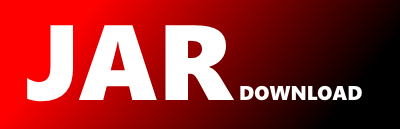
com.maxifier.mxcache.jconsoleplugin.MxCacheJConsolePlugin Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mxcache-jmx Show documentation
Show all versions of mxcache-jmx Show documentation
MxCache management extensions and JConsole plugin
/*
* Copyright (c) 2008-2014 Maxifier Ltd. All Rights Reserved.
*/
package com.maxifier.mxcache.jconsoleplugin;
import com.sun.tools.jconsole.JConsolePlugin;
import javax.management.openmbean.InvalidKeyException;
import javax.swing.*;
import javax.swing.event.ChangeListener;
import javax.swing.event.ChangeEvent;
import javax.swing.table.*;
import javax.management.*;
import javax.management.openmbean.CompositeData;
import java.awt.event.*;
import java.util.*;
import java.util.List;
import java.util.regex.Pattern;
import java.util.regex.PatternSyntaxException;
import java.awt.*;
/**
* @author Alexander Kochurov ([email protected])
*/
@SuppressWarnings("UnusedDeclaration")
// used by JConsole
public class MxCacheJConsolePlugin extends JConsolePlugin {
private final JPanel panel;
private final JPanel resourcesPanel;
private final JList resourcesList;
private final DefaultListModel resourcesListModel;
private final MxCacheTableModel model;
private final JCheckBox regex;
private final JTextField searchText;
private final TableRowSorter sorter;
private final JCheckBox matchCase;
private final JComboBox tagsCombo;
private final JComboBox groupsCombo;
private final JComboBox classesCombo;
private final JComboBox annotationsCombo;
private final Color searchTextBg;
private final Map searchIn;
private final JLabel errorLabel;
private static final String[] STRING_SIGNATURE = { String.class.getCanonicalName() };
private static final ObjectName JMX_NAME;
private static final Color INVALID_PATTERN_BG = new Color(0xFF, 0x80, 0x80);
static {
try {
JMX_NAME = new ObjectName("com.maxifier.mxcache:service=CacheControl");
} catch (MalformedObjectNameException e) {
throw new RuntimeException(e);
}
}
public MxCacheJConsolePlugin() {
panel = new JPanel(new BorderLayout());
resourcesPanel = new JPanel(new BorderLayout());
resourcesListModel = new DefaultListModel();
resourcesList = new JList(resourcesListModel);
resourcesPanel.add(new JScrollPane(resourcesList), BorderLayout.CENTER);
JButton clearResourceButton = new JButton("Clear resource");
resourcesPanel.add(clearResourceButton, BorderLayout.SOUTH);
clearResourceButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String resName = (String) resourcesList.getSelectedValue();
try {
getContext().getMBeanServerConnection().invoke(JMX_NAME, "clearByResource", new Object[] { resName }, STRING_SIGNATURE);
} catch (Exception er) {
er.printStackTrace();
}
}
});
model = new MxCacheTableModel();
final JTable table = new JTable(model);
final JPopupMenu popup = new JPopupMenu();
table.getTableHeader().addMouseListener(new ShowPopupMouseAdapter(popup, table));
Enumeration columnEnumeration = table.getColumnModel().getColumns();
final List columns = new ArrayList();
while (columnEnumeration.hasMoreElements()) {
columns.add(columnEnumeration.nextElement());
}
final boolean[] visible = new boolean[columns.size()];
Arrays.fill(visible, true);
for (final TableColumn column : columns) {
final JCheckBox c = new JCheckBox(column.getIdentifier().toString());
c.setSelected(true);
popup.add(c);
c.addChangeListener(new ColumnVisibilityChangeListener(column, c, visible, table));
}
sorter = new TableRowSorter(model);
for (com.maxifier.mxcache.jconsoleplugin.Attribute attribute : com.maxifier.mxcache.jconsoleplugin.Attribute.values()) {
Comparator comparator = attribute.getComparator();
if (comparator != null) {
sorter.setComparator(attribute.ordinal(), comparator);
}
}
JPanel search = new JPanel(new FlowLayout(FlowLayout.LEFT));
search.add(new JLabel("Search text "));
regex = new JCheckBox("Regex");
matchCase = new JCheckBox("Match case");
ChangeListener listener = new ChangeListener() {
@Override
public void stateChanged(ChangeEvent e) {
updateSorting();
}
};
searchText = new JTextField(10);
search.add(searchText);
search.add(regex);
search.add(matchCase);
search.add(new JLabel("Search in "));
searchIn = new EnumMap(com.maxifier.mxcache.jconsoleplugin.Attribute.class);
for (com.maxifier.mxcache.jconsoleplugin.Attribute attribute : com.maxifier.mxcache.jconsoleplugin.Attribute.values()) {
if (attribute.isSearchable()) {
JCheckBox check = new JCheckBox(attribute.getName(), true);
searchIn.put(attribute, check);
check.addChangeListener(listener);
search.add(check);
}
}
JButton all = new JButton("All");
search.add(all);
all.addActionListener(new SetAllAction(true));
JButton none = new JButton("None");
search.add(none);
none.addActionListener(new SetAllAction(false));
errorLabel = new JLabel();
search.add(errorLabel);
searchText.addKeyListener(new KeyAdapter() {
@Override
public void keyReleased(KeyEvent e) {
updateSorting();
}
});
searchTextBg = searchText.getBackground();
regex.addChangeListener(listener);
matchCase.addChangeListener(listener);
table.setRowSorter(sorter);
table.setDefaultRenderer(Object.class, new MxCacheTableRenderer());
table.setAutoResizeMode(JTable.AUTO_RESIZE_LAST_COLUMN);
JPanel clear = new JPanel(new FlowLayout(FlowLayout.LEFT));
classesCombo = initClearComponent(clear, "Class: ", "clearByClass", true);
groupsCombo = initClearComponent(clear, "Group: ", "clearByGroup", false);
tagsCombo = initClearComponent(clear, "Tag: ", "clearByTag", false);
annotationsCombo = initClearComponent(clear, "Annotation: ", "clearByTag", true);
panel.add(search, BorderLayout.NORTH);
panel.add(new JScrollPane(table), BorderLayout.CENTER);
panel.add(clear, BorderLayout.SOUTH);
}
private JComboBox initClearComponent(JPanel clear, String caption, String method, boolean shortcutClassNames) {
clear.add(new JLabel(caption));
JComboBox combo = new JComboBox();
JButton clearButton = new JButton("Clear");
clearButton.addActionListener(new CleanerAction(combo, method));
clear.add(combo);
clear.add(clearButton);
if (shortcutClassNames) {
combo.setRenderer(new ShortcutListCellRenderer());
}
return combo;
}
static class MxCacheTableRenderer extends DefaultTableCellRenderer {
@Override
public Component getTableCellRendererComponent(JTable table,
Object value, boolean isSelected, boolean hasFocus,
int row, int column) {
Component c = super.getTableCellRendererComponent(table, value, isSelected, hasFocus, row, column);
if (c instanceof JLabel) {
JLabel l = (JLabel) c;
Insets insets = l.getInsets();
int w = l.getPreferredSize().width
+ insets.left + insets.right;
TableColumn col = table.getColumnModel().getColumn(column);
col.setPreferredWidth(Math.max(col.getPreferredWidth(), w));
}
return c;
}
}
private void updateSorting() {
int[] columns = getColumnsToSearch();
boolean ok;
try {
RowFilter
© 2015 - 2025 Weber Informatics LLC | Privacy Policy