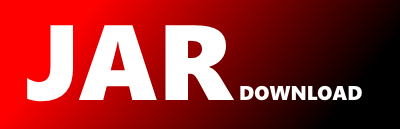
io.swagger.client.model.Product Maven / Gradle / Ivy
/*
* Commspace source data API
* API for Commspace source data management
*
* OpenAPI spec version: 1.0.4
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.client.model.Agreement;
import io.swagger.v3.oas.annotations.media.Schema;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.List;
/**
* Product
*/
@javax.annotation.Generated(value = "io.swagger.codegen.v3.generators.java.JavaClientCodegen", date = "2021-03-31T12:15:05.519Z[GMT]")
public class Product {
@JsonProperty("product_id")
private String productId = null;
@JsonProperty("product_number")
private String productNumber = null;
@JsonProperty("provider_id")
private String providerId = null;
@JsonProperty("product_category_id")
private String productCategoryId = null;
@JsonProperty("agreements")
private List agreements = new ArrayList<>();
@JsonProperty("inception_date")
private LocalDate inceptionDate = null;
@JsonProperty("termination_date")
private LocalDate terminationDate = null;
@JsonProperty("reference_number")
private String referenceNumber = null;
public Product productId(String productId) {
this.productId = productId;
return this;
}
/**
* unique identifier by which this product is known at consumer
* @return productId
**/
@Schema(example = "45678123", required = true, description = "unique identifier by which this product is known at consumer")
public String getProductId() {
return productId;
}
public void setProductId(String productId) {
this.productId = productId;
}
public Product productNumber(String productNumber) {
this.productNumber = productNumber;
return this;
}
/**
* unique product number issued by the product provider
* @return productNumber
**/
@Schema(example = "100015643", required = true, description = "unique product number issued by the product provider")
public String getProductNumber() {
return productNumber;
}
public void setProductNumber(String productNumber) {
this.productNumber = productNumber;
}
public Product providerId(String providerId) {
this.providerId = providerId;
return this;
}
/**
* unique identifier by which the product provider is known by the consumer
* @return providerId
**/
@Schema(example = "SLM", required = true, description = "unique identifier by which the product provider is known by the consumer")
public String getProviderId() {
return providerId;
}
public void setProviderId(String providerId) {
this.providerId = providerId;
}
public Product productCategoryId(String productCategoryId) {
this.productCategoryId = productCategoryId;
return this;
}
/**
* unique identifier by which the product category is known by the consumer
* @return productCategoryId
**/
@Schema(example = "1", required = true, description = "unique identifier by which the product category is known by the consumer")
public String getProductCategoryId() {
return productCategoryId;
}
public void setProductCategoryId(String productCategoryId) {
this.productCategoryId = productCategoryId;
}
public Product agreements(List agreements) {
this.agreements = agreements;
return this;
}
public Product addAgreementsItem(Agreement agreementsItem) {
this.agreements.add(agreementsItem);
return this;
}
/**
* array of agreements for this product
* @return agreements
**/
@Schema(required = true, description = "array of agreements for this product")
public List getAgreements() {
return agreements;
}
public void setAgreements(List agreements) {
this.agreements = agreements;
}
public Product inceptionDate(LocalDate inceptionDate) {
this.inceptionDate = inceptionDate;
return this;
}
/**
* inception date of the product
* @return inceptionDate
**/
@Schema(description = "inception date of the product")
public LocalDate getInceptionDate() {
return inceptionDate;
}
public void setInceptionDate(LocalDate inceptionDate) {
this.inceptionDate = inceptionDate;
}
public Product terminationDate(LocalDate terminationDate) {
this.terminationDate = terminationDate;
return this;
}
/**
* termination date of the product
* @return terminationDate
**/
@Schema(description = "termination date of the product")
public LocalDate getTerminationDate() {
return terminationDate;
}
public void setTerminationDate(LocalDate terminationDate) {
this.terminationDate = terminationDate;
}
public Product referenceNumber(String referenceNumber) {
this.referenceNumber = referenceNumber;
return this;
}
/**
* additional reference number for the product (optional)
* @return referenceNumber
**/
@Schema(description = "additional reference number for the product (optional)")
public String getReferenceNumber() {
return referenceNumber;
}
public void setReferenceNumber(String referenceNumber) {
this.referenceNumber = referenceNumber;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Product product = (Product) o;
return Objects.equals(this.productId, product.productId) &&
Objects.equals(this.productNumber, product.productNumber) &&
Objects.equals(this.providerId, product.providerId) &&
Objects.equals(this.productCategoryId, product.productCategoryId) &&
Objects.equals(this.agreements, product.agreements) &&
Objects.equals(this.inceptionDate, product.inceptionDate) &&
Objects.equals(this.terminationDate, product.terminationDate) &&
Objects.equals(this.referenceNumber, product.referenceNumber);
}
@Override
public int hashCode() {
return Objects.hash(productId, productNumber, providerId, productCategoryId, agreements, inceptionDate, terminationDate, referenceNumber);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Product {\n");
sb.append(" productId: ").append(toIndentedString(productId)).append("\n");
sb.append(" productNumber: ").append(toIndentedString(productNumber)).append("\n");
sb.append(" providerId: ").append(toIndentedString(providerId)).append("\n");
sb.append(" productCategoryId: ").append(toIndentedString(productCategoryId)).append("\n");
sb.append(" agreements: ").append(toIndentedString(agreements)).append("\n");
sb.append(" inceptionDate: ").append(toIndentedString(inceptionDate)).append("\n");
sb.append(" terminationDate: ").append(toIndentedString(terminationDate)).append("\n");
sb.append(" referenceNumber: ").append(toIndentedString(referenceNumber)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy