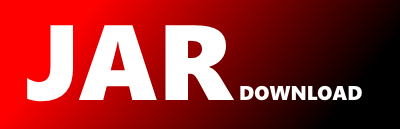
bibliothek.gui.dock.util.UIPriorityValue Maven / Gradle / Ivy
package bibliothek.gui.dock.util;
import bibliothek.util.FrameworkOnly;
/**
* A {@link PriorityValue} that supports working with {@link UIScheme}s.
* @author Benjamin Sigg
* @param the kind of item this {@link PriorityValue} stores
*/
@FrameworkOnly
public class UIPriorityValue extends PriorityValue> {
/**
* Sets the value of this
using a value that may be
* derived from scheme
.
* @param priority the priority of the value to set
* @param value the value that is to be set, can be null
* @param scheme the scheme that created value
or null
, ignored
* if value
is null
* @return true
if the value of this scheme changed because of
* the call to this method
*/
public boolean set( Priority priority, T value, UIScheme, ?, ?> scheme ){
T old = getValue();
if( value == null ){
set( priority, null );
}
else if( scheme == null ){
set( priority, new DirectValue( value ));
}
else{
set( priority, new SchemeValue( value, scheme ));
}
return old != getValue();
}
/**
* Gets the scheme that created the entry with priority
.
* @param priority the priority of the entry that is queried
* @return the scheme that was used to create the value or null
*/
public UIScheme,?,?> getScheme( Priority priority ){
Value value = get( priority );
if( value instanceof SchemeValue> ){
return ((SchemeValue>)value).scheme;
}
return null;
}
/**
* Tells whether all entries of this value are either null
or
* are created by an {@link UIScheme}.
* @return true
if all entries of this value are null
or created
*/
public boolean isAllScheme(){
for( Priority priority : Priority.values() ){
if( getScheme( priority ) == null && get( priority ) != null ){
return false;
}
}
return true;
}
/**
* Gets the current value of this {@link UIPriorityValue}.
* @return the current value or null
*/
public T getValue(){
Value value = get();
if( value == null ){
return null;
}
return value.getValue();
}
/**
* Gets the current value of {@link UIPriorityValue} on level priority
.
* @param priority the level to search, not null
* @return the value or null
*/
public T getValue( Priority priority ){
Value value = get( priority );
if( value == null ){
return null;
}
return value.getValue();
}
/**
* Represents a single entry in this map.
* @author Benjamin Sigg
*
* @param the kind of value this entry represents
*/
public interface Value{
public T getValue();
}
/**
* Represents an entry which was set directly by a client.
* @author Benjamin Sigg
* @param the kind of value this entry represents
*/
private static class DirectValue implements Value{
private T value;
/**
* Creates a new entry.
* @param value the value of this entry, can be null
*/
public DirectValue( T value ){
this.value = value;
}
public T getValue(){
return value;
}
}
/**
* Represents an entry which was created by reading from an {@link UIScheme}.
* @author Benjamin Sigg
* @param the kind of value this entry represents
*/
private static class SchemeValue implements Value{
private T value;
private UIScheme, ?, ?> scheme;
/**
* Creates a new entry.
* @param value the value of this entry, can be null
* @param scheme the scheme that was used to create this entry, must not be null
*/
public SchemeValue( T value, UIScheme, ?, ?> scheme ){
this.value = value;
this.scheme = scheme;
}
public T getValue(){
return value;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy