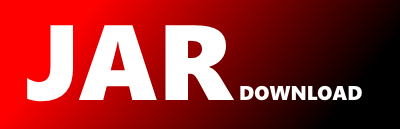
kv-4.0.9.src.oracle.kv.table.ExecutionFuture Maven / Gradle / Ivy
Show all versions of oracle-nosql-client Show documentation
/*-
*
* This file is part of Oracle NoSQL Database
* Copyright (C) 2011, 2016 Oracle and/or its affiliates. All rights reserved.
*
* If you have received this file as part of Oracle NoSQL Database the
* following applies to the work as a whole:
*
* Oracle NoSQL Database server software is free software: you can
* redistribute it and/or modify it under the terms of the GNU Affero
* General Public License as published by the Free Software Foundation,
* version 3.
*
* Oracle NoSQL Database is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Affero General Public License for more details.
*
* If you have received this file as part of Oracle NoSQL Database Client or
* distributed separately the following applies:
*
* Oracle NoSQL Database client software is free software: you can
* redistribute it and/or modify it under the terms of the Apache License
* as published by the Apache Software Foundation, version 2.0.
*
* You should have received a copy of the GNU Affero General Public License
* and/or the Apache License in the LICENSE file along with Oracle NoSQL
* Database client or server distribution. If not, see
*
* or
* .
*
* An active Oracle commercial licensing agreement for this product supersedes
* these licenses and in such case the license notices, but not the copyright
* notice, may be removed by you in connection with your distribution that is
* in accordance with the commercial licensing terms.
*
* For more information please contact:
*
* [email protected]
*
*/
package oracle.kv.table;
import java.util.concurrent.CancellationException;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.Future;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
import oracle.kv.FaultException;
/**
* An ExecutionFuture is a {@link Future} that provides a handle to an
* administrative statement that has been issued and is being processed by the
* kvstore. ExecutionFuture provides a way to check on the interim status of
* the administrative operation, wait for the operation completion, or cancel
* the operation.
* @deprecated since 3.3 in favor of {@link oracle.kv.ExecutionFuture}
*/
@Deprecated
public interface ExecutionFuture extends Future {
/**
* Attempts to cancel execution of this statement. Returns false if the
* statement couldn't be cancelled, possibly because it has already
* finished. If the statement hasn't succeeded already, and is stopped,
* the operation will deem to have failed.
*
* @param mayInterruptIfRunning Since command execution begins immediately,
* if mayInterreuptIfRunning is false, cancel returns false.
* @throws FaultException if the Admin service connection fails.
*/
@Override
public boolean cancel(boolean mayInterruptIfRunning) throws FaultException;
/**
* Block until the command represented by this future completes. Returns
* information about the execution of the statement. This call will result
* in communication with the kvstore server.
*
* Note that ExecutionException is thrown if the statement execution
* threw an exception. Once get() throws ExecutionException, all further
* calls to get() will continue to throw ExecutionException.
*
* @throws ExecutionException if the command failed.
* @throws CancellationException if the command was cancelled.
* @throws InterruptedException if the current thread was interrupted
* @throws FaultException if tbw
*/
@Override
public StatementResult get()
throws CancellationException,
ExecutionException,
InterruptedException;
/**
* Block until the administrative operation has finished or the timeout
* period is exceeded. This call will result in communication with the
* kvstore server.
*
* Note that ExecutionException is thrown if the statement execution
* threw an exception. Once get() throws ExecutionException, all further
* calls to get() will continue to throw ExecutionException.
* @return information about the execution of the statement
* @throws TimeoutException if the timeout is exceeded.
* @throws InterruptedException
*/
@Override
public StatementResult get(long timeout, TimeUnit unit)
throws InterruptedException, TimeoutException, ExecutionException;
/**
* Returns true if the operation was cancelled. The cancellation may
* still be in progress, and the operation may still be running.
*/
@Override
public boolean isCancelled();
/**
* Returns true if the operation has been terminated. If the operation is
* still executing, or if status has never been requested before, this call
* will result in communication with the kvstore server to obtain up to
* date status.
*
* When the statement has terminated, results and status can be obtained
* via {@link StatementResult}
*/
@Override
public boolean isDone();
/**
* Returns information about the execution of the statement. If the
* statement is still executing, this call will result in communication
* with the kvstore server to obtain up to date status, and the status
* returned will reflect interim information.
* @throws FaultException if there was any problem with accessing the
* server. In general, such issues are transient, and the request can
* be retried.
*/
public StatementResult updateStatus()
throws FaultException;
/**
* Returns information about the execution of the statement. The information
* returned is that obtained by the last communication with the kvstore
* server, and will not cause any additional communication. To request a
* current check, use {@link #updateStatus}
*/
public StatementResult getLastStatus();
/**
* Return the statement which has been executed.
*/
public String getStatement();
}