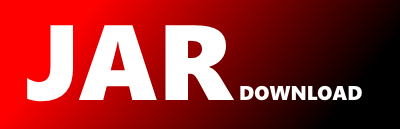
com.github.tonivade.zeromock.api.HttpService Maven / Gradle / Ivy
/*
* Copyright (c) 2018-2020, Antonio Gabriel Muñoz Conejo
* Distributed under the terms of the MIT License
*/
package com.github.tonivade.zeromock.api;
import static com.github.tonivade.purefun.type.IdOf.toId;
import static com.github.tonivade.zeromock.api.PreFilter.filter;
import static java.util.Objects.requireNonNull;
import com.github.tonivade.purefun.Matcher1;
import com.github.tonivade.purefun.instances.IdInstances;
import com.github.tonivade.purefun.type.Id_;
import com.github.tonivade.purefun.type.Option;
public final class HttpService {
private final HttpServiceK serviceK;
public HttpService(String name) {
this(new HttpServiceK<>(name, IdInstances.monad()));
}
private HttpService(HttpServiceK serviceK) {
this.serviceK = requireNonNull(serviceK);
}
public String name() {
return serviceK.name();
}
public HttpServiceK build() {
return serviceK;
}
public HttpService mount(String path, HttpService other) {
return new HttpService(serviceK.mount(path, other.serviceK));
}
public HttpService exec(RequestHandler handler) {
return new HttpService(serviceK.exec(handler.liftId()::apply));
}
public ThenStep preFilter(Matcher1 matcher) {
return handler -> addPreFilter(matcher, handler);
}
public HttpService preFilter(PreFilter filter) {
return new HttpService(serviceK.preFilter(filter.liftId()::apply));
}
public HttpService postFilter(PostFilter filter) {
return new HttpService(serviceK.postFilter(filter.liftId()::apply));
}
public ThenStep when(Matcher1 matcher) {
return handler -> addMapping(matcher, handler);
}
public Option execute(HttpRequest request) {
return serviceK.execute(request).fix(toId()).get();
}
public HttpService combine(HttpService other) {
return new HttpService(serviceK.combine(other.serviceK));
}
protected HttpService addMapping(Matcher1 matcher, RequestHandler handler) {
return new HttpService(serviceK.addMapping(matcher, handler.liftId()::apply));
}
protected HttpService addPreFilter(Matcher1 matcher, RequestHandler handler) {
return preFilter(filter(matcher, handler));
}
@Override
public String toString() {
return "HttpService(" + serviceK.name() + ")";
}
@FunctionalInterface
public interface ThenStep {
T then(RequestHandler handler);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy