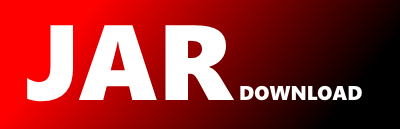
com.github.ykiselev.buffers.SimpleBuffers Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of buffer-pool Show documentation
Show all versions of buffer-pool Show documentation
A simple object pool fro byte buffers
The newest version!
/*
* Copyright 2017 Yuriy Kiselev ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.ykiselev.buffers;
import com.github.ykiselev.buffers.pool.BufferPool;
import java.nio.Buffer;
import java.nio.ByteBuffer;
import java.nio.CharBuffer;
import java.nio.DoubleBuffer;
import java.nio.FloatBuffer;
import java.nio.IntBuffer;
import java.nio.LongBuffer;
import java.nio.ShortBuffer;
import static java.util.Objects.requireNonNull;
/**
* Created by Y.Kiselev on 04.06.2016.
*/
public final class SimpleBuffers implements Buffers {
private final BufferPool pool;
public SimpleBuffers(BufferPool pool) {
this.pool = requireNonNull(pool);
}
@Override
public Pooled byteBuffer(int size) {
final ByteBuffer buffer = acquire(size);
return createPooled(buffer, buffer);
}
@Override
public Pooled floatBuffer(int size) {
final ByteBuffer buffer = acquire(size * Float.BYTES);
return createPooled(buffer, buffer.asFloatBuffer());
}
@Override
public Pooled intBuffer(int size) {
final ByteBuffer buffer = acquire(size * Integer.BYTES);
return createPooled(buffer, buffer.asIntBuffer());
}
@Override
public Pooled charBuffer(int size) {
final ByteBuffer buffer = acquire(size * Character.BYTES);
return createPooled(buffer, buffer.asCharBuffer());
}
@Override
public Pooled shortBuffer(int size) {
final ByteBuffer buffer = acquire(size * Short.BYTES);
return createPooled(buffer, buffer.asShortBuffer());
}
@Override
public Pooled longBuffer(int size) {
final ByteBuffer buffer = acquire(size * Long.BYTES);
return createPooled(buffer, buffer.asLongBuffer());
}
@Override
public Pooled doubleBuffer(int size) {
final ByteBuffer buffer = acquire(size * Double.BYTES);
return createPooled(buffer, buffer.asDoubleBuffer());
}
private ByteBuffer acquire(int size) {
final ByteBuffer result = pool.acquire(size);
if (result == null) {
throw new IllegalStateException("Unable to acquire a buffer of size " + size);
}
result.clear();
return result;
}
private Pooled createPooled(final ByteBuffer pooled, final T buffer) {
return new Pooled() {
@Override
public T buffer() {
return buffer;
}
@Override
public void close() {
pool.release(pooled);
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy