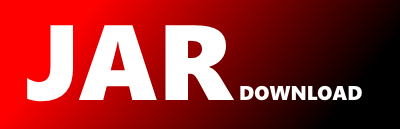
xyz.downgoon.mydk.concurrent.ThreadContext Maven / Gradle / Ivy
package xyz.downgoon.mydk.concurrent;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import xyz.downgoon.mydk.util.CollectionUtils;
/**
* A ThreadContext provides a means of binding and unbinding objects to the
* current thread based on key/value pairs.
*
*
* An internal {@link java.util.HashMap} is used to maintain the key/value pairs
* for each thread.
*
*
*
* If the desired behavior is to ensure that bound data is not shared across
* threads in a pooled or reusable threaded environment, the application (or
* more likely a framework) must bind and remove any necessary values at the
* beginning and end of stack execution, respectively (i.e. individually
* explicitly or all via the clear method).
*
*
* @see #removeAll() or {@link #clear()}
* @since 0.1
*/
public abstract class ThreadContext {
private static final ThreadLocal
© 2015 - 2025 Weber Informatics LLC | Privacy Policy