02000000;
}
while (input.getBytesUntilLimit() > 0) {
repeatedSint64_.add(input.readSInt64());
}
input.popLimit(limit);
break;
}
case 301: {
if (!((mutable_bitField0_ & 0x04000000) == 0x04000000)) {
repeatedFixed32_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x04000000;
}
repeatedFixed32_.add(input.readFixed32());
break;
}
case 298: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x04000000) == 0x04000000) && input.getBytesUntilLimit() > 0) {
repeatedFixed32_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x04000000;
}
while (input.getBytesUntilLimit() > 0) {
repeatedFixed32_.add(input.readFixed32());
}
input.popLimit(limit);
break;
}
case 305: {
if (!((mutable_bitField0_ & 0x08000000) == 0x08000000)) {
repeatedFixed64_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x08000000;
}
repeatedFixed64_.add(input.readFixed64());
break;
}
case 306: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x08000000) == 0x08000000) && input.getBytesUntilLimit() > 0) {
repeatedFixed64_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x08000000;
}
while (input.getBytesUntilLimit() > 0) {
repeatedFixed64_.add(input.readFixed64());
}
input.popLimit(limit);
break;
}
case 317: {
if (!((mutable_bitField0_ & 0x10000000) == 0x10000000)) {
repeatedSfixed32_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x10000000;
}
repeatedSfixed32_.add(input.readSFixed32());
break;
}
case 314: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x10000000) == 0x10000000) && input.getBytesUntilLimit() > 0) {
repeatedSfixed32_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x10000000;
}
while (input.getBytesUntilLimit() > 0) {
repeatedSfixed32_.add(input.readSFixed32());
}
input.popLimit(limit);
break;
}
case 321: {
if (!((mutable_bitField0_ & 0x20000000) == 0x20000000)) {
repeatedSfixed64_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x20000000;
}
repeatedSfixed64_.add(input.readSFixed64());
break;
}
case 322: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x20000000) == 0x20000000) && input.getBytesUntilLimit() > 0) {
repeatedSfixed64_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x20000000;
}
while (input.getBytesUntilLimit() > 0) {
repeatedSfixed64_.add(input.readSFixed64());
}
input.popLimit(limit);
break;
}
case 333: {
if (!((mutable_bitField0_ & 0x40000000) == 0x40000000)) {
repeatedFloat_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x40000000;
}
repeatedFloat_.add(input.readFloat());
break;
}
case 330: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x40000000) == 0x40000000) && input.getBytesUntilLimit() > 0) {
repeatedFloat_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x40000000;
}
while (input.getBytesUntilLimit() > 0) {
repeatedFloat_.add(input.readFloat());
}
input.popLimit(limit);
break;
}
case 337: {
if (!((mutable_bitField0_ & 0x80000000) == 0x80000000)) {
repeatedDouble_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x80000000;
}
repeatedDouble_.add(input.readDouble());
break;
}
case 338: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x80000000) == 0x80000000) && input.getBytesUntilLimit() > 0) {
repeatedDouble_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x80000000;
}
while (input.getBytesUntilLimit() > 0) {
repeatedDouble_.add(input.readDouble());
}
input.popLimit(limit);
break;
}
case 344: {
if (!((mutable_bitField1_ & 0x00000001) == 0x00000001)) {
repeatedBool_ = new java.util.ArrayList();
mutable_bitField1_ |= 0x00000001;
}
repeatedBool_.add(input.readBool());
break;
}
case 346: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField1_ & 0x00000001) == 0x00000001) && input.getBytesUntilLimit() > 0) {
repeatedBool_ = new java.util.ArrayList();
mutable_bitField1_ |= 0x00000001;
}
while (input.getBytesUntilLimit() > 0) {
repeatedBool_.add(input.readBool());
}
input.popLimit(limit);
break;
}
case 354: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField1_ & 0x00000002) == 0x00000002)) {
repeatedString_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField1_ |= 0x00000002;
}
repeatedString_.add(s);
break;
}
case 362: {
if (!((mutable_bitField1_ & 0x00000004) == 0x00000004)) {
repeatedBytes_ = new java.util.ArrayList();
mutable_bitField1_ |= 0x00000004;
}
repeatedBytes_.add(input.readBytes());
break;
}
case 386: {
if (!((mutable_bitField1_ & 0x00000008) == 0x00000008)) {
repeatedNestedMessage_ = new java.util.ArrayList();
mutable_bitField1_ |= 0x00000008;
}
repeatedNestedMessage_.add(
input.readMessage(tensorflow.test.Test.TestAllTypes.NestedMessage.parser(), extensionRegistry));
break;
}
case 408: {
int rawValue = input.readEnum();
if (!((mutable_bitField1_ & 0x00000010) == 0x00000010)) {
repeatedNestedEnum_ = new java.util.ArrayList();
mutable_bitField1_ |= 0x00000010;
}
repeatedNestedEnum_.add(rawValue);
break;
}
case 410: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int rawValue = input.readEnum();
if (!((mutable_bitField1_ & 0x00000010) == 0x00000010)) {
repeatedNestedEnum_ = new java.util.ArrayList();
mutable_bitField1_ |= 0x00000010;
}
repeatedNestedEnum_.add(rawValue);
}
input.popLimit(oldLimit);
break;
}
case 442: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField1_ & 0x00000020) == 0x00000020)) {
repeatedCord_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField1_ |= 0x00000020;
}
repeatedCord_.add(s);
break;
}
case 466: {
if (!((mutable_bitField1_ & 0x00000800) == 0x00000800)) {
mapStringToMessage_ = com.google.protobuf.MapField.newMapField(
MapStringToMessageDefaultEntryHolder.defaultEntry);
mutable_bitField1_ |= 0x00000800;
}
com.google.protobuf.MapEntry
mapStringToMessage__ = input.readMessage(
MapStringToMessageDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
mapStringToMessage_.getMutableMap().put(
mapStringToMessage__.getKey(), mapStringToMessage__.getValue());
break;
}
case 474: {
if (!((mutable_bitField1_ & 0x00001000) == 0x00001000)) {
mapInt32ToMessage_ = com.google.protobuf.MapField.newMapField(
MapInt32ToMessageDefaultEntryHolder.defaultEntry);
mutable_bitField1_ |= 0x00001000;
}
com.google.protobuf.MapEntry
mapInt32ToMessage__ = input.readMessage(
MapInt32ToMessageDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
mapInt32ToMessage_.getMutableMap().put(
mapInt32ToMessage__.getKey(), mapInt32ToMessage__.getValue());
break;
}
case 482: {
if (!((mutable_bitField1_ & 0x00002000) == 0x00002000)) {
mapInt64ToMessage_ = com.google.protobuf.MapField.newMapField(
MapInt64ToMessageDefaultEntryHolder.defaultEntry);
mutable_bitField1_ |= 0x00002000;
}
com.google.protobuf.MapEntry
mapInt64ToMessage__ = input.readMessage(
MapInt64ToMessageDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
mapInt64ToMessage_.getMutableMap().put(
mapInt64ToMessage__.getKey(), mapInt64ToMessage__.getValue());
break;
}
case 490: {
if (!((mutable_bitField1_ & 0x00004000) == 0x00004000)) {
mapBoolToMessage_ = com.google.protobuf.MapField.newMapField(
MapBoolToMessageDefaultEntryHolder.defaultEntry);
mutable_bitField1_ |= 0x00004000;
}
com.google.protobuf.MapEntry
mapBoolToMessage__ = input.readMessage(
MapBoolToMessageDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
mapBoolToMessage_.getMutableMap().put(
mapBoolToMessage__.getKey(), mapBoolToMessage__.getValue());
break;
}
case 498: {
if (!((mutable_bitField1_ & 0x00008000) == 0x00008000)) {
mapStringToInt64_ = com.google.protobuf.MapField.newMapField(
MapStringToInt64DefaultEntryHolder.defaultEntry);
mutable_bitField1_ |= 0x00008000;
}
com.google.protobuf.MapEntry
mapStringToInt64__ = input.readMessage(
MapStringToInt64DefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
mapStringToInt64_.getMutableMap().put(
mapStringToInt64__.getKey(), mapStringToInt64__.getValue());
break;
}
case 506: {
if (!((mutable_bitField1_ & 0x00010000) == 0x00010000)) {
mapInt64ToString_ = com.google.protobuf.MapField.newMapField(
MapInt64ToStringDefaultEntryHolder.defaultEntry);
mutable_bitField1_ |= 0x00010000;
}
com.google.protobuf.MapEntry
mapInt64ToString__ = input.readMessage(
MapInt64ToStringDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
mapInt64ToString_.getMutableMap().put(
mapInt64ToString__.getKey(), mapInt64ToString__.getValue());
break;
}
case 512: {
if (!((mutable_bitField1_ & 0x00040000) == 0x00040000)) {
packedRepeatedInt64_ = new java.util.ArrayList();
mutable_bitField1_ |= 0x00040000;
}
packedRepeatedInt64_.add(input.readInt64());
break;
}
case 514: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField1_ & 0x00040000) == 0x00040000) && input.getBytesUntilLimit() > 0) {
packedRepeatedInt64_ = new java.util.ArrayList();
mutable_bitField1_ |= 0x00040000;
}
while (input.getBytesUntilLimit() > 0) {
packedRepeatedInt64_.add(input.readInt64());
}
input.popLimit(limit);
break;
}
case 522: {
if (!((mutable_bitField1_ & 0x00020000) == 0x00020000)) {
anotherMapStringToMessage_ = com.google.protobuf.MapField.newMapField(
AnotherMapStringToMessageDefaultEntryHolder.defaultEntry);
mutable_bitField1_ |= 0x00020000;
}
com.google.protobuf.MapEntry
anotherMapStringToMessage__ = input.readMessage(
AnotherMapStringToMessageDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
anotherMapStringToMessage_.getMutableMap().put(
anotherMapStringToMessage__.getKey(), anotherMapStringToMessage__.getValue());
break;
}
case 800: {
int rawValue = input.readEnum();
oneofFieldCase_ = 100;
oneofField_ = rawValue;
break;
}
case 888: {
oneofFieldCase_ = 111;
oneofField_ = input.readUInt32();
break;
}
case 898: {
tensorflow.test.Test.TestAllTypes.NestedMessage.Builder subBuilder = null;
if (oneofFieldCase_ == 112) {
subBuilder = ((tensorflow.test.Test.TestAllTypes.NestedMessage) oneofField_).toBuilder();
}
oneofField_ =
input.readMessage(tensorflow.test.Test.TestAllTypes.NestedMessage.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((tensorflow.test.Test.TestAllTypes.NestedMessage) oneofField_);
oneofField_ = subBuilder.buildPartial();
}
oneofFieldCase_ = 112;
break;
}
case 906: {
java.lang.String s = input.readStringRequireUtf8();
oneofFieldCase_ = 113;
oneofField_ = s;
break;
}
case 914: {
oneofFieldCase_ = 114;
oneofField_ = input.readBytes();
break;
}
case 7992: {
optionalUint64_ = input.readUInt64();
break;
}
case 8000: {
optionalInt32_ = input.readInt32();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00100000) == 0x00100000)) {
repeatedInt32_ = java.util.Collections.unmodifiableList(repeatedInt32_);
}
if (((mutable_bitField0_ & 0x00200000) == 0x00200000)) {
repeatedInt64_ = java.util.Collections.unmodifiableList(repeatedInt64_);
}
if (((mutable_bitField0_ & 0x00400000) == 0x00400000)) {
repeatedUint32_ = java.util.Collections.unmodifiableList(repeatedUint32_);
}
if (((mutable_bitField0_ & 0x00800000) == 0x00800000)) {
repeatedUint64_ = java.util.Collections.unmodifiableList(repeatedUint64_);
}
if (((mutable_bitField0_ & 0x01000000) == 0x01000000)) {
repeatedSint32_ = java.util.Collections.unmodifiableList(repeatedSint32_);
}
if (((mutable_bitField0_ & 0x02000000) == 0x02000000)) {
repeatedSint64_ = java.util.Collections.unmodifiableList(repeatedSint64_);
}
if (((mutable_bitField0_ & 0x04000000) == 0x04000000)) {
repeatedFixed32_ = java.util.Collections.unmodifiableList(repeatedFixed32_);
}
if (((mutable_bitField0_ & 0x08000000) == 0x08000000)) {
repeatedFixed64_ = java.util.Collections.unmodifiableList(repeatedFixed64_);
}
if (((mutable_bitField0_ & 0x10000000) == 0x10000000)) {
repeatedSfixed32_ = java.util.Collections.unmodifiableList(repeatedSfixed32_);
}
if (((mutable_bitField0_ & 0x20000000) == 0x20000000)) {
repeatedSfixed64_ = java.util.Collections.unmodifiableList(repeatedSfixed64_);
}
if (((mutable_bitField0_ & 0x40000000) == 0x40000000)) {
repeatedFloat_ = java.util.Collections.unmodifiableList(repeatedFloat_);
}
if (((mutable_bitField0_ & 0x80000000) == 0x80000000)) {
repeatedDouble_ = java.util.Collections.unmodifiableList(repeatedDouble_);
}
if (((mutable_bitField1_ & 0x00000001) == 0x00000001)) {
repeatedBool_ = java.util.Collections.unmodifiableList(repeatedBool_);
}
if (((mutable_bitField1_ & 0x00000002) == 0x00000002)) {
repeatedString_ = repeatedString_.getUnmodifiableView();
}
if (((mutable_bitField1_ & 0x00000004) == 0x00000004)) {
repeatedBytes_ = java.util.Collections.unmodifiableList(repeatedBytes_);
}
if (((mutable_bitField1_ & 0x00000008) == 0x00000008)) {
repeatedNestedMessage_ = java.util.Collections.unmodifiableList(repeatedNestedMessage_);
}
if (((mutable_bitField1_ & 0x00000010) == 0x00000010)) {
repeatedNestedEnum_ = java.util.Collections.unmodifiableList(repeatedNestedEnum_);
}
if (((mutable_bitField1_ & 0x00000020) == 0x00000020)) {
repeatedCord_ = repeatedCord_.getUnmodifiableView();
}
if (((mutable_bitField1_ & 0x00040000) == 0x00040000)) {
packedRepeatedInt64_ = java.util.Collections.unmodifiableList(packedRepeatedInt64_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.test.Test.internal_static_tensorflow_test_TestAllTypes_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 58:
return internalGetMapStringToMessage();
case 59:
return internalGetMapInt32ToMessage();
case 60:
return internalGetMapInt64ToMessage();
case 61:
return internalGetMapBoolToMessage();
case 62:
return internalGetMapStringToInt64();
case 63:
return internalGetMapInt64ToString();
case 65:
return internalGetAnotherMapStringToMessage();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.test.Test.internal_static_tensorflow_test_TestAllTypes_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.test.Test.TestAllTypes.class, tensorflow.test.Test.TestAllTypes.Builder.class);
}
/**
* Protobuf enum {@code tensorflow.test.TestAllTypes.NestedEnum}
*/
public enum NestedEnum
implements com.google.protobuf.ProtocolMessageEnum {
/**
* ZERO = 0;
*/
ZERO(0),
/**
* FOO = 1;
*/
FOO(1),
/**
* BAR = 2;
*/
BAR(2),
/**
* BAZ = 3;
*/
BAZ(3),
/**
*
* Intentionally negative.
*
*
* NEG = -1;
*/
NEG(-1),
UNRECOGNIZED(-1),
;
/**
* ZERO = 0;
*/
public static final int ZERO_VALUE = 0;
/**
* FOO = 1;
*/
public static final int FOO_VALUE = 1;
/**
* BAR = 2;
*/
public static final int BAR_VALUE = 2;
/**
* BAZ = 3;
*/
public static final int BAZ_VALUE = 3;
/**
*
* Intentionally negative.
*
*
* NEG = -1;
*/
public static final int NEG_VALUE = -1;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static NestedEnum valueOf(int value) {
return forNumber(value);
}
public static NestedEnum forNumber(int value) {
switch (value) {
case 0: return ZERO;
case 1: return FOO;
case 2: return BAR;
case 3: return BAZ;
case -1: return NEG;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
NestedEnum> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public NestedEnum findValueByNumber(int number) {
return NestedEnum.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return tensorflow.test.Test.TestAllTypes.getDescriptor().getEnumTypes().get(0);
}
private static final NestedEnum[] VALUES = values();
public static NestedEnum valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private NestedEnum(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:tensorflow.test.TestAllTypes.NestedEnum)
}
public interface NestedMessageOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.test.TestAllTypes.NestedMessage)
com.google.protobuf.MessageOrBuilder {
/**
* int32 optional_int32 = 1;
*/
int getOptionalInt32();
/**
* repeated int32 repeated_int32 = 2;
*/
java.util.List getRepeatedInt32List();
/**
* repeated int32 repeated_int32 = 2;
*/
int getRepeatedInt32Count();
/**
* repeated int32 repeated_int32 = 2;
*/
int getRepeatedInt32(int index);
/**
* .tensorflow.test.TestAllTypes.NestedMessage.DoubleNestedMessage msg = 3;
*/
boolean hasMsg();
/**
* .tensorflow.test.TestAllTypes.NestedMessage.DoubleNestedMessage msg = 3;
*/
tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage getMsg();
/**
* .tensorflow.test.TestAllTypes.NestedMessage.DoubleNestedMessage msg = 3;
*/
tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessageOrBuilder getMsgOrBuilder();
/**
* int64 optional_int64 = 4;
*/
long getOptionalInt64();
}
/**
* Protobuf type {@code tensorflow.test.TestAllTypes.NestedMessage}
*/
public static final class NestedMessage extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.test.TestAllTypes.NestedMessage)
NestedMessageOrBuilder {
private static final long serialVersionUID = 0L;
// Use NestedMessage.newBuilder() to construct.
private NestedMessage(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private NestedMessage() {
optionalInt32_ = 0;
repeatedInt32_ = java.util.Collections.emptyList();
optionalInt64_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private NestedMessage(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
optionalInt32_ = input.readInt32();
break;
}
case 16: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
repeatedInt32_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
repeatedInt32_.add(input.readInt32());
break;
}
case 18: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002) && input.getBytesUntilLimit() > 0) {
repeatedInt32_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
while (input.getBytesUntilLimit() > 0) {
repeatedInt32_.add(input.readInt32());
}
input.popLimit(limit);
break;
}
case 26: {
tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage.Builder subBuilder = null;
if (msg_ != null) {
subBuilder = msg_.toBuilder();
}
msg_ = input.readMessage(tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(msg_);
msg_ = subBuilder.buildPartial();
}
break;
}
case 32: {
optionalInt64_ = input.readInt64();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
repeatedInt32_ = java.util.Collections.unmodifiableList(repeatedInt32_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.test.Test.internal_static_tensorflow_test_TestAllTypes_NestedMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.test.Test.internal_static_tensorflow_test_TestAllTypes_NestedMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.test.Test.TestAllTypes.NestedMessage.class, tensorflow.test.Test.TestAllTypes.NestedMessage.Builder.class);
}
public interface DoubleNestedMessageOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.test.TestAllTypes.NestedMessage.DoubleNestedMessage)
com.google.protobuf.MessageOrBuilder {
/**
* string optional_string = 1;
*/
java.lang.String getOptionalString();
/**
* string optional_string = 1;
*/
com.google.protobuf.ByteString
getOptionalStringBytes();
}
/**
* Protobuf type {@code tensorflow.test.TestAllTypes.NestedMessage.DoubleNestedMessage}
*/
public static final class DoubleNestedMessage extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.test.TestAllTypes.NestedMessage.DoubleNestedMessage)
DoubleNestedMessageOrBuilder {
private static final long serialVersionUID = 0L;
// Use DoubleNestedMessage.newBuilder() to construct.
