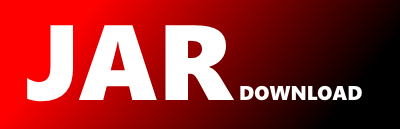
xyz.migoo.framework.infra.convert.developer.dictionary.DictionaryConvertImpl Maven / Gradle / Ivy
The newest version!
package xyz.migoo.framework.infra.convert.developer.dictionary;
import java.util.ArrayList;
import java.util.List;
import javax.annotation.processing.Generated;
import xyz.migoo.framework.common.pojo.PageResult;
import xyz.migoo.framework.infra.controller.developer.dictionary.vo.DictionaryAddReqVO;
import xyz.migoo.framework.infra.controller.developer.dictionary.vo.DictionaryRespVO;
import xyz.migoo.framework.infra.controller.developer.dictionary.vo.DictionaryUpdateReqVO;
import xyz.migoo.framework.infra.controller.developer.dictionary.vo.DictionaryValueAddReqVO;
import xyz.migoo.framework.infra.controller.developer.dictionary.vo.DictionaryValueRespVO;
import xyz.migoo.framework.infra.controller.developer.dictionary.vo.DictionaryValueUpdateReqVO;
import xyz.migoo.framework.infra.dal.dataobject.developer.dictionary.DictionaryDO;
import xyz.migoo.framework.infra.dal.dataobject.developer.dictionary.DictionaryValueDO;
@Generated(
value = "org.mapstruct.ap.MappingProcessor",
date = "2024-11-29T19:16:13+0800",
comments = "version: 1.6.0, compiler: javac, environment: Java 21.0.3 (Oracle Corporation)"
)
public class DictionaryConvertImpl implements DictionaryConvert {
@Override
public DictionaryDO convert(DictionaryAddReqVO bean) {
if ( bean == null ) {
return null;
}
DictionaryDO dictionaryDO = new DictionaryDO();
dictionaryDO.setCode( bean.getCode() );
dictionaryDO.setName( bean.getName() );
dictionaryDO.setSource( bean.getSource() );
dictionaryDO.setStatus( bean.getStatus() );
return dictionaryDO;
}
@Override
public DictionaryDO convert(DictionaryUpdateReqVO bean) {
if ( bean == null ) {
return null;
}
DictionaryDO dictionaryDO = new DictionaryDO();
dictionaryDO.setId( bean.getId() );
dictionaryDO.setCode( bean.getCode() );
dictionaryDO.setName( bean.getName() );
dictionaryDO.setSource( bean.getSource() );
dictionaryDO.setStatus( bean.getStatus() );
return dictionaryDO;
}
@Override
public DictionaryRespVO convert(DictionaryDO bean) {
if ( bean == null ) {
return null;
}
DictionaryRespVO dictionaryRespVO = new DictionaryRespVO();
dictionaryRespVO.setName( bean.getName() );
dictionaryRespVO.setCode( bean.getCode() );
dictionaryRespVO.setStatus( bean.getStatus() );
dictionaryRespVO.setSource( bean.getSource() );
dictionaryRespVO.setId( bean.getId() );
return dictionaryRespVO;
}
@Override
public PageResult convert(PageResult beans) {
if ( beans == null ) {
return null;
}
PageResult pageResult = new PageResult();
pageResult.setList( convert( beans.getList() ) );
pageResult.setTotal( beans.getTotal() );
return pageResult;
}
@Override
public List convert(List beans) {
if ( beans == null ) {
return null;
}
List list = new ArrayList( beans.size() );
for ( DictionaryDO dictionaryDO : beans ) {
list.add( convert( dictionaryDO ) );
}
return list;
}
@Override
public DictionaryValueDO convert(DictionaryValueAddReqVO bean) {
if ( bean == null ) {
return null;
}
DictionaryValueDO dictionaryValueDO = new DictionaryValueDO();
dictionaryValueDO.setLabel( bean.getLabel() );
dictionaryValueDO.setValue( bean.getValue() );
dictionaryValueDO.setDictCode( bean.getDictCode() );
dictionaryValueDO.setStatus( bean.getStatus() );
dictionaryValueDO.setColorType( bean.getColorType() );
dictionaryValueDO.setSort( bean.getSort() );
return dictionaryValueDO;
}
@Override
public DictionaryValueDO convert(DictionaryValueUpdateReqVO bean) {
if ( bean == null ) {
return null;
}
DictionaryValueDO dictionaryValueDO = new DictionaryValueDO();
dictionaryValueDO.setId( bean.getId() );
dictionaryValueDO.setLabel( bean.getLabel() );
dictionaryValueDO.setValue( bean.getValue() );
dictionaryValueDO.setDictCode( bean.getDictCode() );
dictionaryValueDO.setStatus( bean.getStatus() );
dictionaryValueDO.setColorType( bean.getColorType() );
dictionaryValueDO.setSort( bean.getSort() );
return dictionaryValueDO;
}
@Override
public DictionaryValueRespVO convert(DictionaryValueDO bean) {
if ( bean == null ) {
return null;
}
DictionaryValueRespVO dictionaryValueRespVO = new DictionaryValueRespVO();
dictionaryValueRespVO.setLabel( bean.getLabel() );
dictionaryValueRespVO.setValue( bean.getValue() );
dictionaryValueRespVO.setDictCode( bean.getDictCode() );
dictionaryValueRespVO.setStatus( bean.getStatus() );
dictionaryValueRespVO.setColorType( bean.getColorType() );
dictionaryValueRespVO.setSort( bean.getSort() );
dictionaryValueRespVO.setId( bean.getId() );
return dictionaryValueRespVO;
}
@Override
public PageResult convert2(PageResult beans) {
if ( beans == null ) {
return null;
}
PageResult pageResult = new PageResult();
pageResult.setList( convert2( beans.getList() ) );
pageResult.setTotal( beans.getTotal() );
return pageResult;
}
@Override
public List convert2(List beans) {
if ( beans == null ) {
return null;
}
List list = new ArrayList( beans.size() );
for ( DictionaryValueDO dictionaryValueDO : beans ) {
list.add( convert( dictionaryValueDO ) );
}
return list;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy