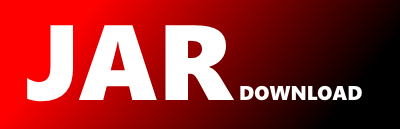
org.restexpress.RestExpress Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of RestExpress Show documentation
Show all versions of RestExpress Show documentation
Internet scale, high-performance RESTful Services in Java
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy