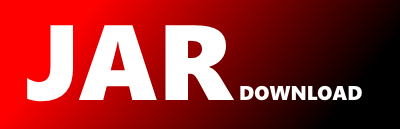
target.apidocs.com.google.api.services.calendar.model.Events.html Maven / Gradle / Ivy
The newest version!
Events (Calendar API v3-rev20241101-2.0.0)
com.google.api.services.calendar.model
Class Events
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.calendar.model.Events
-
public final class Events
extends com.google.api.client.json.GenericJson
Model definition for Events.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Calendar API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
Events()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
Events
clone()
String
getAccessRole()
The user's access role for this calendar.
List<EventReminder>
getDefaultReminders()
The default reminders on the calendar for the authenticated user.
String
getDescription()
Description of the calendar.
String
getEtag()
ETag of the collection.
List<Event>
getItems()
List of events on the calendar.
String
getKind()
Type of the collection ("calendar#events").
String
getNextPageToken()
Token used to access the next page of this result.
String
getNextSyncToken()
Token used at a later point in time to retrieve only the entries that have changed since this
result was returned.
String
getSummary()
Title of the calendar.
String
getTimeZone()
The time zone of the calendar.
com.google.api.client.util.DateTime
getUpdated()
Last modification time of the calendar (as a RFC3339 timestamp).
Events
set(String fieldName,
Object value)
Events
setAccessRole(String accessRole)
The user's access role for this calendar.
Events
setDefaultReminders(List<EventReminder> defaultReminders)
The default reminders on the calendar for the authenticated user.
Events
setDescription(String description)
Description of the calendar.
Events
setEtag(String etag)
ETag of the collection.
Events
setItems(List<Event> items)
List of events on the calendar.
Events
setKind(String kind)
Type of the collection ("calendar#events").
Events
setNextPageToken(String nextPageToken)
Token used to access the next page of this result.
Events
setNextSyncToken(String nextSyncToken)
Token used at a later point in time to retrieve only the entries that have changed since this
result was returned.
Events
setSummary(String summary)
Title of the calendar.
Events
setTimeZone(String timeZone)
The time zone of the calendar.
Events
setUpdated(com.google.api.client.util.DateTime updated)
Last modification time of the calendar (as a RFC3339 timestamp).
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getAccessRole
public String getAccessRole()
The user's access role for this calendar. Read-only. Possible values are: - "none" - The user
has no access. - "freeBusyReader" - The user has read access to free/busy information. -
"reader" - The user has read access to the calendar. Private events will appear to users with
reader access, but event details will be hidden. - "writer" - The user has read and write
access to the calendar. Private events will appear to users with writer access, and event
details will be visible. - "owner" - The user has ownership of the calendar. This role has all
of the permissions of the writer role with the additional ability to see and manipulate ACLs.
- Returns:
- value or
null
for none
-
setAccessRole
public Events setAccessRole(String accessRole)
The user's access role for this calendar. Read-only. Possible values are: - "none" - The user
has no access. - "freeBusyReader" - The user has read access to free/busy information. -
"reader" - The user has read access to the calendar. Private events will appear to users with
reader access, but event details will be hidden. - "writer" - The user has read and write
access to the calendar. Private events will appear to users with writer access, and event
details will be visible. - "owner" - The user has ownership of the calendar. This role has all
of the permissions of the writer role with the additional ability to see and manipulate ACLs.
- Parameters:
accessRole
- accessRole or null
for none
-
getDefaultReminders
public List<EventReminder> getDefaultReminders()
The default reminders on the calendar for the authenticated user. These reminders apply to all
events on this calendar that do not explicitly override them (i.e. do not have
reminders.useDefault set to True).
- Returns:
- value or
null
for none
-
setDefaultReminders
public Events setDefaultReminders(List<EventReminder> defaultReminders)
The default reminders on the calendar for the authenticated user. These reminders apply to all
events on this calendar that do not explicitly override them (i.e. do not have
reminders.useDefault set to True).
- Parameters:
defaultReminders
- defaultReminders or null
for none
-
getDescription
public String getDescription()
Description of the calendar. Read-only.
- Returns:
- value or
null
for none
-
setDescription
public Events setDescription(String description)
Description of the calendar. Read-only.
- Parameters:
description
- description or null
for none
-
getEtag
public String getEtag()
ETag of the collection.
- Returns:
- value or
null
for none
-
setEtag
public Events setEtag(String etag)
ETag of the collection.
- Parameters:
etag
- etag or null
for none
-
getItems
public List<Event> getItems()
List of events on the calendar.
- Returns:
- value or
null
for none
-
setItems
public Events setItems(List<Event> items)
List of events on the calendar.
- Parameters:
items
- items or null
for none
-
getKind
public String getKind()
Type of the collection ("calendar#events").
- Returns:
- value or
null
for none
-
setKind
public Events setKind(String kind)
Type of the collection ("calendar#events").
- Parameters:
kind
- kind or null
for none
-
getNextPageToken
public String getNextPageToken()
Token used to access the next page of this result. Omitted if no further results are available,
in which case nextSyncToken is provided.
- Returns:
- value or
null
for none
-
setNextPageToken
public Events setNextPageToken(String nextPageToken)
Token used to access the next page of this result. Omitted if no further results are available,
in which case nextSyncToken is provided.
- Parameters:
nextPageToken
- nextPageToken or null
for none
-
getNextSyncToken
public String getNextSyncToken()
Token used at a later point in time to retrieve only the entries that have changed since this
result was returned. Omitted if further results are available, in which case nextPageToken is
provided.
- Returns:
- value or
null
for none
-
setNextSyncToken
public Events setNextSyncToken(String nextSyncToken)
Token used at a later point in time to retrieve only the entries that have changed since this
result was returned. Omitted if further results are available, in which case nextPageToken is
provided.
- Parameters:
nextSyncToken
- nextSyncToken or null
for none
-
getSummary
public String getSummary()
Title of the calendar. Read-only.
- Returns:
- value or
null
for none
-
setSummary
public Events setSummary(String summary)
Title of the calendar. Read-only.
- Parameters:
summary
- summary or null
for none
-
getTimeZone
public String getTimeZone()
The time zone of the calendar. Read-only.
- Returns:
- value or
null
for none
-
setTimeZone
public Events setTimeZone(String timeZone)
The time zone of the calendar. Read-only.
- Parameters:
timeZone
- timeZone or null
for none
-
getUpdated
public com.google.api.client.util.DateTime getUpdated()
Last modification time of the calendar (as a RFC3339 timestamp). Read-only.
- Returns:
- value or
null
for none
-
setUpdated
public Events setUpdated(com.google.api.client.util.DateTime updated)
Last modification time of the calendar (as a RFC3339 timestamp). Read-only.
- Parameters:
updated
- updated or null
for none
-
set
public Events set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public Events clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2024 Weber Informatics LLC | Privacy Policy