private DoubleNestedMessage(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DoubleNestedMessage() {
optionalString_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DoubleNestedMessage(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
optionalString_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.test.Test.internal_static_tensorflow_test_TestAllTypes_NestedMessage_DoubleNestedMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.test.Test.internal_static_tensorflow_test_TestAllTypes_NestedMessage_DoubleNestedMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage.class, tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage.Builder.class);
}
public static final int OPTIONAL_STRING_FIELD_NUMBER = 1;
private volatile java.lang.Object optionalString_;
/**
* string optional_string = 1;
*/
public java.lang.String getOptionalString() {
java.lang.Object ref = optionalString_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
optionalString_ = s;
return s;
}
}
/**
* string optional_string = 1;
*/
public com.google.protobuf.ByteString
getOptionalStringBytes() {
java.lang.Object ref = optionalString_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
optionalString_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getOptionalStringBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, optionalString_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getOptionalStringBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, optionalString_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage)) {
return super.equals(obj);
}
tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage other = (tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage) obj;
boolean result = true;
result = result && getOptionalString()
.equals(other.getOptionalString());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + OPTIONAL_STRING_FIELD_NUMBER;
hash = (53 * hash) + getOptionalString().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.test.TestAllTypes.NestedMessage.DoubleNestedMessage}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.test.TestAllTypes.NestedMessage.DoubleNestedMessage)
tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessageOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.test.Test.internal_static_tensorflow_test_TestAllTypes_NestedMessage_DoubleNestedMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.test.Test.internal_static_tensorflow_test_TestAllTypes_NestedMessage_DoubleNestedMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage.class, tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage.Builder.class);
}
// Construct using tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
optionalString_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.test.Test.internal_static_tensorflow_test_TestAllTypes_NestedMessage_DoubleNestedMessage_descriptor;
}
@java.lang.Override
public tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage getDefaultInstanceForType() {
return tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage.getDefaultInstance();
}
@java.lang.Override
public tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage build() {
tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage buildPartial() {
tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage result = new tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage(this);
result.optionalString_ = optionalString_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage) {
return mergeFrom((tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage other) {
if (other == tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage.getDefaultInstance()) return this;
if (!other.getOptionalString().isEmpty()) {
optionalString_ = other.optionalString_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object optionalString_ = "";
/**
* string optional_string = 1;
*/
public java.lang.String getOptionalString() {
java.lang.Object ref = optionalString_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
optionalString_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string optional_string = 1;
*/
public com.google.protobuf.ByteString
getOptionalStringBytes() {
java.lang.Object ref = optionalString_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
optionalString_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string optional_string = 1;
*/
public Builder setOptionalString(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
optionalString_ = value;
onChanged();
return this;
}
/**
* string optional_string = 1;
*/
public Builder clearOptionalString() {
optionalString_ = getDefaultInstance().getOptionalString();
onChanged();
return this;
}
/**
* string optional_string = 1;
*/
public Builder setOptionalStringBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
optionalString_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.test.TestAllTypes.NestedMessage.DoubleNestedMessage)
}
// @@protoc_insertion_point(class_scope:tensorflow.test.TestAllTypes.NestedMessage.DoubleNestedMessage)
private static final tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage();
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DoubleNestedMessage parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DoubleNestedMessage(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int OPTIONAL_INT32_FIELD_NUMBER = 1;
private int optionalInt32_;
/**
* int32 optional_int32 = 1;
*/
public int getOptionalInt32() {
return optionalInt32_;
}
public static final int REPEATED_INT32_FIELD_NUMBER = 2;
private java.util.List repeatedInt32_;
/**
* repeated int32 repeated_int32 = 2;
*/
public java.util.List
getRepeatedInt32List() {
return repeatedInt32_;
}
/**
* repeated int32 repeated_int32 = 2;
*/
public int getRepeatedInt32Count() {
return repeatedInt32_.size();
}
/**
* repeated int32 repeated_int32 = 2;
*/
public int getRepeatedInt32(int index) {
return repeatedInt32_.get(index);
}
private int repeatedInt32MemoizedSerializedSize = -1;
public static final int MSG_FIELD_NUMBER = 3;
private tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage msg_;
/**
* .tensorflow.test.TestAllTypes.NestedMessage.DoubleNestedMessage msg = 3;
*/
public boolean hasMsg() {
return msg_ != null;
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage.DoubleNestedMessage msg = 3;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage getMsg() {
return msg_ == null ? tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage.getDefaultInstance() : msg_;
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage.DoubleNestedMessage msg = 3;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessageOrBuilder getMsgOrBuilder() {
return getMsg();
}
public static final int OPTIONAL_INT64_FIELD_NUMBER = 4;
private long optionalInt64_;
/**
* int64 optional_int64 = 4;
*/
public long getOptionalInt64() {
return optionalInt64_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (optionalInt32_ != 0) {
output.writeInt32(1, optionalInt32_);
}
if (getRepeatedInt32List().size() > 0) {
output.writeUInt32NoTag(18);
output.writeUInt32NoTag(repeatedInt32MemoizedSerializedSize);
}
for (int i = 0; i < repeatedInt32_.size(); i++) {
output.writeInt32NoTag(repeatedInt32_.get(i));
}
if (msg_ != null) {
output.writeMessage(3, getMsg());
}
if (optionalInt64_ != 0L) {
output.writeInt64(4, optionalInt64_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (optionalInt32_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, optionalInt32_);
}
{
int dataSize = 0;
for (int i = 0; i < repeatedInt32_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(repeatedInt32_.get(i));
}
size += dataSize;
if (!getRepeatedInt32List().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
repeatedInt32MemoizedSerializedSize = dataSize;
}
if (msg_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getMsg());
}
if (optionalInt64_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, optionalInt64_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.test.Test.TestAllTypes.NestedMessage)) {
return super.equals(obj);
}
tensorflow.test.Test.TestAllTypes.NestedMessage other = (tensorflow.test.Test.TestAllTypes.NestedMessage) obj;
boolean result = true;
result = result && (getOptionalInt32()
== other.getOptionalInt32());
result = result && getRepeatedInt32List()
.equals(other.getRepeatedInt32List());
result = result && (hasMsg() == other.hasMsg());
if (hasMsg()) {
result = result && getMsg()
.equals(other.getMsg());
}
result = result && (getOptionalInt64()
== other.getOptionalInt64());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + OPTIONAL_INT32_FIELD_NUMBER;
hash = (53 * hash) + getOptionalInt32();
if (getRepeatedInt32Count() > 0) {
hash = (37 * hash) + REPEATED_INT32_FIELD_NUMBER;
hash = (53 * hash) + getRepeatedInt32List().hashCode();
}
if (hasMsg()) {
hash = (37 * hash) + MSG_FIELD_NUMBER;
hash = (53 * hash) + getMsg().hashCode();
}
hash = (37 * hash) + OPTIONAL_INT64_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOptionalInt64());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.test.Test.TestAllTypes.NestedMessage prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.test.TestAllTypes.NestedMessage}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.test.TestAllTypes.NestedMessage)
tensorflow.test.Test.TestAllTypes.NestedMessageOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.test.Test.internal_static_tensorflow_test_TestAllTypes_NestedMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.test.Test.internal_static_tensorflow_test_TestAllTypes_NestedMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.test.Test.TestAllTypes.NestedMessage.class, tensorflow.test.Test.TestAllTypes.NestedMessage.Builder.class);
}
// Construct using tensorflow.test.Test.TestAllTypes.NestedMessage.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
optionalInt32_ = 0;
repeatedInt32_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
if (msgBuilder_ == null) {
msg_ = null;
} else {
msg_ = null;
msgBuilder_ = null;
}
optionalInt64_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.test.Test.internal_static_tensorflow_test_TestAllTypes_NestedMessage_descriptor;
}
@java.lang.Override
public tensorflow.test.Test.TestAllTypes.NestedMessage getDefaultInstanceForType() {
return tensorflow.test.Test.TestAllTypes.NestedMessage.getDefaultInstance();
}
@java.lang.Override
public tensorflow.test.Test.TestAllTypes.NestedMessage build() {
tensorflow.test.Test.TestAllTypes.NestedMessage result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.test.Test.TestAllTypes.NestedMessage buildPartial() {
tensorflow.test.Test.TestAllTypes.NestedMessage result = new tensorflow.test.Test.TestAllTypes.NestedMessage(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.optionalInt32_ = optionalInt32_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
repeatedInt32_ = java.util.Collections.unmodifiableList(repeatedInt32_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.repeatedInt32_ = repeatedInt32_;
if (msgBuilder_ == null) {
result.msg_ = msg_;
} else {
result.msg_ = msgBuilder_.build();
}
result.optionalInt64_ = optionalInt64_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.test.Test.TestAllTypes.NestedMessage) {
return mergeFrom((tensorflow.test.Test.TestAllTypes.NestedMessage)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.test.Test.TestAllTypes.NestedMessage other) {
if (other == tensorflow.test.Test.TestAllTypes.NestedMessage.getDefaultInstance()) return this;
if (other.getOptionalInt32() != 0) {
setOptionalInt32(other.getOptionalInt32());
}
if (!other.repeatedInt32_.isEmpty()) {
if (repeatedInt32_.isEmpty()) {
repeatedInt32_ = other.repeatedInt32_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureRepeatedInt32IsMutable();
repeatedInt32_.addAll(other.repeatedInt32_);
}
onChanged();
}
if (other.hasMsg()) {
mergeMsg(other.getMsg());
}
if (other.getOptionalInt64() != 0L) {
setOptionalInt64(other.getOptionalInt64());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.test.Test.TestAllTypes.NestedMessage parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.test.Test.TestAllTypes.NestedMessage) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int optionalInt32_ ;
/**
* int32 optional_int32 = 1;
*/
public int getOptionalInt32() {
return optionalInt32_;
}
/**
* int32 optional_int32 = 1;
*/
public Builder setOptionalInt32(int value) {
optionalInt32_ = value;
onChanged();
return this;
}
/**
* int32 optional_int32 = 1;
*/
public Builder clearOptionalInt32() {
optionalInt32_ = 0;
onChanged();
return this;
}
private java.util.List repeatedInt32_ = java.util.Collections.emptyList();
private void ensureRepeatedInt32IsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
repeatedInt32_ = new java.util.ArrayList(repeatedInt32_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated int32 repeated_int32 = 2;
*/
public java.util.List
getRepeatedInt32List() {
return java.util.Collections.unmodifiableList(repeatedInt32_);
}
/**
* repeated int32 repeated_int32 = 2;
*/
public int getRepeatedInt32Count() {
return repeatedInt32_.size();
}
/**
* repeated int32 repeated_int32 = 2;
*/
public int getRepeatedInt32(int index) {
return repeatedInt32_.get(index);
}
/**
* repeated int32 repeated_int32 = 2;
*/
public Builder setRepeatedInt32(
int index, int value) {
ensureRepeatedInt32IsMutable();
repeatedInt32_.set(index, value);
onChanged();
return this;
}
/**
* repeated int32 repeated_int32 = 2;
*/
public Builder addRepeatedInt32(int value) {
ensureRepeatedInt32IsMutable();
repeatedInt32_.add(value);
onChanged();
return this;
}
/**
* repeated int32 repeated_int32 = 2;
*/
public Builder addAllRepeatedInt32(
java.lang.Iterable extends java.lang.Integer> values) {
ensureRepeatedInt32IsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, repeatedInt32_);
onChanged();
return this;
}
/**
* repeated int32 repeated_int32 = 2;
*/
public Builder clearRepeatedInt32() {
repeatedInt32_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
private tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage msg_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage, tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage.Builder, tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessageOrBuilder> msgBuilder_;
/**
* .tensorflow.test.TestAllTypes.NestedMessage.DoubleNestedMessage msg = 3;
*/
public boolean hasMsg() {
return msgBuilder_ != null || msg_ != null;
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage.DoubleNestedMessage msg = 3;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage getMsg() {
if (msgBuilder_ == null) {
return msg_ == null ? tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage.getDefaultInstance() : msg_;
} else {
return msgBuilder_.getMessage();
}
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage.DoubleNestedMessage msg = 3;
*/
public Builder setMsg(tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage value) {
if (msgBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
msg_ = value;
onChanged();
} else {
msgBuilder_.setMessage(value);
}
return this;
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage.DoubleNestedMessage msg = 3;
*/
public Builder setMsg(
tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage.Builder builderForValue) {
if (msgBuilder_ == null) {
msg_ = builderForValue.build();
onChanged();
} else {
msgBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage.DoubleNestedMessage msg = 3;
*/
public Builder mergeMsg(tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage value) {
if (msgBuilder_ == null) {
if (msg_ != null) {
msg_ =
tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage.newBuilder(msg_).mergeFrom(value).buildPartial();
} else {
msg_ = value;
}
onChanged();
} else {
msgBuilder_.mergeFrom(value);
}
return this;
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage.DoubleNestedMessage msg = 3;
*/
public Builder clearMsg() {
if (msgBuilder_ == null) {
msg_ = null;
onChanged();
} else {
msg_ = null;
msgBuilder_ = null;
}
return this;
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage.DoubleNestedMessage msg = 3;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage.Builder getMsgBuilder() {
onChanged();
return getMsgFieldBuilder().getBuilder();
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage.DoubleNestedMessage msg = 3;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessageOrBuilder getMsgOrBuilder() {
if (msgBuilder_ != null) {
return msgBuilder_.getMessageOrBuilder();
} else {
return msg_ == null ?
tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage.getDefaultInstance() : msg_;
}
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage.DoubleNestedMessage msg = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage, tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage.Builder, tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessageOrBuilder>
getMsgFieldBuilder() {
if (msgBuilder_ == null) {
msgBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage, tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessage.Builder, tensorflow.test.Test.TestAllTypes.NestedMessage.DoubleNestedMessageOrBuilder>(
getMsg(),
getParentForChildren(),
isClean());
msg_ = null;
}
return msgBuilder_;
}
private long optionalInt64_ ;
/**
* int64 optional_int64 = 4;
*/
public long getOptionalInt64() {
return optionalInt64_;
}
/**
* int64 optional_int64 = 4;
*/
public Builder setOptionalInt64(long value) {
optionalInt64_ = value;
onChanged();
return this;
}
/**
* int64 optional_int64 = 4;
*/
public Builder clearOptionalInt64() {
optionalInt64_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.test.TestAllTypes.NestedMessage)
}
// @@protoc_insertion_point(class_scope:tensorflow.test.TestAllTypes.NestedMessage)
private static final tensorflow.test.Test.TestAllTypes.NestedMessage DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.test.Test.TestAllTypes.NestedMessage();
}
public static tensorflow.test.Test.TestAllTypes.NestedMessage getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public NestedMessage parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new NestedMessage(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.test.Test.TestAllTypes.NestedMessage getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
private int oneofFieldCase_ = 0;
private java.lang.Object oneofField_;
public enum OneofFieldCase
implements com.google.protobuf.Internal.EnumLite {
ONEOF_UINT32(111),
ONEOF_NESTED_MESSAGE(112),
ONEOF_STRING(113),
ONEOF_BYTES(114),
ONEOF_ENUM(100),
ONEOFFIELD_NOT_SET(0);
private final int value;
private OneofFieldCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static OneofFieldCase valueOf(int value) {
return forNumber(value);
}
public static OneofFieldCase forNumber(int value) {
switch (value) {
case 111: return ONEOF_UINT32;
case 112: return ONEOF_NESTED_MESSAGE;
case 113: return ONEOF_STRING;
case 114: return ONEOF_BYTES;
case 100: return ONEOF_ENUM;
case 0: return ONEOFFIELD_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public OneofFieldCase
getOneofFieldCase() {
return OneofFieldCase.forNumber(
oneofFieldCase_);
}
public static final int OPTIONAL_INT32_FIELD_NUMBER = 1000;
private int optionalInt32_;
/**
*
* Singular
*
*
* int32 optional_int32 = 1000;
*/
public int getOptionalInt32() {
return optionalInt32_;
}
public static final int OPTIONAL_INT64_FIELD_NUMBER = 2;
private long optionalInt64_;
/**
* int64 optional_int64 = 2;
*/
public long getOptionalInt64() {
return optionalInt64_;
}
public static final int OPTIONAL_UINT32_FIELD_NUMBER = 3;
private int optionalUint32_;
/**
* uint32 optional_uint32 = 3;
*/
public int getOptionalUint32() {
return optionalUint32_;
}
public static final int OPTIONAL_UINT64_FIELD_NUMBER = 999;
private long optionalUint64_;
/**
*
* use large tag to test output order.
*
*
* uint64 optional_uint64 = 999;
*/
public long getOptionalUint64() {
return optionalUint64_;
}
public static final int OPTIONAL_SINT32_FIELD_NUMBER = 5;
private int optionalSint32_;
/**
* sint32 optional_sint32 = 5;
*/
public int getOptionalSint32() {
return optionalSint32_;
}
public static final int OPTIONAL_SINT64_FIELD_NUMBER = 6;
private long optionalSint64_;
/**
* sint64 optional_sint64 = 6;
*/
public long getOptionalSint64() {
return optionalSint64_;
}
public static final int OPTIONAL_FIXED32_FIELD_NUMBER = 7;
private int optionalFixed32_;
/**
* fixed32 optional_fixed32 = 7;
*/
public int getOptionalFixed32() {
return optionalFixed32_;
}
public static final int OPTIONAL_FIXED64_FIELD_NUMBER = 8;
private long optionalFixed64_;
/**
* fixed64 optional_fixed64 = 8;
*/
public long getOptionalFixed64() {
return optionalFixed64_;
}
public static final int OPTIONAL_SFIXED32_FIELD_NUMBER = 9;
private int optionalSfixed32_;
/**
* sfixed32 optional_sfixed32 = 9;
*/
public int getOptionalSfixed32() {
return optionalSfixed32_;
}
public static final int OPTIONAL_SFIXED64_FIELD_NUMBER = 10;
private long optionalSfixed64_;
/**
* sfixed64 optional_sfixed64 = 10;
*/
public long getOptionalSfixed64() {
return optionalSfixed64_;
}
public static final int OPTIONAL_FLOAT_FIELD_NUMBER = 11;
private float optionalFloat_;
/**
* float optional_float = 11;
*/
public float getOptionalFloat() {
return optionalFloat_;
}
public static final int OPTIONAL_DOUBLE_FIELD_NUMBER = 12;
private double optionalDouble_;
/**
* double optional_double = 12;
*/
public double getOptionalDouble() {
return optionalDouble_;
}
public static final int OPTIONAL_BOOL_FIELD_NUMBER = 13;
private boolean optionalBool_;
/**
* bool optional_bool = 13;
*/
public boolean getOptionalBool() {
return optionalBool_;
}
public static final int OPTIONAL_STRING_FIELD_NUMBER = 14;
private volatile java.lang.Object optionalString_;
/**
* string optional_string = 14;
*/
public java.lang.String getOptionalString() {
java.lang.Object ref = optionalString_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
optionalString_ = s;
return s;
}
}
/**
* string optional_string = 14;
*/
public com.google.protobuf.ByteString
getOptionalStringBytes() {
java.lang.Object ref = optionalString_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
optionalString_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OPTIONAL_BYTES_FIELD_NUMBER = 15;
private com.google.protobuf.ByteString optionalBytes_;
/**
* bytes optional_bytes = 15;
*/
public com.google.protobuf.ByteString getOptionalBytes() {
return optionalBytes_;
}
public static final int OPTIONAL_NESTED_MESSAGE_FIELD_NUMBER = 18;
private tensorflow.test.Test.TestAllTypes.NestedMessage optionalNestedMessage_;
/**
* .tensorflow.test.TestAllTypes.NestedMessage optional_nested_message = 18;
*/
public boolean hasOptionalNestedMessage() {
return optionalNestedMessage_ != null;
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage optional_nested_message = 18;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage getOptionalNestedMessage() {
return optionalNestedMessage_ == null ? tensorflow.test.Test.TestAllTypes.NestedMessage.getDefaultInstance() : optionalNestedMessage_;
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage optional_nested_message = 18;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessageOrBuilder getOptionalNestedMessageOrBuilder() {
return getOptionalNestedMessage();
}
public static final int OPTIONAL_FOREIGN_MESSAGE_FIELD_NUMBER = 19;
private tensorflow.test.Test.ForeignMessage optionalForeignMessage_;
/**
* .tensorflow.test.ForeignMessage optional_foreign_message = 19;
*/
public boolean hasOptionalForeignMessage() {
return optionalForeignMessage_ != null;
}
/**
* .tensorflow.test.ForeignMessage optional_foreign_message = 19;
*/
public tensorflow.test.Test.ForeignMessage getOptionalForeignMessage() {
return optionalForeignMessage_ == null ? tensorflow.test.Test.ForeignMessage.getDefaultInstance() : optionalForeignMessage_;
}
/**
* .tensorflow.test.ForeignMessage optional_foreign_message = 19;
*/
public tensorflow.test.Test.ForeignMessageOrBuilder getOptionalForeignMessageOrBuilder() {
return getOptionalForeignMessage();
}
public static final int OPTIONAL_NESTED_ENUM_FIELD_NUMBER = 21;
private int optionalNestedEnum_;
/**
* .tensorflow.test.TestAllTypes.NestedEnum optional_nested_enum = 21;
*/
public int getOptionalNestedEnumValue() {
return optionalNestedEnum_;
}
/**
* .tensorflow.test.TestAllTypes.NestedEnum optional_nested_enum = 21;
*/
public tensorflow.test.Test.TestAllTypes.NestedEnum getOptionalNestedEnum() {
@SuppressWarnings("deprecation")
tensorflow.test.Test.TestAllTypes.NestedEnum result = tensorflow.test.Test.TestAllTypes.NestedEnum.valueOf(optionalNestedEnum_);
return result == null ? tensorflow.test.Test.TestAllTypes.NestedEnum.UNRECOGNIZED : result;
}
public static final int OPTIONAL_FOREIGN_ENUM_FIELD_NUMBER = 22;
private int optionalForeignEnum_;
/**
* .tensorflow.test.ForeignEnum optional_foreign_enum = 22;
*/
public int getOptionalForeignEnumValue() {
return optionalForeignEnum_;
}
/**
* .tensorflow.test.ForeignEnum optional_foreign_enum = 22;
*/
public tensorflow.test.Test.ForeignEnum getOptionalForeignEnum() {
@SuppressWarnings("deprecation")
tensorflow.test.Test.ForeignEnum result = tensorflow.test.Test.ForeignEnum.valueOf(optionalForeignEnum_);
return result == null ? tensorflow.test.Test.ForeignEnum.UNRECOGNIZED : result;
}
public static final int OPTIONAL_CORD_FIELD_NUMBER = 25;
private volatile java.lang.Object optionalCord_;
/**
* string optional_cord = 25;
*/
public java.lang.String getOptionalCord() {
java.lang.Object ref = optionalCord_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
optionalCord_ = s;
return s;
}
}
/**
* string optional_cord = 25;
*/
public com.google.protobuf.ByteString
getOptionalCordBytes() {
java.lang.Object ref = optionalCord_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
optionalCord_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REPEATED_INT32_FIELD_NUMBER = 31;
private java.util.List repeatedInt32_;
/**
*
* Repeated
*
*
* repeated int32 repeated_int32 = 31;
*/
public java.util.List
getRepeatedInt32List() {
return repeatedInt32_;
}
/**
*
* Repeated
*
*
* repeated int32 repeated_int32 = 31;
*/
public int getRepeatedInt32Count() {
return repeatedInt32_.size();
}
/**
*
* Repeated
*
*
* repeated int32 repeated_int32 = 31;
*/
public int getRepeatedInt32(int index) {
return repeatedInt32_.get(index);
}
private int repeatedInt32MemoizedSerializedSize = -1;
public static final int REPEATED_INT64_FIELD_NUMBER = 32;
private java.util.List repeatedInt64_;
/**
* repeated int64 repeated_int64 = 32;
*/
public java.util.List
getRepeatedInt64List() {
return repeatedInt64_;
}
/**
* repeated int64 repeated_int64 = 32;
*/
public int getRepeatedInt64Count() {
return repeatedInt64_.size();
}
/**
* repeated int64 repeated_int64 = 32;
*/
public long getRepeatedInt64(int index) {
return repeatedInt64_.get(index);
}
private int repeatedInt64MemoizedSerializedSize = -1;
public static final int REPEATED_UINT32_FIELD_NUMBER = 33;
private java.util.List repeatedUint32_;
/**
* repeated uint32 repeated_uint32 = 33;
*/
public java.util.List
getRepeatedUint32List() {
return repeatedUint32_;
}
/**
* repeated uint32 repeated_uint32 = 33;
*/
public int getRepeatedUint32Count() {
return repeatedUint32_.size();
}
/**
* repeated uint32 repeated_uint32 = 33;
*/
public int getRepeatedUint32(int index) {
return repeatedUint32_.get(index);
}
private int repeatedUint32MemoizedSerializedSize = -1;
public static final int REPEATED_UINT64_FIELD_NUMBER = 34;
private java.util.List repeatedUint64_;
/**
* repeated uint64 repeated_uint64 = 34;
*/
public java.util.List
getRepeatedUint64List() {
return repeatedUint64_;
}
/**
* repeated uint64 repeated_uint64 = 34;
*/
public int getRepeatedUint64Count() {
return repeatedUint64_.size();
}
/**
* repeated uint64 repeated_uint64 = 34;
*/
public long getRepeatedUint64(int index) {
return repeatedUint64_.get(index);
}
private int repeatedUint64MemoizedSerializedSize = -1;
public static final int REPEATED_SINT32_FIELD_NUMBER = 35;
private java.util.List repeatedSint32_;
/**
* repeated sint32 repeated_sint32 = 35;
*/
public java.util.List
getRepeatedSint32List() {
return repeatedSint32_;
}
/**
* repeated sint32 repeated_sint32 = 35;
*/
public int getRepeatedSint32Count() {
return repeatedSint32_.size();
}
/**
* repeated sint32 repeated_sint32 = 35;
*/
public int getRepeatedSint32(int index) {
return repeatedSint32_.get(index);
}
private int repeatedSint32MemoizedSerializedSize = -1;
public static final int REPEATED_SINT64_FIELD_NUMBER = 36;
private java.util.List repeatedSint64_;
/**
* repeated sint64 repeated_sint64 = 36;
*/
public java.util.List
getRepeatedSint64List() {
return repeatedSint64_;
}
/**
* repeated sint64 repeated_sint64 = 36;
*/
public int getRepeatedSint64Count() {
return repeatedSint64_.size();
}
/**
* repeated sint64 repeated_sint64 = 36;
*/
public long getRepeatedSint64(int index) {
return repeatedSint64_.get(index);
}
private int repeatedSint64MemoizedSerializedSize = -1;
public static final int REPEATED_FIXED32_FIELD_NUMBER = 37;
private java.util.List repeatedFixed32_;
/**
* repeated fixed32 repeated_fixed32 = 37;
*/
public java.util.List
getRepeatedFixed32List() {
return repeatedFixed32_;
}
/**
* repeated fixed32 repeated_fixed32 = 37;
*/
public int getRepeatedFixed32Count() {
return repeatedFixed32_.size();
}
/**
* repeated fixed32 repeated_fixed32 = 37;
*/
public int getRepeatedFixed32(int index) {
return repeatedFixed32_.get(index);
}
private int repeatedFixed32MemoizedSerializedSize = -1;
public static final int REPEATED_FIXED64_FIELD_NUMBER = 38;
private java.util.List repeatedFixed64_;
/**
* repeated fixed64 repeated_fixed64 = 38;
*/
public java.util.List
getRepeatedFixed64List() {
return repeatedFixed64_;
}
/**
* repeated fixed64 repeated_fixed64 = 38;
*/
public int getRepeatedFixed64Count() {
return repeatedFixed64_.size();
}
/**
* repeated fixed64 repeated_fixed64 = 38;
*/
public long getRepeatedFixed64(int index) {
return repeatedFixed64_.get(index);
}
private int repeatedFixed64MemoizedSerializedSize = -1;
public static final int REPEATED_SFIXED32_FIELD_NUMBER = 39;
private java.util.List repeatedSfixed32_;
/**
* repeated sfixed32 repeated_sfixed32 = 39;
*/
public java.util.List
getRepeatedSfixed32List() {
return repeatedSfixed32_;
}
/**
* repeated sfixed32 repeated_sfixed32 = 39;
*/
public int getRepeatedSfixed32Count() {
return repeatedSfixed32_.size();
}
/**
* repeated sfixed32 repeated_sfixed32 = 39;
*/
public int getRepeatedSfixed32(int index) {
return repeatedSfixed32_.get(index);
}
private int repeatedSfixed32MemoizedSerializedSize = -1;
public static final int REPEATED_SFIXED64_FIELD_NUMBER = 40;
private java.util.List repeatedSfixed64_;
/**
* repeated sfixed64 repeated_sfixed64 = 40;
*/
public java.util.List
getRepeatedSfixed64List() {
return repeatedSfixed64_;
}
/**
* repeated sfixed64 repeated_sfixed64 = 40;
*/
public int getRepeatedSfixed64Count() {
return repeatedSfixed64_.size();
}
/**
* repeated sfixed64 repeated_sfixed64 = 40;
*/
public long getRepeatedSfixed64(int index) {
return repeatedSfixed64_.get(index);
}
private int repeatedSfixed64MemoizedSerializedSize = -1;
public static final int REPEATED_FLOAT_FIELD_NUMBER = 41;
private java.util.List repeatedFloat_;
/**
* repeated float repeated_float = 41;
*/
public java.util.List
getRepeatedFloatList() {
return repeatedFloat_;
}
/**
* repeated float repeated_float = 41;
*/
public int getRepeatedFloatCount() {
return repeatedFloat_.size();
}
/**
* repeated float repeated_float = 41;
*/
public float getRepeatedFloat(int index) {
return repeatedFloat_.get(index);
}
private int repeatedFloatMemoizedSerializedSize = -1;
public static final int REPEATED_DOUBLE_FIELD_NUMBER = 42;
private java.util.List repeatedDouble_;
/**
* repeated double repeated_double = 42;
*/
public java.util.List
getRepeatedDoubleList() {
return repeatedDouble_;
}
/**
* repeated double repeated_double = 42;
*/
public int getRepeatedDoubleCount() {
return repeatedDouble_.size();
}
/**
* repeated double repeated_double = 42;
*/
public double getRepeatedDouble(int index) {
return repeatedDouble_.get(index);
}
private int repeatedDoubleMemoizedSerializedSize = -1;
public static final int REPEATED_BOOL_FIELD_NUMBER = 43;
private java.util.List repeatedBool_;
/**
* repeated bool repeated_bool = 43;
*/
public java.util.List
getRepeatedBoolList() {
return repeatedBool_;
}
/**
* repeated bool repeated_bool = 43;
*/
public int getRepeatedBoolCount() {
return repeatedBool_.size();
}
/**
* repeated bool repeated_bool = 43;
*/
public boolean getRepeatedBool(int index) {
return repeatedBool_.get(index);
}
private int repeatedBoolMemoizedSerializedSize = -1;
public static final int REPEATED_STRING_FIELD_NUMBER = 44;
private com.google.protobuf.LazyStringList repeatedString_;
/**
* repeated string repeated_string = 44;
*/
public com.google.protobuf.ProtocolStringList
getRepeatedStringList() {
return repeatedString_;
}
/**
* repeated string repeated_string = 44;
*/
public int getRepeatedStringCount() {
return repeatedString_.size();
}
/**
* repeated string repeated_string = 44;
*/
public java.lang.String getRepeatedString(int index) {
return repeatedString_.get(index);
}
/**
* repeated string repeated_string = 44;
*/
public com.google.protobuf.ByteString
getRepeatedStringBytes(int index) {
return repeatedString_.getByteString(index);
}
public static final int REPEATED_BYTES_FIELD_NUMBER = 45;
private java.util.List repeatedBytes_;
/**
* repeated bytes repeated_bytes = 45;
*/
public java.util.List
getRepeatedBytesList() {
return repeatedBytes_;
}
/**
* repeated bytes repeated_bytes = 45;
*/
public int getRepeatedBytesCount() {
return repeatedBytes_.size();
}
/**
* repeated bytes repeated_bytes = 45;
*/
public com.google.protobuf.ByteString getRepeatedBytes(int index) {
return repeatedBytes_.get(index);
}
public static final int REPEATED_NESTED_MESSAGE_FIELD_NUMBER = 48;
private java.util.List repeatedNestedMessage_;
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
public java.util.List getRepeatedNestedMessageList() {
return repeatedNestedMessage_;
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
public java.util.List extends tensorflow.test.Test.TestAllTypes.NestedMessageOrBuilder>
getRepeatedNestedMessageOrBuilderList() {
return repeatedNestedMessage_;
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
public int getRepeatedNestedMessageCount() {
return repeatedNestedMessage_.size();
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage getRepeatedNestedMessage(int index) {
return repeatedNestedMessage_.get(index);
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessageOrBuilder getRepeatedNestedMessageOrBuilder(
int index) {
return repeatedNestedMessage_.get(index);
}
public static final int REPEATED_NESTED_ENUM_FIELD_NUMBER = 51;
private java.util.List repeatedNestedEnum_;
private static final com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, tensorflow.test.Test.TestAllTypes.NestedEnum> repeatedNestedEnum_converter_ =
new com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, tensorflow.test.Test.TestAllTypes.NestedEnum>() {
public tensorflow.test.Test.TestAllTypes.NestedEnum convert(java.lang.Integer from) {
@SuppressWarnings("deprecation")
tensorflow.test.Test.TestAllTypes.NestedEnum result = tensorflow.test.Test.TestAllTypes.NestedEnum.valueOf(from);
return result == null ? tensorflow.test.Test.TestAllTypes.NestedEnum.UNRECOGNIZED : result;
}
};
/**
* repeated .tensorflow.test.TestAllTypes.NestedEnum repeated_nested_enum = 51;
*/
public java.util.List getRepeatedNestedEnumList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, tensorflow.test.Test.TestAllTypes.NestedEnum>(repeatedNestedEnum_, repeatedNestedEnum_converter_);
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedEnum repeated_nested_enum = 51;
*/
public int getRepeatedNestedEnumCount() {
return repeatedNestedEnum_.size();
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedEnum repeated_nested_enum = 51;
*/
public tensorflow.test.Test.TestAllTypes.NestedEnum getRepeatedNestedEnum(int index) {
return repeatedNestedEnum_converter_.convert(repeatedNestedEnum_.get(index));
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedEnum repeated_nested_enum = 51;
*/
public java.util.List
getRepeatedNestedEnumValueList() {
return repeatedNestedEnum_;
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedEnum repeated_nested_enum = 51;
*/
public int getRepeatedNestedEnumValue(int index) {
return repeatedNestedEnum_.get(index);
}
private int repeatedNestedEnumMemoizedSerializedSize;
public static final int REPEATED_CORD_FIELD_NUMBER = 55;
private com.google.protobuf.LazyStringList repeatedCord_;
/**
* repeated string repeated_cord = 55;
*/
public com.google.protobuf.ProtocolStringList
getRepeatedCordList() {
return repeatedCord_;
}
/**
* repeated string repeated_cord = 55;
*/
public int getRepeatedCordCount() {
return repeatedCord_.size();
}
/**
* repeated string repeated_cord = 55;
*/
public java.lang.String getRepeatedCord(int index) {
return repeatedCord_.get(index);
}
/**
* repeated string repeated_cord = 55;
*/
public com.google.protobuf.ByteString
getRepeatedCordBytes(int index) {
return repeatedCord_.getByteString(index);
}
public static final int ONEOF_UINT32_FIELD_NUMBER = 111;
/**
* uint32 oneof_uint32 = 111;
*/
public int getOneofUint32() {
if (oneofFieldCase_ == 111) {
return (java.lang.Integer) oneofField_;
}
return 0;
}
public static final int ONEOF_NESTED_MESSAGE_FIELD_NUMBER = 112;
/**
* .tensorflow.test.TestAllTypes.NestedMessage oneof_nested_message = 112;
*/
public boolean hasOneofNestedMessage() {
return oneofFieldCase_ == 112;
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage oneof_nested_message = 112;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage getOneofNestedMessage() {
if (oneofFieldCase_ == 112) {
return (tensorflow.test.Test.TestAllTypes.NestedMessage) oneofField_;
}
return tensorflow.test.Test.TestAllTypes.NestedMessage.getDefaultInstance();
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage oneof_nested_message = 112;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessageOrBuilder getOneofNestedMessageOrBuilder() {
if (oneofFieldCase_ == 112) {
return (tensorflow.test.Test.TestAllTypes.NestedMessage) oneofField_;
}
return tensorflow.test.Test.TestAllTypes.NestedMessage.getDefaultInstance();
}
public static final int ONEOF_STRING_FIELD_NUMBER = 113;
/**
* string oneof_string = 113;
*/
public java.lang.String getOneofString() {
java.lang.Object ref = "";
if (oneofFieldCase_ == 113) {
ref = oneofField_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (oneofFieldCase_ == 113) {
oneofField_ = s;
}
return s;
}
}
/**
* string oneof_string = 113;
*/
public com.google.protobuf.ByteString
getOneofStringBytes() {
java.lang.Object ref = "";
if (oneofFieldCase_ == 113) {
ref = oneofField_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (oneofFieldCase_ == 113) {
oneofField_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ONEOF_BYTES_FIELD_NUMBER = 114;
/**
* bytes oneof_bytes = 114;
*/
public com.google.protobuf.ByteString getOneofBytes() {
if (oneofFieldCase_ == 114) {
return (com.google.protobuf.ByteString) oneofField_;
}
return com.google.protobuf.ByteString.EMPTY;
}
public static final int ONEOF_ENUM_FIELD_NUMBER = 100;
/**
* .tensorflow.test.TestAllTypes.NestedEnum oneof_enum = 100;
*/
public int getOneofEnumValue() {
if (oneofFieldCase_ == 100) {
return (java.lang.Integer) oneofField_;
}
return 0;
}
/**
* .tensorflow.test.TestAllTypes.NestedEnum oneof_enum = 100;
*/
public tensorflow.test.Test.TestAllTypes.NestedEnum getOneofEnum() {
if (oneofFieldCase_ == 100) {
@SuppressWarnings("deprecation")
tensorflow.test.Test.TestAllTypes.NestedEnum result = tensorflow.test.Test.TestAllTypes.NestedEnum.valueOf(
(java.lang.Integer) oneofField_);
return result == null ? tensorflow.test.Test.TestAllTypes.NestedEnum.UNRECOGNIZED : result;
}
return tensorflow.test.Test.TestAllTypes.NestedEnum.ZERO;
}
public static final int MAP_STRING_TO_MESSAGE_FIELD_NUMBER = 58;
private static final class MapStringToMessageDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, tensorflow.test.Test.TestAllTypes.NestedMessage> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
tensorflow.test.Test.internal_static_tensorflow_test_TestAllTypes_MapStringToMessageEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
tensorflow.test.Test.TestAllTypes.NestedMessage.getDefaultInstance());
}
private com.google.protobuf.MapField<
java.lang.String, tensorflow.test.Test.TestAllTypes.NestedMessage> mapStringToMessage_;
private com.google.protobuf.MapField
internalGetMapStringToMessage() {
if (mapStringToMessage_ == null) {
return com.google.protobuf.MapField.emptyMapField(
MapStringToMessageDefaultEntryHolder.defaultEntry);
}
return mapStringToMessage_;
}
public int getMapStringToMessageCount() {
return internalGetMapStringToMessage().getMap().size();
}
/**
* map<string, .tensorflow.test.TestAllTypes.NestedMessage> map_string_to_message = 58;
*/
public boolean containsMapStringToMessage(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetMapStringToMessage().getMap().containsKey(key);
}
/**
* Use {@link #getMapStringToMessageMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getMapStringToMessage() {
return getMapStringToMessageMap();
}
/**
* map<string, .tensorflow.test.TestAllTypes.NestedMessage> map_string_to_message = 58;
*/
public java.util.Map getMapStringToMessageMap() {
return internalGetMapStringToMessage().getMap();
}
/**
* map<string, .tensorflow.test.TestAllTypes.NestedMessage> map_string_to_message = 58;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage getMapStringToMessageOrDefault(
java.lang.String key,
tensorflow.test.Test.TestAllTypes.NestedMessage defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetMapStringToMessage().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, .tensorflow.test.TestAllTypes.NestedMessage> map_string_to_message = 58;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage getMapStringToMessageOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetMapStringToMessage().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int MAP_INT32_TO_MESSAGE_FIELD_NUMBER = 59;
private static final class MapInt32ToMessageDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.Integer, tensorflow.test.Test.TestAllTypes.NestedMessage> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
tensorflow.test.Test.internal_static_tensorflow_test_TestAllTypes_MapInt32ToMessageEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.INT32,
0,
com.google.protobuf.WireFormat.FieldType.MESSAGE,
tensorflow.test.Test.TestAllTypes.NestedMessage.getDefaultInstance());
}
private com.google.protobuf.MapField<
java.lang.Integer, tensorflow.test.Test.TestAllTypes.NestedMessage> mapInt32ToMessage_;
private com.google.protobuf.MapField
internalGetMapInt32ToMessage() {
if (mapInt32ToMessage_ == null) {
return com.google.protobuf.MapField.emptyMapField(
MapInt32ToMessageDefaultEntryHolder.defaultEntry);
}
return mapInt32ToMessage_;
}
public int getMapInt32ToMessageCount() {
return internalGetMapInt32ToMessage().getMap().size();
}
/**
* map<int32, .tensorflow.test.TestAllTypes.NestedMessage> map_int32_to_message = 59;
*/
public boolean containsMapInt32ToMessage(
int key) {
return internalGetMapInt32ToMessage().getMap().containsKey(key);
}
/**
* Use {@link #getMapInt32ToMessageMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getMapInt32ToMessage() {
return getMapInt32ToMessageMap();
}
/**
* map<int32, .tensorflow.test.TestAllTypes.NestedMessage> map_int32_to_message = 59;
*/
public java.util.Map getMapInt32ToMessageMap() {
return internalGetMapInt32ToMessage().getMap();
}
/**
* map<int32, .tensorflow.test.TestAllTypes.NestedMessage> map_int32_to_message = 59;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage getMapInt32ToMessageOrDefault(
int key,
tensorflow.test.Test.TestAllTypes.NestedMessage defaultValue) {
java.util.Map map =
internalGetMapInt32ToMessage().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<int32, .tensorflow.test.TestAllTypes.NestedMessage> map_int32_to_message = 59;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage getMapInt32ToMessageOrThrow(
int key) {
java.util.Map map =
internalGetMapInt32ToMessage().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int MAP_INT64_TO_MESSAGE_FIELD_NUMBER = 60;
private static final class MapInt64ToMessageDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.Long, tensorflow.test.Test.TestAllTypes.NestedMessage> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
tensorflow.test.Test.internal_static_tensorflow_test_TestAllTypes_MapInt64ToMessageEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.INT64,
0L,
com.google.protobuf.WireFormat.FieldType.MESSAGE,
tensorflow.test.Test.TestAllTypes.NestedMessage.getDefaultInstance());
}
private com.google.protobuf.MapField<
java.lang.Long, tensorflow.test.Test.TestAllTypes.NestedMessage> mapInt64ToMessage_;
private com.google.protobuf.MapField
internalGetMapInt64ToMessage() {
if (mapInt64ToMessage_ == null) {
return com.google.protobuf.MapField.emptyMapField(
MapInt64ToMessageDefaultEntryHolder.defaultEntry);
}
return mapInt64ToMessage_;
}
public int getMapInt64ToMessageCount() {
return internalGetMapInt64ToMessage().getMap().size();
}
/**
* map<int64, .tensorflow.test.TestAllTypes.NestedMessage> map_int64_to_message = 60;
*/
public boolean containsMapInt64ToMessage(
long key) {
return internalGetMapInt64ToMessage().getMap().containsKey(key);
}
/**
* Use {@link #getMapInt64ToMessageMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getMapInt64ToMessage() {
return getMapInt64ToMessageMap();
}
/**
* map<int64, .tensorflow.test.TestAllTypes.NestedMessage> map_int64_to_message = 60;
*/
public java.util.Map getMapInt64ToMessageMap() {
return internalGetMapInt64ToMessage().getMap();
}
/**
* map<int64, .tensorflow.test.TestAllTypes.NestedMessage> map_int64_to_message = 60;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage getMapInt64ToMessageOrDefault(
long key,
tensorflow.test.Test.TestAllTypes.NestedMessage defaultValue) {
java.util.Map map =
internalGetMapInt64ToMessage().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<int64, .tensorflow.test.TestAllTypes.NestedMessage> map_int64_to_message = 60;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage getMapInt64ToMessageOrThrow(
long key) {
java.util.Map map =
internalGetMapInt64ToMessage().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int MAP_BOOL_TO_MESSAGE_FIELD_NUMBER = 61;
private static final class MapBoolToMessageDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.Boolean, tensorflow.test.Test.TestAllTypes.NestedMessage> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
tensorflow.test.Test.internal_static_tensorflow_test_TestAllTypes_MapBoolToMessageEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.BOOL,
false,
com.google.protobuf.WireFormat.FieldType.MESSAGE,
tensorflow.test.Test.TestAllTypes.NestedMessage.getDefaultInstance());
}
private com.google.protobuf.MapField<
java.lang.Boolean, tensorflow.test.Test.TestAllTypes.NestedMessage> mapBoolToMessage_;
private com.google.protobuf.MapField
internalGetMapBoolToMessage() {
if (mapBoolToMessage_ == null) {
return com.google.protobuf.MapField.emptyMapField(
MapBoolToMessageDefaultEntryHolder.defaultEntry);
}
return mapBoolToMessage_;
}
public int getMapBoolToMessageCount() {
return internalGetMapBoolToMessage().getMap().size();
}
/**
* map<bool, .tensorflow.test.TestAllTypes.NestedMessage> map_bool_to_message = 61;
*/
public boolean containsMapBoolToMessage(
boolean key) {
return internalGetMapBoolToMessage().getMap().containsKey(key);
}
/**
* Use {@link #getMapBoolToMessageMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getMapBoolToMessage() {
return getMapBoolToMessageMap();
}
/**
* map<bool, .tensorflow.test.TestAllTypes.NestedMessage> map_bool_to_message = 61;
*/
public java.util.Map getMapBoolToMessageMap() {
return internalGetMapBoolToMessage().getMap();
}
/**
* map<bool, .tensorflow.test.TestAllTypes.NestedMessage> map_bool_to_message = 61;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage getMapBoolToMessageOrDefault(
boolean key,
tensorflow.test.Test.TestAllTypes.NestedMessage defaultValue) {
java.util.Map map =
internalGetMapBoolToMessage().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<bool, .tensorflow.test.TestAllTypes.NestedMessage> map_bool_to_message = 61;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage getMapBoolToMessageOrThrow(
boolean key) {
java.util.Map map =
internalGetMapBoolToMessage().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int MAP_STRING_TO_INT64_FIELD_NUMBER = 62;
private static final class MapStringToInt64DefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.Long> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
tensorflow.test.Test.internal_static_tensorflow_test_TestAllTypes_MapStringToInt64Entry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.INT64,
0L);
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.Long> mapStringToInt64_;
private com.google.protobuf.MapField
internalGetMapStringToInt64() {
if (mapStringToInt64_ == null) {
return com.google.protobuf.MapField.emptyMapField(
MapStringToInt64DefaultEntryHolder.defaultEntry);
}
return mapStringToInt64_;
}
public int getMapStringToInt64Count() {
return internalGetMapStringToInt64().getMap().size();
}
/**
* map<string, int64> map_string_to_int64 = 62;
*/
public boolean containsMapStringToInt64(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetMapStringToInt64().getMap().containsKey(key);
}
/**
* Use {@link #getMapStringToInt64Map()} instead.
*/
@java.lang.Deprecated
public java.util.Map getMapStringToInt64() {
return getMapStringToInt64Map();
}
/**
* map<string, int64> map_string_to_int64 = 62;
*/
public java.util.Map getMapStringToInt64Map() {
return internalGetMapStringToInt64().getMap();
}
/**
* map<string, int64> map_string_to_int64 = 62;
*/
public long getMapStringToInt64OrDefault(
java.lang.String key,
long defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetMapStringToInt64().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, int64> map_string_to_int64 = 62;
*/
public long getMapStringToInt64OrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetMapStringToInt64().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int MAP_INT64_TO_STRING_FIELD_NUMBER = 63;
private static final class MapInt64ToStringDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.Long, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
tensorflow.test.Test.internal_static_tensorflow_test_TestAllTypes_MapInt64ToStringEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.INT64,
0L,
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
private com.google.protobuf.MapField<
java.lang.Long, java.lang.String> mapInt64ToString_;
private com.google.protobuf.MapField
internalGetMapInt64ToString() {
if (mapInt64ToString_ == null) {
return com.google.protobuf.MapField.emptyMapField(
MapInt64ToStringDefaultEntryHolder.defaultEntry);
}
return mapInt64ToString_;
}
public int getMapInt64ToStringCount() {
return internalGetMapInt64ToString().getMap().size();
}
/**
* map<int64, string> map_int64_to_string = 63;
*/
public boolean containsMapInt64ToString(
long key) {
return internalGetMapInt64ToString().getMap().containsKey(key);
}
/**
* Use {@link #getMapInt64ToStringMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getMapInt64ToString() {
return getMapInt64ToStringMap();
}
/**
* map<int64, string> map_int64_to_string = 63;
*/
public java.util.Map getMapInt64ToStringMap() {
return internalGetMapInt64ToString().getMap();
}
/**
* map<int64, string> map_int64_to_string = 63;
*/
public java.lang.String getMapInt64ToStringOrDefault(
long key,
java.lang.String defaultValue) {
java.util.Map map =
internalGetMapInt64ToString().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<int64, string> map_int64_to_string = 63;
*/
public java.lang.String getMapInt64ToStringOrThrow(
long key) {
java.util.Map map =
internalGetMapInt64ToString().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int ANOTHER_MAP_STRING_TO_MESSAGE_FIELD_NUMBER = 65;
private static final class AnotherMapStringToMessageDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, tensorflow.test.Test.TestAllTypes.NestedMessage> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
tensorflow.test.Test.internal_static_tensorflow_test_TestAllTypes_AnotherMapStringToMessageEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
tensorflow.test.Test.TestAllTypes.NestedMessage.getDefaultInstance());
}
private com.google.protobuf.MapField<
java.lang.String, tensorflow.test.Test.TestAllTypes.NestedMessage> anotherMapStringToMessage_;
private com.google.protobuf.MapField
internalGetAnotherMapStringToMessage() {
if (anotherMapStringToMessage_ == null) {
return com.google.protobuf.MapField.emptyMapField(
AnotherMapStringToMessageDefaultEntryHolder.defaultEntry);
}
return anotherMapStringToMessage_;
}
public int getAnotherMapStringToMessageCount() {
return internalGetAnotherMapStringToMessage().getMap().size();
}
/**
* map<string, .tensorflow.test.TestAllTypes.NestedMessage> another_map_string_to_message = 65;
*/
public boolean containsAnotherMapStringToMessage(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetAnotherMapStringToMessage().getMap().containsKey(key);
}
/**
* Use {@link #getAnotherMapStringToMessageMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getAnotherMapStringToMessage() {
return getAnotherMapStringToMessageMap();
}
/**
* map<string, .tensorflow.test.TestAllTypes.NestedMessage> another_map_string_to_message = 65;
*/
public java.util.Map getAnotherMapStringToMessageMap() {
return internalGetAnotherMapStringToMessage().getMap();
}
/**
* map<string, .tensorflow.test.TestAllTypes.NestedMessage> another_map_string_to_message = 65;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage getAnotherMapStringToMessageOrDefault(
java.lang.String key,
tensorflow.test.Test.TestAllTypes.NestedMessage defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetAnotherMapStringToMessage().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, .tensorflow.test.TestAllTypes.NestedMessage> another_map_string_to_message = 65;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage getAnotherMapStringToMessageOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetAnotherMapStringToMessage().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int PACKED_REPEATED_INT64_FIELD_NUMBER = 64;
private java.util.List packedRepeatedInt64_;
/**
* repeated int64 packed_repeated_int64 = 64 [packed = true];
*/
public java.util.List
getPackedRepeatedInt64List() {
return packedRepeatedInt64_;
}
/**
* repeated int64 packed_repeated_int64 = 64 [packed = true];
*/
public int getPackedRepeatedInt64Count() {
return packedRepeatedInt64_.size();
}
/**
* repeated int64 packed_repeated_int64 = 64 [packed = true];
*/
public long getPackedRepeatedInt64(int index) {
return packedRepeatedInt64_.get(index);
}
private int packedRepeatedInt64MemoizedSerializedSize = -1;
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (optionalInt64_ != 0L) {
output.writeInt64(2, optionalInt64_);
}
if (optionalUint32_ != 0) {
output.writeUInt32(3, optionalUint32_);
}
if (optionalSint32_ != 0) {
output.writeSInt32(5, optionalSint32_);
}
if (optionalSint64_ != 0L) {
output.writeSInt64(6, optionalSint64_);
}
if (optionalFixed32_ != 0) {
output.writeFixed32(7, optionalFixed32_);
}
if (optionalFixed64_ != 0L) {
output.writeFixed64(8, optionalFixed64_);
}
if (optionalSfixed32_ != 0) {
output.writeSFixed32(9, optionalSfixed32_);
}
if (optionalSfixed64_ != 0L) {
output.writeSFixed64(10, optionalSfixed64_);
}
if (optionalFloat_ != 0F) {
output.writeFloat(11, optionalFloat_);
}
if (optionalDouble_ != 0D) {
output.writeDouble(12, optionalDouble_);
}
if (optionalBool_ != false) {
output.writeBool(13, optionalBool_);
}
if (!getOptionalStringBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 14, optionalString_);
}
if (!optionalBytes_.isEmpty()) {
output.writeBytes(15, optionalBytes_);
}
if (optionalNestedMessage_ != null) {
output.writeMessage(18, getOptionalNestedMessage());
}
if (optionalForeignMessage_ != null) {
output.writeMessage(19, getOptionalForeignMessage());
}
if (optionalNestedEnum_ != tensorflow.test.Test.TestAllTypes.NestedEnum.ZERO.getNumber()) {
output.writeEnum(21, optionalNestedEnum_);
}
if (optionalForeignEnum_ != tensorflow.test.Test.ForeignEnum.FOREIGN_ZERO.getNumber()) {
output.writeEnum(22, optionalForeignEnum_);
}
if (!getOptionalCordBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 25, optionalCord_);
}
if (getRepeatedInt32List().size() > 0) {
output.writeUInt32NoTag(250);
output.writeUInt32NoTag(repeatedInt32MemoizedSerializedSize);
}
for (int i = 0; i < repeatedInt32_.size(); i++) {
output.writeInt32NoTag(repeatedInt32_.get(i));
}
if (getRepeatedInt64List().size() > 0) {
output.writeUInt32NoTag(258);
output.writeUInt32NoTag(repeatedInt64MemoizedSerializedSize);
}
for (int i = 0; i < repeatedInt64_.size(); i++) {
output.writeInt64NoTag(repeatedInt64_.get(i));
}
if (getRepeatedUint32List().size() > 0) {
output.writeUInt32NoTag(266);
output.writeUInt32NoTag(repeatedUint32MemoizedSerializedSize);
}
for (int i = 0; i < repeatedUint32_.size(); i++) {
output.writeUInt32NoTag(repeatedUint32_.get(i));
}
if (getRepeatedUint64List().size() > 0) {
output.writeUInt32NoTag(274);
output.writeUInt32NoTag(repeatedUint64MemoizedSerializedSize);
}
for (int i = 0; i < repeatedUint64_.size(); i++) {
output.writeUInt64NoTag(repeatedUint64_.get(i));
}
if (getRepeatedSint32List().size() > 0) {
output.writeUInt32NoTag(282);
output.writeUInt32NoTag(repeatedSint32MemoizedSerializedSize);
}
for (int i = 0; i < repeatedSint32_.size(); i++) {
output.writeSInt32NoTag(repeatedSint32_.get(i));
}
if (getRepeatedSint64List().size() > 0) {
output.writeUInt32NoTag(290);
output.writeUInt32NoTag(repeatedSint64MemoizedSerializedSize);
}
for (int i = 0; i < repeatedSint64_.size(); i++) {
output.writeSInt64NoTag(repeatedSint64_.get(i));
}
if (getRepeatedFixed32List().size() > 0) {
output.writeUInt32NoTag(298);
output.writeUInt32NoTag(repeatedFixed32MemoizedSerializedSize);
}
for (int i = 0; i < repeatedFixed32_.size(); i++) {
output.writeFixed32NoTag(repeatedFixed32_.get(i));
}
if (getRepeatedFixed64List().size() > 0) {
output.writeUInt32NoTag(306);
output.writeUInt32NoTag(repeatedFixed64MemoizedSerializedSize);
}
for (int i = 0; i < repeatedFixed64_.size(); i++) {
output.writeFixed64NoTag(repeatedFixed64_.get(i));
}
if (getRepeatedSfixed32List().size() > 0) {
output.writeUInt32NoTag(314);
output.writeUInt32NoTag(repeatedSfixed32MemoizedSerializedSize);
}
for (int i = 0; i < repeatedSfixed32_.size(); i++) {
output.writeSFixed32NoTag(repeatedSfixed32_.get(i));
}
if (getRepeatedSfixed64List().size() > 0) {
output.writeUInt32NoTag(322);
output.writeUInt32NoTag(repeatedSfixed64MemoizedSerializedSize);
}
for (int i = 0; i < repeatedSfixed64_.size(); i++) {
output.writeSFixed64NoTag(repeatedSfixed64_.get(i));
}
if (getRepeatedFloatList().size() > 0) {
output.writeUInt32NoTag(330);
output.writeUInt32NoTag(repeatedFloatMemoizedSerializedSize);
}
for (int i = 0; i < repeatedFloat_.size(); i++) {
output.writeFloatNoTag(repeatedFloat_.get(i));
}
if (getRepeatedDoubleList().size() > 0) {
output.writeUInt32NoTag(338);
output.writeUInt32NoTag(repeatedDoubleMemoizedSerializedSize);
}
for (int i = 0; i < repeatedDouble_.size(); i++) {
output.writeDoubleNoTag(repeatedDouble_.get(i));
}
if (getRepeatedBoolList().size() > 0) {
output.writeUInt32NoTag(346);
output.writeUInt32NoTag(repeatedBoolMemoizedSerializedSize);
}
for (int i = 0; i < repeatedBool_.size(); i++) {
output.writeBoolNoTag(repeatedBool_.get(i));
}
for (int i = 0; i < repeatedString_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 44, repeatedString_.getRaw(i));
}
for (int i = 0; i < repeatedBytes_.size(); i++) {
output.writeBytes(45, repeatedBytes_.get(i));
}
for (int i = 0; i < repeatedNestedMessage_.size(); i++) {
output.writeMessage(48, repeatedNestedMessage_.get(i));
}
if (getRepeatedNestedEnumList().size() > 0) {
output.writeUInt32NoTag(410);
output.writeUInt32NoTag(repeatedNestedEnumMemoizedSerializedSize);
}
for (int i = 0; i < repeatedNestedEnum_.size(); i++) {
output.writeEnumNoTag(repeatedNestedEnum_.get(i));
}
for (int i = 0; i < repeatedCord_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 55, repeatedCord_.getRaw(i));
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetMapStringToMessage(),
MapStringToMessageDefaultEntryHolder.defaultEntry,
58);
com.google.protobuf.GeneratedMessageV3
.serializeIntegerMapTo(
output,
internalGetMapInt32ToMessage(),
MapInt32ToMessageDefaultEntryHolder.defaultEntry,
59);
com.google.protobuf.GeneratedMessageV3
.serializeLongMapTo(
output,
internalGetMapInt64ToMessage(),
MapInt64ToMessageDefaultEntryHolder.defaultEntry,
60);
com.google.protobuf.GeneratedMessageV3
.serializeBooleanMapTo(
output,
internalGetMapBoolToMessage(),
MapBoolToMessageDefaultEntryHolder.defaultEntry,
61);
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetMapStringToInt64(),
MapStringToInt64DefaultEntryHolder.defaultEntry,
62);
com.google.protobuf.GeneratedMessageV3
.serializeLongMapTo(
output,
internalGetMapInt64ToString(),
MapInt64ToStringDefaultEntryHolder.defaultEntry,
63);
if (getPackedRepeatedInt64List().size() > 0) {
output.writeUInt32NoTag(514);
output.writeUInt32NoTag(packedRepeatedInt64MemoizedSerializedSize);
}
for (int i = 0; i < packedRepeatedInt64_.size(); i++) {
output.writeInt64NoTag(packedRepeatedInt64_.get(i));
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetAnotherMapStringToMessage(),
AnotherMapStringToMessageDefaultEntryHolder.defaultEntry,
65);
if (oneofFieldCase_ == 100) {
output.writeEnum(100, ((java.lang.Integer) oneofField_));
}
if (oneofFieldCase_ == 111) {
output.writeUInt32(
111, (int)((java.lang.Integer) oneofField_));
}
if (oneofFieldCase_ == 112) {
output.writeMessage(112, (tensorflow.test.Test.TestAllTypes.NestedMessage) oneofField_);
}
if (oneofFieldCase_ == 113) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 113, oneofField_);
}
if (oneofFieldCase_ == 114) {
output.writeBytes(
114, (com.google.protobuf.ByteString) oneofField_);
}
if (optionalUint64_ != 0L) {
output.writeUInt64(999, optionalUint64_);
}
if (optionalInt32_ != 0) {
output.writeInt32(1000, optionalInt32_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (optionalInt64_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, optionalInt64_);
}
if (optionalUint32_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(3, optionalUint32_);
}
if (optionalSint32_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(5, optionalSint32_);
}
if (optionalSint64_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(6, optionalSint64_);
}
if (optionalFixed32_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeFixed32Size(7, optionalFixed32_);
}
if (optionalFixed64_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeFixed64Size(8, optionalFixed64_);
}
if (optionalSfixed32_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeSFixed32Size(9, optionalSfixed32_);
}
if (optionalSfixed64_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSFixed64Size(10, optionalSfixed64_);
}
if (optionalFloat_ != 0F) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(11, optionalFloat_);
}
if (optionalDouble_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(12, optionalDouble_);
}
if (optionalBool_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(13, optionalBool_);
}
if (!getOptionalStringBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(14, optionalString_);
}
if (!optionalBytes_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(15, optionalBytes_);
}
if (optionalNestedMessage_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(18, getOptionalNestedMessage());
}
if (optionalForeignMessage_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(19, getOptionalForeignMessage());
}
if (optionalNestedEnum_ != tensorflow.test.Test.TestAllTypes.NestedEnum.ZERO.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(21, optionalNestedEnum_);
}
if (optionalForeignEnum_ != tensorflow.test.Test.ForeignEnum.FOREIGN_ZERO.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(22, optionalForeignEnum_);
}
if (!getOptionalCordBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(25, optionalCord_);
}
{
int dataSize = 0;
for (int i = 0; i < repeatedInt32_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(repeatedInt32_.get(i));
}
size += dataSize;
if (!getRepeatedInt32List().isEmpty()) {
size += 2;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
repeatedInt32MemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < repeatedInt64_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt64SizeNoTag(repeatedInt64_.get(i));
}
size += dataSize;
if (!getRepeatedInt64List().isEmpty()) {
size += 2;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
repeatedInt64MemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < repeatedUint32_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(repeatedUint32_.get(i));
}
size += dataSize;
if (!getRepeatedUint32List().isEmpty()) {
size += 2;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
repeatedUint32MemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < repeatedUint64_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt64SizeNoTag(repeatedUint64_.get(i));
}
size += dataSize;
if (!getRepeatedUint64List().isEmpty()) {
size += 2;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
repeatedUint64MemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < repeatedSint32_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeSInt32SizeNoTag(repeatedSint32_.get(i));
}
size += dataSize;
if (!getRepeatedSint32List().isEmpty()) {
size += 2;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
repeatedSint32MemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < repeatedSint64_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeSInt64SizeNoTag(repeatedSint64_.get(i));
}
size += dataSize;
if (!getRepeatedSint64List().isEmpty()) {
size += 2;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
repeatedSint64MemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
dataSize = 4 * getRepeatedFixed32List().size();
size += dataSize;
if (!getRepeatedFixed32List().isEmpty()) {
size += 2;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
repeatedFixed32MemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
dataSize = 8 * getRepeatedFixed64List().size();
size += dataSize;
if (!getRepeatedFixed64List().isEmpty()) {
size += 2;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
repeatedFixed64MemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
dataSize = 4 * getRepeatedSfixed32List().size();
size += dataSize;
if (!getRepeatedSfixed32List().isEmpty()) {
size += 2;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
repeatedSfixed32MemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
dataSize = 8 * getRepeatedSfixed64List().size();
size += dataSize;
if (!getRepeatedSfixed64List().isEmpty()) {
size += 2;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
repeatedSfixed64MemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
dataSize = 4 * getRepeatedFloatList().size();
size += dataSize;
if (!getRepeatedFloatList().isEmpty()) {
size += 2;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
repeatedFloatMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
dataSize = 8 * getRepeatedDoubleList().size();
size += dataSize;
if (!getRepeatedDoubleList().isEmpty()) {
size += 2;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
repeatedDoubleMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
dataSize = 1 * getRepeatedBoolList().size();
size += dataSize;
if (!getRepeatedBoolList().isEmpty()) {
size += 2;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
repeatedBoolMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < repeatedString_.size(); i++) {
dataSize += computeStringSizeNoTag(repeatedString_.getRaw(i));
}
size += dataSize;
size += 2 * getRepeatedStringList().size();
}
{
int dataSize = 0;
for (int i = 0; i < repeatedBytes_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(repeatedBytes_.get(i));
}
size += dataSize;
size += 2 * getRepeatedBytesList().size();
}
for (int i = 0; i < repeatedNestedMessage_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(48, repeatedNestedMessage_.get(i));
}
{
int dataSize = 0;
for (int i = 0; i < repeatedNestedEnum_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(repeatedNestedEnum_.get(i));
}
size += dataSize;
if (!getRepeatedNestedEnumList().isEmpty()) { size += 2;
size += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(dataSize);
}repeatedNestedEnumMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < repeatedCord_.size(); i++) {
dataSize += computeStringSizeNoTag(repeatedCord_.getRaw(i));
}
size += dataSize;
size += 2 * getRepeatedCordList().size();
}
for (java.util.Map.Entry entry
: internalGetMapStringToMessage().getMap().entrySet()) {
com.google.protobuf.MapEntry
mapStringToMessage__ = MapStringToMessageDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(58, mapStringToMessage__);
}
for (java.util.Map.Entry entry
: internalGetMapInt32ToMessage().getMap().entrySet()) {
com.google.protobuf.MapEntry
mapInt32ToMessage__ = MapInt32ToMessageDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(59, mapInt32ToMessage__);
}
for (java.util.Map.Entry entry
: internalGetMapInt64ToMessage().getMap().entrySet()) {
com.google.protobuf.MapEntry
mapInt64ToMessage__ = MapInt64ToMessageDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(60, mapInt64ToMessage__);
}
for (java.util.Map.Entry entry
: internalGetMapBoolToMessage().getMap().entrySet()) {
com.google.protobuf.MapEntry
mapBoolToMessage__ = MapBoolToMessageDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(61, mapBoolToMessage__);
}
for (java.util.Map.Entry entry
: internalGetMapStringToInt64().getMap().entrySet()) {
com.google.protobuf.MapEntry
mapStringToInt64__ = MapStringToInt64DefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(62, mapStringToInt64__);
}
for (java.util.Map.Entry entry
: internalGetMapInt64ToString().getMap().entrySet()) {
com.google.protobuf.MapEntry
mapInt64ToString__ = MapInt64ToStringDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(63, mapInt64ToString__);
}
{
int dataSize = 0;
for (int i = 0; i < packedRepeatedInt64_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt64SizeNoTag(packedRepeatedInt64_.get(i));
}
size += dataSize;
if (!getPackedRepeatedInt64List().isEmpty()) {
size += 2;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
packedRepeatedInt64MemoizedSerializedSize = dataSize;
}
for (java.util.Map.Entry entry
: internalGetAnotherMapStringToMessage().getMap().entrySet()) {
com.google.protobuf.MapEntry
anotherMapStringToMessage__ = AnotherMapStringToMessageDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(65, anotherMapStringToMessage__);
}
if (oneofFieldCase_ == 100) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(100, ((java.lang.Integer) oneofField_));
}
if (oneofFieldCase_ == 111) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(
111, (int)((java.lang.Integer) oneofField_));
}
if (oneofFieldCase_ == 112) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(112, (tensorflow.test.Test.TestAllTypes.NestedMessage) oneofField_);
}
if (oneofFieldCase_ == 113) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(113, oneofField_);
}
if (oneofFieldCase_ == 114) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(
114, (com.google.protobuf.ByteString) oneofField_);
}
if (optionalUint64_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(999, optionalUint64_);
}
if (optionalInt32_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1000, optionalInt32_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.test.Test.TestAllTypes)) {
return super.equals(obj);
}
tensorflow.test.Test.TestAllTypes other = (tensorflow.test.Test.TestAllTypes) obj;
boolean result = true;
result = result && (getOptionalInt32()
== other.getOptionalInt32());
result = result && (getOptionalInt64()
== other.getOptionalInt64());
result = result && (getOptionalUint32()
== other.getOptionalUint32());
result = result && (getOptionalUint64()
== other.getOptionalUint64());
result = result && (getOptionalSint32()
== other.getOptionalSint32());
result = result && (getOptionalSint64()
== other.getOptionalSint64());
result = result && (getOptionalFixed32()
== other.getOptionalFixed32());
result = result && (getOptionalFixed64()
== other.getOptionalFixed64());
result = result && (getOptionalSfixed32()
== other.getOptionalSfixed32());
result = result && (getOptionalSfixed64()
== other.getOptionalSfixed64());
result = result && (
java.lang.Float.floatToIntBits(getOptionalFloat())
== java.lang.Float.floatToIntBits(
other.getOptionalFloat()));
result = result && (
java.lang.Double.doubleToLongBits(getOptionalDouble())
== java.lang.Double.doubleToLongBits(
other.getOptionalDouble()));
result = result && (getOptionalBool()
== other.getOptionalBool());
result = result && getOptionalString()
.equals(other.getOptionalString());
result = result && getOptionalBytes()
.equals(other.getOptionalBytes());
result = result && (hasOptionalNestedMessage() == other.hasOptionalNestedMessage());
if (hasOptionalNestedMessage()) {
result = result && getOptionalNestedMessage()
.equals(other.getOptionalNestedMessage());
}
result = result && (hasOptionalForeignMessage() == other.hasOptionalForeignMessage());
if (hasOptionalForeignMessage()) {
result = result && getOptionalForeignMessage()
.equals(other.getOptionalForeignMessage());
}
result = result && optionalNestedEnum_ == other.optionalNestedEnum_;
result = result && optionalForeignEnum_ == other.optionalForeignEnum_;
result = result && getOptionalCord()
.equals(other.getOptionalCord());
result = result && getRepeatedInt32List()
.equals(other.getRepeatedInt32List());
result = result && getRepeatedInt64List()
.equals(other.getRepeatedInt64List());
result = result && getRepeatedUint32List()
.equals(other.getRepeatedUint32List());
result = result && getRepeatedUint64List()
.equals(other.getRepeatedUint64List());
result = result && getRepeatedSint32List()
.equals(other.getRepeatedSint32List());
result = result && getRepeatedSint64List()
.equals(other.getRepeatedSint64List());
result = result && getRepeatedFixed32List()
.equals(other.getRepeatedFixed32List());
result = result && getRepeatedFixed64List()
.equals(other.getRepeatedFixed64List());
result = result && getRepeatedSfixed32List()
.equals(other.getRepeatedSfixed32List());
result = result && getRepeatedSfixed64List()
.equals(other.getRepeatedSfixed64List());
result = result && getRepeatedFloatList()
.equals(other.getRepeatedFloatList());
result = result && getRepeatedDoubleList()
.equals(other.getRepeatedDoubleList());
result = result && getRepeatedBoolList()
.equals(other.getRepeatedBoolList());
result = result && getRepeatedStringList()
.equals(other.getRepeatedStringList());
result = result && getRepeatedBytesList()
.equals(other.getRepeatedBytesList());
result = result && getRepeatedNestedMessageList()
.equals(other.getRepeatedNestedMessageList());
result = result && repeatedNestedEnum_.equals(other.repeatedNestedEnum_);
result = result && getRepeatedCordList()
.equals(other.getRepeatedCordList());
result = result && internalGetMapStringToMessage().equals(
other.internalGetMapStringToMessage());
result = result && internalGetMapInt32ToMessage().equals(
other.internalGetMapInt32ToMessage());
result = result && internalGetMapInt64ToMessage().equals(
other.internalGetMapInt64ToMessage());
result = result && internalGetMapBoolToMessage().equals(
other.internalGetMapBoolToMessage());
result = result && internalGetMapStringToInt64().equals(
other.internalGetMapStringToInt64());
result = result && internalGetMapInt64ToString().equals(
other.internalGetMapInt64ToString());
result = result && internalGetAnotherMapStringToMessage().equals(
other.internalGetAnotherMapStringToMessage());
result = result && getPackedRepeatedInt64List()
.equals(other.getPackedRepeatedInt64List());
result = result && getOneofFieldCase().equals(
other.getOneofFieldCase());
if (!result) return false;
switch (oneofFieldCase_) {
case 111:
result = result && (getOneofUint32()
== other.getOneofUint32());
break;
case 112:
result = result && getOneofNestedMessage()
.equals(other.getOneofNestedMessage());
break;
case 113:
result = result && getOneofString()
.equals(other.getOneofString());
break;
case 114:
result = result && getOneofBytes()
.equals(other.getOneofBytes());
break;
case 100:
result = result && getOneofEnumValue()
== other.getOneofEnumValue();
break;
case 0:
default:
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + OPTIONAL_INT32_FIELD_NUMBER;
hash = (53 * hash) + getOptionalInt32();
hash = (37 * hash) + OPTIONAL_INT64_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOptionalInt64());
hash = (37 * hash) + OPTIONAL_UINT32_FIELD_NUMBER;
hash = (53 * hash) + getOptionalUint32();
hash = (37 * hash) + OPTIONAL_UINT64_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOptionalUint64());
hash = (37 * hash) + OPTIONAL_SINT32_FIELD_NUMBER;
hash = (53 * hash) + getOptionalSint32();
hash = (37 * hash) + OPTIONAL_SINT64_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOptionalSint64());
hash = (37 * hash) + OPTIONAL_FIXED32_FIELD_NUMBER;
hash = (53 * hash) + getOptionalFixed32();
hash = (37 * hash) + OPTIONAL_FIXED64_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOptionalFixed64());
hash = (37 * hash) + OPTIONAL_SFIXED32_FIELD_NUMBER;
hash = (53 * hash) + getOptionalSfixed32();
hash = (37 * hash) + OPTIONAL_SFIXED64_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOptionalSfixed64());
hash = (37 * hash) + OPTIONAL_FLOAT_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getOptionalFloat());
hash = (37 * hash) + OPTIONAL_DOUBLE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getOptionalDouble()));
hash = (37 * hash) + OPTIONAL_BOOL_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getOptionalBool());
hash = (37 * hash) + OPTIONAL_STRING_FIELD_NUMBER;
hash = (53 * hash) + getOptionalString().hashCode();
hash = (37 * hash) + OPTIONAL_BYTES_FIELD_NUMBER;
hash = (53 * hash) + getOptionalBytes().hashCode();
if (hasOptionalNestedMessage()) {
hash = (37 * hash) + OPTIONAL_NESTED_MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getOptionalNestedMessage().hashCode();
}
if (hasOptionalForeignMessage()) {
hash = (37 * hash) + OPTIONAL_FOREIGN_MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getOptionalForeignMessage().hashCode();
}
hash = (37 * hash) + OPTIONAL_NESTED_ENUM_FIELD_NUMBER;
hash = (53 * hash) + optionalNestedEnum_;
hash = (37 * hash) + OPTIONAL_FOREIGN_ENUM_FIELD_NUMBER;
hash = (53 * hash) + optionalForeignEnum_;
hash = (37 * hash) + OPTIONAL_CORD_FIELD_NUMBER;
hash = (53 * hash) + getOptionalCord().hashCode();
if (getRepeatedInt32Count() > 0) {
hash = (37 * hash) + REPEATED_INT32_FIELD_NUMBER;
hash = (53 * hash) + getRepeatedInt32List().hashCode();
}
if (getRepeatedInt64Count() > 0) {
hash = (37 * hash) + REPEATED_INT64_FIELD_NUMBER;
hash = (53 * hash) + getRepeatedInt64List().hashCode();
}
if (getRepeatedUint32Count() > 0) {
hash = (37 * hash) + REPEATED_UINT32_FIELD_NUMBER;
hash = (53 * hash) + getRepeatedUint32List().hashCode();
}
if (getRepeatedUint64Count() > 0) {
hash = (37 * hash) + REPEATED_UINT64_FIELD_NUMBER;
hash = (53 * hash) + getRepeatedUint64List().hashCode();
}
if (getRepeatedSint32Count() > 0) {
hash = (37 * hash) + REPEATED_SINT32_FIELD_NUMBER;
hash = (53 * hash) + getRepeatedSint32List().hashCode();
}
if (getRepeatedSint64Count() > 0) {
hash = (37 * hash) + REPEATED_SINT64_FIELD_NUMBER;
hash = (53 * hash) + getRepeatedSint64List().hashCode();
}
if (getRepeatedFixed32Count() > 0) {
hash = (37 * hash) + REPEATED_FIXED32_FIELD_NUMBER;
hash = (53 * hash) + getRepeatedFixed32List().hashCode();
}
if (getRepeatedFixed64Count() > 0) {
hash = (37 * hash) + REPEATED_FIXED64_FIELD_NUMBER;
hash = (53 * hash) + getRepeatedFixed64List().hashCode();
}
if (getRepeatedSfixed32Count() > 0) {
hash = (37 * hash) + REPEATED_SFIXED32_FIELD_NUMBER;
hash = (53 * hash) + getRepeatedSfixed32List().hashCode();
}
if (getRepeatedSfixed64Count() > 0) {
hash = (37 * hash) + REPEATED_SFIXED64_FIELD_NUMBER;
hash = (53 * hash) + getRepeatedSfixed64List().hashCode();
}
if (getRepeatedFloatCount() > 0) {
hash = (37 * hash) + REPEATED_FLOAT_FIELD_NUMBER;
hash = (53 * hash) + getRepeatedFloatList().hashCode();
}
if (getRepeatedDoubleCount() > 0) {
hash = (37 * hash) + REPEATED_DOUBLE_FIELD_NUMBER;
hash = (53 * hash) + getRepeatedDoubleList().hashCode();
}
if (getRepeatedBoolCount() > 0) {
hash = (37 * hash) + REPEATED_BOOL_FIELD_NUMBER;
hash = (53 * hash) + getRepeatedBoolList().hashCode();
}
if (getRepeatedStringCount() > 0) {
hash = (37 * hash) + REPEATED_STRING_FIELD_NUMBER;
hash = (53 * hash) + getRepeatedStringList().hashCode();
}
if (getRepeatedBytesCount() > 0) {
hash = (37 * hash) + REPEATED_BYTES_FIELD_NUMBER;
hash = (53 * hash) + getRepeatedBytesList().hashCode();
}
if (getRepeatedNestedMessageCount() > 0) {
hash = (37 * hash) + REPEATED_NESTED_MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getRepeatedNestedMessageList().hashCode();
}
if (getRepeatedNestedEnumCount() > 0) {
hash = (37 * hash) + REPEATED_NESTED_ENUM_FIELD_NUMBER;
hash = (53 * hash) + repeatedNestedEnum_.hashCode();
}
if (getRepeatedCordCount() > 0) {
hash = (37 * hash) + REPEATED_CORD_FIELD_NUMBER;
hash = (53 * hash) + getRepeatedCordList().hashCode();
}
if (!internalGetMapStringToMessage().getMap().isEmpty()) {
hash = (37 * hash) + MAP_STRING_TO_MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + internalGetMapStringToMessage().hashCode();
}
if (!internalGetMapInt32ToMessage().getMap().isEmpty()) {
hash = (37 * hash) + MAP_INT32_TO_MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + internalGetMapInt32ToMessage().hashCode();
}
if (!internalGetMapInt64ToMessage().getMap().isEmpty()) {
hash = (37 * hash) + MAP_INT64_TO_MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + internalGetMapInt64ToMessage().hashCode();
}
if (!internalGetMapBoolToMessage().getMap().isEmpty()) {
hash = (37 * hash) + MAP_BOOL_TO_MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + internalGetMapBoolToMessage().hashCode();
}
if (!internalGetMapStringToInt64().getMap().isEmpty()) {
hash = (37 * hash) + MAP_STRING_TO_INT64_FIELD_NUMBER;
hash = (53 * hash) + internalGetMapStringToInt64().hashCode();
}
if (!internalGetMapInt64ToString().getMap().isEmpty()) {
hash = (37 * hash) + MAP_INT64_TO_STRING_FIELD_NUMBER;
hash = (53 * hash) + internalGetMapInt64ToString().hashCode();
}
if (!internalGetAnotherMapStringToMessage().getMap().isEmpty()) {
hash = (37 * hash) + ANOTHER_MAP_STRING_TO_MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + internalGetAnotherMapStringToMessage().hashCode();
}
if (getPackedRepeatedInt64Count() > 0) {
hash = (37 * hash) + PACKED_REPEATED_INT64_FIELD_NUMBER;
hash = (53 * hash) + getPackedRepeatedInt64List().hashCode();
}
switch (oneofFieldCase_) {
case 111:
hash = (37 * hash) + ONEOF_UINT32_FIELD_NUMBER;
hash = (53 * hash) + getOneofUint32();
break;
case 112:
hash = (37 * hash) + ONEOF_NESTED_MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getOneofNestedMessage().hashCode();
break;
case 113:
hash = (37 * hash) + ONEOF_STRING_FIELD_NUMBER;
hash = (53 * hash) + getOneofString().hashCode();
break;
case 114:
hash = (37 * hash) + ONEOF_BYTES_FIELD_NUMBER;
hash = (53 * hash) + getOneofBytes().hashCode();
break;
case 100:
hash = (37 * hash) + ONEOF_ENUM_FIELD_NUMBER;
hash = (53 * hash) + getOneofEnumValue();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.test.Test.TestAllTypes parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.test.Test.TestAllTypes parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.test.Test.TestAllTypes parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.test.Test.TestAllTypes parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.test.Test.TestAllTypes parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.test.Test.TestAllTypes parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.test.Test.TestAllTypes parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.test.Test.TestAllTypes parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.test.Test.TestAllTypes parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.test.Test.TestAllTypes parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.test.Test.TestAllTypes parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.test.Test.TestAllTypes parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.test.Test.TestAllTypes prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.test.TestAllTypes}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.test.TestAllTypes)
tensorflow.test.Test.TestAllTypesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.test.Test.internal_static_tensorflow_test_TestAllTypes_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 58:
return internalGetMapStringToMessage();
case 59:
return internalGetMapInt32ToMessage();
case 60:
return internalGetMapInt64ToMessage();
case 61:
return internalGetMapBoolToMessage();
case 62:
return internalGetMapStringToInt64();
case 63:
return internalGetMapInt64ToString();
case 65:
return internalGetAnotherMapStringToMessage();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 58:
return internalGetMutableMapStringToMessage();
case 59:
return internalGetMutableMapInt32ToMessage();
case 60:
return internalGetMutableMapInt64ToMessage();
case 61:
return internalGetMutableMapBoolToMessage();
case 62:
return internalGetMutableMapStringToInt64();
case 63:
return internalGetMutableMapInt64ToString();
case 65:
return internalGetMutableAnotherMapStringToMessage();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.test.Test.internal_static_tensorflow_test_TestAllTypes_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.test.Test.TestAllTypes.class, tensorflow.test.Test.TestAllTypes.Builder.class);
}
// Construct using tensorflow.test.Test.TestAllTypes.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getRepeatedNestedMessageFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
optionalInt32_ = 0;
optionalInt64_ = 0L;
optionalUint32_ = 0;
optionalUint64_ = 0L;
optionalSint32_ = 0;
optionalSint64_ = 0L;
optionalFixed32_ = 0;
optionalFixed64_ = 0L;
optionalSfixed32_ = 0;
optionalSfixed64_ = 0L;
optionalFloat_ = 0F;
optionalDouble_ = 0D;
optionalBool_ = false;
optionalString_ = "";
optionalBytes_ = com.google.protobuf.ByteString.EMPTY;
if (optionalNestedMessageBuilder_ == null) {
optionalNestedMessage_ = null;
} else {
optionalNestedMessage_ = null;
optionalNestedMessageBuilder_ = null;
}
if (optionalForeignMessageBuilder_ == null) {
optionalForeignMessage_ = null;
} else {
optionalForeignMessage_ = null;
optionalForeignMessageBuilder_ = null;
}
optionalNestedEnum_ = 0;
optionalForeignEnum_ = 0;
optionalCord_ = "";
repeatedInt32_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00100000);
repeatedInt64_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00200000);
repeatedUint32_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00400000);
repeatedUint64_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00800000);
repeatedSint32_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x01000000);
repeatedSint64_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x02000000);
repeatedFixed32_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x04000000);
repeatedFixed64_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x08000000);
repeatedSfixed32_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x10000000);
repeatedSfixed64_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x20000000);
repeatedFloat_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x40000000);
repeatedDouble_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x80000000);
repeatedBool_ = java.util.Collections.emptyList();
bitField1_ = (bitField1_ & ~0x00000001);
repeatedString_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField1_ = (bitField1_ & ~0x00000002);
repeatedBytes_ = java.util.Collections.emptyList();
bitField1_ = (bitField1_ & ~0x00000004);
if (repeatedNestedMessageBuilder_ == null) {
repeatedNestedMessage_ = java.util.Collections.emptyList();
bitField1_ = (bitField1_ & ~0x00000008);
} else {
repeatedNestedMessageBuilder_.clear();
}
repeatedNestedEnum_ = java.util.Collections.emptyList();
bitField1_ = (bitField1_ & ~0x00000010);
repeatedCord_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField1_ = (bitField1_ & ~0x00000020);
internalGetMutableMapStringToMessage().clear();
internalGetMutableMapInt32ToMessage().clear();
internalGetMutableMapInt64ToMessage().clear();
internalGetMutableMapBoolToMessage().clear();
internalGetMutableMapStringToInt64().clear();
internalGetMutableMapInt64ToString().clear();
internalGetMutableAnotherMapStringToMessage().clear();
packedRepeatedInt64_ = java.util.Collections.emptyList();
bitField1_ = (bitField1_ & ~0x00040000);
oneofFieldCase_ = 0;
oneofField_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.test.Test.internal_static_tensorflow_test_TestAllTypes_descriptor;
}
@java.lang.Override
public tensorflow.test.Test.TestAllTypes getDefaultInstanceForType() {
return tensorflow.test.Test.TestAllTypes.getDefaultInstance();
}
@java.lang.Override
public tensorflow.test.Test.TestAllTypes build() {
tensorflow.test.Test.TestAllTypes result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.test.Test.TestAllTypes buildPartial() {
tensorflow.test.Test.TestAllTypes result = new tensorflow.test.Test.TestAllTypes(this);
int from_bitField0_ = bitField0_;
int from_bitField1_ = bitField1_;
int to_bitField0_ = 0;
result.optionalInt32_ = optionalInt32_;
result.optionalInt64_ = optionalInt64_;
result.optionalUint32_ = optionalUint32_;
result.optionalUint64_ = optionalUint64_;
result.optionalSint32_ = optionalSint32_;
result.optionalSint64_ = optionalSint64_;
result.optionalFixed32_ = optionalFixed32_;
result.optionalFixed64_ = optionalFixed64_;
result.optionalSfixed32_ = optionalSfixed32_;
result.optionalSfixed64_ = optionalSfixed64_;
result.optionalFloat_ = optionalFloat_;
result.optionalDouble_ = optionalDouble_;
result.optionalBool_ = optionalBool_;
result.optionalString_ = optionalString_;
result.optionalBytes_ = optionalBytes_;
if (optionalNestedMessageBuilder_ == null) {
result.optionalNestedMessage_ = optionalNestedMessage_;
} else {
result.optionalNestedMessage_ = optionalNestedMessageBuilder_.build();
}
if (optionalForeignMessageBuilder_ == null) {
result.optionalForeignMessage_ = optionalForeignMessage_;
} else {
result.optionalForeignMessage_ = optionalForeignMessageBuilder_.build();
}
result.optionalNestedEnum_ = optionalNestedEnum_;
result.optionalForeignEnum_ = optionalForeignEnum_;
result.optionalCord_ = optionalCord_;
if (((bitField0_ & 0x00100000) == 0x00100000)) {
repeatedInt32_ = java.util.Collections.unmodifiableList(repeatedInt32_);
bitField0_ = (bitField0_ & ~0x00100000);
}
result.repeatedInt32_ = repeatedInt32_;
if (((bitField0_ & 0x00200000) == 0x00200000)) {
repeatedInt64_ = java.util.Collections.unmodifiableList(repeatedInt64_);
bitField0_ = (bitField0_ & ~0x00200000);
}
result.repeatedInt64_ = repeatedInt64_;
if (((bitField0_ & 0x00400000) == 0x00400000)) {
repeatedUint32_ = java.util.Collections.unmodifiableList(repeatedUint32_);
bitField0_ = (bitField0_ & ~0x00400000);
}
result.repeatedUint32_ = repeatedUint32_;
if (((bitField0_ & 0x00800000) == 0x00800000)) {
repeatedUint64_ = java.util.Collections.unmodifiableList(repeatedUint64_);
bitField0_ = (bitField0_ & ~0x00800000);
}
result.repeatedUint64_ = repeatedUint64_;
if (((bitField0_ & 0x01000000) == 0x01000000)) {
repeatedSint32_ = java.util.Collections.unmodifiableList(repeatedSint32_);
bitField0_ = (bitField0_ & ~0x01000000);
}
result.repeatedSint32_ = repeatedSint32_;
if (((bitField0_ & 0x02000000) == 0x02000000)) {
repeatedSint64_ = java.util.Collections.unmodifiableList(repeatedSint64_);
bitField0_ = (bitField0_ & ~0x02000000);
}
result.repeatedSint64_ = repeatedSint64_;
if (((bitField0_ & 0x04000000) == 0x04000000)) {
repeatedFixed32_ = java.util.Collections.unmodifiableList(repeatedFixed32_);
bitField0_ = (bitField0_ & ~0x04000000);
}
result.repeatedFixed32_ = repeatedFixed32_;
if (((bitField0_ & 0x08000000) == 0x08000000)) {
repeatedFixed64_ = java.util.Collections.unmodifiableList(repeatedFixed64_);
bitField0_ = (bitField0_ & ~0x08000000);
}
result.repeatedFixed64_ = repeatedFixed64_;
if (((bitField0_ & 0x10000000) == 0x10000000)) {
repeatedSfixed32_ = java.util.Collections.unmodifiableList(repeatedSfixed32_);
bitField0_ = (bitField0_ & ~0x10000000);
}
result.repeatedSfixed32_ = repeatedSfixed32_;
if (((bitField0_ & 0x20000000) == 0x20000000)) {
repeatedSfixed64_ = java.util.Collections.unmodifiableList(repeatedSfixed64_);
bitField0_ = (bitField0_ & ~0x20000000);
}
result.repeatedSfixed64_ = repeatedSfixed64_;
if (((bitField0_ & 0x40000000) == 0x40000000)) {
repeatedFloat_ = java.util.Collections.unmodifiableList(repeatedFloat_);
bitField0_ = (bitField0_ & ~0x40000000);
}
result.repeatedFloat_ = repeatedFloat_;
if (((bitField0_ & 0x80000000) == 0x80000000)) {
repeatedDouble_ = java.util.Collections.unmodifiableList(repeatedDouble_);
bitField0_ = (bitField0_ & ~0x80000000);
}
result.repeatedDouble_ = repeatedDouble_;
if (((bitField1_ & 0x00000001) == 0x00000001)) {
repeatedBool_ = java.util.Collections.unmodifiableList(repeatedBool_);
bitField1_ = (bitField1_ & ~0x00000001);
}
result.repeatedBool_ = repeatedBool_;
if (((bitField1_ & 0x00000002) == 0x00000002)) {
repeatedString_ = repeatedString_.getUnmodifiableView();
bitField1_ = (bitField1_ & ~0x00000002);
}
result.repeatedString_ = repeatedString_;
if (((bitField1_ & 0x00000004) == 0x00000004)) {
repeatedBytes_ = java.util.Collections.unmodifiableList(repeatedBytes_);
bitField1_ = (bitField1_ & ~0x00000004);
}
result.repeatedBytes_ = repeatedBytes_;
if (repeatedNestedMessageBuilder_ == null) {
if (((bitField1_ & 0x00000008) == 0x00000008)) {
repeatedNestedMessage_ = java.util.Collections.unmodifiableList(repeatedNestedMessage_);
bitField1_ = (bitField1_ & ~0x00000008);
}
result.repeatedNestedMessage_ = repeatedNestedMessage_;
} else {
result.repeatedNestedMessage_ = repeatedNestedMessageBuilder_.build();
}
if (((bitField1_ & 0x00000010) == 0x00000010)) {
repeatedNestedEnum_ = java.util.Collections.unmodifiableList(repeatedNestedEnum_);
bitField1_ = (bitField1_ & ~0x00000010);
}
result.repeatedNestedEnum_ = repeatedNestedEnum_;
if (((bitField1_ & 0x00000020) == 0x00000020)) {
repeatedCord_ = repeatedCord_.getUnmodifiableView();
bitField1_ = (bitField1_ & ~0x00000020);
}
result.repeatedCord_ = repeatedCord_;
if (oneofFieldCase_ == 111) {
result.oneofField_ = oneofField_;
}
if (oneofFieldCase_ == 112) {
if (oneofNestedMessageBuilder_ == null) {
result.oneofField_ = oneofField_;
} else {
result.oneofField_ = oneofNestedMessageBuilder_.build();
}
}
if (oneofFieldCase_ == 113) {
result.oneofField_ = oneofField_;
}
if (oneofFieldCase_ == 114) {
result.oneofField_ = oneofField_;
}
if (oneofFieldCase_ == 100) {
result.oneofField_ = oneofField_;
}
result.mapStringToMessage_ = internalGetMapStringToMessage();
result.mapStringToMessage_.makeImmutable();
result.mapInt32ToMessage_ = internalGetMapInt32ToMessage();
result.mapInt32ToMessage_.makeImmutable();
result.mapInt64ToMessage_ = internalGetMapInt64ToMessage();
result.mapInt64ToMessage_.makeImmutable();
result.mapBoolToMessage_ = internalGetMapBoolToMessage();
result.mapBoolToMessage_.makeImmutable();
result.mapStringToInt64_ = internalGetMapStringToInt64();
result.mapStringToInt64_.makeImmutable();
result.mapInt64ToString_ = internalGetMapInt64ToString();
result.mapInt64ToString_.makeImmutable();
result.anotherMapStringToMessage_ = internalGetAnotherMapStringToMessage();
result.anotherMapStringToMessage_.makeImmutable();
if (((bitField1_ & 0x00040000) == 0x00040000)) {
packedRepeatedInt64_ = java.util.Collections.unmodifiableList(packedRepeatedInt64_);
bitField1_ = (bitField1_ & ~0x00040000);
}
result.packedRepeatedInt64_ = packedRepeatedInt64_;
result.bitField0_ = to_bitField0_;
result.oneofFieldCase_ = oneofFieldCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.test.Test.TestAllTypes) {
return mergeFrom((tensorflow.test.Test.TestAllTypes)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.test.Test.TestAllTypes other) {
if (other == tensorflow.test.Test.TestAllTypes.getDefaultInstance()) return this;
if (other.getOptionalInt32() != 0) {
setOptionalInt32(other.getOptionalInt32());
}
if (other.getOptionalInt64() != 0L) {
setOptionalInt64(other.getOptionalInt64());
}
if (other.getOptionalUint32() != 0) {
setOptionalUint32(other.getOptionalUint32());
}
if (other.getOptionalUint64() != 0L) {
setOptionalUint64(other.getOptionalUint64());
}
if (other.getOptionalSint32() != 0) {
setOptionalSint32(other.getOptionalSint32());
}
if (other.getOptionalSint64() != 0L) {
setOptionalSint64(other.getOptionalSint64());
}
if (other.getOptionalFixed32() != 0) {
setOptionalFixed32(other.getOptionalFixed32());
}
if (other.getOptionalFixed64() != 0L) {
setOptionalFixed64(other.getOptionalFixed64());
}
if (other.getOptionalSfixed32() != 0) {
setOptionalSfixed32(other.getOptionalSfixed32());
}
if (other.getOptionalSfixed64() != 0L) {
setOptionalSfixed64(other.getOptionalSfixed64());
}
if (other.getOptionalFloat() != 0F) {
setOptionalFloat(other.getOptionalFloat());
}
if (other.getOptionalDouble() != 0D) {
setOptionalDouble(other.getOptionalDouble());
}
if (other.getOptionalBool() != false) {
setOptionalBool(other.getOptionalBool());
}
if (!other.getOptionalString().isEmpty()) {
optionalString_ = other.optionalString_;
onChanged();
}
if (other.getOptionalBytes() != com.google.protobuf.ByteString.EMPTY) {
setOptionalBytes(other.getOptionalBytes());
}
if (other.hasOptionalNestedMessage()) {
mergeOptionalNestedMessage(other.getOptionalNestedMessage());
}
if (other.hasOptionalForeignMessage()) {
mergeOptionalForeignMessage(other.getOptionalForeignMessage());
}
if (other.optionalNestedEnum_ != 0) {
setOptionalNestedEnumValue(other.getOptionalNestedEnumValue());
}
if (other.optionalForeignEnum_ != 0) {
setOptionalForeignEnumValue(other.getOptionalForeignEnumValue());
}
if (!other.getOptionalCord().isEmpty()) {
optionalCord_ = other.optionalCord_;
onChanged();
}
if (!other.repeatedInt32_.isEmpty()) {
if (repeatedInt32_.isEmpty()) {
repeatedInt32_ = other.repeatedInt32_;
bitField0_ = (bitField0_ & ~0x00100000);
} else {
ensureRepeatedInt32IsMutable();
repeatedInt32_.addAll(other.repeatedInt32_);
}
onChanged();
}
if (!other.repeatedInt64_.isEmpty()) {
if (repeatedInt64_.isEmpty()) {
repeatedInt64_ = other.repeatedInt64_;
bitField0_ = (bitField0_ & ~0x00200000);
} else {
ensureRepeatedInt64IsMutable();
repeatedInt64_.addAll(other.repeatedInt64_);
}
onChanged();
}
if (!other.repeatedUint32_.isEmpty()) {
if (repeatedUint32_.isEmpty()) {
repeatedUint32_ = other.repeatedUint32_;
bitField0_ = (bitField0_ & ~0x00400000);
} else {
ensureRepeatedUint32IsMutable();
repeatedUint32_.addAll(other.repeatedUint32_);
}
onChanged();
}
if (!other.repeatedUint64_.isEmpty()) {
if (repeatedUint64_.isEmpty()) {
repeatedUint64_ = other.repeatedUint64_;
bitField0_ = (bitField0_ & ~0x00800000);
} else {
ensureRepeatedUint64IsMutable();
repeatedUint64_.addAll(other.repeatedUint64_);
}
onChanged();
}
if (!other.repeatedSint32_.isEmpty()) {
if (repeatedSint32_.isEmpty()) {
repeatedSint32_ = other.repeatedSint32_;
bitField0_ = (bitField0_ & ~0x01000000);
} else {
ensureRepeatedSint32IsMutable();
repeatedSint32_.addAll(other.repeatedSint32_);
}
onChanged();
}
if (!other.repeatedSint64_.isEmpty()) {
if (repeatedSint64_.isEmpty()) {
repeatedSint64_ = other.repeatedSint64_;
bitField0_ = (bitField0_ & ~0x02000000);
} else {
ensureRepeatedSint64IsMutable();
repeatedSint64_.addAll(other.repeatedSint64_);
}
onChanged();
}
if (!other.repeatedFixed32_.isEmpty()) {
if (repeatedFixed32_.isEmpty()) {
repeatedFixed32_ = other.repeatedFixed32_;
bitField0_ = (bitField0_ & ~0x04000000);
} else {
ensureRepeatedFixed32IsMutable();
repeatedFixed32_.addAll(other.repeatedFixed32_);
}
onChanged();
}
if (!other.repeatedFixed64_.isEmpty()) {
if (repeatedFixed64_.isEmpty()) {
repeatedFixed64_ = other.repeatedFixed64_;
bitField0_ = (bitField0_ & ~0x08000000);
} else {
ensureRepeatedFixed64IsMutable();
repeatedFixed64_.addAll(other.repeatedFixed64_);
}
onChanged();
}
if (!other.repeatedSfixed32_.isEmpty()) {
if (repeatedSfixed32_.isEmpty()) {
repeatedSfixed32_ = other.repeatedSfixed32_;
bitField0_ = (bitField0_ & ~0x10000000);
} else {
ensureRepeatedSfixed32IsMutable();
repeatedSfixed32_.addAll(other.repeatedSfixed32_);
}
onChanged();
}
if (!other.repeatedSfixed64_.isEmpty()) {
if (repeatedSfixed64_.isEmpty()) {
repeatedSfixed64_ = other.repeatedSfixed64_;
bitField0_ = (bitField0_ & ~0x20000000);
} else {
ensureRepeatedSfixed64IsMutable();
repeatedSfixed64_.addAll(other.repeatedSfixed64_);
}
onChanged();
}
if (!other.repeatedFloat_.isEmpty()) {
if (repeatedFloat_.isEmpty()) {
repeatedFloat_ = other.repeatedFloat_;
bitField0_ = (bitField0_ & ~0x40000000);
} else {
ensureRepeatedFloatIsMutable();
repeatedFloat_.addAll(other.repeatedFloat_);
}
onChanged();
}
if (!other.repeatedDouble_.isEmpty()) {
if (repeatedDouble_.isEmpty()) {
repeatedDouble_ = other.repeatedDouble_;
bitField0_ = (bitField0_ & ~0x80000000);
} else {
ensureRepeatedDoubleIsMutable();
repeatedDouble_.addAll(other.repeatedDouble_);
}
onChanged();
}
if (!other.repeatedBool_.isEmpty()) {
if (repeatedBool_.isEmpty()) {
repeatedBool_ = other.repeatedBool_;
bitField1_ = (bitField1_ & ~0x00000001);
} else {
ensureRepeatedBoolIsMutable();
repeatedBool_.addAll(other.repeatedBool_);
}
onChanged();
}
if (!other.repeatedString_.isEmpty()) {
if (repeatedString_.isEmpty()) {
repeatedString_ = other.repeatedString_;
bitField1_ = (bitField1_ & ~0x00000002);
} else {
ensureRepeatedStringIsMutable();
repeatedString_.addAll(other.repeatedString_);
}
onChanged();
}
if (!other.repeatedBytes_.isEmpty()) {
if (repeatedBytes_.isEmpty()) {
repeatedBytes_ = other.repeatedBytes_;
bitField1_ = (bitField1_ & ~0x00000004);
} else {
ensureRepeatedBytesIsMutable();
repeatedBytes_.addAll(other.repeatedBytes_);
}
onChanged();
}
if (repeatedNestedMessageBuilder_ == null) {
if (!other.repeatedNestedMessage_.isEmpty()) {
if (repeatedNestedMessage_.isEmpty()) {
repeatedNestedMessage_ = other.repeatedNestedMessage_;
bitField1_ = (bitField1_ & ~0x00000008);
} else {
ensureRepeatedNestedMessageIsMutable();
repeatedNestedMessage_.addAll(other.repeatedNestedMessage_);
}
onChanged();
}
} else {
if (!other.repeatedNestedMessage_.isEmpty()) {
if (repeatedNestedMessageBuilder_.isEmpty()) {
repeatedNestedMessageBuilder_.dispose();
repeatedNestedMessageBuilder_ = null;
repeatedNestedMessage_ = other.repeatedNestedMessage_;
bitField1_ = (bitField1_ & ~0x00000008);
repeatedNestedMessageBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getRepeatedNestedMessageFieldBuilder() : null;
} else {
repeatedNestedMessageBuilder_.addAllMessages(other.repeatedNestedMessage_);
}
}
}
if (!other.repeatedNestedEnum_.isEmpty()) {
if (repeatedNestedEnum_.isEmpty()) {
repeatedNestedEnum_ = other.repeatedNestedEnum_;
bitField1_ = (bitField1_ & ~0x00000010);
} else {
ensureRepeatedNestedEnumIsMutable();
repeatedNestedEnum_.addAll(other.repeatedNestedEnum_);
}
onChanged();
}
if (!other.repeatedCord_.isEmpty()) {
if (repeatedCord_.isEmpty()) {
repeatedCord_ = other.repeatedCord_;
bitField1_ = (bitField1_ & ~0x00000020);
} else {
ensureRepeatedCordIsMutable();
repeatedCord_.addAll(other.repeatedCord_);
}
onChanged();
}
internalGetMutableMapStringToMessage().mergeFrom(
other.internalGetMapStringToMessage());
internalGetMutableMapInt32ToMessage().mergeFrom(
other.internalGetMapInt32ToMessage());
internalGetMutableMapInt64ToMessage().mergeFrom(
other.internalGetMapInt64ToMessage());
internalGetMutableMapBoolToMessage().mergeFrom(
other.internalGetMapBoolToMessage());
internalGetMutableMapStringToInt64().mergeFrom(
other.internalGetMapStringToInt64());
internalGetMutableMapInt64ToString().mergeFrom(
other.internalGetMapInt64ToString());
internalGetMutableAnotherMapStringToMessage().mergeFrom(
other.internalGetAnotherMapStringToMessage());
if (!other.packedRepeatedInt64_.isEmpty()) {
if (packedRepeatedInt64_.isEmpty()) {
packedRepeatedInt64_ = other.packedRepeatedInt64_;
bitField1_ = (bitField1_ & ~0x00040000);
} else {
ensurePackedRepeatedInt64IsMutable();
packedRepeatedInt64_.addAll(other.packedRepeatedInt64_);
}
onChanged();
}
switch (other.getOneofFieldCase()) {
case ONEOF_UINT32: {
setOneofUint32(other.getOneofUint32());
break;
}
case ONEOF_NESTED_MESSAGE: {
mergeOneofNestedMessage(other.getOneofNestedMessage());
break;
}
case ONEOF_STRING: {
oneofFieldCase_ = 113;
oneofField_ = other.oneofField_;
onChanged();
break;
}
case ONEOF_BYTES: {
setOneofBytes(other.getOneofBytes());
break;
}
case ONEOF_ENUM: {
setOneofEnumValue(other.getOneofEnumValue());
break;
}
case ONEOFFIELD_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.test.Test.TestAllTypes parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.test.Test.TestAllTypes) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int oneofFieldCase_ = 0;
private java.lang.Object oneofField_;
public OneofFieldCase
getOneofFieldCase() {
return OneofFieldCase.forNumber(
oneofFieldCase_);
}
public Builder clearOneofField() {
oneofFieldCase_ = 0;
oneofField_ = null;
onChanged();
return this;
}
private int bitField0_;
private int bitField1_;
private int optionalInt32_ ;
/**
*
* Singular
*
*
* int32 optional_int32 = 1000;
*/
public int getOptionalInt32() {
return optionalInt32_;
}
/**
*
* Singular
*
*
* int32 optional_int32 = 1000;
*/
public Builder setOptionalInt32(int value) {
optionalInt32_ = value;
onChanged();
return this;
}
/**
*
* Singular
*
*
* int32 optional_int32 = 1000;
*/
public Builder clearOptionalInt32() {
optionalInt32_ = 0;
onChanged();
return this;
}
private long optionalInt64_ ;
/**
* int64 optional_int64 = 2;
*/
public long getOptionalInt64() {
return optionalInt64_;
}
/**
* int64 optional_int64 = 2;
*/
public Builder setOptionalInt64(long value) {
optionalInt64_ = value;
onChanged();
return this;
}
/**
* int64 optional_int64 = 2;
*/
public Builder clearOptionalInt64() {
optionalInt64_ = 0L;
onChanged();
return this;
}
private int optionalUint32_ ;
/**
* uint32 optional_uint32 = 3;
*/
public int getOptionalUint32() {
return optionalUint32_;
}
/**
* uint32 optional_uint32 = 3;
*/
public Builder setOptionalUint32(int value) {
optionalUint32_ = value;
onChanged();
return this;
}
/**
* uint32 optional_uint32 = 3;
*/
public Builder clearOptionalUint32() {
optionalUint32_ = 0;
onChanged();
return this;
}
private long optionalUint64_ ;
/**
*
* use large tag to test output order.
*
*
* uint64 optional_uint64 = 999;
*/
public long getOptionalUint64() {
return optionalUint64_;
}
/**
*
* use large tag to test output order.
*
*
* uint64 optional_uint64 = 999;
*/
public Builder setOptionalUint64(long value) {
optionalUint64_ = value;
onChanged();
return this;
}
/**
*
* use large tag to test output order.
*
*
* uint64 optional_uint64 = 999;
*/
public Builder clearOptionalUint64() {
optionalUint64_ = 0L;
onChanged();
return this;
}
private int optionalSint32_ ;
/**
* sint32 optional_sint32 = 5;
*/
public int getOptionalSint32() {
return optionalSint32_;
}
/**
* sint32 optional_sint32 = 5;
*/
public Builder setOptionalSint32(int value) {
optionalSint32_ = value;
onChanged();
return this;
}
/**
* sint32 optional_sint32 = 5;
*/
public Builder clearOptionalSint32() {
optionalSint32_ = 0;
onChanged();
return this;
}
private long optionalSint64_ ;
/**
* sint64 optional_sint64 = 6;
*/
public long getOptionalSint64() {
return optionalSint64_;
}
/**
* sint64 optional_sint64 = 6;
*/
public Builder setOptionalSint64(long value) {
optionalSint64_ = value;
onChanged();
return this;
}
/**
* sint64 optional_sint64 = 6;
*/
public Builder clearOptionalSint64() {
optionalSint64_ = 0L;
onChanged();
return this;
}
private int optionalFixed32_ ;
/**
* fixed32 optional_fixed32 = 7;
*/
public int getOptionalFixed32() {
return optionalFixed32_;
}
/**
* fixed32 optional_fixed32 = 7;
*/
public Builder setOptionalFixed32(int value) {
optionalFixed32_ = value;
onChanged();
return this;
}
/**
* fixed32 optional_fixed32 = 7;
*/
public Builder clearOptionalFixed32() {
optionalFixed32_ = 0;
onChanged();
return this;
}
private long optionalFixed64_ ;
/**
* fixed64 optional_fixed64 = 8;
*/
public long getOptionalFixed64() {
return optionalFixed64_;
}
/**
* fixed64 optional_fixed64 = 8;
*/
public Builder setOptionalFixed64(long value) {
optionalFixed64_ = value;
onChanged();
return this;
}
/**
* fixed64 optional_fixed64 = 8;
*/
public Builder clearOptionalFixed64() {
optionalFixed64_ = 0L;
onChanged();
return this;
}
private int optionalSfixed32_ ;
/**
* sfixed32 optional_sfixed32 = 9;
*/
public int getOptionalSfixed32() {
return optionalSfixed32_;
}
/**
* sfixed32 optional_sfixed32 = 9;
*/
public Builder setOptionalSfixed32(int value) {
optionalSfixed32_ = value;
onChanged();
return this;
}
/**
* sfixed32 optional_sfixed32 = 9;
*/
public Builder clearOptionalSfixed32() {
optionalSfixed32_ = 0;
onChanged();
return this;
}
private long optionalSfixed64_ ;
/**
* sfixed64 optional_sfixed64 = 10;
*/
public long getOptionalSfixed64() {
return optionalSfixed64_;
}
/**
* sfixed64 optional_sfixed64 = 10;
*/
public Builder setOptionalSfixed64(long value) {
optionalSfixed64_ = value;
onChanged();
return this;
}
/**
* sfixed64 optional_sfixed64 = 10;
*/
public Builder clearOptionalSfixed64() {
optionalSfixed64_ = 0L;
onChanged();
return this;
}
private float optionalFloat_ ;
/**
* float optional_float = 11;
*/
public float getOptionalFloat() {
return optionalFloat_;
}
/**
* float optional_float = 11;
*/
public Builder setOptionalFloat(float value) {
optionalFloat_ = value;
onChanged();
return this;
}
/**
* float optional_float = 11;
*/
public Builder clearOptionalFloat() {
optionalFloat_ = 0F;
onChanged();
return this;
}
private double optionalDouble_ ;
/**
* double optional_double = 12;
*/
public double getOptionalDouble() {
return optionalDouble_;
}
/**
* double optional_double = 12;
*/
public Builder setOptionalDouble(double value) {
optionalDouble_ = value;
onChanged();
return this;
}
/**
* double optional_double = 12;
*/
public Builder clearOptionalDouble() {
optionalDouble_ = 0D;
onChanged();
return this;
}
private boolean optionalBool_ ;
/**
* bool optional_bool = 13;
*/
public boolean getOptionalBool() {
return optionalBool_;
}
/**
* bool optional_bool = 13;
*/
public Builder setOptionalBool(boolean value) {
optionalBool_ = value;
onChanged();
return this;
}
/**
* bool optional_bool = 13;
*/
public Builder clearOptionalBool() {
optionalBool_ = false;
onChanged();
return this;
}
private java.lang.Object optionalString_ = "";
/**
* string optional_string = 14;
*/
public java.lang.String getOptionalString() {
java.lang.Object ref = optionalString_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
optionalString_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string optional_string = 14;
*/
public com.google.protobuf.ByteString
getOptionalStringBytes() {
java.lang.Object ref = optionalString_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
optionalString_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string optional_string = 14;
*/
public Builder setOptionalString(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
optionalString_ = value;
onChanged();
return this;
}
/**
* string optional_string = 14;
*/
public Builder clearOptionalString() {
optionalString_ = getDefaultInstance().getOptionalString();
onChanged();
return this;
}
/**
* string optional_string = 14;
*/
public Builder setOptionalStringBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
optionalString_ = value;
onChanged();
return this;
}
private com.google.protobuf.ByteString optionalBytes_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes optional_bytes = 15;
*/
public com.google.protobuf.ByteString getOptionalBytes() {
return optionalBytes_;
}
/**
* bytes optional_bytes = 15;
*/
public Builder setOptionalBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
optionalBytes_ = value;
onChanged();
return this;
}
/**
* bytes optional_bytes = 15;
*/
public Builder clearOptionalBytes() {
optionalBytes_ = getDefaultInstance().getOptionalBytes();
onChanged();
return this;
}
private tensorflow.test.Test.TestAllTypes.NestedMessage optionalNestedMessage_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.test.Test.TestAllTypes.NestedMessage, tensorflow.test.Test.TestAllTypes.NestedMessage.Builder, tensorflow.test.Test.TestAllTypes.NestedMessageOrBuilder> optionalNestedMessageBuilder_;
/**
* .tensorflow.test.TestAllTypes.NestedMessage optional_nested_message = 18;
*/
public boolean hasOptionalNestedMessage() {
return optionalNestedMessageBuilder_ != null || optionalNestedMessage_ != null;
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage optional_nested_message = 18;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage getOptionalNestedMessage() {
if (optionalNestedMessageBuilder_ == null) {
return optionalNestedMessage_ == null ? tensorflow.test.Test.TestAllTypes.NestedMessage.getDefaultInstance() : optionalNestedMessage_;
} else {
return optionalNestedMessageBuilder_.getMessage();
}
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage optional_nested_message = 18;
*/
public Builder setOptionalNestedMessage(tensorflow.test.Test.TestAllTypes.NestedMessage value) {
if (optionalNestedMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
optionalNestedMessage_ = value;
onChanged();
} else {
optionalNestedMessageBuilder_.setMessage(value);
}
return this;
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage optional_nested_message = 18;
*/
public Builder setOptionalNestedMessage(
tensorflow.test.Test.TestAllTypes.NestedMessage.Builder builderForValue) {
if (optionalNestedMessageBuilder_ == null) {
optionalNestedMessage_ = builderForValue.build();
onChanged();
} else {
optionalNestedMessageBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage optional_nested_message = 18;
*/
public Builder mergeOptionalNestedMessage(tensorflow.test.Test.TestAllTypes.NestedMessage value) {
if (optionalNestedMessageBuilder_ == null) {
if (optionalNestedMessage_ != null) {
optionalNestedMessage_ =
tensorflow.test.Test.TestAllTypes.NestedMessage.newBuilder(optionalNestedMessage_).mergeFrom(value).buildPartial();
} else {
optionalNestedMessage_ = value;
}
onChanged();
} else {
optionalNestedMessageBuilder_.mergeFrom(value);
}
return this;
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage optional_nested_message = 18;
*/
public Builder clearOptionalNestedMessage() {
if (optionalNestedMessageBuilder_ == null) {
optionalNestedMessage_ = null;
onChanged();
} else {
optionalNestedMessage_ = null;
optionalNestedMessageBuilder_ = null;
}
return this;
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage optional_nested_message = 18;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage.Builder getOptionalNestedMessageBuilder() {
onChanged();
return getOptionalNestedMessageFieldBuilder().getBuilder();
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage optional_nested_message = 18;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessageOrBuilder getOptionalNestedMessageOrBuilder() {
if (optionalNestedMessageBuilder_ != null) {
return optionalNestedMessageBuilder_.getMessageOrBuilder();
} else {
return optionalNestedMessage_ == null ?
tensorflow.test.Test.TestAllTypes.NestedMessage.getDefaultInstance() : optionalNestedMessage_;
}
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage optional_nested_message = 18;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.test.Test.TestAllTypes.NestedMessage, tensorflow.test.Test.TestAllTypes.NestedMessage.Builder, tensorflow.test.Test.TestAllTypes.NestedMessageOrBuilder>
getOptionalNestedMessageFieldBuilder() {
if (optionalNestedMessageBuilder_ == null) {
optionalNestedMessageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.test.Test.TestAllTypes.NestedMessage, tensorflow.test.Test.TestAllTypes.NestedMessage.Builder, tensorflow.test.Test.TestAllTypes.NestedMessageOrBuilder>(
getOptionalNestedMessage(),
getParentForChildren(),
isClean());
optionalNestedMessage_ = null;
}
return optionalNestedMessageBuilder_;
}
private tensorflow.test.Test.ForeignMessage optionalForeignMessage_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.test.Test.ForeignMessage, tensorflow.test.Test.ForeignMessage.Builder, tensorflow.test.Test.ForeignMessageOrBuilder> optionalForeignMessageBuilder_;
/**
* .tensorflow.test.ForeignMessage optional_foreign_message = 19;
*/
public boolean hasOptionalForeignMessage() {
return optionalForeignMessageBuilder_ != null || optionalForeignMessage_ != null;
}
/**
* .tensorflow.test.ForeignMessage optional_foreign_message = 19;
*/
public tensorflow.test.Test.ForeignMessage getOptionalForeignMessage() {
if (optionalForeignMessageBuilder_ == null) {
return optionalForeignMessage_ == null ? tensorflow.test.Test.ForeignMessage.getDefaultInstance() : optionalForeignMessage_;
} else {
return optionalForeignMessageBuilder_.getMessage();
}
}
/**
* .tensorflow.test.ForeignMessage optional_foreign_message = 19;
*/
public Builder setOptionalForeignMessage(tensorflow.test.Test.ForeignMessage value) {
if (optionalForeignMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
optionalForeignMessage_ = value;
onChanged();
} else {
optionalForeignMessageBuilder_.setMessage(value);
}
return this;
}
/**
* .tensorflow.test.ForeignMessage optional_foreign_message = 19;
*/
public Builder setOptionalForeignMessage(
tensorflow.test.Test.ForeignMessage.Builder builderForValue) {
if (optionalForeignMessageBuilder_ == null) {
optionalForeignMessage_ = builderForValue.build();
onChanged();
} else {
optionalForeignMessageBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .tensorflow.test.ForeignMessage optional_foreign_message = 19;
*/
public Builder mergeOptionalForeignMessage(tensorflow.test.Test.ForeignMessage value) {
if (optionalForeignMessageBuilder_ == null) {
if (optionalForeignMessage_ != null) {
optionalForeignMessage_ =
tensorflow.test.Test.ForeignMessage.newBuilder(optionalForeignMessage_).mergeFrom(value).buildPartial();
} else {
optionalForeignMessage_ = value;
}
onChanged();
} else {
optionalForeignMessageBuilder_.mergeFrom(value);
}
return this;
}
/**
* .tensorflow.test.ForeignMessage optional_foreign_message = 19;
*/
public Builder clearOptionalForeignMessage() {
if (optionalForeignMessageBuilder_ == null) {
optionalForeignMessage_ = null;
onChanged();
} else {
optionalForeignMessage_ = null;
optionalForeignMessageBuilder_ = null;
}
return this;
}
/**
* .tensorflow.test.ForeignMessage optional_foreign_message = 19;
*/
public tensorflow.test.Test.ForeignMessage.Builder getOptionalForeignMessageBuilder() {
onChanged();
return getOptionalForeignMessageFieldBuilder().getBuilder();
}
/**
* .tensorflow.test.ForeignMessage optional_foreign_message = 19;
*/
public tensorflow.test.Test.ForeignMessageOrBuilder getOptionalForeignMessageOrBuilder() {
if (optionalForeignMessageBuilder_ != null) {
return optionalForeignMessageBuilder_.getMessageOrBuilder();
} else {
return optionalForeignMessage_ == null ?
tensorflow.test.Test.ForeignMessage.getDefaultInstance() : optionalForeignMessage_;
}
}
/**
* .tensorflow.test.ForeignMessage optional_foreign_message = 19;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.test.Test.ForeignMessage, tensorflow.test.Test.ForeignMessage.Builder, tensorflow.test.Test.ForeignMessageOrBuilder>
getOptionalForeignMessageFieldBuilder() {
if (optionalForeignMessageBuilder_ == null) {
optionalForeignMessageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.test.Test.ForeignMessage, tensorflow.test.Test.ForeignMessage.Builder, tensorflow.test.Test.ForeignMessageOrBuilder>(
getOptionalForeignMessage(),
getParentForChildren(),
isClean());
optionalForeignMessage_ = null;
}
return optionalForeignMessageBuilder_;
}
private int optionalNestedEnum_ = 0;
/**
* .tensorflow.test.TestAllTypes.NestedEnum optional_nested_enum = 21;
*/
public int getOptionalNestedEnumValue() {
return optionalNestedEnum_;
}
/**
* .tensorflow.test.TestAllTypes.NestedEnum optional_nested_enum = 21;
*/
public Builder setOptionalNestedEnumValue(int value) {
optionalNestedEnum_ = value;
onChanged();
return this;
}
/**
* .tensorflow.test.TestAllTypes.NestedEnum optional_nested_enum = 21;
*/
public tensorflow.test.Test.TestAllTypes.NestedEnum getOptionalNestedEnum() {
@SuppressWarnings("deprecation")
tensorflow.test.Test.TestAllTypes.NestedEnum result = tensorflow.test.Test.TestAllTypes.NestedEnum.valueOf(optionalNestedEnum_);
return result == null ? tensorflow.test.Test.TestAllTypes.NestedEnum.UNRECOGNIZED : result;
}
/**
* .tensorflow.test.TestAllTypes.NestedEnum optional_nested_enum = 21;
*/
public Builder setOptionalNestedEnum(tensorflow.test.Test.TestAllTypes.NestedEnum value) {
if (value == null) {
throw new NullPointerException();
}
optionalNestedEnum_ = value.getNumber();
onChanged();
return this;
}
/**
* .tensorflow.test.TestAllTypes.NestedEnum optional_nested_enum = 21;
*/
public Builder clearOptionalNestedEnum() {
optionalNestedEnum_ = 0;
onChanged();
return this;
}
private int optionalForeignEnum_ = 0;
/**
* .tensorflow.test.ForeignEnum optional_foreign_enum = 22;
*/
public int getOptionalForeignEnumValue() {
return optionalForeignEnum_;
}
/**
* .tensorflow.test.ForeignEnum optional_foreign_enum = 22;
*/
public Builder setOptionalForeignEnumValue(int value) {
optionalForeignEnum_ = value;
onChanged();
return this;
}
/**
* .tensorflow.test.ForeignEnum optional_foreign_enum = 22;
*/
public tensorflow.test.Test.ForeignEnum getOptionalForeignEnum() {
@SuppressWarnings("deprecation")
tensorflow.test.Test.ForeignEnum result = tensorflow.test.Test.ForeignEnum.valueOf(optionalForeignEnum_);
return result == null ? tensorflow.test.Test.ForeignEnum.UNRECOGNIZED : result;
}
/**
* .tensorflow.test.ForeignEnum optional_foreign_enum = 22;
*/
public Builder setOptionalForeignEnum(tensorflow.test.Test.ForeignEnum value) {
if (value == null) {
throw new NullPointerException();
}
optionalForeignEnum_ = value.getNumber();
onChanged();
return this;
}
/**
* .tensorflow.test.ForeignEnum optional_foreign_enum = 22;
*/
public Builder clearOptionalForeignEnum() {
optionalForeignEnum_ = 0;
onChanged();
return this;
}
private java.lang.Object optionalCord_ = "";
/**
* string optional_cord = 25;
*/
public java.lang.String getOptionalCord() {
java.lang.Object ref = optionalCord_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
optionalCord_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string optional_cord = 25;
*/
public com.google.protobuf.ByteString
getOptionalCordBytes() {
java.lang.Object ref = optionalCord_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
optionalCord_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string optional_cord = 25;
*/
public Builder setOptionalCord(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
optionalCord_ = value;
onChanged();
return this;
}
/**
* string optional_cord = 25;
*/
public Builder clearOptionalCord() {
optionalCord_ = getDefaultInstance().getOptionalCord();
onChanged();
return this;
}
/**
* string optional_cord = 25;
*/
public Builder setOptionalCordBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
optionalCord_ = value;
onChanged();
return this;
}
private java.util.List repeatedInt32_ = java.util.Collections.emptyList();
private void ensureRepeatedInt32IsMutable() {
if (!((bitField0_ & 0x00100000) == 0x00100000)) {
repeatedInt32_ = new java.util.ArrayList(repeatedInt32_);
bitField0_ |= 0x00100000;
}
}
/**
*
* Repeated
*
*
* repeated int32 repeated_int32 = 31;
*/
public java.util.List
getRepeatedInt32List() {
return java.util.Collections.unmodifiableList(repeatedInt32_);
}
/**
*
* Repeated
*
*
* repeated int32 repeated_int32 = 31;
*/
public int getRepeatedInt32Count() {
return repeatedInt32_.size();
}
/**
*
* Repeated
*
*
* repeated int32 repeated_int32 = 31;
*/
public int getRepeatedInt32(int index) {
return repeatedInt32_.get(index);
}
/**
*
* Repeated
*
*
* repeated int32 repeated_int32 = 31;
*/
public Builder setRepeatedInt32(
int index, int value) {
ensureRepeatedInt32IsMutable();
repeatedInt32_.set(index, value);
onChanged();
return this;
}
/**
*
* Repeated
*
*
* repeated int32 repeated_int32 = 31;
*/
public Builder addRepeatedInt32(int value) {
ensureRepeatedInt32IsMutable();
repeatedInt32_.add(value);
onChanged();
return this;
}
/**
*
* Repeated
*
*
* repeated int32 repeated_int32 = 31;
*/
public Builder addAllRepeatedInt32(
java.lang.Iterable extends java.lang.Integer> values) {
ensureRepeatedInt32IsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, repeatedInt32_);
onChanged();
return this;
}
/**
*
* Repeated
*
*
* repeated int32 repeated_int32 = 31;
*/
public Builder clearRepeatedInt32() {
repeatedInt32_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00100000);
onChanged();
return this;
}
private java.util.List repeatedInt64_ = java.util.Collections.emptyList();
private void ensureRepeatedInt64IsMutable() {
if (!((bitField0_ & 0x00200000) == 0x00200000)) {
repeatedInt64_ = new java.util.ArrayList(repeatedInt64_);
bitField0_ |= 0x00200000;
}
}
/**
* repeated int64 repeated_int64 = 32;
*/
public java.util.List
getRepeatedInt64List() {
return java.util.Collections.unmodifiableList(repeatedInt64_);
}
/**
* repeated int64 repeated_int64 = 32;
*/
public int getRepeatedInt64Count() {
return repeatedInt64_.size();
}
/**
* repeated int64 repeated_int64 = 32;
*/
public long getRepeatedInt64(int index) {
return repeatedInt64_.get(index);
}
/**
* repeated int64 repeated_int64 = 32;
*/
public Builder setRepeatedInt64(
int index, long value) {
ensureRepeatedInt64IsMutable();
repeatedInt64_.set(index, value);
onChanged();
return this;
}
/**
* repeated int64 repeated_int64 = 32;
*/
public Builder addRepeatedInt64(long value) {
ensureRepeatedInt64IsMutable();
repeatedInt64_.add(value);
onChanged();
return this;
}
/**
* repeated int64 repeated_int64 = 32;
*/
public Builder addAllRepeatedInt64(
java.lang.Iterable extends java.lang.Long> values) {
ensureRepeatedInt64IsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, repeatedInt64_);
onChanged();
return this;
}
/**
* repeated int64 repeated_int64 = 32;
*/
public Builder clearRepeatedInt64() {
repeatedInt64_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00200000);
onChanged();
return this;
}
private java.util.List repeatedUint32_ = java.util.Collections.emptyList();
private void ensureRepeatedUint32IsMutable() {
if (!((bitField0_ & 0x00400000) == 0x00400000)) {
repeatedUint32_ = new java.util.ArrayList(repeatedUint32_);
bitField0_ |= 0x00400000;
}
}
/**
* repeated uint32 repeated_uint32 = 33;
*/
public java.util.List
getRepeatedUint32List() {
return java.util.Collections.unmodifiableList(repeatedUint32_);
}
/**
* repeated uint32 repeated_uint32 = 33;
*/
public int getRepeatedUint32Count() {
return repeatedUint32_.size();
}
/**
* repeated uint32 repeated_uint32 = 33;
*/
public int getRepeatedUint32(int index) {
return repeatedUint32_.get(index);
}
/**
* repeated uint32 repeated_uint32 = 33;
*/
public Builder setRepeatedUint32(
int index, int value) {
ensureRepeatedUint32IsMutable();
repeatedUint32_.set(index, value);
onChanged();
return this;
}
/**
* repeated uint32 repeated_uint32 = 33;
*/
public Builder addRepeatedUint32(int value) {
ensureRepeatedUint32IsMutable();
repeatedUint32_.add(value);
onChanged();
return this;
}
/**
* repeated uint32 repeated_uint32 = 33;
*/
public Builder addAllRepeatedUint32(
java.lang.Iterable extends java.lang.Integer> values) {
ensureRepeatedUint32IsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, repeatedUint32_);
onChanged();
return this;
}
/**
* repeated uint32 repeated_uint32 = 33;
*/
public Builder clearRepeatedUint32() {
repeatedUint32_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00400000);
onChanged();
return this;
}
private java.util.List repeatedUint64_ = java.util.Collections.emptyList();
private void ensureRepeatedUint64IsMutable() {
if (!((bitField0_ & 0x00800000) == 0x00800000)) {
repeatedUint64_ = new java.util.ArrayList(repeatedUint64_);
bitField0_ |= 0x00800000;
}
}
/**
* repeated uint64 repeated_uint64 = 34;
*/
public java.util.List
getRepeatedUint64List() {
return java.util.Collections.unmodifiableList(repeatedUint64_);
}
/**
* repeated uint64 repeated_uint64 = 34;
*/
public int getRepeatedUint64Count() {
return repeatedUint64_.size();
}
/**
* repeated uint64 repeated_uint64 = 34;
*/
public long getRepeatedUint64(int index) {
return repeatedUint64_.get(index);
}
/**
* repeated uint64 repeated_uint64 = 34;
*/
public Builder setRepeatedUint64(
int index, long value) {
ensureRepeatedUint64IsMutable();
repeatedUint64_.set(index, value);
onChanged();
return this;
}
/**
* repeated uint64 repeated_uint64 = 34;
*/
public Builder addRepeatedUint64(long value) {
ensureRepeatedUint64IsMutable();
repeatedUint64_.add(value);
onChanged();
return this;
}
/**
* repeated uint64 repeated_uint64 = 34;
*/
public Builder addAllRepeatedUint64(
java.lang.Iterable extends java.lang.Long> values) {
ensureRepeatedUint64IsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, repeatedUint64_);
onChanged();
return this;
}
/**
* repeated uint64 repeated_uint64 = 34;
*/
public Builder clearRepeatedUint64() {
repeatedUint64_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00800000);
onChanged();
return this;
}
private java.util.List repeatedSint32_ = java.util.Collections.emptyList();
private void ensureRepeatedSint32IsMutable() {
if (!((bitField0_ & 0x01000000) == 0x01000000)) {
repeatedSint32_ = new java.util.ArrayList(repeatedSint32_);
bitField0_ |= 0x01000000;
}
}
/**
* repeated sint32 repeated_sint32 = 35;
*/
public java.util.List
getRepeatedSint32List() {
return java.util.Collections.unmodifiableList(repeatedSint32_);
}
/**
* repeated sint32 repeated_sint32 = 35;
*/
public int getRepeatedSint32Count() {
return repeatedSint32_.size();
}
/**
* repeated sint32 repeated_sint32 = 35;
*/
public int getRepeatedSint32(int index) {
return repeatedSint32_.get(index);
}
/**
* repeated sint32 repeated_sint32 = 35;
*/
public Builder setRepeatedSint32(
int index, int value) {
ensureRepeatedSint32IsMutable();
repeatedSint32_.set(index, value);
onChanged();
return this;
}
/**
* repeated sint32 repeated_sint32 = 35;
*/
public Builder addRepeatedSint32(int value) {
ensureRepeatedSint32IsMutable();
repeatedSint32_.add(value);
onChanged();
return this;
}
/**
* repeated sint32 repeated_sint32 = 35;
*/
public Builder addAllRepeatedSint32(
java.lang.Iterable extends java.lang.Integer> values) {
ensureRepeatedSint32IsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, repeatedSint32_);
onChanged();
return this;
}
/**
* repeated sint32 repeated_sint32 = 35;
*/
public Builder clearRepeatedSint32() {
repeatedSint32_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x01000000);
onChanged();
return this;
}
private java.util.List repeatedSint64_ = java.util.Collections.emptyList();
private void ensureRepeatedSint64IsMutable() {
if (!((bitField0_ & 0x02000000) == 0x02000000)) {
repeatedSint64_ = new java.util.ArrayList(repeatedSint64_);
bitField0_ |= 0x02000000;
}
}
/**
* repeated sint64 repeated_sint64 = 36;
*/
public java.util.List
getRepeatedSint64List() {
return java.util.Collections.unmodifiableList(repeatedSint64_);
}
/**
* repeated sint64 repeated_sint64 = 36;
*/
public int getRepeatedSint64Count() {
return repeatedSint64_.size();
}
/**
* repeated sint64 repeated_sint64 = 36;
*/
public long getRepeatedSint64(int index) {
return repeatedSint64_.get(index);
}
/**
* repeated sint64 repeated_sint64 = 36;
*/
public Builder setRepeatedSint64(
int index, long value) {
ensureRepeatedSint64IsMutable();
repeatedSint64_.set(index, value);
onChanged();
return this;
}
/**
* repeated sint64 repeated_sint64 = 36;
*/
public Builder addRepeatedSint64(long value) {
ensureRepeatedSint64IsMutable();
repeatedSint64_.add(value);
onChanged();
return this;
}
/**
* repeated sint64 repeated_sint64 = 36;
*/
public Builder addAllRepeatedSint64(
java.lang.Iterable extends java.lang.Long> values) {
ensureRepeatedSint64IsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, repeatedSint64_);
onChanged();
return this;
}
/**
* repeated sint64 repeated_sint64 = 36;
*/
public Builder clearRepeatedSint64() {
repeatedSint64_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x02000000);
onChanged();
return this;
}
private java.util.List repeatedFixed32_ = java.util.Collections.emptyList();
private void ensureRepeatedFixed32IsMutable() {
if (!((bitField0_ & 0x04000000) == 0x04000000)) {
repeatedFixed32_ = new java.util.ArrayList(repeatedFixed32_);
bitField0_ |= 0x04000000;
}
}
/**
* repeated fixed32 repeated_fixed32 = 37;
*/
public java.util.List
getRepeatedFixed32List() {
return java.util.Collections.unmodifiableList(repeatedFixed32_);
}
/**
* repeated fixed32 repeated_fixed32 = 37;
*/
public int getRepeatedFixed32Count() {
return repeatedFixed32_.size();
}
/**
* repeated fixed32 repeated_fixed32 = 37;
*/
public int getRepeatedFixed32(int index) {
return repeatedFixed32_.get(index);
}
/**
* repeated fixed32 repeated_fixed32 = 37;
*/
public Builder setRepeatedFixed32(
int index, int value) {
ensureRepeatedFixed32IsMutable();
repeatedFixed32_.set(index, value);
onChanged();
return this;
}
/**
* repeated fixed32 repeated_fixed32 = 37;
*/
public Builder addRepeatedFixed32(int value) {
ensureRepeatedFixed32IsMutable();
repeatedFixed32_.add(value);
onChanged();
return this;
}
/**
* repeated fixed32 repeated_fixed32 = 37;
*/
public Builder addAllRepeatedFixed32(
java.lang.Iterable extends java.lang.Integer> values) {
ensureRepeatedFixed32IsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, repeatedFixed32_);
onChanged();
return this;
}
/**
* repeated fixed32 repeated_fixed32 = 37;
*/
public Builder clearRepeatedFixed32() {
repeatedFixed32_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x04000000);
onChanged();
return this;
}
private java.util.List repeatedFixed64_ = java.util.Collections.emptyList();
private void ensureRepeatedFixed64IsMutable() {
if (!((bitField0_ & 0x08000000) == 0x08000000)) {
repeatedFixed64_ = new java.util.ArrayList(repeatedFixed64_);
bitField0_ |= 0x08000000;
}
}
/**
* repeated fixed64 repeated_fixed64 = 38;
*/
public java.util.List
getRepeatedFixed64List() {
return java.util.Collections.unmodifiableList(repeatedFixed64_);
}
/**
* repeated fixed64 repeated_fixed64 = 38;
*/
public int getRepeatedFixed64Count() {
return repeatedFixed64_.size();
}
/**
* repeated fixed64 repeated_fixed64 = 38;
*/
public long getRepeatedFixed64(int index) {
return repeatedFixed64_.get(index);
}
/**
* repeated fixed64 repeated_fixed64 = 38;
*/
public Builder setRepeatedFixed64(
int index, long value) {
ensureRepeatedFixed64IsMutable();
repeatedFixed64_.set(index, value);
onChanged();
return this;
}
/**
* repeated fixed64 repeated_fixed64 = 38;
*/
public Builder addRepeatedFixed64(long value) {
ensureRepeatedFixed64IsMutable();
repeatedFixed64_.add(value);
onChanged();
return this;
}
/**
* repeated fixed64 repeated_fixed64 = 38;
*/
public Builder addAllRepeatedFixed64(
java.lang.Iterable extends java.lang.Long> values) {
ensureRepeatedFixed64IsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, repeatedFixed64_);
onChanged();
return this;
}
/**
* repeated fixed64 repeated_fixed64 = 38;
*/
public Builder clearRepeatedFixed64() {
repeatedFixed64_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x08000000);
onChanged();
return this;
}
private java.util.List repeatedSfixed32_ = java.util.Collections.emptyList();
private void ensureRepeatedSfixed32IsMutable() {
if (!((bitField0_ & 0x10000000) == 0x10000000)) {
repeatedSfixed32_ = new java.util.ArrayList(repeatedSfixed32_);
bitField0_ |= 0x10000000;
}
}
/**
* repeated sfixed32 repeated_sfixed32 = 39;
*/
public java.util.List
getRepeatedSfixed32List() {
return java.util.Collections.unmodifiableList(repeatedSfixed32_);
}
/**
* repeated sfixed32 repeated_sfixed32 = 39;
*/
public int getRepeatedSfixed32Count() {
return repeatedSfixed32_.size();
}
/**
* repeated sfixed32 repeated_sfixed32 = 39;
*/
public int getRepeatedSfixed32(int index) {
return repeatedSfixed32_.get(index);
}
/**
* repeated sfixed32 repeated_sfixed32 = 39;
*/
public Builder setRepeatedSfixed32(
int index, int value) {
ensureRepeatedSfixed32IsMutable();
repeatedSfixed32_.set(index, value);
onChanged();
return this;
}
/**
* repeated sfixed32 repeated_sfixed32 = 39;
*/
public Builder addRepeatedSfixed32(int value) {
ensureRepeatedSfixed32IsMutable();
repeatedSfixed32_.add(value);
onChanged();
return this;
}
/**
* repeated sfixed32 repeated_sfixed32 = 39;
*/
public Builder addAllRepeatedSfixed32(
java.lang.Iterable extends java.lang.Integer> values) {
ensureRepeatedSfixed32IsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, repeatedSfixed32_);
onChanged();
return this;
}
/**
* repeated sfixed32 repeated_sfixed32 = 39;
*/
public Builder clearRepeatedSfixed32() {
repeatedSfixed32_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x10000000);
onChanged();
return this;
}
private java.util.List repeatedSfixed64_ = java.util.Collections.emptyList();
private void ensureRepeatedSfixed64IsMutable() {
if (!((bitField0_ & 0x20000000) == 0x20000000)) {
repeatedSfixed64_ = new java.util.ArrayList(repeatedSfixed64_);
bitField0_ |= 0x20000000;
}
}
/**
* repeated sfixed64 repeated_sfixed64 = 40;
*/
public java.util.List
getRepeatedSfixed64List() {
return java.util.Collections.unmodifiableList(repeatedSfixed64_);
}
/**
* repeated sfixed64 repeated_sfixed64 = 40;
*/
public int getRepeatedSfixed64Count() {
return repeatedSfixed64_.size();
}
/**
* repeated sfixed64 repeated_sfixed64 = 40;
*/
public long getRepeatedSfixed64(int index) {
return repeatedSfixed64_.get(index);
}
/**
* repeated sfixed64 repeated_sfixed64 = 40;
*/
public Builder setRepeatedSfixed64(
int index, long value) {
ensureRepeatedSfixed64IsMutable();
repeatedSfixed64_.set(index, value);
onChanged();
return this;
}
/**
* repeated sfixed64 repeated_sfixed64 = 40;
*/
public Builder addRepeatedSfixed64(long value) {
ensureRepeatedSfixed64IsMutable();
repeatedSfixed64_.add(value);
onChanged();
return this;
}
/**
* repeated sfixed64 repeated_sfixed64 = 40;
*/
public Builder addAllRepeatedSfixed64(
java.lang.Iterable extends java.lang.Long> values) {
ensureRepeatedSfixed64IsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, repeatedSfixed64_);
onChanged();
return this;
}
/**
* repeated sfixed64 repeated_sfixed64 = 40;
*/
public Builder clearRepeatedSfixed64() {
repeatedSfixed64_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x20000000);
onChanged();
return this;
}
private java.util.List repeatedFloat_ = java.util.Collections.emptyList();
private void ensureRepeatedFloatIsMutable() {
if (!((bitField0_ & 0x40000000) == 0x40000000)) {
repeatedFloat_ = new java.util.ArrayList(repeatedFloat_);
bitField0_ |= 0x40000000;
}
}
/**
* repeated float repeated_float = 41;
*/
public java.util.List
getRepeatedFloatList() {
return java.util.Collections.unmodifiableList(repeatedFloat_);
}
/**
* repeated float repeated_float = 41;
*/
public int getRepeatedFloatCount() {
return repeatedFloat_.size();
}
/**
* repeated float repeated_float = 41;
*/
public float getRepeatedFloat(int index) {
return repeatedFloat_.get(index);
}
/**
* repeated float repeated_float = 41;
*/
public Builder setRepeatedFloat(
int index, float value) {
ensureRepeatedFloatIsMutable();
repeatedFloat_.set(index, value);
onChanged();
return this;
}
/**
* repeated float repeated_float = 41;
*/
public Builder addRepeatedFloat(float value) {
ensureRepeatedFloatIsMutable();
repeatedFloat_.add(value);
onChanged();
return this;
}
/**
* repeated float repeated_float = 41;
*/
public Builder addAllRepeatedFloat(
java.lang.Iterable extends java.lang.Float> values) {
ensureRepeatedFloatIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, repeatedFloat_);
onChanged();
return this;
}
/**
* repeated float repeated_float = 41;
*/
public Builder clearRepeatedFloat() {
repeatedFloat_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x40000000);
onChanged();
return this;
}
private java.util.List repeatedDouble_ = java.util.Collections.emptyList();
private void ensureRepeatedDoubleIsMutable() {
if (!((bitField0_ & 0x80000000) == 0x80000000)) {
repeatedDouble_ = new java.util.ArrayList(repeatedDouble_);
bitField0_ |= 0x80000000;
}
}
/**
* repeated double repeated_double = 42;
*/
public java.util.List
getRepeatedDoubleList() {
return java.util.Collections.unmodifiableList(repeatedDouble_);
}
/**
* repeated double repeated_double = 42;
*/
public int getRepeatedDoubleCount() {
return repeatedDouble_.size();
}
/**
* repeated double repeated_double = 42;
*/
public double getRepeatedDouble(int index) {
return repeatedDouble_.get(index);
}
/**
* repeated double repeated_double = 42;
*/
public Builder setRepeatedDouble(
int index, double value) {
ensureRepeatedDoubleIsMutable();
repeatedDouble_.set(index, value);
onChanged();
return this;
}
/**
* repeated double repeated_double = 42;
*/
public Builder addRepeatedDouble(double value) {
ensureRepeatedDoubleIsMutable();
repeatedDouble_.add(value);
onChanged();
return this;
}
/**
* repeated double repeated_double = 42;
*/
public Builder addAllRepeatedDouble(
java.lang.Iterable extends java.lang.Double> values) {
ensureRepeatedDoubleIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, repeatedDouble_);
onChanged();
return this;
}
/**
* repeated double repeated_double = 42;
*/
public Builder clearRepeatedDouble() {
repeatedDouble_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x80000000);
onChanged();
return this;
}
private java.util.List repeatedBool_ = java.util.Collections.emptyList();
private void ensureRepeatedBoolIsMutable() {
if (!((bitField1_ & 0x00000001) == 0x00000001)) {
repeatedBool_ = new java.util.ArrayList(repeatedBool_);
bitField1_ |= 0x00000001;
}
}
/**
* repeated bool repeated_bool = 43;
*/
public java.util.List
getRepeatedBoolList() {
return java.util.Collections.unmodifiableList(repeatedBool_);
}
/**
* repeated bool repeated_bool = 43;
*/
public int getRepeatedBoolCount() {
return repeatedBool_.size();
}
/**
* repeated bool repeated_bool = 43;
*/
public boolean getRepeatedBool(int index) {
return repeatedBool_.get(index);
}
/**
* repeated bool repeated_bool = 43;
*/
public Builder setRepeatedBool(
int index, boolean value) {
ensureRepeatedBoolIsMutable();
repeatedBool_.set(index, value);
onChanged();
return this;
}
/**
* repeated bool repeated_bool = 43;
*/
public Builder addRepeatedBool(boolean value) {
ensureRepeatedBoolIsMutable();
repeatedBool_.add(value);
onChanged();
return this;
}
/**
* repeated bool repeated_bool = 43;
*/
public Builder addAllRepeatedBool(
java.lang.Iterable extends java.lang.Boolean> values) {
ensureRepeatedBoolIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, repeatedBool_);
onChanged();
return this;
}
/**
* repeated bool repeated_bool = 43;
*/
public Builder clearRepeatedBool() {
repeatedBool_ = java.util.Collections.emptyList();
bitField1_ = (bitField1_ & ~0x00000001);
onChanged();
return this;
}
private com.google.protobuf.LazyStringList repeatedString_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureRepeatedStringIsMutable() {
if (!((bitField1_ & 0x00000002) == 0x00000002)) {
repeatedString_ = new com.google.protobuf.LazyStringArrayList(repeatedString_);
bitField1_ |= 0x00000002;
}
}
/**
* repeated string repeated_string = 44;
*/
public com.google.protobuf.ProtocolStringList
getRepeatedStringList() {
return repeatedString_.getUnmodifiableView();
}
/**
* repeated string repeated_string = 44;
*/
public int getRepeatedStringCount() {
return repeatedString_.size();
}
/**
* repeated string repeated_string = 44;
*/
public java.lang.String getRepeatedString(int index) {
return repeatedString_.get(index);
}
/**
* repeated string repeated_string = 44;
*/
public com.google.protobuf.ByteString
getRepeatedStringBytes(int index) {
return repeatedString_.getByteString(index);
}
/**
* repeated string repeated_string = 44;
*/
public Builder setRepeatedString(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureRepeatedStringIsMutable();
repeatedString_.set(index, value);
onChanged();
return this;
}
/**
* repeated string repeated_string = 44;
*/
public Builder addRepeatedString(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureRepeatedStringIsMutable();
repeatedString_.add(value);
onChanged();
return this;
}
/**
* repeated string repeated_string = 44;
*/
public Builder addAllRepeatedString(
java.lang.Iterable values) {
ensureRepeatedStringIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, repeatedString_);
onChanged();
return this;
}
/**
* repeated string repeated_string = 44;
*/
public Builder clearRepeatedString() {
repeatedString_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField1_ = (bitField1_ & ~0x00000002);
onChanged();
return this;
}
/**
* repeated string repeated_string = 44;
*/
public Builder addRepeatedStringBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureRepeatedStringIsMutable();
repeatedString_.add(value);
onChanged();
return this;
}
private java.util.List repeatedBytes_ = java.util.Collections.emptyList();
private void ensureRepeatedBytesIsMutable() {
if (!((bitField1_ & 0x00000004) == 0x00000004)) {
repeatedBytes_ = new java.util.ArrayList(repeatedBytes_);
bitField1_ |= 0x00000004;
}
}
/**
* repeated bytes repeated_bytes = 45;
*/
public java.util.List
getRepeatedBytesList() {
return java.util.Collections.unmodifiableList(repeatedBytes_);
}
/**
* repeated bytes repeated_bytes = 45;
*/
public int getRepeatedBytesCount() {
return repeatedBytes_.size();
}
/**
* repeated bytes repeated_bytes = 45;
*/
public com.google.protobuf.ByteString getRepeatedBytes(int index) {
return repeatedBytes_.get(index);
}
/**
* repeated bytes repeated_bytes = 45;
*/
public Builder setRepeatedBytes(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureRepeatedBytesIsMutable();
repeatedBytes_.set(index, value);
onChanged();
return this;
}
/**
* repeated bytes repeated_bytes = 45;
*/
public Builder addRepeatedBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureRepeatedBytesIsMutable();
repeatedBytes_.add(value);
onChanged();
return this;
}
/**
* repeated bytes repeated_bytes = 45;
*/
public Builder addAllRepeatedBytes(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureRepeatedBytesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, repeatedBytes_);
onChanged();
return this;
}
/**
* repeated bytes repeated_bytes = 45;
*/
public Builder clearRepeatedBytes() {
repeatedBytes_ = java.util.Collections.emptyList();
bitField1_ = (bitField1_ & ~0x00000004);
onChanged();
return this;
}
private java.util.List repeatedNestedMessage_ =
java.util.Collections.emptyList();
private void ensureRepeatedNestedMessageIsMutable() {
if (!((bitField1_ & 0x00000008) == 0x00000008)) {
repeatedNestedMessage_ = new java.util.ArrayList(repeatedNestedMessage_);
bitField1_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.test.Test.TestAllTypes.NestedMessage, tensorflow.test.Test.TestAllTypes.NestedMessage.Builder, tensorflow.test.Test.TestAllTypes.NestedMessageOrBuilder> repeatedNestedMessageBuilder_;
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
public java.util.List getRepeatedNestedMessageList() {
if (repeatedNestedMessageBuilder_ == null) {
return java.util.Collections.unmodifiableList(repeatedNestedMessage_);
} else {
return repeatedNestedMessageBuilder_.getMessageList();
}
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
public int getRepeatedNestedMessageCount() {
if (repeatedNestedMessageBuilder_ == null) {
return repeatedNestedMessage_.size();
} else {
return repeatedNestedMessageBuilder_.getCount();
}
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage getRepeatedNestedMessage(int index) {
if (repeatedNestedMessageBuilder_ == null) {
return repeatedNestedMessage_.get(index);
} else {
return repeatedNestedMessageBuilder_.getMessage(index);
}
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
public Builder setRepeatedNestedMessage(
int index, tensorflow.test.Test.TestAllTypes.NestedMessage value) {
if (repeatedNestedMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRepeatedNestedMessageIsMutable();
repeatedNestedMessage_.set(index, value);
onChanged();
} else {
repeatedNestedMessageBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
public Builder setRepeatedNestedMessage(
int index, tensorflow.test.Test.TestAllTypes.NestedMessage.Builder builderForValue) {
if (repeatedNestedMessageBuilder_ == null) {
ensureRepeatedNestedMessageIsMutable();
repeatedNestedMessage_.set(index, builderForValue.build());
onChanged();
} else {
repeatedNestedMessageBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
public Builder addRepeatedNestedMessage(tensorflow.test.Test.TestAllTypes.NestedMessage value) {
if (repeatedNestedMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRepeatedNestedMessageIsMutable();
repeatedNestedMessage_.add(value);
onChanged();
} else {
repeatedNestedMessageBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
public Builder addRepeatedNestedMessage(
int index, tensorflow.test.Test.TestAllTypes.NestedMessage value) {
if (repeatedNestedMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRepeatedNestedMessageIsMutable();
repeatedNestedMessage_.add(index, value);
onChanged();
} else {
repeatedNestedMessageBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
public Builder addRepeatedNestedMessage(
tensorflow.test.Test.TestAllTypes.NestedMessage.Builder builderForValue) {
if (repeatedNestedMessageBuilder_ == null) {
ensureRepeatedNestedMessageIsMutable();
repeatedNestedMessage_.add(builderForValue.build());
onChanged();
} else {
repeatedNestedMessageBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
public Builder addRepeatedNestedMessage(
int index, tensorflow.test.Test.TestAllTypes.NestedMessage.Builder builderForValue) {
if (repeatedNestedMessageBuilder_ == null) {
ensureRepeatedNestedMessageIsMutable();
repeatedNestedMessage_.add(index, builderForValue.build());
onChanged();
} else {
repeatedNestedMessageBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
public Builder addAllRepeatedNestedMessage(
java.lang.Iterable extends tensorflow.test.Test.TestAllTypes.NestedMessage> values) {
if (repeatedNestedMessageBuilder_ == null) {
ensureRepeatedNestedMessageIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, repeatedNestedMessage_);
onChanged();
} else {
repeatedNestedMessageBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
public Builder clearRepeatedNestedMessage() {
if (repeatedNestedMessageBuilder_ == null) {
repeatedNestedMessage_ = java.util.Collections.emptyList();
bitField1_ = (bitField1_ & ~0x00000008);
onChanged();
} else {
repeatedNestedMessageBuilder_.clear();
}
return this;
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
public Builder removeRepeatedNestedMessage(int index) {
if (repeatedNestedMessageBuilder_ == null) {
ensureRepeatedNestedMessageIsMutable();
repeatedNestedMessage_.remove(index);
onChanged();
} else {
repeatedNestedMessageBuilder_.remove(index);
}
return this;
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage.Builder getRepeatedNestedMessageBuilder(
int index) {
return getRepeatedNestedMessageFieldBuilder().getBuilder(index);
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessageOrBuilder getRepeatedNestedMessageOrBuilder(
int index) {
if (repeatedNestedMessageBuilder_ == null) {
return repeatedNestedMessage_.get(index); } else {
return repeatedNestedMessageBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
public java.util.List extends tensorflow.test.Test.TestAllTypes.NestedMessageOrBuilder>
getRepeatedNestedMessageOrBuilderList() {
if (repeatedNestedMessageBuilder_ != null) {
return repeatedNestedMessageBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(repeatedNestedMessage_);
}
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage.Builder addRepeatedNestedMessageBuilder() {
return getRepeatedNestedMessageFieldBuilder().addBuilder(
tensorflow.test.Test.TestAllTypes.NestedMessage.getDefaultInstance());
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage.Builder addRepeatedNestedMessageBuilder(
int index) {
return getRepeatedNestedMessageFieldBuilder().addBuilder(
index, tensorflow.test.Test.TestAllTypes.NestedMessage.getDefaultInstance());
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedMessage repeated_nested_message = 48;
*/
public java.util.List
getRepeatedNestedMessageBuilderList() {
return getRepeatedNestedMessageFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.test.Test.TestAllTypes.NestedMessage, tensorflow.test.Test.TestAllTypes.NestedMessage.Builder, tensorflow.test.Test.TestAllTypes.NestedMessageOrBuilder>
getRepeatedNestedMessageFieldBuilder() {
if (repeatedNestedMessageBuilder_ == null) {
repeatedNestedMessageBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.test.Test.TestAllTypes.NestedMessage, tensorflow.test.Test.TestAllTypes.NestedMessage.Builder, tensorflow.test.Test.TestAllTypes.NestedMessageOrBuilder>(
repeatedNestedMessage_,
((bitField1_ & 0x00000008) == 0x00000008),
getParentForChildren(),
isClean());
repeatedNestedMessage_ = null;
}
return repeatedNestedMessageBuilder_;
}
private java.util.List repeatedNestedEnum_ =
java.util.Collections.emptyList();
private void ensureRepeatedNestedEnumIsMutable() {
if (!((bitField1_ & 0x00000010) == 0x00000010)) {
repeatedNestedEnum_ = new java.util.ArrayList(repeatedNestedEnum_);
bitField1_ |= 0x00000010;
}
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedEnum repeated_nested_enum = 51;
*/
public java.util.List getRepeatedNestedEnumList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, tensorflow.test.Test.TestAllTypes.NestedEnum>(repeatedNestedEnum_, repeatedNestedEnum_converter_);
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedEnum repeated_nested_enum = 51;
*/
public int getRepeatedNestedEnumCount() {
return repeatedNestedEnum_.size();
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedEnum repeated_nested_enum = 51;
*/
public tensorflow.test.Test.TestAllTypes.NestedEnum getRepeatedNestedEnum(int index) {
return repeatedNestedEnum_converter_.convert(repeatedNestedEnum_.get(index));
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedEnum repeated_nested_enum = 51;
*/
public Builder setRepeatedNestedEnum(
int index, tensorflow.test.Test.TestAllTypes.NestedEnum value) {
if (value == null) {
throw new NullPointerException();
}
ensureRepeatedNestedEnumIsMutable();
repeatedNestedEnum_.set(index, value.getNumber());
onChanged();
return this;
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedEnum repeated_nested_enum = 51;
*/
public Builder addRepeatedNestedEnum(tensorflow.test.Test.TestAllTypes.NestedEnum value) {
if (value == null) {
throw new NullPointerException();
}
ensureRepeatedNestedEnumIsMutable();
repeatedNestedEnum_.add(value.getNumber());
onChanged();
return this;
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedEnum repeated_nested_enum = 51;
*/
public Builder addAllRepeatedNestedEnum(
java.lang.Iterable extends tensorflow.test.Test.TestAllTypes.NestedEnum> values) {
ensureRepeatedNestedEnumIsMutable();
for (tensorflow.test.Test.TestAllTypes.NestedEnum value : values) {
repeatedNestedEnum_.add(value.getNumber());
}
onChanged();
return this;
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedEnum repeated_nested_enum = 51;
*/
public Builder clearRepeatedNestedEnum() {
repeatedNestedEnum_ = java.util.Collections.emptyList();
bitField1_ = (bitField1_ & ~0x00000010);
onChanged();
return this;
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedEnum repeated_nested_enum = 51;
*/
public java.util.List
getRepeatedNestedEnumValueList() {
return java.util.Collections.unmodifiableList(repeatedNestedEnum_);
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedEnum repeated_nested_enum = 51;
*/
public int getRepeatedNestedEnumValue(int index) {
return repeatedNestedEnum_.get(index);
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedEnum repeated_nested_enum = 51;
*/
public Builder setRepeatedNestedEnumValue(
int index, int value) {
ensureRepeatedNestedEnumIsMutable();
repeatedNestedEnum_.set(index, value);
onChanged();
return this;
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedEnum repeated_nested_enum = 51;
*/
public Builder addRepeatedNestedEnumValue(int value) {
ensureRepeatedNestedEnumIsMutable();
repeatedNestedEnum_.add(value);
onChanged();
return this;
}
/**
* repeated .tensorflow.test.TestAllTypes.NestedEnum repeated_nested_enum = 51;
*/
public Builder addAllRepeatedNestedEnumValue(
java.lang.Iterable values) {
ensureRepeatedNestedEnumIsMutable();
for (int value : values) {
repeatedNestedEnum_.add(value);
}
onChanged();
return this;
}
private com.google.protobuf.LazyStringList repeatedCord_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureRepeatedCordIsMutable() {
if (!((bitField1_ & 0x00000020) == 0x00000020)) {
repeatedCord_ = new com.google.protobuf.LazyStringArrayList(repeatedCord_);
bitField1_ |= 0x00000020;
}
}
/**
* repeated string repeated_cord = 55;
*/
public com.google.protobuf.ProtocolStringList
getRepeatedCordList() {
return repeatedCord_.getUnmodifiableView();
}
/**
* repeated string repeated_cord = 55;
*/
public int getRepeatedCordCount() {
return repeatedCord_.size();
}
/**
* repeated string repeated_cord = 55;
*/
public java.lang.String getRepeatedCord(int index) {
return repeatedCord_.get(index);
}
/**
* repeated string repeated_cord = 55;
*/
public com.google.protobuf.ByteString
getRepeatedCordBytes(int index) {
return repeatedCord_.getByteString(index);
}
/**
* repeated string repeated_cord = 55;
*/
public Builder setRepeatedCord(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureRepeatedCordIsMutable();
repeatedCord_.set(index, value);
onChanged();
return this;
}
/**
* repeated string repeated_cord = 55;
*/
public Builder addRepeatedCord(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureRepeatedCordIsMutable();
repeatedCord_.add(value);
onChanged();
return this;
}
/**
* repeated string repeated_cord = 55;
*/
public Builder addAllRepeatedCord(
java.lang.Iterable values) {
ensureRepeatedCordIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, repeatedCord_);
onChanged();
return this;
}
/**
* repeated string repeated_cord = 55;
*/
public Builder clearRepeatedCord() {
repeatedCord_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField1_ = (bitField1_ & ~0x00000020);
onChanged();
return this;
}
/**
* repeated string repeated_cord = 55;
*/
public Builder addRepeatedCordBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureRepeatedCordIsMutable();
repeatedCord_.add(value);
onChanged();
return this;
}
/**
* uint32 oneof_uint32 = 111;
*/
public int getOneofUint32() {
if (oneofFieldCase_ == 111) {
return (java.lang.Integer) oneofField_;
}
return 0;
}
/**
* uint32 oneof_uint32 = 111;
*/
public Builder setOneofUint32(int value) {
oneofFieldCase_ = 111;
oneofField_ = value;
onChanged();
return this;
}
/**
* uint32 oneof_uint32 = 111;
*/
public Builder clearOneofUint32() {
if (oneofFieldCase_ == 111) {
oneofFieldCase_ = 0;
oneofField_ = null;
onChanged();
}
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.test.Test.TestAllTypes.NestedMessage, tensorflow.test.Test.TestAllTypes.NestedMessage.Builder, tensorflow.test.Test.TestAllTypes.NestedMessageOrBuilder> oneofNestedMessageBuilder_;
/**
* .tensorflow.test.TestAllTypes.NestedMessage oneof_nested_message = 112;
*/
public boolean hasOneofNestedMessage() {
return oneofFieldCase_ == 112;
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage oneof_nested_message = 112;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage getOneofNestedMessage() {
if (oneofNestedMessageBuilder_ == null) {
if (oneofFieldCase_ == 112) {
return (tensorflow.test.Test.TestAllTypes.NestedMessage) oneofField_;
}
return tensorflow.test.Test.TestAllTypes.NestedMessage.getDefaultInstance();
} else {
if (oneofFieldCase_ == 112) {
return oneofNestedMessageBuilder_.getMessage();
}
return tensorflow.test.Test.TestAllTypes.NestedMessage.getDefaultInstance();
}
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage oneof_nested_message = 112;
*/
public Builder setOneofNestedMessage(tensorflow.test.Test.TestAllTypes.NestedMessage value) {
if (oneofNestedMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
oneofField_ = value;
onChanged();
} else {
oneofNestedMessageBuilder_.setMessage(value);
}
oneofFieldCase_ = 112;
return this;
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage oneof_nested_message = 112;
*/
public Builder setOneofNestedMessage(
tensorflow.test.Test.TestAllTypes.NestedMessage.Builder builderForValue) {
if (oneofNestedMessageBuilder_ == null) {
oneofField_ = builderForValue.build();
onChanged();
} else {
oneofNestedMessageBuilder_.setMessage(builderForValue.build());
}
oneofFieldCase_ = 112;
return this;
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage oneof_nested_message = 112;
*/
public Builder mergeOneofNestedMessage(tensorflow.test.Test.TestAllTypes.NestedMessage value) {
if (oneofNestedMessageBuilder_ == null) {
if (oneofFieldCase_ == 112 &&
oneofField_ != tensorflow.test.Test.TestAllTypes.NestedMessage.getDefaultInstance()) {
oneofField_ = tensorflow.test.Test.TestAllTypes.NestedMessage.newBuilder((tensorflow.test.Test.TestAllTypes.NestedMessage) oneofField_)
.mergeFrom(value).buildPartial();
} else {
oneofField_ = value;
}
onChanged();
} else {
if (oneofFieldCase_ == 112) {
oneofNestedMessageBuilder_.mergeFrom(value);
}
oneofNestedMessageBuilder_.setMessage(value);
}
oneofFieldCase_ = 112;
return this;
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage oneof_nested_message = 112;
*/
public Builder clearOneofNestedMessage() {
if (oneofNestedMessageBuilder_ == null) {
if (oneofFieldCase_ == 112) {
oneofFieldCase_ = 0;
oneofField_ = null;
onChanged();
}
} else {
if (oneofFieldCase_ == 112) {
oneofFieldCase_ = 0;
oneofField_ = null;
}
oneofNestedMessageBuilder_.clear();
}
return this;
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage oneof_nested_message = 112;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage.Builder getOneofNestedMessageBuilder() {
return getOneofNestedMessageFieldBuilder().getBuilder();
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage oneof_nested_message = 112;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessageOrBuilder getOneofNestedMessageOrBuilder() {
if ((oneofFieldCase_ == 112) && (oneofNestedMessageBuilder_ != null)) {
return oneofNestedMessageBuilder_.getMessageOrBuilder();
} else {
if (oneofFieldCase_ == 112) {
return (tensorflow.test.Test.TestAllTypes.NestedMessage) oneofField_;
}
return tensorflow.test.Test.TestAllTypes.NestedMessage.getDefaultInstance();
}
}
/**
* .tensorflow.test.TestAllTypes.NestedMessage oneof_nested_message = 112;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.test.Test.TestAllTypes.NestedMessage, tensorflow.test.Test.TestAllTypes.NestedMessage.Builder, tensorflow.test.Test.TestAllTypes.NestedMessageOrBuilder>
getOneofNestedMessageFieldBuilder() {
if (oneofNestedMessageBuilder_ == null) {
if (!(oneofFieldCase_ == 112)) {
oneofField_ = tensorflow.test.Test.TestAllTypes.NestedMessage.getDefaultInstance();
}
oneofNestedMessageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.test.Test.TestAllTypes.NestedMessage, tensorflow.test.Test.TestAllTypes.NestedMessage.Builder, tensorflow.test.Test.TestAllTypes.NestedMessageOrBuilder>(
(tensorflow.test.Test.TestAllTypes.NestedMessage) oneofField_,
getParentForChildren(),
isClean());
oneofField_ = null;
}
oneofFieldCase_ = 112;
onChanged();;
return oneofNestedMessageBuilder_;
}
/**
* string oneof_string = 113;
*/
public java.lang.String getOneofString() {
java.lang.Object ref = "";
if (oneofFieldCase_ == 113) {
ref = oneofField_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (oneofFieldCase_ == 113) {
oneofField_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string oneof_string = 113;
*/
public com.google.protobuf.ByteString
getOneofStringBytes() {
java.lang.Object ref = "";
if (oneofFieldCase_ == 113) {
ref = oneofField_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (oneofFieldCase_ == 113) {
oneofField_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string oneof_string = 113;
*/
public Builder setOneofString(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
oneofFieldCase_ = 113;
oneofField_ = value;
onChanged();
return this;
}
/**
* string oneof_string = 113;
*/
public Builder clearOneofString() {
if (oneofFieldCase_ == 113) {
oneofFieldCase_ = 0;
oneofField_ = null;
onChanged();
}
return this;
}
/**
* string oneof_string = 113;
*/
public Builder setOneofStringBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
oneofFieldCase_ = 113;
oneofField_ = value;
onChanged();
return this;
}
/**
* bytes oneof_bytes = 114;
*/
public com.google.protobuf.ByteString getOneofBytes() {
if (oneofFieldCase_ == 114) {
return (com.google.protobuf.ByteString) oneofField_;
}
return com.google.protobuf.ByteString.EMPTY;
}
/**
* bytes oneof_bytes = 114;
*/
public Builder setOneofBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
oneofFieldCase_ = 114;
oneofField_ = value;
onChanged();
return this;
}
/**
* bytes oneof_bytes = 114;
*/
public Builder clearOneofBytes() {
if (oneofFieldCase_ == 114) {
oneofFieldCase_ = 0;
oneofField_ = null;
onChanged();
}
return this;
}
/**
* .tensorflow.test.TestAllTypes.NestedEnum oneof_enum = 100;
*/
public int getOneofEnumValue() {
if (oneofFieldCase_ == 100) {
return ((java.lang.Integer) oneofField_).intValue();
}
return 0;
}
/**
* .tensorflow.test.TestAllTypes.NestedEnum oneof_enum = 100;
*/
public Builder setOneofEnumValue(int value) {
oneofFieldCase_ = 100;
oneofField_ = value;
onChanged();
return this;
}
/**
* .tensorflow.test.TestAllTypes.NestedEnum oneof_enum = 100;
*/
public tensorflow.test.Test.TestAllTypes.NestedEnum getOneofEnum() {
if (oneofFieldCase_ == 100) {
@SuppressWarnings("deprecation")
tensorflow.test.Test.TestAllTypes.NestedEnum result = tensorflow.test.Test.TestAllTypes.NestedEnum.valueOf(
(java.lang.Integer) oneofField_);
return result == null ? tensorflow.test.Test.TestAllTypes.NestedEnum.UNRECOGNIZED : result;
}
return tensorflow.test.Test.TestAllTypes.NestedEnum.ZERO;
}
/**
* .tensorflow.test.TestAllTypes.NestedEnum oneof_enum = 100;
*/
public Builder setOneofEnum(tensorflow.test.Test.TestAllTypes.NestedEnum value) {
if (value == null) {
throw new NullPointerException();
}
oneofFieldCase_ = 100;
oneofField_ = value.getNumber();
onChanged();
return this;
}
/**
* .tensorflow.test.TestAllTypes.NestedEnum oneof_enum = 100;
*/
public Builder clearOneofEnum() {
if (oneofFieldCase_ == 100) {
oneofFieldCase_ = 0;
oneofField_ = null;
onChanged();
}
return this;
}
private com.google.protobuf.MapField<
java.lang.String, tensorflow.test.Test.TestAllTypes.NestedMessage> mapStringToMessage_;
private com.google.protobuf.MapField
internalGetMapStringToMessage() {
if (mapStringToMessage_ == null) {
return com.google.protobuf.MapField.emptyMapField(
MapStringToMessageDefaultEntryHolder.defaultEntry);
}
return mapStringToMessage_;
}
private com.google.protobuf.MapField
internalGetMutableMapStringToMessage() {
onChanged();;
if (mapStringToMessage_ == null) {
mapStringToMessage_ = com.google.protobuf.MapField.newMapField(
MapStringToMessageDefaultEntryHolder.defaultEntry);
}
if (!mapStringToMessage_.isMutable()) {
mapStringToMessage_ = mapStringToMessage_.copy();
}
return mapStringToMessage_;
}
public int getMapStringToMessageCount() {
return internalGetMapStringToMessage().getMap().size();
}
/**
* map<string, .tensorflow.test.TestAllTypes.NestedMessage> map_string_to_message = 58;
*/
public boolean containsMapStringToMessage(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetMapStringToMessage().getMap().containsKey(key);
}
/**
* Use {@link #getMapStringToMessageMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getMapStringToMessage() {
return getMapStringToMessageMap();
}
/**
* map<string, .tensorflow.test.TestAllTypes.NestedMessage> map_string_to_message = 58;
*/
public java.util.Map getMapStringToMessageMap() {
return internalGetMapStringToMessage().getMap();
}
/**
* map<string, .tensorflow.test.TestAllTypes.NestedMessage> map_string_to_message = 58;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage getMapStringToMessageOrDefault(
java.lang.String key,
tensorflow.test.Test.TestAllTypes.NestedMessage defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetMapStringToMessage().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, .tensorflow.test.TestAllTypes.NestedMessage> map_string_to_message = 58;
*/
public tensorflow.test.Test.TestAllTypes.NestedMessage getMapStringToMessageOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetMapStringToMessage().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearMapStringToMessage() {
internalGetMutableMapStringToMessage().getMutableMap()
.clear();
return this;
}
/**
* map<string, .tensorflow.test.TestAllTypes.NestedMessage> map_string_to_message = 58;
*/
public Builder removeMapStringToMessage(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableMapStringToMessage().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableMapStringToMessage() {
return internalGetMutableMapStringToMessage().getMutableMap();
}
/**
* map<string, .tensorflow.test.TestAllTypes.NestedMessage> map_string_to_message = 58;
*/
public Builder putMapStringToMessage(
java.lang.String key,
tensorflow.test.Test.TestAllTypes.NestedMessage value) {
if (key == null) { throw new java.lang.NullPointerException(); }
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutableMapStringToMessage().getMutableMap()
.put(key, value);
return this;
}
/**
* map<string, .tensorflow.test.TestAllTypes.NestedMessage> map_string_to_message = 58;
*/
public Builder putAllMapStringToMessage(
java.util.Map values) {
internalGetMutableMapStringToMessage().getMutableMap()
.putAll(values);
return this;
}
private com.google.protobuf.MapField<
java.lang.Integer, tensorflow.test.Test.TestAllTypes.NestedMessage> mapInt32ToMessage_;
private com.google.protobuf.MapField
internalGetMapInt32ToMessage() {
if (mapInt32ToMessage_ == null) {
return com.google.protobuf.MapField.emptyMapField(
MapInt32ToMessageDefaultEntryHolder.defaultEntry);
}
return mapInt32ToMessage_;
}
private com.google.protobuf.MapField
internalGetMutableMapInt32ToMessage() {
onChanged();;
if (mapInt32ToMessage_ == null) {
mapInt32ToMessage_ = com.google.protobuf.MapField.newMapField(
MapInt32ToMessageDefaultEntryHolder.defaultEntry);
}
if (!mapInt32ToMessage_.isMutable()) {
mapInt32ToMessage_ = mapInt32ToMessage_.copy();
}
return mapInt32ToMessage_;
}
public int getMapInt32ToMessageCount() {
return internalGetMapInt32ToMessage().getMap().size();
}
/**
*