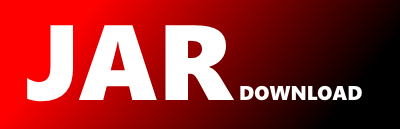
com.github.tonivade.resp.protocol.RedisToken Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of resp-server Show documentation
Show all versions of resp-server Show documentation
Netty implementation of REdis Serialization Protocol, and a simple framework to implement command based protocols
/*
* Copyright (c) 2016-2019, Antonio Gabriel Muñoz Conejo
* Distributed under the terms of the MIT License
*/
package com.github.tonivade.resp.protocol;
import static com.github.tonivade.resp.protocol.SafeString.safeString;
import java.util.Collection;
import java.util.stream.Stream;
import com.github.tonivade.purefun.data.ImmutableList;
import com.github.tonivade.purefun.data.Sequence;
import com.github.tonivade.resp.protocol.AbstractRedisToken.ArrayRedisToken;
import com.github.tonivade.resp.protocol.AbstractRedisToken.ErrorRedisToken;
import com.github.tonivade.resp.protocol.AbstractRedisToken.IntegerRedisToken;
import com.github.tonivade.resp.protocol.AbstractRedisToken.StatusRedisToken;
import com.github.tonivade.resp.protocol.AbstractRedisToken.StringRedisToken;
public interface RedisToken {
RedisToken NULL_STRING = string((SafeString) null);
RedisToken RESPONSE_OK = status("OK");
RedisTokenType getType();
T accept(RedisTokenVisitor visitor);
static RedisToken nullString() {
return NULL_STRING;
}
static RedisToken responseOk() {
return RESPONSE_OK;
}
static RedisToken string(SafeString str) {
return new StringRedisToken(str);
}
static RedisToken string(String str) {
return new StringRedisToken(safeString(str));
}
static RedisToken status(String str) {
return new StatusRedisToken(str);
}
static RedisToken integer(boolean b) {
return new IntegerRedisToken(b ? 1 : 0);
}
static RedisToken integer(int i) {
return new IntegerRedisToken(i);
}
static RedisToken error(String str) {
return new ErrorRedisToken(str);
}
static RedisToken array(RedisToken... redisTokens) {
return new ArrayRedisToken(ImmutableList.of(redisTokens));
}
static RedisToken array(Collection redisTokens) {
return new ArrayRedisToken(ImmutableList.from(redisTokens));
}
static RedisToken array(Sequence redisTokens) {
return new ArrayRedisToken(redisTokens.asArray());
}
static Stream visit(Stream tokens, RedisTokenVisitor visitor) {
return tokens.map(token -> token.accept(visitor));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy