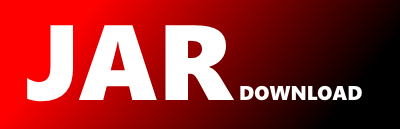
com.founder.core.domain.YpSupplyer Maven / Gradle / Ivy
package com.founder.core.domain;
import com.baomidou.mybatisplus.annotation.TableId;
import com.baomidou.mybatisplus.annotation.TableName;
import java.io.Serializable;
@TableName(value = "yp_supplyer")
public class YpSupplyer implements Serializable {
@TableId
private String supplyer_code;
private String supplyer_name;
private String bank;
private String account;
private String abbr_name;
private String address;
private String zip_code;
private String tel;
private String link;
private String busi_scope;
private String py_code;
private String d_code;
private String relation;
private String comment;
private String deleted_flag;
private String tax_no;
public String getSupplyer_code() {
return supplyer_code;
}
public void setSupplyer_code(String supplyer_code) {
this.supplyer_code = supplyer_code;
}
public String getSupplyer_name() {
return supplyer_name;
}
public void setSupplyer_name(String supplyer_name) {
this.supplyer_name = supplyer_name;
}
public String getBank() {
return bank;
}
public void setBank(String bank) {
this.bank = bank;
}
public String getAccount() {
return account;
}
public void setAccount(String account) {
this.account = account;
}
public String getAbbr_name() {
return abbr_name;
}
public void setAbbr_name(String abbr_name) {
this.abbr_name = abbr_name;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public String getZip_code() {
return zip_code;
}
public void setZip_code(String zip_code) {
this.zip_code = zip_code;
}
public String getTel() {
return tel;
}
public void setTel(String tel) {
this.tel = tel;
}
public String getLink() {
return link;
}
public void setLink(String link) {
this.link = link;
}
public String getBusi_scope() {
return busi_scope;
}
public void setBusi_scope(String busi_scope) {
this.busi_scope = busi_scope;
}
public String getPy_code() {
return py_code;
}
public void setPy_code(String py_code) {
this.py_code = py_code;
}
public String getD_code() {
return d_code;
}
public void setD_code(String d_code) {
this.d_code = d_code;
}
public String getRelation() {
return relation;
}
public void setRelation(String relation) {
this.relation = relation;
}
public String getComment() {
return comment;
}
public void setComment(String comment) {
this.comment = comment;
}
public String getDeleted_flag() {
return deleted_flag;
}
public void setDeleted_flag(String deleted_flag) {
this.deleted_flag = deleted_flag;
}
public String getTax_no() {
return tax_no;
}
public void setTax_no(String tax_no) {
this.tax_no = tax_no;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy