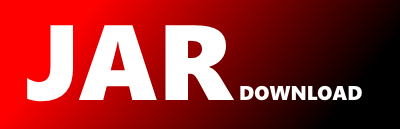
com.founder.core.domain.MzReceipt Maven / Gradle / Ivy
package com.founder.core.domain;
import com.baomidou.mybatisplus.annotation.IdType;
import com.baomidou.mybatisplus.annotation.TableId;
import com.baomidou.mybatisplus.annotation.TableName;
import java.io.Serializable;
import java.math.BigDecimal;
import java.util.Date;
@TableName(value = "mz_receipt")
public class MzReceipt implements Serializable {
@TableId(value = "patient_id",type = IdType.INPUT)
private String patient_id;
@TableId(value = "ledger_sn",type = IdType.INPUT)
private Short ledger_sn;
@TableId(value = "receipt_sn",type = IdType.INPUT)
private Integer receipt_sn;
private String pay_unit;
private BigDecimal charge_total;
private String charge_status;
private Date cash_date;
private String cash_opera;
private Byte windows_no;
private Date report_date;
private String receipt_no;
private String mz_dept_no;
private Date group_date;
private String contract_code;
private String responce_type;
public String getPatient_id() {
return patient_id;
}
public void setPatient_id(String patient_id) {
this.patient_id = patient_id;
}
public Short getLedger_sn() {
return ledger_sn;
}
public void setLedger_sn(Short ledger_sn) {
this.ledger_sn = ledger_sn;
}
public Integer getReceipt_sn() {
return receipt_sn;
}
public void setReceipt_sn(Integer receipt_sn) {
this.receipt_sn = receipt_sn;
}
public String getPay_unit() {
return pay_unit;
}
public void setPay_unit(String pay_unit) {
this.pay_unit = pay_unit;
}
public BigDecimal getCharge_total() {
return charge_total;
}
public void setCharge_total(BigDecimal charge_total) {
this.charge_total = charge_total;
}
public String getCharge_status() {
return charge_status;
}
public void setCharge_status(String charge_status) {
this.charge_status = charge_status;
}
public Date getCash_date() {
return cash_date;
}
public void setCash_date(Date cash_date) {
this.cash_date = cash_date;
}
public String getCash_opera() {
return cash_opera;
}
public void setCash_opera(String cash_opera) {
this.cash_opera = cash_opera;
}
public Byte getWindows_no() {
return windows_no;
}
public void setWindows_no(Byte windows_no) {
this.windows_no = windows_no;
}
public Date getReport_date() {
return report_date;
}
public void setReport_date(Date report_date) {
this.report_date = report_date;
}
public String getReceipt_no() {
return receipt_no;
}
public void setReceipt_no(String receipt_no) {
this.receipt_no = receipt_no;
}
public String getMz_dept_no() {
return mz_dept_no;
}
public void setMz_dept_no(String mz_dept_no) {
this.mz_dept_no = mz_dept_no;
}
public Date getGroup_date() {
return group_date;
}
public void setGroup_date(Date group_date) {
this.group_date = group_date;
}
public String getContract_code() {
return contract_code;
}
public void setContract_code(String contract_code) {
this.contract_code = contract_code;
}
public String getResponce_type() {
return responce_type;
}
public void setResponce_type(String responce_type) {
this.responce_type = responce_type;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy