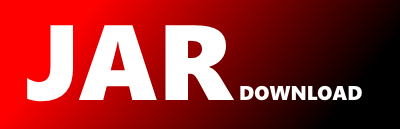
xla.XlaServiceGrpc Maven / Gradle / Ivy
The newest version!
package xla;
import static io.grpc.MethodDescriptor.generateFullMethodName;
import static io.grpc.stub.ClientCalls.asyncBidiStreamingCall;
import static io.grpc.stub.ClientCalls.asyncClientStreamingCall;
import static io.grpc.stub.ClientCalls.asyncServerStreamingCall;
import static io.grpc.stub.ClientCalls.asyncUnaryCall;
import static io.grpc.stub.ClientCalls.blockingServerStreamingCall;
import static io.grpc.stub.ClientCalls.blockingUnaryCall;
import static io.grpc.stub.ClientCalls.futureUnaryCall;
import static io.grpc.stub.ServerCalls.asyncBidiStreamingCall;
import static io.grpc.stub.ServerCalls.asyncClientStreamingCall;
import static io.grpc.stub.ServerCalls.asyncServerStreamingCall;
import static io.grpc.stub.ServerCalls.asyncUnaryCall;
import static io.grpc.stub.ServerCalls.asyncUnimplementedStreamingCall;
import static io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall;
/**
*
*///////////////////////
* Global data requests
*
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.18.0)",
comments = "Source: tensorflow/compiler/xla/rpc/xla_service.proto")
public final class XlaServiceGrpc {
private XlaServiceGrpc() {}
public static final String SERVICE_NAME = "xla.XlaService";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getUnregisterMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Unregister",
requestType = xla.Xla.UnregisterRequest.class,
responseType = xla.Xla.UnregisterResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getUnregisterMethod() {
io.grpc.MethodDescriptor getUnregisterMethod;
if ((getUnregisterMethod = XlaServiceGrpc.getUnregisterMethod) == null) {
synchronized (XlaServiceGrpc.class) {
if ((getUnregisterMethod = XlaServiceGrpc.getUnregisterMethod) == null) {
XlaServiceGrpc.getUnregisterMethod = getUnregisterMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"xla.XlaService", "Unregister"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.UnregisterRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.UnregisterResponse.getDefaultInstance()))
.setSchemaDescriptor(new XlaServiceMethodDescriptorSupplier("Unregister"))
.build();
}
}
}
return getUnregisterMethod;
}
private static volatile io.grpc.MethodDescriptor getDeconstructTupleMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "DeconstructTuple",
requestType = xla.Xla.DeconstructTupleRequest.class,
responseType = xla.Xla.DeconstructTupleResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getDeconstructTupleMethod() {
io.grpc.MethodDescriptor getDeconstructTupleMethod;
if ((getDeconstructTupleMethod = XlaServiceGrpc.getDeconstructTupleMethod) == null) {
synchronized (XlaServiceGrpc.class) {
if ((getDeconstructTupleMethod = XlaServiceGrpc.getDeconstructTupleMethod) == null) {
XlaServiceGrpc.getDeconstructTupleMethod = getDeconstructTupleMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"xla.XlaService", "DeconstructTuple"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.DeconstructTupleRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.DeconstructTupleResponse.getDefaultInstance()))
.setSchemaDescriptor(new XlaServiceMethodDescriptorSupplier("DeconstructTuple"))
.build();
}
}
}
return getDeconstructTupleMethod;
}
private static volatile io.grpc.MethodDescriptor getUnpackMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Unpack",
requestType = xla.Xla.UnpackRequest.class,
responseType = xla.Xla.UnpackResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getUnpackMethod() {
io.grpc.MethodDescriptor getUnpackMethod;
if ((getUnpackMethod = XlaServiceGrpc.getUnpackMethod) == null) {
synchronized (XlaServiceGrpc.class) {
if ((getUnpackMethod = XlaServiceGrpc.getUnpackMethod) == null) {
XlaServiceGrpc.getUnpackMethod = getUnpackMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"xla.XlaService", "Unpack"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.UnpackRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.UnpackResponse.getDefaultInstance()))
.setSchemaDescriptor(new XlaServiceMethodDescriptorSupplier("Unpack"))
.build();
}
}
}
return getUnpackMethod;
}
private static volatile io.grpc.MethodDescriptor getGetShapeMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetShape",
requestType = xla.Xla.GetShapeRequest.class,
responseType = xla.Xla.GetShapeResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetShapeMethod() {
io.grpc.MethodDescriptor getGetShapeMethod;
if ((getGetShapeMethod = XlaServiceGrpc.getGetShapeMethod) == null) {
synchronized (XlaServiceGrpc.class) {
if ((getGetShapeMethod = XlaServiceGrpc.getGetShapeMethod) == null) {
XlaServiceGrpc.getGetShapeMethod = getGetShapeMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"xla.XlaService", "GetShape"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.GetShapeRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.GetShapeResponse.getDefaultInstance()))
.setSchemaDescriptor(new XlaServiceMethodDescriptorSupplier("GetShape"))
.build();
}
}
}
return getGetShapeMethod;
}
private static volatile io.grpc.MethodDescriptor getGetComputationGraphStatsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetComputationGraphStats",
requestType = xla.Xla.ComputationGraphStatsRequest.class,
responseType = xla.Xla.ComputationStatsResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetComputationGraphStatsMethod() {
io.grpc.MethodDescriptor getGetComputationGraphStatsMethod;
if ((getGetComputationGraphStatsMethod = XlaServiceGrpc.getGetComputationGraphStatsMethod) == null) {
synchronized (XlaServiceGrpc.class) {
if ((getGetComputationGraphStatsMethod = XlaServiceGrpc.getGetComputationGraphStatsMethod) == null) {
XlaServiceGrpc.getGetComputationGraphStatsMethod = getGetComputationGraphStatsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"xla.XlaService", "GetComputationGraphStats"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.ComputationGraphStatsRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.ComputationStatsResponse.getDefaultInstance()))
.setSchemaDescriptor(new XlaServiceMethodDescriptorSupplier("GetComputationGraphStats"))
.build();
}
}
}
return getGetComputationGraphStatsMethod;
}
private static volatile io.grpc.MethodDescriptor getLoadDataMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "LoadData",
requestType = xla.Xla.LoadDataRequest.class,
responseType = xla.Xla.LoadDataResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getLoadDataMethod() {
io.grpc.MethodDescriptor getLoadDataMethod;
if ((getLoadDataMethod = XlaServiceGrpc.getLoadDataMethod) == null) {
synchronized (XlaServiceGrpc.class) {
if ((getLoadDataMethod = XlaServiceGrpc.getLoadDataMethod) == null) {
XlaServiceGrpc.getLoadDataMethod = getLoadDataMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"xla.XlaService", "LoadData"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.LoadDataRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.LoadDataResponse.getDefaultInstance()))
.setSchemaDescriptor(new XlaServiceMethodDescriptorSupplier("LoadData"))
.build();
}
}
}
return getLoadDataMethod;
}
private static volatile io.grpc.MethodDescriptor getTransferToClientMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "TransferToClient",
requestType = xla.Xla.TransferToClientRequest.class,
responseType = xla.Xla.TransferToClientResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getTransferToClientMethod() {
io.grpc.MethodDescriptor getTransferToClientMethod;
if ((getTransferToClientMethod = XlaServiceGrpc.getTransferToClientMethod) == null) {
synchronized (XlaServiceGrpc.class) {
if ((getTransferToClientMethod = XlaServiceGrpc.getTransferToClientMethod) == null) {
XlaServiceGrpc.getTransferToClientMethod = getTransferToClientMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"xla.XlaService", "TransferToClient"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.TransferToClientRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.TransferToClientResponse.getDefaultInstance()))
.setSchemaDescriptor(new XlaServiceMethodDescriptorSupplier("TransferToClient"))
.build();
}
}
}
return getTransferToClientMethod;
}
private static volatile io.grpc.MethodDescriptor getTransferToServerMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "TransferToServer",
requestType = xla.Xla.TransferToServerRequest.class,
responseType = xla.Xla.TransferToServerResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getTransferToServerMethod() {
io.grpc.MethodDescriptor getTransferToServerMethod;
if ((getTransferToServerMethod = XlaServiceGrpc.getTransferToServerMethod) == null) {
synchronized (XlaServiceGrpc.class) {
if ((getTransferToServerMethod = XlaServiceGrpc.getTransferToServerMethod) == null) {
XlaServiceGrpc.getTransferToServerMethod = getTransferToServerMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"xla.XlaService", "TransferToServer"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.TransferToServerRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.TransferToServerResponse.getDefaultInstance()))
.setSchemaDescriptor(new XlaServiceMethodDescriptorSupplier("TransferToServer"))
.build();
}
}
}
return getTransferToServerMethod;
}
private static volatile io.grpc.MethodDescriptor getTransferToInfeedMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "TransferToInfeed",
requestType = xla.Xla.TransferToInfeedRequest.class,
responseType = xla.Xla.TransferToInfeedResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getTransferToInfeedMethod() {
io.grpc.MethodDescriptor getTransferToInfeedMethod;
if ((getTransferToInfeedMethod = XlaServiceGrpc.getTransferToInfeedMethod) == null) {
synchronized (XlaServiceGrpc.class) {
if ((getTransferToInfeedMethod = XlaServiceGrpc.getTransferToInfeedMethod) == null) {
XlaServiceGrpc.getTransferToInfeedMethod = getTransferToInfeedMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"xla.XlaService", "TransferToInfeed"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.TransferToInfeedRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.TransferToInfeedResponse.getDefaultInstance()))
.setSchemaDescriptor(new XlaServiceMethodDescriptorSupplier("TransferToInfeed"))
.build();
}
}
}
return getTransferToInfeedMethod;
}
private static volatile io.grpc.MethodDescriptor getTransferFromOutfeedMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "TransferFromOutfeed",
requestType = xla.Xla.TransferFromOutfeedRequest.class,
responseType = xla.Xla.TransferFromOutfeedResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getTransferFromOutfeedMethod() {
io.grpc.MethodDescriptor getTransferFromOutfeedMethod;
if ((getTransferFromOutfeedMethod = XlaServiceGrpc.getTransferFromOutfeedMethod) == null) {
synchronized (XlaServiceGrpc.class) {
if ((getTransferFromOutfeedMethod = XlaServiceGrpc.getTransferFromOutfeedMethod) == null) {
XlaServiceGrpc.getTransferFromOutfeedMethod = getTransferFromOutfeedMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"xla.XlaService", "TransferFromOutfeed"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.TransferFromOutfeedRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.TransferFromOutfeedResponse.getDefaultInstance()))
.setSchemaDescriptor(new XlaServiceMethodDescriptorSupplier("TransferFromOutfeed"))
.build();
}
}
}
return getTransferFromOutfeedMethod;
}
private static volatile io.grpc.MethodDescriptor getResetDeviceMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ResetDevice",
requestType = xla.Xla.ResetDeviceRequest.class,
responseType = xla.Xla.ResetDeviceResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getResetDeviceMethod() {
io.grpc.MethodDescriptor getResetDeviceMethod;
if ((getResetDeviceMethod = XlaServiceGrpc.getResetDeviceMethod) == null) {
synchronized (XlaServiceGrpc.class) {
if ((getResetDeviceMethod = XlaServiceGrpc.getResetDeviceMethod) == null) {
XlaServiceGrpc.getResetDeviceMethod = getResetDeviceMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"xla.XlaService", "ResetDevice"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.ResetDeviceRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.ResetDeviceResponse.getDefaultInstance()))
.setSchemaDescriptor(new XlaServiceMethodDescriptorSupplier("ResetDevice"))
.build();
}
}
}
return getResetDeviceMethod;
}
private static volatile io.grpc.MethodDescriptor getComputeConstantGraphMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ComputeConstantGraph",
requestType = xla.Xla.ComputeConstantGraphRequest.class,
responseType = xla.Xla.ComputeConstantResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getComputeConstantGraphMethod() {
io.grpc.MethodDescriptor getComputeConstantGraphMethod;
if ((getComputeConstantGraphMethod = XlaServiceGrpc.getComputeConstantGraphMethod) == null) {
synchronized (XlaServiceGrpc.class) {
if ((getComputeConstantGraphMethod = XlaServiceGrpc.getComputeConstantGraphMethod) == null) {
XlaServiceGrpc.getComputeConstantGraphMethod = getComputeConstantGraphMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"xla.XlaService", "ComputeConstantGraph"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.ComputeConstantGraphRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.ComputeConstantResponse.getDefaultInstance()))
.setSchemaDescriptor(new XlaServiceMethodDescriptorSupplier("ComputeConstantGraph"))
.build();
}
}
}
return getComputeConstantGraphMethod;
}
private static volatile io.grpc.MethodDescriptor getGetDeviceHandlesMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetDeviceHandles",
requestType = xla.Xla.GetDeviceHandlesRequest.class,
responseType = xla.Xla.GetDeviceHandlesResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetDeviceHandlesMethod() {
io.grpc.MethodDescriptor getGetDeviceHandlesMethod;
if ((getGetDeviceHandlesMethod = XlaServiceGrpc.getGetDeviceHandlesMethod) == null) {
synchronized (XlaServiceGrpc.class) {
if ((getGetDeviceHandlesMethod = XlaServiceGrpc.getGetDeviceHandlesMethod) == null) {
XlaServiceGrpc.getGetDeviceHandlesMethod = getGetDeviceHandlesMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"xla.XlaService", "GetDeviceHandles"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.GetDeviceHandlesRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.GetDeviceHandlesResponse.getDefaultInstance()))
.setSchemaDescriptor(new XlaServiceMethodDescriptorSupplier("GetDeviceHandles"))
.build();
}
}
}
return getGetDeviceHandlesMethod;
}
private static volatile io.grpc.MethodDescriptor getCreateChannelHandleMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "CreateChannelHandle",
requestType = xla.Xla.CreateChannelHandleRequest.class,
responseType = xla.Xla.CreateChannelHandleResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getCreateChannelHandleMethod() {
io.grpc.MethodDescriptor getCreateChannelHandleMethod;
if ((getCreateChannelHandleMethod = XlaServiceGrpc.getCreateChannelHandleMethod) == null) {
synchronized (XlaServiceGrpc.class) {
if ((getCreateChannelHandleMethod = XlaServiceGrpc.getCreateChannelHandleMethod) == null) {
XlaServiceGrpc.getCreateChannelHandleMethod = getCreateChannelHandleMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"xla.XlaService", "CreateChannelHandle"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.CreateChannelHandleRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.CreateChannelHandleResponse.getDefaultInstance()))
.setSchemaDescriptor(new XlaServiceMethodDescriptorSupplier("CreateChannelHandle"))
.build();
}
}
}
return getCreateChannelHandleMethod;
}
private static volatile io.grpc.MethodDescriptor getExecuteGraphMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ExecuteGraph",
requestType = xla.Xla.ExecuteGraphRequest.class,
responseType = xla.Xla.ExecuteResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getExecuteGraphMethod() {
io.grpc.MethodDescriptor getExecuteGraphMethod;
if ((getExecuteGraphMethod = XlaServiceGrpc.getExecuteGraphMethod) == null) {
synchronized (XlaServiceGrpc.class) {
if ((getExecuteGraphMethod = XlaServiceGrpc.getExecuteGraphMethod) == null) {
XlaServiceGrpc.getExecuteGraphMethod = getExecuteGraphMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"xla.XlaService", "ExecuteGraph"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.ExecuteGraphRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.ExecuteResponse.getDefaultInstance()))
.setSchemaDescriptor(new XlaServiceMethodDescriptorSupplier("ExecuteGraph"))
.build();
}
}
}
return getExecuteGraphMethod;
}
private static volatile io.grpc.MethodDescriptor getExecuteGraphParallelMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ExecuteGraphParallel",
requestType = xla.Xla.ExecuteGraphParallelRequest.class,
responseType = xla.Xla.ExecuteParallelResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getExecuteGraphParallelMethod() {
io.grpc.MethodDescriptor getExecuteGraphParallelMethod;
if ((getExecuteGraphParallelMethod = XlaServiceGrpc.getExecuteGraphParallelMethod) == null) {
synchronized (XlaServiceGrpc.class) {
if ((getExecuteGraphParallelMethod = XlaServiceGrpc.getExecuteGraphParallelMethod) == null) {
XlaServiceGrpc.getExecuteGraphParallelMethod = getExecuteGraphParallelMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"xla.XlaService", "ExecuteGraphParallel"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.ExecuteGraphParallelRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.ExecuteParallelResponse.getDefaultInstance()))
.setSchemaDescriptor(new XlaServiceMethodDescriptorSupplier("ExecuteGraphParallel"))
.build();
}
}
}
return getExecuteGraphParallelMethod;
}
private static volatile io.grpc.MethodDescriptor getWaitForExecutionMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "WaitForExecution",
requestType = xla.Xla.WaitForExecutionRequest.class,
responseType = xla.Xla.WaitForExecutionResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getWaitForExecutionMethod() {
io.grpc.MethodDescriptor getWaitForExecutionMethod;
if ((getWaitForExecutionMethod = XlaServiceGrpc.getWaitForExecutionMethod) == null) {
synchronized (XlaServiceGrpc.class) {
if ((getWaitForExecutionMethod = XlaServiceGrpc.getWaitForExecutionMethod) == null) {
XlaServiceGrpc.getWaitForExecutionMethod = getWaitForExecutionMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"xla.XlaService", "WaitForExecution"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.WaitForExecutionRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
xla.Xla.WaitForExecutionResponse.getDefaultInstance()))
.setSchemaDescriptor(new XlaServiceMethodDescriptorSupplier("WaitForExecution"))
.build();
}
}
}
return getWaitForExecutionMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static XlaServiceStub newStub(io.grpc.Channel channel) {
return new XlaServiceStub(channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static XlaServiceBlockingStub newBlockingStub(
io.grpc.Channel channel) {
return new XlaServiceBlockingStub(channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static XlaServiceFutureStub newFutureStub(
io.grpc.Channel channel) {
return new XlaServiceFutureStub(channel);
}
/**
*
*///////////////////////
* Global data requests
*
*/
public static abstract class XlaServiceImplBase implements io.grpc.BindableService {
/**
*
* Unregisters a global allocation.
* If the handle given is not currently allocated, a NOT_FOUND status is
* returned.
*
*/
public void unregister(xla.Xla.UnregisterRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getUnregisterMethod(), responseObserver);
}
/**
*
* Deconstructs a tuple. Returns a newly created GlobalDataHandle for each
* element in the tuple.
*
*/
public void deconstructTuple(xla.Xla.DeconstructTupleRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getDeconstructTupleMethod(), responseObserver);
}
/**
*
* Unpack requests that a global data handle, with a tuple shape, has global
* data handles created for each of its constituent members. This is the
* equivalent of the "destructuring assignment" present in various programming
* languages.
*
*/
public void unpack(xla.Xla.UnpackRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getUnpackMethod(), responseObserver);
}
/**
*
* Requests the shape of the referenced global data.
*
*/
public void getShape(xla.Xla.GetShapeRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getGetShapeMethod(), responseObserver);
}
/**
*
* Requests the statistics of the given computation.
*
*/
public void getComputationGraphStats(xla.Xla.ComputationGraphStatsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getGetComputationGraphStatsMethod(), responseObserver);
}
/**
*
* Loads a variable number of values with a given element type from ColumnIO.
*
*/
public void loadData(xla.Xla.LoadDataRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getLoadDataMethod(), responseObserver);
}
/**
*
* Transfers the given global data to the client in the form of a Literal.
*
*/
public void transferToClient(xla.Xla.TransferToClientRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getTransferToClientMethod(), responseObserver);
}
/**
*
* Transfers the given literal to the server to be stored in a global
* allocation, which is returned.
*
*/
public void transferToServer(xla.Xla.TransferToServerRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getTransferToServerMethod(), responseObserver);
}
/**
*
* Transfers the given literal to the Infeed buffer of the device.
*
*/
public void transferToInfeed(xla.Xla.TransferToInfeedRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getTransferToInfeedMethod(), responseObserver);
}
/**
*
* Transferred literal from the Outfeed buffer of the device.
*
*/
public void transferFromOutfeed(xla.Xla.TransferFromOutfeedRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getTransferFromOutfeedMethod(), responseObserver);
}
/**
*
* Resets the device, clearing all existing state on the device.
*
*/
public void resetDevice(xla.Xla.ResetDeviceRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getResetDeviceMethod(), responseObserver);
}
/**
*
* Computes the value of a constant expression. The request contains the
* computation graph for the constant expression.
*
*/
public void computeConstantGraph(xla.Xla.ComputeConstantGraphRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getComputeConstantGraphMethod(), responseObserver);
}
/**
*
* Requests one or more device handles from the target. The returned device
* handles can be used to specify the device on which to execute computations
* or transfer data.
*
*/
public void getDeviceHandles(xla.Xla.GetDeviceHandlesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getGetDeviceHandlesMethod(), responseObserver);
}
/**
*
* Creates a channel handle that can be used to transfer data between
* two computations via a pair of Send and Recv instructions.
*
*/
public void createChannelHandle(xla.Xla.CreateChannelHandleRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getCreateChannelHandleMethod(), responseObserver);
}
/**
*
* Invokes the provided computation with the provided global data passed as
* immutable arguments. The request contains the whole computation graph.
* Returns global data output and execution timing.
*
*/
public void executeGraph(xla.Xla.ExecuteGraphRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getExecuteGraphMethod(), responseObserver);
}
/**
*
* Invokes the provided list of computations in parallel with the provided
* global data for each computation. Returns a list of global data output and
* execution timing.
*
*/
public void executeGraphParallel(xla.Xla.ExecuteGraphParallelRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getExecuteGraphParallelMethod(), responseObserver);
}
/**
*
* Waits until the given execution (aysnchronously launched) is complete, and
* returns the global data output.
*
*/
public void waitForExecution(xla.Xla.WaitForExecutionRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getWaitForExecutionMethod(), responseObserver);
}
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getUnregisterMethod(),
asyncUnaryCall(
new MethodHandlers<
xla.Xla.UnregisterRequest,
xla.Xla.UnregisterResponse>(
this, METHODID_UNREGISTER)))
.addMethod(
getDeconstructTupleMethod(),
asyncUnaryCall(
new MethodHandlers<
xla.Xla.DeconstructTupleRequest,
xla.Xla.DeconstructTupleResponse>(
this, METHODID_DECONSTRUCT_TUPLE)))
.addMethod(
getUnpackMethod(),
asyncUnaryCall(
new MethodHandlers<
xla.Xla.UnpackRequest,
xla.Xla.UnpackResponse>(
this, METHODID_UNPACK)))
.addMethod(
getGetShapeMethod(),
asyncUnaryCall(
new MethodHandlers<
xla.Xla.GetShapeRequest,
xla.Xla.GetShapeResponse>(
this, METHODID_GET_SHAPE)))
.addMethod(
getGetComputationGraphStatsMethod(),
asyncUnaryCall(
new MethodHandlers<
xla.Xla.ComputationGraphStatsRequest,
xla.Xla.ComputationStatsResponse>(
this, METHODID_GET_COMPUTATION_GRAPH_STATS)))
.addMethod(
getLoadDataMethod(),
asyncUnaryCall(
new MethodHandlers<
xla.Xla.LoadDataRequest,
xla.Xla.LoadDataResponse>(
this, METHODID_LOAD_DATA)))
.addMethod(
getTransferToClientMethod(),
asyncUnaryCall(
new MethodHandlers<
xla.Xla.TransferToClientRequest,
xla.Xla.TransferToClientResponse>(
this, METHODID_TRANSFER_TO_CLIENT)))
.addMethod(
getTransferToServerMethod(),
asyncUnaryCall(
new MethodHandlers<
xla.Xla.TransferToServerRequest,
xla.Xla.TransferToServerResponse>(
this, METHODID_TRANSFER_TO_SERVER)))
.addMethod(
getTransferToInfeedMethod(),
asyncUnaryCall(
new MethodHandlers<
xla.Xla.TransferToInfeedRequest,
xla.Xla.TransferToInfeedResponse>(
this, METHODID_TRANSFER_TO_INFEED)))
.addMethod(
getTransferFromOutfeedMethod(),
asyncUnaryCall(
new MethodHandlers<
xla.Xla.TransferFromOutfeedRequest,
xla.Xla.TransferFromOutfeedResponse>(
this, METHODID_TRANSFER_FROM_OUTFEED)))
.addMethod(
getResetDeviceMethod(),
asyncUnaryCall(
new MethodHandlers<
xla.Xla.ResetDeviceRequest,
xla.Xla.ResetDeviceResponse>(
this, METHODID_RESET_DEVICE)))
.addMethod(
getComputeConstantGraphMethod(),
asyncUnaryCall(
new MethodHandlers<
xla.Xla.ComputeConstantGraphRequest,
xla.Xla.ComputeConstantResponse>(
this, METHODID_COMPUTE_CONSTANT_GRAPH)))
.addMethod(
getGetDeviceHandlesMethod(),
asyncUnaryCall(
new MethodHandlers<
xla.Xla.GetDeviceHandlesRequest,
xla.Xla.GetDeviceHandlesResponse>(
this, METHODID_GET_DEVICE_HANDLES)))
.addMethod(
getCreateChannelHandleMethod(),
asyncUnaryCall(
new MethodHandlers<
xla.Xla.CreateChannelHandleRequest,
xla.Xla.CreateChannelHandleResponse>(
this, METHODID_CREATE_CHANNEL_HANDLE)))
.addMethod(
getExecuteGraphMethod(),
asyncUnaryCall(
new MethodHandlers<
xla.Xla.ExecuteGraphRequest,
xla.Xla.ExecuteResponse>(
this, METHODID_EXECUTE_GRAPH)))
.addMethod(
getExecuteGraphParallelMethod(),
asyncUnaryCall(
new MethodHandlers<
xla.Xla.ExecuteGraphParallelRequest,
xla.Xla.ExecuteParallelResponse>(
this, METHODID_EXECUTE_GRAPH_PARALLEL)))
.addMethod(
getWaitForExecutionMethod(),
asyncUnaryCall(
new MethodHandlers<
xla.Xla.WaitForExecutionRequest,
xla.Xla.WaitForExecutionResponse>(
this, METHODID_WAIT_FOR_EXECUTION)))
.build();
}
}
/**
*
*///////////////////////
* Global data requests
*
*/
public static final class XlaServiceStub extends io.grpc.stub.AbstractStub {
private XlaServiceStub(io.grpc.Channel channel) {
super(channel);
}
private XlaServiceStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected XlaServiceStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new XlaServiceStub(channel, callOptions);
}
/**
*
* Unregisters a global allocation.
* If the handle given is not currently allocated, a NOT_FOUND status is
* returned.
*
*/
public void unregister(xla.Xla.UnregisterRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getUnregisterMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Deconstructs a tuple. Returns a newly created GlobalDataHandle for each
* element in the tuple.
*
*/
public void deconstructTuple(xla.Xla.DeconstructTupleRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getDeconstructTupleMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Unpack requests that a global data handle, with a tuple shape, has global
* data handles created for each of its constituent members. This is the
* equivalent of the "destructuring assignment" present in various programming
* languages.
*
*/
public void unpack(xla.Xla.UnpackRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getUnpackMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Requests the shape of the referenced global data.
*
*/
public void getShape(xla.Xla.GetShapeRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getGetShapeMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Requests the statistics of the given computation.
*
*/
public void getComputationGraphStats(xla.Xla.ComputationGraphStatsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getGetComputationGraphStatsMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Loads a variable number of values with a given element type from ColumnIO.
*
*/
public void loadData(xla.Xla.LoadDataRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getLoadDataMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Transfers the given global data to the client in the form of a Literal.
*
*/
public void transferToClient(xla.Xla.TransferToClientRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getTransferToClientMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Transfers the given literal to the server to be stored in a global
* allocation, which is returned.
*
*/
public void transferToServer(xla.Xla.TransferToServerRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getTransferToServerMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Transfers the given literal to the Infeed buffer of the device.
*
*/
public void transferToInfeed(xla.Xla.TransferToInfeedRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getTransferToInfeedMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Transferred literal from the Outfeed buffer of the device.
*
*/
public void transferFromOutfeed(xla.Xla.TransferFromOutfeedRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getTransferFromOutfeedMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Resets the device, clearing all existing state on the device.
*
*/
public void resetDevice(xla.Xla.ResetDeviceRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getResetDeviceMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Computes the value of a constant expression. The request contains the
* computation graph for the constant expression.
*
*/
public void computeConstantGraph(xla.Xla.ComputeConstantGraphRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getComputeConstantGraphMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Requests one or more device handles from the target. The returned device
* handles can be used to specify the device on which to execute computations
* or transfer data.
*
*/
public void getDeviceHandles(xla.Xla.GetDeviceHandlesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getGetDeviceHandlesMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Creates a channel handle that can be used to transfer data between
* two computations via a pair of Send and Recv instructions.
*
*/
public void createChannelHandle(xla.Xla.CreateChannelHandleRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getCreateChannelHandleMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Invokes the provided computation with the provided global data passed as
* immutable arguments. The request contains the whole computation graph.
* Returns global data output and execution timing.
*
*/
public void executeGraph(xla.Xla.ExecuteGraphRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getExecuteGraphMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Invokes the provided list of computations in parallel with the provided
* global data for each computation. Returns a list of global data output and
* execution timing.
*
*/
public void executeGraphParallel(xla.Xla.ExecuteGraphParallelRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getExecuteGraphParallelMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Waits until the given execution (aysnchronously launched) is complete, and
* returns the global data output.
*
*/
public void waitForExecution(xla.Xla.WaitForExecutionRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getWaitForExecutionMethod(), getCallOptions()), request, responseObserver);
}
}
/**
*
*///////////////////////
* Global data requests
*
*/
public static final class XlaServiceBlockingStub extends io.grpc.stub.AbstractStub {
private XlaServiceBlockingStub(io.grpc.Channel channel) {
super(channel);
}
private XlaServiceBlockingStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected XlaServiceBlockingStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new XlaServiceBlockingStub(channel, callOptions);
}
/**
*
* Unregisters a global allocation.
* If the handle given is not currently allocated, a NOT_FOUND status is
* returned.
*
*/
public xla.Xla.UnregisterResponse unregister(xla.Xla.UnregisterRequest request) {
return blockingUnaryCall(
getChannel(), getUnregisterMethod(), getCallOptions(), request);
}
/**
*
* Deconstructs a tuple. Returns a newly created GlobalDataHandle for each
* element in the tuple.
*
*/
public xla.Xla.DeconstructTupleResponse deconstructTuple(xla.Xla.DeconstructTupleRequest request) {
return blockingUnaryCall(
getChannel(), getDeconstructTupleMethod(), getCallOptions(), request);
}
/**
*
* Unpack requests that a global data handle, with a tuple shape, has global
* data handles created for each of its constituent members. This is the
* equivalent of the "destructuring assignment" present in various programming
* languages.
*
*/
public xla.Xla.UnpackResponse unpack(xla.Xla.UnpackRequest request) {
return blockingUnaryCall(
getChannel(), getUnpackMethod(), getCallOptions(), request);
}
/**
*
* Requests the shape of the referenced global data.
*
*/
public xla.Xla.GetShapeResponse getShape(xla.Xla.GetShapeRequest request) {
return blockingUnaryCall(
getChannel(), getGetShapeMethod(), getCallOptions(), request);
}
/**
*
* Requests the statistics of the given computation.
*
*/
public xla.Xla.ComputationStatsResponse getComputationGraphStats(xla.Xla.ComputationGraphStatsRequest request) {
return blockingUnaryCall(
getChannel(), getGetComputationGraphStatsMethod(), getCallOptions(), request);
}
/**
*
* Loads a variable number of values with a given element type from ColumnIO.
*
*/
public xla.Xla.LoadDataResponse loadData(xla.Xla.LoadDataRequest request) {
return blockingUnaryCall(
getChannel(), getLoadDataMethod(), getCallOptions(), request);
}
/**
*
* Transfers the given global data to the client in the form of a Literal.
*
*/
public xla.Xla.TransferToClientResponse transferToClient(xla.Xla.TransferToClientRequest request) {
return blockingUnaryCall(
getChannel(), getTransferToClientMethod(), getCallOptions(), request);
}
/**
*
* Transfers the given literal to the server to be stored in a global
* allocation, which is returned.
*
*/
public xla.Xla.TransferToServerResponse transferToServer(xla.Xla.TransferToServerRequest request) {
return blockingUnaryCall(
getChannel(), getTransferToServerMethod(), getCallOptions(), request);
}
/**
*
* Transfers the given literal to the Infeed buffer of the device.
*
*/
public xla.Xla.TransferToInfeedResponse transferToInfeed(xla.Xla.TransferToInfeedRequest request) {
return blockingUnaryCall(
getChannel(), getTransferToInfeedMethod(), getCallOptions(), request);
}
/**
*
* Transferred literal from the Outfeed buffer of the device.
*
*/
public xla.Xla.TransferFromOutfeedResponse transferFromOutfeed(xla.Xla.TransferFromOutfeedRequest request) {
return blockingUnaryCall(
getChannel(), getTransferFromOutfeedMethod(), getCallOptions(), request);
}
/**
*
* Resets the device, clearing all existing state on the device.
*
*/
public xla.Xla.ResetDeviceResponse resetDevice(xla.Xla.ResetDeviceRequest request) {
return blockingUnaryCall(
getChannel(), getResetDeviceMethod(), getCallOptions(), request);
}
/**
*
* Computes the value of a constant expression. The request contains the
* computation graph for the constant expression.
*
*/
public xla.Xla.ComputeConstantResponse computeConstantGraph(xla.Xla.ComputeConstantGraphRequest request) {
return blockingUnaryCall(
getChannel(), getComputeConstantGraphMethod(), getCallOptions(), request);
}
/**
*
* Requests one or more device handles from the target. The returned device
* handles can be used to specify the device on which to execute computations
* or transfer data.
*
*/
public xla.Xla.GetDeviceHandlesResponse getDeviceHandles(xla.Xla.GetDeviceHandlesRequest request) {
return blockingUnaryCall(
getChannel(), getGetDeviceHandlesMethod(), getCallOptions(), request);
}
/**
*
* Creates a channel handle that can be used to transfer data between
* two computations via a pair of Send and Recv instructions.
*
*/
public xla.Xla.CreateChannelHandleResponse createChannelHandle(xla.Xla.CreateChannelHandleRequest request) {
return blockingUnaryCall(
getChannel(), getCreateChannelHandleMethod(), getCallOptions(), request);
}
/**
*
* Invokes the provided computation with the provided global data passed as
* immutable arguments. The request contains the whole computation graph.
* Returns global data output and execution timing.
*
*/
public xla.Xla.ExecuteResponse executeGraph(xla.Xla.ExecuteGraphRequest request) {
return blockingUnaryCall(
getChannel(), getExecuteGraphMethod(), getCallOptions(), request);
}
/**
*
* Invokes the provided list of computations in parallel with the provided
* global data for each computation. Returns a list of global data output and
* execution timing.
*
*/
public xla.Xla.ExecuteParallelResponse executeGraphParallel(xla.Xla.ExecuteGraphParallelRequest request) {
return blockingUnaryCall(
getChannel(), getExecuteGraphParallelMethod(), getCallOptions(), request);
}
/**
*
* Waits until the given execution (aysnchronously launched) is complete, and
* returns the global data output.
*
*/
public xla.Xla.WaitForExecutionResponse waitForExecution(xla.Xla.WaitForExecutionRequest request) {
return blockingUnaryCall(
getChannel(), getWaitForExecutionMethod(), getCallOptions(), request);
}
}
/**
*
*///////////////////////
* Global data requests
*
*/
public static final class XlaServiceFutureStub extends io.grpc.stub.AbstractStub {
private XlaServiceFutureStub(io.grpc.Channel channel) {
super(channel);
}
private XlaServiceFutureStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected XlaServiceFutureStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new XlaServiceFutureStub(channel, callOptions);
}
/**
*
* Unregisters a global allocation.
* If the handle given is not currently allocated, a NOT_FOUND status is
* returned.
*
*/
public com.google.common.util.concurrent.ListenableFuture unregister(
xla.Xla.UnregisterRequest request) {
return futureUnaryCall(
getChannel().newCall(getUnregisterMethod(), getCallOptions()), request);
}
/**
*
* Deconstructs a tuple. Returns a newly created GlobalDataHandle for each
* element in the tuple.
*
*/
public com.google.common.util.concurrent.ListenableFuture deconstructTuple(
xla.Xla.DeconstructTupleRequest request) {
return futureUnaryCall(
getChannel().newCall(getDeconstructTupleMethod(), getCallOptions()), request);
}
/**
*
* Unpack requests that a global data handle, with a tuple shape, has global
* data handles created for each of its constituent members. This is the
* equivalent of the "destructuring assignment" present in various programming
* languages.
*
*/
public com.google.common.util.concurrent.ListenableFuture unpack(
xla.Xla.UnpackRequest request) {
return futureUnaryCall(
getChannel().newCall(getUnpackMethod(), getCallOptions()), request);
}
/**
*
* Requests the shape of the referenced global data.
*
*/
public com.google.common.util.concurrent.ListenableFuture getShape(
xla.Xla.GetShapeRequest request) {
return futureUnaryCall(
getChannel().newCall(getGetShapeMethod(), getCallOptions()), request);
}
/**
*
* Requests the statistics of the given computation.
*
*/
public com.google.common.util.concurrent.ListenableFuture getComputationGraphStats(
xla.Xla.ComputationGraphStatsRequest request) {
return futureUnaryCall(
getChannel().newCall(getGetComputationGraphStatsMethod(), getCallOptions()), request);
}
/**
*
* Loads a variable number of values with a given element type from ColumnIO.
*
*/
public com.google.common.util.concurrent.ListenableFuture loadData(
xla.Xla.LoadDataRequest request) {
return futureUnaryCall(
getChannel().newCall(getLoadDataMethod(), getCallOptions()), request);
}
/**
*
* Transfers the given global data to the client in the form of a Literal.
*
*/
public com.google.common.util.concurrent.ListenableFuture transferToClient(
xla.Xla.TransferToClientRequest request) {
return futureUnaryCall(
getChannel().newCall(getTransferToClientMethod(), getCallOptions()), request);
}
/**
*
* Transfers the given literal to the server to be stored in a global
* allocation, which is returned.
*
*/
public com.google.common.util.concurrent.ListenableFuture transferToServer(
xla.Xla.TransferToServerRequest request) {
return futureUnaryCall(
getChannel().newCall(getTransferToServerMethod(), getCallOptions()), request);
}
/**
*
* Transfers the given literal to the Infeed buffer of the device.
*
*/
public com.google.common.util.concurrent.ListenableFuture transferToInfeed(
xla.Xla.TransferToInfeedRequest request) {
return futureUnaryCall(
getChannel().newCall(getTransferToInfeedMethod(), getCallOptions()), request);
}
/**
*
* Transferred literal from the Outfeed buffer of the device.
*
*/
public com.google.common.util.concurrent.ListenableFuture transferFromOutfeed(
xla.Xla.TransferFromOutfeedRequest request) {
return futureUnaryCall(
getChannel().newCall(getTransferFromOutfeedMethod(), getCallOptions()), request);
}
/**
*
* Resets the device, clearing all existing state on the device.
*
*/
public com.google.common.util.concurrent.ListenableFuture resetDevice(
xla.Xla.ResetDeviceRequest request) {
return futureUnaryCall(
getChannel().newCall(getResetDeviceMethod(), getCallOptions()), request);
}
/**
*
* Computes the value of a constant expression. The request contains the
* computation graph for the constant expression.
*
*/
public com.google.common.util.concurrent.ListenableFuture computeConstantGraph(
xla.Xla.ComputeConstantGraphRequest request) {
return futureUnaryCall(
getChannel().newCall(getComputeConstantGraphMethod(), getCallOptions()), request);
}
/**
*
* Requests one or more device handles from the target. The returned device
* handles can be used to specify the device on which to execute computations
* or transfer data.
*
*/
public com.google.common.util.concurrent.ListenableFuture getDeviceHandles(
xla.Xla.GetDeviceHandlesRequest request) {
return futureUnaryCall(
getChannel().newCall(getGetDeviceHandlesMethod(), getCallOptions()), request);
}
/**
*
* Creates a channel handle that can be used to transfer data between
* two computations via a pair of Send and Recv instructions.
*
*/
public com.google.common.util.concurrent.ListenableFuture createChannelHandle(
xla.Xla.CreateChannelHandleRequest request) {
return futureUnaryCall(
getChannel().newCall(getCreateChannelHandleMethod(), getCallOptions()), request);
}
/**
*
* Invokes the provided computation with the provided global data passed as
* immutable arguments. The request contains the whole computation graph.
* Returns global data output and execution timing.
*
*/
public com.google.common.util.concurrent.ListenableFuture executeGraph(
xla.Xla.ExecuteGraphRequest request) {
return futureUnaryCall(
getChannel().newCall(getExecuteGraphMethod(), getCallOptions()), request);
}
/**
*
* Invokes the provided list of computations in parallel with the provided
* global data for each computation. Returns a list of global data output and
* execution timing.
*
*/
public com.google.common.util.concurrent.ListenableFuture executeGraphParallel(
xla.Xla.ExecuteGraphParallelRequest request) {
return futureUnaryCall(
getChannel().newCall(getExecuteGraphParallelMethod(), getCallOptions()), request);
}
/**
*
* Waits until the given execution (aysnchronously launched) is complete, and
* returns the global data output.
*
*/
public com.google.common.util.concurrent.ListenableFuture waitForExecution(
xla.Xla.WaitForExecutionRequest request) {
return futureUnaryCall(
getChannel().newCall(getWaitForExecutionMethod(), getCallOptions()), request);
}
}
private static final int METHODID_UNREGISTER = 0;
private static final int METHODID_DECONSTRUCT_TUPLE = 1;
private static final int METHODID_UNPACK = 2;
private static final int METHODID_GET_SHAPE = 3;
private static final int METHODID_GET_COMPUTATION_GRAPH_STATS = 4;
private static final int METHODID_LOAD_DATA = 5;
private static final int METHODID_TRANSFER_TO_CLIENT = 6;
private static final int METHODID_TRANSFER_TO_SERVER = 7;
private static final int METHODID_TRANSFER_TO_INFEED = 8;
private static final int METHODID_TRANSFER_FROM_OUTFEED = 9;
private static final int METHODID_RESET_DEVICE = 10;
private static final int METHODID_COMPUTE_CONSTANT_GRAPH = 11;
private static final int METHODID_GET_DEVICE_HANDLES = 12;
private static final int METHODID_CREATE_CHANNEL_HANDLE = 13;
private static final int METHODID_EXECUTE_GRAPH = 14;
private static final int METHODID_EXECUTE_GRAPH_PARALLEL = 15;
private static final int METHODID_WAIT_FOR_EXECUTION = 16;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final XlaServiceImplBase serviceImpl;
private final int methodId;
MethodHandlers(XlaServiceImplBase serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_UNREGISTER:
serviceImpl.unregister((xla.Xla.UnregisterRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_DECONSTRUCT_TUPLE:
serviceImpl.deconstructTuple((xla.Xla.DeconstructTupleRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_UNPACK:
serviceImpl.unpack((xla.Xla.UnpackRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_SHAPE:
serviceImpl.getShape((xla.Xla.GetShapeRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_COMPUTATION_GRAPH_STATS:
serviceImpl.getComputationGraphStats((xla.Xla.ComputationGraphStatsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_LOAD_DATA:
serviceImpl.loadData((xla.Xla.LoadDataRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_TRANSFER_TO_CLIENT:
serviceImpl.transferToClient((xla.Xla.TransferToClientRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_TRANSFER_TO_SERVER:
serviceImpl.transferToServer((xla.Xla.TransferToServerRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_TRANSFER_TO_INFEED:
serviceImpl.transferToInfeed((xla.Xla.TransferToInfeedRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_TRANSFER_FROM_OUTFEED:
serviceImpl.transferFromOutfeed((xla.Xla.TransferFromOutfeedRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_RESET_DEVICE:
serviceImpl.resetDevice((xla.Xla.ResetDeviceRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_COMPUTE_CONSTANT_GRAPH:
serviceImpl.computeConstantGraph((xla.Xla.ComputeConstantGraphRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_DEVICE_HANDLES:
serviceImpl.getDeviceHandles((xla.Xla.GetDeviceHandlesRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_CREATE_CHANNEL_HANDLE:
serviceImpl.createChannelHandle((xla.Xla.CreateChannelHandleRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_EXECUTE_GRAPH:
serviceImpl.executeGraph((xla.Xla.ExecuteGraphRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_EXECUTE_GRAPH_PARALLEL:
serviceImpl.executeGraphParallel((xla.Xla.ExecuteGraphParallelRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_WAIT_FOR_EXECUTION:
serviceImpl.waitForExecution((xla.Xla.WaitForExecutionRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
private static abstract class XlaServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
XlaServiceBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return xla.XlaServiceOuterClass.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("XlaService");
}
}
private static final class XlaServiceFileDescriptorSupplier
extends XlaServiceBaseDescriptorSupplier {
XlaServiceFileDescriptorSupplier() {}
}
private static final class XlaServiceMethodDescriptorSupplier
extends XlaServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final String methodName;
XlaServiceMethodDescriptorSupplier(String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (XlaServiceGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new XlaServiceFileDescriptorSupplier())
.addMethod(getUnregisterMethod())
.addMethod(getDeconstructTupleMethod())
.addMethod(getUnpackMethod())
.addMethod(getGetShapeMethod())
.addMethod(getGetComputationGraphStatsMethod())
.addMethod(getLoadDataMethod())
.addMethod(getTransferToClientMethod())
.addMethod(getTransferToServerMethod())
.addMethod(getTransferToInfeedMethod())
.addMethod(getTransferFromOutfeedMethod())
.addMethod(getResetDeviceMethod())
.addMethod(getComputeConstantGraphMethod())
.addMethod(getGetDeviceHandlesMethod())
.addMethod(getCreateChannelHandleMethod())
.addMethod(getExecuteGraphMethod())
.addMethod(getExecuteGraphParallelMethod())
.addMethod(getWaitForExecutionMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy