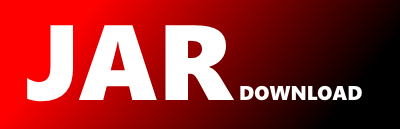
target.apidocs.com.google.api.services.content.model.Order.html Maven / Gradle / Ivy
Order (Content API for Shopping v2.1-rev20240609-2.0.0)
com.google.api.services.content.model
Class Order
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.content.model.Order
-
public final class Order
extends com.google.api.client.json.GenericJson
Order. Production access (all methods) requires the order manager role. Sandbox access does not.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Content API for Shopping. For a detailed explanation
see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
Order()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
Order
clone()
Boolean
getAcknowledged()
Whether the order was acknowledged.
List<OrderOrderAnnotation>
getAnnotations()
List of key-value pairs that are attached to a given order.
OrderAddress
getBillingAddress()
The billing address.
OrderCustomer
getCustomer()
The details of the customer who placed the order.
OrderDeliveryDetails
getDeliveryDetails()
Delivery details for shipments of type `delivery`.
String
getId()
The REST ID of the order.
String
getKind()
Identifies what kind of resource this is.
List<OrderLineItem>
getLineItems()
Line items that are ordered.
BigInteger
getMerchantId()
String
getMerchantOrderId()
Merchant-provided ID of the order.
Price
getNetPriceAmount()
The net amount for the order (price part).
Price
getNetTaxAmount()
The net amount for the order (tax part).
String
getPaymentStatus()
The status of the payment.
OrderPickupDetails
getPickupDetails()
Pickup details for shipments of type `pickup`.
String
getPlacedDate()
The date when the order was placed, in ISO 8601 format.
List<OrderPromotion>
getPromotions()
Promotions associated with the order.
List<OrderRefund>
getRefunds()
Refunds for the order.
List<OrderShipment>
getShipments()
Shipments of the order.
Price
getShippingCost()
The total cost of shipping for all items.
Price
getShippingCostTax()
The tax for the total shipping cost.
String
getStatus()
The status of the order.
String
getTaxCollector()
The party responsible for collecting and remitting taxes.
Order
set(String fieldName,
Object value)
Order
setAcknowledged(Boolean acknowledged)
Whether the order was acknowledged.
Order
setAnnotations(List<OrderOrderAnnotation> annotations)
List of key-value pairs that are attached to a given order.
Order
setBillingAddress(OrderAddress billingAddress)
The billing address.
Order
setCustomer(OrderCustomer customer)
The details of the customer who placed the order.
Order
setDeliveryDetails(OrderDeliveryDetails deliveryDetails)
Delivery details for shipments of type `delivery`.
Order
setId(String id)
The REST ID of the order.
Order
setKind(String kind)
Identifies what kind of resource this is.
Order
setLineItems(List<OrderLineItem> lineItems)
Line items that are ordered.
Order
setMerchantId(BigInteger merchantId)
Order
setMerchantOrderId(String merchantOrderId)
Merchant-provided ID of the order.
Order
setNetPriceAmount(Price netPriceAmount)
The net amount for the order (price part).
Order
setNetTaxAmount(Price netTaxAmount)
The net amount for the order (tax part).
Order
setPaymentStatus(String paymentStatus)
The status of the payment.
Order
setPickupDetails(OrderPickupDetails pickupDetails)
Pickup details for shipments of type `pickup`.
Order
setPlacedDate(String placedDate)
The date when the order was placed, in ISO 8601 format.
Order
setPromotions(List<OrderPromotion> promotions)
Promotions associated with the order.
Order
setRefunds(List<OrderRefund> refunds)
Refunds for the order.
Order
setShipments(List<OrderShipment> shipments)
Shipments of the order.
Order
setShippingCost(Price shippingCost)
The total cost of shipping for all items.
Order
setShippingCostTax(Price shippingCostTax)
The tax for the total shipping cost.
Order
setStatus(String status)
The status of the order.
Order
setTaxCollector(String taxCollector)
The party responsible for collecting and remitting taxes.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getAcknowledged
public Boolean getAcknowledged()
Whether the order was acknowledged.
- Returns:
- value or
null
for none
-
setAcknowledged
public Order setAcknowledged(Boolean acknowledged)
Whether the order was acknowledged.
- Parameters:
acknowledged
- acknowledged or null
for none
-
getAnnotations
public List<OrderOrderAnnotation> getAnnotations()
List of key-value pairs that are attached to a given order.
- Returns:
- value or
null
for none
-
setAnnotations
public Order setAnnotations(List<OrderOrderAnnotation> annotations)
List of key-value pairs that are attached to a given order.
- Parameters:
annotations
- annotations or null
for none
-
getBillingAddress
public OrderAddress getBillingAddress()
The billing address.
- Returns:
- value or
null
for none
-
setBillingAddress
public Order setBillingAddress(OrderAddress billingAddress)
The billing address.
- Parameters:
billingAddress
- billingAddress or null
for none
-
getCustomer
public OrderCustomer getCustomer()
The details of the customer who placed the order.
- Returns:
- value or
null
for none
-
setCustomer
public Order setCustomer(OrderCustomer customer)
The details of the customer who placed the order.
- Parameters:
customer
- customer or null
for none
-
getDeliveryDetails
public OrderDeliveryDetails getDeliveryDetails()
Delivery details for shipments of type `delivery`.
- Returns:
- value or
null
for none
-
setDeliveryDetails
public Order setDeliveryDetails(OrderDeliveryDetails deliveryDetails)
Delivery details for shipments of type `delivery`.
- Parameters:
deliveryDetails
- deliveryDetails or null
for none
-
getId
public String getId()
The REST ID of the order. Globally unique.
- Returns:
- value or
null
for none
-
setId
public Order setId(String id)
The REST ID of the order. Globally unique.
- Parameters:
id
- id or null
for none
-
getKind
public String getKind()
Identifies what kind of resource this is. Value: the fixed string "`content#order`"
- Returns:
- value or
null
for none
-
setKind
public Order setKind(String kind)
Identifies what kind of resource this is. Value: the fixed string "`content#order`"
- Parameters:
kind
- kind or null
for none
-
getLineItems
public List<OrderLineItem> getLineItems()
Line items that are ordered.
- Returns:
- value or
null
for none
-
setLineItems
public Order setLineItems(List<OrderLineItem> lineItems)
Line items that are ordered.
- Parameters:
lineItems
- lineItems or null
for none
-
getMerchantId
public BigInteger getMerchantId()
- Returns:
- value or
null
for none
-
setMerchantId
public Order setMerchantId(BigInteger merchantId)
- Parameters:
merchantId
- merchantId or null
for none
-
getMerchantOrderId
public String getMerchantOrderId()
Merchant-provided ID of the order.
- Returns:
- value or
null
for none
-
setMerchantOrderId
public Order setMerchantOrderId(String merchantOrderId)
Merchant-provided ID of the order.
- Parameters:
merchantOrderId
- merchantOrderId or null
for none
-
getNetPriceAmount
public Price getNetPriceAmount()
The net amount for the order (price part). For example, if an order was originally for $100 and
a refund was issued for $20, the net amount will be $80.
- Returns:
- value or
null
for none
-
setNetPriceAmount
public Order setNetPriceAmount(Price netPriceAmount)
The net amount for the order (price part). For example, if an order was originally for $100 and
a refund was issued for $20, the net amount will be $80.
- Parameters:
netPriceAmount
- netPriceAmount or null
for none
-
getNetTaxAmount
public Price getNetTaxAmount()
The net amount for the order (tax part). Note that in certain cases due to taxable base
adjustment `netTaxAmount` might not match to a sum of tax field across all lineItems and
refunds.
- Returns:
- value or
null
for none
-
setNetTaxAmount
public Order setNetTaxAmount(Price netTaxAmount)
The net amount for the order (tax part). Note that in certain cases due to taxable base
adjustment `netTaxAmount` might not match to a sum of tax field across all lineItems and
refunds.
- Parameters:
netTaxAmount
- netTaxAmount or null
for none
-
getPaymentStatus
public String getPaymentStatus()
The status of the payment. Acceptable values are: - "`paymentCaptured`" - "`paymentRejected`" -
"`paymentSecured`" - "`pendingAuthorization`"
- Returns:
- value or
null
for none
-
setPaymentStatus
public Order setPaymentStatus(String paymentStatus)
The status of the payment. Acceptable values are: - "`paymentCaptured`" - "`paymentRejected`" -
"`paymentSecured`" - "`pendingAuthorization`"
- Parameters:
paymentStatus
- paymentStatus or null
for none
-
getPickupDetails
public OrderPickupDetails getPickupDetails()
Pickup details for shipments of type `pickup`.
- Returns:
- value or
null
for none
-
setPickupDetails
public Order setPickupDetails(OrderPickupDetails pickupDetails)
Pickup details for shipments of type `pickup`.
- Parameters:
pickupDetails
- pickupDetails or null
for none
-
getPlacedDate
public String getPlacedDate()
The date when the order was placed, in ISO 8601 format.
- Returns:
- value or
null
for none
-
setPlacedDate
public Order setPlacedDate(String placedDate)
The date when the order was placed, in ISO 8601 format.
- Parameters:
placedDate
- placedDate or null
for none
-
getPromotions
public List<OrderPromotion> getPromotions()
Promotions associated with the order. To determine which promotions apply to which products,
check the `Promotions[].appliedItems[].lineItemId` field against the `LineItems[].id` field for
each promotion. If a promotion is applied to more than 1 offerId, divide the discount value by
the number of affected offers to determine how much discount to apply to each offerId.
Examples: 1. To calculate price paid by the customer for a single line item including the
discount: For each promotion, subtract the `LineItems[].adjustments[].priceAdjustment.value`
amount from the `LineItems[].Price.value`. 2. To calculate price paid by the customer for a
single line item including the discount in case of multiple quantity: For each promotion,
divide the `LineItems[].adjustments[].priceAdjustment.value` by the quantity of products then
subtract the resulting value from the `LineItems[].Product.Price.value` for each quantity item.
Only 1 promotion can be applied to an offerId in a given order. To refund an item which had a
promotion applied to it, make sure to refund the amount after first subtracting the promotion
discount from the item price. More details about the program are here.
- Returns:
- value or
null
for none
-
setPromotions
public Order setPromotions(List<OrderPromotion> promotions)
Promotions associated with the order. To determine which promotions apply to which products,
check the `Promotions[].appliedItems[].lineItemId` field against the `LineItems[].id` field for
each promotion. If a promotion is applied to more than 1 offerId, divide the discount value by
the number of affected offers to determine how much discount to apply to each offerId.
Examples: 1. To calculate price paid by the customer for a single line item including the
discount: For each promotion, subtract the `LineItems[].adjustments[].priceAdjustment.value`
amount from the `LineItems[].Price.value`. 2. To calculate price paid by the customer for a
single line item including the discount in case of multiple quantity: For each promotion,
divide the `LineItems[].adjustments[].priceAdjustment.value` by the quantity of products then
subtract the resulting value from the `LineItems[].Product.Price.value` for each quantity item.
Only 1 promotion can be applied to an offerId in a given order. To refund an item which had a
promotion applied to it, make sure to refund the amount after first subtracting the promotion
discount from the item price. More details about the program are here.
- Parameters:
promotions
- promotions or null
for none
-
getRefunds
public List<OrderRefund> getRefunds()
Refunds for the order.
- Returns:
- value or
null
for none
-
setRefunds
public Order setRefunds(List<OrderRefund> refunds)
Refunds for the order.
- Parameters:
refunds
- refunds or null
for none
-
getShipments
public List<OrderShipment> getShipments()
Shipments of the order.
- Returns:
- value or
null
for none
-
setShipments
public Order setShipments(List<OrderShipment> shipments)
Shipments of the order.
- Parameters:
shipments
- shipments or null
for none
-
getShippingCost
public Price getShippingCost()
The total cost of shipping for all items.
- Returns:
- value or
null
for none
-
setShippingCost
public Order setShippingCost(Price shippingCost)
The total cost of shipping for all items.
- Parameters:
shippingCost
- shippingCost or null
for none
-
getShippingCostTax
public Price getShippingCostTax()
The tax for the total shipping cost.
- Returns:
- value or
null
for none
-
setShippingCostTax
public Order setShippingCostTax(Price shippingCostTax)
The tax for the total shipping cost.
- Parameters:
shippingCostTax
- shippingCostTax or null
for none
-
getStatus
public String getStatus()
The status of the order. Acceptable values are: - "`canceled`" - "`delivered`" - "`inProgress`"
- "`partiallyDelivered`" - "`partiallyReturned`" - "`partiallyShipped`" - "`pendingShipment`" -
"`returned`" - "`shipped`"
- Returns:
- value or
null
for none
-
setStatus
public Order setStatus(String status)
The status of the order. Acceptable values are: - "`canceled`" - "`delivered`" - "`inProgress`"
- "`partiallyDelivered`" - "`partiallyReturned`" - "`partiallyShipped`" - "`pendingShipment`" -
"`returned`" - "`shipped`"
- Parameters:
status
- status or null
for none
-
getTaxCollector
public String getTaxCollector()
The party responsible for collecting and remitting taxes. Acceptable values are: -
"`marketplaceFacilitator`" - "`merchant`"
- Returns:
- value or
null
for none
-
setTaxCollector
public Order setTaxCollector(String taxCollector)
The party responsible for collecting and remitting taxes. Acceptable values are: -
"`marketplaceFacilitator`" - "`merchant`"
- Parameters:
taxCollector
- taxCollector or null
for none
-
set
public Order set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public Order clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy