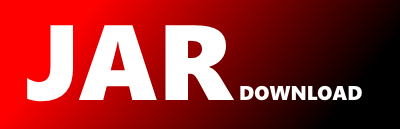
tensorflow.tpu.op_profile.OpProfile Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: tensorflow/contrib/tpu/profiler/op_profile.proto
package tensorflow.tpu.op_profile;
public final class OpProfile {
private OpProfile() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface ProfileOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.tpu.op_profile.Profile)
com.google.protobuf.MessageOrBuilder {
/**
*
* Root of a profile broken down by instruction category.
*
*
* .tensorflow.tpu.op_profile.Node by_category = 1;
*/
boolean hasByCategory();
/**
*
* Root of a profile broken down by instruction category.
*
*
* .tensorflow.tpu.op_profile.Node by_category = 1;
*/
tensorflow.tpu.op_profile.OpProfile.Node getByCategory();
/**
*
* Root of a profile broken down by instruction category.
*
*
* .tensorflow.tpu.op_profile.Node by_category = 1;
*/
tensorflow.tpu.op_profile.OpProfile.NodeOrBuilder getByCategoryOrBuilder();
/**
*
* Root of a profile broken down by program.
*
*
* .tensorflow.tpu.op_profile.Node by_program = 4;
*/
boolean hasByProgram();
/**
*
* Root of a profile broken down by program.
*
*
* .tensorflow.tpu.op_profile.Node by_program = 4;
*/
tensorflow.tpu.op_profile.OpProfile.Node getByProgram();
/**
*
* Root of a profile broken down by program.
*
*
* .tensorflow.tpu.op_profile.Node by_program = 4;
*/
tensorflow.tpu.op_profile.OpProfile.NodeOrBuilder getByProgramOrBuilder();
}
/**
*
* Profile is the top-level data that summarizes a program.
*
*
* Protobuf type {@code tensorflow.tpu.op_profile.Profile}
*/
public static final class Profile extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.tpu.op_profile.Profile)
ProfileOrBuilder {
private static final long serialVersionUID = 0L;
// Use Profile.newBuilder() to construct.
private Profile(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Profile() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Profile(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
tensorflow.tpu.op_profile.OpProfile.Node.Builder subBuilder = null;
if (byCategory_ != null) {
subBuilder = byCategory_.toBuilder();
}
byCategory_ = input.readMessage(tensorflow.tpu.op_profile.OpProfile.Node.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(byCategory_);
byCategory_ = subBuilder.buildPartial();
}
break;
}
case 34: {
tensorflow.tpu.op_profile.OpProfile.Node.Builder subBuilder = null;
if (byProgram_ != null) {
subBuilder = byProgram_.toBuilder();
}
byProgram_ = input.readMessage(tensorflow.tpu.op_profile.OpProfile.Node.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(byProgram_);
byProgram_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Profile_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Profile_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.tpu.op_profile.OpProfile.Profile.class, tensorflow.tpu.op_profile.OpProfile.Profile.Builder.class);
}
public static final int BY_CATEGORY_FIELD_NUMBER = 1;
private tensorflow.tpu.op_profile.OpProfile.Node byCategory_;
/**
*
* Root of a profile broken down by instruction category.
*
*
* .tensorflow.tpu.op_profile.Node by_category = 1;
*/
public boolean hasByCategory() {
return byCategory_ != null;
}
/**
*
* Root of a profile broken down by instruction category.
*
*
* .tensorflow.tpu.op_profile.Node by_category = 1;
*/
public tensorflow.tpu.op_profile.OpProfile.Node getByCategory() {
return byCategory_ == null ? tensorflow.tpu.op_profile.OpProfile.Node.getDefaultInstance() : byCategory_;
}
/**
*
* Root of a profile broken down by instruction category.
*
*
* .tensorflow.tpu.op_profile.Node by_category = 1;
*/
public tensorflow.tpu.op_profile.OpProfile.NodeOrBuilder getByCategoryOrBuilder() {
return getByCategory();
}
public static final int BY_PROGRAM_FIELD_NUMBER = 4;
private tensorflow.tpu.op_profile.OpProfile.Node byProgram_;
/**
*
* Root of a profile broken down by program.
*
*
* .tensorflow.tpu.op_profile.Node by_program = 4;
*/
public boolean hasByProgram() {
return byProgram_ != null;
}
/**
*
* Root of a profile broken down by program.
*
*
* .tensorflow.tpu.op_profile.Node by_program = 4;
*/
public tensorflow.tpu.op_profile.OpProfile.Node getByProgram() {
return byProgram_ == null ? tensorflow.tpu.op_profile.OpProfile.Node.getDefaultInstance() : byProgram_;
}
/**
*
* Root of a profile broken down by program.
*
*
* .tensorflow.tpu.op_profile.Node by_program = 4;
*/
public tensorflow.tpu.op_profile.OpProfile.NodeOrBuilder getByProgramOrBuilder() {
return getByProgram();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (byCategory_ != null) {
output.writeMessage(1, getByCategory());
}
if (byProgram_ != null) {
output.writeMessage(4, getByProgram());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (byCategory_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getByCategory());
}
if (byProgram_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getByProgram());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.tpu.op_profile.OpProfile.Profile)) {
return super.equals(obj);
}
tensorflow.tpu.op_profile.OpProfile.Profile other = (tensorflow.tpu.op_profile.OpProfile.Profile) obj;
boolean result = true;
result = result && (hasByCategory() == other.hasByCategory());
if (hasByCategory()) {
result = result && getByCategory()
.equals(other.getByCategory());
}
result = result && (hasByProgram() == other.hasByProgram());
if (hasByProgram()) {
result = result && getByProgram()
.equals(other.getByProgram());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasByCategory()) {
hash = (37 * hash) + BY_CATEGORY_FIELD_NUMBER;
hash = (53 * hash) + getByCategory().hashCode();
}
if (hasByProgram()) {
hash = (37 * hash) + BY_PROGRAM_FIELD_NUMBER;
hash = (53 * hash) + getByProgram().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.tpu.op_profile.OpProfile.Profile parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tpu.op_profile.OpProfile.Profile parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Profile parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tpu.op_profile.OpProfile.Profile parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Profile parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tpu.op_profile.OpProfile.Profile parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Profile parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.tpu.op_profile.OpProfile.Profile parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Profile parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.tpu.op_profile.OpProfile.Profile parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Profile parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.tpu.op_profile.OpProfile.Profile parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.tpu.op_profile.OpProfile.Profile prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Profile is the top-level data that summarizes a program.
*
*
* Protobuf type {@code tensorflow.tpu.op_profile.Profile}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.tpu.op_profile.Profile)
tensorflow.tpu.op_profile.OpProfile.ProfileOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Profile_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Profile_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.tpu.op_profile.OpProfile.Profile.class, tensorflow.tpu.op_profile.OpProfile.Profile.Builder.class);
}
// Construct using tensorflow.tpu.op_profile.OpProfile.Profile.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (byCategoryBuilder_ == null) {
byCategory_ = null;
} else {
byCategory_ = null;
byCategoryBuilder_ = null;
}
if (byProgramBuilder_ == null) {
byProgram_ = null;
} else {
byProgram_ = null;
byProgramBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Profile_descriptor;
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Profile getDefaultInstanceForType() {
return tensorflow.tpu.op_profile.OpProfile.Profile.getDefaultInstance();
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Profile build() {
tensorflow.tpu.op_profile.OpProfile.Profile result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Profile buildPartial() {
tensorflow.tpu.op_profile.OpProfile.Profile result = new tensorflow.tpu.op_profile.OpProfile.Profile(this);
if (byCategoryBuilder_ == null) {
result.byCategory_ = byCategory_;
} else {
result.byCategory_ = byCategoryBuilder_.build();
}
if (byProgramBuilder_ == null) {
result.byProgram_ = byProgram_;
} else {
result.byProgram_ = byProgramBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.tpu.op_profile.OpProfile.Profile) {
return mergeFrom((tensorflow.tpu.op_profile.OpProfile.Profile)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.tpu.op_profile.OpProfile.Profile other) {
if (other == tensorflow.tpu.op_profile.OpProfile.Profile.getDefaultInstance()) return this;
if (other.hasByCategory()) {
mergeByCategory(other.getByCategory());
}
if (other.hasByProgram()) {
mergeByProgram(other.getByProgram());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.tpu.op_profile.OpProfile.Profile parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.tpu.op_profile.OpProfile.Profile) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private tensorflow.tpu.op_profile.OpProfile.Node byCategory_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Node, tensorflow.tpu.op_profile.OpProfile.Node.Builder, tensorflow.tpu.op_profile.OpProfile.NodeOrBuilder> byCategoryBuilder_;
/**
*
* Root of a profile broken down by instruction category.
*
*
* .tensorflow.tpu.op_profile.Node by_category = 1;
*/
public boolean hasByCategory() {
return byCategoryBuilder_ != null || byCategory_ != null;
}
/**
*
* Root of a profile broken down by instruction category.
*
*
* .tensorflow.tpu.op_profile.Node by_category = 1;
*/
public tensorflow.tpu.op_profile.OpProfile.Node getByCategory() {
if (byCategoryBuilder_ == null) {
return byCategory_ == null ? tensorflow.tpu.op_profile.OpProfile.Node.getDefaultInstance() : byCategory_;
} else {
return byCategoryBuilder_.getMessage();
}
}
/**
*
* Root of a profile broken down by instruction category.
*
*
* .tensorflow.tpu.op_profile.Node by_category = 1;
*/
public Builder setByCategory(tensorflow.tpu.op_profile.OpProfile.Node value) {
if (byCategoryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
byCategory_ = value;
onChanged();
} else {
byCategoryBuilder_.setMessage(value);
}
return this;
}
/**
*
* Root of a profile broken down by instruction category.
*
*
* .tensorflow.tpu.op_profile.Node by_category = 1;
*/
public Builder setByCategory(
tensorflow.tpu.op_profile.OpProfile.Node.Builder builderForValue) {
if (byCategoryBuilder_ == null) {
byCategory_ = builderForValue.build();
onChanged();
} else {
byCategoryBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Root of a profile broken down by instruction category.
*
*
* .tensorflow.tpu.op_profile.Node by_category = 1;
*/
public Builder mergeByCategory(tensorflow.tpu.op_profile.OpProfile.Node value) {
if (byCategoryBuilder_ == null) {
if (byCategory_ != null) {
byCategory_ =
tensorflow.tpu.op_profile.OpProfile.Node.newBuilder(byCategory_).mergeFrom(value).buildPartial();
} else {
byCategory_ = value;
}
onChanged();
} else {
byCategoryBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Root of a profile broken down by instruction category.
*
*
* .tensorflow.tpu.op_profile.Node by_category = 1;
*/
public Builder clearByCategory() {
if (byCategoryBuilder_ == null) {
byCategory_ = null;
onChanged();
} else {
byCategory_ = null;
byCategoryBuilder_ = null;
}
return this;
}
/**
*
* Root of a profile broken down by instruction category.
*
*
* .tensorflow.tpu.op_profile.Node by_category = 1;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.Builder getByCategoryBuilder() {
onChanged();
return getByCategoryFieldBuilder().getBuilder();
}
/**
*
* Root of a profile broken down by instruction category.
*
*
* .tensorflow.tpu.op_profile.Node by_category = 1;
*/
public tensorflow.tpu.op_profile.OpProfile.NodeOrBuilder getByCategoryOrBuilder() {
if (byCategoryBuilder_ != null) {
return byCategoryBuilder_.getMessageOrBuilder();
} else {
return byCategory_ == null ?
tensorflow.tpu.op_profile.OpProfile.Node.getDefaultInstance() : byCategory_;
}
}
/**
*
* Root of a profile broken down by instruction category.
*
*
* .tensorflow.tpu.op_profile.Node by_category = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Node, tensorflow.tpu.op_profile.OpProfile.Node.Builder, tensorflow.tpu.op_profile.OpProfile.NodeOrBuilder>
getByCategoryFieldBuilder() {
if (byCategoryBuilder_ == null) {
byCategoryBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Node, tensorflow.tpu.op_profile.OpProfile.Node.Builder, tensorflow.tpu.op_profile.OpProfile.NodeOrBuilder>(
getByCategory(),
getParentForChildren(),
isClean());
byCategory_ = null;
}
return byCategoryBuilder_;
}
private tensorflow.tpu.op_profile.OpProfile.Node byProgram_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Node, tensorflow.tpu.op_profile.OpProfile.Node.Builder, tensorflow.tpu.op_profile.OpProfile.NodeOrBuilder> byProgramBuilder_;
/**
*
* Root of a profile broken down by program.
*
*
* .tensorflow.tpu.op_profile.Node by_program = 4;
*/
public boolean hasByProgram() {
return byProgramBuilder_ != null || byProgram_ != null;
}
/**
*
* Root of a profile broken down by program.
*
*
* .tensorflow.tpu.op_profile.Node by_program = 4;
*/
public tensorflow.tpu.op_profile.OpProfile.Node getByProgram() {
if (byProgramBuilder_ == null) {
return byProgram_ == null ? tensorflow.tpu.op_profile.OpProfile.Node.getDefaultInstance() : byProgram_;
} else {
return byProgramBuilder_.getMessage();
}
}
/**
*
* Root of a profile broken down by program.
*
*
* .tensorflow.tpu.op_profile.Node by_program = 4;
*/
public Builder setByProgram(tensorflow.tpu.op_profile.OpProfile.Node value) {
if (byProgramBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
byProgram_ = value;
onChanged();
} else {
byProgramBuilder_.setMessage(value);
}
return this;
}
/**
*
* Root of a profile broken down by program.
*
*
* .tensorflow.tpu.op_profile.Node by_program = 4;
*/
public Builder setByProgram(
tensorflow.tpu.op_profile.OpProfile.Node.Builder builderForValue) {
if (byProgramBuilder_ == null) {
byProgram_ = builderForValue.build();
onChanged();
} else {
byProgramBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Root of a profile broken down by program.
*
*
* .tensorflow.tpu.op_profile.Node by_program = 4;
*/
public Builder mergeByProgram(tensorflow.tpu.op_profile.OpProfile.Node value) {
if (byProgramBuilder_ == null) {
if (byProgram_ != null) {
byProgram_ =
tensorflow.tpu.op_profile.OpProfile.Node.newBuilder(byProgram_).mergeFrom(value).buildPartial();
} else {
byProgram_ = value;
}
onChanged();
} else {
byProgramBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Root of a profile broken down by program.
*
*
* .tensorflow.tpu.op_profile.Node by_program = 4;
*/
public Builder clearByProgram() {
if (byProgramBuilder_ == null) {
byProgram_ = null;
onChanged();
} else {
byProgram_ = null;
byProgramBuilder_ = null;
}
return this;
}
/**
*
* Root of a profile broken down by program.
*
*
* .tensorflow.tpu.op_profile.Node by_program = 4;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.Builder getByProgramBuilder() {
onChanged();
return getByProgramFieldBuilder().getBuilder();
}
/**
*
* Root of a profile broken down by program.
*
*
* .tensorflow.tpu.op_profile.Node by_program = 4;
*/
public tensorflow.tpu.op_profile.OpProfile.NodeOrBuilder getByProgramOrBuilder() {
if (byProgramBuilder_ != null) {
return byProgramBuilder_.getMessageOrBuilder();
} else {
return byProgram_ == null ?
tensorflow.tpu.op_profile.OpProfile.Node.getDefaultInstance() : byProgram_;
}
}
/**
*
* Root of a profile broken down by program.
*
*
* .tensorflow.tpu.op_profile.Node by_program = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Node, tensorflow.tpu.op_profile.OpProfile.Node.Builder, tensorflow.tpu.op_profile.OpProfile.NodeOrBuilder>
getByProgramFieldBuilder() {
if (byProgramBuilder_ == null) {
byProgramBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Node, tensorflow.tpu.op_profile.OpProfile.Node.Builder, tensorflow.tpu.op_profile.OpProfile.NodeOrBuilder>(
getByProgram(),
getParentForChildren(),
isClean());
byProgram_ = null;
}
return byProgramBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.tpu.op_profile.Profile)
}
// @@protoc_insertion_point(class_scope:tensorflow.tpu.op_profile.Profile)
private static final tensorflow.tpu.op_profile.OpProfile.Profile DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.tpu.op_profile.OpProfile.Profile();
}
public static tensorflow.tpu.op_profile.OpProfile.Profile getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Profile parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Profile(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Profile getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface NodeOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.tpu.op_profile.Node)
com.google.protobuf.MessageOrBuilder {
/**
*
* Semantics depend on contents.
*
*
* string name = 1;
*/
java.lang.String getName();
/**
*
* Semantics depend on contents.
*
*
* string name = 1;
*/
com.google.protobuf.ByteString
getNameBytes();
/**
*
* May be omitted e.g. for fused instructions.
*
*
* .tensorflow.tpu.op_profile.Metrics metrics = 2;
*/
boolean hasMetrics();
/**
*
* May be omitted e.g. for fused instructions.
*
*
* .tensorflow.tpu.op_profile.Metrics metrics = 2;
*/
tensorflow.tpu.op_profile.OpProfile.Metrics getMetrics();
/**
*
* May be omitted e.g. for fused instructions.
*
*
* .tensorflow.tpu.op_profile.Metrics metrics = 2;
*/
tensorflow.tpu.op_profile.OpProfile.MetricsOrBuilder getMetricsOrBuilder();
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
java.util.List
getChildrenList();
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
tensorflow.tpu.op_profile.OpProfile.Node getChildren(int index);
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
int getChildrenCount();
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
java.util.List extends tensorflow.tpu.op_profile.OpProfile.NodeOrBuilder>
getChildrenOrBuilderList();
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
tensorflow.tpu.op_profile.OpProfile.NodeOrBuilder getChildrenOrBuilder(
int index);
/**
* .tensorflow.tpu.op_profile.Node.InstructionCategory category = 4;
*/
boolean hasCategory();
/**
* .tensorflow.tpu.op_profile.Node.InstructionCategory category = 4;
*/
tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory getCategory();
/**
* .tensorflow.tpu.op_profile.Node.InstructionCategory category = 4;
*/
tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategoryOrBuilder getCategoryOrBuilder();
/**
* .tensorflow.tpu.op_profile.Node.XLAInstruction xla = 5;
*/
boolean hasXla();
/**
* .tensorflow.tpu.op_profile.Node.XLAInstruction xla = 5;
*/
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction getXla();
/**
* .tensorflow.tpu.op_profile.Node.XLAInstruction xla = 5;
*/
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstructionOrBuilder getXlaOrBuilder();
/**
*
* Total number of children before pruning.
*
*
* int32 num_children = 6;
*/
int getNumChildren();
public tensorflow.tpu.op_profile.OpProfile.Node.ContentsCase getContentsCase();
}
/**
*
* An entry in the profile tree. (An instruction, or set of instructions).
*
*
* Protobuf type {@code tensorflow.tpu.op_profile.Node}
*/
public static final class Node extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.tpu.op_profile.Node)
NodeOrBuilder {
private static final long serialVersionUID = 0L;
// Use Node.newBuilder() to construct.
private Node(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Node() {
name_ = "";
children_ = java.util.Collections.emptyList();
numChildren_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Node(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 18: {
tensorflow.tpu.op_profile.OpProfile.Metrics.Builder subBuilder = null;
if (metrics_ != null) {
subBuilder = metrics_.toBuilder();
}
metrics_ = input.readMessage(tensorflow.tpu.op_profile.OpProfile.Metrics.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(metrics_);
metrics_ = subBuilder.buildPartial();
}
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
children_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
children_.add(
input.readMessage(tensorflow.tpu.op_profile.OpProfile.Node.parser(), extensionRegistry));
break;
}
case 34: {
tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory.Builder subBuilder = null;
if (contentsCase_ == 4) {
subBuilder = ((tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory) contents_).toBuilder();
}
contents_ =
input.readMessage(tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory) contents_);
contents_ = subBuilder.buildPartial();
}
contentsCase_ = 4;
break;
}
case 42: {
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.Builder subBuilder = null;
if (contentsCase_ == 5) {
subBuilder = ((tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction) contents_).toBuilder();
}
contents_ =
input.readMessage(tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction) contents_);
contents_ = subBuilder.buildPartial();
}
contentsCase_ = 5;
break;
}
case 48: {
numChildren_ = input.readInt32();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
children_ = java.util.Collections.unmodifiableList(children_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Node_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Node_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.tpu.op_profile.OpProfile.Node.class, tensorflow.tpu.op_profile.OpProfile.Node.Builder.class);
}
public interface InstructionCategoryOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.tpu.op_profile.Node.InstructionCategory)
com.google.protobuf.MessageOrBuilder {
}
/**
*
* A category of XLA instructions.
* name is a descriptive string, like "data formatting".
*
*
* Protobuf type {@code tensorflow.tpu.op_profile.Node.InstructionCategory}
*/
public static final class InstructionCategory extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.tpu.op_profile.Node.InstructionCategory)
InstructionCategoryOrBuilder {
private static final long serialVersionUID = 0L;
// Use InstructionCategory.newBuilder() to construct.
private InstructionCategory(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private InstructionCategory() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private InstructionCategory(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Node_InstructionCategory_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Node_InstructionCategory_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory.class, tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory.Builder.class);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory)) {
return super.equals(obj);
}
tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory other = (tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory) obj;
boolean result = true;
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A category of XLA instructions.
* name is a descriptive string, like "data formatting".
*
*
* Protobuf type {@code tensorflow.tpu.op_profile.Node.InstructionCategory}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.tpu.op_profile.Node.InstructionCategory)
tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategoryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Node_InstructionCategory_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Node_InstructionCategory_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory.class, tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory.Builder.class);
}
// Construct using tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Node_InstructionCategory_descriptor;
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory getDefaultInstanceForType() {
return tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory.getDefaultInstance();
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory build() {
tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory buildPartial() {
tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory result = new tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory(this);
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory) {
return mergeFrom((tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory other) {
if (other == tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory.getDefaultInstance()) return this;
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.tpu.op_profile.Node.InstructionCategory)
}
// @@protoc_insertion_point(class_scope:tensorflow.tpu.op_profile.Node.InstructionCategory)
private static final tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory();
}
public static tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public InstructionCategory parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new InstructionCategory(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface XLAInstructionOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.tpu.op_profile.Node.XLAInstruction)
com.google.protobuf.MessageOrBuilder {
/**
*
* Opcode like %multiply
*
*
* string op = 1;
*/
java.lang.String getOp();
/**
*
* Opcode like %multiply
*
*
* string op = 1;
*/
com.google.protobuf.ByteString
getOpBytes();
/**
*
* %multiply = [shape]multiply(operand1, operand2)
*
*
* string expression = 2;
*/
java.lang.String getExpression();
/**
*
* %multiply = [shape]multiply(operand1, operand2)
*
*
* string expression = 2;
*/
com.google.protobuf.ByteString
getExpressionBytes();
/**
*
* Typically the TensorFlow operation name.
*
*
* string provenance = 3;
*/
java.lang.String getProvenance();
/**
*
* Typically the TensorFlow operation name.
*
*
* string provenance = 3;
*/
com.google.protobuf.ByteString
getProvenanceBytes();
/**
* string category = 4;
*/
java.lang.String getCategory();
/**
* string category = 4;
*/
com.google.protobuf.ByteString
getCategoryBytes();
/**
*
* Describes the physical memory layout of the instruction's primary input.
* e.g. for a convolution, this analyzes the image and ignores the kernel.
*
*
* .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis layout = 5;
*/
boolean hasLayout();
/**
*
* Describes the physical memory layout of the instruction's primary input.
* e.g. for a convolution, this analyzes the image and ignores the kernel.
*
*
* .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis layout = 5;
*/
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis getLayout();
/**
*
* Describes the physical memory layout of the instruction's primary input.
* e.g. for a convolution, this analyzes the image and ignores the kernel.
*
*
* .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis layout = 5;
*/
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysisOrBuilder getLayoutOrBuilder();
}
/**
*
* A single XLA instruction.
* name is the unique instruction id, like "%multiply.5".
*
*
* Protobuf type {@code tensorflow.tpu.op_profile.Node.XLAInstruction}
*/
public static final class XLAInstruction extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.tpu.op_profile.Node.XLAInstruction)
XLAInstructionOrBuilder {
private static final long serialVersionUID = 0L;
// Use XLAInstruction.newBuilder() to construct.
private XLAInstruction(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private XLAInstruction() {
op_ = "";
expression_ = "";
provenance_ = "";
category_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private XLAInstruction(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
op_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
expression_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
provenance_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
category_ = s;
break;
}
case 42: {
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Builder subBuilder = null;
if (layout_ != null) {
subBuilder = layout_.toBuilder();
}
layout_ = input.readMessage(tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(layout_);
layout_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.class, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.Builder.class);
}
public interface LayoutAnalysisOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis)
com.google.protobuf.MessageOrBuilder {
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
java.util.List
getDimensionsList();
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension getDimensions(int index);
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
int getDimensionsCount();
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
java.util.List extends tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.DimensionOrBuilder>
getDimensionsOrBuilderList();
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.DimensionOrBuilder getDimensionsOrBuilder(
int index);
}
/**
* Protobuf type {@code tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis}
*/
public static final class LayoutAnalysis extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis)
LayoutAnalysisOrBuilder {
private static final long serialVersionUID = 0L;
// Use LayoutAnalysis.newBuilder() to construct.
private LayoutAnalysis(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LayoutAnalysis() {
dimensions_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LayoutAnalysis(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
dimensions_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
dimensions_.add(
input.readMessage(tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
dimensions_ = java.util.Collections.unmodifiableList(dimensions_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_LayoutAnalysis_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_LayoutAnalysis_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.class, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Builder.class);
}
public interface DimensionOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension)
com.google.protobuf.MessageOrBuilder {
/**
*
* Size of the data in this dimension.
*
*
* int32 size = 1;
*/
int getSize();
/**
*
* Data must be padded to a multiple of alignment.
*
*
* int32 alignment = 2;
*/
int getAlignment();
/**
*
* What the dimension represents, e.g. "spatial".
*
*
* string semantics = 3;
*/
java.lang.String getSemantics();
/**
*
* What the dimension represents, e.g. "spatial".
*
*
* string semantics = 3;
*/
com.google.protobuf.ByteString
getSemanticsBytes();
}
/**
* Protobuf type {@code tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension}
*/
public static final class Dimension extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension)
DimensionOrBuilder {
private static final long serialVersionUID = 0L;
// Use Dimension.newBuilder() to construct.
private Dimension(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Dimension() {
size_ = 0;
alignment_ = 0;
semantics_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Dimension(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
size_ = input.readInt32();
break;
}
case 16: {
alignment_ = input.readInt32();
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
semantics_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_LayoutAnalysis_Dimension_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_LayoutAnalysis_Dimension_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension.class, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension.Builder.class);
}
public static final int SIZE_FIELD_NUMBER = 1;
private int size_;
/**
*
* Size of the data in this dimension.
*
*
* int32 size = 1;
*/
public int getSize() {
return size_;
}
public static final int ALIGNMENT_FIELD_NUMBER = 2;
private int alignment_;
/**
*
* Data must be padded to a multiple of alignment.
*
*
* int32 alignment = 2;
*/
public int getAlignment() {
return alignment_;
}
public static final int SEMANTICS_FIELD_NUMBER = 3;
private volatile java.lang.Object semantics_;
/**
*
* What the dimension represents, e.g. "spatial".
*
*
* string semantics = 3;
*/
public java.lang.String getSemantics() {
java.lang.Object ref = semantics_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
semantics_ = s;
return s;
}
}
/**
*
* What the dimension represents, e.g. "spatial".
*
*
* string semantics = 3;
*/
public com.google.protobuf.ByteString
getSemanticsBytes() {
java.lang.Object ref = semantics_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
semantics_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (size_ != 0) {
output.writeInt32(1, size_);
}
if (alignment_ != 0) {
output.writeInt32(2, alignment_);
}
if (!getSemanticsBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, semantics_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (size_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, size_);
}
if (alignment_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, alignment_);
}
if (!getSemanticsBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, semantics_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension)) {
return super.equals(obj);
}
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension other = (tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension) obj;
boolean result = true;
result = result && (getSize()
== other.getSize());
result = result && (getAlignment()
== other.getAlignment());
result = result && getSemantics()
.equals(other.getSemantics());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + SIZE_FIELD_NUMBER;
hash = (53 * hash) + getSize();
hash = (37 * hash) + ALIGNMENT_FIELD_NUMBER;
hash = (53 * hash) + getAlignment();
hash = (37 * hash) + SEMANTICS_FIELD_NUMBER;
hash = (53 * hash) + getSemantics().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension)
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.DimensionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_LayoutAnalysis_Dimension_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_LayoutAnalysis_Dimension_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension.class, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension.Builder.class);
}
// Construct using tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
size_ = 0;
alignment_ = 0;
semantics_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_LayoutAnalysis_Dimension_descriptor;
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension getDefaultInstanceForType() {
return tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension.getDefaultInstance();
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension build() {
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension buildPartial() {
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension result = new tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension(this);
result.size_ = size_;
result.alignment_ = alignment_;
result.semantics_ = semantics_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension) {
return mergeFrom((tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension other) {
if (other == tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension.getDefaultInstance()) return this;
if (other.getSize() != 0) {
setSize(other.getSize());
}
if (other.getAlignment() != 0) {
setAlignment(other.getAlignment());
}
if (!other.getSemantics().isEmpty()) {
semantics_ = other.semantics_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int size_ ;
/**
*
* Size of the data in this dimension.
*
*
* int32 size = 1;
*/
public int getSize() {
return size_;
}
/**
*
* Size of the data in this dimension.
*
*
* int32 size = 1;
*/
public Builder setSize(int value) {
size_ = value;
onChanged();
return this;
}
/**
*
* Size of the data in this dimension.
*
*
* int32 size = 1;
*/
public Builder clearSize() {
size_ = 0;
onChanged();
return this;
}
private int alignment_ ;
/**
*
* Data must be padded to a multiple of alignment.
*
*
* int32 alignment = 2;
*/
public int getAlignment() {
return alignment_;
}
/**
*
* Data must be padded to a multiple of alignment.
*
*
* int32 alignment = 2;
*/
public Builder setAlignment(int value) {
alignment_ = value;
onChanged();
return this;
}
/**
*
* Data must be padded to a multiple of alignment.
*
*
* int32 alignment = 2;
*/
public Builder clearAlignment() {
alignment_ = 0;
onChanged();
return this;
}
private java.lang.Object semantics_ = "";
/**
*
* What the dimension represents, e.g. "spatial".
*
*
* string semantics = 3;
*/
public java.lang.String getSemantics() {
java.lang.Object ref = semantics_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
semantics_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* What the dimension represents, e.g. "spatial".
*
*
* string semantics = 3;
*/
public com.google.protobuf.ByteString
getSemanticsBytes() {
java.lang.Object ref = semantics_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
semantics_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* What the dimension represents, e.g. "spatial".
*
*
* string semantics = 3;
*/
public Builder setSemantics(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
semantics_ = value;
onChanged();
return this;
}
/**
*
* What the dimension represents, e.g. "spatial".
*
*
* string semantics = 3;
*/
public Builder clearSemantics() {
semantics_ = getDefaultInstance().getSemantics();
onChanged();
return this;
}
/**
*
* What the dimension represents, e.g. "spatial".
*
*
* string semantics = 3;
*/
public Builder setSemanticsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
semantics_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension)
}
// @@protoc_insertion_point(class_scope:tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension)
private static final tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension();
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Dimension parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Dimension(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int DIMENSIONS_FIELD_NUMBER = 1;
private java.util.List dimensions_;
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
public java.util.List getDimensionsList() {
return dimensions_;
}
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
public java.util.List extends tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.DimensionOrBuilder>
getDimensionsOrBuilderList() {
return dimensions_;
}
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
public int getDimensionsCount() {
return dimensions_.size();
}
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension getDimensions(int index) {
return dimensions_.get(index);
}
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.DimensionOrBuilder getDimensionsOrBuilder(
int index) {
return dimensions_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < dimensions_.size(); i++) {
output.writeMessage(1, dimensions_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < dimensions_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, dimensions_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis)) {
return super.equals(obj);
}
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis other = (tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis) obj;
boolean result = true;
result = result && getDimensionsList()
.equals(other.getDimensionsList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getDimensionsCount() > 0) {
hash = (37 * hash) + DIMENSIONS_FIELD_NUMBER;
hash = (53 * hash) + getDimensionsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis)
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysisOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_LayoutAnalysis_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_LayoutAnalysis_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.class, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Builder.class);
}
// Construct using tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getDimensionsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (dimensionsBuilder_ == null) {
dimensions_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
dimensionsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_LayoutAnalysis_descriptor;
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis getDefaultInstanceForType() {
return tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.getDefaultInstance();
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis build() {
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis buildPartial() {
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis result = new tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis(this);
int from_bitField0_ = bitField0_;
if (dimensionsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
dimensions_ = java.util.Collections.unmodifiableList(dimensions_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.dimensions_ = dimensions_;
} else {
result.dimensions_ = dimensionsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis) {
return mergeFrom((tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis other) {
if (other == tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.getDefaultInstance()) return this;
if (dimensionsBuilder_ == null) {
if (!other.dimensions_.isEmpty()) {
if (dimensions_.isEmpty()) {
dimensions_ = other.dimensions_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureDimensionsIsMutable();
dimensions_.addAll(other.dimensions_);
}
onChanged();
}
} else {
if (!other.dimensions_.isEmpty()) {
if (dimensionsBuilder_.isEmpty()) {
dimensionsBuilder_.dispose();
dimensionsBuilder_ = null;
dimensions_ = other.dimensions_;
bitField0_ = (bitField0_ & ~0x00000001);
dimensionsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getDimensionsFieldBuilder() : null;
} else {
dimensionsBuilder_.addAllMessages(other.dimensions_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List dimensions_ =
java.util.Collections.emptyList();
private void ensureDimensionsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
dimensions_ = new java.util.ArrayList(dimensions_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension.Builder, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.DimensionOrBuilder> dimensionsBuilder_;
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
public java.util.List getDimensionsList() {
if (dimensionsBuilder_ == null) {
return java.util.Collections.unmodifiableList(dimensions_);
} else {
return dimensionsBuilder_.getMessageList();
}
}
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
public int getDimensionsCount() {
if (dimensionsBuilder_ == null) {
return dimensions_.size();
} else {
return dimensionsBuilder_.getCount();
}
}
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension getDimensions(int index) {
if (dimensionsBuilder_ == null) {
return dimensions_.get(index);
} else {
return dimensionsBuilder_.getMessage(index);
}
}
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
public Builder setDimensions(
int index, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension value) {
if (dimensionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDimensionsIsMutable();
dimensions_.set(index, value);
onChanged();
} else {
dimensionsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
public Builder setDimensions(
int index, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension.Builder builderForValue) {
if (dimensionsBuilder_ == null) {
ensureDimensionsIsMutable();
dimensions_.set(index, builderForValue.build());
onChanged();
} else {
dimensionsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
public Builder addDimensions(tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension value) {
if (dimensionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDimensionsIsMutable();
dimensions_.add(value);
onChanged();
} else {
dimensionsBuilder_.addMessage(value);
}
return this;
}
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
public Builder addDimensions(
int index, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension value) {
if (dimensionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDimensionsIsMutable();
dimensions_.add(index, value);
onChanged();
} else {
dimensionsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
public Builder addDimensions(
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension.Builder builderForValue) {
if (dimensionsBuilder_ == null) {
ensureDimensionsIsMutable();
dimensions_.add(builderForValue.build());
onChanged();
} else {
dimensionsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
public Builder addDimensions(
int index, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension.Builder builderForValue) {
if (dimensionsBuilder_ == null) {
ensureDimensionsIsMutable();
dimensions_.add(index, builderForValue.build());
onChanged();
} else {
dimensionsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
public Builder addAllDimensions(
java.lang.Iterable extends tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension> values) {
if (dimensionsBuilder_ == null) {
ensureDimensionsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, dimensions_);
onChanged();
} else {
dimensionsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
public Builder clearDimensions() {
if (dimensionsBuilder_ == null) {
dimensions_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
dimensionsBuilder_.clear();
}
return this;
}
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
public Builder removeDimensions(int index) {
if (dimensionsBuilder_ == null) {
ensureDimensionsIsMutable();
dimensions_.remove(index);
onChanged();
} else {
dimensionsBuilder_.remove(index);
}
return this;
}
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension.Builder getDimensionsBuilder(
int index) {
return getDimensionsFieldBuilder().getBuilder(index);
}
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.DimensionOrBuilder getDimensionsOrBuilder(
int index) {
if (dimensionsBuilder_ == null) {
return dimensions_.get(index); } else {
return dimensionsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
public java.util.List extends tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.DimensionOrBuilder>
getDimensionsOrBuilderList() {
if (dimensionsBuilder_ != null) {
return dimensionsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(dimensions_);
}
}
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension.Builder addDimensionsBuilder() {
return getDimensionsFieldBuilder().addBuilder(
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension.getDefaultInstance());
}
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension.Builder addDimensionsBuilder(
int index) {
return getDimensionsFieldBuilder().addBuilder(
index, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension.getDefaultInstance());
}
/**
*
* The physical data layout, from most-minor to most-major dimensions.
*
*
* repeated .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis.Dimension dimensions = 1;
*/
public java.util.List
getDimensionsBuilderList() {
return getDimensionsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension.Builder, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.DimensionOrBuilder>
getDimensionsFieldBuilder() {
if (dimensionsBuilder_ == null) {
dimensionsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Dimension.Builder, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.DimensionOrBuilder>(
dimensions_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
dimensions_ = null;
}
return dimensionsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis)
}
// @@protoc_insertion_point(class_scope:tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis)
private static final tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis();
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LayoutAnalysis parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LayoutAnalysis(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int OP_FIELD_NUMBER = 1;
private volatile java.lang.Object op_;
/**
*
* Opcode like %multiply
*
*
* string op = 1;
*/
public java.lang.String getOp() {
java.lang.Object ref = op_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
op_ = s;
return s;
}
}
/**
*
* Opcode like %multiply
*
*
* string op = 1;
*/
public com.google.protobuf.ByteString
getOpBytes() {
java.lang.Object ref = op_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
op_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int EXPRESSION_FIELD_NUMBER = 2;
private volatile java.lang.Object expression_;
/**
*
* %multiply = [shape]multiply(operand1, operand2)
*
*
* string expression = 2;
*/
public java.lang.String getExpression() {
java.lang.Object ref = expression_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
expression_ = s;
return s;
}
}
/**
*
* %multiply = [shape]multiply(operand1, operand2)
*
*
* string expression = 2;
*/
public com.google.protobuf.ByteString
getExpressionBytes() {
java.lang.Object ref = expression_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
expression_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PROVENANCE_FIELD_NUMBER = 3;
private volatile java.lang.Object provenance_;
/**
*
* Typically the TensorFlow operation name.
*
*
* string provenance = 3;
*/
public java.lang.String getProvenance() {
java.lang.Object ref = provenance_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
provenance_ = s;
return s;
}
}
/**
*
* Typically the TensorFlow operation name.
*
*
* string provenance = 3;
*/
public com.google.protobuf.ByteString
getProvenanceBytes() {
java.lang.Object ref = provenance_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
provenance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CATEGORY_FIELD_NUMBER = 4;
private volatile java.lang.Object category_;
/**
* string category = 4;
*/
public java.lang.String getCategory() {
java.lang.Object ref = category_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
category_ = s;
return s;
}
}
/**
* string category = 4;
*/
public com.google.protobuf.ByteString
getCategoryBytes() {
java.lang.Object ref = category_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
category_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LAYOUT_FIELD_NUMBER = 5;
private tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis layout_;
/**
*
* Describes the physical memory layout of the instruction's primary input.
* e.g. for a convolution, this analyzes the image and ignores the kernel.
*
*
* .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis layout = 5;
*/
public boolean hasLayout() {
return layout_ != null;
}
/**
*
* Describes the physical memory layout of the instruction's primary input.
* e.g. for a convolution, this analyzes the image and ignores the kernel.
*
*
* .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis layout = 5;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis getLayout() {
return layout_ == null ? tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.getDefaultInstance() : layout_;
}
/**
*
* Describes the physical memory layout of the instruction's primary input.
* e.g. for a convolution, this analyzes the image and ignores the kernel.
*
*
* .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis layout = 5;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysisOrBuilder getLayoutOrBuilder() {
return getLayout();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getOpBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, op_);
}
if (!getExpressionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, expression_);
}
if (!getProvenanceBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, provenance_);
}
if (!getCategoryBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, category_);
}
if (layout_ != null) {
output.writeMessage(5, getLayout());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getOpBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, op_);
}
if (!getExpressionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, expression_);
}
if (!getProvenanceBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, provenance_);
}
if (!getCategoryBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, category_);
}
if (layout_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getLayout());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction)) {
return super.equals(obj);
}
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction other = (tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction) obj;
boolean result = true;
result = result && getOp()
.equals(other.getOp());
result = result && getExpression()
.equals(other.getExpression());
result = result && getProvenance()
.equals(other.getProvenance());
result = result && getCategory()
.equals(other.getCategory());
result = result && (hasLayout() == other.hasLayout());
if (hasLayout()) {
result = result && getLayout()
.equals(other.getLayout());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + OP_FIELD_NUMBER;
hash = (53 * hash) + getOp().hashCode();
hash = (37 * hash) + EXPRESSION_FIELD_NUMBER;
hash = (53 * hash) + getExpression().hashCode();
hash = (37 * hash) + PROVENANCE_FIELD_NUMBER;
hash = (53 * hash) + getProvenance().hashCode();
hash = (37 * hash) + CATEGORY_FIELD_NUMBER;
hash = (53 * hash) + getCategory().hashCode();
if (hasLayout()) {
hash = (37 * hash) + LAYOUT_FIELD_NUMBER;
hash = (53 * hash) + getLayout().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A single XLA instruction.
* name is the unique instruction id, like "%multiply.5".
*
*
* Protobuf type {@code tensorflow.tpu.op_profile.Node.XLAInstruction}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.tpu.op_profile.Node.XLAInstruction)
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstructionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.class, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.Builder.class);
}
// Construct using tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
op_ = "";
expression_ = "";
provenance_ = "";
category_ = "";
if (layoutBuilder_ == null) {
layout_ = null;
} else {
layout_ = null;
layoutBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_descriptor;
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction getDefaultInstanceForType() {
return tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.getDefaultInstance();
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction build() {
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction buildPartial() {
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction result = new tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction(this);
result.op_ = op_;
result.expression_ = expression_;
result.provenance_ = provenance_;
result.category_ = category_;
if (layoutBuilder_ == null) {
result.layout_ = layout_;
} else {
result.layout_ = layoutBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction) {
return mergeFrom((tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction other) {
if (other == tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.getDefaultInstance()) return this;
if (!other.getOp().isEmpty()) {
op_ = other.op_;
onChanged();
}
if (!other.getExpression().isEmpty()) {
expression_ = other.expression_;
onChanged();
}
if (!other.getProvenance().isEmpty()) {
provenance_ = other.provenance_;
onChanged();
}
if (!other.getCategory().isEmpty()) {
category_ = other.category_;
onChanged();
}
if (other.hasLayout()) {
mergeLayout(other.getLayout());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object op_ = "";
/**
*
* Opcode like %multiply
*
*
* string op = 1;
*/
public java.lang.String getOp() {
java.lang.Object ref = op_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
op_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Opcode like %multiply
*
*
* string op = 1;
*/
public com.google.protobuf.ByteString
getOpBytes() {
java.lang.Object ref = op_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
op_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Opcode like %multiply
*
*
* string op = 1;
*/
public Builder setOp(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
op_ = value;
onChanged();
return this;
}
/**
*
* Opcode like %multiply
*
*
* string op = 1;
*/
public Builder clearOp() {
op_ = getDefaultInstance().getOp();
onChanged();
return this;
}
/**
*
* Opcode like %multiply
*
*
* string op = 1;
*/
public Builder setOpBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
op_ = value;
onChanged();
return this;
}
private java.lang.Object expression_ = "";
/**
*
* %multiply = [shape]multiply(operand1, operand2)
*
*
* string expression = 2;
*/
public java.lang.String getExpression() {
java.lang.Object ref = expression_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
expression_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* %multiply = [shape]multiply(operand1, operand2)
*
*
* string expression = 2;
*/
public com.google.protobuf.ByteString
getExpressionBytes() {
java.lang.Object ref = expression_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
expression_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* %multiply = [shape]multiply(operand1, operand2)
*
*
* string expression = 2;
*/
public Builder setExpression(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
expression_ = value;
onChanged();
return this;
}
/**
*
* %multiply = [shape]multiply(operand1, operand2)
*
*
* string expression = 2;
*/
public Builder clearExpression() {
expression_ = getDefaultInstance().getExpression();
onChanged();
return this;
}
/**
*
* %multiply = [shape]multiply(operand1, operand2)
*
*
* string expression = 2;
*/
public Builder setExpressionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
expression_ = value;
onChanged();
return this;
}
private java.lang.Object provenance_ = "";
/**
*
* Typically the TensorFlow operation name.
*
*
* string provenance = 3;
*/
public java.lang.String getProvenance() {
java.lang.Object ref = provenance_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
provenance_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Typically the TensorFlow operation name.
*
*
* string provenance = 3;
*/
public com.google.protobuf.ByteString
getProvenanceBytes() {
java.lang.Object ref = provenance_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
provenance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Typically the TensorFlow operation name.
*
*
* string provenance = 3;
*/
public Builder setProvenance(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
provenance_ = value;
onChanged();
return this;
}
/**
*
* Typically the TensorFlow operation name.
*
*
* string provenance = 3;
*/
public Builder clearProvenance() {
provenance_ = getDefaultInstance().getProvenance();
onChanged();
return this;
}
/**
*
* Typically the TensorFlow operation name.
*
*
* string provenance = 3;
*/
public Builder setProvenanceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
provenance_ = value;
onChanged();
return this;
}
private java.lang.Object category_ = "";
/**
* string category = 4;
*/
public java.lang.String getCategory() {
java.lang.Object ref = category_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
category_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string category = 4;
*/
public com.google.protobuf.ByteString
getCategoryBytes() {
java.lang.Object ref = category_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
category_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string category = 4;
*/
public Builder setCategory(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
category_ = value;
onChanged();
return this;
}
/**
* string category = 4;
*/
public Builder clearCategory() {
category_ = getDefaultInstance().getCategory();
onChanged();
return this;
}
/**
* string category = 4;
*/
public Builder setCategoryBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
category_ = value;
onChanged();
return this;
}
private tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis layout_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Builder, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysisOrBuilder> layoutBuilder_;
/**
*
* Describes the physical memory layout of the instruction's primary input.
* e.g. for a convolution, this analyzes the image and ignores the kernel.
*
*
* .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis layout = 5;
*/
public boolean hasLayout() {
return layoutBuilder_ != null || layout_ != null;
}
/**
*
* Describes the physical memory layout of the instruction's primary input.
* e.g. for a convolution, this analyzes the image and ignores the kernel.
*
*
* .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis layout = 5;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis getLayout() {
if (layoutBuilder_ == null) {
return layout_ == null ? tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.getDefaultInstance() : layout_;
} else {
return layoutBuilder_.getMessage();
}
}
/**
*
* Describes the physical memory layout of the instruction's primary input.
* e.g. for a convolution, this analyzes the image and ignores the kernel.
*
*
* .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis layout = 5;
*/
public Builder setLayout(tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis value) {
if (layoutBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
layout_ = value;
onChanged();
} else {
layoutBuilder_.setMessage(value);
}
return this;
}
/**
*
* Describes the physical memory layout of the instruction's primary input.
* e.g. for a convolution, this analyzes the image and ignores the kernel.
*
*
* .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis layout = 5;
*/
public Builder setLayout(
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Builder builderForValue) {
if (layoutBuilder_ == null) {
layout_ = builderForValue.build();
onChanged();
} else {
layoutBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Describes the physical memory layout of the instruction's primary input.
* e.g. for a convolution, this analyzes the image and ignores the kernel.
*
*
* .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis layout = 5;
*/
public Builder mergeLayout(tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis value) {
if (layoutBuilder_ == null) {
if (layout_ != null) {
layout_ =
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.newBuilder(layout_).mergeFrom(value).buildPartial();
} else {
layout_ = value;
}
onChanged();
} else {
layoutBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Describes the physical memory layout of the instruction's primary input.
* e.g. for a convolution, this analyzes the image and ignores the kernel.
*
*
* .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis layout = 5;
*/
public Builder clearLayout() {
if (layoutBuilder_ == null) {
layout_ = null;
onChanged();
} else {
layout_ = null;
layoutBuilder_ = null;
}
return this;
}
/**
*
* Describes the physical memory layout of the instruction's primary input.
* e.g. for a convolution, this analyzes the image and ignores the kernel.
*
*
* .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis layout = 5;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Builder getLayoutBuilder() {
onChanged();
return getLayoutFieldBuilder().getBuilder();
}
/**
*
* Describes the physical memory layout of the instruction's primary input.
* e.g. for a convolution, this analyzes the image and ignores the kernel.
*
*
* .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis layout = 5;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysisOrBuilder getLayoutOrBuilder() {
if (layoutBuilder_ != null) {
return layoutBuilder_.getMessageOrBuilder();
} else {
return layout_ == null ?
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.getDefaultInstance() : layout_;
}
}
/**
*
* Describes the physical memory layout of the instruction's primary input.
* e.g. for a convolution, this analyzes the image and ignores the kernel.
*
*
* .tensorflow.tpu.op_profile.Node.XLAInstruction.LayoutAnalysis layout = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Builder, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysisOrBuilder>
getLayoutFieldBuilder() {
if (layoutBuilder_ == null) {
layoutBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysis.Builder, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.LayoutAnalysisOrBuilder>(
getLayout(),
getParentForChildren(),
isClean());
layout_ = null;
}
return layoutBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.tpu.op_profile.Node.XLAInstruction)
}
// @@protoc_insertion_point(class_scope:tensorflow.tpu.op_profile.Node.XLAInstruction)
private static final tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction();
}
public static tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public XLAInstruction parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new XLAInstruction(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
private int contentsCase_ = 0;
private java.lang.Object contents_;
public enum ContentsCase
implements com.google.protobuf.Internal.EnumLite {
CATEGORY(4),
XLA(5),
CONTENTS_NOT_SET(0);
private final int value;
private ContentsCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ContentsCase valueOf(int value) {
return forNumber(value);
}
public static ContentsCase forNumber(int value) {
switch (value) {
case 4: return CATEGORY;
case 5: return XLA;
case 0: return CONTENTS_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ContentsCase
getContentsCase() {
return ContentsCase.forNumber(
contentsCase_);
}
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
*
* Semantics depend on contents.
*
*
* string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
* Semantics depend on contents.
*
*
* string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int METRICS_FIELD_NUMBER = 2;
private tensorflow.tpu.op_profile.OpProfile.Metrics metrics_;
/**
*
* May be omitted e.g. for fused instructions.
*
*
* .tensorflow.tpu.op_profile.Metrics metrics = 2;
*/
public boolean hasMetrics() {
return metrics_ != null;
}
/**
*
* May be omitted e.g. for fused instructions.
*
*
* .tensorflow.tpu.op_profile.Metrics metrics = 2;
*/
public tensorflow.tpu.op_profile.OpProfile.Metrics getMetrics() {
return metrics_ == null ? tensorflow.tpu.op_profile.OpProfile.Metrics.getDefaultInstance() : metrics_;
}
/**
*
* May be omitted e.g. for fused instructions.
*
*
* .tensorflow.tpu.op_profile.Metrics metrics = 2;
*/
public tensorflow.tpu.op_profile.OpProfile.MetricsOrBuilder getMetricsOrBuilder() {
return getMetrics();
}
public static final int CHILDREN_FIELD_NUMBER = 3;
private java.util.List children_;
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
public java.util.List getChildrenList() {
return children_;
}
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
public java.util.List extends tensorflow.tpu.op_profile.OpProfile.NodeOrBuilder>
getChildrenOrBuilderList() {
return children_;
}
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
public int getChildrenCount() {
return children_.size();
}
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
public tensorflow.tpu.op_profile.OpProfile.Node getChildren(int index) {
return children_.get(index);
}
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
public tensorflow.tpu.op_profile.OpProfile.NodeOrBuilder getChildrenOrBuilder(
int index) {
return children_.get(index);
}
public static final int CATEGORY_FIELD_NUMBER = 4;
/**
* .tensorflow.tpu.op_profile.Node.InstructionCategory category = 4;
*/
public boolean hasCategory() {
return contentsCase_ == 4;
}
/**
* .tensorflow.tpu.op_profile.Node.InstructionCategory category = 4;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory getCategory() {
if (contentsCase_ == 4) {
return (tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory) contents_;
}
return tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory.getDefaultInstance();
}
/**
* .tensorflow.tpu.op_profile.Node.InstructionCategory category = 4;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategoryOrBuilder getCategoryOrBuilder() {
if (contentsCase_ == 4) {
return (tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory) contents_;
}
return tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory.getDefaultInstance();
}
public static final int XLA_FIELD_NUMBER = 5;
/**
* .tensorflow.tpu.op_profile.Node.XLAInstruction xla = 5;
*/
public boolean hasXla() {
return contentsCase_ == 5;
}
/**
* .tensorflow.tpu.op_profile.Node.XLAInstruction xla = 5;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction getXla() {
if (contentsCase_ == 5) {
return (tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction) contents_;
}
return tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.getDefaultInstance();
}
/**
* .tensorflow.tpu.op_profile.Node.XLAInstruction xla = 5;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstructionOrBuilder getXlaOrBuilder() {
if (contentsCase_ == 5) {
return (tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction) contents_;
}
return tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.getDefaultInstance();
}
public static final int NUM_CHILDREN_FIELD_NUMBER = 6;
private int numChildren_;
/**
*
* Total number of children before pruning.
*
*
* int32 num_children = 6;
*/
public int getNumChildren() {
return numChildren_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (metrics_ != null) {
output.writeMessage(2, getMetrics());
}
for (int i = 0; i < children_.size(); i++) {
output.writeMessage(3, children_.get(i));
}
if (contentsCase_ == 4) {
output.writeMessage(4, (tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory) contents_);
}
if (contentsCase_ == 5) {
output.writeMessage(5, (tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction) contents_);
}
if (numChildren_ != 0) {
output.writeInt32(6, numChildren_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (metrics_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getMetrics());
}
for (int i = 0; i < children_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, children_.get(i));
}
if (contentsCase_ == 4) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, (tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory) contents_);
}
if (contentsCase_ == 5) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, (tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction) contents_);
}
if (numChildren_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(6, numChildren_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.tpu.op_profile.OpProfile.Node)) {
return super.equals(obj);
}
tensorflow.tpu.op_profile.OpProfile.Node other = (tensorflow.tpu.op_profile.OpProfile.Node) obj;
boolean result = true;
result = result && getName()
.equals(other.getName());
result = result && (hasMetrics() == other.hasMetrics());
if (hasMetrics()) {
result = result && getMetrics()
.equals(other.getMetrics());
}
result = result && getChildrenList()
.equals(other.getChildrenList());
result = result && (getNumChildren()
== other.getNumChildren());
result = result && getContentsCase().equals(
other.getContentsCase());
if (!result) return false;
switch (contentsCase_) {
case 4:
result = result && getCategory()
.equals(other.getCategory());
break;
case 5:
result = result && getXla()
.equals(other.getXla());
break;
case 0:
default:
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
if (hasMetrics()) {
hash = (37 * hash) + METRICS_FIELD_NUMBER;
hash = (53 * hash) + getMetrics().hashCode();
}
if (getChildrenCount() > 0) {
hash = (37 * hash) + CHILDREN_FIELD_NUMBER;
hash = (53 * hash) + getChildrenList().hashCode();
}
hash = (37 * hash) + NUM_CHILDREN_FIELD_NUMBER;
hash = (53 * hash) + getNumChildren();
switch (contentsCase_) {
case 4:
hash = (37 * hash) + CATEGORY_FIELD_NUMBER;
hash = (53 * hash) + getCategory().hashCode();
break;
case 5:
hash = (37 * hash) + XLA_FIELD_NUMBER;
hash = (53 * hash) + getXla().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.tpu.op_profile.OpProfile.Node parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tpu.op_profile.OpProfile.Node parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Node parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tpu.op_profile.OpProfile.Node parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Node parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tpu.op_profile.OpProfile.Node parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Node parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.tpu.op_profile.OpProfile.Node parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Node parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.tpu.op_profile.OpProfile.Node parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Node parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.tpu.op_profile.OpProfile.Node parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.tpu.op_profile.OpProfile.Node prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* An entry in the profile tree. (An instruction, or set of instructions).
*
*
* Protobuf type {@code tensorflow.tpu.op_profile.Node}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.tpu.op_profile.Node)
tensorflow.tpu.op_profile.OpProfile.NodeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Node_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Node_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.tpu.op_profile.OpProfile.Node.class, tensorflow.tpu.op_profile.OpProfile.Node.Builder.class);
}
// Construct using tensorflow.tpu.op_profile.OpProfile.Node.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getChildrenFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
name_ = "";
if (metricsBuilder_ == null) {
metrics_ = null;
} else {
metrics_ = null;
metricsBuilder_ = null;
}
if (childrenBuilder_ == null) {
children_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
childrenBuilder_.clear();
}
numChildren_ = 0;
contentsCase_ = 0;
contents_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Node_descriptor;
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Node getDefaultInstanceForType() {
return tensorflow.tpu.op_profile.OpProfile.Node.getDefaultInstance();
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Node build() {
tensorflow.tpu.op_profile.OpProfile.Node result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Node buildPartial() {
tensorflow.tpu.op_profile.OpProfile.Node result = new tensorflow.tpu.op_profile.OpProfile.Node(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.name_ = name_;
if (metricsBuilder_ == null) {
result.metrics_ = metrics_;
} else {
result.metrics_ = metricsBuilder_.build();
}
if (childrenBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004)) {
children_ = java.util.Collections.unmodifiableList(children_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.children_ = children_;
} else {
result.children_ = childrenBuilder_.build();
}
if (contentsCase_ == 4) {
if (categoryBuilder_ == null) {
result.contents_ = contents_;
} else {
result.contents_ = categoryBuilder_.build();
}
}
if (contentsCase_ == 5) {
if (xlaBuilder_ == null) {
result.contents_ = contents_;
} else {
result.contents_ = xlaBuilder_.build();
}
}
result.numChildren_ = numChildren_;
result.bitField0_ = to_bitField0_;
result.contentsCase_ = contentsCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.tpu.op_profile.OpProfile.Node) {
return mergeFrom((tensorflow.tpu.op_profile.OpProfile.Node)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.tpu.op_profile.OpProfile.Node other) {
if (other == tensorflow.tpu.op_profile.OpProfile.Node.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (other.hasMetrics()) {
mergeMetrics(other.getMetrics());
}
if (childrenBuilder_ == null) {
if (!other.children_.isEmpty()) {
if (children_.isEmpty()) {
children_ = other.children_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureChildrenIsMutable();
children_.addAll(other.children_);
}
onChanged();
}
} else {
if (!other.children_.isEmpty()) {
if (childrenBuilder_.isEmpty()) {
childrenBuilder_.dispose();
childrenBuilder_ = null;
children_ = other.children_;
bitField0_ = (bitField0_ & ~0x00000004);
childrenBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getChildrenFieldBuilder() : null;
} else {
childrenBuilder_.addAllMessages(other.children_);
}
}
}
if (other.getNumChildren() != 0) {
setNumChildren(other.getNumChildren());
}
switch (other.getContentsCase()) {
case CATEGORY: {
mergeCategory(other.getCategory());
break;
}
case XLA: {
mergeXla(other.getXla());
break;
}
case CONTENTS_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.tpu.op_profile.OpProfile.Node parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.tpu.op_profile.OpProfile.Node) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int contentsCase_ = 0;
private java.lang.Object contents_;
public ContentsCase
getContentsCase() {
return ContentsCase.forNumber(
contentsCase_);
}
public Builder clearContents() {
contentsCase_ = 0;
contents_ = null;
onChanged();
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
*
* Semantics depend on contents.
*
*
* string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Semantics depend on contents.
*
*
* string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Semantics depend on contents.
*
*
* string name = 1;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
*
* Semantics depend on contents.
*
*
* string name = 1;
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
*
* Semantics depend on contents.
*
*
* string name = 1;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private tensorflow.tpu.op_profile.OpProfile.Metrics metrics_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Metrics, tensorflow.tpu.op_profile.OpProfile.Metrics.Builder, tensorflow.tpu.op_profile.OpProfile.MetricsOrBuilder> metricsBuilder_;
/**
*
* May be omitted e.g. for fused instructions.
*
*
* .tensorflow.tpu.op_profile.Metrics metrics = 2;
*/
public boolean hasMetrics() {
return metricsBuilder_ != null || metrics_ != null;
}
/**
*
* May be omitted e.g. for fused instructions.
*
*
* .tensorflow.tpu.op_profile.Metrics metrics = 2;
*/
public tensorflow.tpu.op_profile.OpProfile.Metrics getMetrics() {
if (metricsBuilder_ == null) {
return metrics_ == null ? tensorflow.tpu.op_profile.OpProfile.Metrics.getDefaultInstance() : metrics_;
} else {
return metricsBuilder_.getMessage();
}
}
/**
*
* May be omitted e.g. for fused instructions.
*
*
* .tensorflow.tpu.op_profile.Metrics metrics = 2;
*/
public Builder setMetrics(tensorflow.tpu.op_profile.OpProfile.Metrics value) {
if (metricsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
metrics_ = value;
onChanged();
} else {
metricsBuilder_.setMessage(value);
}
return this;
}
/**
*
* May be omitted e.g. for fused instructions.
*
*
* .tensorflow.tpu.op_profile.Metrics metrics = 2;
*/
public Builder setMetrics(
tensorflow.tpu.op_profile.OpProfile.Metrics.Builder builderForValue) {
if (metricsBuilder_ == null) {
metrics_ = builderForValue.build();
onChanged();
} else {
metricsBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* May be omitted e.g. for fused instructions.
*
*
* .tensorflow.tpu.op_profile.Metrics metrics = 2;
*/
public Builder mergeMetrics(tensorflow.tpu.op_profile.OpProfile.Metrics value) {
if (metricsBuilder_ == null) {
if (metrics_ != null) {
metrics_ =
tensorflow.tpu.op_profile.OpProfile.Metrics.newBuilder(metrics_).mergeFrom(value).buildPartial();
} else {
metrics_ = value;
}
onChanged();
} else {
metricsBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* May be omitted e.g. for fused instructions.
*
*
* .tensorflow.tpu.op_profile.Metrics metrics = 2;
*/
public Builder clearMetrics() {
if (metricsBuilder_ == null) {
metrics_ = null;
onChanged();
} else {
metrics_ = null;
metricsBuilder_ = null;
}
return this;
}
/**
*
* May be omitted e.g. for fused instructions.
*
*
* .tensorflow.tpu.op_profile.Metrics metrics = 2;
*/
public tensorflow.tpu.op_profile.OpProfile.Metrics.Builder getMetricsBuilder() {
onChanged();
return getMetricsFieldBuilder().getBuilder();
}
/**
*
* May be omitted e.g. for fused instructions.
*
*
* .tensorflow.tpu.op_profile.Metrics metrics = 2;
*/
public tensorflow.tpu.op_profile.OpProfile.MetricsOrBuilder getMetricsOrBuilder() {
if (metricsBuilder_ != null) {
return metricsBuilder_.getMessageOrBuilder();
} else {
return metrics_ == null ?
tensorflow.tpu.op_profile.OpProfile.Metrics.getDefaultInstance() : metrics_;
}
}
/**
*
* May be omitted e.g. for fused instructions.
*
*
* .tensorflow.tpu.op_profile.Metrics metrics = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Metrics, tensorflow.tpu.op_profile.OpProfile.Metrics.Builder, tensorflow.tpu.op_profile.OpProfile.MetricsOrBuilder>
getMetricsFieldBuilder() {
if (metricsBuilder_ == null) {
metricsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Metrics, tensorflow.tpu.op_profile.OpProfile.Metrics.Builder, tensorflow.tpu.op_profile.OpProfile.MetricsOrBuilder>(
getMetrics(),
getParentForChildren(),
isClean());
metrics_ = null;
}
return metricsBuilder_;
}
private java.util.List children_ =
java.util.Collections.emptyList();
private void ensureChildrenIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
children_ = new java.util.ArrayList(children_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Node, tensorflow.tpu.op_profile.OpProfile.Node.Builder, tensorflow.tpu.op_profile.OpProfile.NodeOrBuilder> childrenBuilder_;
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
public java.util.List getChildrenList() {
if (childrenBuilder_ == null) {
return java.util.Collections.unmodifiableList(children_);
} else {
return childrenBuilder_.getMessageList();
}
}
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
public int getChildrenCount() {
if (childrenBuilder_ == null) {
return children_.size();
} else {
return childrenBuilder_.getCount();
}
}
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
public tensorflow.tpu.op_profile.OpProfile.Node getChildren(int index) {
if (childrenBuilder_ == null) {
return children_.get(index);
} else {
return childrenBuilder_.getMessage(index);
}
}
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
public Builder setChildren(
int index, tensorflow.tpu.op_profile.OpProfile.Node value) {
if (childrenBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureChildrenIsMutable();
children_.set(index, value);
onChanged();
} else {
childrenBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
public Builder setChildren(
int index, tensorflow.tpu.op_profile.OpProfile.Node.Builder builderForValue) {
if (childrenBuilder_ == null) {
ensureChildrenIsMutable();
children_.set(index, builderForValue.build());
onChanged();
} else {
childrenBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
public Builder addChildren(tensorflow.tpu.op_profile.OpProfile.Node value) {
if (childrenBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureChildrenIsMutable();
children_.add(value);
onChanged();
} else {
childrenBuilder_.addMessage(value);
}
return this;
}
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
public Builder addChildren(
int index, tensorflow.tpu.op_profile.OpProfile.Node value) {
if (childrenBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureChildrenIsMutable();
children_.add(index, value);
onChanged();
} else {
childrenBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
public Builder addChildren(
tensorflow.tpu.op_profile.OpProfile.Node.Builder builderForValue) {
if (childrenBuilder_ == null) {
ensureChildrenIsMutable();
children_.add(builderForValue.build());
onChanged();
} else {
childrenBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
public Builder addChildren(
int index, tensorflow.tpu.op_profile.OpProfile.Node.Builder builderForValue) {
if (childrenBuilder_ == null) {
ensureChildrenIsMutable();
children_.add(index, builderForValue.build());
onChanged();
} else {
childrenBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
public Builder addAllChildren(
java.lang.Iterable extends tensorflow.tpu.op_profile.OpProfile.Node> values) {
if (childrenBuilder_ == null) {
ensureChildrenIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, children_);
onChanged();
} else {
childrenBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
public Builder clearChildren() {
if (childrenBuilder_ == null) {
children_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
childrenBuilder_.clear();
}
return this;
}
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
public Builder removeChildren(int index) {
if (childrenBuilder_ == null) {
ensureChildrenIsMutable();
children_.remove(index);
onChanged();
} else {
childrenBuilder_.remove(index);
}
return this;
}
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.Builder getChildrenBuilder(
int index) {
return getChildrenFieldBuilder().getBuilder(index);
}
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
public tensorflow.tpu.op_profile.OpProfile.NodeOrBuilder getChildrenOrBuilder(
int index) {
if (childrenBuilder_ == null) {
return children_.get(index); } else {
return childrenBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
public java.util.List extends tensorflow.tpu.op_profile.OpProfile.NodeOrBuilder>
getChildrenOrBuilderList() {
if (childrenBuilder_ != null) {
return childrenBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(children_);
}
}
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.Builder addChildrenBuilder() {
return getChildrenFieldBuilder().addBuilder(
tensorflow.tpu.op_profile.OpProfile.Node.getDefaultInstance());
}
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.Builder addChildrenBuilder(
int index) {
return getChildrenFieldBuilder().addBuilder(
index, tensorflow.tpu.op_profile.OpProfile.Node.getDefaultInstance());
}
/**
*
* Subjected to pruning.
*
*
* repeated .tensorflow.tpu.op_profile.Node children = 3;
*/
public java.util.List
getChildrenBuilderList() {
return getChildrenFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Node, tensorflow.tpu.op_profile.OpProfile.Node.Builder, tensorflow.tpu.op_profile.OpProfile.NodeOrBuilder>
getChildrenFieldBuilder() {
if (childrenBuilder_ == null) {
childrenBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Node, tensorflow.tpu.op_profile.OpProfile.Node.Builder, tensorflow.tpu.op_profile.OpProfile.NodeOrBuilder>(
children_,
((bitField0_ & 0x00000004) == 0x00000004),
getParentForChildren(),
isClean());
children_ = null;
}
return childrenBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory, tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory.Builder, tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategoryOrBuilder> categoryBuilder_;
/**
* .tensorflow.tpu.op_profile.Node.InstructionCategory category = 4;
*/
public boolean hasCategory() {
return contentsCase_ == 4;
}
/**
* .tensorflow.tpu.op_profile.Node.InstructionCategory category = 4;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory getCategory() {
if (categoryBuilder_ == null) {
if (contentsCase_ == 4) {
return (tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory) contents_;
}
return tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory.getDefaultInstance();
} else {
if (contentsCase_ == 4) {
return categoryBuilder_.getMessage();
}
return tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory.getDefaultInstance();
}
}
/**
* .tensorflow.tpu.op_profile.Node.InstructionCategory category = 4;
*/
public Builder setCategory(tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory value) {
if (categoryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
contents_ = value;
onChanged();
} else {
categoryBuilder_.setMessage(value);
}
contentsCase_ = 4;
return this;
}
/**
* .tensorflow.tpu.op_profile.Node.InstructionCategory category = 4;
*/
public Builder setCategory(
tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory.Builder builderForValue) {
if (categoryBuilder_ == null) {
contents_ = builderForValue.build();
onChanged();
} else {
categoryBuilder_.setMessage(builderForValue.build());
}
contentsCase_ = 4;
return this;
}
/**
* .tensorflow.tpu.op_profile.Node.InstructionCategory category = 4;
*/
public Builder mergeCategory(tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory value) {
if (categoryBuilder_ == null) {
if (contentsCase_ == 4 &&
contents_ != tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory.getDefaultInstance()) {
contents_ = tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory.newBuilder((tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory) contents_)
.mergeFrom(value).buildPartial();
} else {
contents_ = value;
}
onChanged();
} else {
if (contentsCase_ == 4) {
categoryBuilder_.mergeFrom(value);
}
categoryBuilder_.setMessage(value);
}
contentsCase_ = 4;
return this;
}
/**
* .tensorflow.tpu.op_profile.Node.InstructionCategory category = 4;
*/
public Builder clearCategory() {
if (categoryBuilder_ == null) {
if (contentsCase_ == 4) {
contentsCase_ = 0;
contents_ = null;
onChanged();
}
} else {
if (contentsCase_ == 4) {
contentsCase_ = 0;
contents_ = null;
}
categoryBuilder_.clear();
}
return this;
}
/**
* .tensorflow.tpu.op_profile.Node.InstructionCategory category = 4;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory.Builder getCategoryBuilder() {
return getCategoryFieldBuilder().getBuilder();
}
/**
* .tensorflow.tpu.op_profile.Node.InstructionCategory category = 4;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategoryOrBuilder getCategoryOrBuilder() {
if ((contentsCase_ == 4) && (categoryBuilder_ != null)) {
return categoryBuilder_.getMessageOrBuilder();
} else {
if (contentsCase_ == 4) {
return (tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory) contents_;
}
return tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory.getDefaultInstance();
}
}
/**
* .tensorflow.tpu.op_profile.Node.InstructionCategory category = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory, tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory.Builder, tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategoryOrBuilder>
getCategoryFieldBuilder() {
if (categoryBuilder_ == null) {
if (!(contentsCase_ == 4)) {
contents_ = tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory.getDefaultInstance();
}
categoryBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory, tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory.Builder, tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategoryOrBuilder>(
(tensorflow.tpu.op_profile.OpProfile.Node.InstructionCategory) contents_,
getParentForChildren(),
isClean());
contents_ = null;
}
contentsCase_ = 4;
onChanged();;
return categoryBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.Builder, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstructionOrBuilder> xlaBuilder_;
/**
* .tensorflow.tpu.op_profile.Node.XLAInstruction xla = 5;
*/
public boolean hasXla() {
return contentsCase_ == 5;
}
/**
* .tensorflow.tpu.op_profile.Node.XLAInstruction xla = 5;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction getXla() {
if (xlaBuilder_ == null) {
if (contentsCase_ == 5) {
return (tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction) contents_;
}
return tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.getDefaultInstance();
} else {
if (contentsCase_ == 5) {
return xlaBuilder_.getMessage();
}
return tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.getDefaultInstance();
}
}
/**
* .tensorflow.tpu.op_profile.Node.XLAInstruction xla = 5;
*/
public Builder setXla(tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction value) {
if (xlaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
contents_ = value;
onChanged();
} else {
xlaBuilder_.setMessage(value);
}
contentsCase_ = 5;
return this;
}
/**
* .tensorflow.tpu.op_profile.Node.XLAInstruction xla = 5;
*/
public Builder setXla(
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.Builder builderForValue) {
if (xlaBuilder_ == null) {
contents_ = builderForValue.build();
onChanged();
} else {
xlaBuilder_.setMessage(builderForValue.build());
}
contentsCase_ = 5;
return this;
}
/**
* .tensorflow.tpu.op_profile.Node.XLAInstruction xla = 5;
*/
public Builder mergeXla(tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction value) {
if (xlaBuilder_ == null) {
if (contentsCase_ == 5 &&
contents_ != tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.getDefaultInstance()) {
contents_ = tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.newBuilder((tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction) contents_)
.mergeFrom(value).buildPartial();
} else {
contents_ = value;
}
onChanged();
} else {
if (contentsCase_ == 5) {
xlaBuilder_.mergeFrom(value);
}
xlaBuilder_.setMessage(value);
}
contentsCase_ = 5;
return this;
}
/**
* .tensorflow.tpu.op_profile.Node.XLAInstruction xla = 5;
*/
public Builder clearXla() {
if (xlaBuilder_ == null) {
if (contentsCase_ == 5) {
contentsCase_ = 0;
contents_ = null;
onChanged();
}
} else {
if (contentsCase_ == 5) {
contentsCase_ = 0;
contents_ = null;
}
xlaBuilder_.clear();
}
return this;
}
/**
* .tensorflow.tpu.op_profile.Node.XLAInstruction xla = 5;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.Builder getXlaBuilder() {
return getXlaFieldBuilder().getBuilder();
}
/**
* .tensorflow.tpu.op_profile.Node.XLAInstruction xla = 5;
*/
public tensorflow.tpu.op_profile.OpProfile.Node.XLAInstructionOrBuilder getXlaOrBuilder() {
if ((contentsCase_ == 5) && (xlaBuilder_ != null)) {
return xlaBuilder_.getMessageOrBuilder();
} else {
if (contentsCase_ == 5) {
return (tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction) contents_;
}
return tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.getDefaultInstance();
}
}
/**
* .tensorflow.tpu.op_profile.Node.XLAInstruction xla = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.Builder, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstructionOrBuilder>
getXlaFieldBuilder() {
if (xlaBuilder_ == null) {
if (!(contentsCase_ == 5)) {
contents_ = tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.getDefaultInstance();
}
xlaBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction.Builder, tensorflow.tpu.op_profile.OpProfile.Node.XLAInstructionOrBuilder>(
(tensorflow.tpu.op_profile.OpProfile.Node.XLAInstruction) contents_,
getParentForChildren(),
isClean());
contents_ = null;
}
contentsCase_ = 5;
onChanged();;
return xlaBuilder_;
}
private int numChildren_ ;
/**
*
* Total number of children before pruning.
*
*
* int32 num_children = 6;
*/
public int getNumChildren() {
return numChildren_;
}
/**
*
* Total number of children before pruning.
*
*
* int32 num_children = 6;
*/
public Builder setNumChildren(int value) {
numChildren_ = value;
onChanged();
return this;
}
/**
*
* Total number of children before pruning.
*
*
* int32 num_children = 6;
*/
public Builder clearNumChildren() {
numChildren_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.tpu.op_profile.Node)
}
// @@protoc_insertion_point(class_scope:tensorflow.tpu.op_profile.Node)
private static final tensorflow.tpu.op_profile.OpProfile.Node DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.tpu.op_profile.OpProfile.Node();
}
public static tensorflow.tpu.op_profile.OpProfile.Node getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Node parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Node(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Node getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MetricsOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.tpu.op_profile.Metrics)
com.google.protobuf.MessageOrBuilder {
/**
*
* Core-time taken by this operation, as a fraction of all operations.
*
*
* double time = 1;
*/
double getTime();
/**
*
* Floating point computations performed by this operation, as a fraction of
* peak core FLOPS * program time. This representation has useful properties:
* - it is proportional to the number of floating point operations performed
* - utilization is flops/time
* - wasted potential flops is proportional to time - flops
* - it does not reveal the peak core FLOPS of the hardware
*
*
* double flops = 2;
*/
double getFlops();
/**
*
* The memory bandwidth used to load operands, as a fraction of
* thereotical memory bandwidth on the specific hardware.
*
*
* double memory_bandwidth = 3;
*/
double getMemoryBandwidth();
/**
*
* Elapsed core-time in picoseconds.
*
*
* double raw_time = 11;
*/
double getRawTime();
/**
*
* Total floating-point operations performed.
*
*
* double raw_flops = 12;
*/
double getRawFlops();
/**
*
* Total bytes accessed (include read/write).
*
*
* double raw_bytes_accessed = 13;
*/
double getRawBytesAccessed();
}
/**
*
* Measurements of an operation (or aggregated set of operations).
* Metrics are always "total" rather than "self".
*
*
* Protobuf type {@code tensorflow.tpu.op_profile.Metrics}
*/
public static final class Metrics extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.tpu.op_profile.Metrics)
MetricsOrBuilder {
private static final long serialVersionUID = 0L;
// Use Metrics.newBuilder() to construct.
private Metrics(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Metrics() {
time_ = 0D;
flops_ = 0D;
memoryBandwidth_ = 0D;
rawTime_ = 0D;
rawFlops_ = 0D;
rawBytesAccessed_ = 0D;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Metrics(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 9: {
time_ = input.readDouble();
break;
}
case 17: {
flops_ = input.readDouble();
break;
}
case 25: {
memoryBandwidth_ = input.readDouble();
break;
}
case 89: {
rawTime_ = input.readDouble();
break;
}
case 97: {
rawFlops_ = input.readDouble();
break;
}
case 105: {
rawBytesAccessed_ = input.readDouble();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Metrics_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Metrics_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.tpu.op_profile.OpProfile.Metrics.class, tensorflow.tpu.op_profile.OpProfile.Metrics.Builder.class);
}
public static final int TIME_FIELD_NUMBER = 1;
private double time_;
/**
*
* Core-time taken by this operation, as a fraction of all operations.
*
*
* double time = 1;
*/
public double getTime() {
return time_;
}
public static final int FLOPS_FIELD_NUMBER = 2;
private double flops_;
/**
*
* Floating point computations performed by this operation, as a fraction of
* peak core FLOPS * program time. This representation has useful properties:
* - it is proportional to the number of floating point operations performed
* - utilization is flops/time
* - wasted potential flops is proportional to time - flops
* - it does not reveal the peak core FLOPS of the hardware
*
*
* double flops = 2;
*/
public double getFlops() {
return flops_;
}
public static final int MEMORY_BANDWIDTH_FIELD_NUMBER = 3;
private double memoryBandwidth_;
/**
*
* The memory bandwidth used to load operands, as a fraction of
* thereotical memory bandwidth on the specific hardware.
*
*
* double memory_bandwidth = 3;
*/
public double getMemoryBandwidth() {
return memoryBandwidth_;
}
public static final int RAW_TIME_FIELD_NUMBER = 11;
private double rawTime_;
/**
*
* Elapsed core-time in picoseconds.
*
*
* double raw_time = 11;
*/
public double getRawTime() {
return rawTime_;
}
public static final int RAW_FLOPS_FIELD_NUMBER = 12;
private double rawFlops_;
/**
*
* Total floating-point operations performed.
*
*
* double raw_flops = 12;
*/
public double getRawFlops() {
return rawFlops_;
}
public static final int RAW_BYTES_ACCESSED_FIELD_NUMBER = 13;
private double rawBytesAccessed_;
/**
*
* Total bytes accessed (include read/write).
*
*
* double raw_bytes_accessed = 13;
*/
public double getRawBytesAccessed() {
return rawBytesAccessed_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (time_ != 0D) {
output.writeDouble(1, time_);
}
if (flops_ != 0D) {
output.writeDouble(2, flops_);
}
if (memoryBandwidth_ != 0D) {
output.writeDouble(3, memoryBandwidth_);
}
if (rawTime_ != 0D) {
output.writeDouble(11, rawTime_);
}
if (rawFlops_ != 0D) {
output.writeDouble(12, rawFlops_);
}
if (rawBytesAccessed_ != 0D) {
output.writeDouble(13, rawBytesAccessed_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (time_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(1, time_);
}
if (flops_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(2, flops_);
}
if (memoryBandwidth_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(3, memoryBandwidth_);
}
if (rawTime_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(11, rawTime_);
}
if (rawFlops_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(12, rawFlops_);
}
if (rawBytesAccessed_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(13, rawBytesAccessed_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.tpu.op_profile.OpProfile.Metrics)) {
return super.equals(obj);
}
tensorflow.tpu.op_profile.OpProfile.Metrics other = (tensorflow.tpu.op_profile.OpProfile.Metrics) obj;
boolean result = true;
result = result && (
java.lang.Double.doubleToLongBits(getTime())
== java.lang.Double.doubleToLongBits(
other.getTime()));
result = result && (
java.lang.Double.doubleToLongBits(getFlops())
== java.lang.Double.doubleToLongBits(
other.getFlops()));
result = result && (
java.lang.Double.doubleToLongBits(getMemoryBandwidth())
== java.lang.Double.doubleToLongBits(
other.getMemoryBandwidth()));
result = result && (
java.lang.Double.doubleToLongBits(getRawTime())
== java.lang.Double.doubleToLongBits(
other.getRawTime()));
result = result && (
java.lang.Double.doubleToLongBits(getRawFlops())
== java.lang.Double.doubleToLongBits(
other.getRawFlops()));
result = result && (
java.lang.Double.doubleToLongBits(getRawBytesAccessed())
== java.lang.Double.doubleToLongBits(
other.getRawBytesAccessed()));
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getTime()));
hash = (37 * hash) + FLOPS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getFlops()));
hash = (37 * hash) + MEMORY_BANDWIDTH_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getMemoryBandwidth()));
hash = (37 * hash) + RAW_TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getRawTime()));
hash = (37 * hash) + RAW_FLOPS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getRawFlops()));
hash = (37 * hash) + RAW_BYTES_ACCESSED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getRawBytesAccessed()));
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.tpu.op_profile.OpProfile.Metrics parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tpu.op_profile.OpProfile.Metrics parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Metrics parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tpu.op_profile.OpProfile.Metrics parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Metrics parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.tpu.op_profile.OpProfile.Metrics parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Metrics parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.tpu.op_profile.OpProfile.Metrics parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Metrics parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.tpu.op_profile.OpProfile.Metrics parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.tpu.op_profile.OpProfile.Metrics parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.tpu.op_profile.OpProfile.Metrics parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.tpu.op_profile.OpProfile.Metrics prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Measurements of an operation (or aggregated set of operations).
* Metrics are always "total" rather than "self".
*
*
* Protobuf type {@code tensorflow.tpu.op_profile.Metrics}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.tpu.op_profile.Metrics)
tensorflow.tpu.op_profile.OpProfile.MetricsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Metrics_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Metrics_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.tpu.op_profile.OpProfile.Metrics.class, tensorflow.tpu.op_profile.OpProfile.Metrics.Builder.class);
}
// Construct using tensorflow.tpu.op_profile.OpProfile.Metrics.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
time_ = 0D;
flops_ = 0D;
memoryBandwidth_ = 0D;
rawTime_ = 0D;
rawFlops_ = 0D;
rawBytesAccessed_ = 0D;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.tpu.op_profile.OpProfile.internal_static_tensorflow_tpu_op_profile_Metrics_descriptor;
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Metrics getDefaultInstanceForType() {
return tensorflow.tpu.op_profile.OpProfile.Metrics.getDefaultInstance();
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Metrics build() {
tensorflow.tpu.op_profile.OpProfile.Metrics result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Metrics buildPartial() {
tensorflow.tpu.op_profile.OpProfile.Metrics result = new tensorflow.tpu.op_profile.OpProfile.Metrics(this);
result.time_ = time_;
result.flops_ = flops_;
result.memoryBandwidth_ = memoryBandwidth_;
result.rawTime_ = rawTime_;
result.rawFlops_ = rawFlops_;
result.rawBytesAccessed_ = rawBytesAccessed_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.tpu.op_profile.OpProfile.Metrics) {
return mergeFrom((tensorflow.tpu.op_profile.OpProfile.Metrics)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.tpu.op_profile.OpProfile.Metrics other) {
if (other == tensorflow.tpu.op_profile.OpProfile.Metrics.getDefaultInstance()) return this;
if (other.getTime() != 0D) {
setTime(other.getTime());
}
if (other.getFlops() != 0D) {
setFlops(other.getFlops());
}
if (other.getMemoryBandwidth() != 0D) {
setMemoryBandwidth(other.getMemoryBandwidth());
}
if (other.getRawTime() != 0D) {
setRawTime(other.getRawTime());
}
if (other.getRawFlops() != 0D) {
setRawFlops(other.getRawFlops());
}
if (other.getRawBytesAccessed() != 0D) {
setRawBytesAccessed(other.getRawBytesAccessed());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.tpu.op_profile.OpProfile.Metrics parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.tpu.op_profile.OpProfile.Metrics) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private double time_ ;
/**
*
* Core-time taken by this operation, as a fraction of all operations.
*
*
* double time = 1;
*/
public double getTime() {
return time_;
}
/**
*
* Core-time taken by this operation, as a fraction of all operations.
*
*
* double time = 1;
*/
public Builder setTime(double value) {
time_ = value;
onChanged();
return this;
}
/**
*
* Core-time taken by this operation, as a fraction of all operations.
*
*
* double time = 1;
*/
public Builder clearTime() {
time_ = 0D;
onChanged();
return this;
}
private double flops_ ;
/**
*
* Floating point computations performed by this operation, as a fraction of
* peak core FLOPS * program time. This representation has useful properties:
* - it is proportional to the number of floating point operations performed
* - utilization is flops/time
* - wasted potential flops is proportional to time - flops
* - it does not reveal the peak core FLOPS of the hardware
*
*
* double flops = 2;
*/
public double getFlops() {
return flops_;
}
/**
*
* Floating point computations performed by this operation, as a fraction of
* peak core FLOPS * program time. This representation has useful properties:
* - it is proportional to the number of floating point operations performed
* - utilization is flops/time
* - wasted potential flops is proportional to time - flops
* - it does not reveal the peak core FLOPS of the hardware
*
*
* double flops = 2;
*/
public Builder setFlops(double value) {
flops_ = value;
onChanged();
return this;
}
/**
*
* Floating point computations performed by this operation, as a fraction of
* peak core FLOPS * program time. This representation has useful properties:
* - it is proportional to the number of floating point operations performed
* - utilization is flops/time
* - wasted potential flops is proportional to time - flops
* - it does not reveal the peak core FLOPS of the hardware
*
*
* double flops = 2;
*/
public Builder clearFlops() {
flops_ = 0D;
onChanged();
return this;
}
private double memoryBandwidth_ ;
/**
*
* The memory bandwidth used to load operands, as a fraction of
* thereotical memory bandwidth on the specific hardware.
*
*
* double memory_bandwidth = 3;
*/
public double getMemoryBandwidth() {
return memoryBandwidth_;
}
/**
*
* The memory bandwidth used to load operands, as a fraction of
* thereotical memory bandwidth on the specific hardware.
*
*
* double memory_bandwidth = 3;
*/
public Builder setMemoryBandwidth(double value) {
memoryBandwidth_ = value;
onChanged();
return this;
}
/**
*
* The memory bandwidth used to load operands, as a fraction of
* thereotical memory bandwidth on the specific hardware.
*
*
* double memory_bandwidth = 3;
*/
public Builder clearMemoryBandwidth() {
memoryBandwidth_ = 0D;
onChanged();
return this;
}
private double rawTime_ ;
/**
*
* Elapsed core-time in picoseconds.
*
*
* double raw_time = 11;
*/
public double getRawTime() {
return rawTime_;
}
/**
*
* Elapsed core-time in picoseconds.
*
*
* double raw_time = 11;
*/
public Builder setRawTime(double value) {
rawTime_ = value;
onChanged();
return this;
}
/**
*
* Elapsed core-time in picoseconds.
*
*
* double raw_time = 11;
*/
public Builder clearRawTime() {
rawTime_ = 0D;
onChanged();
return this;
}
private double rawFlops_ ;
/**
*
* Total floating-point operations performed.
*
*
* double raw_flops = 12;
*/
public double getRawFlops() {
return rawFlops_;
}
/**
*
* Total floating-point operations performed.
*
*
* double raw_flops = 12;
*/
public Builder setRawFlops(double value) {
rawFlops_ = value;
onChanged();
return this;
}
/**
*
* Total floating-point operations performed.
*
*
* double raw_flops = 12;
*/
public Builder clearRawFlops() {
rawFlops_ = 0D;
onChanged();
return this;
}
private double rawBytesAccessed_ ;
/**
*
* Total bytes accessed (include read/write).
*
*
* double raw_bytes_accessed = 13;
*/
public double getRawBytesAccessed() {
return rawBytesAccessed_;
}
/**
*
* Total bytes accessed (include read/write).
*
*
* double raw_bytes_accessed = 13;
*/
public Builder setRawBytesAccessed(double value) {
rawBytesAccessed_ = value;
onChanged();
return this;
}
/**
*
* Total bytes accessed (include read/write).
*
*
* double raw_bytes_accessed = 13;
*/
public Builder clearRawBytesAccessed() {
rawBytesAccessed_ = 0D;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.tpu.op_profile.Metrics)
}
// @@protoc_insertion_point(class_scope:tensorflow.tpu.op_profile.Metrics)
private static final tensorflow.tpu.op_profile.OpProfile.Metrics DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.tpu.op_profile.OpProfile.Metrics();
}
public static tensorflow.tpu.op_profile.OpProfile.Metrics getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Metrics parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Metrics(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public tensorflow.tpu.op_profile.OpProfile.Metrics getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_tpu_op_profile_Profile_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_tpu_op_profile_Profile_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_tpu_op_profile_Node_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_tpu_op_profile_Node_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_tpu_op_profile_Node_InstructionCategory_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_tpu_op_profile_Node_InstructionCategory_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_LayoutAnalysis_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_LayoutAnalysis_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_LayoutAnalysis_Dimension_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_LayoutAnalysis_Dimension_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_tpu_op_profile_Metrics_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_tpu_op_profile_Metrics_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n0tensorflow/contrib/tpu/profiler/op_pro" +
"file.proto\022\031tensorflow.tpu.op_profile\"\243\001" +
"\n\007Profile\0224\n\013by_category\030\001 \001(\0132\037.tensorf" +
"low.tpu.op_profile.Node\0223\n\nby_program\030\004 " +
"\001(\0132\037.tensorflow.tpu.op_profile.NodeJ\004\010\002" +
"\020\003J\004\010\003\020\004R\024by_program_structureR\013per_prog" +
"ram\"\226\005\n\004Node\022\014\n\004name\030\001 \001(\t\0223\n\007metrics\030\002 " +
"\001(\0132\".tensorflow.tpu.op_profile.Metrics\022" +
"1\n\010children\030\003 \003(\0132\037.tensorflow.tpu.op_pr" +
"ofile.Node\022G\n\010category\030\004 \001(\01323.tensorflo" +
"w.tpu.op_profile.Node.InstructionCategor" +
"yH\000\022=\n\003xla\030\005 \001(\0132..tensorflow.tpu.op_pro" +
"file.Node.XLAInstructionH\000\022\024\n\014num_childr" +
"en\030\006 \001(\005\032\025\n\023InstructionCategory\032\326\002\n\016XLAI" +
"nstruction\022\n\n\002op\030\001 \001(\t\022\022\n\nexpression\030\002 \001" +
"(\t\022\022\n\nprovenance\030\003 \001(\t\022\020\n\010category\030\004 \001(\t" +
"\022M\n\006layout\030\005 \001(\0132=.tensorflow.tpu.op_pro" +
"file.Node.XLAInstruction.LayoutAnalysis\032" +
"\256\001\n\016LayoutAnalysis\022[\n\ndimensions\030\001 \003(\0132G" +
".tensorflow.tpu.op_profile.Node.XLAInstr" +
"uction.LayoutAnalysis.Dimension\032?\n\tDimen" +
"sion\022\014\n\004size\030\001 \001(\005\022\021\n\talignment\030\002 \001(\005\022\021\n" +
"\tsemantics\030\003 \001(\tB\n\n\010contents\"\201\001\n\007Metrics" +
"\022\014\n\004time\030\001 \001(\001\022\r\n\005flops\030\002 \001(\001\022\030\n\020memory_" +
"bandwidth\030\003 \001(\001\022\020\n\010raw_time\030\013 \001(\001\022\021\n\traw" +
"_flops\030\014 \001(\001\022\032\n\022raw_bytes_accessed\030\r \001(\001" +
"b\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
internal_static_tensorflow_tpu_op_profile_Profile_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_tensorflow_tpu_op_profile_Profile_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_tpu_op_profile_Profile_descriptor,
new java.lang.String[] { "ByCategory", "ByProgram", });
internal_static_tensorflow_tpu_op_profile_Node_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_tensorflow_tpu_op_profile_Node_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_tpu_op_profile_Node_descriptor,
new java.lang.String[] { "Name", "Metrics", "Children", "Category", "Xla", "NumChildren", "Contents", });
internal_static_tensorflow_tpu_op_profile_Node_InstructionCategory_descriptor =
internal_static_tensorflow_tpu_op_profile_Node_descriptor.getNestedTypes().get(0);
internal_static_tensorflow_tpu_op_profile_Node_InstructionCategory_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_tpu_op_profile_Node_InstructionCategory_descriptor,
new java.lang.String[] { });
internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_descriptor =
internal_static_tensorflow_tpu_op_profile_Node_descriptor.getNestedTypes().get(1);
internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_descriptor,
new java.lang.String[] { "Op", "Expression", "Provenance", "Category", "Layout", });
internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_LayoutAnalysis_descriptor =
internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_descriptor.getNestedTypes().get(0);
internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_LayoutAnalysis_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_LayoutAnalysis_descriptor,
new java.lang.String[] { "Dimensions", });
internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_LayoutAnalysis_Dimension_descriptor =
internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_LayoutAnalysis_descriptor.getNestedTypes().get(0);
internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_LayoutAnalysis_Dimension_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_tpu_op_profile_Node_XLAInstruction_LayoutAnalysis_Dimension_descriptor,
new java.lang.String[] { "Size", "Alignment", "Semantics", });
internal_static_tensorflow_tpu_op_profile_Metrics_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_tensorflow_tpu_op_profile_Metrics_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_tpu_op_profile_Metrics_descriptor,
new java.lang.String[] { "Time", "Flops", "MemoryBandwidth", "RawTime", "RawFlops", "RawBytesAccessed", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy