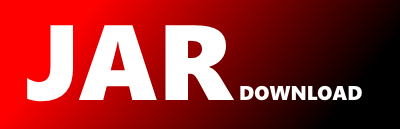
target.apidocs.com.google.api.services.drive.model.File.html Maven / Gradle / Ivy
File (Google Drive API v2-rev20240809-2.0.0)
com.google.api.services.drive.model
Class File
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.drive.model.File
-
public final class File
extends com.google.api.client.json.GenericJson
The metadata for a file. Some resource methods (such as `files.update`) require a `fileId`. Use
the `files.list` method to retrieve the ID for a file.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Google Drive API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
Nested Classes
Modifier and Type
Class and Description
static class
File.Capabilities
Output only.
static class
File.ImageMediaMetadata
Output only.
static class
File.IndexableText
Indexable text attributes for the file (can only be written)
static class
File.LabelInfo
Output only.
static class
File.Labels
A group of labels for the file.
static class
File.LinkShareMetadata
Contains details about the link URLs that clients are using to refer to this item.
static class
File.ShortcutDetails
Shortcut file details.
static class
File.Thumbnail
A thumbnail for the file.
static class
File.VideoMediaMetadata
Output only.
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
File()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
File
clone()
String
getAlternateLink()
Output only.
Boolean
getAppDataContents()
Output only.
Boolean
getCanComment()
Output only.
Boolean
getCanReadRevisions()
Output only.
File.Capabilities
getCapabilities()
Output only.
List<ContentRestriction>
getContentRestrictions()
Restrictions for accessing the content of the file.
Boolean
getCopyable()
Output only.
Boolean
getCopyRequiresWriterPermission()
Whether the options to copy, print, or download this file, should be disabled for readers and
commenters.
com.google.api.client.util.DateTime
getCreatedDate()
Create time for this file (formatted RFC 3339 timestamp).
String
getDefaultOpenWithLink()
Output only.
String
getDescription()
A short description of the file.
String
getDownloadUrl()
Output only.
String
getDriveId()
Output only.
Boolean
getEditable()
Output only.
String
getEmbedLink()
Output only.
String
getEtag()
Output only.
Boolean
getExplicitlyTrashed()
Output only.
Map<String,String>
getExportLinks()
Output only.
String
getFileExtension()
Output only.
Long
getFileSize()
Output only.
String
getFolderColorRgb()
Folder color as an RGB hex string if the file is a folder or a shortcut to a folder.
String
getFullFileExtension()
Output only.
Boolean
getHasAugmentedPermissions()
Output only.
Boolean
getHasThumbnail()
Output only.
String
getHeadRevisionId()
Output only.
String
getIconLink()
Output only.
String
getId()
The ID of the file.
File.ImageMediaMetadata
getImageMediaMetadata()
Output only.
File.IndexableText
getIndexableText()
Indexable text attributes for the file (can only be written)
Boolean
getIsAppAuthorized()
Output only.
String
getKind()
Output only.
File.LabelInfo
getLabelInfo()
Output only.
File.Labels
getLabels()
A group of labels for the file.
User
getLastModifyingUser()
Output only.
String
getLastModifyingUserName()
Output only.
com.google.api.client.util.DateTime
getLastViewedByMeDate()
Last time this file was viewed by the user (formatted RFC 3339 timestamp).
File.LinkShareMetadata
getLinkShareMetadata()
Contains details about the link URLs that clients are using to refer to this item.
com.google.api.client.util.DateTime
getMarkedViewedByMeDate()
Deprecated.
String
getMd5Checksum()
Output only.
String
getMimeType()
The MIME type of the file.
com.google.api.client.util.DateTime
getModifiedByMeDate()
Last time this file was modified by the user (formatted RFC 3339 timestamp).
com.google.api.client.util.DateTime
getModifiedDate()
Last time this file was modified by anyone (formatted RFC 3339 timestamp).
Map<String,String>
getOpenWithLinks()
Output only.
String
getOriginalFilename()
The original filename of the uploaded content if available, or else the original value of the
`title` field.
Boolean
getOwnedByMe()
Output only.
List<String>
getOwnerNames()
Output only.
List<User>
getOwners()
Output only.
List<ParentReference>
getParents()
The ID of the parent folder containing the file.
List<String>
getPermissionIds()
Output only.
List<Permission>
getPermissions()
Output only.
List<Property>
getProperties()
The list of properties.
Long
getQuotaBytesUsed()
Output only.
String
getResourceKey()
Output only.
String
getSelfLink()
Output only.
String
getSha1Checksum()
Output only.
String
getSha256Checksum()
Output only.
Boolean
getShareable()
Output only.
Boolean
getShared()
Output only.
com.google.api.client.util.DateTime
getSharedWithMeDate()
Time at which this file was shared with the user (formatted RFC 3339 timestamp).
User
getSharingUser()
Output only.
File.ShortcutDetails
getShortcutDetails()
Shortcut file details.
List<String>
getSpaces()
Output only.
String
getTeamDriveId()
Output only.
File.Thumbnail
getThumbnail()
A thumbnail for the file.
String
getThumbnailLink()
Output only.
Long
getThumbnailVersion()
Output only.
String
getTitle()
The title of this file.
com.google.api.client.util.DateTime
getTrashedDate()
The time that the item was trashed (formatted RFC 3339 timestamp).
User
getTrashingUser()
Output only.
Permission
getUserPermission()
Output only.
Long
getVersion()
Output only.
File.VideoMediaMetadata
getVideoMediaMetadata()
Output only.
String
getWebContentLink()
Output only.
String
getWebViewLink()
Output only.
Boolean
getWritersCanShare()
Whether writers can share the document with other users.
File
set(String fieldName,
Object value)
File
setAlternateLink(String alternateLink)
Output only.
File
setAppDataContents(Boolean appDataContents)
Output only.
File
setCanComment(Boolean canComment)
Output only.
File
setCanReadRevisions(Boolean canReadRevisions)
Output only.
File
setCapabilities(File.Capabilities capabilities)
Output only.
File
setContentRestrictions(List<ContentRestriction> contentRestrictions)
Restrictions for accessing the content of the file.
File
setCopyable(Boolean copyable)
Output only.
File
setCopyRequiresWriterPermission(Boolean copyRequiresWriterPermission)
Whether the options to copy, print, or download this file, should be disabled for readers and
commenters.
File
setCreatedDate(com.google.api.client.util.DateTime createdDate)
Create time for this file (formatted RFC 3339 timestamp).
File
setDefaultOpenWithLink(String defaultOpenWithLink)
Output only.
File
setDescription(String description)
A short description of the file.
File
setDownloadUrl(String downloadUrl)
Output only.
File
setDriveId(String driveId)
Output only.
File
setEditable(Boolean editable)
Output only.
File
setEmbedLink(String embedLink)
Output only.
File
setEtag(String etag)
Output only.
File
setExplicitlyTrashed(Boolean explicitlyTrashed)
Output only.
File
setExportLinks(Map<String,String> exportLinks)
Output only.
File
setFileExtension(String fileExtension)
Output only.
File
setFileSize(Long fileSize)
Output only.
File
setFolderColorRgb(String folderColorRgb)
Folder color as an RGB hex string if the file is a folder or a shortcut to a folder.
File
setFullFileExtension(String fullFileExtension)
Output only.
File
setHasAugmentedPermissions(Boolean hasAugmentedPermissions)
Output only.
File
setHasThumbnail(Boolean hasThumbnail)
Output only.
File
setHeadRevisionId(String headRevisionId)
Output only.
File
setIconLink(String iconLink)
Output only.
File
setId(String id)
The ID of the file.
File
setImageMediaMetadata(File.ImageMediaMetadata imageMediaMetadata)
Output only.
File
setIndexableText(File.IndexableText indexableText)
Indexable text attributes for the file (can only be written)
File
setIsAppAuthorized(Boolean isAppAuthorized)
Output only.
File
setKind(String kind)
Output only.
File
setLabelInfo(File.LabelInfo labelInfo)
Output only.
File
setLabels(File.Labels labels)
A group of labels for the file.
File
setLastModifyingUser(User lastModifyingUser)
Output only.
File
setLastModifyingUserName(String lastModifyingUserName)
Output only.
File
setLastViewedByMeDate(com.google.api.client.util.DateTime lastViewedByMeDate)
Last time this file was viewed by the user (formatted RFC 3339 timestamp).
File
setLinkShareMetadata(File.LinkShareMetadata linkShareMetadata)
Contains details about the link URLs that clients are using to refer to this item.
File
setMarkedViewedByMeDate(com.google.api.client.util.DateTime markedViewedByMeDate)
Deprecated.
File
setMd5Checksum(String md5Checksum)
Output only.
File
setMimeType(String mimeType)
The MIME type of the file.
File
setModifiedByMeDate(com.google.api.client.util.DateTime modifiedByMeDate)
Last time this file was modified by the user (formatted RFC 3339 timestamp).
File
setModifiedDate(com.google.api.client.util.DateTime modifiedDate)
Last time this file was modified by anyone (formatted RFC 3339 timestamp).
File
setOpenWithLinks(Map<String,String> openWithLinks)
Output only.
File
setOriginalFilename(String originalFilename)
The original filename of the uploaded content if available, or else the original value of the
`title` field.
File
setOwnedByMe(Boolean ownedByMe)
Output only.
File
setOwnerNames(List<String> ownerNames)
Output only.
File
setOwners(List<User> owners)
Output only.
File
setParents(List<ParentReference> parents)
The ID of the parent folder containing the file.
File
setPermissionIds(List<String> permissionIds)
Output only.
File
setPermissions(List<Permission> permissions)
Output only.
File
setProperties(List<Property> properties)
The list of properties.
File
setQuotaBytesUsed(Long quotaBytesUsed)
Output only.
File
setResourceKey(String resourceKey)
Output only.
File
setSelfLink(String selfLink)
Output only.
File
setSha1Checksum(String sha1Checksum)
Output only.
File
setSha256Checksum(String sha256Checksum)
Output only.
File
setShareable(Boolean shareable)
Output only.
File
setShared(Boolean shared)
Output only.
File
setSharedWithMeDate(com.google.api.client.util.DateTime sharedWithMeDate)
Time at which this file was shared with the user (formatted RFC 3339 timestamp).
File
setSharingUser(User sharingUser)
Output only.
File
setShortcutDetails(File.ShortcutDetails shortcutDetails)
Shortcut file details.
File
setSpaces(List<String> spaces)
Output only.
File
setTeamDriveId(String teamDriveId)
Output only.
File
setThumbnail(File.Thumbnail thumbnail)
A thumbnail for the file.
File
setThumbnailLink(String thumbnailLink)
Output only.
File
setThumbnailVersion(Long thumbnailVersion)
Output only.
File
setTitle(String title)
The title of this file.
File
setTrashedDate(com.google.api.client.util.DateTime trashedDate)
The time that the item was trashed (formatted RFC 3339 timestamp).
File
setTrashingUser(User trashingUser)
Output only.
File
setUserPermission(Permission userPermission)
Output only.
File
setVersion(Long version)
Output only.
File
setVideoMediaMetadata(File.VideoMediaMetadata videoMediaMetadata)
Output only.
File
setWebContentLink(String webContentLink)
Output only.
File
setWebViewLink(String webViewLink)
Output only.
File
setWritersCanShare(Boolean writersCanShare)
Whether writers can share the document with other users.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getAlternateLink
public String getAlternateLink()
Output only. A link for opening the file in a relevant Google editor or viewer.
- Returns:
- value or
null
for none
-
setAlternateLink
public File setAlternateLink(String alternateLink)
Output only. A link for opening the file in a relevant Google editor or viewer.
- Parameters:
alternateLink
- alternateLink or null
for none
-
getAppDataContents
public Boolean getAppDataContents()
Output only. Whether this file is in the Application Data folder.
- Returns:
- value or
null
for none
-
setAppDataContents
public File setAppDataContents(Boolean appDataContents)
Output only. Whether this file is in the Application Data folder.
- Parameters:
appDataContents
- appDataContents or null
for none
-
getCanComment
public Boolean getCanComment()
Output only. Deprecated: Use `capabilities/canComment` instead.
- Returns:
- value or
null
for none
-
setCanComment
public File setCanComment(Boolean canComment)
Output only. Deprecated: Use `capabilities/canComment` instead.
- Parameters:
canComment
- canComment or null
for none
-
getCanReadRevisions
public Boolean getCanReadRevisions()
Output only. Deprecated: Use `capabilities/canReadRevisions` instead.
- Returns:
- value or
null
for none
-
setCanReadRevisions
public File setCanReadRevisions(Boolean canReadRevisions)
Output only. Deprecated: Use `capabilities/canReadRevisions` instead.
- Parameters:
canReadRevisions
- canReadRevisions or null
for none
-
getCapabilities
public File.Capabilities getCapabilities()
Output only. Capabilities the current user has on this file. Each capability corresponds to a
fine-grained action that a user may take.
- Returns:
- value or
null
for none
-
setCapabilities
public File setCapabilities(File.Capabilities capabilities)
Output only. Capabilities the current user has on this file. Each capability corresponds to a
fine-grained action that a user may take.
- Parameters:
capabilities
- capabilities or null
for none
-
getContentRestrictions
public List<ContentRestriction> getContentRestrictions()
Restrictions for accessing the content of the file. Only populated if such a restriction
exists.
- Returns:
- value or
null
for none
-
setContentRestrictions
public File setContentRestrictions(List<ContentRestriction> contentRestrictions)
Restrictions for accessing the content of the file. Only populated if such a restriction
exists.
- Parameters:
contentRestrictions
- contentRestrictions or null
for none
-
getCopyRequiresWriterPermission
public Boolean getCopyRequiresWriterPermission()
Whether the options to copy, print, or download this file, should be disabled for readers and
commenters.
- Returns:
- value or
null
for none
-
setCopyRequiresWriterPermission
public File setCopyRequiresWriterPermission(Boolean copyRequiresWriterPermission)
Whether the options to copy, print, or download this file, should be disabled for readers and
commenters.
- Parameters:
copyRequiresWriterPermission
- copyRequiresWriterPermission or null
for none
-
getCopyable
public Boolean getCopyable()
Output only. Deprecated: Use `capabilities/canCopy` instead.
- Returns:
- value or
null
for none
-
setCopyable
public File setCopyable(Boolean copyable)
Output only. Deprecated: Use `capabilities/canCopy` instead.
- Parameters:
copyable
- copyable or null
for none
-
getCreatedDate
public com.google.api.client.util.DateTime getCreatedDate()
Create time for this file (formatted RFC 3339 timestamp).
- Returns:
- value or
null
for none
-
setCreatedDate
public File setCreatedDate(com.google.api.client.util.DateTime createdDate)
Create time for this file (formatted RFC 3339 timestamp).
- Parameters:
createdDate
- createdDate or null
for none
-
getDefaultOpenWithLink
public String getDefaultOpenWithLink()
Output only. A link to open this file with the user's default app for this file. Only populated
when the drive.apps.readonly scope is used.
- Returns:
- value or
null
for none
-
setDefaultOpenWithLink
public File setDefaultOpenWithLink(String defaultOpenWithLink)
Output only. A link to open this file with the user's default app for this file. Only populated
when the drive.apps.readonly scope is used.
- Parameters:
defaultOpenWithLink
- defaultOpenWithLink or null
for none
-
getDescription
public String getDescription()
A short description of the file.
- Returns:
- value or
null
for none
-
setDescription
public File setDescription(String description)
A short description of the file.
- Parameters:
description
- description or null
for none
-
getDownloadUrl
public String getDownloadUrl()
Output only. Short lived download URL for the file. This field is only populated for files with
content stored in Google Drive; it is not populated for Google Docs or shortcut files.
- Returns:
- value or
null
for none
-
setDownloadUrl
public File setDownloadUrl(String downloadUrl)
Output only. Short lived download URL for the file. This field is only populated for files with
content stored in Google Drive; it is not populated for Google Docs or shortcut files.
- Parameters:
downloadUrl
- downloadUrl or null
for none
-
getDriveId
public String getDriveId()
Output only. ID of the shared drive the file resides in. Only populated for items in shared
drives.
- Returns:
- value or
null
for none
-
setDriveId
public File setDriveId(String driveId)
Output only. ID of the shared drive the file resides in. Only populated for items in shared
drives.
- Parameters:
driveId
- driveId or null
for none
-
getEditable
public Boolean getEditable()
Output only. Deprecated: Use `capabilities/canEdit` instead.
- Returns:
- value or
null
for none
-
setEditable
public File setEditable(Boolean editable)
Output only. Deprecated: Use `capabilities/canEdit` instead.
- Parameters:
editable
- editable or null
for none
-
getEmbedLink
public String getEmbedLink()
Output only. A link for embedding the file.
- Returns:
- value or
null
for none
-
setEmbedLink
public File setEmbedLink(String embedLink)
Output only. A link for embedding the file.
- Parameters:
embedLink
- embedLink or null
for none
-
getEtag
public String getEtag()
Output only. ETag of the file.
- Returns:
- value or
null
for none
-
setEtag
public File setEtag(String etag)
Output only. ETag of the file.
- Parameters:
etag
- etag or null
for none
-
getExplicitlyTrashed
public Boolean getExplicitlyTrashed()
Output only. Whether this file has been explicitly trashed, as opposed to recursively trashed.
- Returns:
- value or
null
for none
-
setExplicitlyTrashed
public File setExplicitlyTrashed(Boolean explicitlyTrashed)
Output only. Whether this file has been explicitly trashed, as opposed to recursively trashed.
- Parameters:
explicitlyTrashed
- explicitlyTrashed or null
for none
-
getExportLinks
public Map<String,String> getExportLinks()
Output only. Links for exporting Docs Editors files to specific formats.
- Returns:
- value or
null
for none
-
setExportLinks
public File setExportLinks(Map<String,String> exportLinks)
Output only. Links for exporting Docs Editors files to specific formats.
- Parameters:
exportLinks
- exportLinks or null
for none
-
getFileExtension
public String getFileExtension()
Output only. The final component of `fullFileExtension` with trailing text that does not appear
to be part of the extension removed. This field is only populated for files with content stored
in Google Drive; it is not populated for Docs Editors or shortcut files.
- Returns:
- value or
null
for none
-
setFileExtension
public File setFileExtension(String fileExtension)
Output only. The final component of `fullFileExtension` with trailing text that does not appear
to be part of the extension removed. This field is only populated for files with content stored
in Google Drive; it is not populated for Docs Editors or shortcut files.
- Parameters:
fileExtension
- fileExtension or null
for none
-
getFileSize
public Long getFileSize()
Output only. Size in bytes of blobs and first party editor files. Won't be populated for files
that have no size, like shortcuts and folders.
- Returns:
- value or
null
for none
-
setFileSize
public File setFileSize(Long fileSize)
Output only. Size in bytes of blobs and first party editor files. Won't be populated for files
that have no size, like shortcuts and folders.
- Parameters:
fileSize
- fileSize or null
for none
-
getFolderColorRgb
public String getFolderColorRgb()
Folder color as an RGB hex string if the file is a folder or a shortcut to a folder. The list
of supported colors is available in the folderColorPalette field of the About resource. If an
unsupported color is specified, it will be changed to the closest color in the palette.
- Returns:
- value or
null
for none
-
setFolderColorRgb
public File setFolderColorRgb(String folderColorRgb)
Folder color as an RGB hex string if the file is a folder or a shortcut to a folder. The list
of supported colors is available in the folderColorPalette field of the About resource. If an
unsupported color is specified, it will be changed to the closest color in the palette.
- Parameters:
folderColorRgb
- folderColorRgb or null
for none
-
getFullFileExtension
public String getFullFileExtension()
Output only. The full file extension; extracted from the title. May contain multiple
concatenated extensions, such as "tar.gz". Removing an extension from the title does not clear
this field; however, changing the extension on the title does update this field. This field is
only populated for files with content stored in Google Drive; it is not populated for Docs
Editors or shortcut files.
- Returns:
- value or
null
for none
-
setFullFileExtension
public File setFullFileExtension(String fullFileExtension)
Output only. The full file extension; extracted from the title. May contain multiple
concatenated extensions, such as "tar.gz". Removing an extension from the title does not clear
this field; however, changing the extension on the title does update this field. This field is
only populated for files with content stored in Google Drive; it is not populated for Docs
Editors or shortcut files.
- Parameters:
fullFileExtension
- fullFileExtension or null
for none
-
getHasAugmentedPermissions
public Boolean getHasAugmentedPermissions()
Output only. Whether there are permissions directly on this file. This field is only populated
for items in shared drives.
- Returns:
- value or
null
for none
-
setHasAugmentedPermissions
public File setHasAugmentedPermissions(Boolean hasAugmentedPermissions)
Output only. Whether there are permissions directly on this file. This field is only populated
for items in shared drives.
- Parameters:
hasAugmentedPermissions
- hasAugmentedPermissions or null
for none
-
getHasThumbnail
public Boolean getHasThumbnail()
Output only. Whether this file has a thumbnail. This does not indicate whether the requesting
app has access to the thumbnail. To check access, look for the presence of the thumbnailLink
field.
- Returns:
- value or
null
for none
-
setHasThumbnail
public File setHasThumbnail(Boolean hasThumbnail)
Output only. Whether this file has a thumbnail. This does not indicate whether the requesting
app has access to the thumbnail. To check access, look for the presence of the thumbnailLink
field.
- Parameters:
hasThumbnail
- hasThumbnail or null
for none
-
getHeadRevisionId
public String getHeadRevisionId()
Output only. The ID of the file's head revision. This field is only populated for files with
content stored in Google Drive; it is not populated for Docs Editors or shortcut files.
- Returns:
- value or
null
for none
-
setHeadRevisionId
public File setHeadRevisionId(String headRevisionId)
Output only. The ID of the file's head revision. This field is only populated for files with
content stored in Google Drive; it is not populated for Docs Editors or shortcut files.
- Parameters:
headRevisionId
- headRevisionId or null
for none
-
getIconLink
public String getIconLink()
Output only. A link to the file's icon.
- Returns:
- value or
null
for none
-
setIconLink
public File setIconLink(String iconLink)
Output only. A link to the file's icon.
- Parameters:
iconLink
- iconLink or null
for none
-
getId
public String getId()
The ID of the file.
- Returns:
- value or
null
for none
-
getImageMediaMetadata
public File.ImageMediaMetadata getImageMediaMetadata()
Output only. Metadata about image media. This will only be present for image types, and its
contents will depend on what can be parsed from the image content.
- Returns:
- value or
null
for none
-
setImageMediaMetadata
public File setImageMediaMetadata(File.ImageMediaMetadata imageMediaMetadata)
Output only. Metadata about image media. This will only be present for image types, and its
contents will depend on what can be parsed from the image content.
- Parameters:
imageMediaMetadata
- imageMediaMetadata or null
for none
-
getIndexableText
public File.IndexableText getIndexableText()
Indexable text attributes for the file (can only be written)
- Returns:
- value or
null
for none
-
setIndexableText
public File setIndexableText(File.IndexableText indexableText)
Indexable text attributes for the file (can only be written)
- Parameters:
indexableText
- indexableText or null
for none
-
getIsAppAuthorized
public Boolean getIsAppAuthorized()
Output only. Whether the file was created or opened by the requesting app.
- Returns:
- value or
null
for none
-
setIsAppAuthorized
public File setIsAppAuthorized(Boolean isAppAuthorized)
Output only. Whether the file was created or opened by the requesting app.
- Parameters:
isAppAuthorized
- isAppAuthorized or null
for none
-
getKind
public String getKind()
Output only. The type of file. This is always `drive#file`.
- Returns:
- value or
null
for none
-
setKind
public File setKind(String kind)
Output only. The type of file. This is always `drive#file`.
- Parameters:
kind
- kind or null
for none
-
getLabelInfo
public File.LabelInfo getLabelInfo()
Output only. An overview of the labels on the file.
- Returns:
- value or
null
for none
-
setLabelInfo
public File setLabelInfo(File.LabelInfo labelInfo)
Output only. An overview of the labels on the file.
- Parameters:
labelInfo
- labelInfo or null
for none
-
getLabels
public File.Labels getLabels()
A group of labels for the file.
- Returns:
- value or
null
for none
-
setLabels
public File setLabels(File.Labels labels)
A group of labels for the file.
- Parameters:
labels
- labels or null
for none
-
getLastModifyingUser
public User getLastModifyingUser()
Output only. The last user to modify this file. This field is only populated when the last
modification was performed by a signed-in user.
- Returns:
- value or
null
for none
-
setLastModifyingUser
public File setLastModifyingUser(User lastModifyingUser)
Output only. The last user to modify this file. This field is only populated when the last
modification was performed by a signed-in user.
- Parameters:
lastModifyingUser
- lastModifyingUser or null
for none
-
getLastModifyingUserName
public String getLastModifyingUserName()
Output only. Name of the last user to modify this file.
- Returns:
- value or
null
for none
-
setLastModifyingUserName
public File setLastModifyingUserName(String lastModifyingUserName)
Output only. Name of the last user to modify this file.
- Parameters:
lastModifyingUserName
- lastModifyingUserName or null
for none
-
getLastViewedByMeDate
public com.google.api.client.util.DateTime getLastViewedByMeDate()
Last time this file was viewed by the user (formatted RFC 3339 timestamp).
- Returns:
- value or
null
for none
-
setLastViewedByMeDate
public File setLastViewedByMeDate(com.google.api.client.util.DateTime lastViewedByMeDate)
Last time this file was viewed by the user (formatted RFC 3339 timestamp).
- Parameters:
lastViewedByMeDate
- lastViewedByMeDate or null
for none
-
getLinkShareMetadata
public File.LinkShareMetadata getLinkShareMetadata()
Contains details about the link URLs that clients are using to refer to this item.
- Returns:
- value or
null
for none
-
setLinkShareMetadata
public File setLinkShareMetadata(File.LinkShareMetadata linkShareMetadata)
Contains details about the link URLs that clients are using to refer to this item.
- Parameters:
linkShareMetadata
- linkShareMetadata or null
for none
-
getMarkedViewedByMeDate
public com.google.api.client.util.DateTime getMarkedViewedByMeDate()
Deprecated.
- Returns:
- value or
null
for none
-
setMarkedViewedByMeDate
public File setMarkedViewedByMeDate(com.google.api.client.util.DateTime markedViewedByMeDate)
Deprecated.
- Parameters:
markedViewedByMeDate
- markedViewedByMeDate or null
for none
-
getMd5Checksum
public String getMd5Checksum()
Output only. An MD5 checksum for the content of this file. This field is only populated for
files with content stored in Google Drive; it is not populated for Docs Editors or shortcut
files.
- Returns:
- value or
null
for none
-
setMd5Checksum
public File setMd5Checksum(String md5Checksum)
Output only. An MD5 checksum for the content of this file. This field is only populated for
files with content stored in Google Drive; it is not populated for Docs Editors or shortcut
files.
- Parameters:
md5Checksum
- md5Checksum or null
for none
-
getMimeType
public String getMimeType()
The MIME type of the file. This is only mutable on update when uploading new content. This
field can be left blank, and the mimetype will be determined from the uploaded content's MIME
type.
- Returns:
- value or
null
for none
-
setMimeType
public File setMimeType(String mimeType)
The MIME type of the file. This is only mutable on update when uploading new content. This
field can be left blank, and the mimetype will be determined from the uploaded content's MIME
type.
- Parameters:
mimeType
- mimeType or null
for none
-
getModifiedByMeDate
public com.google.api.client.util.DateTime getModifiedByMeDate()
Last time this file was modified by the user (formatted RFC 3339 timestamp). Note that setting
modifiedDate will also update the modifiedByMe date for the user which set the date.
- Returns:
- value or
null
for none
-
setModifiedByMeDate
public File setModifiedByMeDate(com.google.api.client.util.DateTime modifiedByMeDate)
Last time this file was modified by the user (formatted RFC 3339 timestamp). Note that setting
modifiedDate will also update the modifiedByMe date for the user which set the date.
- Parameters:
modifiedByMeDate
- modifiedByMeDate or null
for none
-
getModifiedDate
public com.google.api.client.util.DateTime getModifiedDate()
Last time this file was modified by anyone (formatted RFC 3339 timestamp). This is only mutable
on update when the setModifiedDate parameter is set.
- Returns:
- value or
null
for none
-
setModifiedDate
public File setModifiedDate(com.google.api.client.util.DateTime modifiedDate)
Last time this file was modified by anyone (formatted RFC 3339 timestamp). This is only mutable
on update when the setModifiedDate parameter is set.
- Parameters:
modifiedDate
- modifiedDate or null
for none
-
getOpenWithLinks
public Map<String,String> getOpenWithLinks()
Output only. A map of the id of each of the user's apps to a link to open this file with that
app. Only populated when the drive.apps.readonly scope is used.
- Returns:
- value or
null
for none
-
setOpenWithLinks
public File setOpenWithLinks(Map<String,String> openWithLinks)
Output only. A map of the id of each of the user's apps to a link to open this file with that
app. Only populated when the drive.apps.readonly scope is used.
- Parameters:
openWithLinks
- openWithLinks or null
for none
-
getOriginalFilename
public String getOriginalFilename()
The original filename of the uploaded content if available, or else the original value of the
`title` field. This is only available for files with binary content in Google Drive.
- Returns:
- value or
null
for none
-
setOriginalFilename
public File setOriginalFilename(String originalFilename)
The original filename of the uploaded content if available, or else the original value of the
`title` field. This is only available for files with binary content in Google Drive.
- Parameters:
originalFilename
- originalFilename or null
for none
-
getOwnedByMe
public Boolean getOwnedByMe()
Output only. Whether the file is owned by the current user. Not populated for items in shared
drives.
- Returns:
- value or
null
for none
-
setOwnedByMe
public File setOwnedByMe(Boolean ownedByMe)
Output only. Whether the file is owned by the current user. Not populated for items in shared
drives.
- Parameters:
ownedByMe
- ownedByMe or null
for none
-
getOwnerNames
public List<String> getOwnerNames()
Output only. Name(s) of the owner(s) of this file. Not populated for items in shared drives.
- Returns:
- value or
null
for none
-
setOwnerNames
public File setOwnerNames(List<String> ownerNames)
Output only. Name(s) of the owner(s) of this file. Not populated for items in shared drives.
- Parameters:
ownerNames
- ownerNames or null
for none
-
getOwners
public List<User> getOwners()
Output only. The owner of this file. Only certain legacy files may have more than one owner.
This field isn't populated for items in shared drives.
- Returns:
- value or
null
for none
-
setOwners
public File setOwners(List<User> owners)
Output only. The owner of this file. Only certain legacy files may have more than one owner.
This field isn't populated for items in shared drives.
- Parameters:
owners
- owners or null
for none
-
getParents
public List<ParentReference> getParents()
The ID of the parent folder containing the file. A file can only have one parent folder;
specifying multiple parents isn't supported. If not specified as part of an insert request, the
file is placed directly in the user's My Drive folder. If not specified as part of a copy
request, the file inherits any discoverable parent of the source file. Update requests must use
the `addParents` and `removeParents` parameters to modify the parents list.
- Returns:
- value or
null
for none
-
setParents
public File setParents(List<ParentReference> parents)
The ID of the parent folder containing the file. A file can only have one parent folder;
specifying multiple parents isn't supported. If not specified as part of an insert request, the
file is placed directly in the user's My Drive folder. If not specified as part of a copy
request, the file inherits any discoverable parent of the source file. Update requests must use
the `addParents` and `removeParents` parameters to modify the parents list.
- Parameters:
parents
- parents or null
for none
-
getPermissionIds
public List<String> getPermissionIds()
Output only. List of permission IDs for users with access to this file.
- Returns:
- value or
null
for none
-
setPermissionIds
public File setPermissionIds(List<String> permissionIds)
Output only. List of permission IDs for users with access to this file.
- Parameters:
permissionIds
- permissionIds or null
for none
-
getPermissions
public List<Permission> getPermissions()
Output only. The list of permissions for users with access to this file. Not populated for
items in shared drives.
- Returns:
- value or
null
for none
-
setPermissions
public File setPermissions(List<Permission> permissions)
Output only. The list of permissions for users with access to this file. Not populated for
items in shared drives.
- Parameters:
permissions
- permissions or null
for none
-
getProperties
public List<Property> getProperties()
The list of properties.
- Returns:
- value or
null
for none
-
setProperties
public File setProperties(List<Property> properties)
The list of properties.
- Parameters:
properties
- properties or null
for none
-
getQuotaBytesUsed
public Long getQuotaBytesUsed()
Output only. The number of quota bytes used by this file.
- Returns:
- value or
null
for none
-
setQuotaBytesUsed
public File setQuotaBytesUsed(Long quotaBytesUsed)
Output only. The number of quota bytes used by this file.
- Parameters:
quotaBytesUsed
- quotaBytesUsed or null
for none
-
getResourceKey
public String getResourceKey()
Output only. A key needed to access the item via a shared link.
- Returns:
- value or
null
for none
-
setResourceKey
public File setResourceKey(String resourceKey)
Output only. A key needed to access the item via a shared link.
- Parameters:
resourceKey
- resourceKey or null
for none
-
getSelfLink
public String getSelfLink()
Output only. A link back to this file.
- Returns:
- value or
null
for none
-
setSelfLink
public File setSelfLink(String selfLink)
Output only. A link back to this file.
- Parameters:
selfLink
- selfLink or null
for none
-
getSha1Checksum
public String getSha1Checksum()
Output only. The SHA1 checksum associated with this file, if available. This field is only
populated for files with content stored in Google Drive; it is not populated for Docs Editors
or shortcut files.
- Returns:
- value or
null
for none
-
setSha1Checksum
public File setSha1Checksum(String sha1Checksum)
Output only. The SHA1 checksum associated with this file, if available. This field is only
populated for files with content stored in Google Drive; it is not populated for Docs Editors
or shortcut files.
- Parameters:
sha1Checksum
- sha1Checksum or null
for none
-
getSha256Checksum
public String getSha256Checksum()
Output only. The SHA256 checksum associated with this file, if available. This field is only
populated for files with content stored in Google Drive; it is not populated for Docs Editors
or shortcut files.
- Returns:
- value or
null
for none
-
setSha256Checksum
public File setSha256Checksum(String sha256Checksum)
Output only. The SHA256 checksum associated with this file, if available. This field is only
populated for files with content stored in Google Drive; it is not populated for Docs Editors
or shortcut files.
- Parameters:
sha256Checksum
- sha256Checksum or null
for none
-
getShareable
public Boolean getShareable()
Output only. Deprecated: Use `capabilities/canShare` instead.
- Returns:
- value or
null
for none
-
setShareable
public File setShareable(Boolean shareable)
Output only. Deprecated: Use `capabilities/canShare` instead.
- Parameters:
shareable
- shareable or null
for none
-
getShared
public Boolean getShared()
Output only. Whether the file has been shared. Not populated for items in shared drives.
- Returns:
- value or
null
for none
-
setShared
public File setShared(Boolean shared)
Output only. Whether the file has been shared. Not populated for items in shared drives.
- Parameters:
shared
- shared or null
for none
-
getSharedWithMeDate
public com.google.api.client.util.DateTime getSharedWithMeDate()
Time at which this file was shared with the user (formatted RFC 3339 timestamp).
- Returns:
- value or
null
for none
-
setSharedWithMeDate
public File setSharedWithMeDate(com.google.api.client.util.DateTime sharedWithMeDate)
Time at which this file was shared with the user (formatted RFC 3339 timestamp).
- Parameters:
sharedWithMeDate
- sharedWithMeDate or null
for none
-
getSharingUser
public User getSharingUser()
Output only. User that shared the item with the current user, if available.
- Returns:
- value or
null
for none
-
setSharingUser
public File setSharingUser(User sharingUser)
Output only. User that shared the item with the current user, if available.
- Parameters:
sharingUser
- sharingUser or null
for none
-
getShortcutDetails
public File.ShortcutDetails getShortcutDetails()
Shortcut file details. Only populated for shortcut files, which have the mimeType field set to
`application/vnd.google-apps.shortcut`. Can only be set on `files.insert` requests.
- Returns:
- value or
null
for none
-
setShortcutDetails
public File setShortcutDetails(File.ShortcutDetails shortcutDetails)
Shortcut file details. Only populated for shortcut files, which have the mimeType field set to
`application/vnd.google-apps.shortcut`. Can only be set on `files.insert` requests.
- Parameters:
shortcutDetails
- shortcutDetails or null
for none
-
getSpaces
public List<String> getSpaces()
Output only. The list of spaces which contain the file. Supported values are `drive`,
`appDataFolder` and `photos`.
- Returns:
- value or
null
for none
-
setSpaces
public File setSpaces(List<String> spaces)
Output only. The list of spaces which contain the file. Supported values are `drive`,
`appDataFolder` and `photos`.
- Parameters:
spaces
- spaces or null
for none
-
getTeamDriveId
public String getTeamDriveId()
Output only. Deprecated: Use `driveId` instead.
- Returns:
- value or
null
for none
-
setTeamDriveId
public File setTeamDriveId(String teamDriveId)
Output only. Deprecated: Use `driveId` instead.
- Parameters:
teamDriveId
- teamDriveId or null
for none
-
getThumbnail
public File.Thumbnail getThumbnail()
A thumbnail for the file. This will only be used if a standard thumbnail cannot be generated.
- Returns:
- value or
null
for none
-
setThumbnail
public File setThumbnail(File.Thumbnail thumbnail)
A thumbnail for the file. This will only be used if a standard thumbnail cannot be generated.
- Parameters:
thumbnail
- thumbnail or null
for none
-
getThumbnailLink
public String getThumbnailLink()
Output only. A short-lived link to the file's thumbnail, if available. Typically lasts on the
order of hours. Not intended for direct usage on web applications due to [Cross-Origin Resource
Sharing (CORS)](https://developer.mozilla.org/en-US/docs/Web/HTTP/CORS), consider using a proxy
server. Only populated when the requesting app can access the file's content. If the file isn't
shared publicly, the URL returned in `Files.thumbnailLink` must be fetched using a credentialed
request.
- Returns:
- value or
null
for none
-
setThumbnailLink
public File setThumbnailLink(String thumbnailLink)
Output only. A short-lived link to the file's thumbnail, if available. Typically lasts on the
order of hours. Not intended for direct usage on web applications due to [Cross-Origin Resource
Sharing (CORS)](https://developer.mozilla.org/en-US/docs/Web/HTTP/CORS), consider using a proxy
server. Only populated when the requesting app can access the file's content. If the file isn't
shared publicly, the URL returned in `Files.thumbnailLink` must be fetched using a credentialed
request.
- Parameters:
thumbnailLink
- thumbnailLink or null
for none
-
getThumbnailVersion
public Long getThumbnailVersion()
Output only. The thumbnail version for use in thumbnail cache invalidation.
- Returns:
- value or
null
for none
-
setThumbnailVersion
public File setThumbnailVersion(Long thumbnailVersion)
Output only. The thumbnail version for use in thumbnail cache invalidation.
- Parameters:
thumbnailVersion
- thumbnailVersion or null
for none
-
getTitle
public String getTitle()
The title of this file. Note that for immutable items such as the top level folders of shared
drives, My Drive root folder, and Application Data folder the title is constant.
- Returns:
- value or
null
for none
-
setTitle
public File setTitle(String title)
The title of this file. Note that for immutable items such as the top level folders of shared
drives, My Drive root folder, and Application Data folder the title is constant.
- Parameters:
title
- title or null
for none
-
getTrashedDate
public com.google.api.client.util.DateTime getTrashedDate()
The time that the item was trashed (formatted RFC 3339 timestamp). Only populated for items in
shared drives.
- Returns:
- value or
null
for none
-
setTrashedDate
public File setTrashedDate(com.google.api.client.util.DateTime trashedDate)
The time that the item was trashed (formatted RFC 3339 timestamp). Only populated for items in
shared drives.
- Parameters:
trashedDate
- trashedDate or null
for none
-
getTrashingUser
public User getTrashingUser()
Output only. If the file has been explicitly trashed, the user who trashed it. Only populated
for items in shared drives.
- Returns:
- value or
null
for none
-
setTrashingUser
public File setTrashingUser(User trashingUser)
Output only. If the file has been explicitly trashed, the user who trashed it. Only populated
for items in shared drives.
- Parameters:
trashingUser
- trashingUser or null
for none
-
getUserPermission
public Permission getUserPermission()
Output only. The permissions for the authenticated user on this file.
- Returns:
- value or
null
for none
-
setUserPermission
public File setUserPermission(Permission userPermission)
Output only. The permissions for the authenticated user on this file.
- Parameters:
userPermission
- userPermission or null
for none
-
getVersion
public Long getVersion()
Output only. A monotonically increasing version number for the file. This reflects every change
made to the file on the server, even those not visible to the requesting user.
- Returns:
- value or
null
for none
-
setVersion
public File setVersion(Long version)
Output only. A monotonically increasing version number for the file. This reflects every change
made to the file on the server, even those not visible to the requesting user.
- Parameters:
version
- version or null
for none
-
getVideoMediaMetadata
public File.VideoMediaMetadata getVideoMediaMetadata()
Output only. Metadata about video media. This will only be present for video types.
- Returns:
- value or
null
for none
-
setVideoMediaMetadata
public File setVideoMediaMetadata(File.VideoMediaMetadata videoMediaMetadata)
Output only. Metadata about video media. This will only be present for video types.
- Parameters:
videoMediaMetadata
- videoMediaMetadata or null
for none
-
getWebContentLink
public String getWebContentLink()
Output only. A link for downloading the content of the file in a browser using cookie based
authentication. In cases where the content is shared publicly, the content can be downloaded
without any credentials.
- Returns:
- value or
null
for none
-
setWebContentLink
public File setWebContentLink(String webContentLink)
Output only. A link for downloading the content of the file in a browser using cookie based
authentication. In cases where the content is shared publicly, the content can be downloaded
without any credentials.
- Parameters:
webContentLink
- webContentLink or null
for none
-
getWebViewLink
public String getWebViewLink()
Output only. A link only available on public folders for viewing their static web assets (HTML,
CSS, JS, etc) via Google Drive's Website Hosting.
- Returns:
- value or
null
for none
-
setWebViewLink
public File setWebViewLink(String webViewLink)
Output only. A link only available on public folders for viewing their static web assets (HTML,
CSS, JS, etc) via Google Drive's Website Hosting.
- Parameters:
webViewLink
- webViewLink or null
for none
-
getWritersCanShare
public Boolean getWritersCanShare()
Whether writers can share the document with other users. Not populated for items in shared
drives.
- Returns:
- value or
null
for none
-
setWritersCanShare
public File setWritersCanShare(Boolean writersCanShare)
Whether writers can share the document with other users. Not populated for items in shared
drives.
- Parameters:
writersCanShare
- writersCanShare or null
for none
-
set
public File set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public File clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy