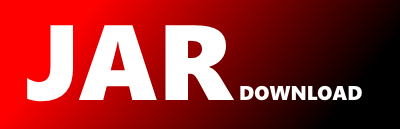
tensorflow.tfprof.TfprofOptions Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: tensorflow/core/profiler/tfprof_options.proto
package tensorflow.tfprof;
public final class TfprofOptions {
private TfprofOptions() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface OptionsProtoOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.tfprof.OptionsProto)
com.google.protobuf.MessageOrBuilder {
/**
* int64 max_depth = 1;
*/
long getMaxDepth();
/**
* int64 min_bytes = 2;
*/
long getMinBytes();
/**
* int64 min_peak_bytes = 19;
*/
long getMinPeakBytes();
/**
* int64 min_residual_bytes = 20;
*/
long getMinResidualBytes();
/**
* int64 min_output_bytes = 21;
*/
long getMinOutputBytes();
/**
* int64 min_micros = 3;
*/
long getMinMicros();
/**
* int64 min_accelerator_micros = 22;
*/
long getMinAcceleratorMicros();
/**
* int64 min_cpu_micros = 23;
*/
long getMinCpuMicros();
/**
* int64 min_params = 4;
*/
long getMinParams();
/**
* int64 min_float_ops = 5;
*/
long getMinFloatOps();
/**
* int64 min_occurrence = 17;
*/
long getMinOccurrence();
/**
* int64 step = 18;
*/
long getStep();
/**
* string order_by = 7;
*/
java.lang.String getOrderBy();
/**
* string order_by = 7;
*/
com.google.protobuf.ByteString
getOrderByBytes();
/**
* repeated string account_type_regexes = 8;
*/
java.util.List
getAccountTypeRegexesList();
/**
* repeated string account_type_regexes = 8;
*/
int getAccountTypeRegexesCount();
/**
* repeated string account_type_regexes = 8;
*/
java.lang.String getAccountTypeRegexes(int index);
/**
* repeated string account_type_regexes = 8;
*/
com.google.protobuf.ByteString
getAccountTypeRegexesBytes(int index);
/**
* repeated string start_name_regexes = 9;
*/
java.util.List
getStartNameRegexesList();
/**
* repeated string start_name_regexes = 9;
*/
int getStartNameRegexesCount();
/**
* repeated string start_name_regexes = 9;
*/
java.lang.String getStartNameRegexes(int index);
/**
* repeated string start_name_regexes = 9;
*/
com.google.protobuf.ByteString
getStartNameRegexesBytes(int index);
/**
* repeated string trim_name_regexes = 10;
*/
java.util.List
getTrimNameRegexesList();
/**
* repeated string trim_name_regexes = 10;
*/
int getTrimNameRegexesCount();
/**
* repeated string trim_name_regexes = 10;
*/
java.lang.String getTrimNameRegexes(int index);
/**
* repeated string trim_name_regexes = 10;
*/
com.google.protobuf.ByteString
getTrimNameRegexesBytes(int index);
/**
* repeated string show_name_regexes = 11;
*/
java.util.List
getShowNameRegexesList();
/**
* repeated string show_name_regexes = 11;
*/
int getShowNameRegexesCount();
/**
* repeated string show_name_regexes = 11;
*/
java.lang.String getShowNameRegexes(int index);
/**
* repeated string show_name_regexes = 11;
*/
com.google.protobuf.ByteString
getShowNameRegexesBytes(int index);
/**
* repeated string hide_name_regexes = 12;
*/
java.util.List
getHideNameRegexesList();
/**
* repeated string hide_name_regexes = 12;
*/
int getHideNameRegexesCount();
/**
* repeated string hide_name_regexes = 12;
*/
java.lang.String getHideNameRegexes(int index);
/**
* repeated string hide_name_regexes = 12;
*/
com.google.protobuf.ByteString
getHideNameRegexesBytes(int index);
/**
* bool account_displayed_op_only = 13;
*/
boolean getAccountDisplayedOpOnly();
/**
* repeated string select = 14;
*/
java.util.List
getSelectList();
/**
* repeated string select = 14;
*/
int getSelectCount();
/**
* repeated string select = 14;
*/
java.lang.String getSelect(int index);
/**
* repeated string select = 14;
*/
com.google.protobuf.ByteString
getSelectBytes(int index);
/**
* string output = 15;
*/
java.lang.String getOutput();
/**
* string output = 15;
*/
com.google.protobuf.ByteString
getOutputBytes();
/**
* string dump_to_file = 16;
*/
java.lang.String getDumpToFile();
/**
* string dump_to_file = 16;
*/
com.google.protobuf.ByteString
getDumpToFileBytes();
}
/**
*
* Refers to tfprof_options.h/cc for documentation.
* Only used to pass tfprof options from Python to C++.
*
*
* Protobuf type {@code tensorflow.tfprof.OptionsProto}
*/
public static final class OptionsProto extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.tfprof.OptionsProto)
OptionsProtoOrBuilder {
private static final long serialVersionUID = 0L;
// Use OptionsProto.newBuilder() to construct.
private OptionsProto(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private OptionsProto() {
maxDepth_ = 0L;
minBytes_ = 0L;
minPeakBytes_ = 0L;
minResidualBytes_ = 0L;
minOutputBytes_ = 0L;
minMicros_ = 0L;
minAcceleratorMicros_ = 0L;
minCpuMicros_ = 0L;
minParams_ = 0L;
minFloatOps_ = 0L;
minOccurrence_ = 0L;
step_ = 0L;
orderBy_ = "";
accountTypeRegexes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
startNameRegexes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
trimNameRegexes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
showNameRegexes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
hideNameRegexes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
accountDisplayedOpOnly_ = false;
select_ = com.google.protobuf.LazyStringArrayList.EMPTY;
output_ = "";
dumpToFile_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private OptionsProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
maxDepth_ = input.readInt64();
break;
}
case 16: {
minBytes_ = input.readInt64();
break;
}
case 24: {
minMicros_ = input.readInt64();
break;
}
case 32: {
minParams_ = input.readInt64();
break;
}
case 40: {
minFloatOps_ = input.readInt64();
break;
}
case 58: {
java.lang.String s = input.readStringRequireUtf8();
orderBy_ = s;
break;
}
case 66: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00002000) == 0x00002000)) {
accountTypeRegexes_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00002000;
}
accountTypeRegexes_.add(s);
break;
}
case 74: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00004000) == 0x00004000)) {
startNameRegexes_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00004000;
}
startNameRegexes_.add(s);
break;
}
case 82: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00008000) == 0x00008000)) {
trimNameRegexes_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00008000;
}
trimNameRegexes_.add(s);
break;
}
case 90: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00010000) == 0x00010000)) {
showNameRegexes_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00010000;
}
showNameRegexes_.add(s);
break;
}
case 98: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00020000) == 0x00020000)) {
hideNameRegexes_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00020000;
}
hideNameRegexes_.add(s);
break;
}
case 104: {
accountDisplayedOpOnly_ = input.readBool();
break;
}
case 114: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00080000) == 0x00080000)) {
select_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00080000;
}
select_.add(s);
break;
}
case 122: {
java.lang.String s = input.readStringRequireUtf8();
output_ = s;
break;
}
case 130: {
java.lang.String s = input.readStringRequireUtf8();
dumpToFile_ = s;
break;
}
case 136: {
minOccurrence_ = input.readInt64();
break;
}
case 144: {
step_ = input.readInt64();
break;
}
case 152: {
minPeakBytes_ = input.readInt64();
break;
}
case 160: {
minResidualBytes_ = input.readInt64();
break;
}
case 168: {
minOutputBytes_ = input.readInt64();
break;
}
case 176: {
minAcceleratorMicros_ = input.readInt64();
break;
}
case 184: {
minCpuMicros_ = input.readInt64();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00002000) == 0x00002000)) {
accountTypeRegexes_ = accountTypeRegexes_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00004000) == 0x00004000)) {
startNameRegexes_ = startNameRegexes_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00008000) == 0x00008000)) {
trimNameRegexes_ = trimNameRegexes_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00010000) == 0x00010000)) {
showNameRegexes_ = showNameRegexes_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00020000) == 0x00020000)) {
hideNameRegexes_ = hideNameRegexes_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00080000) == 0x00080000)) {
select_ = select_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.tfprof.TfprofOptions.internal_static_tensorflow_tfprof_OptionsProto_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.tfprof.TfprofOptions.internal_static_tensorflow_tfprof_OptionsProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.tfprof.TfprofOptions.OptionsProto.class, tensorflow.tfprof.TfprofOptions.OptionsProto.Builder.class);
}
private int bitField0_;
public static final int MAX_DEPTH_FIELD_NUMBER = 1;
private long maxDepth_;
/**
* int64 max_depth = 1;
*/
public long getMaxDepth() {
return maxDepth_;
}
public static final int MIN_BYTES_FIELD_NUMBER = 2;
private long minBytes_;
/**
* int64 min_bytes = 2;
*/
public long getMinBytes() {
return minBytes_;
}
public static final int MIN_PEAK_BYTES_FIELD_NUMBER = 19;
private long minPeakBytes_;
/**
* int64 min_peak_bytes = 19;
*/
public long getMinPeakBytes() {
return minPeakBytes_;
}
public static final int MIN_RESIDUAL_BYTES_FIELD_NUMBER = 20;
private long minResidualBytes_;
/**
* int64 min_residual_bytes = 20;
*/
public long getMinResidualBytes() {
return minResidualBytes_;
}
public static final int MIN_OUTPUT_BYTES_FIELD_NUMBER = 21;
private long minOutputBytes_;
/**
* int64 min_output_bytes = 21;
*/
public long getMinOutputBytes() {
return minOutputBytes_;
}
public static final int MIN_MICROS_FIELD_NUMBER = 3;
private long minMicros_;
/**
* int64 min_micros = 3;
*/
public long getMinMicros() {
return minMicros_;
}
public static final int MIN_ACCELERATOR_MICROS_FIELD_NUMBER = 22;
private long minAcceleratorMicros_;
/**
* int64 min_accelerator_micros = 22;
*/
public long getMinAcceleratorMicros() {
return minAcceleratorMicros_;
}
public static final int MIN_CPU_MICROS_FIELD_NUMBER = 23;
private long minCpuMicros_;
/**
* int64 min_cpu_micros = 23;
*/
public long getMinCpuMicros() {
return minCpuMicros_;
}
public static final int MIN_PARAMS_FIELD_NUMBER = 4;
private long minParams_;
/**
* int64 min_params = 4;
*/
public long getMinParams() {
return minParams_;
}
public static final int MIN_FLOAT_OPS_FIELD_NUMBER = 5;
private long minFloatOps_;
/**
* int64 min_float_ops = 5;
*/
public long getMinFloatOps() {
return minFloatOps_;
}
public static final int MIN_OCCURRENCE_FIELD_NUMBER = 17;
private long minOccurrence_;
/**
* int64 min_occurrence = 17;
*/
public long getMinOccurrence() {
return minOccurrence_;
}
public static final int STEP_FIELD_NUMBER = 18;
private long step_;
/**
* int64 step = 18;
*/
public long getStep() {
return step_;
}
public static final int ORDER_BY_FIELD_NUMBER = 7;
private volatile java.lang.Object orderBy_;
/**
* string order_by = 7;
*/
public java.lang.String getOrderBy() {
java.lang.Object ref = orderBy_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
orderBy_ = s;
return s;
}
}
/**
* string order_by = 7;
*/
public com.google.protobuf.ByteString
getOrderByBytes() {
java.lang.Object ref = orderBy_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
orderBy_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ACCOUNT_TYPE_REGEXES_FIELD_NUMBER = 8;
private com.google.protobuf.LazyStringList accountTypeRegexes_;
/**
* repeated string account_type_regexes = 8;
*/
public com.google.protobuf.ProtocolStringList
getAccountTypeRegexesList() {
return accountTypeRegexes_;
}
/**
* repeated string account_type_regexes = 8;
*/
public int getAccountTypeRegexesCount() {
return accountTypeRegexes_.size();
}
/**
* repeated string account_type_regexes = 8;
*/
public java.lang.String getAccountTypeRegexes(int index) {
return accountTypeRegexes_.get(index);
}
/**
* repeated string account_type_regexes = 8;
*/
public com.google.protobuf.ByteString
getAccountTypeRegexesBytes(int index) {
return accountTypeRegexes_.getByteString(index);
}
public static final int START_NAME_REGEXES_FIELD_NUMBER = 9;
private com.google.protobuf.LazyStringList startNameRegexes_;
/**
* repeated string start_name_regexes = 9;
*/
public com.google.protobuf.ProtocolStringList
getStartNameRegexesList() {
return startNameRegexes_;
}
/**
* repeated string start_name_regexes = 9;
*/
public int getStartNameRegexesCount() {
return startNameRegexes_.size();
}
/**
* repeated string start_name_regexes = 9;
*/
public java.lang.String getStartNameRegexes(int index) {
return startNameRegexes_.get(index);
}
/**
* repeated string start_name_regexes = 9;
*/
public com.google.protobuf.ByteString
getStartNameRegexesBytes(int index) {
return startNameRegexes_.getByteString(index);
}
public static final int TRIM_NAME_REGEXES_FIELD_NUMBER = 10;
private com.google.protobuf.LazyStringList trimNameRegexes_;
/**
* repeated string trim_name_regexes = 10;
*/
public com.google.protobuf.ProtocolStringList
getTrimNameRegexesList() {
return trimNameRegexes_;
}
/**
* repeated string trim_name_regexes = 10;
*/
public int getTrimNameRegexesCount() {
return trimNameRegexes_.size();
}
/**
* repeated string trim_name_regexes = 10;
*/
public java.lang.String getTrimNameRegexes(int index) {
return trimNameRegexes_.get(index);
}
/**
* repeated string trim_name_regexes = 10;
*/
public com.google.protobuf.ByteString
getTrimNameRegexesBytes(int index) {
return trimNameRegexes_.getByteString(index);
}
public static final int SHOW_NAME_REGEXES_FIELD_NUMBER = 11;
private com.google.protobuf.LazyStringList showNameRegexes_;
/**
* repeated string show_name_regexes = 11;
*/
public com.google.protobuf.ProtocolStringList
getShowNameRegexesList() {
return showNameRegexes_;
}
/**
* repeated string show_name_regexes = 11;
*/
public int getShowNameRegexesCount() {
return showNameRegexes_.size();
}
/**
* repeated string show_name_regexes = 11;
*/
public java.lang.String getShowNameRegexes(int index) {
return showNameRegexes_.get(index);
}
/**
* repeated string show_name_regexes = 11;
*/
public com.google.protobuf.ByteString
getShowNameRegexesBytes(int index) {
return showNameRegexes_.getByteString(index);
}
public static final int HIDE_NAME_REGEXES_FIELD_NUMBER = 12;
private com.google.protobuf.LazyStringList hideNameRegexes_;
/**
* repeated string hide_name_regexes = 12;
*/
public com.google.protobuf.ProtocolStringList
getHideNameRegexesList() {
return hideNameRegexes_;
}
/**
* repeated string hide_name_regexes = 12;
*/
public int getHideNameRegexesCount() {
return hideNameRegexes_.size();
}
/**
* repeated string hide_name_regexes = 12;
*/
public java.lang.String getHideNameRegexes(int index) {
return hideNameRegexes_.get(index);
}
/**
* repeated string hide_name_regexes = 12;
*/
public com.google.protobuf.ByteString
getHideNameRegexesBytes(int index) {
return hideNameRegexes_.getByteString(index);
}
public static final int ACCOUNT_DISPLAYED_OP_ONLY_FIELD_NUMBER = 13;
private boolean accountDisplayedOpOnly_;
/**
* bool account_displayed_op_only = 13;
*/
public boolean getAccountDisplayedOpOnly() {
return accountDisplayedOpOnly_;
}
public static final int SELECT_FIELD_NUMBER = 14;
private com.google.protobuf.LazyStringList select_;
/**
* repeated string select = 14;
*/
public com.google.protobuf.ProtocolStringList
getSelectList() {
return select_;
}
/**
* repeated string select = 14;
*/
public int getSelectCount() {
return select_.size();
}
/**
* repeated string select = 14;
*/
public java.lang.String getSelect(int index) {
return select_.get(index);
}
/**
* repeated string select = 14;
*/
public com.google.protobuf.ByteString
getSelectBytes(int index) {
return select_.getByteString(index);
}
public static final int OUTPUT_FIELD_NUMBER = 15;
private volatile java.lang.Object output_;
/**
* string output = 15;
*/
public java.lang.String getOutput() {
java.lang.Object ref = output_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
output_ = s;
return s;
}
}
/**
* string output = 15;
*/
public com.google.protobuf.ByteString
getOutputBytes() {
java.lang.Object ref = output_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
output_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DUMP_TO_FILE_FIELD_NUMBER = 16;
private volatile java.lang.Object dumpToFile_;
/**
* string dump_to_file = 16;
*/
public java.lang.String getDumpToFile() {
java.lang.Object ref = dumpToFile_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
dumpToFile_ = s;
return s;
}
}
/**
* string dump_to_file = 16;
*/
public com.google.protobuf.ByteString
getDumpToFileBytes() {
java.lang.Object ref = dumpToFile_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
dumpToFile_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (maxDepth_ != 0L) {
output.writeInt64(1, maxDepth_);
}
if (minBytes_ != 0L) {
output.writeInt64(2, minBytes_);
}
if (minMicros_ != 0L) {
output.writeInt64(3, minMicros_);
}
if (minParams_ != 0L) {
output.writeInt64(4, minParams_);
}
if (minFloatOps_ != 0L) {
output.writeInt64(5, minFloatOps_);
}
if (!getOrderByBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, orderBy_);
}
for (int i = 0; i < accountTypeRegexes_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, accountTypeRegexes_.getRaw(i));
}
for (int i = 0; i < startNameRegexes_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, startNameRegexes_.getRaw(i));
}
for (int i = 0; i < trimNameRegexes_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, trimNameRegexes_.getRaw(i));
}
for (int i = 0; i < showNameRegexes_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 11, showNameRegexes_.getRaw(i));
}
for (int i = 0; i < hideNameRegexes_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 12, hideNameRegexes_.getRaw(i));
}
if (accountDisplayedOpOnly_ != false) {
output.writeBool(13, accountDisplayedOpOnly_);
}
for (int i = 0; i < select_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 14, select_.getRaw(i));
}
if (!getOutputBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 15, output_);
}
if (!getDumpToFileBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 16, dumpToFile_);
}
if (minOccurrence_ != 0L) {
output.writeInt64(17, minOccurrence_);
}
if (step_ != 0L) {
output.writeInt64(18, step_);
}
if (minPeakBytes_ != 0L) {
output.writeInt64(19, minPeakBytes_);
}
if (minResidualBytes_ != 0L) {
output.writeInt64(20, minResidualBytes_);
}
if (minOutputBytes_ != 0L) {
output.writeInt64(21, minOutputBytes_);
}
if (minAcceleratorMicros_ != 0L) {
output.writeInt64(22, minAcceleratorMicros_);
}
if (minCpuMicros_ != 0L) {
output.writeInt64(23, minCpuMicros_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (maxDepth_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, maxDepth_);
}
if (minBytes_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, minBytes_);
}
if (minMicros_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, minMicros_);
}
if (minParams_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, minParams_);
}
if (minFloatOps_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(5, minFloatOps_);
}
if (!getOrderByBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, orderBy_);
}
{
int dataSize = 0;
for (int i = 0; i < accountTypeRegexes_.size(); i++) {
dataSize += computeStringSizeNoTag(accountTypeRegexes_.getRaw(i));
}
size += dataSize;
size += 1 * getAccountTypeRegexesList().size();
}
{
int dataSize = 0;
for (int i = 0; i < startNameRegexes_.size(); i++) {
dataSize += computeStringSizeNoTag(startNameRegexes_.getRaw(i));
}
size += dataSize;
size += 1 * getStartNameRegexesList().size();
}
{
int dataSize = 0;
for (int i = 0; i < trimNameRegexes_.size(); i++) {
dataSize += computeStringSizeNoTag(trimNameRegexes_.getRaw(i));
}
size += dataSize;
size += 1 * getTrimNameRegexesList().size();
}
{
int dataSize = 0;
for (int i = 0; i < showNameRegexes_.size(); i++) {
dataSize += computeStringSizeNoTag(showNameRegexes_.getRaw(i));
}
size += dataSize;
size += 1 * getShowNameRegexesList().size();
}
{
int dataSize = 0;
for (int i = 0; i < hideNameRegexes_.size(); i++) {
dataSize += computeStringSizeNoTag(hideNameRegexes_.getRaw(i));
}
size += dataSize;
size += 1 * getHideNameRegexesList().size();
}
if (accountDisplayedOpOnly_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(13, accountDisplayedOpOnly_);
}
{
int dataSize = 0;
for (int i = 0; i < select_.size(); i++) {
dataSize += computeStringSizeNoTag(select_.getRaw(i));
}
size += dataSize;
size += 1 * getSelectList().size();
}
if (!getOutputBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(15, output_);
}
if (!getDumpToFileBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(16, dumpToFile_);
}
if (minOccurrence_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(17, minOccurrence_);
}
if (step_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(18, step_);
}
if (minPeakBytes_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(19, minPeakBytes_);
}
if (minResidualBytes_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(20, minResidualBytes_);
}
if (minOutputBytes_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(21, minOutputBytes_);
}
if (minAcceleratorMicros_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(22, minAcceleratorMicros_);
}
if (minCpuMicros_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(23, minCpuMicros_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.tfprof.TfprofOptions.OptionsProto)) {
return super.equals(obj);
}
tensorflow.tfprof.TfprofOptions.OptionsProto other = (tensorflow.tfprof.TfprofOptions.OptionsProto) obj;
boolean result = true;
result = result && (getMaxDepth()
== other.getMaxDepth());
result = result && (getMinBytes()
== other.getMinBytes());
result = result && (getMinPeakBytes()
== other.getMinPeakBytes());
result = result && (getMinResidualBytes()
== other.getMinResidualBytes());
result = result && (getMinOutputBytes()
== other.getMinOutputBytes());
result = result && (getMinMicros()
== other.getMinMicros());
result = result && (getMinAcceleratorMicros()
== other.getMinAcceleratorMicros());
result = result && (getMinCpuMicros()
== other.getMinCpuMicros());
result = result && (getMinParams()
== other.getMinParams());
result = result && (getMinFloatOps()
== other.getMinFloatOps());
result = result && (getMinOccurrence()
== other.getMinOccurrence());
result = result && (getStep()
== other.getStep());
result = result && getOrderBy()
.equals(other.getOrderBy());
result = result && getAccountTypeRegexesList()
.equals(other.getAccountTypeRegexesList());
result = result && getStartNameRegexesList()
.equals(other.getStartNameRegexesList());
result = result && getTrimNameRegexesList()
.equals(other.getTrimNameRegexesList());
result = result && getShowNameRegexesList()
.equals(other.getShowNameRegexesList());
result = result && getHideNameRegexesList()
.equals(other.getHideNameRegexesList());
result = result && (getAccountDisplayedOpOnly()
== other.getAccountDisplayedOpOnly());
result = result && getSelectList()
.equals(other.getSelectList());
result = result && getOutput()
.equals(other.getOutput());
result = result && getDumpToFile()
.equals(other.getDumpToFile());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + MAX_DEPTH_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMaxDepth());
hash = (37 * hash) + MIN_BYTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMinBytes());
hash = (37 * hash) + MIN_PEAK_BYTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMinPeakBytes());
hash = (37 * hash) + MIN_RESIDUAL_BYTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMinResidualBytes());
hash = (37 * hash) + MIN_OUTPUT_BYTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMinOutputBytes());
hash = (37 * hash) + MIN_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMinMicros());
hash = (37 * hash) + MIN_ACCELERATOR_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMinAcceleratorMicros());
hash = (37 * hash) + MIN_CPU_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMinCpuMicros());
hash = (37 * hash) + MIN_PARAMS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMinParams());
hash = (37 * hash) + MIN_FLOAT_OPS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMinFloatOps());
hash = (37 * hash) + MIN_OCCURRENCE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMinOccurrence());
hash = (37 * hash) + STEP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getStep());
hash = (37 * hash) + ORDER_BY_FIELD_NUMBER;
hash = (53 * hash) + getOrderBy().hashCode();
if (getAccountTypeRegexesCount() > 0) {
hash = (37 * hash) + ACCOUNT_TYPE_REGEXES_FIELD_NUMBER;
hash = (53 * hash) + getAccountTypeRegexesList().hashCode();
}
if (getStartNameRegexesCount() > 0) {
hash = (37 * hash) + START_NAME_REGEXES_FIELD_NUMBER;
hash = (53 * hash) + getStartNameRegexesList().hashCode();
}
if (getTrimNameRegexesCount() > 0) {
hash = (37 * hash) + TRIM_NAME_REGEXES_FIELD_NUMBER;
hash = (53 * hash) + getTrimNameRegexesList().hashCode();
}
if (getShowNameRegexesCount() > 0) {
hash = (37 * hash) + SHOW_NAME_REGEXES_FIELD_NUMBER;
hash = (53 * hash) + getShowNameRegexesList().hashCode();
}
if (getHideNameRegexesCount() > 0) {
hash = (37 * hash) + HIDE_NAME_REGEXES_FIELD_NUMBER;
hash = (53 * hash) + getHideNameRegexesList().hashCode();
}
hash = (37 * hash) + ACCOUNT_DISPLAYED_OP_ONLY_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getAccountDisplayedOpOnly());
if (getSelectCount() > 0) {
hash = (37 * hash) + SELECT_FIELD_NUMBER;
hash = (53 * hash) + getSelectList().hashCode();
}
hash = (37 * hash) + OUTPUT_FIELD_NUMBER;
hash = (53 * hash) + getOutput().hashCode();
hash = (37 * hash) + DUMP_TO_FILE_FIELD_NUMBER;
hash = (53 * hash) + getDumpToFile().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.tfprof.TfprofOptions.OptionsProto parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tfprof.TfprofOptions.OptionsProto parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tfprof.TfprofOptions.OptionsProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tfprof.TfprofOptions.OptionsProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tfprof.TfprofOptions.OptionsProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tfprof.TfprofOptions.OptionsProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tfprof.TfprofOptions.OptionsProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.tfprof.TfprofOptions.OptionsProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.tfprof.TfprofOptions.OptionsProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.tfprof.TfprofOptions.OptionsProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.tfprof.TfprofOptions.OptionsProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.tfprof.TfprofOptions.OptionsProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.tfprof.TfprofOptions.OptionsProto prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Refers to tfprof_options.h/cc for documentation.
* Only used to pass tfprof options from Python to C++.
*
*
* Protobuf type {@code tensorflow.tfprof.OptionsProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.tfprof.OptionsProto)
tensorflow.tfprof.TfprofOptions.OptionsProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.tfprof.TfprofOptions.internal_static_tensorflow_tfprof_OptionsProto_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.tfprof.TfprofOptions.internal_static_tensorflow_tfprof_OptionsProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.tfprof.TfprofOptions.OptionsProto.class, tensorflow.tfprof.TfprofOptions.OptionsProto.Builder.class);
}
// Construct using tensorflow.tfprof.TfprofOptions.OptionsProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
maxDepth_ = 0L;
minBytes_ = 0L;
minPeakBytes_ = 0L;
minResidualBytes_ = 0L;
minOutputBytes_ = 0L;
minMicros_ = 0L;
minAcceleratorMicros_ = 0L;
minCpuMicros_ = 0L;
minParams_ = 0L;
minFloatOps_ = 0L;
minOccurrence_ = 0L;
step_ = 0L;
orderBy_ = "";
accountTypeRegexes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00002000);
startNameRegexes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00004000);
trimNameRegexes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00008000);
showNameRegexes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00010000);
hideNameRegexes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00020000);
accountDisplayedOpOnly_ = false;
select_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00080000);
output_ = "";
dumpToFile_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.tfprof.TfprofOptions.internal_static_tensorflow_tfprof_OptionsProto_descriptor;
}
@java.lang.Override
public tensorflow.tfprof.TfprofOptions.OptionsProto getDefaultInstanceForType() {
return tensorflow.tfprof.TfprofOptions.OptionsProto.getDefaultInstance();
}
@java.lang.Override
public tensorflow.tfprof.TfprofOptions.OptionsProto build() {
tensorflow.tfprof.TfprofOptions.OptionsProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.tfprof.TfprofOptions.OptionsProto buildPartial() {
tensorflow.tfprof.TfprofOptions.OptionsProto result = new tensorflow.tfprof.TfprofOptions.OptionsProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.maxDepth_ = maxDepth_;
result.minBytes_ = minBytes_;
result.minPeakBytes_ = minPeakBytes_;
result.minResidualBytes_ = minResidualBytes_;
result.minOutputBytes_ = minOutputBytes_;
result.minMicros_ = minMicros_;
result.minAcceleratorMicros_ = minAcceleratorMicros_;
result.minCpuMicros_ = minCpuMicros_;
result.minParams_ = minParams_;
result.minFloatOps_ = minFloatOps_;
result.minOccurrence_ = minOccurrence_;
result.step_ = step_;
result.orderBy_ = orderBy_;
if (((bitField0_ & 0x00002000) == 0x00002000)) {
accountTypeRegexes_ = accountTypeRegexes_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00002000);
}
result.accountTypeRegexes_ = accountTypeRegexes_;
if (((bitField0_ & 0x00004000) == 0x00004000)) {
startNameRegexes_ = startNameRegexes_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00004000);
}
result.startNameRegexes_ = startNameRegexes_;
if (((bitField0_ & 0x00008000) == 0x00008000)) {
trimNameRegexes_ = trimNameRegexes_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00008000);
}
result.trimNameRegexes_ = trimNameRegexes_;
if (((bitField0_ & 0x00010000) == 0x00010000)) {
showNameRegexes_ = showNameRegexes_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00010000);
}
result.showNameRegexes_ = showNameRegexes_;
if (((bitField0_ & 0x00020000) == 0x00020000)) {
hideNameRegexes_ = hideNameRegexes_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00020000);
}
result.hideNameRegexes_ = hideNameRegexes_;
result.accountDisplayedOpOnly_ = accountDisplayedOpOnly_;
if (((bitField0_ & 0x00080000) == 0x00080000)) {
select_ = select_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00080000);
}
result.select_ = select_;
result.output_ = output_;
result.dumpToFile_ = dumpToFile_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.tfprof.TfprofOptions.OptionsProto) {
return mergeFrom((tensorflow.tfprof.TfprofOptions.OptionsProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.tfprof.TfprofOptions.OptionsProto other) {
if (other == tensorflow.tfprof.TfprofOptions.OptionsProto.getDefaultInstance()) return this;
if (other.getMaxDepth() != 0L) {
setMaxDepth(other.getMaxDepth());
}
if (other.getMinBytes() != 0L) {
setMinBytes(other.getMinBytes());
}
if (other.getMinPeakBytes() != 0L) {
setMinPeakBytes(other.getMinPeakBytes());
}
if (other.getMinResidualBytes() != 0L) {
setMinResidualBytes(other.getMinResidualBytes());
}
if (other.getMinOutputBytes() != 0L) {
setMinOutputBytes(other.getMinOutputBytes());
}
if (other.getMinMicros() != 0L) {
setMinMicros(other.getMinMicros());
}
if (other.getMinAcceleratorMicros() != 0L) {
setMinAcceleratorMicros(other.getMinAcceleratorMicros());
}
if (other.getMinCpuMicros() != 0L) {
setMinCpuMicros(other.getMinCpuMicros());
}
if (other.getMinParams() != 0L) {
setMinParams(other.getMinParams());
}
if (other.getMinFloatOps() != 0L) {
setMinFloatOps(other.getMinFloatOps());
}
if (other.getMinOccurrence() != 0L) {
setMinOccurrence(other.getMinOccurrence());
}
if (other.getStep() != 0L) {
setStep(other.getStep());
}
if (!other.getOrderBy().isEmpty()) {
orderBy_ = other.orderBy_;
onChanged();
}
if (!other.accountTypeRegexes_.isEmpty()) {
if (accountTypeRegexes_.isEmpty()) {
accountTypeRegexes_ = other.accountTypeRegexes_;
bitField0_ = (bitField0_ & ~0x00002000);
} else {
ensureAccountTypeRegexesIsMutable();
accountTypeRegexes_.addAll(other.accountTypeRegexes_);
}
onChanged();
}
if (!other.startNameRegexes_.isEmpty()) {
if (startNameRegexes_.isEmpty()) {
startNameRegexes_ = other.startNameRegexes_;
bitField0_ = (bitField0_ & ~0x00004000);
} else {
ensureStartNameRegexesIsMutable();
startNameRegexes_.addAll(other.startNameRegexes_);
}
onChanged();
}
if (!other.trimNameRegexes_.isEmpty()) {
if (trimNameRegexes_.isEmpty()) {
trimNameRegexes_ = other.trimNameRegexes_;
bitField0_ = (bitField0_ & ~0x00008000);
} else {
ensureTrimNameRegexesIsMutable();
trimNameRegexes_.addAll(other.trimNameRegexes_);
}
onChanged();
}
if (!other.showNameRegexes_.isEmpty()) {
if (showNameRegexes_.isEmpty()) {
showNameRegexes_ = other.showNameRegexes_;
bitField0_ = (bitField0_ & ~0x00010000);
} else {
ensureShowNameRegexesIsMutable();
showNameRegexes_.addAll(other.showNameRegexes_);
}
onChanged();
}
if (!other.hideNameRegexes_.isEmpty()) {
if (hideNameRegexes_.isEmpty()) {
hideNameRegexes_ = other.hideNameRegexes_;
bitField0_ = (bitField0_ & ~0x00020000);
} else {
ensureHideNameRegexesIsMutable();
hideNameRegexes_.addAll(other.hideNameRegexes_);
}
onChanged();
}
if (other.getAccountDisplayedOpOnly() != false) {
setAccountDisplayedOpOnly(other.getAccountDisplayedOpOnly());
}
if (!other.select_.isEmpty()) {
if (select_.isEmpty()) {
select_ = other.select_;
bitField0_ = (bitField0_ & ~0x00080000);
} else {
ensureSelectIsMutable();
select_.addAll(other.select_);
}
onChanged();
}
if (!other.getOutput().isEmpty()) {
output_ = other.output_;
onChanged();
}
if (!other.getDumpToFile().isEmpty()) {
dumpToFile_ = other.dumpToFile_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.tfprof.TfprofOptions.OptionsProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.tfprof.TfprofOptions.OptionsProto) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long maxDepth_ ;
/**
* int64 max_depth = 1;
*/
public long getMaxDepth() {
return maxDepth_;
}
/**
* int64 max_depth = 1;
*/
public Builder setMaxDepth(long value) {
maxDepth_ = value;
onChanged();
return this;
}
/**
* int64 max_depth = 1;
*/
public Builder clearMaxDepth() {
maxDepth_ = 0L;
onChanged();
return this;
}
private long minBytes_ ;
/**
* int64 min_bytes = 2;
*/
public long getMinBytes() {
return minBytes_;
}
/**
* int64 min_bytes = 2;
*/
public Builder setMinBytes(long value) {
minBytes_ = value;
onChanged();
return this;
}
/**
* int64 min_bytes = 2;
*/
public Builder clearMinBytes() {
minBytes_ = 0L;
onChanged();
return this;
}
private long minPeakBytes_ ;
/**
* int64 min_peak_bytes = 19;
*/
public long getMinPeakBytes() {
return minPeakBytes_;
}
/**
* int64 min_peak_bytes = 19;
*/
public Builder setMinPeakBytes(long value) {
minPeakBytes_ = value;
onChanged();
return this;
}
/**
* int64 min_peak_bytes = 19;
*/
public Builder clearMinPeakBytes() {
minPeakBytes_ = 0L;
onChanged();
return this;
}
private long minResidualBytes_ ;
/**
* int64 min_residual_bytes = 20;
*/
public long getMinResidualBytes() {
return minResidualBytes_;
}
/**
* int64 min_residual_bytes = 20;
*/
public Builder setMinResidualBytes(long value) {
minResidualBytes_ = value;
onChanged();
return this;
}
/**
* int64 min_residual_bytes = 20;
*/
public Builder clearMinResidualBytes() {
minResidualBytes_ = 0L;
onChanged();
return this;
}
private long minOutputBytes_ ;
/**
* int64 min_output_bytes = 21;
*/
public long getMinOutputBytes() {
return minOutputBytes_;
}
/**
* int64 min_output_bytes = 21;
*/
public Builder setMinOutputBytes(long value) {
minOutputBytes_ = value;
onChanged();
return this;
}
/**
* int64 min_output_bytes = 21;
*/
public Builder clearMinOutputBytes() {
minOutputBytes_ = 0L;
onChanged();
return this;
}
private long minMicros_ ;
/**
* int64 min_micros = 3;
*/
public long getMinMicros() {
return minMicros_;
}
/**
* int64 min_micros = 3;
*/
public Builder setMinMicros(long value) {
minMicros_ = value;
onChanged();
return this;
}
/**
* int64 min_micros = 3;
*/
public Builder clearMinMicros() {
minMicros_ = 0L;
onChanged();
return this;
}
private long minAcceleratorMicros_ ;
/**
* int64 min_accelerator_micros = 22;
*/
public long getMinAcceleratorMicros() {
return minAcceleratorMicros_;
}
/**
* int64 min_accelerator_micros = 22;
*/
public Builder setMinAcceleratorMicros(long value) {
minAcceleratorMicros_ = value;
onChanged();
return this;
}
/**
* int64 min_accelerator_micros = 22;
*/
public Builder clearMinAcceleratorMicros() {
minAcceleratorMicros_ = 0L;
onChanged();
return this;
}
private long minCpuMicros_ ;
/**
* int64 min_cpu_micros = 23;
*/
public long getMinCpuMicros() {
return minCpuMicros_;
}
/**
* int64 min_cpu_micros = 23;
*/
public Builder setMinCpuMicros(long value) {
minCpuMicros_ = value;
onChanged();
return this;
}
/**
* int64 min_cpu_micros = 23;
*/
public Builder clearMinCpuMicros() {
minCpuMicros_ = 0L;
onChanged();
return this;
}
private long minParams_ ;
/**
* int64 min_params = 4;
*/
public long getMinParams() {
return minParams_;
}
/**
* int64 min_params = 4;
*/
public Builder setMinParams(long value) {
minParams_ = value;
onChanged();
return this;
}
/**
* int64 min_params = 4;
*/
public Builder clearMinParams() {
minParams_ = 0L;
onChanged();
return this;
}
private long minFloatOps_ ;
/**
* int64 min_float_ops = 5;
*/
public long getMinFloatOps() {
return minFloatOps_;
}
/**
* int64 min_float_ops = 5;
*/
public Builder setMinFloatOps(long value) {
minFloatOps_ = value;
onChanged();
return this;
}
/**
* int64 min_float_ops = 5;
*/
public Builder clearMinFloatOps() {
minFloatOps_ = 0L;
onChanged();
return this;
}
private long minOccurrence_ ;
/**
* int64 min_occurrence = 17;
*/
public long getMinOccurrence() {
return minOccurrence_;
}
/**
* int64 min_occurrence = 17;
*/
public Builder setMinOccurrence(long value) {
minOccurrence_ = value;
onChanged();
return this;
}
/**
* int64 min_occurrence = 17;
*/
public Builder clearMinOccurrence() {
minOccurrence_ = 0L;
onChanged();
return this;
}
private long step_ ;
/**
* int64 step = 18;
*/
public long getStep() {
return step_;
}
/**
* int64 step = 18;
*/
public Builder setStep(long value) {
step_ = value;
onChanged();
return this;
}
/**
* int64 step = 18;
*/
public Builder clearStep() {
step_ = 0L;
onChanged();
return this;
}
private java.lang.Object orderBy_ = "";
/**
* string order_by = 7;
*/
public java.lang.String getOrderBy() {
java.lang.Object ref = orderBy_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
orderBy_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string order_by = 7;
*/
public com.google.protobuf.ByteString
getOrderByBytes() {
java.lang.Object ref = orderBy_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
orderBy_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string order_by = 7;
*/
public Builder setOrderBy(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
orderBy_ = value;
onChanged();
return this;
}
/**
* string order_by = 7;
*/
public Builder clearOrderBy() {
orderBy_ = getDefaultInstance().getOrderBy();
onChanged();
return this;
}
/**
* string order_by = 7;
*/
public Builder setOrderByBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
orderBy_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList accountTypeRegexes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureAccountTypeRegexesIsMutable() {
if (!((bitField0_ & 0x00002000) == 0x00002000)) {
accountTypeRegexes_ = new com.google.protobuf.LazyStringArrayList(accountTypeRegexes_);
bitField0_ |= 0x00002000;
}
}
/**
* repeated string account_type_regexes = 8;
*/
public com.google.protobuf.ProtocolStringList
getAccountTypeRegexesList() {
return accountTypeRegexes_.getUnmodifiableView();
}
/**
* repeated string account_type_regexes = 8;
*/
public int getAccountTypeRegexesCount() {
return accountTypeRegexes_.size();
}
/**
* repeated string account_type_regexes = 8;
*/
public java.lang.String getAccountTypeRegexes(int index) {
return accountTypeRegexes_.get(index);
}
/**
* repeated string account_type_regexes = 8;
*/
public com.google.protobuf.ByteString
getAccountTypeRegexesBytes(int index) {
return accountTypeRegexes_.getByteString(index);
}
/**
* repeated string account_type_regexes = 8;
*/
public Builder setAccountTypeRegexes(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureAccountTypeRegexesIsMutable();
accountTypeRegexes_.set(index, value);
onChanged();
return this;
}
/**
* repeated string account_type_regexes = 8;
*/
public Builder addAccountTypeRegexes(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureAccountTypeRegexesIsMutable();
accountTypeRegexes_.add(value);
onChanged();
return this;
}
/**
* repeated string account_type_regexes = 8;
*/
public Builder addAllAccountTypeRegexes(
java.lang.Iterable values) {
ensureAccountTypeRegexesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, accountTypeRegexes_);
onChanged();
return this;
}
/**
* repeated string account_type_regexes = 8;
*/
public Builder clearAccountTypeRegexes() {
accountTypeRegexes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00002000);
onChanged();
return this;
}
/**
* repeated string account_type_regexes = 8;
*/
public Builder addAccountTypeRegexesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureAccountTypeRegexesIsMutable();
accountTypeRegexes_.add(value);
onChanged();
return this;
}
private com.google.protobuf.LazyStringList startNameRegexes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureStartNameRegexesIsMutable() {
if (!((bitField0_ & 0x00004000) == 0x00004000)) {
startNameRegexes_ = new com.google.protobuf.LazyStringArrayList(startNameRegexes_);
bitField0_ |= 0x00004000;
}
}
/**
* repeated string start_name_regexes = 9;
*/
public com.google.protobuf.ProtocolStringList
getStartNameRegexesList() {
return startNameRegexes_.getUnmodifiableView();
}
/**
* repeated string start_name_regexes = 9;
*/
public int getStartNameRegexesCount() {
return startNameRegexes_.size();
}
/**
* repeated string start_name_regexes = 9;
*/
public java.lang.String getStartNameRegexes(int index) {
return startNameRegexes_.get(index);
}
/**
* repeated string start_name_regexes = 9;
*/
public com.google.protobuf.ByteString
getStartNameRegexesBytes(int index) {
return startNameRegexes_.getByteString(index);
}
/**
* repeated string start_name_regexes = 9;
*/
public Builder setStartNameRegexes(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureStartNameRegexesIsMutable();
startNameRegexes_.set(index, value);
onChanged();
return this;
}
/**
* repeated string start_name_regexes = 9;
*/
public Builder addStartNameRegexes(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureStartNameRegexesIsMutable();
startNameRegexes_.add(value);
onChanged();
return this;
}
/**
* repeated string start_name_regexes = 9;
*/
public Builder addAllStartNameRegexes(
java.lang.Iterable values) {
ensureStartNameRegexesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, startNameRegexes_);
onChanged();
return this;
}
/**
* repeated string start_name_regexes = 9;
*/
public Builder clearStartNameRegexes() {
startNameRegexes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00004000);
onChanged();
return this;
}
/**
* repeated string start_name_regexes = 9;
*/
public Builder addStartNameRegexesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureStartNameRegexesIsMutable();
startNameRegexes_.add(value);
onChanged();
return this;
}
private com.google.protobuf.LazyStringList trimNameRegexes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureTrimNameRegexesIsMutable() {
if (!((bitField0_ & 0x00008000) == 0x00008000)) {
trimNameRegexes_ = new com.google.protobuf.LazyStringArrayList(trimNameRegexes_);
bitField0_ |= 0x00008000;
}
}
/**
* repeated string trim_name_regexes = 10;
*/
public com.google.protobuf.ProtocolStringList
getTrimNameRegexesList() {
return trimNameRegexes_.getUnmodifiableView();
}
/**
* repeated string trim_name_regexes = 10;
*/
public int getTrimNameRegexesCount() {
return trimNameRegexes_.size();
}
/**
* repeated string trim_name_regexes = 10;
*/
public java.lang.String getTrimNameRegexes(int index) {
return trimNameRegexes_.get(index);
}
/**
* repeated string trim_name_regexes = 10;
*/
public com.google.protobuf.ByteString
getTrimNameRegexesBytes(int index) {
return trimNameRegexes_.getByteString(index);
}
/**
* repeated string trim_name_regexes = 10;
*/
public Builder setTrimNameRegexes(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureTrimNameRegexesIsMutable();
trimNameRegexes_.set(index, value);
onChanged();
return this;
}
/**
* repeated string trim_name_regexes = 10;
*/
public Builder addTrimNameRegexes(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureTrimNameRegexesIsMutable();
trimNameRegexes_.add(value);
onChanged();
return this;
}
/**
* repeated string trim_name_regexes = 10;
*/
public Builder addAllTrimNameRegexes(
java.lang.Iterable values) {
ensureTrimNameRegexesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, trimNameRegexes_);
onChanged();
return this;
}
/**
* repeated string trim_name_regexes = 10;
*/
public Builder clearTrimNameRegexes() {
trimNameRegexes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00008000);
onChanged();
return this;
}
/**
* repeated string trim_name_regexes = 10;
*/
public Builder addTrimNameRegexesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureTrimNameRegexesIsMutable();
trimNameRegexes_.add(value);
onChanged();
return this;
}
private com.google.protobuf.LazyStringList showNameRegexes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureShowNameRegexesIsMutable() {
if (!((bitField0_ & 0x00010000) == 0x00010000)) {
showNameRegexes_ = new com.google.protobuf.LazyStringArrayList(showNameRegexes_);
bitField0_ |= 0x00010000;
}
}
/**
* repeated string show_name_regexes = 11;
*/
public com.google.protobuf.ProtocolStringList
getShowNameRegexesList() {
return showNameRegexes_.getUnmodifiableView();
}
/**
* repeated string show_name_regexes = 11;
*/
public int getShowNameRegexesCount() {
return showNameRegexes_.size();
}
/**
* repeated string show_name_regexes = 11;
*/
public java.lang.String getShowNameRegexes(int index) {
return showNameRegexes_.get(index);
}
/**
* repeated string show_name_regexes = 11;
*/
public com.google.protobuf.ByteString
getShowNameRegexesBytes(int index) {
return showNameRegexes_.getByteString(index);
}
/**
* repeated string show_name_regexes = 11;
*/
public Builder setShowNameRegexes(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureShowNameRegexesIsMutable();
showNameRegexes_.set(index, value);
onChanged();
return this;
}
/**
* repeated string show_name_regexes = 11;
*/
public Builder addShowNameRegexes(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureShowNameRegexesIsMutable();
showNameRegexes_.add(value);
onChanged();
return this;
}
/**
* repeated string show_name_regexes = 11;
*/
public Builder addAllShowNameRegexes(
java.lang.Iterable values) {
ensureShowNameRegexesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, showNameRegexes_);
onChanged();
return this;
}
/**
* repeated string show_name_regexes = 11;
*/
public Builder clearShowNameRegexes() {
showNameRegexes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00010000);
onChanged();
return this;
}
/**
* repeated string show_name_regexes = 11;
*/
public Builder addShowNameRegexesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureShowNameRegexesIsMutable();
showNameRegexes_.add(value);
onChanged();
return this;
}
private com.google.protobuf.LazyStringList hideNameRegexes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureHideNameRegexesIsMutable() {
if (!((bitField0_ & 0x00020000) == 0x00020000)) {
hideNameRegexes_ = new com.google.protobuf.LazyStringArrayList(hideNameRegexes_);
bitField0_ |= 0x00020000;
}
}
/**
* repeated string hide_name_regexes = 12;
*/
public com.google.protobuf.ProtocolStringList
getHideNameRegexesList() {
return hideNameRegexes_.getUnmodifiableView();
}
/**
* repeated string hide_name_regexes = 12;
*/
public int getHideNameRegexesCount() {
return hideNameRegexes_.size();
}
/**
* repeated string hide_name_regexes = 12;
*/
public java.lang.String getHideNameRegexes(int index) {
return hideNameRegexes_.get(index);
}
/**
* repeated string hide_name_regexes = 12;
*/
public com.google.protobuf.ByteString
getHideNameRegexesBytes(int index) {
return hideNameRegexes_.getByteString(index);
}
/**
* repeated string hide_name_regexes = 12;
*/
public Builder setHideNameRegexes(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureHideNameRegexesIsMutable();
hideNameRegexes_.set(index, value);
onChanged();
return this;
}
/**
* repeated string hide_name_regexes = 12;
*/
public Builder addHideNameRegexes(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureHideNameRegexesIsMutable();
hideNameRegexes_.add(value);
onChanged();
return this;
}
/**
* repeated string hide_name_regexes = 12;
*/
public Builder addAllHideNameRegexes(
java.lang.Iterable values) {
ensureHideNameRegexesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, hideNameRegexes_);
onChanged();
return this;
}
/**
* repeated string hide_name_regexes = 12;
*/
public Builder clearHideNameRegexes() {
hideNameRegexes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00020000);
onChanged();
return this;
}
/**
* repeated string hide_name_regexes = 12;
*/
public Builder addHideNameRegexesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureHideNameRegexesIsMutable();
hideNameRegexes_.add(value);
onChanged();
return this;
}
private boolean accountDisplayedOpOnly_ ;
/**
* bool account_displayed_op_only = 13;
*/
public boolean getAccountDisplayedOpOnly() {
return accountDisplayedOpOnly_;
}
/**
* bool account_displayed_op_only = 13;
*/
public Builder setAccountDisplayedOpOnly(boolean value) {
accountDisplayedOpOnly_ = value;
onChanged();
return this;
}
/**
* bool account_displayed_op_only = 13;
*/
public Builder clearAccountDisplayedOpOnly() {
accountDisplayedOpOnly_ = false;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList select_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureSelectIsMutable() {
if (!((bitField0_ & 0x00080000) == 0x00080000)) {
select_ = new com.google.protobuf.LazyStringArrayList(select_);
bitField0_ |= 0x00080000;
}
}
/**
* repeated string select = 14;
*/
public com.google.protobuf.ProtocolStringList
getSelectList() {
return select_.getUnmodifiableView();
}
/**
* repeated string select = 14;
*/
public int getSelectCount() {
return select_.size();
}
/**
* repeated string select = 14;
*/
public java.lang.String getSelect(int index) {
return select_.get(index);
}
/**
* repeated string select = 14;
*/
public com.google.protobuf.ByteString
getSelectBytes(int index) {
return select_.getByteString(index);
}
/**
* repeated string select = 14;
*/
public Builder setSelect(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSelectIsMutable();
select_.set(index, value);
onChanged();
return this;
}
/**
* repeated string select = 14;
*/
public Builder addSelect(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSelectIsMutable();
select_.add(value);
onChanged();
return this;
}
/**
* repeated string select = 14;
*/
public Builder addAllSelect(
java.lang.Iterable values) {
ensureSelectIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, select_);
onChanged();
return this;
}
/**
* repeated string select = 14;
*/
public Builder clearSelect() {
select_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00080000);
onChanged();
return this;
}
/**
* repeated string select = 14;
*/
public Builder addSelectBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureSelectIsMutable();
select_.add(value);
onChanged();
return this;
}
private java.lang.Object output_ = "";
/**
* string output = 15;
*/
public java.lang.String getOutput() {
java.lang.Object ref = output_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
output_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string output = 15;
*/
public com.google.protobuf.ByteString
getOutputBytes() {
java.lang.Object ref = output_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
output_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string output = 15;
*/
public Builder setOutput(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
output_ = value;
onChanged();
return this;
}
/**
* string output = 15;
*/
public Builder clearOutput() {
output_ = getDefaultInstance().getOutput();
onChanged();
return this;
}
/**
* string output = 15;
*/
public Builder setOutputBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
output_ = value;
onChanged();
return this;
}
private java.lang.Object dumpToFile_ = "";
/**
* string dump_to_file = 16;
*/
public java.lang.String getDumpToFile() {
java.lang.Object ref = dumpToFile_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
dumpToFile_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string dump_to_file = 16;
*/
public com.google.protobuf.ByteString
getDumpToFileBytes() {
java.lang.Object ref = dumpToFile_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
dumpToFile_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string dump_to_file = 16;
*/
public Builder setDumpToFile(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
dumpToFile_ = value;
onChanged();
return this;
}
/**
* string dump_to_file = 16;
*/
public Builder clearDumpToFile() {
dumpToFile_ = getDefaultInstance().getDumpToFile();
onChanged();
return this;
}
/**
* string dump_to_file = 16;
*/
public Builder setDumpToFileBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
dumpToFile_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.tfprof.OptionsProto)
}
// @@protoc_insertion_point(class_scope:tensorflow.tfprof.OptionsProto)
private static final tensorflow.tfprof.TfprofOptions.OptionsProto DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.tfprof.TfprofOptions.OptionsProto();
}
public static tensorflow.tfprof.TfprofOptions.OptionsProto getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public OptionsProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new OptionsProto(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.tfprof.TfprofOptions.OptionsProto getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AdvisorOptionsProtoOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.tfprof.AdvisorOptionsProto)
com.google.protobuf.MessageOrBuilder {
/**
*
* checker name -> a dict of key-value options.
*
*
* map<string, .tensorflow.tfprof.AdvisorOptionsProto.CheckerOption> checkers = 1;
*/
int getCheckersCount();
/**
*
* checker name -> a dict of key-value options.
*
*
* map<string, .tensorflow.tfprof.AdvisorOptionsProto.CheckerOption> checkers = 1;
*/
boolean containsCheckers(
java.lang.String key);
/**
* Use {@link #getCheckersMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getCheckers();
/**
*
* checker name -> a dict of key-value options.
*
*
* map<string, .tensorflow.tfprof.AdvisorOptionsProto.CheckerOption> checkers = 1;
*/
java.util.Map
getCheckersMap();
/**
*
* checker name -> a dict of key-value options.
*
*
* map<string, .tensorflow.tfprof.AdvisorOptionsProto.CheckerOption> checkers = 1;
*/
tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption getCheckersOrDefault(
java.lang.String key,
tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption defaultValue);
/**
*
* checker name -> a dict of key-value options.
*
*
* map<string, .tensorflow.tfprof.AdvisorOptionsProto.CheckerOption> checkers = 1;
*/
tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption getCheckersOrThrow(
java.lang.String key);
}
/**
* Protobuf type {@code tensorflow.tfprof.AdvisorOptionsProto}
*/
public static final class AdvisorOptionsProto extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.tfprof.AdvisorOptionsProto)
AdvisorOptionsProtoOrBuilder {
private static final long serialVersionUID = 0L;
// Use AdvisorOptionsProto.newBuilder() to construct.
private AdvisorOptionsProto(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AdvisorOptionsProto() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private AdvisorOptionsProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
checkers_ = com.google.protobuf.MapField.newMapField(
CheckersDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000001;
}
com.google.protobuf.MapEntry
checkers__ = input.readMessage(
CheckersDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
checkers_.getMutableMap().put(
checkers__.getKey(), checkers__.getValue());
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.tfprof.TfprofOptions.internal_static_tensorflow_tfprof_AdvisorOptionsProto_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 1:
return internalGetCheckers();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.tfprof.TfprofOptions.internal_static_tensorflow_tfprof_AdvisorOptionsProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.class, tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.Builder.class);
}
public interface CheckerOptionOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.tfprof.AdvisorOptionsProto.CheckerOption)
com.google.protobuf.MessageOrBuilder {
/**
* map<string, string> options = 1;
*/
int getOptionsCount();
/**
* map<string, string> options = 1;
*/
boolean containsOptions(
java.lang.String key);
/**
* Use {@link #getOptionsMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getOptions();
/**
* map<string, string> options = 1;
*/
java.util.Map
getOptionsMap();
/**
* map<string, string> options = 1;
*/
java.lang.String getOptionsOrDefault(
java.lang.String key,
java.lang.String defaultValue);
/**
* map<string, string> options = 1;
*/
java.lang.String getOptionsOrThrow(
java.lang.String key);
}
/**
* Protobuf type {@code tensorflow.tfprof.AdvisorOptionsProto.CheckerOption}
*/
public static final class CheckerOption extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.tfprof.AdvisorOptionsProto.CheckerOption)
CheckerOptionOrBuilder {
private static final long serialVersionUID = 0L;
// Use CheckerOption.newBuilder() to construct.
private CheckerOption(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CheckerOption() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CheckerOption(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
options_ = com.google.protobuf.MapField.newMapField(
OptionsDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000001;
}
com.google.protobuf.MapEntry
options__ = input.readMessage(
OptionsDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
options_.getMutableMap().put(
options__.getKey(), options__.getValue());
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.tfprof.TfprofOptions.internal_static_tensorflow_tfprof_AdvisorOptionsProto_CheckerOption_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 1:
return internalGetOptions();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.tfprof.TfprofOptions.internal_static_tensorflow_tfprof_AdvisorOptionsProto_CheckerOption_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption.class, tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption.Builder.class);
}
public static final int OPTIONS_FIELD_NUMBER = 1;
private static final class OptionsDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
tensorflow.tfprof.TfprofOptions.internal_static_tensorflow_tfprof_AdvisorOptionsProto_CheckerOption_OptionsEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> options_;
private com.google.protobuf.MapField
internalGetOptions() {
if (options_ == null) {
return com.google.protobuf.MapField.emptyMapField(
OptionsDefaultEntryHolder.defaultEntry);
}
return options_;
}
public int getOptionsCount() {
return internalGetOptions().getMap().size();
}
/**
* map<string, string> options = 1;
*/
public boolean containsOptions(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetOptions().getMap().containsKey(key);
}
/**
* Use {@link #getOptionsMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getOptions() {
return getOptionsMap();
}
/**
* map<string, string> options = 1;
*/
public java.util.Map getOptionsMap() {
return internalGetOptions().getMap();
}
/**
* map<string, string> options = 1;
*/
public java.lang.String getOptionsOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetOptions().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, string> options = 1;
*/
public java.lang.String getOptionsOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetOptions().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetOptions(),
OptionsDefaultEntryHolder.defaultEntry,
1);
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (java.util.Map.Entry entry
: internalGetOptions().getMap().entrySet()) {
com.google.protobuf.MapEntry
options__ = OptionsDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, options__);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption)) {
return super.equals(obj);
}
tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption other = (tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption) obj;
boolean result = true;
result = result && internalGetOptions().equals(
other.internalGetOptions());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (!internalGetOptions().getMap().isEmpty()) {
hash = (37 * hash) + OPTIONS_FIELD_NUMBER;
hash = (53 * hash) + internalGetOptions().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.tfprof.AdvisorOptionsProto.CheckerOption}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.tfprof.AdvisorOptionsProto.CheckerOption)
tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOptionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.tfprof.TfprofOptions.internal_static_tensorflow_tfprof_AdvisorOptionsProto_CheckerOption_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 1:
return internalGetOptions();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 1:
return internalGetMutableOptions();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.tfprof.TfprofOptions.internal_static_tensorflow_tfprof_AdvisorOptionsProto_CheckerOption_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption.class, tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption.Builder.class);
}
// Construct using tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
internalGetMutableOptions().clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.tfprof.TfprofOptions.internal_static_tensorflow_tfprof_AdvisorOptionsProto_CheckerOption_descriptor;
}
@java.lang.Override
public tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption getDefaultInstanceForType() {
return tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption.getDefaultInstance();
}
@java.lang.Override
public tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption build() {
tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption buildPartial() {
tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption result = new tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption(this);
int from_bitField0_ = bitField0_;
result.options_ = internalGetOptions();
result.options_.makeImmutable();
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption) {
return mergeFrom((tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption other) {
if (other == tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption.getDefaultInstance()) return this;
internalGetMutableOptions().mergeFrom(
other.internalGetOptions());
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> options_;
private com.google.protobuf.MapField
internalGetOptions() {
if (options_ == null) {
return com.google.protobuf.MapField.emptyMapField(
OptionsDefaultEntryHolder.defaultEntry);
}
return options_;
}
private com.google.protobuf.MapField
internalGetMutableOptions() {
onChanged();;
if (options_ == null) {
options_ = com.google.protobuf.MapField.newMapField(
OptionsDefaultEntryHolder.defaultEntry);
}
if (!options_.isMutable()) {
options_ = options_.copy();
}
return options_;
}
public int getOptionsCount() {
return internalGetOptions().getMap().size();
}
/**
* map<string, string> options = 1;
*/
public boolean containsOptions(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetOptions().getMap().containsKey(key);
}
/**
* Use {@link #getOptionsMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getOptions() {
return getOptionsMap();
}
/**
* map<string, string> options = 1;
*/
public java.util.Map getOptionsMap() {
return internalGetOptions().getMap();
}
/**
* map<string, string> options = 1;
*/
public java.lang.String getOptionsOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetOptions().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, string> options = 1;
*/
public java.lang.String getOptionsOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetOptions().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearOptions() {
internalGetMutableOptions().getMutableMap()
.clear();
return this;
}
/**
* map<string, string> options = 1;
*/
public Builder removeOptions(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableOptions().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableOptions() {
return internalGetMutableOptions().getMutableMap();
}
/**
* map<string, string> options = 1;
*/
public Builder putOptions(
java.lang.String key,
java.lang.String value) {
if (key == null) { throw new java.lang.NullPointerException(); }
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutableOptions().getMutableMap()
.put(key, value);
return this;
}
/**
* map<string, string> options = 1;
*/
public Builder putAllOptions(
java.util.Map values) {
internalGetMutableOptions().getMutableMap()
.putAll(values);
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.tfprof.AdvisorOptionsProto.CheckerOption)
}
// @@protoc_insertion_point(class_scope:tensorflow.tfprof.AdvisorOptionsProto.CheckerOption)
private static final tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption();
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CheckerOption parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CheckerOption(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int CHECKERS_FIELD_NUMBER = 1;
private static final class CheckersDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
tensorflow.tfprof.TfprofOptions.internal_static_tensorflow_tfprof_AdvisorOptionsProto_CheckersEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption.getDefaultInstance());
}
private com.google.protobuf.MapField<
java.lang.String, tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption> checkers_;
private com.google.protobuf.MapField
internalGetCheckers() {
if (checkers_ == null) {
return com.google.protobuf.MapField.emptyMapField(
CheckersDefaultEntryHolder.defaultEntry);
}
return checkers_;
}
public int getCheckersCount() {
return internalGetCheckers().getMap().size();
}
/**
*
* checker name -> a dict of key-value options.
*
*
* map<string, .tensorflow.tfprof.AdvisorOptionsProto.CheckerOption> checkers = 1;
*/
public boolean containsCheckers(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetCheckers().getMap().containsKey(key);
}
/**
* Use {@link #getCheckersMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getCheckers() {
return getCheckersMap();
}
/**
*
* checker name -> a dict of key-value options.
*
*
* map<string, .tensorflow.tfprof.AdvisorOptionsProto.CheckerOption> checkers = 1;
*/
public java.util.Map getCheckersMap() {
return internalGetCheckers().getMap();
}
/**
*
* checker name -> a dict of key-value options.
*
*
* map<string, .tensorflow.tfprof.AdvisorOptionsProto.CheckerOption> checkers = 1;
*/
public tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption getCheckersOrDefault(
java.lang.String key,
tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetCheckers().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* checker name -> a dict of key-value options.
*
*
* map<string, .tensorflow.tfprof.AdvisorOptionsProto.CheckerOption> checkers = 1;
*/
public tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption getCheckersOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetCheckers().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetCheckers(),
CheckersDefaultEntryHolder.defaultEntry,
1);
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (java.util.Map.Entry entry
: internalGetCheckers().getMap().entrySet()) {
com.google.protobuf.MapEntry
checkers__ = CheckersDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, checkers__);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto)) {
return super.equals(obj);
}
tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto other = (tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto) obj;
boolean result = true;
result = result && internalGetCheckers().equals(
other.internalGetCheckers());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (!internalGetCheckers().getMap().isEmpty()) {
hash = (37 * hash) + CHECKERS_FIELD_NUMBER;
hash = (53 * hash) + internalGetCheckers().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.tfprof.AdvisorOptionsProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.tfprof.AdvisorOptionsProto)
tensorflow.tfprof.TfprofOptions.AdvisorOptionsProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.tfprof.TfprofOptions.internal_static_tensorflow_tfprof_AdvisorOptionsProto_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 1:
return internalGetCheckers();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 1:
return internalGetMutableCheckers();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.tfprof.TfprofOptions.internal_static_tensorflow_tfprof_AdvisorOptionsProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.class, tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.Builder.class);
}
// Construct using tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
internalGetMutableCheckers().clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.tfprof.TfprofOptions.internal_static_tensorflow_tfprof_AdvisorOptionsProto_descriptor;
}
@java.lang.Override
public tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto getDefaultInstanceForType() {
return tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.getDefaultInstance();
}
@java.lang.Override
public tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto build() {
tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto buildPartial() {
tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto result = new tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto(this);
int from_bitField0_ = bitField0_;
result.checkers_ = internalGetCheckers();
result.checkers_.makeImmutable();
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto) {
return mergeFrom((tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto other) {
if (other == tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.getDefaultInstance()) return this;
internalGetMutableCheckers().mergeFrom(
other.internalGetCheckers());
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.MapField<
java.lang.String, tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption> checkers_;
private com.google.protobuf.MapField
internalGetCheckers() {
if (checkers_ == null) {
return com.google.protobuf.MapField.emptyMapField(
CheckersDefaultEntryHolder.defaultEntry);
}
return checkers_;
}
private com.google.protobuf.MapField
internalGetMutableCheckers() {
onChanged();;
if (checkers_ == null) {
checkers_ = com.google.protobuf.MapField.newMapField(
CheckersDefaultEntryHolder.defaultEntry);
}
if (!checkers_.isMutable()) {
checkers_ = checkers_.copy();
}
return checkers_;
}
public int getCheckersCount() {
return internalGetCheckers().getMap().size();
}
/**
*
* checker name -> a dict of key-value options.
*
*
* map<string, .tensorflow.tfprof.AdvisorOptionsProto.CheckerOption> checkers = 1;
*/
public boolean containsCheckers(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetCheckers().getMap().containsKey(key);
}
/**
* Use {@link #getCheckersMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getCheckers() {
return getCheckersMap();
}
/**
*
* checker name -> a dict of key-value options.
*
*
* map<string, .tensorflow.tfprof.AdvisorOptionsProto.CheckerOption> checkers = 1;
*/
public java.util.Map getCheckersMap() {
return internalGetCheckers().getMap();
}
/**
*
* checker name -> a dict of key-value options.
*
*
* map<string, .tensorflow.tfprof.AdvisorOptionsProto.CheckerOption> checkers = 1;
*/
public tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption getCheckersOrDefault(
java.lang.String key,
tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetCheckers().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* checker name -> a dict of key-value options.
*
*
* map<string, .tensorflow.tfprof.AdvisorOptionsProto.CheckerOption> checkers = 1;
*/
public tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption getCheckersOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetCheckers().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearCheckers() {
internalGetMutableCheckers().getMutableMap()
.clear();
return this;
}
/**
*
* checker name -> a dict of key-value options.
*
*
* map<string, .tensorflow.tfprof.AdvisorOptionsProto.CheckerOption> checkers = 1;
*/
public Builder removeCheckers(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableCheckers().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableCheckers() {
return internalGetMutableCheckers().getMutableMap();
}
/**
*
* checker name -> a dict of key-value options.
*
*
* map<string, .tensorflow.tfprof.AdvisorOptionsProto.CheckerOption> checkers = 1;
*/
public Builder putCheckers(
java.lang.String key,
tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto.CheckerOption value) {
if (key == null) { throw new java.lang.NullPointerException(); }
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutableCheckers().getMutableMap()
.put(key, value);
return this;
}
/**
*
* checker name -> a dict of key-value options.
*
*
* map<string, .tensorflow.tfprof.AdvisorOptionsProto.CheckerOption> checkers = 1;
*/
public Builder putAllCheckers(
java.util.Map values) {
internalGetMutableCheckers().getMutableMap()
.putAll(values);
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.tfprof.AdvisorOptionsProto)
}
// @@protoc_insertion_point(class_scope:tensorflow.tfprof.AdvisorOptionsProto)
private static final tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto();
}
public static tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AdvisorOptionsProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new AdvisorOptionsProto(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.tfprof.TfprofOptions.AdvisorOptionsProto getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_tfprof_OptionsProto_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_tfprof_OptionsProto_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_tfprof_AdvisorOptionsProto_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_tfprof_AdvisorOptionsProto_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_tfprof_AdvisorOptionsProto_CheckersEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_tfprof_AdvisorOptionsProto_CheckersEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_tfprof_AdvisorOptionsProto_CheckerOption_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_tfprof_AdvisorOptionsProto_CheckerOption_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_tfprof_AdvisorOptionsProto_CheckerOption_OptionsEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_tfprof_AdvisorOptionsProto_CheckerOption_OptionsEntry_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n-tensorflow/core/profiler/tfprof_option" +
"s.proto\022\021tensorflow.tfprof\"\225\004\n\014OptionsPr" +
"oto\022\021\n\tmax_depth\030\001 \001(\003\022\021\n\tmin_bytes\030\002 \001(" +
"\003\022\026\n\016min_peak_bytes\030\023 \001(\003\022\032\n\022min_residua" +
"l_bytes\030\024 \001(\003\022\030\n\020min_output_bytes\030\025 \001(\003\022" +
"\022\n\nmin_micros\030\003 \001(\003\022\036\n\026min_accelerator_m" +
"icros\030\026 \001(\003\022\026\n\016min_cpu_micros\030\027 \001(\003\022\022\n\nm" +
"in_params\030\004 \001(\003\022\025\n\rmin_float_ops\030\005 \001(\003\022\026" +
"\n\016min_occurrence\030\021 \001(\003\022\014\n\004step\030\022 \001(\003\022\020\n\010" +
"order_by\030\007 \001(\t\022\034\n\024account_type_regexes\030\010" +
" \003(\t\022\032\n\022start_name_regexes\030\t \003(\t\022\031\n\021trim" +
"_name_regexes\030\n \003(\t\022\031\n\021show_name_regexes" +
"\030\013 \003(\t\022\031\n\021hide_name_regexes\030\014 \003(\t\022!\n\031acc" +
"ount_displayed_op_only\030\r \001(\010\022\016\n\006select\030\016" +
" \003(\t\022\016\n\006output\030\017 \001(\t\022\024\n\014dump_to_file\030\020 \001" +
"(\t\"\332\002\n\023AdvisorOptionsProto\022F\n\010checkers\030\001" +
" \003(\01324.tensorflow.tfprof.AdvisorOptionsP" +
"roto.CheckersEntry\032e\n\rCheckersEntry\022\013\n\003k" +
"ey\030\001 \001(\t\022C\n\005value\030\002 \001(\01324.tensorflow.tfp" +
"rof.AdvisorOptionsProto.CheckerOption:\0028" +
"\001\032\223\001\n\rCheckerOption\022R\n\007options\030\001 \003(\0132A.t" +
"ensorflow.tfprof.AdvisorOptionsProto.Che" +
"ckerOption.OptionsEntry\032.\n\014OptionsEntry\022" +
"\013\n\003key\030\001 \001(\t\022\r\n\005value\030\002 \001(\t:\0028\001b\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
internal_static_tensorflow_tfprof_OptionsProto_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_tensorflow_tfprof_OptionsProto_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_tfprof_OptionsProto_descriptor,
new java.lang.String[] { "MaxDepth", "MinBytes", "MinPeakBytes", "MinResidualBytes", "MinOutputBytes", "MinMicros", "MinAcceleratorMicros", "MinCpuMicros", "MinParams", "MinFloatOps", "MinOccurrence", "Step", "OrderBy", "AccountTypeRegexes", "StartNameRegexes", "TrimNameRegexes", "ShowNameRegexes", "HideNameRegexes", "AccountDisplayedOpOnly", "Select", "Output", "DumpToFile", });
internal_static_tensorflow_tfprof_AdvisorOptionsProto_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_tensorflow_tfprof_AdvisorOptionsProto_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_tfprof_AdvisorOptionsProto_descriptor,
new java.lang.String[] { "Checkers", });
internal_static_tensorflow_tfprof_AdvisorOptionsProto_CheckersEntry_descriptor =
internal_static_tensorflow_tfprof_AdvisorOptionsProto_descriptor.getNestedTypes().get(0);
internal_static_tensorflow_tfprof_AdvisorOptionsProto_CheckersEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_tfprof_AdvisorOptionsProto_CheckersEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_tensorflow_tfprof_AdvisorOptionsProto_CheckerOption_descriptor =
internal_static_tensorflow_tfprof_AdvisorOptionsProto_descriptor.getNestedTypes().get(1);
internal_static_tensorflow_tfprof_AdvisorOptionsProto_CheckerOption_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_tfprof_AdvisorOptionsProto_CheckerOption_descriptor,
new java.lang.String[] { "Options", });
internal_static_tensorflow_tfprof_AdvisorOptionsProto_CheckerOption_OptionsEntry_descriptor =
internal_static_tensorflow_tfprof_AdvisorOptionsProto_CheckerOption_descriptor.getNestedTypes().get(0);
internal_static_tensorflow_tfprof_AdvisorOptionsProto_CheckerOption_OptionsEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_tfprof_AdvisorOptionsProto_CheckerOption_OptionsEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy