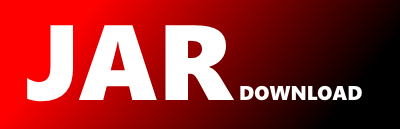
com.founder.core.domain.MzPayChargePercent Maven / Gradle / Ivy
package com.founder.core.domain;
import com.alibaba.fastjson.annotation.JSONField;
import com.baomidou.mybatisplus.annotation.TableId;
import com.baomidou.mybatisplus.annotation.TableName;
import java.io.Serializable;
import java.util.Date;
@TableName(value = "mz_pay_charge_percent")
public class MzPayChargePercent implements Serializable {
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column mz_pay_charge_percent.pay_unit
*
* @mbggenerated Fri Jan 19 16:02:33 CST 2018
*/
@TableId
private String pay_unit;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column mz_pay_charge_percent.responce_group
*
* @mbggenerated Fri Jan 19 16:02:33 CST 2018
*/
@TableId
private String responce_group;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column mz_pay_charge_percent.charge_group
*
* @mbggenerated Fri Jan 19 16:02:33 CST 2018
*/
@TableId
private String charge_group;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column mz_pay_charge_percent.type
*
* @mbggenerated Fri Jan 19 16:02:33 CST 2018
*/
@TableId
private String type;
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column mz_pay_charge_percent.pay_unit
*
* @return the value of mz_pay_charge_percent.pay_unit
* @mbggenerated Fri Jan 19 16:02:33 CST 2018
*/
public String getPay_unit() {
return pay_unit;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column mz_pay_charge_percent.pay_unit
*
* @param pay_unit the value for mz_pay_charge_percent.pay_unit
* @mbggenerated Fri Jan 19 16:02:33 CST 2018
*/
public void setPay_unit(String pay_unit) {
this.pay_unit = pay_unit;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column mz_pay_charge_percent.responce_group
*
* @return the value of mz_pay_charge_percent.responce_group
* @mbggenerated Fri Jan 19 16:02:33 CST 2018
*/
public String getResponce_group() {
return responce_group;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column mz_pay_charge_percent.responce_group
*
* @param responce_group the value for mz_pay_charge_percent.responce_group
* @mbggenerated Fri Jan 19 16:02:33 CST 2018
*/
public void setResponce_group(String responce_group) {
this.responce_group = responce_group;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column mz_pay_charge_percent.charge_group
*
* @return the value of mz_pay_charge_percent.charge_group
* @mbggenerated Fri Jan 19 16:02:33 CST 2018
*/
public String getCharge_group() {
return charge_group;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column mz_pay_charge_percent.charge_group
*
* @param charge_group the value for mz_pay_charge_percent.charge_group
* @mbggenerated Fri Jan 19 16:02:33 CST 2018
*/
public void setCharge_group(String charge_group) {
this.charge_group = charge_group;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column mz_pay_charge_percent.type
*
* @return the value of mz_pay_charge_percent.type
* @mbggenerated Fri Jan 19 16:02:33 CST 2018
*/
public String getType() {
return type;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column mz_pay_charge_percent.type
*
* @param type the value for mz_pay_charge_percent.type
* @mbggenerated Fri Jan 19 16:02:33 CST 2018
*/
public void setType(String type) {
this.type = type;
}
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column mz_pay_charge_percent.start_date
*
* @mbggenerated Fri Jan 19 16:02:33 CST 2018
*/
@JSONField(format = "yyyy-MM-dd HH:mm:ss")
private Date start_date;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column mz_pay_charge_percent.end_date
*
* @mbggenerated Fri Jan 19 16:02:33 CST 2018
*/
@JSONField(format = "yyyy-MM-dd HH:mm:ss")
private Date end_date;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column mz_pay_charge_percent.charge_percent
*
* @mbggenerated Fri Jan 19 16:02:33 CST 2018
*/
private Double charge_percent;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column mz_pay_charge_percent.charge_amount
*
* @mbggenerated Fri Jan 19 16:02:33 CST 2018
*/
private Double charge_amount;
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column mz_pay_charge_percent.start_date
*
* @return the value of mz_pay_charge_percent.start_date
* @mbggenerated Fri Jan 19 16:02:33 CST 2018
*/
public Date getStart_date() {
return start_date;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column mz_pay_charge_percent.start_date
*
* @param start_date the value for mz_pay_charge_percent.start_date
* @mbggenerated Fri Jan 19 16:02:33 CST 2018
*/
public void setStart_date(Date start_date) {
this.start_date = start_date;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column mz_pay_charge_percent.end_date
*
* @return the value of mz_pay_charge_percent.end_date
* @mbggenerated Fri Jan 19 16:02:33 CST 2018
*/
public Date getEnd_date() {
return end_date;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column mz_pay_charge_percent.end_date
*
* @param end_date the value for mz_pay_charge_percent.end_date
* @mbggenerated Fri Jan 19 16:02:33 CST 2018
*/
public void setEnd_date(Date end_date) {
this.end_date = end_date;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column mz_pay_charge_percent.charge_percent
*
* @return the value of mz_pay_charge_percent.charge_percent
* @mbggenerated Fri Jan 19 16:02:33 CST 2018
*/
public Double getCharge_percent() {
return charge_percent;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column mz_pay_charge_percent.charge_percent
*
* @param charge_percent the value for mz_pay_charge_percent.charge_percent
* @mbggenerated Fri Jan 19 16:02:33 CST 2018
*/
public void setCharge_percent(Double charge_percent) {
this.charge_percent = charge_percent;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column mz_pay_charge_percent.charge_amount
*
* @return the value of mz_pay_charge_percent.charge_amount
* @mbggenerated Fri Jan 19 16:02:33 CST 2018
*/
public Double getCharge_amount() {
return charge_amount;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column mz_pay_charge_percent.charge_amount
*
* @param charge_amount the value for mz_pay_charge_percent.charge_amount
* @mbggenerated Fri Jan 19 16:02:33 CST 2018
*/
public void setCharge_amount(Double charge_amount) {
this.charge_amount = charge_amount;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy