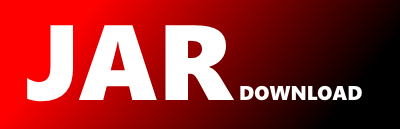
com.axellience.vueroutergwt.client.Route Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vue-router-gwt Show documentation
Show all versions of vue-router-gwt Show documentation
Vue Router JsInterop classes to be used with Vue GWT.
The newest version!
package com.axellience.vueroutergwt.client;
import static com.axellience.vuegwt.core.client.tools.JsUtils.map;
import elemental2.core.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsPackage;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
import jsinterop.base.JsPropertyMap;
/**
* @author Adrien Baron
*/
@JsType(isNative = true, namespace = JsPackage.GLOBAL, name = "Object")
public final class Route {
@JsProperty
private String name;
@JsProperty
private String path;
@JsProperty
private String hash;
@JsProperty
private JsPropertyMap query;
@JsProperty
private JsPropertyMap params;
@JsProperty
private String fullPath;
@JsProperty
private JsArray matched;
@JsProperty
private String redirectedFrom;
@JsProperty
private Object meta;
@JsOverlay
public static Route of(String name, String path) {
return new Route().setName(name).setPath(path);
}
@JsOverlay
public final String getName() {
return name;
}
@JsOverlay
public final Route setName(String name) {
this.name = name;
return this;
}
@JsOverlay
public final String getPath() {
return path;
}
@JsOverlay
public final Route setPath(String path) {
this.path = path;
return this;
}
@JsOverlay
public final String getHash() {
return hash;
}
@JsOverlay
public final Route setHash(String hash) {
this.hash = hash;
return this;
}
@JsOverlay
public final JsPropertyMap getQuery() {
return query;
}
@JsOverlay
public final Route setQuery(JsPropertyMap query) {
this.query = query;
return this;
}
@JsOverlay
public final Route setQueryParameter(String key, String value) {
if (this.query == null) {
this.query = map();
}
this.query.set(key, value);
return this;
}
@JsOverlay
public final JsPropertyMap getParams() {
return params;
}
@JsOverlay
public final Route setParams(JsPropertyMap params) {
this.params = params;
return this;
}
@JsOverlay
public final Route setParam(String key, String value) {
if (this.params == null) {
this.params = map();
}
this.params.set(key, value);
return this;
}
@JsOverlay
public final String getFullPath() {
return fullPath;
}
@JsOverlay
public final Route setFullPath(String fullPath) {
this.fullPath = fullPath;
return this;
}
@JsOverlay
public final JsArray getMatched() {
return matched;
}
@JsOverlay
public final Route setMatched(JsArray matched) {
this.matched = matched;
return this;
}
@JsOverlay
public final String getRedirectedFrom() {
return redirectedFrom;
}
@JsOverlay
public final Route setRedirectedFrom(String redirectedFrom) {
this.redirectedFrom = redirectedFrom;
return this;
}
@JsOverlay
public final Object getMeta() {
return meta;
}
@JsOverlay
public final Route setMeta(Object meta) {
this.meta = meta;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy