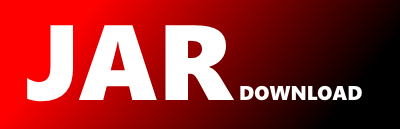
com.tecacet.finance.io.parser.OtherAssetParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jfapi Show documentation
Show all versions of jfapi Show documentation
JFApi connects to various Web Services that provide financial data such as stock prices,
splits, dividends, and FX rates.
package com.tecacet.finance.io.parser;
import com.tecacet.finance.model.Asset;
import com.tecacet.finance.model.AssetType;
import com.tecacet.finance.model.Exchange;
import com.tecacet.jflat.CSVReader;
import org.apache.commons.csv.CSVFormat;
import java.io.IOException;
import java.io.InputStream;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class OtherAssetParser {
private static final Map EXCHNAGE_MAP = new HashMap<>();
static {
EXCHNAGE_MAP.put("A", Exchange.NYSE_MKT);
EXCHNAGE_MAP.put("N", Exchange.NYSE);
EXCHNAGE_MAP.put("P", Exchange.NYSE_ARCA);
EXCHNAGE_MAP.put("Z", Exchange.BATS);
}
private final CSVReader reader = CSVReader
.readerWithHeaderMapping(Asset.class, new String[] {"ACT Symbol", "Security Name", "Round Lot Size", "ETF", "Exchange"},
new String[] {"symbol", "name", "roundLotSize", "assetType", "exchange"})
.withFormat(CSVFormat.DEFAULT.withFirstRecordAsHeader().withDelimiter('|'))
.registerConverter(AssetType.class, s -> isETF(s) ? AssetType.ETF : AssetType.STOCK)
.registerConverter(Exchange.class, EXCHNAGE_MAP::get);
public List parse(InputStream fis) throws IOException {
List assets = reader.readAll(fis);
// The last one is a footer
assets.remove(assets.size() - 1);
return assets;
}
private boolean isETF(String token) {
return "Y".equals(token.trim());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy