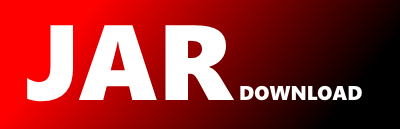
com.jillesvangurp.iterables.PagingIterable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iterables-support Show documentation
Show all versions of iterables-support Show documentation
Misc classes to make processing utf-8 content with iterators a bit less painful.
The newest version!
package com.jillesvangurp.iterables;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.NoSuchElementException;
import org.apache.commons.lang.Validate;
class PagingIterable implements Iterable> {
private final Iterable input;
private final int pageSize;
public PagingIterable(Iterable input, int pageSize) {
Validate.isTrue(pageSize > 0);
this.input = input;
this.pageSize = pageSize;
}
@Override
public Iterator> iterator() {
final Iterator it = input.iterator();
return new Iterator>() {
List next=null;
@Override
public boolean hasNext() {
if(next != null) {
return true;
} else if(it.hasNext()) {
next = new ArrayList<>(pageSize);
int i = 0;
while(i < pageSize && it.hasNext()) {
next.add(it.next());
i++;
}
if(next.size() > 0) {
return true;
} else {
return false;
}
} else {
return false;
}
}
@Override
public List next() {
if(hasNext()) {
List result = next;
next=null;
return result;
} else {
throw new NoSuchElementException();
}
}
@Override
public void remove() {
throw new UnsupportedOperationException();
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy