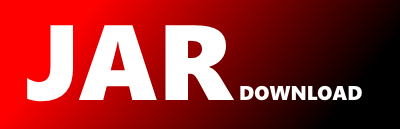
com.jillesvangurp.iterables.CSVLineIterable Maven / Gradle / Ivy
package com.jillesvangurp.iterables;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.NoSuchElementException;
import com.google.common.base.Splitter;
/**
* Simple iterable that breaks lines into fields based on a separator.
* Note. this class is fairly simplistic compared to solutions like open csv and doesn't support things like escaping
* currently. Feel free to contribute patches to fix this.
*/
public class CSVLineIterable implements Iterable> {
private final Iterable lineIterator;
private final char delimiter;
public CSVLineIterable(Iterable lineIterator, char delimiter) {
this.lineIterator = lineIterator;
this.delimiter = delimiter;
}
@Override
public Iterator> iterator() {
final Iterator iterator = lineIterator.iterator();
return new Iterator>() {
@Override
public boolean hasNext() {
return iterator.hasNext();
}
@Override
public List next() {
String line = iterator.next();
if (line != null) {
List result = new ArrayList<>();
for (String field : Splitter.on(delimiter).trimResults().split(line)) {
result.add(field);
}
return result;
} else {
throw new NoSuchElementException();
}
}
@Override
public void remove() {
iterator.remove();
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy