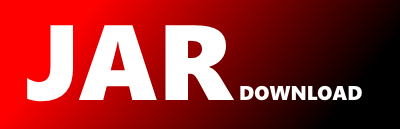
target.apidocs.com.google.api.services.compute.model.Router.html Maven / Gradle / Ivy
Router (Compute Engine API alpha-rev20200526-1.30.9)
com.google.api.services.compute.model
Class Router
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.compute.model.Router
-
public final class Router
extends GenericJson
Represents a Cloud Router resource.
For more information about Cloud Router, read the Cloud Router overview.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
Router()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
Router
clone()
RouterBgp
getBgp()
BGP information specific to this router.
List<RouterBgpPeer>
getBgpPeers()
BGP information that must be configured into the routing stack to establish BGP peering.
String
getCreationTimestamp()
[Output Only] Creation timestamp in RFC3339 text format.
String
getDescription()
An optional description of this resource.
BigInteger
getId()
[Output Only] The unique identifier for the resource.
List<RouterInterface>
getInterfaces()
Router interfaces.
String
getKind()
[Output Only] Type of resource.
String
getName()
Name of the resource.
List<RouterNat>
getNats()
A list of NAT services created in this router.
String
getNetwork()
URI of the network to which this router belongs.
String
getRegion()
[Output Only] URI of the region where the router resides.
String
getSelfLink()
[Output Only] Server-defined URL for the resource.
String
getSelfLinkWithId()
[Output Only] Server-defined URL for this resource with the resource id.
Router
set(String fieldName,
Object value)
Router
setBgp(RouterBgp bgp)
BGP information specific to this router.
Router
setBgpPeers(List<RouterBgpPeer> bgpPeers)
BGP information that must be configured into the routing stack to establish BGP peering.
Router
setCreationTimestamp(String creationTimestamp)
[Output Only] Creation timestamp in RFC3339 text format.
Router
setDescription(String description)
An optional description of this resource.
Router
setId(BigInteger id)
[Output Only] The unique identifier for the resource.
Router
setInterfaces(List<RouterInterface> interfaces)
Router interfaces.
Router
setKind(String kind)
[Output Only] Type of resource.
Router
setName(String name)
Name of the resource.
Router
setNats(List<RouterNat> nats)
A list of NAT services created in this router.
Router
setNetwork(String network)
URI of the network to which this router belongs.
Router
setRegion(String region)
[Output Only] URI of the region where the router resides.
Router
setSelfLink(String selfLink)
[Output Only] Server-defined URL for the resource.
Router
setSelfLinkWithId(String selfLinkWithId)
[Output Only] Server-defined URL for this resource with the resource id.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getBgp
public RouterBgp getBgp()
BGP information specific to this router.
- Returns:
- value or
null
for none
-
setBgp
public Router setBgp(RouterBgp bgp)
BGP information specific to this router.
- Parameters:
bgp
- bgp or null
for none
-
getBgpPeers
public List<RouterBgpPeer> getBgpPeers()
BGP information that must be configured into the routing stack to establish BGP peering. This
information must specify the peer ASN and either the interface name, IP address, or peer IP
address. Please refer to RFC4273.
- Returns:
- value or
null
for none
-
setBgpPeers
public Router setBgpPeers(List<RouterBgpPeer> bgpPeers)
BGP information that must be configured into the routing stack to establish BGP peering. This
information must specify the peer ASN and either the interface name, IP address, or peer IP
address. Please refer to RFC4273.
- Parameters:
bgpPeers
- bgpPeers or null
for none
-
getCreationTimestamp
public String getCreationTimestamp()
[Output Only] Creation timestamp in RFC3339 text format.
- Returns:
- value or
null
for none
-
setCreationTimestamp
public Router setCreationTimestamp(String creationTimestamp)
[Output Only] Creation timestamp in RFC3339 text format.
- Parameters:
creationTimestamp
- creationTimestamp or null
for none
-
getDescription
public String getDescription()
An optional description of this resource. Provide this property when you create the resource.
- Returns:
- value or
null
for none
-
setDescription
public Router setDescription(String description)
An optional description of this resource. Provide this property when you create the resource.
- Parameters:
description
- description or null
for none
-
getId
public BigInteger getId()
[Output Only] The unique identifier for the resource. This identifier is defined by the server.
- Returns:
- value or
null
for none
-
setId
public Router setId(BigInteger id)
[Output Only] The unique identifier for the resource. This identifier is defined by the server.
- Parameters:
id
- id or null
for none
-
getInterfaces
public List<RouterInterface> getInterfaces()
Router interfaces. Each interface requires either one linked resource, (for example,
linkedVpnTunnel), or IP address and IP address range (for example, ipRange), or both.
- Returns:
- value or
null
for none
-
setInterfaces
public Router setInterfaces(List<RouterInterface> interfaces)
Router interfaces. Each interface requires either one linked resource, (for example,
linkedVpnTunnel), or IP address and IP address range (for example, ipRange), or both.
- Parameters:
interfaces
- interfaces or null
for none
-
getKind
public String getKind()
[Output Only] Type of resource. Always compute#router for routers.
- Returns:
- value or
null
for none
-
setKind
public Router setKind(String kind)
[Output Only] Type of resource. Always compute#router for routers.
- Parameters:
kind
- kind or null
for none
-
getName
public String getName()
Name of the resource. Provided by the client when the resource is created. The name must be
1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first
character must be a lowercase letter, and all following characters must be a dash, lowercase
letter, or digit, except the last character, which cannot be a dash.
- Returns:
- value or
null
for none
-
setName
public Router setName(String name)
Name of the resource. Provided by the client when the resource is created. The name must be
1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first
character must be a lowercase letter, and all following characters must be a dash, lowercase
letter, or digit, except the last character, which cannot be a dash.
- Parameters:
name
- name or null
for none
-
getNats
public List<RouterNat> getNats()
A list of NAT services created in this router.
- Returns:
- value or
null
for none
-
setNats
public Router setNats(List<RouterNat> nats)
A list of NAT services created in this router.
- Parameters:
nats
- nats or null
for none
-
getNetwork
public String getNetwork()
URI of the network to which this router belongs.
- Returns:
- value or
null
for none
-
setNetwork
public Router setNetwork(String network)
URI of the network to which this router belongs.
- Parameters:
network
- network or null
for none
-
getRegion
public String getRegion()
[Output Only] URI of the region where the router resides. You must specify this field as part
of the HTTP request URL. It is not settable as a field in the request body.
- Returns:
- value or
null
for none
-
setRegion
public Router setRegion(String region)
[Output Only] URI of the region where the router resides. You must specify this field as part
of the HTTP request URL. It is not settable as a field in the request body.
- Parameters:
region
- region or null
for none
-
getSelfLink
public String getSelfLink()
[Output Only] Server-defined URL for the resource.
- Returns:
- value or
null
for none
-
setSelfLink
public Router setSelfLink(String selfLink)
[Output Only] Server-defined URL for the resource.
- Parameters:
selfLink
- selfLink or null
for none
-
getSelfLinkWithId
public String getSelfLinkWithId()
[Output Only] Server-defined URL for this resource with the resource id.
- Returns:
- value or
null
for none
-
setSelfLinkWithId
public Router setSelfLinkWithId(String selfLinkWithId)
[Output Only] Server-defined URL for this resource with the resource id.
- Parameters:
selfLinkWithId
- selfLinkWithId or null
for none
-
set
public Router set(String fieldName,
Object value)
- Overrides:
set
in class GenericJson
-
clone
public Router clone()
- Overrides:
clone
in class GenericJson
Copyright © 2011–2020 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy