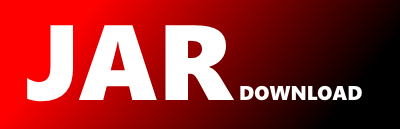
target.apidocs.com.google.api.services.content.model.AccountsCustomBatchRequestEntry.html Maven / Gradle / Ivy
AccountsCustomBatchRequestEntry (Content API for Shopping v2.1-rev20240609-2.0.0)
com.google.api.services.content.model
Class AccountsCustomBatchRequestEntry
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.content.model.AccountsCustomBatchRequestEntry
-
public final class AccountsCustomBatchRequestEntry
extends com.google.api.client.json.GenericJson
A batch entry encoding a single non-batch accounts request.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Content API for Shopping. For a detailed explanation
see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
AccountsCustomBatchRequestEntry()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
AccountsCustomBatchRequestEntry
clone()
Account
getAccount()
The account to create or update.
BigInteger
getAccountId()
The ID of the targeted account.
Long
getBatchId()
An entry ID, unique within the batch request.
Boolean
getForce()
Whether the account should be deleted if the account has offers.
List<BigInteger>
getLabelIds()
Label IDs for the 'updatelabels' request.
AccountsCustomBatchRequestEntryLinkRequest
getLinkRequest()
Details about the `link` request.
BigInteger
getMerchantId()
The ID of the managing account.
String
getMethod()
The method of the batch entry.
Boolean
getOverwrite()
Only applicable if the method is `claimwebsite`.
String
getView()
Controls which fields are visible.
AccountsCustomBatchRequestEntry
set(String fieldName,
Object value)
AccountsCustomBatchRequestEntry
setAccount(Account account)
The account to create or update.
AccountsCustomBatchRequestEntry
setAccountId(BigInteger accountId)
The ID of the targeted account.
AccountsCustomBatchRequestEntry
setBatchId(Long batchId)
An entry ID, unique within the batch request.
AccountsCustomBatchRequestEntry
setForce(Boolean force)
Whether the account should be deleted if the account has offers.
AccountsCustomBatchRequestEntry
setLabelIds(List<BigInteger> labelIds)
Label IDs for the 'updatelabels' request.
AccountsCustomBatchRequestEntry
setLinkRequest(AccountsCustomBatchRequestEntryLinkRequest linkRequest)
Details about the `link` request.
AccountsCustomBatchRequestEntry
setMerchantId(BigInteger merchantId)
The ID of the managing account.
AccountsCustomBatchRequestEntry
setMethod(String method)
The method of the batch entry.
AccountsCustomBatchRequestEntry
setOverwrite(Boolean overwrite)
Only applicable if the method is `claimwebsite`.
AccountsCustomBatchRequestEntry
setView(String view)
Controls which fields are visible.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getAccount
public Account getAccount()
The account to create or update. Only defined if the method is `insert` or `update`.
- Returns:
- value or
null
for none
-
setAccount
public AccountsCustomBatchRequestEntry setAccount(Account account)
The account to create or update. Only defined if the method is `insert` or `update`.
- Parameters:
account
- account or null
for none
-
getAccountId
public BigInteger getAccountId()
The ID of the targeted account. Only defined if the method is not `insert`.
- Returns:
- value or
null
for none
-
setAccountId
public AccountsCustomBatchRequestEntry setAccountId(BigInteger accountId)
The ID of the targeted account. Only defined if the method is not `insert`.
- Parameters:
accountId
- accountId or null
for none
-
getBatchId
public Long getBatchId()
An entry ID, unique within the batch request.
- Returns:
- value or
null
for none
-
setBatchId
public AccountsCustomBatchRequestEntry setBatchId(Long batchId)
An entry ID, unique within the batch request.
- Parameters:
batchId
- batchId or null
for none
-
getForce
public Boolean getForce()
Whether the account should be deleted if the account has offers. Only applicable if the method
is `delete`.
- Returns:
- value or
null
for none
-
setForce
public AccountsCustomBatchRequestEntry setForce(Boolean force)
Whether the account should be deleted if the account has offers. Only applicable if the method
is `delete`.
- Parameters:
force
- force or null
for none
-
getLabelIds
public List<BigInteger> getLabelIds()
Label IDs for the 'updatelabels' request.
- Returns:
- value or
null
for none
-
setLabelIds
public AccountsCustomBatchRequestEntry setLabelIds(List<BigInteger> labelIds)
Label IDs for the 'updatelabels' request.
- Parameters:
labelIds
- labelIds or null
for none
-
getLinkRequest
public AccountsCustomBatchRequestEntryLinkRequest getLinkRequest()
Details about the `link` request.
- Returns:
- value or
null
for none
-
setLinkRequest
public AccountsCustomBatchRequestEntry setLinkRequest(AccountsCustomBatchRequestEntryLinkRequest linkRequest)
Details about the `link` request.
- Parameters:
linkRequest
- linkRequest or null
for none
-
getMerchantId
public BigInteger getMerchantId()
The ID of the managing account.
- Returns:
- value or
null
for none
-
setMerchantId
public AccountsCustomBatchRequestEntry setMerchantId(BigInteger merchantId)
The ID of the managing account.
- Parameters:
merchantId
- merchantId or null
for none
-
getMethod
public String getMethod()
The method of the batch entry. Acceptable values are: - "`claimWebsite`" - "`delete`" - "`get`"
- "`insert`" - "`link`" - "`update`"
- Returns:
- value or
null
for none
-
setMethod
public AccountsCustomBatchRequestEntry setMethod(String method)
The method of the batch entry. Acceptable values are: - "`claimWebsite`" - "`delete`" - "`get`"
- "`insert`" - "`link`" - "`update`"
- Parameters:
method
- method or null
for none
-
getOverwrite
public Boolean getOverwrite()
Only applicable if the method is `claimwebsite`. Indicates whether or not to take the claim
from another account in case there is a conflict.
- Returns:
- value or
null
for none
-
setOverwrite
public AccountsCustomBatchRequestEntry setOverwrite(Boolean overwrite)
Only applicable if the method is `claimwebsite`. Indicates whether or not to take the claim
from another account in case there is a conflict.
- Parameters:
overwrite
- overwrite or null
for none
-
getView
public String getView()
Controls which fields are visible. Only applicable if the method is 'get'.
- Returns:
- value or
null
for none
-
setView
public AccountsCustomBatchRequestEntry setView(String view)
Controls which fields are visible. Only applicable if the method is 'get'.
- Parameters:
view
- view or null
for none
-
set
public AccountsCustomBatchRequestEntry set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public AccountsCustomBatchRequestEntry clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy