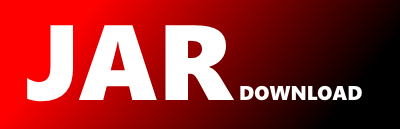
com.founder.core.utils.IpUtils Maven / Gradle / Ivy
package com.founder.core.utils;
import java.net.*;
import java.util.ArrayList;
import java.util.Enumeration;
import java.util.List;
/**
* @author dingzhiwei [email protected]
* @version V1.0
* @Description: IP地址工具类
* @date 2017-07-05
* @Copyright: www.xxpay.org
*/
public class IpUtils {
/**
* getLocalhostIp(获取本机ip地址)
*
* @throws UnknownHostException
* @Exception 异常对象
* @since CodingExample Ver(编码范例查看) 1.1
*/
public static String getLocalhostIp() {
String ip = "";
try {
ip = InetAddress.getLocalHost().getHostAddress();
} catch (Exception e) {
return null;
}
return ip;
}
public static List getIpAddrs() throws Exception {
List IPs = new ArrayList();
Enumeration allNetInterfaces = null;
allNetInterfaces = NetworkInterface.getNetworkInterfaces();
InetAddress ip = null;
while (allNetInterfaces.hasMoreElements()) {
NetworkInterface netInterface = (NetworkInterface) allNetInterfaces.nextElement();
Enumeration> addresses = netInterface.getInetAddresses();
while (addresses.hasMoreElements()) {
ip = (InetAddress) addresses.nextElement();
if (ip != null && ip instanceof Inet4Address && ip.getHostAddress().indexOf(".") != -1) {
IPs.add(ip.getHostAddress());
}
}
}
return IPs;
}
/**
* 兼容Linux系统
*
* @return
*/
public static String getLocalIP() {
String ip = "";
try {
Enumeration> e1 = (Enumeration>) NetworkInterface
.getNetworkInterfaces();
while (e1.hasMoreElements()) {
NetworkInterface ni = (NetworkInterface) e1.nextElement();
Enumeration> e2 = ni.getInetAddresses();
while (e2.hasMoreElements()) {
InetAddress ia = (InetAddress) e2.nextElement();
if (ia instanceof Inet6Address)
continue;
if (!ia.isLoopbackAddress()) {
ip = ia.getHostAddress();
break;
}
}
}
} catch (SocketException e) {
e.printStackTrace();
return "";
}
return ip;
}
public static void main(String[] args) throws Exception {
System.out.println(IpUtils.getLocalIP());
System.out.println(IpUtils.getLocalhostIp());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy