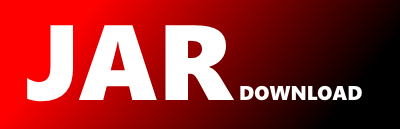
javadoc.com.google.common.collect.ImmutableSortedSet.html Maven / Gradle / Ivy
ImmutableSortedSet (Guava: Google Core Libraries for Java 11.0.1 API)
Overview
Package
Class
Use
Tree
Deprecated
Index
Help
PREV CLASS
NEXT CLASS
FRAMES
NO FRAMES
SUMMARY: NESTED | FIELD | CONSTR | METHOD
DETAIL: FIELD | CONSTR | METHOD
com.google.common.collect
Class ImmutableSortedSet<E>
java.lang.Object
com.google.common.collect.ImmutableCollection<E>
com.google.common.collect.ImmutableSet<E>
com.google.common.collect.ImmutableSortedSet<E>
- All Implemented Interfaces:
- Serializable, Iterable<E>, Collection<E>, Set<E>, SortedSet<E>
- Direct Known Subclasses:
- ContiguousSet
@GwtCompatible(serializable=true,
emulated=true)
public abstract class ImmutableSortedSet<E>
- extends ImmutableSet<E>
- implements SortedSet<E>
An immutable SortedSet
that stores its elements in a sorted array.
Some instances are ordered by an explicit comparator, while others follow the
natural sort ordering of their elements. Either way, null elements are not
supported.
Unlike Collections.unmodifiableSortedSet(java.util.SortedSet
, which is a view
of a separate collection that can still change, an instance of ImmutableSortedSet
contains its own private data and will never
change. This class is convenient for public static final
sets
("constant sets") and also lets you easily make a "defensive copy" of a set
provided to your class by a caller.
The sets returned by the headSet(E)
, tailSet(E)
, and
subSet(E, E)
methods share the same array as the original set, preventing
that array from being garbage collected. If this is a concern, the data may
be copied into a correctly-sized array by calling copyOfSorted(java.util.SortedSet
.
Note on element equivalence: The ImmutableCollection.contains(Object)
,
ImmutableCollection.containsAll(Collection)
, and ImmutableSet.equals(Object)
implementations must check whether a provided object is equivalent to an
element in the collection. Unlike most collections, an
ImmutableSortedSet
doesn't use Object.equals(java.lang.Object)
to determine if
two elements are equivalent. Instead, with an explicit comparator, the
following relation determines whether elements x
and y
are
equivalent:
{(x, y) | comparator.compare(x, y) == 0}
With natural ordering of elements, the following relation determines whether
two elements are equivalent: {(x, y) | x.compareTo(y) == 0}
Warning: Like most sets, an ImmutableSortedSet
will not
function correctly if an element is modified after being placed in the set.
For this reason, and to avoid general confusion, it is strongly recommended
to place only immutable objects into this collection.
Note: Although this class is not final, it cannot be subclassed as it has no public or protected constructors. Thus, instances of this type are guaranteed to be immutable.
- Since:
- 2.0 (imported from Google Collections Library)
- Author:
- Jared Levy, Louis Wasserman
- See Also:
ImmutableSet
, Serialized Form
Nested Class Summary | |
---|---|
static class |
ImmutableSortedSet.Builder<E>
A builder for creating immutable sorted set instances, especially public static final sets ("constant sets"), with a given comparator. |
Method Summary | ||
---|---|---|
static
|
builder()
Deprecated. Use naturalOrder() , which offers
better type-safety. |
|
Comparator<? super E> |
comparator()
Returns the comparator that orders the elements, which is Ordering.natural() when the natural ordering of the
elements is used. |
|
static
|
copyOf(Collection<? extends E> elements)
Returns an immutable sorted set containing the given elements sorted by their natural ordering. |
|
static
|
copyOf(Comparator<? super E> comparator,
Collection<? extends E> elements)
Returns an immutable sorted set containing the given elements sorted by the given Comparator . |
|
static
|
copyOf(Comparator<? super E> comparator,
Iterable<? extends E> elements)
Returns an immutable sorted set containing the given elements sorted by the given Comparator . |
|
static
|
copyOf(Comparator<? super E> comparator,
Iterator<? extends E> elements)
Returns an immutable sorted set containing the given elements sorted by the given Comparator . |
|
static
|
copyOf(E[] elements)
Returns an immutable sorted set containing the given elements sorted by their natural ordering. |
|
static
|
copyOf(Iterable<? extends E> elements)
Returns an immutable sorted set containing the given elements sorted by their natural ordering. |
|
static
|
copyOf(Iterator<? extends E> elements)
Returns an immutable sorted set containing the given elements sorted by their natural ordering. |
|
static
|
copyOfSorted(SortedSet<E> sortedSet)
Returns an immutable sorted set containing the elements of a sorted set, sorted by the same Comparator . |
|
ImmutableSortedSet<E> |
headSet(E toElement)
|
|
abstract UnmodifiableIterator<E> |
iterator()
Returns an unmodifiable iterator across the elements in this collection. |
|
static
|
naturalOrder()
Returns a builder that creates immutable sorted sets whose elements are ordered by their natural ordering. |
|
static
|
of()
Returns the empty immutable sorted set. |
|
static
|
of(E element)
Returns an immutable sorted set containing a single element. |
|
static
|
of(E e1,
E e2)
Returns an immutable sorted set containing the given elements sorted by their natural ordering. |
|
static
|
of(E e1,
E e2,
E e3)
Returns an immutable sorted set containing the given elements sorted by their natural ordering. |
|
static
|
of(E e1,
E e2,
E e3,
E e4)
Returns an immutable sorted set containing the given elements sorted by their natural ordering. |
|
static
|
of(E e1,
E e2,
E e3,
E e4,
E e5)
Returns an immutable sorted set containing the given elements sorted by their natural ordering. |
|
static
|
of(E e1,
E e2,
E e3,
E e4,
E e5,
E e6,
E... remaining)
Returns an immutable sorted set containing the given elements sorted by their natural ordering. |
|
static
|
orderedBy(Comparator<E> comparator)
Returns a builder that creates immutable sorted sets with an explicit comparator. |
|
static
|
reverseOrder()
Returns a builder that creates immutable sorted sets whose elements are ordered by the reverse of their natural ordering. |
|
ImmutableSortedSet<E> |
subSet(E fromElement,
E toElement)
|
|
ImmutableSortedSet<E> |
tailSet(E fromElement)
|
Methods inherited from class com.google.common.collect.ImmutableSet |
---|
equals, hashCode |
Methods inherited from class com.google.common.collect.ImmutableCollection |
---|
add, addAll, asList, clear, contains, containsAll, isEmpty, remove, removeAll, retainAll, toArray, toArray, toString |
Methods inherited from class java.lang.Object |
---|
clone, finalize, getClass, notify, notifyAll, wait, wait, wait |
Methods inherited from interface java.util.SortedSet |
---|
first, last |
Methods inherited from interface java.util.Set |
---|
add, addAll, clear, contains, containsAll, equals, hashCode, isEmpty, remove, removeAll, retainAll, size, toArray, toArray |
Method Detail |
---|
of
public static <E> ImmutableSortedSet<E> of()
- Returns the empty immutable sorted set.
of
public static <E extends Comparable<? super E>> ImmutableSortedSet<E> of(E element)
- Returns an immutable sorted set containing a single element.
of
public static <E extends Comparable<? super E>> ImmutableSortedSet<E> of(E e1, E e2)
- Returns an immutable sorted set containing the given elements sorted by
their natural ordering. When multiple elements are equivalent according to
Comparable.compareTo(T)
, only the first one specified is included.- Throws:
NullPointerException
- if any element is null
of
public static <E extends Comparable<? super E>> ImmutableSortedSet<E> of(E e1, E e2, E e3)
- Returns an immutable sorted set containing the given elements sorted by
their natural ordering. When multiple elements are equivalent according to
Comparable.compareTo(T)
, only the first one specified is included.- Throws:
NullPointerException
- if any element is null
of
public static <E extends Comparable<? super E>> ImmutableSortedSet<E> of(E e1, E e2, E e3, E e4)
- Returns an immutable sorted set containing the given elements sorted by
their natural ordering. When multiple elements are equivalent according to
Comparable.compareTo(T)
, only the first one specified is included.- Throws:
NullPointerException
- if any element is null
of
public static <E extends Comparable<? super E>> ImmutableSortedSet<E> of(E e1, E e2, E e3, E e4, E e5)
- Returns an immutable sorted set containing the given elements sorted by
their natural ordering. When multiple elements are equivalent according to
Comparable.compareTo(T)
, only the first one specified is included.- Throws:
NullPointerException
- if any element is null
of
public static <E extends Comparable<? super E>> ImmutableSortedSet<E> of(E e1, E e2, E e3, E e4, E e5, E e6, E... remaining)
- Returns an immutable sorted set containing the given elements sorted by
their natural ordering. When multiple elements are equivalent according to
Comparable.compareTo(T)
, only the first one specified is included.- Throws:
NullPointerException
- if any element is null- Since:
- 3.0 (source-compatible since 2.0)
copyOf
public static <E extends Comparable<? super E>> ImmutableSortedSet<E> copyOf(E[] elements)
- Returns an immutable sorted set containing the given elements sorted by
their natural ordering. When multiple elements are equivalent according to
Comparable.compareTo(T)
, only the first one specified is included.- Throws:
NullPointerException
- if any ofelements
is null- Since:
- 3.0
copyOf
public static <E> ImmutableSortedSet<E> copyOf(Iterable<? extends E> elements)
- Returns an immutable sorted set containing the given elements sorted by
their natural ordering. When multiple elements are equivalent according to
compareTo()
, only the first one specified is included. To create a copy of aSortedSet
that preserves the comparator, callcopyOfSorted(java.util.SortedSet
instead. This method iterates over) elements
at most once.Note that if
s
is aSet<String>
, thenImmutableSortedSet.copyOf(s)
returns anImmutableSortedSet<String>
containing each of the strings ins
, whileImmutableSortedSet.of(s)
returns anImmutableSortedSet<Set<String>>
containing one element (the given set itself).Despite the method name, this method attempts to avoid actually copying the data when it is safe to do so. The exact circumstances under which a copy will or will not be performed are undocumented and subject to change.
This method is not type-safe, as it may be called on elements that are not mutually comparable.
- Throws:
ClassCastException
- if the elements are not mutually comparableNullPointerException
- if any ofelements
is null
copyOf
public static <E> ImmutableSortedSet<E> copyOf(Collection<? extends E> elements)
- Returns an immutable sorted set containing the given elements sorted by
their natural ordering. When multiple elements are equivalent according to
compareTo()
, only the first one specified is included. To create a copy of aSortedSet
that preserves the comparator, callcopyOfSorted(java.util.SortedSet
instead. This method iterates over) elements
at most once.Note that if
s
is aSet<String>
, thenImmutableSortedSet.copyOf(s)
returns anImmutableSortedSet<String>
containing each of the strings ins
, whileImmutableSortedSet.of(s)
returns anImmutableSortedSet<Set<String>>
containing one element (the given set itself).Note: Despite what the method name suggests, if
elements
is anImmutableSortedSet
, it may be returned instead of a copy.This method is not type-safe, as it may be called on elements that are not mutually comparable.
This method is safe to use even when
elements
is a synchronized or concurrent collection that is currently being modified by another thread.- Throws:
ClassCastException
- if the elements are not mutually comparableNullPointerException
- if any ofelements
is null- Since:
- 7.0 (source-compatible since 2.0)
copyOf
public static <E> ImmutableSortedSet<E> copyOf(Iterator<? extends E> elements)
- Returns an immutable sorted set containing the given elements sorted by
their natural ordering. When multiple elements are equivalent according to
compareTo()
, only the first one specified is included.This method is not type-safe, as it may be called on elements that are not mutually comparable.
- Throws:
ClassCastException
- if the elements are not mutually comparableNullPointerException
- if any ofelements
is null
copyOf
public static <E> ImmutableSortedSet<E> copyOf(Comparator<? super E> comparator, Iterator<? extends E> elements)
- Returns an immutable sorted set containing the given elements sorted by
the given
Comparator
. When multiple elements are equivalent according tocompareTo()
, only the first one specified is included.- Throws:
NullPointerException
- ifcomparator
or any ofelements
is null
copyOf
public static <E> ImmutableSortedSet<E> copyOf(Comparator<? super E> comparator, Iterable<? extends E> elements)
- Returns an immutable sorted set containing the given elements sorted by
the given
Comparator
. When multiple elements are equivalent according tocompare()
, only the first one specified is included. This method iterates overelements
at most once.Despite the method name, this method attempts to avoid actually copying the data when it is safe to do so. The exact circumstances under which a copy will or will not be performed are undocumented and subject to change.
- Throws:
NullPointerException
- ifcomparator
or any ofelements
is null
copyOf
public static <E> ImmutableSortedSet<E> copyOf(Comparator<? super E> comparator, Collection<? extends E> elements)
- Returns an immutable sorted set containing the given elements sorted by
the given
Comparator
. When multiple elements are equivalent according tocompareTo()
, only the first one specified is included.Despite the method name, this method attempts to avoid actually copying the data when it is safe to do so. The exact circumstances under which a copy will or will not be performed are undocumented and subject to change.
This method is safe to use even when
elements
is a synchronized or concurrent collection that is currently being modified by another thread.- Throws:
NullPointerException
- ifcomparator
or any ofelements
is null- Since:
- 7.0 (source-compatible since 2.0)
copyOfSorted
public static <E> ImmutableSortedSet<E> copyOfSorted(SortedSet<E> sortedSet)
- Returns an immutable sorted set containing the elements of a sorted set,
sorted by the same
Comparator
. That behavior differs fromcopyOf(Iterable)
, which always uses the natural ordering of the elements.Despite the method name, this method attempts to avoid actually copying the data when it is safe to do so. The exact circumstances under which a copy will or will not be performed are undocumented and subject to change.
This method is safe to use even when
sortedSet
is a synchronized or concurrent collection that is currently being modified by another thread.- Throws:
NullPointerException
- ifsortedSet
or any of its elements is null
orderedBy
public static <E> ImmutableSortedSet.Builder<E> orderedBy(Comparator<E> comparator)
- Returns a builder that creates immutable sorted sets with an explicit
comparator. If the comparator has a more general type than the set being
generated, such as creating a
SortedSet<Integer>
with aComparator<Number>
, use theImmutableSortedSet.Builder
constructor instead.- Throws:
NullPointerException
- ifcomparator
is null
reverseOrder
public static <E extends Comparable<E>> ImmutableSortedSet.Builder<E> reverseOrder()
- Returns a builder that creates immutable sorted sets whose elements are
ordered by the reverse of their natural ordering.
Note: the type parameter
E
extendsComparable<E>
rather thanComparable<? super E>
as a workaround for javac bug 6468354.
naturalOrder
public static <E extends Comparable<E>> ImmutableSortedSet.Builder<E> naturalOrder()
- Returns a builder that creates immutable sorted sets whose elements are
ordered by their natural ordering. The sorted sets use
Ordering.natural()
as the comparator. This method provides more type-safety thanbuilder()
, as it can be called only for classes that implementComparable
.Note: the type parameter
E
extendsComparable<E>
rather thanComparable<? super E>
as a workaround for javac bug 6468354.
comparator
public Comparator<? super E> comparator()
- Returns the comparator that orders the elements, which is
Ordering.natural()
when the natural ordering of the elements is used. Note that its behavior is not consistent withSortedSet.comparator()
, which returnsnull
to indicate natural ordering.- Specified by:
comparator
in interfaceSortedSet<E>
iterator
public abstract UnmodifiableIterator<E> iterator()
- Description copied from class:
ImmutableCollection
- Returns an unmodifiable iterator across the elements in this collection.
headSet
public ImmutableSortedSet<E> headSet(E toElement)
-
This method returns a serializable
ImmutableSortedSet
.The
SortedSet.headSet(E)
documentation states that a subset of a subset throws anIllegalArgumentException
if passed atoElement
greater than an earliertoElement
. However, this method doesn't throw an exception in that situation, but instead keeps the originaltoElement
.
subSet
public ImmutableSortedSet<E> subSet(E fromElement, E toElement)
-
This method returns a serializable
ImmutableSortedSet
.The
SortedSet.subSet(E, E)
documentation states that a subset of a subset throws anIllegalArgumentException
if passed afromElement
smaller than an earlierfromElement
. However, this method doesn't throw an exception in that situation, but instead keeps the originalfromElement
. Similarly, this method keeps the originaltoElement
, instead of throwing an exception, if passed atoElement
greater than an earliertoElement
.
tailSet
public ImmutableSortedSet<E> tailSet(E fromElement)
-
This method returns a serializable
ImmutableSortedSet
.The
SortedSet.tailSet(E)
documentation states that a subset of a subset throws anIllegalArgumentException
if passed afromElement
smaller than an earlierfromElement
. However, this method doesn't throw an exception in that situation, but instead keeps the originalfromElement
.
builder
@Deprecated public static <E> ImmutableSortedSet.Builder<E> builder()
- Deprecated. Use
naturalOrder()
, which offers better type-safety.- Not supported. Use
naturalOrder()
, which offers better type-safety, instead. This method exists only to hideImmutableSet.builder()
from consumers ofImmutableSortedSet
.- Throws:
UnsupportedOperationException
- always
- Not supported. Use
|
||||||||||
PREV CLASS NEXT CLASS | FRAMES NO FRAMES | |||||||||
SUMMARY: NESTED | FIELD | CONSTR | METHOD | DETAIL: FIELD | CONSTR | METHOD |
Copyright © 2010-2012. All Rights Reserved.