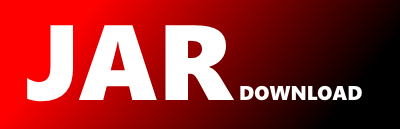
io.swagger.client.model.IntermediarySplit Maven / Gradle / Ivy
/*
* Commspace source data API
* API for Commspace source data management
*
* OpenAPI spec version: 1.0.4
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.v3.oas.annotations.media.Schema;
/**
* IntermediarySplit
*/
@javax.annotation.Generated(value = "io.swagger.codegen.v3.generators.java.JavaClientCodegen", date = "2021-03-31T12:15:05.519Z[GMT]")
public class IntermediarySplit {
@JsonProperty("intermediary_id")
private String intermediaryId = null;
@JsonProperty("split_percentage")
private Double splitPercentage = null;
@JsonProperty("commission_type_id")
private String commissionTypeId = null;
@JsonProperty("responsible")
private Boolean responsible = null;
@JsonProperty("match_broker_on_statement")
private Boolean matchBrokerOnStatement = null;
public IntermediarySplit intermediaryId(String intermediaryId) {
this.intermediaryId = intermediaryId;
return this;
}
/**
* unique identifier by which this intermediary is known at consumer
* @return intermediaryId
**/
@Schema(example = "abc1234", required = true, description = "unique identifier by which this intermediary is known at consumer")
public String getIntermediaryId() {
return intermediaryId;
}
public void setIntermediaryId(String intermediaryId) {
this.intermediaryId = intermediaryId;
}
public IntermediarySplit splitPercentage(Double splitPercentage) {
this.splitPercentage = splitPercentage;
return this;
}
/**
* percentage of commission that the intermediary is entitled to
* minimum: 0
* maximum: 100
* @return splitPercentage
**/
@Schema(example = "66.667", required = true, description = "percentage of commission that the intermediary is entitled to")
public Double getSplitPercentage() {
return splitPercentage;
}
public void setSplitPercentage(Double splitPercentage) {
this.splitPercentage = splitPercentage;
}
public IntermediarySplit commissionTypeId(String commissionTypeId) {
this.commissionTypeId = commissionTypeId;
return this;
}
/**
* (optional) commission type identifier as it is known by consumer
* @return commissionTypeId
**/
@Schema(example = "initial", description = "(optional) commission type identifier as it is known by consumer")
public String getCommissionTypeId() {
return commissionTypeId;
}
public void setCommissionTypeId(String commissionTypeId) {
this.commissionTypeId = commissionTypeId;
}
public IntermediarySplit responsible(Boolean responsible) {
this.responsible = responsible;
return this;
}
/**
* indicates whether this intermediary is the main intermediary on the agreement (if commission_type_id is not provided) or the commission type (if commission_type_id is provided)
* @return responsible
**/
@Schema(example = "true", required = true, description = "indicates whether this intermediary is the main intermediary on the agreement (if commission_type_id is not provided) or the commission type (if commission_type_id is provided)")
public Boolean isResponsible() {
return responsible;
}
public void setResponsible(Boolean responsible) {
this.responsible = responsible;
}
public IntermediarySplit matchBrokerOnStatement(Boolean matchBrokerOnStatement) {
this.matchBrokerOnStatement = matchBrokerOnStatement;
return this;
}
/**
* match the intermediary specified on this split with the intermediary on the statement.
* @return matchBrokerOnStatement
**/
@Schema(example = "true", description = "match the intermediary specified on this split with the intermediary on the statement.")
public Boolean isMatchBrokerOnStatement() {
return matchBrokerOnStatement;
}
public void setMatchBrokerOnStatement(Boolean matchBrokerOnStatement) {
this.matchBrokerOnStatement = matchBrokerOnStatement;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
IntermediarySplit intermediarySplit = (IntermediarySplit) o;
return Objects.equals(this.intermediaryId, intermediarySplit.intermediaryId) &&
Objects.equals(this.splitPercentage, intermediarySplit.splitPercentage) &&
Objects.equals(this.commissionTypeId, intermediarySplit.commissionTypeId) &&
Objects.equals(this.responsible, intermediarySplit.responsible) &&
Objects.equals(this.matchBrokerOnStatement, intermediarySplit.matchBrokerOnStatement);
}
@Override
public int hashCode() {
return Objects.hash(intermediaryId, splitPercentage, commissionTypeId, responsible, matchBrokerOnStatement);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class IntermediarySplit {\n");
sb.append(" intermediaryId: ").append(toIndentedString(intermediaryId)).append("\n");
sb.append(" splitPercentage: ").append(toIndentedString(splitPercentage)).append("\n");
sb.append(" commissionTypeId: ").append(toIndentedString(commissionTypeId)).append("\n");
sb.append(" responsible: ").append(toIndentedString(responsible)).append("\n");
sb.append(" matchBrokerOnStatement: ").append(toIndentedString(matchBrokerOnStatement)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy