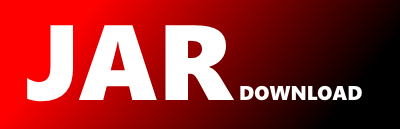
io.swagger.client.model.CreateReportRequest Maven / Gradle / Ivy
/*
* Commspace source data API
* API for Commspace source data management
*
* OpenAPI spec version: 1.0.4
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.client.model.ReportFilter;
import io.swagger.v3.oas.annotations.media.Schema;
import java.util.ArrayList;
import java.util.List;
/**
* CreateReportRequest
*/
@javax.annotation.Generated(value = "io.swagger.codegen.v3.generators.java.JavaClientCodegen", date = "2021-03-31T12:15:05.519Z[GMT]")
public class CreateReportRequest {
@JsonProperty("report_id")
private String reportId = null;
/**
* specify the format of the report output document
*/
public enum FormatEnum {
PDF("pdf"),
XLS("xls"),
CSV("csv");
private String value;
FormatEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static FormatEnum fromValue(String text) {
for (FormatEnum b : FormatEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
} @JsonProperty("format")
private FormatEnum format = null;
@JsonProperty("callback_url")
private String callbackUrl = null;
@JsonProperty("exclude_payload_from_callback")
private Boolean excludePayloadFromCallback = null;
@JsonProperty("compress")
private Boolean compress = null;
@JsonProperty("filters")
private List filters = null;
public CreateReportRequest reportId(String reportId) {
this.reportId = reportId;
return this;
}
/**
* Unique identifier of the report template to use
* @return reportId
**/
@Schema(required = true, description = "Unique identifier of the report template to use")
public String getReportId() {
return reportId;
}
public void setReportId(String reportId) {
this.reportId = reportId;
}
public CreateReportRequest format(FormatEnum format) {
this.format = format;
return this;
}
/**
* specify the format of the report output document
* @return format
**/
@Schema(description = "specify the format of the report output document")
public FormatEnum getFormat() {
return format;
}
public void setFormat(FormatEnum format) {
this.format = format;
}
public CreateReportRequest callbackUrl(String callbackUrl) {
this.callbackUrl = callbackUrl;
return this;
}
/**
* url to post report results to
* @return callbackUrl
**/
@Schema(description = "url to post report results to")
public String getCallbackUrl() {
return callbackUrl;
}
public void setCallbackUrl(String callbackUrl) {
this.callbackUrl = callbackUrl;
}
public CreateReportRequest excludePayloadFromCallback(Boolean excludePayloadFromCallback) {
this.excludePayloadFromCallback = excludePayloadFromCallback;
return this;
}
/**
* exclude the payload from the callback. This will require a GET to .../reports/{report_token} to retrieve the report content
* @return excludePayloadFromCallback
**/
@Schema(description = "exclude the payload from the callback. This will require a GET to .../reports/{report_token} to retrieve the report content")
public Boolean isExcludePayloadFromCallback() {
return excludePayloadFromCallback;
}
public void setExcludePayloadFromCallback(Boolean excludePayloadFromCallback) {
this.excludePayloadFromCallback = excludePayloadFromCallback;
}
public CreateReportRequest compress(Boolean compress) {
this.compress = compress;
return this;
}
/**
* compress the result in zip format
* @return compress
**/
@Schema(description = "compress the result in zip format")
public Boolean isCompress() {
return compress;
}
public void setCompress(Boolean compress) {
this.compress = compress;
}
public CreateReportRequest filters(List filters) {
this.filters = filters;
return this;
}
public CreateReportRequest addFiltersItem(ReportFilter filtersItem) {
if (this.filters == null) {
this.filters = new ArrayList<>();
}
this.filters.add(filtersItem);
return this;
}
/**
* Get filters
* @return filters
**/
@Schema(description = "")
public List getFilters() {
return filters;
}
public void setFilters(List filters) {
this.filters = filters;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreateReportRequest createReportRequest = (CreateReportRequest) o;
return Objects.equals(this.reportId, createReportRequest.reportId) &&
Objects.equals(this.format, createReportRequest.format) &&
Objects.equals(this.callbackUrl, createReportRequest.callbackUrl) &&
Objects.equals(this.excludePayloadFromCallback, createReportRequest.excludePayloadFromCallback) &&
Objects.equals(this.compress, createReportRequest.compress) &&
Objects.equals(this.filters, createReportRequest.filters);
}
@Override
public int hashCode() {
return Objects.hash(reportId, format, callbackUrl, excludePayloadFromCallback, compress, filters);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreateReportRequest {\n");
sb.append(" reportId: ").append(toIndentedString(reportId)).append("\n");
sb.append(" format: ").append(toIndentedString(format)).append("\n");
sb.append(" callbackUrl: ").append(toIndentedString(callbackUrl)).append("\n");
sb.append(" excludePayloadFromCallback: ").append(toIndentedString(excludePayloadFromCallback)).append("\n");
sb.append(" compress: ").append(toIndentedString(compress)).append("\n");
sb.append(" filters: ").append(toIndentedString(filters)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy